Note
Go to the end to download the full example code.
ChemicalDrift - Transport and fate of organic compounds
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.chemicaldrift import ChemicalDrift
from opendrift.readers.reader_constant import Reader as ConstantReader
from datetime import timedelta, datetime
import matplotlib.pyplot as plt
import numpy as np
o = ChemicalDrift(loglevel=0, seed=0)
# Norkyst
reader_norkyst = reader_netCDF_CF_generic.Reader('https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be')
mixed_layer = ConstantReader({'ocean_mixed_layer_thickness': 40})
o.add_reader([reader_norkyst,mixed_layer])
o.set_config('drift:vertical_mixing', True)
o.set_config('vertical_mixing:diffusivitymodel', 'windspeed_Large1994')
o.set_config('chemical:particle_diameter',30.e-6) # m
o.set_config('chemical:particle_diameter_uncertainty',5.e-6) # m
o.set_config('chemical:sediment:resuspension_critvel',0.15) # m/s
o.set_config('chemical:transformations:volatilization', True)
o.set_config('chemical:transformations:degradation', True)
o.set_config('chemical:transformations:degradation_mode', 'OverallRateConstants')
o.set_config('seed:LMM_fraction',.9)
o.set_config('seed:particle_fraction',.1)
o.set_config('general:coastline_action', 'previous')
o.init_chemical_compound("Phenanthrene")
# Modify half-life times with unrealistic values for this demo
o.set_config('chemical:transformations:t12_W_tot', 6.) # hours
o.set_config('chemical:transformations:t12_S_tot', 12.) # hours
o.list_configspec()
07:27:50 DEBUG opendrift.config:168: Adding 18 config items from __init__
07:27:50 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
07:27:50 DEBUG opendrift.config:168: Adding 13 config items from __init__
07:27:51 INFO opendrift:513: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-90-g1dd1995)
07:27:51 DEBUG opendrift.config:168: Adding 19 config items from oceandrift
07:27:51 DEBUG opendrift.config:178: Overwriting config item seed:z
07:27:51 DEBUG opendrift.config:168: Adding 71 config items from chemicaldrift
07:27:51 INFO opendrift.readers:61: Opening file with xr.open_dataset
07:27:51 DEBUG gribapi.bindings:58: eccodes lib search: trying to find binary wheel
07:27:51 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes.libs
07:27:51 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes/.dylibs
07:27:51 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes
07:27:51 DEBUG gribapi.bindings:91: eccodes lib search: did not find library from wheel; try to find as separate lib
07:27:51 DEBUG gribapi.bindings:99: eccodes lib search: findlibs returned /opt/conda/envs/opendrift/lib/libeccodes.so
07:27:52 DEBUG opendrift.readers.reader_netCDF_CF_generic:128: Finding coordinate variables.
07:27:52 DEBUG opendrift.readers.reader_netCDF_CF_generic:143: Parsing CF grid mapping dictionary: {'grid_mapping_name': 'polar_stereographic', 'straight_vertical_longitude_from_pole': np.float64(70.0), 'latitude_of_projection_origin': np.float64(90.0), 'standard_parallel': np.float64(60.0), 'false_easting': np.float64(3192800.0), 'false_northing': np.float64(1784000.0), 'semi_major_axis': np.float64(6378137.0), 'semi_minor_axis': np.float64(6356752.3142), 'proj4': '+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs'}
07:27:52 DEBUG pyproj:40: PROJ_ERROR: proj_create: several objects matching this name: Krovak (Greenwich), Equal Earth Greenwich, Laborde Grid (Greenwich), Modified Krovak (Greenwich), Krovak East North (Greenwich), Modified Krovak East North (Greenwich), ...
07:27:52 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
07:27:52 DEBUG opendrift.readers.reader_netCDF_CF_generic:368: Skipped variables without standard_name: ['angle', 'tke', 'ubar', 'vbar']
07:27:52 DEBUG opendrift.readers.basereader.variables:614: Setting buffer size 25 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 5 m/s and time span of 1:00:00
07:27:52 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
07:27:52 DEBUG opendrift.readers.basereader.variables:569: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
07:27:52 DEBUG opendrift.readers.basereader.variables:569: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
07:27:52 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
07:27:52 DEBUG opendrift.models.basemodel.environment:312: Added reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:27:52 DEBUG opendrift.models.basemodel.environment:312: Added reader constant_reader
environment:constant:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:sea_surface_height [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_surface_height [0] float min: None, max: None [None] Fallback value for s...
environment:constant:x_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:x_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:y_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:y_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:fallback:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:constant:sea_floor_depth_below_sea_level [None] float min: -20, max: 12000 [None] Depth of seafloor...
environment:fallback:sea_floor_depth_below_sea_level [10000] float min: -20, max: 12000 [None] Depth of seafloor...
environment:constant:ocean_vertical_diffusivity [None] float min: 0, max: 1 [None] Use constant value f...
environment:fallback:ocean_vertical_diffusivity [0.0001] float min: 0, max: 1 [None] Fallback value for o...
environment:constant:sea_water_temperature [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_temperature [10] float min: None, max: None [None] Fallback value for s...
environment:constant:sea_water_salinity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_salinity [34] float min: None, max: None [None] Fallback value for s...
environment:constant:upward_sea_water_velocity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:upward_sea_water_velocity [0] float min: None, max: None [None] Fallback value for u...
environment:constant:spm [None] float min: None, max: None [None] Use constant value f...
environment:fallback:spm [1] float min: None, max: None [None] Fallback value for s...
environment:constant:ocean_mixed_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:ocean_mixed_layer_thickness [50] float min: None, max: None [None] Fallback value for o...
environment:constant:active_sediment_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:active_sediment_layer_thickness [0.03] float min: None, max: None [None] Fallback value for a...
environment:constant:doc [None] float min: None, max: None [None] Use constant value f...
environment:fallback:doc [0.0] float min: None, max: None [None] Fallback value for d...
environment:constant:sea_water_ph_reported_on_total_scale [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_ph_reported_on_total_scale [8.1] float min: None, max: None [None] Fallback value for s...
environment:constant:pH_sediment [None] float min: None, max: None [None] Use constant value f...
environment:fallback:pH_sediment [6.9] float min: None, max: None [None] Fallback value for p...
general:use_auto_landmask [True] bool A built-in GSHHG glo...
drift:current_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:current_uncertainty_uniform [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:max_speed [2.0] float min: 0, max: inf [seconds] Typical maximum spee...
readers:max_number_of_fails [1] int min: 0, max: 1000000.0 [number] Readers are discarde...
general:simulation_name [] str min length 0, max length 64 Name of simulation...
general:coastline_action [previous] enum ['none', 'stranding', 'previous'] None means that obje...
general:coastline_approximation_precision [0.001] float min: 0.0001, max: 0.005 [degrees] The precision of the...
general:time_step_minutes [60] float min: 0.01, max: 1440 [minutes] Calculation time ste...
general:time_step_output_minutes [None] float min: 1, max: 1440 [minutes] Output time step, i....
seed:ocean_only [True] bool If True, elements se...
seed:number [1] int min: 1, max: 100000000 [1] The number of elemen...
drift:max_age_seconds [None] float min: 0, max: inf [seconds] Elements will be dea...
drift:advection_scheme [euler] enum ['euler', 'runge-kutta', 'runge-kutta4'] Numerical advection ...
drift:horizontal_diffusivity [0] float min: 0, max: 100000 [m2/s] Add horizontal diffu...
drift:profiles_depth [50] float min: 0, max: None [meters] Environment profiles...
drift:wind_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:relative_wind [False] bool If True, wind drift ...
drift:deactivate_north_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_south_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_east_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
drift:deactivate_west_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
seed:origin_marker [0] float min: None, max: None [None] An integer kept cons...
seed:z [0] float min: -10000, max: 0 [m] Depth below sea leve...
seed:wind_drift_factor [0.02] float min: None, max: None [1] Elements at surface ...
seed:current_drift_factor [1] float min: None, max: None [1] Elements are moved w...
seed:terminal_velocity [0.0] float min: None, max: None [m/s] Terminal rise/sinkin...
seed:diameter [0.0] float min: None, max: None [m] Seeding value of dia...
seed:density [2650.0] float min: None, max: None [kg/m^3] Seeding value of den...
seed:specie [0] float min: None, max: None [] Seeding value of spe...
seed:mass [1000.0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_water [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_sediment [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_volatilized [0] float min: None, max: None [ug] Seeding value of mas...
drift:vertical_advection [True] bool Advect elements with...
drift:vertical_advection_at_surface [True] bool If vertical advectio...
drift:water_column_stretching [False] bool If sea surface eleva...
drift:vertical_advection_correction [False] bool The vertical velocit...
drift:vertical_mixing [True] bool Activate vertical mi...
drift:vertical_mixing_at_surface [True] bool If vertical mixing i...
vertical_mixing:timestep [60] float min: 0.1, max: 3600 [seconds] Time step used for i...
vertical_mixing:diffusivitymodel [windspeed_Large1994] enum ['environment', 'stepfunction', 'windspeed_Sundby1983', 'windspeed_Large1994', 'constant'] Algorithm/source use...
vertical_mixing:background_diffusivity [1.2e-05] float min: 0, max: 1 [m2s-1] Background diffusivi...
vertical_mixing:TSprofiles [False] bool Update T and S profi...
drift:wind_drift_depth [0.1] float min: 0, max: 10 [meters] The direct wind drif...
drift:stokes_drift [True] bool Advection elements w...
drift:stokes_drift_profile [Phillips] enum ['monochromatic', 'exponential', 'Phillips', 'windsea_swell'] Algorithm to calcula...
drift:use_tabularised_stokes_drift [False] bool If True, Stokes drif...
drift:tabularised_stokes_drift_fetch [25000] enum ['5000', '25000', '50000'] The fetch length whe...
general:seafloor_action [lift_to_seafloor] enum ['none', 'lift_to_seafloor', 'deactivate', 'previous'] "deactivate": elemen...
drift:truncate_ocean_model_below_m [None] float min: 0, max: 10000 [m] Ocean model data are...
seed:seafloor [False] bool Elements are seeded ...
chemical:transfer_setup [organics] enum ['Sandnesfj_Al', 'metals', '137Cs_rev', 'custom', 'organics'] ...
chemical:dynamic_partitioning [True] bool Toggle dynamic parti...
chemical:slowly_fraction [False] bool ...
chemical:irreversible_fraction [False] bool ...
chemical:dissolved_diameter [0] float min: 0, max: 0.0001 [m] ...
chemical:particle_diameter [3e-05] float min: 0, max: 0.0001 [m] ...
chemical:particle_concentration_half_depth [20] float min: 0, max: 100 [m] ...
chemical:doc_concentration_half_depth [1000] float min: 0, max: 1000 [m] ...
chemical:particle_diameter_uncertainty [5e-06] float min: 0, max: 0.0001 [m] ...
seed:LMM_fraction [0.9] float min: 0, max: 1 [] ...
seed:particle_fraction [0.1] float min: 0, max: 1 [] ...
chemical:species:LMM [True] bool Toggle LMM species...
chemical:species:LMMcation [False] bool ...
chemical:species:LMManion [False] bool ...
chemical:species:Colloid [False] bool ...
chemical:species:Humic_colloid [False] bool ...
chemical:species:Polymer [False] bool ...
chemical:species:Particle_reversible [True] bool ...
chemical:species:Particle_slowly_reversible [False] bool ...
chemical:species:Particle_irreversible [False] bool ...
chemical:species:Sediment_reversible [True] bool ...
chemical:species:Sediment_slowly_reversible [False] bool ...
chemical:species:Sediment_irreversible [False] bool ...
chemical:transformations:Kd [2.0] float min: 0, max: 1000000000.0 [m3/kg] ...
chemical:transformations:S0 [0.0] float min: 0, max: 100 [PSU] parameter controllin...
chemical:transformations:Dc [1.16e-05] float min: 0, max: 1000000.0 [] ...
chemical:transformations:slow_coeff [0] float min: 0, max: 1000000.0 [] ...
chemical:transformations:volatilization [True] bool Chemical is evaporat...
chemical:transformations:degradation [True] bool Chemical mass is deg...
chemical:transformations:degradation_mode [OverallRateConstants] enum ['OverallRateConstants'] ...
chemical:transformations:dissociation [nondiss] enum ['nondiss', 'acid', 'base', 'amphoter'] ...
chemical:transformations:LogKOW [4.505] float min: -3, max: 10 [Log L/Kg] ...
chemical:transformations:TrefKOW [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_KOC_Sed [-24900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:DeltaH_KOC_DOM [-25900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:Setchenow [0.3026] float min: 0, max: 1 [L/mol] ...
chemical:transformations:pKa_acid [-1] float min: -1, max: 14 [] ...
chemical:transformations:pKa_base [-1] float min: -1, max: 14 [] ...
chemical:transformations:KOC_DOM [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:KOC_sed [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:fOC_SPM [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:fOC_sed [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:aggregation_rate [0] float min: 0, max: 1 [s-1] ...
chemical:transformations:t12_W_tot [6.0] float min: 1, max: None [hours] half life in water, ...
chemical:transformations:Tref_kWt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kWt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:t12_S_tot [12.0] float min: 1, max: None [hours] half life in sedimen...
chemical:transformations:Tref_kSt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kSt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:MolWt [178.226] float min: 50, max: 1000 [amu] molecular weight...
chemical:transformations:Henry [4.294e-05] float min: None, max: None [atm m3 mol-1] Henry constant...
chemical:transformations:Vpress [0.0222] float min: None, max: None [Pa] Vapour pressure...
chemical:transformations:Tref_Vpress [25.0] float min: None, max: None [C] Vapour pressure ref ...
chemical:transformations:DeltaH_Vpress [71733.0] float min: -100000.0, max: 115000.0 [J/mol] Enthalpy of volatili...
chemical:transformations:Solub [1.09] float min: None, max: None [g/m3] Solubility...
chemical:transformations:Tref_Solub [25.0] float min: None, max: None [C] Solubility ref temp...
chemical:transformations:DeltaH_Solub [34800.0] float min: -100000.0, max: 100000.0 [J/mol] Enthalpy of solubili...
chemical:sediment:mixing_depth [0.03] float min: 0, max: 100 [m] ...
chemical:sediment:density [2600] float min: 0, max: 10000 [kg/m3] ...
chemical:sediment:effective_fraction [0.9] float min: 0, max: 1 [] ...
chemical:sediment:corr_factor [0.1] float min: 0, max: 10 [] ...
chemical:sediment:porosity [0.6] float min: 0, max: 1 [] ...
chemical:sediment:layer_thickness [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_critvel [0.15] float min: 0, max: 1 [m/s] ...
chemical:sediment:burial_rate [3e-05] float min: 0, max: 10 [m/year] ...
chemical:sediment:buried_leaking_rate [0] float min: 0, max: 10 [s-1] ...
chemical:compound [Phenanthrene] enum ['Naphthalene', 'Phenanthrene', 'Fluoranthene', 'Benzo-a-anthracene', 'Benzo-a-pyrene', 'Dibenzo-ah-anthracene', 'C1-Naphthalene', 'Acenaphthene', 'Acenaphthylene', 'Fluorene', 'Dibenzothiophene', 'C2-Naphthalene', 'Anthracene', 'C3-Naphthalene', 'C1-Dibenzothiophene', 'Pyrene', 'C1-Phenanthrene', 'C2-Dibenzothiophene', 'C2-Phenanthrene', 'Benzo-b-fluoranthene', 'Chrysene', 'C3-Dibenzothiophene', 'C3-Phenanthrene', 'Benzo-k-fluoranthene', 'Benzo-ghi-perylene', 'Indeno-123cd-pyrene', 'Copper', 'Cadmium', 'Chromium', 'Lead', 'Vanadium', 'Zinc', 'Nickel', None] ...
Seeding 500 lagrangian elements each representign 2mg og target chemical
td=datetime.today()
time = td - timedelta(days=10)
latseed= 57.6; lonseed= 10.6 # Skagen
ntraj=500
iniz=np.random.rand(ntraj) * -10. # seeding the chemicals in the upper 10m
o.seed_elements(lonseed, latseed, z=iniz, radius=2000,number=ntraj,time=time, mass=2e3)
07:27:52 DEBUG opendrift.models.chemicaldrift:894: Partitioning coefficients (Tref,freshwater)
07:27:52 DEBUG opendrift.models.chemicaldrift:895: KOC_sed: 12953.922406542462 L/KgOC
07:27:52 DEBUG opendrift.models.chemicaldrift:896: KOC_SPM: 12953.922406542462 L/KgOC
07:27:52 DEBUG opendrift.models.chemicaldrift:897: KOC_DOM: 3004.29439651874 L/KgOC
07:27:52 DEBUG opendrift.models.chemicaldrift:909: Kd_sed: 647.6961203271231 L/Kg
07:27:52 DEBUG opendrift.models.chemicaldrift:910: Kd_SPM: 647.6961203271231 L/Kg
07:27:52 DEBUG opendrift.models.chemicaldrift:911: Kd_DOM: 1580.2588525688573 L/Kg
07:27:52 DEBUG opendrift.models.chemicaldrift:1128: nspecies: 5
07:27:52 DEBUG opendrift.models.chemicaldrift:1129: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
07:27:52 DEBUG opendrift.models.chemicaldrift:533: Initial partitioning:
07:27:52 DEBUG opendrift.models.chemicaldrift:535: 449 0 LMM
07:27:52 DEBUG opendrift.models.chemicaldrift:535: 0 1 Humic colloid
07:27:52 DEBUG opendrift.models.chemicaldrift:535: 51 2 Particle reversible
07:27:52 DEBUG opendrift.models.chemicaldrift:535: 0 3 Sediment reversible
07:27:52 DEBUG opendrift.models.chemicaldrift:535: 0 4 Sediment slowly reversible
07:27:52 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
07:27:52 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
07:27:56 DEBUG opendrift.models.basemodel.environment:312: Added reader global_landmask
07:27:56 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
07:27:56 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.000100
07:27:56 INFO opendrift.models.basemodel.environment:232: spm: 1.000000
07:27:56 INFO opendrift.models.basemodel.environment:232: active_sediment_layer_thickness: 0.030000
07:27:56 INFO opendrift.models.basemodel.environment:232: doc: 0.000000
07:27:56 INFO opendrift.models.basemodel.environment:232: sea_water_ph_reported_on_total_scale: 8.100000
07:27:56 INFO opendrift.models.basemodel.environment:232: pH_sediment: 6.900000
07:27:56 DEBUG opendrift:101: Changed mode from Mode.Config to Mode.Ready
Running model
o.run(steps=48*2, time_step=1800, time_step_output=1800)
07:27:56 DEBUG opendrift:101: Changed mode from Mode.Ready to Mode.Run
07:27:56 DEBUG opendrift:1704:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.14.2
Platform: Linux, 6.8.0-1031-aws
4.0 GB memory
36 processors (x86_64)
NumPy version 2.3.1
SciPy version 1.16.0
Matplotlib version 3.10.3
NetCDF4 version 1.7.2
Xarray version 2025.7.1
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 2.2.0
Python version 3.13.5 | packaged by conda-forge | (main, Jun 16 2025, 08:27:50) [GCC 13.3.0]
------------------------------------------------------
07:27:56 DEBUG opendrift:1717: No output file is specified, neglecting export_buffer_length
07:27:56 INFO opendrift:1743: Storing previous values of element property lon because of condition (('general:coastline_action', 'in', ['stranding', 'previous']), 'or', ('general:seafloor_action', 'in', ['previous']))
07:27:56 INFO opendrift:1743: Storing previous values of element property lat because of condition (('general:coastline_action', 'in', ['stranding', 'previous']), 'or', ('general:seafloor_action', 'in', ['previous']))
07:27:56 DEBUG opendrift:1866: Finalizing environment and preparing readers for simulation coverage ([ 4.69639997 54.43270917 16.51679392 60.76164202]) and time (2025-07-07 07:27:52.765301 to 2025-07-09 07:27:52.765301)
07:27:56 DEBUG opendrift.models.basemodel.environment:168: Preparing https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be for extent [ 4.69639997 54.43270917 16.51679392 60.76164202]
07:27:56 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be before starting new simulation
07:27:56 DEBUG opendrift.readers.basereader.variables:614: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
07:27:56 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:27:56 DEBUG opendrift.models.basemodel.environment:168: Preparing constant_reader for extent [ 4.69639997 54.43270917 16.51679392 60.76164202]
07:27:56 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for constant_reader
07:27:56 DEBUG opendrift.models.basemodel.environment:168: Preparing global_landmask for extent [ 4.69639997 54.43270917 16.51679392 60.76164202]
07:27:56 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for global_landmask
07:27:56 DEBUG opendrift:1953: Initial self.result, size Frozen({'trajectory': 500, 'time': 97})
07:27:56 INFO opendrift:899: Using existing reader for land_binary_mask
07:27:56 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:27:56 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:27:56 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:27:56 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:27:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:27:56 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:27:56 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:27:56 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:27:56 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:27:56 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:27:56 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:27:56 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:27:56 INFO opendrift:928: All points are in ocean
07:27:56 INFO opendrift.models.chemicaldrift:355: Number of species: 5
07:27:56 INFO opendrift.models.chemicaldrift:357: 0 LMM
07:27:56 INFO opendrift.models.chemicaldrift:357: 1 Humic colloid
07:27:56 INFO opendrift.models.chemicaldrift:357: 2 Particle reversible
07:27:56 INFO opendrift.models.chemicaldrift:357: 3 Sediment reversible
07:27:56 INFO opendrift.models.chemicaldrift:357: 4 Sediment slowly reversible
07:27:56 INFO opendrift.models.chemicaldrift:360: transfer setup: organics
07:27:56 INFO opendrift.models.chemicaldrift:362: nspecies: 5
07:27:56 INFO opendrift.models.chemicaldrift:363: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
07:27:56 DEBUG opendrift:856: to be seeded: 500, already seeded 0
07:27:56 DEBUG opendrift:878: Released 500 new elements.
07:27:56 DEBUG opendrift:2034: ======================================================================
07:27:56 INFO opendrift:2035: 2025-07-07 07:27:52.765301 - step 1 of 96 - 500 active elements (0 deactivated)
07:27:56 DEBUG opendrift:2041: 0 elements scheduled.
07:27:56 DEBUG opendrift:2043: ======================================================================
07:27:56 DEBUG opendrift:2054: 57.54622268676758 <- latitude -> 57.648128509521484
07:27:56 DEBUG opendrift:2054: 10.507113456726074 <- longitude -> 10.706080436706543
07:27:56 DEBUG opendrift:2054: -9.988470077514648 <- z -> -0.04695476219058037
07:27:56 DEBUG opendrift:2055: ---------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:27:56 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:27:56 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:27:56 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:27:56 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:27:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 07:00:00 (before)
2025-07-07 08:00:00 (after)
07:27:59 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:27:59 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:27:59 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:27:59 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:27:59 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:27:59 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:27:59 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 37x35x7) for time before (2025-07-07 07:00:00)
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:01 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:01 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 37x35x7) for time after (2025-07-07 08:00:00)
07:28:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 07:00:00) in space (linearNDFast)
07:28:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 08:00:00) in space (linearNDFast)
07:28:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 07:00:00, weight 0.54) and
after (2025-07-07 08:00:00, weight 0.46) in time
07:28:01 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:01 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.4929004930107 and -59.29392585004879 degrees.
07:28:01 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.4929004930107 and -59.29392585004879 degrees.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0471336 (min) 0.147604 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.139886 (min) 0.250241 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.358316 (min) -0.33576 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.646354 (min) 0.530744 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.50032 (min) 2.86846 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.53077 (min) 23.882 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6103 (min) 16.134 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1609 (min) 31.605 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.18768e-05 (min) 4.71995e-05 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:01 DEBUG opendrift:685: No elements hit coastline.
07:28:01 DEBUG opendrift:714: No elements hit seafloor.
07:28:01 DEBUG opendrift:1636: No elements to deactivate
07:28:01 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:01 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:01 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:01 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:28:01 DEBUG opendrift.models.chemicaldrift:1476: old species: [2]
07:28:01 DEBUG opendrift.models.chemicaldrift:1477: new species: [0]
07:28:01 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]]
07:28:01 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:01 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:01 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:01 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:01 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005961349609897279
07:28:01 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:01 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:01 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:01 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:01 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:01 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:01 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(450), np.int64(0), np.int64(50), np.int64(0), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:01 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:01 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:01 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:01 DEBUG opendrift:2034: ======================================================================
07:28:01 INFO opendrift:2035: 2025-07-07 07:57:52.765301 - step 2 of 96 - 500 active elements (0 deactivated)
07:28:01 DEBUG opendrift:2041: 0 elements scheduled.
07:28:01 DEBUG opendrift:2043: ======================================================================
07:28:01 DEBUG opendrift:2054: 57.54983874727096 <- latitude -> 57.65166668727702
07:28:01 DEBUG opendrift:2054: 10.508136099841403 <- longitude -> 10.707891893850942
07:28:01 DEBUG opendrift:2054: -20.318856751972397 <- z -> -0.14409695677136392
07:28:01 DEBUG opendrift:2055: ---------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 07:00:00 (before)
2025-07-07 08:00:00 (after)
07:28:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 07:00:00) in space (linearNDFast)
07:28:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 08:00:00) in space (linearNDFast)
07:28:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 07:00:00, weight 0.04) and
after (2025-07-07 08:00:00, weight 0.96) in time
07:28:01 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:01 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.491874988001676 and -59.29211916800444 degrees.
07:28:01 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.491874988001676 and -59.29211916800444 degrees.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:01 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0583624 (min) 0.152791 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.125367 (min) 0.24688 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.367684 (min) -0.345338 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.27839 (min) 0.224865 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.10867 (min) 2.80757 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.50779 (min) 23.8285 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.59 (min) 16.1459 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1627 (min) 31.8254 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.15177e-05 (min) 5.67649e-05 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:01 DEBUG opendrift:685: No elements hit coastline.
07:28:01 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:01 DEBUG opendrift:1636: No elements to deactivate
07:28:01 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:01 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:01 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:01 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 8
07:28:01 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 2 0 0]
07:28:01 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 0 3 3]
07:28:01 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[0. 0. 0. 7. 0.]
[0. 0. 0. 0. 0.]
[2. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]]
07:28:01 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:01 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:01 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:01 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:01 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005890156607091151
07:28:01 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:01 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:01 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:01 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:01 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:01 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:01 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:01 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:01 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:01 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:01 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:01 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:01 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:01 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:01 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(444), np.int64(0), np.int64(46), np.int64(10), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:01 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:01 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:01 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:01 DEBUG opendrift:2034: ======================================================================
07:28:01 INFO opendrift:2035: 2025-07-07 08:27:52.765301 - step 3 of 96 - 500 active elements (0 deactivated)
07:28:01 DEBUG opendrift:2041: 0 elements scheduled.
07:28:01 DEBUG opendrift:2043: ======================================================================
07:28:01 DEBUG opendrift:2054: 57.55382889726922 <- latitude -> 57.655004342402066
07:28:01 DEBUG opendrift:2054: 10.509302980729913 <- longitude -> 10.70983543534054
07:28:01 DEBUG opendrift:2054: -20.958398818969727 <- z -> -0.09427655616855814
07:28:01 DEBUG opendrift:2055: ---------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 08:00:00 (before)
2025-07-07 09:00:00 (after)
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:03 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:03 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x34x7) for time after (2025-07-07 09:00:00)
07:28:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 08:00:00) in space (linearNDFast)
07:28:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 09:00:00) in space (linearNDFast)
07:28:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 08:00:00, weight 0.54) and
after (2025-07-07 09:00:00, weight 0.46) in time
07:28:03 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:03 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.490708096902715 and -59.29017561854913 degrees.
07:28:03 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.490708096902715 and -59.29017561854913 degrees.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:03 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0549596 (min) 0.150737 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.114878 (min) 0.232672 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.361661 (min) -0.339905 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.94443 (min) 0.00313593 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.02553 (min) 2.5598 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.48267 (min) 23.7823 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5829 (min) 16.1296 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1651 (min) 31.8351 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.70325e-05 (min) 6.78277e-05 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:03 DEBUG opendrift:685: No elements hit coastline.
07:28:03 DEBUG opendrift:714: No elements hit seafloor.
07:28:03 DEBUG opendrift:1636: No elements to deactivate
07:28:03 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:03 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:03 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:03 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:03 DEBUG opendrift.models.chemicaldrift:1476: old species: [2 0 0 0 0 0]
07:28:03 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3 3 3 3 3]
07:28:03 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 12. 0.]
[ 0. 0. 0. 0. 0.]
[ 3. 0. 0. 3. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:03 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:03 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:03 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:03 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:03 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005596450331429396
07:28:03 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:03 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:03 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:03 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:03 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:03 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:03 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:03 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:03 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:03 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:03 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(440), np.int64(0), np.int64(43), np.int64(17), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:03 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:03 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:03 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:03 DEBUG opendrift:2034: ======================================================================
07:28:03 INFO opendrift:2035: 2025-07-07 08:57:52.765301 - step 4 of 96 - 500 active elements (0 deactivated)
07:28:03 DEBUG opendrift:2041: 0 elements scheduled.
07:28:03 DEBUG opendrift:2043: ======================================================================
07:28:03 DEBUG opendrift:2054: 57.55747418742786 <- latitude -> 57.65795316829585
07:28:03 DEBUG opendrift:2054: 10.510495841791503 <- longitude -> 10.711687101894954
07:28:03 DEBUG opendrift:2054: -20.958398818969727 <- z -> -0.1433944415517745
07:28:03 DEBUG opendrift:2055: ---------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 08:00:00 (before)
2025-07-07 09:00:00 (after)
07:28:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 08:00:00) in space (linearNDFast)
07:28:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 09:00:00) in space (linearNDFast)
07:28:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 08:00:00, weight 0.04) and
after (2025-07-07 09:00:00, weight 0.96) in time
07:28:03 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:03 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48951524767088 and -59.28832396676054 degrees.
07:28:03 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48951524767088 and -59.28832396676054 degrees.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:03 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:03 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:03 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0620571 (min) 0.147164 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.103894 (min) 0.227141 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.355 (min) -0.3331 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.60425 (min) -0.182603 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.920186 (min) 2.50496 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.46032 (min) 23.7218 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5871 (min) 16.149 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1673 (min) 31.8426 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.7771e-05 (min) 8.03152e-05 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:03 DEBUG opendrift:685: No elements hit coastline.
07:28:03 DEBUG opendrift:714: No elements hit seafloor.
07:28:03 DEBUG opendrift:1636: No elements to deactivate
07:28:03 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:03 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:03 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:03 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 9
07:28:03 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 2 0 0 2 0 0]
07:28:03 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 0 3 3 0 3 3]
07:28:03 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 19. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 5. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:03 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:03 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:03 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:03 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:03 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0060441189833982875
07:28:03 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:03 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:03 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:03 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:03 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:03 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:03 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:03 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:03 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:03 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:03 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(435), np.int64(0), np.int64(41), np.int64(24), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:03 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:03 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:03 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:03 DEBUG opendrift:2034: ======================================================================
07:28:03 INFO opendrift:2035: 2025-07-07 09:27:52.765301 - step 5 of 96 - 500 active elements (0 deactivated)
07:28:03 DEBUG opendrift:2041: 0 elements scheduled.
07:28:03 DEBUG opendrift:2043: ======================================================================
07:28:03 DEBUG opendrift:2054: 57.5611453229737 <- latitude -> 57.66049997853574
07:28:03 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.713582315202306
07:28:03 DEBUG opendrift:2054: -20.958398818969727 <- z -> -0.182315760053171
07:28:03 DEBUG opendrift:2055: ---------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:03 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 09:00:00 (before)
2025-07-07 10:00:00 (after)
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:05 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:05 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x34x7) for time after (2025-07-07 10:00:00)
07:28:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 09:00:00) in space (linearNDFast)
07:28:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 10:00:00) in space (linearNDFast)
07:28:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 09:00:00, weight 0.54) and
after (2025-07-07 10:00:00, weight 0.46) in time
07:28:05 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:05 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2864287484331 degrees.
07:28:05 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2864287484331 degrees.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:05 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:05 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0509637 (min) 0.132322 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0865198 (min) 0.193721 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.321625 (min) -0.301218 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.71167 (min) -0.991961 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.30659 (min) 2.07273 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.6669 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.58 (min) 16.1655 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1701 (min) 31.8477 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.38732e-05 (min) 9.60349e-05 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:05 DEBUG opendrift:685: No elements hit coastline.
07:28:05 DEBUG opendrift:714: No elements hit seafloor.
07:28:05 DEBUG opendrift:1636: No elements to deactivate
07:28:05 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:05 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:05 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:05 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 12
07:28:05 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 2 0 0 0 0 0 0 0 0]
07:28:05 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 0 3 3 3 3 3 3 3 3]
07:28:05 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 30. 0.]
[ 0. 0. 0. 0. 0.]
[ 6. 0. 0. 5. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:05 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:05 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:05 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:05 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:05 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.006733259465902438
07:28:05 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:05 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:05 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:548: 11 elements reached seafloor, interacting with bottom
07:28:05 DEBUG opendrift:719: Lifting 11 elements to seafloor.
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:05 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:05 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:05 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:05 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:05 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(425), np.int64(0), np.int64(39), np.int64(36), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:05 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:05 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:05 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:05 DEBUG opendrift:2034: ======================================================================
07:28:05 INFO opendrift:2035: 2025-07-07 09:57:52.765301 - step 6 of 96 - 500 active elements (0 deactivated)
07:28:05 DEBUG opendrift:2041: 0 elements scheduled.
07:28:05 DEBUG opendrift:2043: ======================================================================
07:28:05 DEBUG opendrift:2054: 57.5640084639195 <- latitude -> 57.66234116488163
07:28:05 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.715603841503206
07:28:05 DEBUG opendrift:2054: -20.958398818969727 <- z -> -0.062148856576980105
07:28:05 DEBUG opendrift:2055: ---------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 09:00:00 (before)
2025-07-07 10:00:00 (after)
07:28:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 09:00:00) in space (linearNDFast)
07:28:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 10:00:00) in space (linearNDFast)
07:28:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 09:00:00, weight 0.04) and
after (2025-07-07 10:00:00, weight 0.96) in time
07:28:05 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:05 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.284407225157764 degrees.
07:28:05 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.284407225157764 degrees.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:05 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:05 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:05 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:05 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:05 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:05 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0458659 (min) 0.120298 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0597051 (min) 0.170772 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.286632 (min) -0.265101 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.7752 (min) -1.87933 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.11682 (min) 1.78684 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.6362 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5874 (min) 16.1858 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1725 (min) 31.9512 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.45426e-05 (min) 9.23173e-05 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:05 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:05 DEBUG opendrift:685: No elements hit coastline.
07:28:05 DEBUG opendrift:714: No elements hit seafloor.
07:28:05 DEBUG opendrift:1636: No elements to deactivate
07:28:05 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:05 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:05 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:05 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:05 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 2 0 0]
07:28:05 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3]
07:28:05 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 33. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 6. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:05 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:05 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:05 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:05 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:05 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.007648099900615213
07:28:05 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:05 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:05 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:05 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:28:05 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:05 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:05 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:05 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:05 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:05 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:05 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:05 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(423), np.int64(0), np.int64(37), np.int64(40), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:05 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:05 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:05 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:05 DEBUG opendrift:2034: ======================================================================
07:28:05 INFO opendrift:2035: 2025-07-07 10:27:52.765301 - step 7 of 96 - 500 active elements (0 deactivated)
07:28:05 DEBUG opendrift:2041: 0 elements scheduled.
07:28:05 DEBUG opendrift:2043: ======================================================================
07:28:05 DEBUG opendrift:2054: 57.56638511384269 <- latitude -> 57.66351341791315
07:28:05 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.71789560860608
07:28:05 DEBUG opendrift:2054: -21.36562728881836 <- z -> 0.0
07:28:05 DEBUG opendrift:2055: ---------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:05 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 10:00:00 (before)
2025-07-07 11:00:00 (after)
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:07 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:07 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x34x7) for time after (2025-07-07 11:00:00)
07:28:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 10:00:00) in space (linearNDFast)
07:28:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 11:00:00) in space (linearNDFast)
07:28:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 10:00:00, weight 0.54) and
after (2025-07-07 11:00:00, weight 0.46) in time
07:28:07 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:07 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.282115449930814 degrees.
07:28:07 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.282115449930814 degrees.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0592572 (min) 0.10555 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0227428 (min) 0.136457 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.232351 (min) -0.213528 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.63682 (min) -2.07679 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.33806 (min) 1.92123 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.6251 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5846 (min) 16.201 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1745 (min) 31.9453 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.90001e-05 (min) 0.000108042 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:07 DEBUG opendrift:685: No elements hit coastline.
07:28:07 DEBUG opendrift:714: No elements hit seafloor.
07:28:07 DEBUG opendrift:1636: No elements to deactivate
07:28:07 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:07 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:07 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:07 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 8
07:28:07 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0]
07:28:07 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3]
07:28:07 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 41. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 7. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:07 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:07 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:07 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:07 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:07 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.007526209966997204
07:28:07 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:07 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:07 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 8 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 8 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:07 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:07 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:07 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:07 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(415), np.int64(0), np.int64(35), np.int64(50), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:07 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:07 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:07 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:07 DEBUG opendrift:2034: ======================================================================
07:28:07 INFO opendrift:2035: 2025-07-07 10:57:52.765301 - step 8 of 96 - 500 active elements (0 deactivated)
07:28:07 DEBUG opendrift:2041: 0 elements scheduled.
07:28:07 DEBUG opendrift:2043: ======================================================================
07:28:07 DEBUG opendrift:2054: 57.56844798076591 <- latitude -> 57.66389432173953
07:28:07 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.720286033310206
07:28:07 DEBUG opendrift:2054: -21.36562728881836 <- z -> -0.09099001185914668
07:28:07 DEBUG opendrift:2055: ---------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 10:00:00 (before)
2025-07-07 11:00:00 (after)
07:28:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 10:00:00) in space (linearNDFast)
07:28:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 11:00:00) in space (linearNDFast)
07:28:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 10:00:00, weight 0.04) and
after (2025-07-07 11:00:00, weight 0.96) in time
07:28:07 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:07 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27972502441569 degrees.
07:28:07 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27972502441569 degrees.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:07 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0631123 (min) 0.0936446 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0209559 (min) 0.11854 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.1765 (min) -0.161206 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.48308 (min) -2.05669 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.58873 (min) 2.0442 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.6549 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5895 (min) 16.2298 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1777 (min) 31.9416 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.90665e-05 (min) 0.000112583 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:07 DEBUG opendrift:685: No elements hit coastline.
07:28:07 DEBUG opendrift:714: No elements hit seafloor.
07:28:07 DEBUG opendrift:1636: No elements to deactivate
07:28:07 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:07 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:07 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:07 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:07 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0]
07:28:07 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3]
07:28:07 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 47. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 9. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:07 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:07 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:07 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:07 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:07 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.007376150511642552
07:28:07 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:07 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:07 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:07 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:07 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:07 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:07 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:07 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:07 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:07 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(409), np.int64(0), np.int64(32), np.int64(59), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:07 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:07 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:07 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:07 DEBUG opendrift:2034: ======================================================================
07:28:07 INFO opendrift:2035: 2025-07-07 11:27:52.765301 - step 9 of 96 - 500 active elements (0 deactivated)
07:28:07 DEBUG opendrift:2041: 0 elements scheduled.
07:28:07 DEBUG opendrift:2043: ======================================================================
07:28:07 DEBUG opendrift:2054: 57.57036386480739 <- latitude -> 57.663666876068575
07:28:07 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.72272148102675
07:28:07 DEBUG opendrift:2054: -21.36562728881836 <- z -> -0.10257311144290215
07:28:07 DEBUG opendrift:2055: ---------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 11:00:00 (before)
2025-07-07 12:00:00 (after)
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:09 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:09 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x35x7) for time after (2025-07-07 12:00:00)
07:28:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 11:00:00) in space (linearNDFast)
07:28:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 12:00:00) in space (linearNDFast)
07:28:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 11:00:00, weight 0.54) and
after (2025-07-07 12:00:00, weight 0.46) in time
07:28:09 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.277289579545645 degrees.
07:28:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.277289579545645 degrees.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0589286 (min) 0.0863122 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0444307 (min) 0.0889262 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.124628 (min) -0.114931 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.30303 (min) -1.74896 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.54947 (min) 2.40695 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.7198 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.59 (min) 16.2537 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2257 (min) 31.9353 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.04675e-05 (min) 0.000102909 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:09 DEBUG opendrift:685: No elements hit coastline.
07:28:09 DEBUG opendrift:714: No elements hit seafloor.
07:28:09 DEBUG opendrift:1636: No elements to deactivate
07:28:09 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:09 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:09 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:09 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 15
07:28:09 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
07:28:09 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
07:28:09 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 62. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 12. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:09 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:09 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:09 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.008023117470760902
07:28:09 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:09 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:09 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 15 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 15 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:09 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:09 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(394), np.int64(0), np.int64(29), np.int64(77), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:09 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:09 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:09 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:09 DEBUG opendrift:2034: ======================================================================
07:28:09 INFO opendrift:2035: 2025-07-07 11:57:52.765301 - step 10 of 96 - 500 active elements (0 deactivated)
07:28:09 DEBUG opendrift:2041: 0 elements scheduled.
07:28:09 DEBUG opendrift:2043: ======================================================================
07:28:09 DEBUG opendrift:2054: 57.57169582862139 <- latitude -> 57.66294876808988
07:28:09 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.725107629309932
07:28:09 DEBUG opendrift:2054: -21.36562728881836 <- z -> -0.13682267358957323
07:28:09 DEBUG opendrift:2055: ---------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 11:00:00 (before)
2025-07-07 12:00:00 (after)
07:28:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 11:00:00) in space (linearNDFast)
07:28:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 12:00:00) in space (linearNDFast)
07:28:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 11:00:00, weight 0.04) and
after (2025-07-07 12:00:00, weight 0.96) in time
07:28:09 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.274903431359384 degrees.
07:28:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.274903431359384 degrees.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.054366 (min) 0.0823359 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0661125 (min) 0.0705866 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0733793 (min) -0.0692329 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.13233 (min) -1.2399 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.46987 (min) 2.8237 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.8106 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.59 (min) 16.2763 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1829 (min) 31.8649 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.01516e-05 (min) 9.25287e-05 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:09 DEBUG opendrift:685: No elements hit coastline.
07:28:09 DEBUG opendrift:714: No elements hit seafloor.
07:28:09 DEBUG opendrift:1636: No elements to deactivate
07:28:09 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:09 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:09 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:09 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 9
07:28:09 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0]
07:28:09 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3]
07:28:09 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 71. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 15. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:09 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:09 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:09 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.009016340244014414
07:28:09 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:09 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:09 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:09 DEBUG opendrift.models.oceandrift:548: 9 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 9 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:09 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:09 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:09 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:09 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(385), np.int64(0), np.int64(26), np.int64(89), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:09 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:09 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:09 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:09 DEBUG opendrift:2034: ======================================================================
07:28:09 INFO opendrift:2035: 2025-07-07 12:27:52.765301 - step 11 of 96 - 500 active elements (0 deactivated)
07:28:09 DEBUG opendrift:2041: 0 elements scheduled.
07:28:09 DEBUG opendrift:2043: ======================================================================
07:28:09 DEBUG opendrift:2054: 57.572648042755894 <- latitude -> 57.661880243231245
07:28:09 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.727191262859858
07:28:09 DEBUG opendrift:2054: -21.423494338989258 <- z -> -0.09564935167273117
07:28:09 DEBUG opendrift:2055: ---------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 12:00:00 (before)
2025-07-07 13:00:00 (after)
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:11 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:11 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x35x7) for time after (2025-07-07 13:00:00)
07:28:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 12:00:00) in space (linearNDFast)
07:28:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 13:00:00) in space (linearNDFast)
07:28:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 12:00:00, weight 0.54) and
after (2025-07-07 13:00:00, weight 0.46) in time
07:28:11 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27281980006234 degrees.
07:28:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27281980006234 degrees.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:11 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0551207 (min) 0.0775456 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0777939 (min) 0.0625125 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0466949 (min) -0.0428378 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.51778 (min) -1.03071 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.32994 (min) 2.26868 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 23.9271 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5849 (min) 16.2545 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1856 (min) 31.8769 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.55414e-05 (min) 7.79951e-05 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:11 DEBUG opendrift:685: No elements hit coastline.
07:28:11 DEBUG opendrift:714: No elements hit seafloor.
07:28:11 DEBUG opendrift:1636: No elements to deactivate
07:28:11 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:11 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:11 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:11 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 8
07:28:11 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0]
07:28:11 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3]
07:28:11 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 79. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 18. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:11 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:11 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:11 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0052264998506523636
07:28:11 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:11 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:11 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:11 DEBUG opendrift.models.oceandrift:548: 8 elements reached seafloor, interacting with bottom
07:28:11 DEBUG opendrift:719: Lifting 8 elements to seafloor.
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:11 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:11 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:11 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(377), np.int64(0), np.int64(26), np.int64(97), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:11 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:11 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:11 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:11 DEBUG opendrift:2034: ======================================================================
07:28:11 INFO opendrift:2035: 2025-07-07 12:57:52.765301 - step 12 of 96 - 500 active elements (0 deactivated)
07:28:11 DEBUG opendrift:2041: 0 elements scheduled.
07:28:11 DEBUG opendrift:2043: ======================================================================
07:28:11 DEBUG opendrift:2054: 57.57345184654709 <- latitude -> 57.661204423536034
07:28:11 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.72895331745727
07:28:11 DEBUG opendrift:2054: -21.423494338989258 <- z -> -0.19439120570813523
07:28:11 DEBUG opendrift:2055: ---------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 12:00:00 (before)
2025-07-07 13:00:00 (after)
07:28:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 12:00:00) in space (linearNDFast)
07:28:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 13:00:00) in space (linearNDFast)
07:28:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 12:00:00, weight 0.04) and
after (2025-07-07 13:00:00, weight 0.96) in time
07:28:11 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27105773565039 degrees.
07:28:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.27105773565039 degrees.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:11 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:11 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:11 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:11 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0459682 (min) 0.0731153 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0946258 (min) 0.0604407 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.025894 (min) -0.0161979 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.1592 (min) -0.599581 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.16062 (min) 1.70542 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.0491 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5899 (min) 16.2892 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2175 (min) 31.8772 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000105328 (min) 6.31457e-05 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:11 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:11 DEBUG opendrift:685: No elements hit coastline.
07:28:11 DEBUG opendrift:714: No elements hit seafloor.
07:28:11 DEBUG opendrift:1636: No elements to deactivate
07:28:11 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:11 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:11 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:11 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 13
07:28:11 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0]
07:28:11 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3]
07:28:11 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 92. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 18. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:11 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:11 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:11 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0030766208131817467
07:28:11 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:11 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:11 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:11 DEBUG opendrift.models.oceandrift:548: 13 elements reached seafloor, interacting with bottom
07:28:11 DEBUG opendrift:719: Lifting 13 elements to seafloor.
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:11 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:11 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:11 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:11 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(364), np.int64(0), np.int64(25), np.int64(111), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:11 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:11 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:11 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:11 DEBUG opendrift:2034: ======================================================================
07:28:11 INFO opendrift:2035: 2025-07-07 13:27:52.765301 - step 13 of 96 - 500 active elements (0 deactivated)
07:28:11 DEBUG opendrift:2041: 0 elements scheduled.
07:28:11 DEBUG opendrift:2043: ======================================================================
07:28:11 DEBUG opendrift:2054: 57.57407921198555 <- latitude -> 57.66041158209429
07:28:11 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.730201879312714
07:28:11 DEBUG opendrift:2054: -21.926971435546875 <- z -> -0.26859258533360814
07:28:11 DEBUG opendrift:2055: ---------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 13:00:00 (before)
2025-07-07 14:00:00 (after)
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:13 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:13 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x35x7) for time after (2025-07-07 14:00:00)
07:28:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 13:00:00) in space (linearNDFast)
07:28:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 14:00:00) in space (linearNDFast)
07:28:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 13:00:00, weight 0.54) and
after (2025-07-07 14:00:00, weight 0.46) in time
07:28:13 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.269809170397615 degrees.
07:28:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.269809170397615 degrees.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:13 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0358628 (min) 0.068163 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0809774 (min) 0.0735693 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0366888 (min) -0.0245273 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.70741 (min) -0.92484 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.0725385 (min) 0.652953 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.172 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5906 (min) 16.3004 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2198 (min) 31.8783 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00010369 (min) 4.86517e-05 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:13 DEBUG opendrift:685: No elements hit coastline.
07:28:13 DEBUG opendrift:719: Lifting 14 elements to seafloor.
07:28:13 DEBUG opendrift:1636: No elements to deactivate
07:28:13 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:13 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:13 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:13 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 9
07:28:13 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0]
07:28:13 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3]
07:28:13 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 101. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 19. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:13 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:13 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:13 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0021453906700503547
07:28:13 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:13 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:13 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:13 DEBUG opendrift.models.oceandrift:548: 9 elements reached seafloor, interacting with bottom
07:28:13 DEBUG opendrift:719: Lifting 9 elements to seafloor.
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:13 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:13 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:13 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(355), np.int64(0), np.int64(24), np.int64(121), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:13 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:13 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:13 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:13 DEBUG opendrift:2034: ======================================================================
07:28:13 INFO opendrift:2035: 2025-07-07 13:57:52.765301 - step 14 of 96 - 500 active elements (0 deactivated)
07:28:13 DEBUG opendrift:2041: 0 elements scheduled.
07:28:13 DEBUG opendrift:2043: ======================================================================
07:28:13 DEBUG opendrift:2054: 57.57473118725124 <- latitude -> 57.65994822557066
07:28:13 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.731374842150869
07:28:13 DEBUG opendrift:2054: -22.229304205169647 <- z -> -0.32934685496671745
07:28:13 DEBUG opendrift:2055: ---------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 13:00:00 (before)
2025-07-07 14:00:00 (after)
07:28:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 13:00:00) in space (linearNDFast)
07:28:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 14:00:00) in space (linearNDFast)
07:28:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 13:00:00, weight 0.04) and
after (2025-07-07 14:00:00, weight 0.96) in time
07:28:13 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.268636209651035 degrees.
07:28:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.268636209651035 degrees.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:13 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:13 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:13 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0257422 (min) 0.0692736 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0665391 (min) 0.0873514 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0498293 (min) -0.0348613 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.32125 (min) -0.868715 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: y_wind: -1.3997 (min) -0.0184011 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.278 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5909 (min) 16.3342 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1963 (min) 31.8792 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00010243 (min) 3.41848e-05 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:13 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:13 DEBUG opendrift:685: No elements hit coastline.
07:28:13 DEBUG opendrift:719: Lifting 26 elements to seafloor.
07:28:13 DEBUG opendrift:1636: No elements to deactivate
07:28:13 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:13 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:13 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:13 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:13 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0]
07:28:13 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3]
07:28:13 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 107. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 20. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:13 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:13 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:13 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0050938693821924825
07:28:13 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:13 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:13 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:13 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:13 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:13 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:13 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:13 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(349), np.int64(0), np.int64(24), np.int64(127), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:13 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:13 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:13 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:13 DEBUG opendrift:2034: ======================================================================
07:28:13 INFO opendrift:2035: 2025-07-07 14:27:52.765301 - step 15 of 96 - 500 active elements (0 deactivated)
07:28:13 DEBUG opendrift:2041: 0 elements scheduled.
07:28:13 DEBUG opendrift:2043: ======================================================================
07:28:13 DEBUG opendrift:2054: 57.575454123638124 <- latitude -> 57.65979382671069
07:28:13 DEBUG opendrift:2054: 10.51172184560699 <- longitude -> 10.732635578814259
07:28:13 DEBUG opendrift:2054: -22.328767776489258 <- z -> -0.28312608363546143
07:28:13 DEBUG opendrift:2055: ---------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 14:00:00 (before)
2025-07-07 15:00:00 (after)
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:15 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x36x7) for time after (2025-07-07 15:00:00)
07:28:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 14:00:00) in space (linearNDFast)
07:28:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 15:00:00) in space (linearNDFast)
07:28:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 14:00:00, weight 0.54) and
after (2025-07-07 15:00:00, weight 0.46) in time
07:28:15 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.267375482885555 degrees.
07:28:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.267375482885555 degrees.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0150895 (min) 0.0780579 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0467172 (min) 0.104958 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0868701 (min) -0.071669 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.22026 (min) -1.45296 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -1.14625 (min) -0.33225 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3704 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5923 (min) 16.3785 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.1965 (min) 31.8789 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.26478e-05 (min) 2.05912e-05 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:15 DEBUG opendrift:685: No elements hit coastline.
07:28:15 DEBUG opendrift:719: Lifting 51 elements to seafloor.
07:28:15 DEBUG opendrift:1636: No elements to deactivate
07:28:15 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:15 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:15 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:15 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:15 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:15 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:15 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 114. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 20. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:15 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:15 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:15 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004126484263385597
07:28:15 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:15 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:15 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:15 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:15 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:15 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:15 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:15 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(342), np.int64(0), np.int64(23), np.int64(135), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:15 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:15 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:15 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:15 DEBUG opendrift:2034: ======================================================================
07:28:15 INFO opendrift:2035: 2025-07-07 14:57:52.765301 - step 16 of 96 - 500 active elements (0 deactivated)
07:28:15 DEBUG opendrift:2041: 0 elements scheduled.
07:28:15 DEBUG opendrift:2043: ======================================================================
07:28:15 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.660089522816655
07:28:15 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.733672922649632
07:28:15 DEBUG opendrift:2054: -22.292556762695312 <- z -> -0.18189965632162935
07:28:15 DEBUG opendrift:2055: ---------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 14:00:00 (before)
2025-07-07 15:00:00 (after)
07:28:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 14:00:00) in space (linearNDFast)
07:28:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 15:00:00) in space (linearNDFast)
07:28:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 14:00:00, weight 0.04) and
after (2025-07-07 15:00:00, weight 0.96) in time
07:28:15 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26633814098439 degrees.
07:28:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26633814098439 degrees.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:15 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00439631 (min) 0.0893741 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0287272 (min) 0.123602 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.127151 (min) -0.110202 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.5737 (min) -1.78939 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.908891 (min) -0.369464 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4399 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5938 (min) 16.4168 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2255 (min) 31.8784 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.15146e-05 (min) 7.06406e-06 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:15 DEBUG opendrift:685: No elements hit coastline.
07:28:15 DEBUG opendrift:719: Lifting 65 elements to seafloor.
07:28:15 DEBUG opendrift:1636: No elements to deactivate
07:28:15 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:15 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:15 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:15 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 15
07:28:15 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 2 0 0 0 0 0 0]
07:28:15 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 0 3 3 3 3 3 3]
07:28:15 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 128. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 21. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:15 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:15 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:15 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005019146804332312
07:28:15 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:15 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:15 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:15 DEBUG opendrift.models.oceandrift:548: 14 elements reached seafloor, interacting with bottom
07:28:15 DEBUG opendrift:719: Lifting 14 elements to seafloor.
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:15 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:15 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:15 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(329), np.int64(0), np.int64(22), np.int64(149), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:15 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:15 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:15 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:15 DEBUG opendrift:2034: ======================================================================
07:28:15 INFO opendrift:2035: 2025-07-07 15:27:52.765301 - step 17 of 96 - 500 active elements (0 deactivated)
07:28:15 DEBUG opendrift:2041: 0 elements scheduled.
07:28:15 DEBUG opendrift:2043: ======================================================================
07:28:15 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.660940194216494
07:28:15 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.733610730841342
07:28:15 DEBUG opendrift:2054: -22.254467010498047 <- z -> -0.23425274829619847
07:28:15 DEBUG opendrift:2055: ---------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 15:00:00 (before)
2025-07-07 16:00:00 (after)
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:17 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:17 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 38x36x7) for time after (2025-07-07 16:00:00)
07:28:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 15:00:00) in space (linearNDFast)
07:28:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 16:00:00) in space (linearNDFast)
07:28:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 15:00:00, weight 0.54) and
after (2025-07-07 16:00:00, weight 0.46) in time
07:28:17 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26640032352513 degrees.
07:28:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26640032352513 degrees.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00245937 (min) 0.0957239 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.00570321 (min) 0.135138 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.16952 (min) -0.152497 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.32511 (min) -1.7584 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.596983 (min) -0.306739 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4638 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5971 (min) 16.4378 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2279 (min) 31.8792 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.21956e-05 (min) 6.13439e-08 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:17 DEBUG opendrift:685: No elements hit coastline.
07:28:17 DEBUG opendrift:719: Lifting 91 elements to seafloor.
07:28:17 DEBUG opendrift:1636: No elements to deactivate
07:28:17 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:17 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:17 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:17 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 13
07:28:17 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 3 0 0 0 0]
07:28:17 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 0 3 3 3 3]
07:28:17 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 140. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 21. 0.]
[ 1. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:17 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:17 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:17 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004059921897118865
07:28:17 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:17 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:17 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:548: 12 elements reached seafloor, interacting with bottom
07:28:17 DEBUG opendrift:719: Lifting 12 elements to seafloor.
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:17 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:17 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:17 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:17 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(318), np.int64(0), np.int64(20), np.int64(162), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:17 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:17 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:17 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:17 DEBUG opendrift:2034: ======================================================================
07:28:17 INFO opendrift:2035: 2025-07-07 15:57:52.765301 - step 18 of 96 - 500 active elements (0 deactivated)
07:28:17 DEBUG opendrift:2041: 0 elements scheduled.
07:28:17 DEBUG opendrift:2043: ======================================================================
07:28:17 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.662351764228596
07:28:17 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.733655409750355
07:28:17 DEBUG opendrift:2054: -22.213123321533203 <- z -> -0.2544076397446664
07:28:17 DEBUG opendrift:2055: ---------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 15:00:00 (before)
2025-07-07 16:00:00 (after)
07:28:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 15:00:00) in space (linearNDFast)
07:28:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 16:00:00) in space (linearNDFast)
07:28:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 15:00:00, weight 0.04) and
after (2025-07-07 16:00:00, weight 0.96) in time
07:28:17 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.266355640441915 degrees.
07:28:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.266355640441915 degrees.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:17 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00913018 (min) 0.101995 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0174431 (min) 0.148195 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.21202 (min) -0.195003 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.10691 (min) -1.49559 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.420112 (min) -0.0598764 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4701 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6007 (min) 16.4494 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2311 (min) 31.8801 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.50226e-05 (min) 1.23087e-05 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:17 DEBUG opendrift:685: No elements hit coastline.
07:28:17 DEBUG opendrift:719: Lifting 114 elements to seafloor.
07:28:17 DEBUG opendrift:1636: No elements to deactivate
07:28:17 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:17 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:17 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:17 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 12
07:28:17 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0 0 0 0 0 0 0 0 0 0]
07:28:17 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3 3 3 3 3 3 3 3 3]
07:28:17 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 151. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 23. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:17 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:17 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:17 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0033391990435806883
07:28:17 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:17 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:17 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:548: 11 elements reached seafloor, interacting with bottom
07:28:17 DEBUG opendrift:719: Lifting 11 elements to seafloor.
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:17 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:17 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:17 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:17 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(308), np.int64(0), np.int64(19), np.int64(173), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:17 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:17 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:17 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:17 DEBUG opendrift:2034: ======================================================================
07:28:17 INFO opendrift:2035: 2025-07-07 16:27:52.765301 - step 19 of 96 - 500 active elements (0 deactivated)
07:28:17 DEBUG opendrift:2041: 0 elements scheduled.
07:28:17 DEBUG opendrift:2043: ======================================================================
07:28:17 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.6641838829907
07:28:17 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.73350159936494
07:28:17 DEBUG opendrift:2054: -22.321734339124703 <- z -> -0.3383848989481274
07:28:17 DEBUG opendrift:2055: ---------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 16:00:00 (before)
2025-07-07 17:00:00 (after)
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:20 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:20 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 39x36x7) for time after (2025-07-07 17:00:00)
07:28:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 16:00:00) in space (linearNDFast)
07:28:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 17:00:00) in space (linearNDFast)
07:28:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 16:00:00, weight 0.54) and
after (2025-07-07 17:00:00, weight 0.46) in time
07:28:20 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:20 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26650945600665 degrees.
07:28:20 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26650945600665 degrees.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:20 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.002153 (min) 0.103451 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.02902 (min) 0.152686 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.241171 (min) -0.224513 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.26662 (min) -1.66057 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.562765 (min) -0.153861 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4504 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6023 (min) 16.4426 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.232 (min) 31.8822 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.6117e-05 (min) 1.52018e-05 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:20 DEBUG opendrift:685: No elements hit coastline.
07:28:20 DEBUG opendrift:719: Lifting 132 elements to seafloor.
07:28:20 DEBUG opendrift:1636: No elements to deactivate
07:28:20 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:20 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:20 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:20 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:20 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 2]
07:28:20 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 0]
07:28:20 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 155. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 24. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:20 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:20 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:20 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:20 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:20 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0037662822588456005
07:28:20 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:20 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:20 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:20 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:20 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:20 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:20 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:20 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:20 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:20 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(305), np.int64(0), np.int64(17), np.int64(178), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:20 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:20 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:20 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:20 DEBUG opendrift:2034: ======================================================================
07:28:20 INFO opendrift:2035: 2025-07-07 16:57:52.765301 - step 20 of 96 - 500 active elements (0 deactivated)
07:28:20 DEBUG opendrift:2041: 0 elements scheduled.
07:28:20 DEBUG opendrift:2043: ======================================================================
07:28:20 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.665961644699486
07:28:20 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.734003213254526
07:28:20 DEBUG opendrift:2054: -22.49344825744629 <- z -> -0.341356562238306
07:28:20 DEBUG opendrift:2055: ---------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 16:00:00 (before)
2025-07-07 17:00:00 (after)
07:28:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 16:00:00) in space (linearNDFast)
07:28:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 17:00:00) in space (linearNDFast)
07:28:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 16:00:00, weight 0.04) and
after (2025-07-07 17:00:00, weight 0.96) in time
07:28:20 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:20 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26600785053887 degrees.
07:28:20 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26600785053887 degrees.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:20 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:20 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:20 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00156567 (min) 0.105838 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0425998 (min) 0.158877 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.269306 (min) -0.253057 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.53187 (min) -1.59819 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.820861 (min) -0.22681 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4382 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6038 (min) 16.4388 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2346 (min) 31.8844 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.61894e-05 (min) 2.53301e-05 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:20 DEBUG opendrift:685: No elements hit coastline.
07:28:20 DEBUG opendrift:719: Lifting 138 elements to seafloor.
07:28:20 DEBUG opendrift:1636: No elements to deactivate
07:28:20 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:20 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:20 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:20 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:20 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:20 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:20 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 162. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 25. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:20 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:20 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:20 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:20 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:20 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004673358334531014
07:28:20 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:20 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:20 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:20 DEBUG opendrift.models.oceandrift:548: 8 elements reached seafloor, interacting with bottom
07:28:20 DEBUG opendrift:719: Lifting 8 elements to seafloor.
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:20 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:20 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:20 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:20 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:20 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:20 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(298), np.int64(0), np.int64(16), np.int64(186), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:20 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:20 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:20 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:20 DEBUG opendrift:2034: ======================================================================
07:28:20 INFO opendrift:2035: 2025-07-07 17:27:52.765301 - step 21 of 96 - 500 active elements (0 deactivated)
07:28:20 DEBUG opendrift:2041: 0 elements scheduled.
07:28:20 DEBUG opendrift:2043: ======================================================================
07:28:20 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.66787637645123
07:28:20 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.734437644716479
07:28:20 DEBUG opendrift:2054: -22.737038944406176 <- z -> -0.2841343073647997
07:28:20 DEBUG opendrift:2055: ---------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:20 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 17:00:00 (before)
2025-07-07 18:00:00 (after)
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:22 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:22 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 39x36x7) for time after (2025-07-07 18:00:00)
07:28:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 17:00:00) in space (linearNDFast)
07:28:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 18:00:00) in space (linearNDFast)
07:28:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 17:00:00, weight 0.54) and
after (2025-07-07 18:00:00, weight 0.46) in time
07:28:22 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:22 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2655733979396 degrees.
07:28:22 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2655733979396 degrees.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:22 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00419658 (min) 0.105876 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0522945 (min) 0.163105 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.286967 (min) -0.270082 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.82223 (min) -1.38404 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: -1.35807 (min) -0.472585 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4121 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6039 (min) 16.4264 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2349 (min) 31.8933 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.41259e-05 (min) 3.87614e-05 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:22 DEBUG opendrift:685: No elements hit coastline.
07:28:22 DEBUG opendrift:719: Lifting 148 elements to seafloor.
07:28:22 DEBUG opendrift:1636: No elements to deactivate
07:28:22 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:22 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:22 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:22 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:22 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0]
07:28:22 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3]
07:28:22 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 167. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 26. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:22 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:22 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:22 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:22 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:22 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0061389867940219
07:28:22 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:22 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:22 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:22 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:22 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:22 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:22 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:22 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(293), np.int64(0), np.int64(14), np.int64(193), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:22 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:22 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:22 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:22 DEBUG opendrift:2034: ======================================================================
07:28:22 INFO opendrift:2035: 2025-07-07 17:57:52.765301 - step 22 of 96 - 500 active elements (0 deactivated)
07:28:22 DEBUG opendrift:2041: 0 elements scheduled.
07:28:22 DEBUG opendrift:2043: ======================================================================
07:28:22 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.66990718171578
07:28:22 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.735150770636846
07:28:22 DEBUG opendrift:2054: -22.800186157226562 <- z -> -0.18977063601666394
07:28:22 DEBUG opendrift:2055: ---------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 17:00:00 (before)
2025-07-07 18:00:00 (after)
07:28:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 17:00:00) in space (linearNDFast)
07:28:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 18:00:00) in space (linearNDFast)
07:28:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 17:00:00, weight 0.04) and
after (2025-07-07 18:00:00, weight 0.96) in time
07:28:22 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:22 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.264860288719376 degrees.
07:28:22 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.264860288719376 degrees.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:22 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:22 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:22 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00864344 (min) 0.105735 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0639188 (min) 0.167167 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.303832 (min) -0.286244 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.22264 (min) -1.14552 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: -1.97704 (min) -0.737536 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3852 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6039 (min) 16.4438 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2372 (min) 31.9042 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.35197e-05 (min) 4.69925e-05 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:22 DEBUG opendrift:685: No elements hit coastline.
07:28:22 DEBUG opendrift:719: Lifting 157 elements to seafloor.
07:28:22 DEBUG opendrift:1636: No elements to deactivate
07:28:22 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:22 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:22 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:22 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:22 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0]
07:28:22 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3]
07:28:22 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 172. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 28. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:22 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:22 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:22 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:22 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:22 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.009112685551172277
07:28:22 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:22 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:22 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:22 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:22 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:22 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:22 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:22 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:22 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:22 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(288), np.int64(0), np.int64(12), np.int64(200), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:22 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:22 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:22 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:22 DEBUG opendrift:2034: ======================================================================
07:28:22 INFO opendrift:2035: 2025-07-07 18:27:52.765301 - step 23 of 96 - 500 active elements (0 deactivated)
07:28:22 DEBUG opendrift:2041: 0 elements scheduled.
07:28:22 DEBUG opendrift:2043: ======================================================================
07:28:22 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.67185091163117
07:28:22 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.73594612467628
07:28:22 DEBUG opendrift:2054: -22.7841854095459 <- z -> -0.30546333727822494
07:28:22 DEBUG opendrift:2055: ---------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:22 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 18:00:00 (before)
2025-07-07 19:00:00 (after)
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:24 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:24 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 40x36x7) for time after (2025-07-07 19:00:00)
07:28:24 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 18:00:00) in space (linearNDFast)
07:28:24 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:24 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 19:00:00) in space (linearNDFast)
07:28:24 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:24 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 18:00:00, weight 0.54) and
after (2025-07-07 19:00:00, weight 0.46) in time
07:28:24 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:24 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26406493353198 degrees.
07:28:24 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26406493353198 degrees.
07:28:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:24 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:24 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:24 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:24 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:24 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:24 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:24 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:24 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:24 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:24 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:24 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:24 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:24 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00677354 (min) 0.105267 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0781704 (min) 0.171479 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.316213 (min) -0.297659 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.02559 (min) -1.55351 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.39598 (min) -1.41697 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3503 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6069 (min) 16.4359 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2393 (min) 31.9179 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.20142e-05 (min) 5.26862e-05 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:24 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:25 DEBUG opendrift:685: No elements hit coastline.
07:28:25 DEBUG opendrift:719: Lifting 166 elements to seafloor.
07:28:25 DEBUG opendrift:1636: No elements to deactivate
07:28:25 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:25 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:25 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:25 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 8
07:28:25 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0]
07:28:25 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3]
07:28:25 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 180. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 30. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:25 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:25 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:25 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.00924948549013861
07:28:25 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:25 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:25 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:548: 9 elements reached seafloor, interacting with bottom
07:28:25 DEBUG opendrift:719: Lifting 9 elements to seafloor.
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:25 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:25 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:25 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(280), np.int64(0), np.int64(10), np.int64(210), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:25 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:25 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:25 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:25 DEBUG opendrift:2034: ======================================================================
07:28:25 INFO opendrift:2035: 2025-07-07 18:57:52.765301 - step 24 of 96 - 500 active elements (0 deactivated)
07:28:25 DEBUG opendrift:2041: 0 elements scheduled.
07:28:25 DEBUG opendrift:2043: ======================================================================
07:28:25 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.673956354198005
07:28:25 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.736846779954476
07:28:25 DEBUG opendrift:2054: -23.134864807128906 <- z -> -0.28119141403656267
07:28:25 DEBUG opendrift:2055: ---------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 18:00:00 (before)
2025-07-07 19:00:00 (after)
07:28:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 18:00:00) in space (linearNDFast)
07:28:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 19:00:00) in space (linearNDFast)
07:28:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 18:00:00, weight 0.04) and
after (2025-07-07 19:00:00, weight 0.96) in time
07:28:25 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26316427705221 degrees.
07:28:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26316427705221 degrees.
07:28:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:25 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00488685 (min) 0.104773 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0858748 (min) 0.179773 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.328253 (min) -0.308221 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.01174 (min) -2.01809 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.80792 (min) -2.11257 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3061 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.61 (min) 16.4266 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2559 (min) 31.9327 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.40037e-05 (min) 5.75232e-05 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:25 DEBUG opendrift:685: No elements hit coastline.
07:28:25 DEBUG opendrift:719: Lifting 186 elements to seafloor.
07:28:25 DEBUG opendrift:1636: No elements to deactivate
07:28:25 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:25 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:25 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:25 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:25 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0]
07:28:25 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3]
07:28:25 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 186. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 32. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:25 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:25 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:25 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.011807794569782828
07:28:25 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:25 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:25 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:25 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:25 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:25 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:25 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:25 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(274), np.int64(0), np.int64(10), np.int64(216), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:25 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:25 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:25 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:25 DEBUG opendrift:2034: ======================================================================
07:28:25 INFO opendrift:2035: 2025-07-07 19:27:52.765301 - step 25 of 96 - 500 active elements (0 deactivated)
07:28:25 DEBUG opendrift:2041: 0 elements scheduled.
07:28:25 DEBUG opendrift:2043: ======================================================================
07:28:25 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.67628499460845
07:28:25 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.737864798476032
07:28:25 DEBUG opendrift:2054: -23.12386131286621 <- z -> -0.2177928609627176
07:28:25 DEBUG opendrift:2055: ---------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 19:00:00 (before)
2025-07-07 20:00:00 (after)
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:27 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:27 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 40x36x7) for time after (2025-07-07 20:00:00)
07:28:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 19:00:00) in space (linearNDFast)
07:28:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 20:00:00) in space (linearNDFast)
07:28:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 19:00:00, weight 0.54) and
after (2025-07-07 20:00:00, weight 0.46) in time
07:28:27 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:27 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26214625679244 degrees.
07:28:27 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.26214625679244 degrees.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:27 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.00184643 (min) 0.1021 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0906063 (min) 0.184931 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.336538 (min) -0.315432 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.84406 (min) -2.11299 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.03892 (min) -2.35581 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.2554 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6107 (min) 16.405 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2524 (min) 31.9486 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.18643e-05 (min) 6.04662e-05 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:27 DEBUG opendrift:685: No elements hit coastline.
07:28:27 DEBUG opendrift:719: Lifting 192 elements to seafloor.
07:28:27 DEBUG opendrift:1636: No elements to deactivate
07:28:27 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:27 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:27 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:27 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:27 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:27 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:27 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 193. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 32. 0.]
[ 2. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:27 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:27 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:27 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:27 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:27 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.011714367185887947
07:28:27 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:27 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:27 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:27 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:27 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:27 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:27 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:27 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:27 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:27 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:27 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:27 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(267), np.int64(0), np.int64(9), np.int64(224), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:27 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:27 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:27 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:27 DEBUG opendrift:2034: ======================================================================
07:28:27 INFO opendrift:2035: 2025-07-07 19:57:52.765301 - step 26 of 96 - 500 active elements (0 deactivated)
07:28:27 DEBUG opendrift:2041: 0 elements scheduled.
07:28:27 DEBUG opendrift:2043: ======================================================================
07:28:27 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.67925090040949
07:28:27 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.738977301595565
07:28:27 DEBUG opendrift:2054: -23.390483856201172 <- z -> -0.2559709783021936
07:28:27 DEBUG opendrift:2055: ---------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 19:00:00 (before)
2025-07-07 20:00:00 (after)
07:28:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 19:00:00) in space (linearNDFast)
07:28:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 20:00:00) in space (linearNDFast)
07:28:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 19:00:00, weight 0.04) and
after (2025-07-07 20:00:00, weight 0.96) in time
07:28:27 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:27 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.261033746060995 degrees.
07:28:27 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.261033746060995 degrees.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:27 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:27 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:27 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00128174 (min) 0.102616 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0911021 (min) 0.191455 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.344538 (min) -0.322338 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.7817 (min) -2.19334 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.29313 (min) -2.47353 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.2001 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6109 (min) 16.3897 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2281 (min) 31.959 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.01135e-05 (min) 6.33796e-05 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:27 DEBUG opendrift:685: No elements hit coastline.
07:28:27 DEBUG opendrift:719: Lifting 200 elements to seafloor.
07:28:27 DEBUG opendrift:1636: No elements to deactivate
07:28:27 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:27 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:27 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:27 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:27 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 3 0 3]
07:28:27 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 0 3 0]
07:28:27 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 198. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 33. 0.]
[ 4. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:27 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:27 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:27 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:27 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:27 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.012296241331744062
07:28:27 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:27 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:27 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:27 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:27 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:27 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:27 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:27 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:27 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:27 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:27 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(264), np.int64(0), np.int64(8), np.int64(228), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:27 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:27 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:27 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:27 DEBUG opendrift:2034: ======================================================================
07:28:27 INFO opendrift:2035: 2025-07-07 20:27:52.765301 - step 27 of 96 - 500 active elements (0 deactivated)
07:28:27 DEBUG opendrift:2041: 0 elements scheduled.
07:28:27 DEBUG opendrift:2043: ======================================================================
07:28:27 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.68228750537293
07:28:27 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.740194005671352
07:28:27 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.1344804376449148
07:28:27 DEBUG opendrift:2055: ---------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:27 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 20:00:00 (before)
2025-07-07 21:00:00 (after)
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:29 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:29 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 41x36x7) for time after (2025-07-07 21:00:00)
07:28:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 20:00:00) in space (linearNDFast)
07:28:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 21:00:00) in space (linearNDFast)
07:28:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 20:00:00, weight 0.54) and
after (2025-07-07 21:00:00, weight 0.46) in time
07:28:29 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:29 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.259817047010586 degrees.
07:28:29 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.259817047010586 degrees.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:29 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:29 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00442233 (min) 0.106055 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.086184 (min) 0.188472 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.340424 (min) -0.318253 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.39142 (min) -1.82969 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.60357 (min) -2.46958 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.1372 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6123 (min) 16.3823 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2456 (min) 31.9194 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.51917e-05 (min) 6.30664e-05 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:29 DEBUG opendrift:685: No elements hit coastline.
07:28:29 DEBUG opendrift:714: No elements hit seafloor.
07:28:29 DEBUG opendrift:1636: No elements to deactivate
07:28:29 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:29 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:29 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:29 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 14
07:28:29 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3 0 0 0 0 0 0 0 0 0 0 0]
07:28:29 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0 3 3 3 3 3 3 3 3 3 3 3]
07:28:29 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 211. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 34. 0.]
[ 5. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:29 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:29 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:29 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:29 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:29 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.012796623688467942
07:28:29 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:29 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:29 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:29 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:548: 13 elements reached seafloor, interacting with bottom
07:28:29 DEBUG opendrift:719: Lifting 13 elements to seafloor.
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:29 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:29 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:29 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:29 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(252), np.int64(0), np.int64(8), np.int64(240), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:29 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:29 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:29 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:29 DEBUG opendrift:2034: ======================================================================
07:28:29 INFO opendrift:2035: 2025-07-07 20:57:52.765301 - step 28 of 96 - 500 active elements (0 deactivated)
07:28:29 DEBUG opendrift:2041: 0 elements scheduled.
07:28:29 DEBUG opendrift:2043: ======================================================================
07:28:29 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.68516604824171
07:28:29 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.741548740495153
07:28:29 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.18157654589135086
07:28:29 DEBUG opendrift:2055: ---------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 20:00:00 (before)
2025-07-07 21:00:00 (after)
07:28:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 20:00:00) in space (linearNDFast)
07:28:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 21:00:00) in space (linearNDFast)
07:28:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 20:00:00, weight 0.04) and
after (2025-07-07 21:00:00, weight 0.96) in time
07:28:29 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:29 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.258462306632204 degrees.
07:28:29 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.258462306632204 degrees.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:29 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:29 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:29 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:29 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:29 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:29 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.012592 (min) 0.111379 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0808756 (min) 0.185568 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.337424 (min) -0.313292 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.07469 (min) -1.33855 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.92983 (min) -2.45638 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.078 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6128 (min) 16.3687 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2423 (min) 31.9234 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.03757e-05 (min) 6.21871e-05 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:29 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:29 DEBUG opendrift:685: No elements hit coastline.
07:28:29 DEBUG opendrift:714: No elements hit seafloor.
07:28:29 DEBUG opendrift:1636: No elements to deactivate
07:28:29 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:29 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:29 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:29 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:29 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0]
07:28:29 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3]
07:28:29 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 215. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 34. 0.]
[ 5. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:29 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:29 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:29 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:29 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:29 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.013676806502863858
07:28:29 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:29 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:29 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:29 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:29 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:29 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:29 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:29 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:29 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:29 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:29 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(248), np.int64(0), np.int64(7), np.int64(245), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:29 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:29 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:29 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:29 DEBUG opendrift:2034: ======================================================================
07:28:29 INFO opendrift:2035: 2025-07-07 21:27:52.765301 - step 29 of 96 - 500 active elements (0 deactivated)
07:28:29 DEBUG opendrift:2041: 0 elements scheduled.
07:28:29 DEBUG opendrift:2043: ======================================================================
07:28:29 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.688001327405175
07:28:29 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.743032884099259
07:28:29 DEBUG opendrift:2054: -23.38407325744629 <- z -> 0.0
07:28:29 DEBUG opendrift:2055: ---------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:29 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 21:00:00 (before)
2025-07-07 22:00:00 (after)
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:31 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:31 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 42x36x7) for time after (2025-07-07 22:00:00)
07:28:31 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 21:00:00) in space (linearNDFast)
07:28:31 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:31 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 22:00:00) in space (linearNDFast)
07:28:31 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:31 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 21:00:00, weight 0.54) and
after (2025-07-07 22:00:00, weight 0.46) in time
07:28:31 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:31 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25697816689888 degrees.
07:28:31 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25697816689888 degrees.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:31 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:31 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:31 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0223433 (min) 0.107254 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0690382 (min) 0.171506 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.310726 (min) -0.286132 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.93018 (min) -1.22561 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.40255 (min) -2.6606 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.0364 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.612 (min) 16.3524 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2393 (min) 31.9347 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.02528e-05 (min) 5.39898e-05 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:31 DEBUG opendrift:685: No elements hit coastline.
07:28:31 DEBUG opendrift:714: No elements hit seafloor.
07:28:31 DEBUG opendrift:1636: No elements to deactivate
07:28:31 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:31 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:31 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:31 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:31 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 2 0 0]
07:28:31 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 0 3 3]
07:28:31 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 220. 0.]
[ 0. 0. 0. 0. 0.]
[ 10. 0. 0. 35. 0.]
[ 5. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:31 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:31 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:31 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:31 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:31 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.016702286923944754
07:28:31 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:31 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:31 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:31 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:31 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:31 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:31 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:31 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(244), np.int64(0), np.int64(6), np.int64(250), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:31 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:31 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:31 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:31 DEBUG opendrift:2034: ======================================================================
07:28:31 INFO opendrift:2035: 2025-07-07 21:57:52.765301 - step 30 of 96 - 500 active elements (0 deactivated)
07:28:31 DEBUG opendrift:2041: 0 elements scheduled.
07:28:31 DEBUG opendrift:2043: ======================================================================
07:28:31 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.69023165596159
07:28:31 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.744657077997585
07:28:31 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.007979730566539445
07:28:31 DEBUG opendrift:2055: ---------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 21:00:00 (before)
2025-07-07 22:00:00 (after)
07:28:31 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 21:00:00) in space (linearNDFast)
07:28:31 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:31 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 22:00:00) in space (linearNDFast)
07:28:31 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:31 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 21:00:00, weight 0.04) and
after (2025-07-07 22:00:00, weight 0.96) in time
07:28:31 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:31 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25535396553452 degrees.
07:28:31 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25535396553452 degrees.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:31 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:31 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:31 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:31 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:31 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:31 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:31 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:31 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0321582 (min) 0.107753 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0567041 (min) 0.158661 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.282226 (min) -0.255744 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.01758 (min) -1.0392 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.87047 (min) -2.80671 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.0185 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6103 (min) 16.3321 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2363 (min) 31.9992 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -4.97264e-05 (min) 6.80647e-05 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:31 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:31 DEBUG opendrift:685: No elements hit coastline.
07:28:31 DEBUG opendrift:714: No elements hit seafloor.
07:28:31 DEBUG opendrift:1636: No elements to deactivate
07:28:31 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:31 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:31 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:31 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:31 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:31 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:31 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 227. 0.]
[ 0. 0. 0. 0. 0.]
[ 10. 0. 0. 35. 0.]
[ 5. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:31 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:31 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:31 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:31 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:31 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.020087067317631352
07:28:31 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:31 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:31 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:31 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:31 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:31 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:31 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:31 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:31 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:31 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:31 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:31 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:31 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(237), np.int64(0), np.int64(5), np.int64(258), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:31 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:31 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:31 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:31 DEBUG opendrift:2034: ======================================================================
07:28:31 INFO opendrift:2035: 2025-07-07 22:27:52.765301 - step 31 of 96 - 500 active elements (0 deactivated)
07:28:31 DEBUG opendrift:2041: 0 elements scheduled.
07:28:31 DEBUG opendrift:2043: ======================================================================
07:28:31 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.69201432223719
07:28:31 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.746201803042817
07:28:31 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.24629374690190603
07:28:31 DEBUG opendrift:2055: ---------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:31 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:31 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:31 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:31 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 22:00:00 (before)
2025-07-07 23:00:00 (after)
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:33 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:33 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x35x7) for time after (2025-07-07 23:00:00)
07:28:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 22:00:00) in space (linearNDFast)
07:28:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 23:00:00) in space (linearNDFast)
07:28:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 22:00:00, weight 0.54) and
after (2025-07-07 23:00:00, weight 0.46) in time
07:28:33 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:33 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.253809251412356 degrees.
07:28:33 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.253809251412356 degrees.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:33 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:33 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0370499 (min) 0.0948293 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0233755 (min) 0.135435 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.231647 (min) -0.205388 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.20459 (min) -0.913017 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.82587 (min) -2.80232 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.0013 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6128 (min) 16.2998 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2351 (min) 31.9514 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -4.28497e-05 (min) 7.54572e-05 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:33 DEBUG opendrift:685: No elements hit coastline.
07:28:33 DEBUG opendrift:714: No elements hit seafloor.
07:28:33 DEBUG opendrift:1636: No elements to deactivate
07:28:33 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:33 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:33 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:33 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:33 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0 0 0 3 0]
07:28:33 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3 3 3 0 3]
07:28:33 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 231. 0.]
[ 0. 0. 0. 0. 0.]
[ 10. 0. 0. 36. 0.]
[ 7. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:33 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:33 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:33 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:33 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:33 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.020345166721170642
07:28:33 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:33 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:33 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:33 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:33 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:33 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:33 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:33 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:33 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:33 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(235), np.int64(0), np.int64(5), np.int64(260), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:33 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:33 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:33 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:33 DEBUG opendrift:2034: ======================================================================
07:28:33 INFO opendrift:2035: 2025-07-07 22:57:52.765301 - step 32 of 96 - 500 active elements (0 deactivated)
07:28:33 DEBUG opendrift:2041: 0 elements scheduled.
07:28:33 DEBUG opendrift:2043: ======================================================================
07:28:33 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.69321030968304
07:28:33 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.747669870261962
07:28:33 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.02008196587614841
07:28:33 DEBUG opendrift:2055: ---------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 22:00:00 (before)
2025-07-07 23:00:00 (after)
07:28:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 22:00:00) in space (linearNDFast)
07:28:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-07 23:00:00) in space (linearNDFast)
07:28:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 22:00:00, weight 0.04) and
after (2025-07-07 23:00:00, weight 0.96) in time
07:28:33 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:33 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25234117848414 degrees.
07:28:33 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25234117848414 degrees.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:33 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:33 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:33 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:33 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:33 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:33 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.041567 (min) 0.0867569 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.00846413 (min) 0.111522 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.179388 (min) -0.153983 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.3884 (min) -0.760613 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.72007 (min) -2.7875 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.026 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6128 (min) 16.2897 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2341 (min) 31.9565 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -4.25838e-05 (min) 8.23271e-05 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:33 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:33 DEBUG opendrift:685: No elements hit coastline.
07:28:33 DEBUG opendrift:714: No elements hit seafloor.
07:28:33 DEBUG opendrift:1636: No elements to deactivate
07:28:33 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:33 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:33 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:33 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 10
07:28:33 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 2 0 3 3 0 0]
07:28:33 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 0 3 0 0 3 3]
07:28:33 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 238. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 9. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:33 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:33 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:33 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:33 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:33 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.020225378292479786
07:28:33 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:33 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:33 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:33 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:33 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:33 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:33 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:33 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:33 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:33 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:33 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(231), np.int64(0), np.int64(4), np.int64(265), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:33 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:33 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:33 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:33 DEBUG opendrift:2034: ======================================================================
07:28:33 INFO opendrift:2035: 2025-07-07 23:27:52.765301 - step 33 of 96 - 500 active elements (0 deactivated)
07:28:33 DEBUG opendrift:2041: 0 elements scheduled.
07:28:33 DEBUG opendrift:2043: ======================================================================
07:28:33 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.693752965215516
07:28:33 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.749321872736312
07:28:33 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.10849433920425877
07:28:33 DEBUG opendrift:2055: ---------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:33 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 23:00:00 (before)
2025-07-08 00:00:00 (after)
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:35 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:35 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x36x7) for time after (2025-07-08 00:00:00)
07:28:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 23:00:00) in space (linearNDFast)
07:28:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 00:00:00) in space (linearNDFast)
07:28:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 23:00:00, weight 0.54) and
after (2025-07-08 00:00:00, weight 0.46) in time
07:28:35 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:35 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25068916907304 degrees.
07:28:35 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.25068916907304 degrees.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:35 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:35 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0398716 (min) 0.0779896 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0341667 (min) 0.0908841 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.120077 (min) -0.103202 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.27264 (min) -0.285742 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.32263 (min) -2.65854 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.0732 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6135 (min) 16.2998 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2356 (min) 31.954 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.08235e-05 (min) 7.66159e-05 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:35 DEBUG opendrift:685: No elements hit coastline.
07:28:35 DEBUG opendrift:714: No elements hit seafloor.
07:28:35 DEBUG opendrift:1636: No elements to deactivate
07:28:35 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:35 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:35 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:35 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:35 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:35 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:35 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 245. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 9. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:35 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:35 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:35 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.017238402807959952
07:28:35 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:35 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:35 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:35 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:35 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:35 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(224), np.int64(0), np.int64(4), np.int64(272), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:35 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:35 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:35 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:35 DEBUG opendrift:2034: ======================================================================
07:28:35 INFO opendrift:2035: 2025-07-07 23:57:52.765301 - step 34 of 96 - 500 active elements (0 deactivated)
07:28:35 DEBUG opendrift:2041: 0 elements scheduled.
07:28:35 DEBUG opendrift:2043: ======================================================================
07:28:35 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.69358093123131
07:28:35 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.750917350812506
07:28:35 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.42804484404276943
07:28:35 DEBUG opendrift:2055: ---------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-07 23:00:00 (before)
2025-07-08 00:00:00 (after)
07:28:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-07 23:00:00) in space (linearNDFast)
07:28:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 00:00:00) in space (linearNDFast)
07:28:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-07 23:00:00, weight 0.04) and
after (2025-07-08 00:00:00, weight 0.96) in time
07:28:35 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:35 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24909370306242 degrees.
07:28:35 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24909370306242 degrees.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:35 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:35 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:35 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:35 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:35 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:35 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0398944 (min) 0.0711625 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0873958 (min) 0.0703495 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0610279 (min) -0.0525767 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.13495 (min) 0.213656 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.11102 (min) -2.52091 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.1265 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6139 (min) 16.2892 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2373 (min) 31.9509 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.68621e-05 (min) 6.99477e-05 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:35 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:35 DEBUG opendrift:685: No elements hit coastline.
07:28:35 DEBUG opendrift:714: No elements hit seafloor.
07:28:35 DEBUG opendrift:1636: No elements to deactivate
07:28:35 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:35 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:35 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:35 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:35 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:35 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:35 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 252. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 9. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:35 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:35 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:35 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.014275209368909286
07:28:35 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:35 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:35 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:35 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:35 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:35 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:35 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(217), np.int64(0), np.int64(4), np.int64(279), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:35 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:35 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:35 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:35 DEBUG opendrift:2034: ======================================================================
07:28:35 INFO opendrift:2035: 2025-07-08 00:27:52.765301 - step 35 of 96 - 500 active elements (0 deactivated)
07:28:35 DEBUG opendrift:2041: 0 elements scheduled.
07:28:35 DEBUG opendrift:2043: ======================================================================
07:28:35 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.6923153399495
07:28:35 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.751551804806406
07:28:35 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.2802913855421454
07:28:35 DEBUG opendrift:2055: ---------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 00:00:00 (before)
2025-07-08 01:00:00 (after)
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:37 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:37 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x36x7) for time after (2025-07-08 01:00:00)
07:28:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 00:00:00) in space (linearNDFast)
07:28:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 01:00:00) in space (linearNDFast)
07:28:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 00:00:00, weight 0.54) and
after (2025-07-08 01:00:00, weight 0.46) in time
07:28:37 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24845924218665 degrees.
07:28:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24845924218665 degrees.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0366489 (min) 0.0633571 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0751274 (min) 0.0638617 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0228622 (min) -0.0173352 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.94827 (min) -0.546862 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.17742 (min) -3.2862 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.1976 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.614 (min) 16.2597 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2407 (min) 31.943 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -5.94989e-05 (min) 6.36952e-05 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:37 DEBUG opendrift:685: No elements hit coastline.
07:28:37 DEBUG opendrift:714: No elements hit seafloor.
07:28:37 DEBUG opendrift:1636: No elements to deactivate
07:28:37 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:37 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:37 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:37 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:37 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0]
07:28:37 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3]
07:28:37 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 257. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 9. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:37 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:37 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:37 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.018895507845635828
07:28:37 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:37 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:37 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:37 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:37 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:37 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(212), np.int64(0), np.int64(4), np.int64(284), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:37 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:37 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:37 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:37 DEBUG opendrift:2034: ======================================================================
07:28:37 INFO opendrift:2035: 2025-07-08 00:57:52.765301 - step 36 of 96 - 500 active elements (0 deactivated)
07:28:37 DEBUG opendrift:2041: 0 elements scheduled.
07:28:37 DEBUG opendrift:2043: ======================================================================
07:28:37 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.69116015120562
07:28:37 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.752852278250433
07:28:37 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.19285877720277317
07:28:37 DEBUG opendrift:2055: ---------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 00:00:00 (before)
2025-07-08 01:00:00 (after)
07:28:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 00:00:00) in space (linearNDFast)
07:28:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 01:00:00) in space (linearNDFast)
07:28:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 00:00:00, weight 0.04) and
after (2025-07-08 01:00:00, weight 0.96) in time
07:28:37 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24715877155951 degrees.
07:28:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24715877155951 degrees.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:37 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0342445 (min) 0.0611296 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0833772 (min) 0.0606116 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0.00632957 (min) 0.0242706 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.10618 (min) -1.24834 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.76431 (min) -3.57666 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.279 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6141 (min) 16.2497 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2442 (min) 32.034 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.35622e-05 (min) 6.17528e-05 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:37 DEBUG opendrift:685: No elements hit coastline.
07:28:37 DEBUG opendrift:714: No elements hit seafloor.
07:28:37 DEBUG opendrift:1636: No elements to deactivate
07:28:37 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:37 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:37 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:37 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 20
07:28:37 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 3 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
07:28:37 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 0 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
07:28:37 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 276. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 10. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:37 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:37 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:37 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.025411481154370023
07:28:37 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:37 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:37 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:37 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:37 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:37 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:37 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(194), np.int64(0), np.int64(4), np.int64(302), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:37 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:37 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:37 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:37 DEBUG opendrift:2034: ======================================================================
07:28:37 INFO opendrift:2035: 2025-07-08 01:27:52.765301 - step 37 of 96 - 500 active elements (0 deactivated)
07:28:37 DEBUG opendrift:2041: 0 elements scheduled.
07:28:37 DEBUG opendrift:2043: ======================================================================
07:28:37 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.69017857300896
07:28:37 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.754262426006736
07:28:37 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.04749477001652569
07:28:37 DEBUG opendrift:2055: ---------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 01:00:00 (before)
2025-07-08 02:00:00 (after)
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:39 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:39 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x36x7) for time after (2025-07-08 02:00:00)
07:28:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 01:00:00) in space (linearNDFast)
07:28:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 02:00:00) in space (linearNDFast)
07:28:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 01:00:00, weight 0.54) and
after (2025-07-08 02:00:00, weight 0.46) in time
07:28:39 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24574861939535 degrees.
07:28:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24574861939535 degrees.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0530507 (min) 0.0610569 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0510884 (min) 0.0725602 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.00178925 (min) 0.019072 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.13814 (min) -2.26622 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.73929 (min) -4.07297 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.349 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6161 (min) 16.2397 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2483 (min) 32.0359 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.95204e-05 (min) 5.24598e-05 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:39 DEBUG opendrift:685: No elements hit coastline.
07:28:39 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:39 DEBUG opendrift:1636: No elements to deactivate
07:28:39 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:39 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:39 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:39 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 12
07:28:39 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 3 0 0 0 0]
07:28:39 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 0 3 3 3 3]
07:28:39 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 287. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:39 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:39 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:39 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.02732732637365201
07:28:39 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:39 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:39 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:39 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 13 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 13 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 12 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:39 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:39 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:39 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(184), np.int64(0), np.int64(4), np.int64(312), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:39 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:39 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:39 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:39 DEBUG opendrift:2034: ======================================================================
07:28:39 INFO opendrift:2035: 2025-07-08 01:57:52.765301 - step 38 of 96 - 500 active elements (0 deactivated)
07:28:39 DEBUG opendrift:2041: 0 elements scheduled.
07:28:39 DEBUG opendrift:2043: ======================================================================
07:28:39 DEBUG opendrift:2054: 57.57618781848219 <- latitude -> 57.69020205881602
07:28:39 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.755640903650567
07:28:39 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.14534572332823636
07:28:39 DEBUG opendrift:2055: ---------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 01:00:00 (before)
2025-07-08 02:00:00 (after)
07:28:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 01:00:00) in space (linearNDFast)
07:28:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 02:00:00) in space (linearNDFast)
07:28:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 01:00:00, weight 0.04) and
after (2025-07-08 02:00:00, weight 0.96) in time
07:28:39 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2443701478794 degrees.
07:28:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2443701478794 degrees.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:39 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0494116 (min) 0.0627572 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0406082 (min) 0.0864163 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0129008 (min) 0.0104962 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.21339 (min) -3.27926 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.81732 (min) -4.38023 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4101 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6161 (min) 16.2297 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2525 (min) 32.0367 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.87419e-05 (min) 4.2454e-05 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:39 DEBUG opendrift:685: No elements hit coastline.
07:28:39 DEBUG opendrift:719: Lifting 25 elements to seafloor.
07:28:39 DEBUG opendrift:1636: No elements to deactivate
07:28:39 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:39 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:39 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:39 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:39 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:39 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:39 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 294. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:39 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:39 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:39 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.02901650738447468
07:28:39 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:39 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:39 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:39 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 13 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:39 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:39 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:39 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:39 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(177), np.int64(0), np.int64(4), np.int64(319), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:39 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:39 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:39 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:39 DEBUG opendrift:2034: ======================================================================
07:28:39 INFO opendrift:2035: 2025-07-08 02:27:52.765301 - step 39 of 96 - 500 active elements (0 deactivated)
07:28:39 DEBUG opendrift:2041: 0 elements scheduled.
07:28:39 DEBUG opendrift:2043: ======================================================================
07:28:39 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.69034697702153
07:28:39 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.756991097744406
07:28:39 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.2161052472596281
07:28:39 DEBUG opendrift:2055: ---------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 02:00:00 (before)
2025-07-08 03:00:00 (after)
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:41 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:41 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x37x7) for time after (2025-07-08 03:00:00)
07:28:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 02:00:00) in space (linearNDFast)
07:28:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:41 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 03:00:00) in space (linearNDFast)
07:28:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:41 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 02:00:00, weight 0.54) and
after (2025-07-08 03:00:00, weight 0.46) in time
07:28:41 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2430199569675 degrees.
07:28:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2430199569675 degrees.
07:28:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:41 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:41 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:41 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:41 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0672067 (min) 0.0672026 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0314095 (min) 0.105162 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0444774 (min) -0.0201984 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.08382 (min) -3.46516 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.19019 (min) -4.64963 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4599 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6161 (min) 16.2271 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2562 (min) 31.9472 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.00674e-05 (min) 2.29133e-05 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:41 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:41 DEBUG opendrift:685: No elements hit coastline.
07:28:41 DEBUG opendrift:719: Lifting 42 elements to seafloor.
07:28:41 DEBUG opendrift:1636: No elements to deactivate
07:28:41 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:41 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:41 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:41 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:41 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:41 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:41 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 301. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:41 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:41 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:41 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03055561217026877
07:28:41 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:41 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:41 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:41 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:41 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 12 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:41 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:41 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:41 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:41 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(170), np.int64(0), np.int64(4), np.int64(326), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:41 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:41 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:41 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:41 DEBUG opendrift:2034: ======================================================================
07:28:41 INFO opendrift:2035: 2025-07-08 02:57:52.765301 - step 40 of 96 - 500 active elements (0 deactivated)
07:28:41 DEBUG opendrift:2041: 0 elements scheduled.
07:28:41 DEBUG opendrift:2043: ======================================================================
07:28:41 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.691430124138094
07:28:41 DEBUG opendrift:2054: 10.511721845606996 <- longitude -> 10.757786435030557
07:28:41 DEBUG opendrift:2054: -23.437851384937634 <- z -> -0.22287597016465122
07:28:41 DEBUG opendrift:2055: ---------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 02:00:00 (before)
2025-07-08 03:00:00 (after)
07:28:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 02:00:00) in space (linearNDFast)
07:28:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:41 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 03:00:00) in space (linearNDFast)
07:28:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:42 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 02:00:00, weight 0.04) and
after (2025-07-08 03:00:00, weight 0.96) in time
07:28:42 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:42 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.242224612723476 degrees.
07:28:42 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.242224612723476 degrees.
07:28:42 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:42 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:42 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:42 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:42 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:42 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:42 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:42 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:42 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:42 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:42 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:42 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:42 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:42 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:42 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:42 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:42 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:42 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:42 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:42 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:42 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.065182 (min) 0.0804575 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.00604523 (min) 0.123965 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0784494 (min) -0.0527741 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.0086 (min) -3.59708 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.54587 (min) -4.73939 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.498 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6161 (min) 16.2168 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2599 (min) 32.0443 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.07923e-05 (min) 4.7587e-06 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:42 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:42 DEBUG opendrift:685: No elements hit coastline.
07:28:42 DEBUG opendrift:719: Lifting 57 elements to seafloor.
07:28:42 DEBUG opendrift:1636: No elements to deactivate
07:28:42 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:42 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:42 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:42 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 14
07:28:42 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0]
07:28:42 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3]
07:28:42 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 315. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:42 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:42 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:42 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:42 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:42 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03250641951184152
07:28:42 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:42 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:42 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:548: 14 elements reached seafloor, interacting with bottom
07:28:42 DEBUG opendrift:719: Lifting 14 elements to seafloor.
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 12 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:42 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:42 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:42 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:42 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:42 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:42 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(156), np.int64(0), np.int64(4), np.int64(340), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:42 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:42 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:42 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:42 DEBUG opendrift:2034: ======================================================================
07:28:42 INFO opendrift:2035: 2025-07-08 03:27:52.765301 - step 41 of 96 - 500 active elements (0 deactivated)
07:28:42 DEBUG opendrift:2041: 0 elements scheduled.
07:28:42 DEBUG opendrift:2043: ======================================================================
07:28:42 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.693091952884124
07:28:42 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.759137654592967
07:28:42 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.2307603757099097
07:28:42 DEBUG opendrift:2055: ---------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:42 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:42 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:42 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:42 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 03:00:00 (before)
2025-07-08 04:00:00 (after)
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:44 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:44 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 43x37x7) for time after (2025-07-08 04:00:00)
07:28:44 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 03:00:00) in space (linearNDFast)
07:28:44 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:44 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 04:00:00) in space (linearNDFast)
07:28:44 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:44 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 03:00:00, weight 0.54) and
after (2025-07-08 04:00:00, weight 0.46) in time
07:28:44 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:44 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.240873392372684 degrees.
07:28:44 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.240873392372684 degrees.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:44 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:44 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:44 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0816197 (min) 0.0939583 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0163283 (min) 0.140777 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.126708 (min) -0.102037 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.60405 (min) -3.30879 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.92331 (min) -5.32286 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.5158 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6179 (min) 16.205 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2631 (min) 32.0391 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.69454e-05 (min) 9.26987e-06 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:44 DEBUG opendrift:685: No elements hit coastline.
07:28:44 DEBUG opendrift:719: Lifting 76 elements to seafloor.
07:28:44 DEBUG opendrift:1636: No elements to deactivate
07:28:44 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:44 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:44 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:44 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:44 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0]
07:28:44 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3]
07:28:44 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 319. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:44 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:44 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:44 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:44 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:44 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.034588259789779176
07:28:44 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:44 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:44 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:44 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:44 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:44 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:44 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:44 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(152), np.int64(0), np.int64(4), np.int64(344), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:44 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:44 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:44 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:44 DEBUG opendrift:2034: ======================================================================
07:28:44 INFO opendrift:2035: 2025-07-08 03:57:52.765301 - step 42 of 96 - 500 active elements (0 deactivated)
07:28:44 DEBUG opendrift:2041: 0 elements scheduled.
07:28:44 DEBUG opendrift:2043: ======================================================================
07:28:44 DEBUG opendrift:2054: 57.57618781848219 <- latitude -> 57.69526422509805
07:28:44 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.760530393574221
07:28:44 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.3991029008163265
07:28:44 DEBUG opendrift:2055: ---------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 03:00:00 (before)
2025-07-08 04:00:00 (after)
07:28:44 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 03:00:00) in space (linearNDFast)
07:28:44 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:44 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 04:00:00) in space (linearNDFast)
07:28:44 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:44 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 03:00:00, weight 0.04) and
after (2025-07-08 04:00:00, weight 0.96) in time
07:28:44 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:44 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2394806650336 degrees.
07:28:44 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2394806650336 degrees.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:44 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:44 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:44 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:44 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:44 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:44 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:44 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:44 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.088466 (min) 0.107953 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0190911 (min) 0.197528 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.176525 (min) -0.15257 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.23095 (min) -2.82891 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.89974 (min) -5.44767 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.5218 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6199 (min) 16.1862 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2663 (min) 31.9489 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.95611e-05 (min) -5.92744e-06 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:44 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:44 DEBUG opendrift:685: No elements hit coastline.
07:28:44 DEBUG opendrift:719: Lifting 87 elements to seafloor.
07:28:44 DEBUG opendrift:1636: No elements to deactivate
07:28:44 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:44 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:44 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:44 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 9
07:28:44 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0 0 0]
07:28:44 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3 3 3]
07:28:44 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 328. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:44 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:44 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:44 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:44 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:44 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.041667473488795355
07:28:44 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:44 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:44 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:548: 9 elements reached seafloor, interacting with bottom
07:28:44 DEBUG opendrift:719: Lifting 9 elements to seafloor.
07:28:44 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:44 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:44 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:44 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:44 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:44 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:44 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(143), np.int64(0), np.int64(4), np.int64(353), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:44 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:44 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:44 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:44 DEBUG opendrift:2034: ======================================================================
07:28:44 INFO opendrift:2035: 2025-07-08 04:27:52.765301 - step 43 of 96 - 500 active elements (0 deactivated)
07:28:44 DEBUG opendrift:2041: 0 elements scheduled.
07:28:44 DEBUG opendrift:2043: ======================================================================
07:28:44 DEBUG opendrift:2054: 57.57618781848219 <- latitude -> 57.69793134145213
07:28:44 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.75786544260848
07:28:44 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.23429709213578964
07:28:44 DEBUG opendrift:2055: ---------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:44 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:44 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:44 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:44 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 04:00:00 (before)
2025-07-08 05:00:00 (after)
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:46 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:46 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 44x37x7) for time after (2025-07-08 05:00:00)
07:28:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 04:00:00) in space (linearNDFast)
07:28:46 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:46 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 05:00:00) in space (linearNDFast)
07:28:46 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:46 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 04:00:00, weight 0.54) and
after (2025-07-08 05:00:00, weight 0.46) in time
07:28:46 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:46 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24214560206903 degrees.
07:28:46 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24214560206903 degrees.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:46 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:46 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:46 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.103382 (min) 0.116436 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0325423 (min) 0.21045 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.22328 (min) -0.19982 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.12944 (min) -2.84066 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.09189 (min) -6.17828 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4494 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6204 (min) 16.1731 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2687 (min) 31.9491 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000108054 (min) 1.3649e-05 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:46 DEBUG opendrift:685: No elements hit coastline.
07:28:46 DEBUG opendrift:719: Lifting 99 elements to seafloor.
07:28:46 DEBUG opendrift:1636: No elements to deactivate
07:28:46 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:46 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:46 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:46 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:46 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0]
07:28:46 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3]
07:28:46 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 334. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:46 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:46 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:46 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:46 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:46 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0434249776816469
07:28:46 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:46 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:46 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:46 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:46 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:46 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 12 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:46 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:46 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:46 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:46 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(137), np.int64(0), np.int64(4), np.int64(359), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:46 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:46 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:46 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:46 DEBUG opendrift:2034: ======================================================================
07:28:46 INFO opendrift:2035: 2025-07-08 04:57:52.765301 - step 44 of 96 - 500 active elements (0 deactivated)
07:28:46 DEBUG opendrift:2041: 0 elements scheduled.
07:28:46 DEBUG opendrift:2043: ======================================================================
07:28:46 DEBUG opendrift:2054: 57.57618781848219 <- latitude -> 57.70133263464303
07:28:46 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.754751111870851
07:28:46 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.1504379528502664
07:28:46 DEBUG opendrift:2055: ---------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 04:00:00 (before)
2025-07-08 05:00:00 (after)
07:28:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 04:00:00) in space (linearNDFast)
07:28:46 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:46 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 05:00:00) in space (linearNDFast)
07:28:46 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:46 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 04:00:00, weight 0.04) and
after (2025-07-08 05:00:00, weight 0.96) in time
07:28:46 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:46 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24525993830237 degrees.
07:28:46 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24525993830237 degrees.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:46 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:46 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:46 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:46 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:46 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:46 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:46 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:46 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0620106 (min) 0.129889 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0381172 (min) 0.246886 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.269685 (min) -0.24682 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.13707 (min) -2.72748 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.25438 (min) -6.6109 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3472 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6207 (min) 16.1599 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2711 (min) 31.9602 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000116233 (min) 1.36169e-05 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:46 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:46 DEBUG opendrift:685: No elements hit coastline.
07:28:46 DEBUG opendrift:719: Lifting 107 elements to seafloor.
07:28:46 DEBUG opendrift:1636: No elements to deactivate
07:28:46 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:46 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:46 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:46 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:28:46 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0 0]
07:28:46 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3 3]
07:28:46 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 341. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:46 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:46 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:46 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:46 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:46 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.04514399635545456
07:28:46 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:46 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:46 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:46 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 13 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:46 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:46 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:46 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:46 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:46 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:46 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(130), np.int64(0), np.int64(4), np.int64(366), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:46 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:46 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:46 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:46 DEBUG opendrift:2034: ======================================================================
07:28:46 INFO opendrift:2035: 2025-07-08 05:27:52.765301 - step 45 of 96 - 500 active elements (0 deactivated)
07:28:46 DEBUG opendrift:2041: 0 elements scheduled.
07:28:46 DEBUG opendrift:2043: ======================================================================
07:28:46 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.70460638284541
07:28:46 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.756323773644302
07:28:46 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.536149425054147
07:28:46 DEBUG opendrift:2055: ---------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:46 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:46 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:46 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:46 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 05:00:00 (before)
2025-07-08 06:00:00 (after)
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:48 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:48 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 45x36x7) for time after (2025-07-08 06:00:00)
07:28:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 05:00:00) in space (linearNDFast)
07:28:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:48 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 06:00:00) in space (linearNDFast)
07:28:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:48 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 05:00:00, weight 0.54) and
after (2025-07-08 06:00:00, weight 0.46) in time
07:28:48 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:48 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24368728505594 degrees.
07:28:48 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24368728505594 degrees.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0314355 (min) 0.135221 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0407442 (min) 0.247002 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.305925 (min) -0.283037 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.24436 (min) -2.79623 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.38431 (min) -6.88739 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3265 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6251 (min) 16.1527 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2735 (min) 32.0102 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000119719 (min) 2.00229e-05 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:48 DEBUG opendrift:685: No elements hit coastline.
07:28:48 DEBUG opendrift:719: Lifting 123 elements to seafloor.
07:28:48 DEBUG opendrift:1636: No elements to deactivate
07:28:48 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:48 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:48 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:48 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:48 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0]
07:28:48 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3]
07:28:48 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 346. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:48 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:48 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:48 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:48 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:48 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.04701321835076686
07:28:48 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:48 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:48 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:28:48 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 14 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:48 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:48 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:48 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:48 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(125), np.int64(0), np.int64(4), np.int64(371), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:48 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:48 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:48 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:48 DEBUG opendrift:2034: ======================================================================
07:28:48 INFO opendrift:2035: 2025-07-08 05:57:52.765301 - step 46 of 96 - 500 active elements (0 deactivated)
07:28:48 DEBUG opendrift:2041: 0 elements scheduled.
07:28:48 DEBUG opendrift:2043: ======================================================================
07:28:48 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.70795871775461
07:28:48 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.758487062354922
07:28:48 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.5604108052210164
07:28:48 DEBUG opendrift:2055: ---------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 05:00:00 (before)
2025-07-08 06:00:00 (after)
07:28:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 05:00:00) in space (linearNDFast)
07:28:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:48 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 06:00:00) in space (linearNDFast)
07:28:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:48 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 05:00:00, weight 0.04) and
after (2025-07-08 06:00:00, weight 0.96) in time
07:28:48 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:48 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24152398364925 degrees.
07:28:48 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.24152398364925 degrees.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0411676 (min) 0.142966 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0431469 (min) 0.26883 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.341425 (min) -0.318435 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.35123 (min) -2.77681 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.50976 (min) -7.11291 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3303 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6297 (min) 16.1458 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2758 (min) 32.0159 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000122848 (min) 2.03237e-05 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:48 DEBUG opendrift:685: No elements hit coastline.
07:28:48 DEBUG opendrift:719: Lifting 142 elements to seafloor.
07:28:48 DEBUG opendrift:1636: No elements to deactivate
07:28:48 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:48 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:48 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:48 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:48 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0]
07:28:48 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3]
07:28:48 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 350. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 11. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:48 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:48 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:48 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:48 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:48 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.04883069795849021
07:28:48 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:48 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:48 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:48 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:48 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:48 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:48 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:48 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:48 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:48 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(121), np.int64(0), np.int64(4), np.int64(375), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:48 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:48 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:48 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:48 DEBUG opendrift:2034: ======================================================================
07:28:48 INFO opendrift:2035: 2025-07-08 06:27:52.765301 - step 47 of 96 - 500 active elements (0 deactivated)
07:28:48 DEBUG opendrift:2041: 0 elements scheduled.
07:28:48 DEBUG opendrift:2043: ======================================================================
07:28:48 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.71230353391076
07:28:48 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.76084989834401
07:28:48 DEBUG opendrift:2054: -23.38407325744629 <- z -> -0.16874447612710028
07:28:48 DEBUG opendrift:2055: ---------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 06:00:00 (before)
2025-07-08 07:00:00 (after)
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:51 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:51 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 46x36x7) for time after (2025-07-08 07:00:00)
07:28:51 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 06:00:00) in space (linearNDFast)
07:28:51 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:51 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 07:00:00) in space (linearNDFast)
07:28:51 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:51 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 06:00:00, weight 0.54) and
after (2025-07-08 07:00:00, weight 0.46) in time
07:28:51 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:51 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23916114509249 degrees.
07:28:51 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23916114509249 degrees.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:51 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0259884 (min) 0.145623 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.042084 (min) 0.245309 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.364466 (min) -0.341538 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.47006 (min) -2.96669 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.74669 (min) -7.38463 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3416 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.63 (min) 16.1361 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2778 (min) 32.0216 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000118078 (min) 2.4353e-05 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:51 DEBUG opendrift:685: No elements hit coastline.
07:28:51 DEBUG opendrift:719: Lifting 364 elements to seafloor.
07:28:51 DEBUG opendrift:1636: No elements to deactivate
07:28:51 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:51 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:51 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:51 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 8
07:28:51 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0 0 0 0 0 0]
07:28:51 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3 3 3 3 3]
07:28:51 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 357. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 12. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:51 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:51 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:51 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:51 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:51 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.051875887159505286
07:28:51 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:51 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:51 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:548: 7 elements reached seafloor, interacting with bottom
07:28:51 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:28:51 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 14 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:51 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:51 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:51 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:51 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(115), np.int64(0), np.int64(4), np.int64(381), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:51 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:51 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:51 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:51 DEBUG opendrift:2034: ======================================================================
07:28:51 INFO opendrift:2035: 2025-07-08 06:57:52.765301 - step 48 of 96 - 500 active elements (0 deactivated)
07:28:51 DEBUG opendrift:2041: 0 elements scheduled.
07:28:51 DEBUG opendrift:2043: ======================================================================
07:28:51 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.71613839077241
07:28:51 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.76338501078317
07:28:51 DEBUG opendrift:2054: -23.365463256835938 <- z -> -0.2152774131683115
07:28:51 DEBUG opendrift:2055: ---------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 06:00:00 (before)
2025-07-08 07:00:00 (after)
07:28:51 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 06:00:00) in space (linearNDFast)
07:28:51 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:51 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 07:00:00) in space (linearNDFast)
07:28:51 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:51 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 06:00:00, weight 0.04) and
after (2025-07-08 07:00:00, weight 0.96) in time
07:28:51 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:51 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23662602863941 degrees.
07:28:51 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23662602863941 degrees.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:51 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0166024 (min) 0.150927 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0407576 (min) 0.279858 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.386558 (min) -0.363573 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.58356 (min) -3.13946 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.99761 (min) -7.59155 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3539 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.63 (min) 16.1262 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2796 (min) 32.0276 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000116485 (min) 2.79476e-05 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:51 DEBUG opendrift:685: No elements hit coastline.
07:28:51 DEBUG opendrift:719: Lifting 381 elements to seafloor.
07:28:51 DEBUG opendrift:1636: No elements to deactivate
07:28:51 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:51 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:51 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:51 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 6
07:28:51 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0 0]
07:28:51 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3 3]
07:28:51 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 363. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 12. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:51 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:51 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:51 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:51 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:51 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.05508699021864965
07:28:51 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:51 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:51 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:28:51 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:51 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:51 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:51 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:51 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:51 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(109), np.int64(0), np.int64(4), np.int64(387), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:51 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:51 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:51 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:51 DEBUG opendrift:2034: ======================================================================
07:28:51 INFO opendrift:2035: 2025-07-08 07:27:52.765301 - step 49 of 96 - 500 active elements (0 deactivated)
07:28:51 DEBUG opendrift:2041: 0 elements scheduled.
07:28:51 DEBUG opendrift:2043: ======================================================================
07:28:51 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.7200454819019
07:28:51 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.766105657008204
07:28:51 DEBUG opendrift:2054: -23.4953212395696 <- z -> -0.33147750476953064
07:28:51 DEBUG opendrift:2055: ---------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 07:00:00 (before)
2025-07-08 08:00:00 (after)
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:53 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:53 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 47x36x7) for time after (2025-07-08 08:00:00)
07:28:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 07:00:00) in space (linearNDFast)
07:28:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:53 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 08:00:00) in space (linearNDFast)
07:28:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:53 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 07:00:00, weight 0.54) and
after (2025-07-08 08:00:00, weight 0.46) in time
07:28:53 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:53 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23390539181266 degrees.
07:28:53 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23390539181266 degrees.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0182218 (min) 0.155334 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0355895 (min) 0.296149 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.402323 (min) -0.379157 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.00258 (min) -3.3557 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.04785 (min) -7.70288 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.3807 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6346 (min) 16.1254 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.282 (min) 32.0352 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00011451 (min) 3.06643e-05 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:53 DEBUG opendrift:685: No elements hit coastline.
07:28:53 DEBUG opendrift:719: Lifting 387 elements to seafloor.
07:28:53 DEBUG opendrift:1636: No elements to deactivate
07:28:53 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:53 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:53 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:53 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:53 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0 0]
07:28:53 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3]
07:28:53 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 366. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 13. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:53 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:53 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:53 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:53 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:53 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.057810537073779644
07:28:53 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:53 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:53 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:53 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:28:53 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:28:53 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:53 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:53 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:53 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:53 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(107), np.int64(0), np.int64(4), np.int64(389), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:53 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:53 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:53 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:53 DEBUG opendrift:2034: ======================================================================
07:28:53 INFO opendrift:2035: 2025-07-08 07:57:52.765301 - step 50 of 96 - 500 active elements (0 deactivated)
07:28:53 DEBUG opendrift:2041: 0 elements scheduled.
07:28:53 DEBUG opendrift:2043: ======================================================================
07:28:53 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.72483182828294
07:28:53 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.768960744824694
07:28:53 DEBUG opendrift:2054: -23.903553009033203 <- z -> -0.20619877937128284
07:28:53 DEBUG opendrift:2055: ---------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 07:00:00 (before)
2025-07-08 08:00:00 (after)
07:28:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 07:00:00) in space (linearNDFast)
07:28:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:53 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 08:00:00) in space (linearNDFast)
07:28:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:53 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 07:00:00, weight 0.04) and
after (2025-07-08 08:00:00, weight 0.96) in time
07:28:53 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:53 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23105029459758 degrees.
07:28:53 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.23105029459758 degrees.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0294657 (min) 0.160176 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0301293 (min) 0.263272 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.417606 (min) -0.394044 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.46098 (min) -3.53495 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.11626 (min) -7.75892 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.4762 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6396 (min) 16.1254 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2843 (min) 31.9498 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000112816 (min) 2.27554e-05 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:53 DEBUG opendrift:685: No elements hit coastline.
07:28:53 DEBUG opendrift:719: Lifting 389 elements to seafloor.
07:28:53 DEBUG opendrift:1636: No elements to deactivate
07:28:53 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:53 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:53 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:53 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:53 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0]
07:28:53 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3]
07:28:53 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 370. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 36. 0.]
[ 13. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:53 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:53 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:53 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:53 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:53 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06060347154392556
07:28:53 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:53 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:53 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:53 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:53 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:53 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:53 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:53 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:53 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:53 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(103), np.int64(0), np.int64(4), np.int64(393), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:53 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:53 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:53 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:53 DEBUG opendrift:2034: ======================================================================
07:28:53 INFO opendrift:2035: 2025-07-08 08:27:52.765301 - step 51 of 96 - 500 active elements (0 deactivated)
07:28:53 DEBUG opendrift:2041: 0 elements scheduled.
07:28:53 DEBUG opendrift:2043: ======================================================================
07:28:53 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.72908681542871
07:28:53 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.768072791054692
07:28:53 DEBUG opendrift:2054: -23.888315200805664 <- z -> -0.5737464599312727
07:28:53 DEBUG opendrift:2055: ---------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 08:00:00 (before)
2025-07-08 09:00:00 (after)
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:55 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:55 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x36x7) for time after (2025-07-08 09:00:00)
07:28:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 08:00:00) in space (linearNDFast)
07:28:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 09:00:00) in space (linearNDFast)
07:28:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 08:00:00, weight 0.54) and
after (2025-07-08 09:00:00, weight 0.46) in time
07:28:55 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:55 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2319382623913 degrees.
07:28:55 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.2319382623913 degrees.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.00482894 (min) 0.16027 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.020988 (min) 0.244476 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.420578 (min) -0.399465 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.72221 (min) -3.62026 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.99934 (min) -7.66955 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 24.6698 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6421 (min) 16.1286 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2862 (min) 32.0356 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00014521 (min) 3.41444e-05 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:55 DEBUG opendrift:685: No elements hit coastline.
07:28:55 DEBUG opendrift:719: Lifting 393 elements to seafloor.
07:28:55 DEBUG opendrift:1636: No elements to deactivate
07:28:55 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:55 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:55 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:55 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:55 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 2 0 0 0]
07:28:55 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3 3]
07:28:55 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 374. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 13. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:55 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:55 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:55 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:55 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:55 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.061972201738326266
07:28:55 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:55 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:55 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:55 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 13 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:55 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:55 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:55 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:55 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:55 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(100), np.int64(0), np.int64(3), np.int64(397), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:55 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:55 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:55 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:55 DEBUG opendrift:2034: ======================================================================
07:28:55 INFO opendrift:2035: 2025-07-08 08:57:52.765301 - step 52 of 96 - 500 active elements (0 deactivated)
07:28:55 DEBUG opendrift:2041: 0 elements scheduled.
07:28:55 DEBUG opendrift:2043: ======================================================================
07:28:55 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.73216865591646
07:28:55 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.771179367879865
07:28:55 DEBUG opendrift:2054: -23.886178970336914 <- z -> -0.28562765669113954
07:28:55 DEBUG opendrift:2055: ---------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 08:00:00 (before)
2025-07-08 09:00:00 (after)
07:28:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 08:00:00) in space (linearNDFast)
07:28:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 09:00:00) in space (linearNDFast)
07:28:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 08:00:00, weight 0.04) and
after (2025-07-08 09:00:00, weight 0.96) in time
07:28:55 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:55 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22883168123569 degrees.
07:28:55 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22883168123569 degrees.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:55 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0101472 (min) 0.161076 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0115666 (min) 0.230008 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.424663 (min) -0.403897 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.97036 (min) -3.69843 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.98796 (min) -7.51165 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.1391 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6427 (min) 16.1316 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2881 (min) 32.0919 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000133928 (min) 4.29787e-05 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:55 DEBUG opendrift:685: No elements hit coastline.
07:28:55 DEBUG opendrift:719: Lifting 397 elements to seafloor.
07:28:55 DEBUG opendrift:1636: No elements to deactivate
07:28:55 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:55 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:55 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:55 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:28:55 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 3]
07:28:55 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 0]
07:28:55 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 378. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 14. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:55 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:55 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:55 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:55 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:55 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06339782172759653
07:28:55 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:55 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:55 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:28:55 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:55 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:55 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:55 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:55 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:55 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:55 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(97), np.int64(0), np.int64(3), np.int64(400), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:55 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:55 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:55 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:55 DEBUG opendrift:2034: ======================================================================
07:28:55 INFO opendrift:2035: 2025-07-08 09:27:52.765301 - step 53 of 96 - 500 active elements (0 deactivated)
07:28:55 DEBUG opendrift:2041: 0 elements scheduled.
07:28:55 DEBUG opendrift:2043: ======================================================================
07:28:55 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.735886017679654
07:28:55 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.772333048913866
07:28:55 DEBUG opendrift:2054: -23.885034561157227 <- z -> -0.1346346881981466
07:28:55 DEBUG opendrift:2055: ---------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 09:00:00 (before)
2025-07-08 10:00:00 (after)
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:57 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:57 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x36x7) for time after (2025-07-08 10:00:00)
07:28:57 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 09:00:00) in space (linearNDFast)
07:28:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:57 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 10:00:00) in space (linearNDFast)
07:28:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:57 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 09:00:00, weight 0.54) and
after (2025-07-08 10:00:00, weight 0.46) in time
07:28:57 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:57 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227677990723286 degrees.
07:28:57 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227677990723286 degrees.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:57 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:57 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0185301 (min) 0.153676 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.00766447 (min) 0.180888 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.404889 (min) -0.384138 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.12137 (min) -4.14684 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.86934 (min) -7.38599 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.5719 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6469 (min) 16.1317 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2904 (min) 31.9713 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000112824 (min) 3.90399e-05 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:57 DEBUG opendrift:685: No elements hit coastline.
07:28:57 DEBUG opendrift:714: No elements hit seafloor.
07:28:57 DEBUG opendrift:1636: No elements to deactivate
07:28:57 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:57 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:57 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:57 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:57 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0 0]
07:28:57 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 3]
07:28:57 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 381. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 15. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:57 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:57 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:57 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:57 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:57 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06286916393540595
07:28:57 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:57 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:57 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:57 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:28:57 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:57 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:57 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:57 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:57 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:57 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(95), np.int64(0), np.int64(3), np.int64(402), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:57 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:57 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:57 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:57 DEBUG opendrift:2034: ======================================================================
07:28:57 INFO opendrift:2035: 2025-07-08 09:57:52.765301 - step 54 of 96 - 500 active elements (0 deactivated)
07:28:57 DEBUG opendrift:2041: 0 elements scheduled.
07:28:57 DEBUG opendrift:2043: ======================================================================
07:28:57 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.737838626860054
07:28:57 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.772191012532677
07:28:57 DEBUG opendrift:2054: -23.885034561157227 <- z -> -0.05577517649114894
07:28:57 DEBUG opendrift:2055: ---------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 09:00:00 (before)
2025-07-08 10:00:00 (after)
07:28:57 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 09:00:00) in space (linearNDFast)
07:28:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:57 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 10:00:00) in space (linearNDFast)
07:28:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:57 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 09:00:00, weight 0.04) and
after (2025-07-08 10:00:00, weight 0.96) in time
07:28:57 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:57 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22782002955038 degrees.
07:28:57 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22782002955038 degrees.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:57 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:57 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:57 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:57 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:57 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.027146 (min) 0.14676 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0276417 (min) 0.141911 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.385378 (min) -0.354668 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.23765 (min) -4.60913 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.9141 (min) -7.25906 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 26.2066 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6514 (min) 16.1316 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2928 (min) 32.037 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000102229 (min) 5.24013e-05 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:57 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:57 DEBUG opendrift:685: No elements hit coastline.
07:28:57 DEBUG opendrift:714: No elements hit seafloor.
07:28:57 DEBUG opendrift:1636: No elements to deactivate
07:28:57 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:57 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:57 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:57 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:28:57 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3]
07:28:57 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0]
07:28:57 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 383. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 16. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:57 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:57 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:57 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:57 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:57 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.061839174093378683
07:28:57 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:57 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:57 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:28:57 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 12 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:57 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:57 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:57 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:57 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:57 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:57 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(94), np.int64(0), np.int64(3), np.int64(403), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:57 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:57 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:57 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:57 DEBUG opendrift:2034: ======================================================================
07:28:57 INFO opendrift:2035: 2025-07-08 10:27:52.765301 - step 55 of 96 - 500 active elements (0 deactivated)
07:28:57 DEBUG opendrift:2041: 0 elements scheduled.
07:28:57 DEBUG opendrift:2043: ======================================================================
07:28:57 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.73979886953207
07:28:57 DEBUG opendrift:2054: 10.511721845606994 <- longitude -> 10.775388265540625
07:28:57 DEBUG opendrift:2054: -23.885034561157227 <- z -> -0.16137927778157257
07:28:57 DEBUG opendrift:2055: ---------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 10:00:00 (before)
2025-07-08 11:00:00 (after)
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:28:59 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:28:59 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x36x7) for time after (2025-07-08 11:00:00)
07:28:59 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 10:00:00) in space (linearNDFast)
07:28:59 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:59 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 11:00:00) in space (linearNDFast)
07:28:59 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:59 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 10:00:00, weight 0.54) and
after (2025-07-08 11:00:00, weight 0.46) in time
07:28:59 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:59 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22462277524428 degrees.
07:28:59 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22462277524428 degrees.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:59 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:59 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0489843 (min) 0.13069 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0546299 (min) 0.110434 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.339261 (min) -0.30561 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.20484 (min) -4.51909 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.80149 (min) -7.34119 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 26.5167 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6564 (min) 16.1363 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2961 (min) 31.9787 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.30905e-05 (min) 4.9165e-05 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:59 DEBUG opendrift:685: No elements hit coastline.
07:28:59 DEBUG opendrift:714: No elements hit seafloor.
07:28:59 DEBUG opendrift:1636: No elements to deactivate
07:28:59 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:59 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:59 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:59 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:28:59 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0]
07:28:59 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3]
07:28:59 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 386. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 16. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:59 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:59 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:59 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:59 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:59 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.062146902905592355
07:28:59 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:59 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:59 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:28:59 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:59 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:59 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:59 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:59 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:59 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(91), np.int64(0), np.int64(3), np.int64(406), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:59 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:59 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:59 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:59 DEBUG opendrift:2034: ======================================================================
07:28:59 INFO opendrift:2035: 2025-07-08 10:57:52.765301 - step 56 of 96 - 500 active elements (0 deactivated)
07:28:59 DEBUG opendrift:2041: 0 elements scheduled.
07:28:59 DEBUG opendrift:2043: ======================================================================
07:28:59 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.74056766510617
07:28:59 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.775295522884871
07:28:59 DEBUG opendrift:2054: -23.885034561157227 <- z -> -0.04808516584482847
07:28:59 DEBUG opendrift:2055: ---------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 10:00:00 (before)
2025-07-08 11:00:00 (after)
07:28:59 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 10:00:00) in space (linearNDFast)
07:28:59 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:59 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 11:00:00) in space (linearNDFast)
07:28:59 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:28:59 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 10:00:00, weight 0.04) and
after (2025-07-08 11:00:00, weight 0.96) in time
07:28:59 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:28:59 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22471551831625 degrees.
07:28:59 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22471551831625 degrees.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:28:59 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:28:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:28:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:28:59 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:28:59 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:28:59 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:28:59 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0880994 (min) 0.116609 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0821514 (min) 0.0779414 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.292272 (min) -0.255244 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.15263 (min) -4.38703 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.7654 (min) -7.44895 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 26.5378 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6598 (min) 16.1413 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2994 (min) 31.9982 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.86931e-05 (min) 9.83611e-05 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:28:59 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:28:59 DEBUG opendrift:685: No elements hit coastline.
07:28:59 DEBUG opendrift:714: No elements hit seafloor.
07:28:59 DEBUG opendrift:1636: No elements to deactivate
07:28:59 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:28:59 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:28:59 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:28:59 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:28:59 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3 3]
07:28:59 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0 0]
07:28:59 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 388. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 36. 0.]
[ 18. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:28:59 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:59 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:59 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:28:59 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:28:59 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06271203738641347
07:28:59 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:28:59 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:28:59 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:28:59 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:28:59 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:28:59 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:28:59 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:28:59 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:28:59 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:28:59 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:28:59 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(91), np.int64(0), np.int64(2), np.int64(407), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:28:59 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:28:59 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:28:59 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:28:59 DEBUG opendrift:2034: ======================================================================
07:28:59 INFO opendrift:2035: 2025-07-08 11:27:52.765301 - step 57 of 96 - 500 active elements (0 deactivated)
07:28:59 DEBUG opendrift:2041: 0 elements scheduled.
07:28:59 DEBUG opendrift:2043: ======================================================================
07:28:59 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.74006112198885
07:28:59 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.777818356159466
07:28:59 DEBUG opendrift:2054: -24.437157150452595 <- z -> -0.2249924387353076
07:28:59 DEBUG opendrift:2055: ---------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:28:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:28:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:28:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:28:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:28:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 11:00:00 (before)
2025-07-08 12:00:00 (after)
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:02 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:02 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x36x7) for time after (2025-07-08 12:00:00)
07:29:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 11:00:00) in space (linearNDFast)
07:29:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 12:00:00) in space (linearNDFast)
07:29:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 11:00:00, weight 0.54) and
after (2025-07-08 12:00:00, weight 0.46) in time
07:29:02 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:02 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.222192676320354 degrees.
07:29:02 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.222192676320354 degrees.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:02 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:02 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0864978 (min) 0.0979902 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.113032 (min) 0.0444707 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.231502 (min) -0.204835 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.05136 (min) -4.59174 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.07013 (min) -7.56054 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 26.4176 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6623 (min) 16.1493 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2993 (min) 32.4937 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.68019e-05 (min) 0.000237983 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:02 DEBUG opendrift:685: No elements hit coastline.
07:29:02 DEBUG opendrift:714: No elements hit seafloor.
07:29:02 DEBUG opendrift:1636: No elements to deactivate
07:29:02 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:02 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:02 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:02 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:29:02 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0]
07:29:02 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3]
07:29:02 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 392. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 37. 0.]
[ 18. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:02 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:02 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:02 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:02 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:02 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06546488350973402
07:29:02 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:02 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:02 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:29:02 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:02 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:02 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:02 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:02 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:02 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(87), np.int64(0), np.int64(1), np.int64(412), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:02 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:02 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:02 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:02 DEBUG opendrift:2034: ======================================================================
07:29:02 INFO opendrift:2035: 2025-07-08 11:57:52.765301 - step 58 of 96 - 500 active elements (0 deactivated)
07:29:02 DEBUG opendrift:2041: 0 elements scheduled.
07:29:02 DEBUG opendrift:2043: ======================================================================
07:29:02 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.738934080329834
07:29:02 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.777053948385552
07:29:02 DEBUG opendrift:2054: -24.098880767822266 <- z -> -0.5218765177481077
07:29:02 DEBUG opendrift:2055: ---------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 11:00:00 (before)
2025-07-08 12:00:00 (after)
07:29:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 11:00:00) in space (linearNDFast)
07:29:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 12:00:00) in space (linearNDFast)
07:29:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 11:00:00, weight 0.04) and
after (2025-07-08 12:00:00, weight 0.96) in time
07:29:02 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:02 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.222957098674705 degrees.
07:29:02 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.222957098674705 degrees.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:02 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:02 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:02 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:02 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:02 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:02 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.118993 (min) 0.085473 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.14297 (min) 0.0119202 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.170354 (min) -0.154354 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.06769 (min) -4.81859 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.38907 (min) -7.61554 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.9852 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6629 (min) 16.1576 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.296 (min) 32.2689 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.6641e-05 (min) 0.000376203 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:02 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:02 DEBUG opendrift:685: No elements hit coastline.
07:29:02 DEBUG opendrift:714: No elements hit seafloor.
07:29:02 DEBUG opendrift:1636: No elements to deactivate
07:29:02 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:02 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:02 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:02 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:02 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0]
07:29:02 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3]
07:29:02 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 395. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 18. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:02 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:02 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:02 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:02 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:02 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06855179565947495
07:29:02 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:02 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:02 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:02 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:29:02 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 10 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:02 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:02 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:02 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:02 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:02 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:02 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(84), np.int64(0), np.int64(1), np.int64(415), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:02 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:02 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:02 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:02 DEBUG opendrift:2034: ======================================================================
07:29:02 INFO opendrift:2035: 2025-07-08 12:27:52.765301 - step 59 of 96 - 500 active elements (0 deactivated)
07:29:02 DEBUG opendrift:2041: 0 elements scheduled.
07:29:02 DEBUG opendrift:2043: ======================================================================
07:29:02 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.737020237951526
07:29:02 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.777613313902336
07:29:02 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.036339498485453925
07:29:02 DEBUG opendrift:2055: ---------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:02 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 12:00:00 (before)
2025-07-08 13:00:00 (after)
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:04 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:04 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x36x7) for time after (2025-07-08 13:00:00)
07:29:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 12:00:00) in space (linearNDFast)
07:29:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 13:00:00) in space (linearNDFast)
07:29:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 12:00:00, weight 0.54) and
after (2025-07-08 13:00:00, weight 0.46) in time
07:29:04 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:04 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22239772611489 degrees.
07:29:04 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22239772611489 degrees.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.169904 (min) 0.0709817 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.14976 (min) -0.00652418 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.127989 (min) -0.113341 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.12608 (min) -4.81225 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.32752 (min) -7.50123 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.398 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6683 (min) 16.1675 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2966 (min) 32.1823 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -7.86017e-05 (min) 0.000465655 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:04 DEBUG opendrift:685: No elements hit coastline.
07:29:04 DEBUG opendrift:714: No elements hit seafloor.
07:29:04 DEBUG opendrift:1636: No elements to deactivate
07:29:04 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:04 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:04 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:04 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:04 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0]
07:29:04 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3]
07:29:04 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 397. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 19. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:04 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:04 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:04 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:04 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:04 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06848932068057388
07:29:04 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:04 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:04 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:04 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:04 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 11 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:04 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:04 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:04 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:04 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(83), np.int64(0), np.int64(1), np.int64(416), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:04 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:04 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:04 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:04 DEBUG opendrift:2034: ======================================================================
07:29:04 INFO opendrift:2035: 2025-07-08 12:57:52.765301 - step 60 of 96 - 500 active elements (0 deactivated)
07:29:04 DEBUG opendrift:2041: 0 elements scheduled.
07:29:04 DEBUG opendrift:2043: ======================================================================
07:29:04 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.735237442578146
07:29:04 DEBUG opendrift:2054: 10.511721845606997 <- longitude -> 10.778652367003616
07:29:04 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.13222420369158105
07:29:04 DEBUG opendrift:2055: ---------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 12:00:00 (before)
2025-07-08 13:00:00 (after)
07:29:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 12:00:00) in space (linearNDFast)
07:29:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 13:00:00) in space (linearNDFast)
07:29:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 12:00:00, weight 0.04) and
after (2025-07-08 13:00:00, weight 0.96) in time
07:29:04 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:04 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.221358671925245 degrees.
07:29:04 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.221358671925245 degrees.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:04 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.207912 (min) 0.0593352 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.171733 (min) -0.018879 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0907504 (min) -0.0687471 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.18659 (min) -4.78417 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.23634 (min) -7.25954 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.3291 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6737 (min) 16.1775 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.2975 (min) 32.1037 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -8.38652e-05 (min) 0.000480608 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:04 DEBUG opendrift:685: No elements hit coastline.
07:29:04 DEBUG opendrift:714: No elements hit seafloor.
07:29:04 DEBUG opendrift:1636: No elements to deactivate
07:29:04 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:04 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:04 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:04 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:04 DEBUG opendrift.models.chemicaldrift:1476: old species: [0]
07:29:04 DEBUG opendrift.models.chemicaldrift:1477: new species: [3]
07:29:04 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 398. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 19. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:04 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:04 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:04 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:04 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:04 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06820826800650309
07:29:04 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:04 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:04 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:04 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:04 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:04 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:04 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:04 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:04 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(82), np.int64(0), np.int64(1), np.int64(417), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:04 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:04 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:04 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:04 DEBUG opendrift:2034: ======================================================================
07:29:04 INFO opendrift:2035: 2025-07-08 13:27:52.765301 - step 61 of 96 - 500 active elements (0 deactivated)
07:29:04 DEBUG opendrift:2041: 0 elements scheduled.
07:29:04 DEBUG opendrift:2043: ======================================================================
07:29:04 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.73390254161621
07:29:04 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.776406678133958
07:29:04 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.6793776886404859
07:29:04 DEBUG opendrift:2055: ---------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 13:00:00 (before)
2025-07-08 14:00:00 (after)
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:06 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:06 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x37x7) for time after (2025-07-08 14:00:00)
07:29:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 13:00:00) in space (linearNDFast)
07:29:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:06 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 14:00:00) in space (linearNDFast)
07:29:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:06 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 13:00:00, weight 0.54) and
after (2025-07-08 14:00:00, weight 0.46) in time
07:29:06 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:06 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22360436780916 degrees.
07:29:06 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22360436780916 degrees.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:06 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.209715 (min) 0.055573 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.159925 (min) -0.0111922 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0890608 (min) -0.0546656 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.04409 (min) -4.72332 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.05529 (min) -7.34418 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.1927 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6788 (min) 16.1875 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3018 (min) 32.1245 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.03506e-05 (min) 0.00051717 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:06 DEBUG opendrift:685: No elements hit coastline.
07:29:06 DEBUG opendrift:714: No elements hit seafloor.
07:29:06 DEBUG opendrift:1636: No elements to deactivate
07:29:06 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:06 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:06 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:06 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 0
07:29:06 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:06 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06513431969732074
07:29:06 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:06 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:06 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:06 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:06 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:06 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:06 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:06 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(82), np.int64(0), np.int64(1), np.int64(417), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:06 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:06 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:06 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:06 DEBUG opendrift:2034: ======================================================================
07:29:06 INFO opendrift:2035: 2025-07-08 13:57:52.765301 - step 62 of 96 - 500 active elements (0 deactivated)
07:29:06 DEBUG opendrift:2041: 0 elements scheduled.
07:29:06 DEBUG opendrift:2043: ======================================================================
07:29:06 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.73329594342955
07:29:06 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.774439035427834
07:29:06 DEBUG opendrift:2054: -24.916128158569336 <- z -> 0.0
07:29:06 DEBUG opendrift:2055: ---------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 13:00:00 (before)
2025-07-08 14:00:00 (after)
07:29:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 13:00:00) in space (linearNDFast)
07:29:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:06 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 14:00:00) in space (linearNDFast)
07:29:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:06 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 13:00:00, weight 0.04) and
after (2025-07-08 14:00:00, weight 0.96) in time
07:29:06 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:06 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22557199290903 degrees.
07:29:06 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22557199290903 degrees.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:06 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:06 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:06 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:06 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.195462 (min) 0.0609826 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.155843 (min) 0.0230676 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.089992 (min) -0.0429242 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.00219 (min) -4.65669 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.86581 (min) -7.46033 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.1284 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6833 (min) 16.1975 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3064 (min) 32.3365 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.62181e-05 (min) 0.000499061 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:06 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:06 DEBUG opendrift:685: No elements hit coastline.
07:29:06 DEBUG opendrift:714: No elements hit seafloor.
07:29:06 DEBUG opendrift:1636: No elements to deactivate
07:29:06 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:06 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:06 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:06 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:29:06 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0 0 0]
07:29:06 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3 3 3]
07:29:06 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 403. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 19. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:06 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:06 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:06 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:06 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:06 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.06210148709814528
07:29:06 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:06 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:06 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:548: 5 elements reached seafloor, interacting with bottom
07:29:06 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:06 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:06 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:06 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:06 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:06 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:06 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(77), np.int64(0), np.int64(1), np.int64(422), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:06 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:06 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:06 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:06 DEBUG opendrift:2034: ======================================================================
07:29:06 INFO opendrift:2035: 2025-07-08 14:27:52.765301 - step 63 of 96 - 500 active elements (0 deactivated)
07:29:06 DEBUG opendrift:2041: 0 elements scheduled.
07:29:06 DEBUG opendrift:2043: ======================================================================
07:29:06 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.733295943429546
07:29:06 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.77221474815016
07:29:06 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.04507384978473894
07:29:06 DEBUG opendrift:2055: ---------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 14:00:00 (before)
2025-07-08 15:00:00 (after)
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:08 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:08 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x37x7) for time after (2025-07-08 15:00:00)
07:29:08 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 14:00:00) in space (linearNDFast)
07:29:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:08 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 15:00:00) in space (linearNDFast)
07:29:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:08 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 14:00:00, weight 0.54) and
after (2025-07-08 15:00:00, weight 0.46) in time
07:29:08 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:08 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227796291319336 degrees.
07:29:08 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227796291319336 degrees.
07:29:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:08 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:08 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:08 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:08 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.207882 (min) 0.0661583 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.139755 (min) 0.0801019 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.114815 (min) -0.0657918 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.19739 (min) -4.68483 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.45239 (min) -7.05427 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6805 (min) 16.2046 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3106 (min) 32.3598 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.17339e-05 (min) 0.000411211 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:08 DEBUG opendrift:685: No elements hit coastline.
07:29:08 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:29:09 DEBUG opendrift:1636: No elements to deactivate
07:29:09 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:09 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:09 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:09 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:29:09 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0 0 0 0 0 0]
07:29:09 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3 3 3 3 3 3]
07:29:09 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 409. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 20. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:09 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:09 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:09 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.057971271750509305
07:29:09 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:09 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:09 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:29:09 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 8 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:09 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:09 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(72), np.int64(0), np.int64(1), np.int64(427), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:09 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:09 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:09 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:09 DEBUG opendrift:2034: ======================================================================
07:29:09 INFO opendrift:2035: 2025-07-08 14:57:52.765301 - step 64 of 96 - 500 active elements (0 deactivated)
07:29:09 DEBUG opendrift:2041: 0 elements scheduled.
07:29:09 DEBUG opendrift:2043: ======================================================================
07:29:09 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.73329594342954
07:29:09 DEBUG opendrift:2054: 10.511721845607005 <- longitude -> 10.77221474815016
07:29:09 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.42800711958833915
07:29:09 DEBUG opendrift:2055: ---------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 14:00:00 (before)
2025-07-08 15:00:00 (after)
07:29:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 14:00:00) in space (linearNDFast)
07:29:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 15:00:00) in space (linearNDFast)
07:29:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 14:00:00, weight 0.04) and
after (2025-07-08 15:00:00, weight 0.96) in time
07:29:09 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227796291319336 degrees.
07:29:09 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.227796291319336 degrees.
07:29:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:09 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.252134 (min) 0.071214 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.122754 (min) 0.208764 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.141474 (min) -0.0912918 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.46982 (min) -4.65261 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.06646 (min) -6.6072 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6773 (min) 16.2114 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3148 (min) 32.3781 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -9.54128e-05 (min) 0.000318544 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:09 DEBUG opendrift:685: No elements hit coastline.
07:29:09 DEBUG opendrift:719: Lifting 13 elements to seafloor.
07:29:09 DEBUG opendrift:1636: No elements to deactivate
07:29:09 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:09 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:09 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:09 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:09 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0]
07:29:09 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3]
07:29:09 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 412. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 20. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:09 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:09 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:09 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.05561167271550554
07:29:09 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:09 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:09 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:09 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:29:09 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 9 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:09 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:09 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:09 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:09 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:09 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:09 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(69), np.int64(0), np.int64(1), np.int64(430), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:09 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:09 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:09 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:09 DEBUG opendrift:2034: ======================================================================
07:29:09 INFO opendrift:2035: 2025-07-08 15:27:52.765301 - step 65 of 96 - 500 active elements (0 deactivated)
07:29:09 DEBUG opendrift:2041: 0 elements scheduled.
07:29:09 DEBUG opendrift:2043: ======================================================================
07:29:09 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.73329594342953
07:29:09 DEBUG opendrift:2054: 10.511721845607 <- longitude -> 10.77221474815016
07:29:09 DEBUG opendrift:2054: -24.916128158569336 <- z -> -0.011278129324804542
07:29:09 DEBUG opendrift:2055: ---------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 15:00:00 (before)
2025-07-08 16:00:00 (after)
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:11 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:11 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x37x7) for time after (2025-07-08 16:00:00)
07:29:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 15:00:00) in space (linearNDFast)
07:29:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 16:00:00) in space (linearNDFast)
07:29:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 15:00:00, weight 0.54) and
after (2025-07-08 16:00:00, weight 0.46) in time
07:29:11 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629131932 degrees.
07:29:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629131932 degrees.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:11 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.284509 (min) 0.0790567 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.101192 (min) 0.239066 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.18206 (min) -0.137868 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.07987 (min) -4.11135 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.909 (min) -6.14963 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6758 (min) 16.2166 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3183 (min) 32.346 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000147996 (min) 0.000275855 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:11 DEBUG opendrift:685: No elements hit coastline.
07:29:11 DEBUG opendrift:719: Lifting 21 elements to seafloor.
07:29:11 DEBUG opendrift:1636: No elements to deactivate
07:29:11 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:11 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:11 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:11 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 7
07:29:11 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0 0 0 0 0 0]
07:29:11 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3 3 3 3 3 3]
07:29:11 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 418. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 21. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:11 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:11 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:11 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.049259073100608175
07:29:11 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:11 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:11 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:548: 6 elements reached seafloor, interacting with bottom
07:29:11 DEBUG opendrift:719: Lifting 6 elements to seafloor.
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:11 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:11 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(64), np.int64(0), np.int64(1), np.int64(435), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:11 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:11 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:11 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:11 DEBUG opendrift:2034: ======================================================================
07:29:11 INFO opendrift:2035: 2025-07-08 15:57:52.765301 - step 66 of 96 - 500 active elements (0 deactivated)
07:29:11 DEBUG opendrift:2041: 0 elements scheduled.
07:29:11 DEBUG opendrift:2043: ======================================================================
07:29:11 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.734414130162925
07:29:11 DEBUG opendrift:2054: 10.511721845607003 <- longitude -> 10.77221474815016
07:29:11 DEBUG opendrift:2054: -24.90389633178711 <- z -> -0.4496022668390762
07:29:11 DEBUG opendrift:2055: ---------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 15:00:00 (before)
2025-07-08 16:00:00 (after)
07:29:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 15:00:00) in space (linearNDFast)
07:29:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 16:00:00) in space (linearNDFast)
07:29:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 15:00:00, weight 0.04) and
after (2025-07-08 16:00:00, weight 0.96) in time
07:29:11 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:11 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:11 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:11 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:11 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:11 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.183579 (min) 0.101277 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0792825 (min) 0.322531 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.227991 (min) -0.186048 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.75277 (min) -3.50287 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.82608 (min) -5.49896 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.677 (min) 16.2217 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3218 (min) 32.3101 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000165722 (min) 0.000231036 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:11 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:11 DEBUG opendrift:685: No elements hit coastline.
07:29:11 DEBUG opendrift:719: Lifting 27 elements to seafloor.
07:29:11 DEBUG opendrift:1636: No elements to deactivate
07:29:11 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:11 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:11 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:11 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:11 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:11 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:11 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 419. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 22. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:11 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:11 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:11 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.04301727689963544
07:29:11 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:11 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:11 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:11 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 7 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:11 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:11 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:11 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:11 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:11 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:11 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(64), np.int64(0), np.int64(1), np.int64(435), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:11 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:11 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:11 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:11 DEBUG opendrift:2034: ======================================================================
07:29:11 INFO opendrift:2035: 2025-07-08 16:27:52.765301 - step 67 of 96 - 500 active elements (0 deactivated)
07:29:11 DEBUG opendrift:2041: 0 elements scheduled.
07:29:11 DEBUG opendrift:2043: ======================================================================
07:29:11 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.739605833868644
07:29:11 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.77221474815016
07:29:11 DEBUG opendrift:2054: -24.862407684326172 <- z -> -0.13609170061352271
07:29:11 DEBUG opendrift:2055: ---------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 16:00:00 (before)
2025-07-08 17:00:00 (after)
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:13 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:13 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 48x37x7) for time after (2025-07-08 17:00:00)
07:29:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 16:00:00) in space (linearNDFast)
07:29:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 17:00:00) in space (linearNDFast)
07:29:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 16:00:00, weight 0.54) and
after (2025-07-08 17:00:00, weight 0.46) in time
07:29:13 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:13 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.316814 (min) 0.113021 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0626594 (min) 0.404761 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.270063 (min) -0.232133 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.60741 (min) -3.06244 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.9591 (min) -5.51927 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6802 (min) 16.2267 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3244 (min) 32.2556 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000195041 (min) 0.000191288 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:13 DEBUG opendrift:685: No elements hit coastline.
07:29:13 DEBUG opendrift:719: Lifting 32 elements to seafloor.
07:29:13 DEBUG opendrift:1636: No elements to deactivate
07:29:13 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:13 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:13 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:13 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:29:13 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3 0]
07:29:13 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0 3]
07:29:13 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 422. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 23. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:13 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:13 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:13 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.042082425830857834
07:29:13 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:13 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:13 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:29:13 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:13 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:13 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(62), np.int64(0), np.int64(1), np.int64(437), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:13 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:13 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:13 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:13 DEBUG opendrift:2034: ======================================================================
07:29:13 INFO opendrift:2035: 2025-07-08 16:57:52.765301 - step 68 of 96 - 500 active elements (0 deactivated)
07:29:13 DEBUG opendrift:2041: 0 elements scheduled.
07:29:13 DEBUG opendrift:2043: ======================================================================
07:29:13 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.74614718170603
07:29:13 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.77221474815016
07:29:13 DEBUG opendrift:2054: -24.82370948791504 <- z -> -0.4494140779153848
07:29:13 DEBUG opendrift:2055: ---------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 16:00:00 (before)
2025-07-08 17:00:00 (after)
07:29:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 16:00:00) in space (linearNDFast)
07:29:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 17:00:00) in space (linearNDFast)
07:29:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 16:00:00, weight 0.04) and
after (2025-07-08 17:00:00, weight 0.96) in time
07:29:13 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:13 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:13 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:13 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:13 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.354401 (min) 0.133597 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0464384 (min) 0.482348 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.312632 (min) -0.276926 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.47587 (min) -2.47284 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.06322 (min) -5.53069 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 25.0742 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6805 (min) 16.2317 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3269 (min) 32.1996 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000262688 (min) 0.000151925 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:13 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:13 DEBUG opendrift:685: No elements hit coastline.
07:29:13 DEBUG opendrift:719: Lifting 39 elements to seafloor.
07:29:13 DEBUG opendrift:1636: No elements to deactivate
07:29:13 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:13 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:13 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:13 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:13 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3]
07:29:13 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0]
07:29:13 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 424. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 24. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:13 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:13 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:13 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.04155853768697734
07:29:13 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:13 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:13 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:13 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:13 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:13 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:13 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:13 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:13 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:13 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(61), np.int64(0), np.int64(1), np.int64(438), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:13 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:13 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:13 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:13 DEBUG opendrift:2034: ======================================================================
07:29:13 INFO opendrift:2035: 2025-07-08 17:27:52.765301 - step 69 of 96 - 500 active elements (0 deactivated)
07:29:13 DEBUG opendrift:2041: 0 elements scheduled.
07:29:13 DEBUG opendrift:2043: ======================================================================
07:29:13 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.753942368862326
07:29:13 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.772214748150164
07:29:13 DEBUG opendrift:2054: -24.785221099853516 <- z -> -0.42716406811354424
07:29:13 DEBUG opendrift:2055: ---------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 17:00:00 (before)
2025-07-08 18:00:00 (after)
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:15 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x38x7) for time after (2025-07-08 18:00:00)
07:29:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 17:00:00) in space (linearNDFast)
07:29:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 18:00:00) in space (linearNDFast)
07:29:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 17:00:00, weight 0.54) and
after (2025-07-08 18:00:00, weight 0.46) in time
07:29:15 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.326219 (min) 0.124552 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0365913 (min) 0.498318 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.339469 (min) -0.30935 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.63505 (min) -2.15035 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.98716 (min) -5.7145 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 26.4287 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6836 (min) 16.2321 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3287 (min) 32.1798 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000969502 (min) 0.000123495 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:15 DEBUG opendrift:685: No elements hit coastline.
07:29:15 DEBUG opendrift:719: Lifting 41 elements to seafloor.
07:29:15 DEBUG opendrift:1636: No elements to deactivate
07:29:15 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:15 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:15 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:15 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:15 DEBUG opendrift.models.chemicaldrift:1476: old species: [3]
07:29:15 DEBUG opendrift.models.chemicaldrift:1477: new species: [0]
07:29:15 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 424. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 25. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:15 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:15 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:15 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03976047206597286
07:29:15 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:15 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:15 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:15 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:15 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(62), np.int64(0), np.int64(1), np.int64(437), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:15 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:15 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:15 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:15 DEBUG opendrift:2034: ======================================================================
07:29:15 INFO opendrift:2035: 2025-07-08 17:57:52.765301 - step 70 of 96 - 500 active elements (0 deactivated)
07:29:15 DEBUG opendrift:2041: 0 elements scheduled.
07:29:15 DEBUG opendrift:2043: ======================================================================
07:29:15 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.7596536948195
07:29:15 DEBUG opendrift:2054: 10.51172184560701 <- longitude -> 10.772214748150164
07:29:15 DEBUG opendrift:2054: -24.756946563720703 <- z -> -0.09747410397976841
07:29:15 DEBUG opendrift:2055: ---------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 17:00:00 (before)
2025-07-08 18:00:00 (after)
07:29:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 17:00:00) in space (linearNDFast)
07:29:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 18:00:00) in space (linearNDFast)
07:29:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 17:00:00, weight 0.04) and
after (2025-07-08 18:00:00, weight 0.96) in time
07:29:15 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:15 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:15 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.331166 (min) 0.120134 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0272291 (min) 0.540319 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.367858 (min) -0.33895 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.74027 (min) -1.36101 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.97108 (min) -5.84236 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 31.4028 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.687 (min) 16.2321 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3304 (min) 32.1711 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000391636 (min) 0.000107162 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:15 DEBUG opendrift:685: No elements hit coastline.
07:29:15 DEBUG opendrift:719: Lifting 43 elements to seafloor.
07:29:15 DEBUG opendrift:1636: No elements to deactivate
07:29:15 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:15 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:15 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:15 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:15 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0]
07:29:15 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3]
07:29:15 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 426. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 38. 0.]
[ 25. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:15 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:15 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:15 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03847357788032707
07:29:15 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:15 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:15 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:15 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:15 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:15 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:15 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:15 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:15 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:15 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(60), np.int64(0), np.int64(1), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:15 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:15 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:15 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:15 DEBUG opendrift:2034: ======================================================================
07:29:15 INFO opendrift:2035: 2025-07-08 18:27:52.765301 - step 71 of 96 - 500 active elements (0 deactivated)
07:29:15 DEBUG opendrift:2041: 0 elements scheduled.
07:29:15 DEBUG opendrift:2043: ======================================================================
07:29:15 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.76455204036852
07:29:15 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.772214748150166
07:29:15 DEBUG opendrift:2054: -24.72944450378418 <- z -> -0.9659244558016764
07:29:15 DEBUG opendrift:2055: ---------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 18:00:00 (before)
2025-07-08 19:00:00 (after)
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:17 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:17 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x40x7) for time after (2025-07-08 19:00:00)
07:29:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 18:00:00) in space (linearNDFast)
07:29:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 19:00:00) in space (linearNDFast)
07:29:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 18:00:00, weight 0.54) and
after (2025-07-08 19:00:00, weight 0.46) in time
07:29:17 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.343472 (min) 0.115689 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0199443 (min) 0.462393 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.386954 (min) -0.357728 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.93179 (min) -1.66993 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.70457 (min) -5.64498 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 36.8491 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6894 (min) 16.2321 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3317 (min) 32.1671 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00100252 (min) 0.000335887 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:17 DEBUG opendrift:685: No elements hit coastline.
07:29:17 DEBUG opendrift:719: Lifting 47 elements to seafloor.
07:29:17 DEBUG opendrift:1636: No elements to deactivate
07:29:17 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:17 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:17 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:17 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 0
07:29:17 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:17 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03680674643177458
07:29:17 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:17 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:17 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:17 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:17 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(60), np.int64(0), np.int64(1), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:17 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:17 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:17 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:17 DEBUG opendrift:2034: ======================================================================
07:29:17 INFO opendrift:2035: 2025-07-08 18:57:52.765301 - step 72 of 96 - 500 active elements (0 deactivated)
07:29:17 DEBUG opendrift:2041: 0 elements scheduled.
07:29:17 DEBUG opendrift:2043: ======================================================================
07:29:17 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.7684320144618
07:29:17 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.772214748150168
07:29:17 DEBUG opendrift:2054: -25.372790781416754 <- z -> -0.2933139783108101
07:29:17 DEBUG opendrift:2055: ---------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 18:00:00 (before)
2025-07-08 19:00:00 (after)
07:29:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 18:00:00) in space (linearNDFast)
07:29:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 19:00:00) in space (linearNDFast)
07:29:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 18:00:00, weight 0.04) and
after (2025-07-08 19:00:00, weight 0.96) in time
07:29:17 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:17 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:17 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.42782 (min) 0.111151 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0128175 (min) 0.457071 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.404744 (min) -0.375728 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.20594 (min) -2.0826 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.46404 (min) -5.33526 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 42.0687 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6916 (min) 16.2321 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.333 (min) 32.7013 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000278804 (min) 0.000640471 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:17 DEBUG opendrift:685: No elements hit coastline.
07:29:17 DEBUG opendrift:719: Lifting 47 elements to seafloor.
07:29:17 DEBUG opendrift:1636: No elements to deactivate
07:29:17 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:17 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:17 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:17 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:17 DEBUG opendrift.models.chemicaldrift:1476: old species: [2]
07:29:17 DEBUG opendrift.models.chemicaldrift:1477: new species: [0]
07:29:17 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 426. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 25. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:17 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:17 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:17 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03547186979595675
07:29:17 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:17 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:17 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 6 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:17 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:17 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:17 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:17 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:17 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:17 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(61), np.int64(0), np.int64(0), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:17 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:17 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:17 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:17 DEBUG opendrift:2034: ======================================================================
07:29:17 INFO opendrift:2035: 2025-07-08 19:27:52.765301 - step 73 of 96 - 500 active elements (0 deactivated)
07:29:17 DEBUG opendrift:2041: 0 elements scheduled.
07:29:17 DEBUG opendrift:2043: ======================================================================
07:29:17 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.77167201761346
07:29:17 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.772214748150168
07:29:17 DEBUG opendrift:2054: -24.69017219543457 <- z -> -0.3329891356698996
07:29:17 DEBUG opendrift:2055: ---------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 19:00:00 (before)
2025-07-08 20:00:00 (after)
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:19 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:19 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x42x7) for time after (2025-07-08 20:00:00)
07:29:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 19:00:00) in space (linearNDFast)
07:29:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 20:00:00) in space (linearNDFast)
07:29:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 19:00:00, weight 0.54) and
after (2025-07-08 20:00:00, weight 0.46) in time
07:29:19 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:19 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:19 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.466334 (min) 0.128602 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.00449299 (min) 0.421322 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.418068 (min) -0.388119 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.28258 (min) -2.08923 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.94787 (min) -4.99302 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 47.1342 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.6982 (min) 16.2274 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.334 (min) 32.9066 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000702073 (min) 0.00162613 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:19 DEBUG opendrift:685: No elements hit coastline.
07:29:19 DEBUG opendrift:719: Lifting 50 elements to seafloor.
07:29:19 DEBUG opendrift:1636: No elements to deactivate
07:29:19 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:19 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:19 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:19 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:19 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 3 3]
07:29:19 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 0 0]
07:29:19 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 426. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 28. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:19 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:19 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:19 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:19 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:19 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.03025776157861145
07:29:19 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:19 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:19 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:19 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:19 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:19 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:19 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(64), np.int64(0), np.int64(0), np.int64(436), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:19 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:19 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:19 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:19 DEBUG opendrift:2034: ======================================================================
07:29:19 INFO opendrift:2035: 2025-07-08 19:57:52.765301 - step 74 of 96 - 500 active elements (0 deactivated)
07:29:19 DEBUG opendrift:2041: 0 elements scheduled.
07:29:19 DEBUG opendrift:2043: ======================================================================
07:29:19 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.77207897553395
07:29:19 DEBUG opendrift:2054: 10.511721845607001 <- longitude -> 10.772214748150168
07:29:19 DEBUG opendrift:2054: -30.06762481101424 <- z -> -0.20290026010459195
07:29:19 DEBUG opendrift:2055: ---------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 19:00:00 (before)
2025-07-08 20:00:00 (after)
07:29:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 19:00:00) in space (linearNDFast)
07:29:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 20:00:00) in space (linearNDFast)
07:29:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 19:00:00, weight 0.04) and
after (2025-07-08 20:00:00, weight 0.96) in time
07:29:19 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:19 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:19 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.478636 (min) 0.145757 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0170359 (min) 0.425972 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.432945 (min) -0.400083 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.34419 (min) -1.98834 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.43572 (min) -4.37559 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 49.1981 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.3064 (min) 16.2224 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.335 (min) 33.3606 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000561806 (min) 0.00134279 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:19 DEBUG opendrift:685: No elements hit coastline.
07:29:19 DEBUG opendrift:719: Lifting 51 elements to seafloor.
07:29:19 DEBUG opendrift:1636: No elements to deactivate
07:29:19 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:19 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:19 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:19 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:19 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 0]
07:29:19 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 3]
07:29:19 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 429. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 28. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:19 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:19 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:19 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:19 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:19 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.02530309080826179
07:29:19 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:19 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:19 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:19 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:29:19 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:19 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:19 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:19 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:19 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:19 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:19 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(61), np.int64(0), np.int64(0), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:19 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:19 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:19 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:19 DEBUG opendrift:2034: ======================================================================
07:29:19 INFO opendrift:2035: 2025-07-08 20:27:52.765301 - step 75 of 96 - 500 active elements (0 deactivated)
07:29:19 DEBUG opendrift:2041: 0 elements scheduled.
07:29:19 DEBUG opendrift:2043: ======================================================================
07:29:19 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.77223075782479
07:29:19 DEBUG opendrift:2054: 10.511721845607005 <- longitude -> 10.772214748150168
07:29:19 DEBUG opendrift:2054: -25.822361400011854 <- z -> -0.5843891880725
07:29:19 DEBUG opendrift:2055: ---------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 20:00:00 (before)
2025-07-08 21:00:00 (after)
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:21 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:21 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x44x7) for time after (2025-07-08 21:00:00)
07:29:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 20:00:00) in space (linearNDFast)
07:29:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:21 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 21:00:00) in space (linearNDFast)
07:29:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:21 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 20:00:00, weight 0.54) and
after (2025-07-08 21:00:00, weight 0.46) in time
07:29:21 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:21 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:21 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:21 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:21 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.449498 (min) 0.16288 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0588715 (min) 0.392179 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.430458 (min) -0.401858 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.73539 (min) -0.317206 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.97233 (min) -3.85362 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 51.3298 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 14.8852 (min) 16.2128 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3356 (min) 33.5732 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000680209 (min) 0.00124115 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:21 DEBUG opendrift:685: No elements hit coastline.
07:29:21 DEBUG opendrift:719: Lifting 34 elements to seafloor.
07:29:21 DEBUG opendrift:1636: No elements to deactivate
07:29:21 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:21 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:21 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:21 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:21 DEBUG opendrift.models.chemicaldrift:1476: old species: [0]
07:29:21 DEBUG opendrift.models.chemicaldrift:1477: new species: [3]
07:29:21 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 430. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 28. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:21 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:21 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:21 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:21 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:21 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.021472145296265857
07:29:21 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:21 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:21 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:21 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:21 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:21 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:21 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:21 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(60), np.int64(0), np.int64(0), np.int64(440), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:21 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:21 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:21 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:21 DEBUG opendrift:2034: ======================================================================
07:29:21 INFO opendrift:2035: 2025-07-08 20:57:52.765301 - step 76 of 96 - 500 active elements (0 deactivated)
07:29:21 DEBUG opendrift:2041: 0 elements scheduled.
07:29:21 DEBUG opendrift:2043: ======================================================================
07:29:21 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.77310650516157
07:29:21 DEBUG opendrift:2054: 10.51172184560701 <- longitude -> 10.772214748150168
07:29:21 DEBUG opendrift:2054: -24.664377212524414 <- z -> -1.3217278803723311
07:29:21 DEBUG opendrift:2055: ---------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 20:00:00 (before)
2025-07-08 21:00:00 (after)
07:29:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 20:00:00) in space (linearNDFast)
07:29:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:21 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 21:00:00) in space (linearNDFast)
07:29:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:21 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 20:00:00, weight 0.04) and
after (2025-07-08 21:00:00, weight 0.96) in time
07:29:21 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:21 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:21 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:21 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:21 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:21 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:21 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:21 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:21 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.441695 (min) 0.129368 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.00519579 (min) 0.347896 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.426135 (min) -0.402858 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.15165 (min) 1.66828 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.38493 (min) -3.12083 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 54.5062 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.4052 (min) 16.2028 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3361 (min) 33.1322 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00078321 (min) 0.00130914 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:21 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:21 DEBUG opendrift:685: No elements hit coastline.
07:29:21 DEBUG opendrift:719: Lifting 26 elements to seafloor.
07:29:21 DEBUG opendrift:1636: No elements to deactivate
07:29:21 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:21 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:21 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:21 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:21 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:21 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:21 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 431. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 29. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:21 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:21 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:21 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:21 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:21 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.02296924116626294
07:29:21 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:21 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:21 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:21 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 5 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:21 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:21 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:21 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:21 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:21 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(60), np.int64(0), np.int64(0), np.int64(440), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:21 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:21 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:21 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:21 DEBUG opendrift:2034: ======================================================================
07:29:21 INFO opendrift:2035: 2025-07-08 21:27:52.765301 - step 77 of 96 - 500 active elements (0 deactivated)
07:29:21 DEBUG opendrift:2041: 0 elements scheduled.
07:29:21 DEBUG opendrift:2043: ======================================================================
07:29:21 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.773754182653875
07:29:21 DEBUG opendrift:2054: 10.51172184560701 <- longitude -> 10.772214748150168
07:29:21 DEBUG opendrift:2054: -25.9318929267378 <- z -> 0.0
07:29:21 DEBUG opendrift:2055: ---------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:21 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 21:00:00 (before)
2025-07-08 22:00:00 (after)
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:23 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:23 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x45x7) for time after (2025-07-08 22:00:00)
07:29:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 21:00:00) in space (linearNDFast)
07:29:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 22:00:00) in space (linearNDFast)
07:29:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 21:00:00, weight 0.54) and
after (2025-07-08 22:00:00, weight 0.46) in time
07:29:23 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:23 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:23 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:23 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.459036 (min) 0.18532 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.042726 (min) 0.304361 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.407666 (min) -0.384273 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.57748 (min) 1.41868 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.95929 (min) -3.22006 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 57.6611 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 14.7882 (min) 16.1945 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3369 (min) 33.5849 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000591324 (min) 0.0015052 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:23 DEBUG opendrift:685: No elements hit coastline.
07:29:23 DEBUG opendrift:714: No elements hit seafloor.
07:29:23 DEBUG opendrift:1636: No elements to deactivate
07:29:23 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:23 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:23 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:23 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 0
07:29:23 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:23 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.019230857193418695
07:29:23 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:23 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:23 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:23 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:23 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:23 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:23 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(60), np.int64(0), np.int64(0), np.int64(440), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:23 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:23 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:23 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:23 DEBUG opendrift:2034: ======================================================================
07:29:23 INFO opendrift:2035: 2025-07-08 21:57:52.765301 - step 78 of 96 - 500 active elements (0 deactivated)
07:29:23 DEBUG opendrift:2041: 0 elements scheduled.
07:29:23 DEBUG opendrift:2043: ======================================================================
07:29:23 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.77395311420998
07:29:23 DEBUG opendrift:2054: 10.51172184560701 <- longitude -> 10.772214748150168
07:29:23 DEBUG opendrift:2054: -24.6913148139212 <- z -> -0.5022200119110204
07:29:23 DEBUG opendrift:2055: ---------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 21:00:00 (before)
2025-07-08 22:00:00 (after)
07:29:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 21:00:00) in space (linearNDFast)
07:29:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 22:00:00) in space (linearNDFast)
07:29:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 21:00:00, weight 0.04) and
after (2025-07-08 22:00:00, weight 0.96) in time
07:29:23 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:23 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:23 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:23 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:23 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:23 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.470595 (min) 0.158524 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0137675 (min) 0.254036 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.391166 (min) -0.353723 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.92798 (min) 1.00397 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.45247 (min) -2.97317 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 60.572 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.7381 (min) 16.1863 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3376 (min) 32.9347 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000421018 (min) 0.00133337 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:23 DEBUG opendrift:685: No elements hit coastline.
07:29:23 DEBUG opendrift:714: No elements hit seafloor.
07:29:23 DEBUG opendrift:1636: No elements to deactivate
07:29:23 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:23 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:23 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:23 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:23 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 3 3]
07:29:23 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 0 0]
07:29:23 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 431. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 32. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:23 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:23 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:23 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:23 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:23 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.01704652772936564
07:29:23 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:23 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:23 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:23 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:23 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:23 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:23 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:23 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(63), np.int64(0), np.int64(0), np.int64(437), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:23 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:23 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:23 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:23 DEBUG opendrift:2034: ======================================================================
07:29:23 INFO opendrift:2035: 2025-07-08 22:27:52.765301 - step 79 of 96 - 500 active elements (0 deactivated)
07:29:23 DEBUG opendrift:2041: 0 elements scheduled.
07:29:23 DEBUG opendrift:2043: ======================================================================
07:29:23 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.774069584747764
07:29:23 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.772214748150168
07:29:23 DEBUG opendrift:2054: -24.664377212524414 <- z -> -0.2005080087000064
07:29:23 DEBUG opendrift:2055: ---------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:23 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 22:00:00 (before)
2025-07-08 23:00:00 (after)
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:25 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:25 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x47x7) for time after (2025-07-08 23:00:00)
07:29:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 22:00:00) in space (linearNDFast)
07:29:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 23:00:00) in space (linearNDFast)
07:29:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 22:00:00, weight 0.54) and
after (2025-07-08 23:00:00, weight 0.46) in time
07:29:25 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.458616 (min) 0.125753 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0371145 (min) 0.184297 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.348263 (min) -0.306021 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.1473 (min) 0.710639 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.07606 (min) -2.7549 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 63.5998 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5916 (min) 16.1794 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3388 (min) 32.9584 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000530788 (min) 0.00146981 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:25 DEBUG opendrift:685: No elements hit coastline.
07:29:25 DEBUG opendrift:714: No elements hit seafloor.
07:29:25 DEBUG opendrift:1636: No elements to deactivate
07:29:25 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:25 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:25 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:25 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:25 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 3]
07:29:25 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 0]
07:29:25 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 431. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 34. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:25 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:25 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:25 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.012810655492471236
07:29:25 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:25 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:25 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:25 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:25 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:25 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(65), np.int64(0), np.int64(0), np.int64(435), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:25 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:25 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:25 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:25 DEBUG opendrift:2034: ======================================================================
07:29:25 INFO opendrift:2035: 2025-07-08 22:57:52.765301 - step 80 of 96 - 500 active elements (0 deactivated)
07:29:25 DEBUG opendrift:2041: 0 elements scheduled.
07:29:25 DEBUG opendrift:2043: ======================================================================
07:29:25 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77423193291305
07:29:25 DEBUG opendrift:2054: 10.511721845607006 <- longitude -> 10.77221474815017
07:29:25 DEBUG opendrift:2054: -29.066822840729806 <- z -> 0.0
07:29:25 DEBUG opendrift:2055: ---------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 22:00:00 (before)
2025-07-08 23:00:00 (after)
07:29:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 22:00:00) in space (linearNDFast)
07:29:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-08 23:00:00) in space (linearNDFast)
07:29:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 22:00:00, weight 0.04) and
after (2025-07-08 23:00:00, weight 0.96) in time
07:29:25 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:25 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:25 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:25 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.453731 (min) 0.109359 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0546238 (min) 0.161548 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.303579 (min) -0.256059 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.35665 (min) 0.369795 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.0544 (min) -2.42677 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 66.0586 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 15.5992 (min) 16.1725 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.34 (min) 33.1611 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000252389 (min) 0.00169189 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:25 DEBUG opendrift:685: No elements hit coastline.
07:29:25 DEBUG opendrift:714: No elements hit seafloor.
07:29:25 DEBUG opendrift:1636: No elements to deactivate
07:29:25 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:25 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:25 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:25 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:25 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 3]
07:29:25 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 0]
07:29:25 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 432. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 36. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:25 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:25 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:25 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.012100069686167232
07:29:25 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:25 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:25 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:25 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:25 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:25 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:25 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:25 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:25 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:25 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:25 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:25 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:25 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:25 DEBUG opendrift:2034: ======================================================================
07:29:25 INFO opendrift:2035: 2025-07-08 23:27:52.765301 - step 81 of 96 - 500 active elements (0 deactivated)
07:29:25 DEBUG opendrift:2041: 0 elements scheduled.
07:29:25 DEBUG opendrift:2043: ======================================================================
07:29:25 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77443382521116
07:29:25 DEBUG opendrift:2054: 10.51172184560701 <- longitude -> 10.77221474815017
07:29:25 DEBUG opendrift:2054: -27.577979727680365 <- z -> -0.07429310084928178
07:29:25 DEBUG opendrift:2055: ---------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:25 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 23:00:00 (before)
2025-07-09 00:00:00 (after)
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:28 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:28 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x49x7) for time after (2025-07-09 00:00:00)
07:29:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 23:00:00) in space (linearNDFast)
07:29:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 00:00:00) in space (linearNDFast)
07:29:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 23:00:00, weight 0.54) and
after (2025-07-09 00:00:00, weight 0.46) in time
07:29:28 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:28 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:28 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.48828924298985 and -59.22779629352177 degrees.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:28 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:28 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.453777 (min) 0.0765044 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0724826 (min) 0.137694 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.24647 (min) -0.2018 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.391347 (min) 0.826966 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.596 (min) -2.12516 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 68.013 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 14.7434 (min) 16.1645 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.342 (min) 33.6044 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -6.70136e-05 (min) 0.00109357 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:28 DEBUG opendrift:685: No elements hit coastline.
07:29:28 DEBUG opendrift:714: No elements hit seafloor.
07:29:28 DEBUG opendrift:1636: No elements to deactivate
07:29:28 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:28 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:28 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:28 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:28 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:28 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:28 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 433. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 37. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:28 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:28 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:28 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:28 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:28 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005277956712477381
07:29:28 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:28 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:28 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:28 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:28 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:28 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:28 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:28 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:28 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:28 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:28 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:28 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:28 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:28 DEBUG opendrift:2034: ======================================================================
07:29:28 INFO opendrift:2035: 2025-07-08 23:57:52.765301 - step 82 of 96 - 500 active elements (0 deactivated)
07:29:28 DEBUG opendrift:2041: 0 elements scheduled.
07:29:28 DEBUG opendrift:2043: ======================================================================
07:29:28 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77509244791574
07:29:28 DEBUG opendrift:2054: 10.502770067158107 <- longitude -> 10.772214748150173
07:29:28 DEBUG opendrift:2054: -27.5347202833454 <- z -> -0.02913436135642522
07:29:28 DEBUG opendrift:2055: ---------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-08 23:00:00 (before)
2025-07-09 00:00:00 (after)
07:29:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-08 23:00:00) in space (linearNDFast)
07:29:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 00:00:00) in space (linearNDFast)
07:29:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-08 23:00:00, weight 0.04) and
after (2025-07-09 00:00:00, weight 0.96) in time
07:29:28 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:28 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.49724095962218 and -59.22779629352177 degrees.
07:29:28 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.49724095962218 and -59.22779629352177 degrees.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:28 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:28 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:28 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:28 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:28 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:28 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.452241 (min) 0.07193 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0877974 (min) 0.115241 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.188945 (min) -0.148014 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.569588 (min) 1.67439 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.26372 (min) -0.812688 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 69.8946 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 14.1657 (min) 16.1563 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3441 (min) 33.7917 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -1.75128e-05 (min) 0.00137229 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:28 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:28 DEBUG opendrift:685: No elements hit coastline.
07:29:28 DEBUG opendrift:714: No elements hit seafloor.
07:29:28 DEBUG opendrift:1636: No elements to deactivate
07:29:28 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:28 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:28 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:28 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:28 DEBUG opendrift.models.chemicaldrift:1476: old species: [0]
07:29:28 DEBUG opendrift.models.chemicaldrift:1477: new species: [3]
07:29:28 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 434. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 37. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:28 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:28 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:28 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:28 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:28 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.005306783781954776
07:29:28 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:28 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:28 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:28 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:28 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:28 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:28 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:28 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:28 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:28 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(65), np.int64(0), np.int64(0), np.int64(435), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:28 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:28 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:28 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:28 DEBUG opendrift:2034: ======================================================================
07:29:28 INFO opendrift:2035: 2025-07-09 00:27:52.765301 - step 83 of 96 - 500 active elements (0 deactivated)
07:29:28 DEBUG opendrift:2041: 0 elements scheduled.
07:29:28 DEBUG opendrift:2043: ======================================================================
07:29:28 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77551762927487
07:29:28 DEBUG opendrift:2054: 10.489892554455501 <- longitude -> 10.772214748150173
07:29:28 DEBUG opendrift:2054: -24.664377212524414 <- z -> -0.3799716998700726
07:29:28 DEBUG opendrift:2055: ---------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:28 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 00:00:00 (before)
2025-07-09 01:00:00 (after)
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:30 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:30 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x50x7) for time after (2025-07-09 01:00:00)
07:29:30 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 00:00:00) in space (linearNDFast)
07:29:30 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:30 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 01:00:00) in space (linearNDFast)
07:29:30 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:30 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 00:00:00, weight 0.54) and
after (2025-07-09 01:00:00, weight 0.46) in time
07:29:30 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:30 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.510118477245484 and -59.22779629352177 degrees.
07:29:30 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.510118477245484 and -59.22779629352177 degrees.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:30 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:30 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:30 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.495325 (min) 0.0573567 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.10715 (min) 0.0966585 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.134508 (min) -0.107308 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.388071 (min) 1.72356 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.32886 (min) -0.880251 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 71.6667 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 14.2746 (min) 16.1511 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3464 (min) 33.5693 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -1.69302e-05 (min) 0.000969432 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:30 DEBUG opendrift:685: No elements hit coastline.
07:29:30 DEBUG opendrift:714: No elements hit seafloor.
07:29:30 DEBUG opendrift:1636: No elements to deactivate
07:29:30 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:30 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:30 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:30 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 3
07:29:30 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 3 0]
07:29:30 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 0 3]
07:29:30 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 435. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 39. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:30 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:30 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:30 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:30 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:30 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.0049139012133922605
07:29:30 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:30 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:30 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:30 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:30 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:30 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:30 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:30 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:30 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:30 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:30 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:30 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:30 DEBUG opendrift:2034: ======================================================================
07:29:30 INFO opendrift:2035: 2025-07-09 00:57:52.765301 - step 84 of 96 - 500 active elements (0 deactivated)
07:29:30 DEBUG opendrift:2041: 0 elements scheduled.
07:29:30 DEBUG opendrift:2043: ======================================================================
07:29:30 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77579600655574
07:29:30 DEBUG opendrift:2054: 10.476176637446985 <- longitude -> 10.77221474815017
07:29:30 DEBUG opendrift:2054: -28.461433422741095 <- z -> -0.3390807890733144
07:29:30 DEBUG opendrift:2055: ---------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 00:00:00 (before)
2025-07-09 01:00:00 (after)
07:29:30 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 00:00:00) in space (linearNDFast)
07:29:30 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:30 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 01:00:00) in space (linearNDFast)
07:29:30 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:30 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 00:00:00, weight 0.04) and
after (2025-07-09 01:00:00, weight 0.96) in time
07:29:30 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:30 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.5238344039454 and -59.22779629352177 degrees.
07:29:30 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.5238344039454 and -59.22779629352177 degrees.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:30 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:30 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:30 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:30 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:30 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:30 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:30 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:30 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.483143 (min) 0.0424852 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.143938 (min) 0.0781228 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.101724 (min) -0.0669607 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.0388588 (min) 1.71012 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.42455 (min) -0.703977 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 73.4154 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.7818 (min) 16.1461 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3488 (min) 33.8974 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -2.61547e-05 (min) 0.00102667 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:30 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:30 DEBUG opendrift:685: No elements hit coastline.
07:29:30 DEBUG opendrift:714: No elements hit seafloor.
07:29:30 DEBUG opendrift:1636: No elements to deactivate
07:29:30 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:30 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:30 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:30 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 0
07:29:30 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:30 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.00481949147965113
07:29:30 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:30 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:30 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:30 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:30 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:30 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:30 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:30 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:30 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:30 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:30 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:30 DEBUG opendrift:2034: ======================================================================
07:29:30 INFO opendrift:2035: 2025-07-09 01:27:52.765301 - step 85 of 96 - 500 active elements (0 deactivated)
07:29:30 DEBUG opendrift:2041: 0 elements scheduled.
07:29:30 DEBUG opendrift:2043: ======================================================================
07:29:30 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77614507862908
07:29:30 DEBUG opendrift:2054: 10.463282032202295 <- longitude -> 10.77221474815017
07:29:30 DEBUG opendrift:2054: -26.631867923493843 <- z -> -0.7662424635974736
07:29:30 DEBUG opendrift:2055: ---------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:30 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:30 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:30 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:30 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 01:00:00 (before)
2025-07-09 02:00:00 (after)
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:32 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:32 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x52x7) for time after (2025-07-09 02:00:00)
07:29:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 01:00:00) in space (linearNDFast)
07:29:32 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:32 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 02:00:00) in space (linearNDFast)
07:29:32 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:32 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 01:00:00, weight 0.54) and
after (2025-07-09 02:00:00, weight 0.46) in time
07:29:32 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:32 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.536729011034545 and -59.22779629352177 degrees.
07:29:32 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.536729011034545 and -59.22779629352177 degrees.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:32 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:32 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:32 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.534407 (min) 0.0204212 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.153788 (min) 0.0806659 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0997822 (min) -0.0471514 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.514047 (min) 1.44752 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.20377 (min) -0.418117 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 74.6462 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.8015 (min) 16.1381 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3518 (min) 33.8893 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -3.44592e-05 (min) 0.000846837 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:32 DEBUG opendrift:685: No elements hit coastline.
07:29:32 DEBUG opendrift:714: No elements hit seafloor.
07:29:32 DEBUG opendrift:1636: No elements to deactivate
07:29:32 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:32 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:32 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:32 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:29:32 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 0 3]
07:29:32 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 3 0]
07:29:32 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 437. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 41. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:32 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:32 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:32 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:32 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:32 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.003531582066772051
07:29:32 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:32 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:32 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:32 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:32 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:32 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:32 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:32 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:32 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:32 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:32 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:32 DEBUG opendrift:2034: ======================================================================
07:29:32 INFO opendrift:2035: 2025-07-09 01:57:52.765301 - step 86 of 96 - 500 active elements (0 deactivated)
07:29:32 DEBUG opendrift:2041: 0 elements scheduled.
07:29:32 DEBUG opendrift:2043: ======================================================================
07:29:32 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77669800490229
07:29:32 DEBUG opendrift:2054: 10.450659008066673 <- longitude -> 10.772214748150168
07:29:32 DEBUG opendrift:2054: -27.289805044446478 <- z -> -0.30341727412423874
07:29:32 DEBUG opendrift:2055: ---------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 01:00:00 (before)
2025-07-09 02:00:00 (after)
07:29:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 01:00:00) in space (linearNDFast)
07:29:32 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:32 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 02:00:00) in space (linearNDFast)
07:29:32 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:32 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 01:00:00, weight 0.04) and
after (2025-07-09 02:00:00, weight 0.96) in time
07:29:32 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:32 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.549352032743855 and -59.22779629352177 degrees.
07:29:32 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.549352032743855 and -59.22779629352177 degrees.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:32 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:32 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:32 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:32 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:32 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:32 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:32 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:32 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.509447 (min) 0.0178645 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.161757 (min) 0.113392 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.0998551 (min) -0.0253309 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.20912 (min) 1.16596 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: y_wind: -1.97163 (min) -0.114574 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 75.3218 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.7645 (min) 16.13 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3547 (min) 33.8963 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -4.26937e-05 (min) 0.00074202 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:32 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:32 DEBUG opendrift:685: No elements hit coastline.
07:29:32 DEBUG opendrift:714: No elements hit seafloor.
07:29:32 DEBUG opendrift:1636: No elements to deactivate
07:29:32 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:32 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:32 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:32 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:32 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:32 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:32 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 438. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 42. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:32 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:32 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:32 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:32 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:32 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.003099537234561327
07:29:32 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:32 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:32 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:32 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:32 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:32 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:32 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:32 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:32 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:32 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:32 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:32 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:32 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:32 DEBUG opendrift:2034: ======================================================================
07:29:32 INFO opendrift:2035: 2025-07-09 02:27:52.765301 - step 87 of 96 - 500 active elements (0 deactivated)
07:29:32 DEBUG opendrift:2041: 0 elements scheduled.
07:29:32 DEBUG opendrift:2043: ======================================================================
07:29:32 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77742832537007
07:29:32 DEBUG opendrift:2054: 10.43809082512225 <- longitude -> 10.772214748150171
07:29:32 DEBUG opendrift:2054: -28.2402460586089 <- z -> -0.3117441190095559
07:29:32 DEBUG opendrift:2055: ---------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:32 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:32 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:32 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:32 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 02:00:00 (before)
2025-07-09 03:00:00 (after)
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:34 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:34 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x54x7) for time after (2025-07-09 03:00:00)
07:29:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 02:00:00) in space (linearNDFast)
07:29:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 03:00:00) in space (linearNDFast)
07:29:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 02:00:00, weight 0.54) and
after (2025-07-09 03:00:00, weight 0.46) in time
07:29:34 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:34 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.561920218603255 and -59.22779629352177 degrees.
07:29:34 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.561920218603255 and -59.22779629352177 degrees.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:34 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:34 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:34 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.507039 (min) 0.0250952 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.166553 (min) 0.187101 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.1232 (min) -0.0421505 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.69813 (min) 0.66757 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.974748 (min) 0.668412 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 75.8457 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.4958 (min) 16.1247 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.357 (min) 33.9448 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000301983 (min) 0.000578149 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:34 DEBUG opendrift:685: No elements hit coastline.
07:29:34 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:34 DEBUG opendrift:1636: No elements to deactivate
07:29:34 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:34 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:34 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:34 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:29:34 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 3 3 0]
07:29:34 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 0 0 3]
07:29:34 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 440. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 44. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:34 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:34 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:34 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:34 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:34 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.002539201087018863
07:29:34 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:34 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:34 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:34 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:34 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:34 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:34 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:34 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:34 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:34 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(66), np.int64(0), np.int64(0), np.int64(434), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:34 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:34 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:34 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:34 DEBUG opendrift:2034: ======================================================================
07:29:34 INFO opendrift:2035: 2025-07-09 02:57:52.765301 - step 88 of 96 - 500 active elements (0 deactivated)
07:29:34 DEBUG opendrift:2041: 0 elements scheduled.
07:29:34 DEBUG opendrift:2043: ======================================================================
07:29:34 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77814945825255
07:29:34 DEBUG opendrift:2054: 10.425597955674167 <- longitude -> 10.772214748150171
07:29:34 DEBUG opendrift:2054: -28.798489745407036 <- z -> -0.17116885690537986
07:29:34 DEBUG opendrift:2055: ---------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:34 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 02:00:00 (before)
2025-07-09 03:00:00 (after)
07:29:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 02:00:00) in space (linearNDFast)
07:29:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 03:00:00) in space (linearNDFast)
07:29:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 02:00:00, weight 0.04) and
after (2025-07-09 03:00:00, weight 0.96) in time
07:29:34 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:34 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.57441309110491 and -59.22779629352177 degrees.
07:29:34 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.57441309110491 and -59.22779629352177 degrees.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:34 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:34 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:34 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:34 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:34 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.501583 (min) 0.0700284 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.174565 (min) 0.295211 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.147922 (min) -0.0619091 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.85884 (min) 0.152682 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.666122 (min) 1.46734 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 75.7067 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.353 (min) 16.1197 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3592 (min) 33.9825 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000604314 (min) 0.000555569 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:34 DEBUG opendrift:685: No elements hit coastline.
07:29:34 DEBUG opendrift:719: Lifting 5 elements to seafloor.
07:29:35 DEBUG opendrift:1636: No elements to deactivate
07:29:35 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:35 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:35 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:35 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:35 DEBUG opendrift.models.chemicaldrift:1476: old species: [0]
07:29:35 DEBUG opendrift.models.chemicaldrift:1477: new species: [3]
07:29:35 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 441. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 44. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:35 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:35 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:35 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.006045704158891426
07:29:35 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:35 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:35 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:35 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:35 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:35 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:35 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:35 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:35 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:35 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(65), np.int64(0), np.int64(0), np.int64(435), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:35 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:35 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:35 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:35 DEBUG opendrift:2034: ======================================================================
07:29:35 INFO opendrift:2035: 2025-07-09 03:27:52.765301 - step 89 of 96 - 500 active elements (0 deactivated)
07:29:35 DEBUG opendrift:2041: 0 elements scheduled.
07:29:35 DEBUG opendrift:2043: ======================================================================
07:29:35 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77879306244178
07:29:35 DEBUG opendrift:2054: 10.413745476010742 <- longitude -> 10.772214748150171
07:29:35 DEBUG opendrift:2054: -31.28427435592696 <- z -> -0.4768751610636851
07:29:35 DEBUG opendrift:2055: ---------------------------------
07:29:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:35 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 03:00:00 (before)
2025-07-09 04:00:00 (after)
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:37 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:37 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 49x55x7) for time after (2025-07-09 04:00:00)
07:29:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 03:00:00) in space (linearNDFast)
07:29:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 04:00:00) in space (linearNDFast)
07:29:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 03:00:00, weight 0.54) and
after (2025-07-09 04:00:00, weight 0.46) in time
07:29:37 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.58626557092583 and -59.22779629352177 degrees.
07:29:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.58626557092583 and -59.22779629352177 degrees.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.541748 (min) 0.122965 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.169459 (min) 0.384908 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.182272 (min) -0.102072 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.35885 (min) 0.528181 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.412279 (min) 1.82768 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 75.9238 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.3307 (min) 16.1147 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3609 (min) 33.9977 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000554336 (min) 0.000515869 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:37 DEBUG opendrift:685: No elements hit coastline.
07:29:37 DEBUG opendrift:719: Lifting 7 elements to seafloor.
07:29:37 DEBUG opendrift:1636: No elements to deactivate
07:29:37 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:37 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:37 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:37 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:37 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0]
07:29:37 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3]
07:29:37 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 443. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 44. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:37 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:37 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:37 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004474700364141171
07:29:37 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:37 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:37 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:37 DEBUG opendrift.models.oceandrift:548: 2 elements reached seafloor, interacting with bottom
07:29:37 DEBUG opendrift:719: Lifting 2 elements to seafloor.
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:37 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:37 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(63), np.int64(0), np.int64(0), np.int64(437), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:37 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:37 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:37 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:37 DEBUG opendrift:2034: ======================================================================
07:29:37 INFO opendrift:2035: 2025-07-09 03:57:52.765301 - step 90 of 96 - 500 active elements (0 deactivated)
07:29:37 DEBUG opendrift:2041: 0 elements scheduled.
07:29:37 DEBUG opendrift:2043: ======================================================================
07:29:37 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77918080481406
07:29:37 DEBUG opendrift:2054: 10.402671714044487 <- longitude -> 10.772214748150171
07:29:37 DEBUG opendrift:2054: -29.717339959436057 <- z -> -0.4704738763134345
07:29:37 DEBUG opendrift:2055: ---------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 03:00:00 (before)
2025-07-09 04:00:00 (after)
07:29:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 03:00:00) in space (linearNDFast)
07:29:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 04:00:00) in space (linearNDFast)
07:29:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 03:00:00, weight 0.04) and
after (2025-07-09 04:00:00, weight 0.96) in time
07:29:37 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.59733933203792 and -59.22779629352177 degrees.
07:29:37 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.59733933203792 and -59.22779629352177 degrees.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:37 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:37 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.542831 (min) 0.184233 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.196224 (min) 0.363259 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.217189 (min) -0.142969 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.92052 (min) 0.971405 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.156467 (min) 2.12022 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 76.4322 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 13.1849 (min) 16.1097 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3626 (min) 34.0445 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000723364 (min) 0.000609977 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:37 DEBUG opendrift:685: No elements hit coastline.
07:29:37 DEBUG opendrift:719: Lifting 9 elements to seafloor.
07:29:37 DEBUG opendrift:1636: No elements to deactivate
07:29:37 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:37 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:37 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:37 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:37 DEBUG opendrift.models.chemicaldrift:1476: old species: [3]
07:29:37 DEBUG opendrift.models.chemicaldrift:1477: new species: [0]
07:29:37 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 443. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 45. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:37 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:37 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:37 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004866273132908688
07:29:37 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:37 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:37 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:37 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:37 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:37 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:37 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:37 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(64), np.int64(0), np.int64(0), np.int64(436), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:37 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:37 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:37 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:37 DEBUG opendrift:2034: ======================================================================
07:29:37 INFO opendrift:2035: 2025-07-09 04:27:52.765301 - step 91 of 96 - 500 active elements (0 deactivated)
07:29:37 DEBUG opendrift:2041: 0 elements scheduled.
07:29:37 DEBUG opendrift:2043: ======================================================================
07:29:37 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77922266328193
07:29:37 DEBUG opendrift:2054: 10.392967537623782 <- longitude -> 10.77221474815017
07:29:37 DEBUG opendrift:2054: -28.28702700056075 <- z -> -0.30334529325382076
07:29:37 DEBUG opendrift:2055: ---------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:37 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 04:00:00 (before)
2025-07-09 05:00:00 (after)
07:29:38 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:38 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:38 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:38 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:38 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:38 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:38 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x56x7) for time after (2025-07-09 05:00:00)
07:29:38 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 04:00:00) in space (linearNDFast)
07:29:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:38 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 05:00:00) in space (linearNDFast)
07:29:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:38 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 04:00:00, weight 0.54) and
after (2025-07-09 05:00:00, weight 0.46) in time
07:29:38 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.60704350953825 and -59.22779629352177 degrees.
07:29:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.60704350953825 and -59.22779629352177 degrees.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:39 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.570445 (min) 0.257178 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.197703 (min) 0.373578 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.255857 (min) -0.192837 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.17836 (min) 0.911763 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.348127 (min) 2.31539 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 76.667 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 12.7657 (min) 16.1094 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3634 (min) 34.1392 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000532315 (min) 0.000713479 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:39 DEBUG opendrift:685: No elements hit coastline.
07:29:39 DEBUG opendrift:719: Lifting 10 elements to seafloor.
07:29:39 DEBUG opendrift:1636: No elements to deactivate
07:29:39 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:39 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:39 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:39 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:39 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:39 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:39 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 444. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 46. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:39 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:39 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:39 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.004987002869875398
07:29:39 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:39 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:39 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:39 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:39 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:39 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(64), np.int64(0), np.int64(0), np.int64(436), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:39 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:39 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:39 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:39 DEBUG opendrift:2034: ======================================================================
07:29:39 INFO opendrift:2035: 2025-07-09 04:57:52.765301 - step 92 of 96 - 500 active elements (0 deactivated)
07:29:39 DEBUG opendrift:2041: 0 elements scheduled.
07:29:39 DEBUG opendrift:2043: ======================================================================
07:29:39 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77892872921456
07:29:39 DEBUG opendrift:2054: 10.381162236612287 <- longitude -> 10.77221474815017
07:29:39 DEBUG opendrift:2054: -26.559681310114286 <- z -> -0.3417981655874549
07:29:39 DEBUG opendrift:2055: ---------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 04:00:00 (before)
2025-07-09 05:00:00 (after)
07:29:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 04:00:00) in space (linearNDFast)
07:29:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 05:00:00) in space (linearNDFast)
07:29:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 04:00:00, weight 0.04) and
after (2025-07-09 05:00:00, weight 0.96) in time
07:29:39 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.618848811780765 and -59.227796300126535 degrees.
07:29:39 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.618848811780765 and -59.227796300126535 degrees.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:39 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:39 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.575263 (min) 0.241171 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.19854 (min) 0.400714 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.294412 (min) -0.241474 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.54057 (min) 0.825052 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.871646 (min) 2.55109 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 78.0433 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 12.2962 (min) 16.1094 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3642 (min) 34.2429 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000588657 (min) 0.000979046 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:39 DEBUG opendrift:685: No elements hit coastline.
07:29:39 DEBUG opendrift:719: Lifting 12 elements to seafloor.
07:29:39 DEBUG opendrift:1636: No elements to deactivate
07:29:39 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:39 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:39 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:39 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 4
07:29:39 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0 0 0]
07:29:39 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3 3 3]
07:29:39 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 447. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 47. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:39 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:39 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:39 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.007012754766834224
07:29:39 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:39 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:39 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:39 DEBUG opendrift.models.oceandrift:548: 3 elements reached seafloor, interacting with bottom
07:29:39 DEBUG opendrift:719: Lifting 3 elements to seafloor.
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:39 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:39 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:39 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:39 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:39 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(62), np.int64(0), np.int64(0), np.int64(438), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:39 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:39 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:39 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:39 DEBUG opendrift:2034: ======================================================================
07:29:39 INFO opendrift:2035: 2025-07-09 05:27:52.765301 - step 93 of 96 - 500 active elements (0 deactivated)
07:29:39 DEBUG opendrift:2041: 0 elements scheduled.
07:29:39 DEBUG opendrift:2043: ======================================================================
07:29:39 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.77954647965144
07:29:39 DEBUG opendrift:2054: 10.370286511067567 <- longitude -> 10.77221474815017
07:29:39 DEBUG opendrift:2054: -27.571363041300057 <- z -> -0.3744591444857309
07:29:39 DEBUG opendrift:2055: ---------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:39 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 05:00:00 (before)
2025-07-09 06:00:00 (after)
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:41 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:41 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x57x7) for time after (2025-07-09 06:00:00)
07:29:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 05:00:00) in space (linearNDFast)
07:29:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:41 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 06:00:00) in space (linearNDFast)
07:29:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
07:29:41 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 05:00:00, weight 0.54) and
after (2025-07-09 06:00:00, weight 0.46) in time
07:29:41 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.629724541446315 and -59.227796300126535 degrees.
07:29:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.629724541446315 and -59.227796300126535 degrees.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:41 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.570189 (min) 0.138644 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.195472 (min) 0.49351 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.33154 (min) -0.282997 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.12908 (min) 0.896325 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.33057 (min) 2.46278 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 80.4171 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 11.9076 (min) 16.1169 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3647 (min) 34.3273 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000482278 (min) 0.00128405 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:41 DEBUG opendrift:685: No elements hit coastline.
07:29:41 DEBUG opendrift:719: Lifting 16 elements to seafloor.
07:29:41 DEBUG opendrift:1636: No elements to deactivate
07:29:41 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:41 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:41 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:41 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 1
07:29:41 DEBUG opendrift.models.chemicaldrift:1476: old species: [0]
07:29:41 DEBUG opendrift.models.chemicaldrift:1477: new species: [3]
07:29:41 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 448. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 47. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:41 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:41 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:41 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.006789466999827578
07:29:41 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:41 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:41 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:41 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:41 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:41 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:41 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(61), np.int64(0), np.int64(0), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:41 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:41 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:41 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:41 DEBUG opendrift:2034: ======================================================================
07:29:41 INFO opendrift:2035: 2025-07-09 05:57:52.765301 - step 94 of 96 - 500 active elements (0 deactivated)
07:29:41 DEBUG opendrift:2041: 0 elements scheduled.
07:29:41 DEBUG opendrift:2043: ======================================================================
07:29:41 DEBUG opendrift:2054: 57.576187818482204 <- latitude -> 57.780665924854134
07:29:41 DEBUG opendrift:2054: 10.360405899568166 <- longitude -> 10.77221474815017
07:29:41 DEBUG opendrift:2054: -26.786027589651283 <- z -> -0.25159057665762463
07:29:41 DEBUG opendrift:2055: ---------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 05:00:00 (before)
2025-07-09 06:00:00 (after)
07:29:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 05:00:00) in space (linearNDFast)
07:29:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:41 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 06:00:00) in space (linearNDFast)
07:29:41 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:41 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 05:00:00, weight 0.04) and
after (2025-07-09 06:00:00, weight 0.96) in time
07:29:41 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.639605160632584 and -59.22779629660365 degrees.
07:29:41 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.639605160632584 and -59.22779629660365 degrees.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:41 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:41 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:41 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:41 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:41 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:41 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.565725 (min) 0.145779 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.174523 (min) 0.481844 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.368867 (min) -0.323978 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.77126 (min) 2.44081 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.61014 (min) 2.87761 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 82.2185 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 12.2532 (min) 16.1249 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3652 (min) 34.2207 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000940299 (min) 0.0015275 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:41 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:41 DEBUG opendrift:685: No elements hit coastline.
07:29:41 DEBUG opendrift:719: Lifting 17 elements to seafloor.
07:29:41 DEBUG opendrift:1636: No elements to deactivate
07:29:41 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:41 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:41 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:41 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:41 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:41 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:41 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 449. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 48. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:41 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:41 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:41 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.008180818601738936
07:29:41 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:41 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:41 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:41 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:41 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:41 DEBUG opendrift.models.oceandrift:528: 4 elements penetrated seafloor, lifting up
07:29:41 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:41 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:41 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:41 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:41 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(61), np.int64(0), np.int64(0), np.int64(439), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:41 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:41 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:41 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:41 DEBUG opendrift:2034: ======================================================================
07:29:41 INFO opendrift:2035: 2025-07-09 06:27:52.765301 - step 95 of 96 - 500 active elements (0 deactivated)
07:29:41 DEBUG opendrift:2041: 0 elements scheduled.
07:29:41 DEBUG opendrift:2043: ======================================================================
07:29:41 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.78158343090836
07:29:41 DEBUG opendrift:2054: 10.347317028150538 <- longitude -> 10.77221474815017
07:29:41 DEBUG opendrift:2054: -27.50693241868163 <- z -> -0.7767397933771045
07:29:41 DEBUG opendrift:2055: ---------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:41 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:41 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:41 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:41 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 06:00:00 (before)
2025-07-09 07:00:00 (after)
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking sea_floor_depth_below_sea_level for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking x_wind for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking y_wind for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
07:29:43 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'sea_water_temperature', 'upward_sea_water_velocity', 'sea_water_salinity', 'y_sea_water_velocity']
07:29:43 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 50x58x7) for time after (2025-07-09 07:00:00)
07:29:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 06:00:00) in space (linearNDFast)
07:29:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 07:00:00) in space (linearNDFast)
07:29:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 06:00:00, weight 0.54) and
after (2025-07-09 07:00:00, weight 0.46) in time
07:29:43 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:43 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.652694023342036 and -59.22779629660365 degrees.
07:29:43 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.652694023342036 and -59.22779629660365 degrees.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:43 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:43 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.537935 (min) 0.14692 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.179111 (min) 0.398925 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.401473 (min) -0.355676 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.46494 (min) 3.02928 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.7755 (min) 3.04121 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 83.475 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 11.6932 (min) 16.1412 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3654 (min) 34.3523 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.000651805 (min) 0.0013593 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:43 DEBUG opendrift:685: No elements hit coastline.
07:29:43 DEBUG opendrift:719: Lifting 18 elements to seafloor.
07:29:43 DEBUG opendrift:1636: No elements to deactivate
07:29:43 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:43 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:43 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:43 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 5
07:29:43 DEBUG opendrift.models.chemicaldrift:1476: old species: [0 0 3 0 0]
07:29:43 DEBUG opendrift.models.chemicaldrift:1477: new species: [3 3 0 3 3]
07:29:43 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 453. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 49. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:43 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:43 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:43 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:43 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:43 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.009138988343416723
07:29:43 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:43 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:43 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:548: 4 elements reached seafloor, interacting with bottom
07:29:43 DEBUG opendrift:719: Lifting 4 elements to seafloor.
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:43 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:43 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:43 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:43 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:43 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(58), np.int64(0), np.int64(0), np.int64(442), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:43 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:43 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:43 DEBUG opendrift:856: to be seeded: 0, already seeded 500
07:29:43 DEBUG opendrift:2034: ======================================================================
07:29:43 INFO opendrift:2035: 2025-07-09 06:57:52.765301 - step 96 of 96 - 500 active elements (0 deactivated)
07:29:43 DEBUG opendrift:2041: 0 elements scheduled.
07:29:43 DEBUG opendrift:2043: ======================================================================
07:29:43 DEBUG opendrift:2054: 57.5761878184822 <- latitude -> 57.78241887707213
07:29:43 DEBUG opendrift:2054: 10.334042543170392 <- longitude -> 10.772214748150168
07:29:43 DEBUG opendrift:2054: -27.830822176921778 <- z -> -0.4160965985262335
07:29:43 DEBUG opendrift:2055: ---------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-07-09 06:00:00 (before)
2025-07-09 07:00:00 (after)
07:29:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-07-09 06:00:00) in space (linearNDFast)
07:29:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-07-09 07:00:00) in space (linearNDFast)
07:29:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
07:29:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-07-09 06:00:00, weight 0.04) and
after (2025-07-09 07:00:00, weight 0.96) in time
07:29:43 DEBUG opendrift.readers.basereader.structured:370: Interpolating profiles in time
07:29:43 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.665968526926946 and -59.22779629660365 degrees.
07:29:43 DEBUG opendrift.readers.basereader.variables:100: Rotating vectors between -59.665968526926946 and -59.22779629660365 degrees.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from constant_reader covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:43 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 10 for sea_water_temperature for 0 profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:798: Using fallback value 34 for sea_water_salinity for 0 profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
07:29:43 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:43 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.55528 (min) 0.159536 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.198175 (min) 0.358928 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: -0.433918 (min) -0.386667 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.2234 (min) 3.49039 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.56022 (min) 3.27511 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 9.44687 (min) 84.1184 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 12.3835 (min) 16.1582 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 31.3657 (min) 34.2318 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: -0.00073181 (min) 0.00216883 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: spm: 1 (min) 1 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 40 (min) 40 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: doc: 0 (min) 0 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:889: pH_sediment: 6.9 (min) 6.9 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:43 DEBUG opendrift:685: No elements hit coastline.
07:29:43 DEBUG opendrift:719: Lifting 22 elements to seafloor.
07:29:43 DEBUG opendrift:1636: No elements to deactivate
07:29:43 DEBUG opendrift:2089: Calling ChemicalDrift.update()
07:29:43 DEBUG opendrift.models.chemicaldrift:1691: Calculating overall degradation using overall rate constants
07:29:43 DEBUG opendrift.models.chemicaldrift:1748: Calculating: volatilization
07:29:43 INFO opendrift.models.chemicaldrift:1459: Number of transformations: 2
07:29:43 DEBUG opendrift.models.chemicaldrift:1476: old species: [3 0]
07:29:43 DEBUG opendrift.models.chemicaldrift:1477: new species: [0 3]
07:29:43 DEBUG opendrift.models.chemicaldrift:1484: Number of transformations total:
[[ 0. 0. 0. 454. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 38. 0.]
[ 50. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
07:29:43 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:43 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:43 DEBUG opendrift.models.chemicaldrift:1528: Adding uncertainty for desorption from sediments: 0.5 m
07:29:43 DEBUG opendrift.models.oceandrift:440: Using functional expression for diffusivity
07:29:43 DEBUG opendrift.models.oceandrift:445: Diffusivities are in range 0.0 to 0.01132835930102034
07:29:43 DEBUG opendrift.models.oceandrift:464: TSprofiles deactivated for vertical mixing
07:29:43 DEBUG opendrift.models.oceandrift:478: Vertical mixing module:windspeed_Large1994
07:29:43 DEBUG opendrift.models.oceandrift:481: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
07:29:43 DEBUG opendrift.models.oceandrift:548: 1 elements reached seafloor, interacting with bottom
07:29:43 DEBUG opendrift:719: Lifting 1 elements to seafloor.
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 3 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 2 elements penetrated seafloor, lifting up
07:29:43 DEBUG opendrift.models.oceandrift:528: 1 elements penetrated seafloor, lifting up
07:29:43 INFO opendrift.models.chemicaldrift:1657: Number of resuspended particles: 0
07:29:43 DEBUG opendrift.models.chemicaldrift:1662: Adding uncertainty for resuspension from sediments: 0.5 m
07:29:43 DEBUG opendrift.models.chemicaldrift:1563: Updated particle diameter for 0 elements
07:29:43 DEBUG opendrift.models.chemicaldrift:1567: Adding uncertainty for particle diameter: 5e-06 m
07:29:43 INFO opendrift.models.chemicaldrift:1887: partitioning: [np.int64(58), np.int64(0), np.int64(0), np.int64(442), np.int64(0)] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
07:29:43 DEBUG opendrift.models.oceandrift:321: Vertical advection, including elements at surface
07:29:43 DEBUG opendrift:1587: Horizontal diffusivity is 0, no random walk.
07:29:43 DEBUG opendrift:2127: Cleaning up
07:29:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
07:29:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
07:29:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 500 elements
07:29:43 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 500 elements
07:29:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
07:29:43 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
07:29:43 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
07:29:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
07:29:43 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
07:29:43 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
07:29:43 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
07:29:43 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
07:29:43 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
07:29:43 DEBUG opendrift:685: No elements hit coastline.
07:29:43 DEBUG opendrift:2213: Updating minval and maxval
07:29:43 DEBUG opendrift:2293: Writing to file
07:29:43 DEBUG opendrift:1636: No elements to deactivate
07:29:43 DEBUG opendrift:101: Changed mode from Mode.Run to Mode.Result
Print and plot results
print('Final speciation:')
for isp,sp in enumerate(o.name_species):
print ('{:32}: {:>6}'.format(sp,sum(o.elements.specie==isp)))
print('Number of transformations:')
for isp in range(o.nspecies):
print('{}'.format(['{:>9}'.format(np.int32(item)) for item in o.ntransformations[isp,:]]) )
m_pre = o.result.mass.isel(time=-1).sum()
m_deg = o.result.mass_degraded.isel(time=-1).sum()
m_vol = o.result.mass_volatilized.isel(time=-1).sum()
m_tot = m_pre + m_deg + m_vol
print('Mass budget for target chemical:')
print('mass preserved : {:.3f}'.format(m_pre * 1e-6),' g {:.3f}'.format(m_pre/m_tot*100),'%')
print('mass degraded : {:.3f}'.format(m_deg * 1e-6),' g {:.3f}'.format(m_deg/m_tot*100),'%')
print('mass volatilized : {:.3f}'.format(m_vol * 1e-6),' g {:.3f}'.format(m_vol/m_tot*100),'%')
legend=['dissolved', '', 'SPM', 'sediment', '']
o.animation_profile(color='specie',
markersize='mass',
markersize_scaling=30,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
legend = legend,
legend_loc = 3,
fps = 10
)
Final speciation:
LMM : 58
Humic colloid : 0
Particle reversible : 0
Sediment reversible : 442
Sediment slowly reversible : 0
Number of transformations:
[' 0', ' 0', ' 0', ' 454', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
[' 13', ' 0', ' 0', ' 38', ' 0']
[' 50', ' 0', ' 0', ' 0', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
Mass budget for target chemical:
mass preserved : 0.155 g 15.478 %
mass degraded : 0.844 g 84.394 %
mass volatilized : 0.001 g 0.129 %
07:29:43 DEBUG opendrift:3001: Saving animation..
07:29:43 INFO opendrift:4553: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_0.gif...
07:29:59 DEBUG opendrift:4591: MPLBACKEND = agg
07:29:59 DEBUG opendrift:4592: DISPLAY = None
07:29:59 DEBUG opendrift:4593: Time to save animation: 0:00:15.649444
07:29:59 INFO opendrift:3204: Time to make animation: 0:00:15.771930
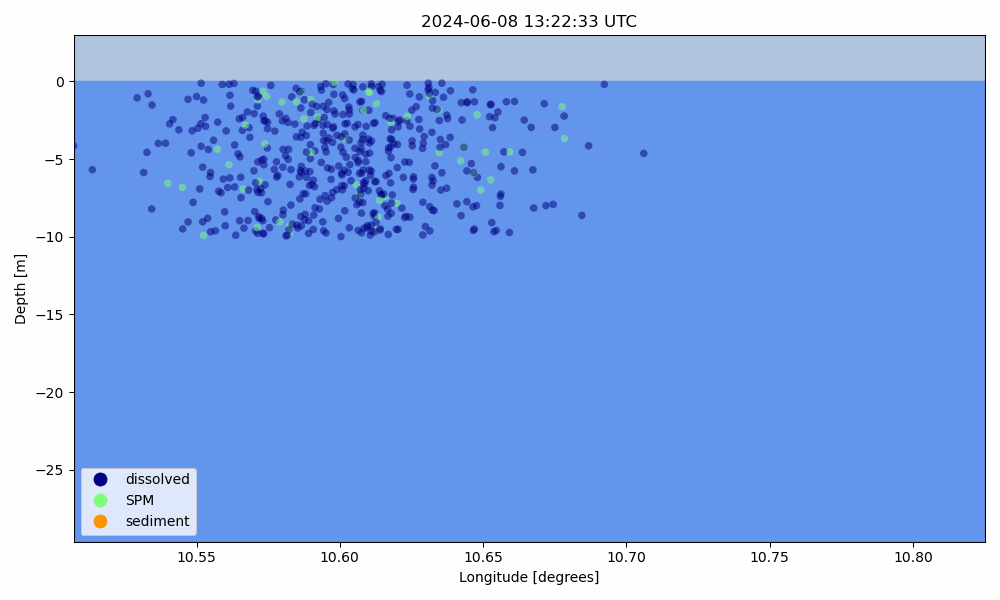
o.animation(color='specie',
markersize='mass',
markersize_scaling=100,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
colorbar=False,
fast = True,
legend = legend,
legend_loc = 3,
fps=10
)
07:29:59 DEBUG opendrift:2360: Setting up map: corners=None, fast=True, lscale=None
07:29:59 WARNING opendrift:2391: Plotting fast. This will make your plots less accurate.
07:30:00 DEBUG opendrift:3001: Saving animation..
07:30:00 INFO opendrift:4553: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_1.gif...
07:31:05 DEBUG opendrift:4591: MPLBACKEND = agg
07:31:05 DEBUG opendrift:4592: DISPLAY = None
07:31:05 DEBUG opendrift:4593: Time to save animation: 0:01:05.106904
07:31:05 INFO opendrift:2994: Time to make animation: 0:01:06.273602
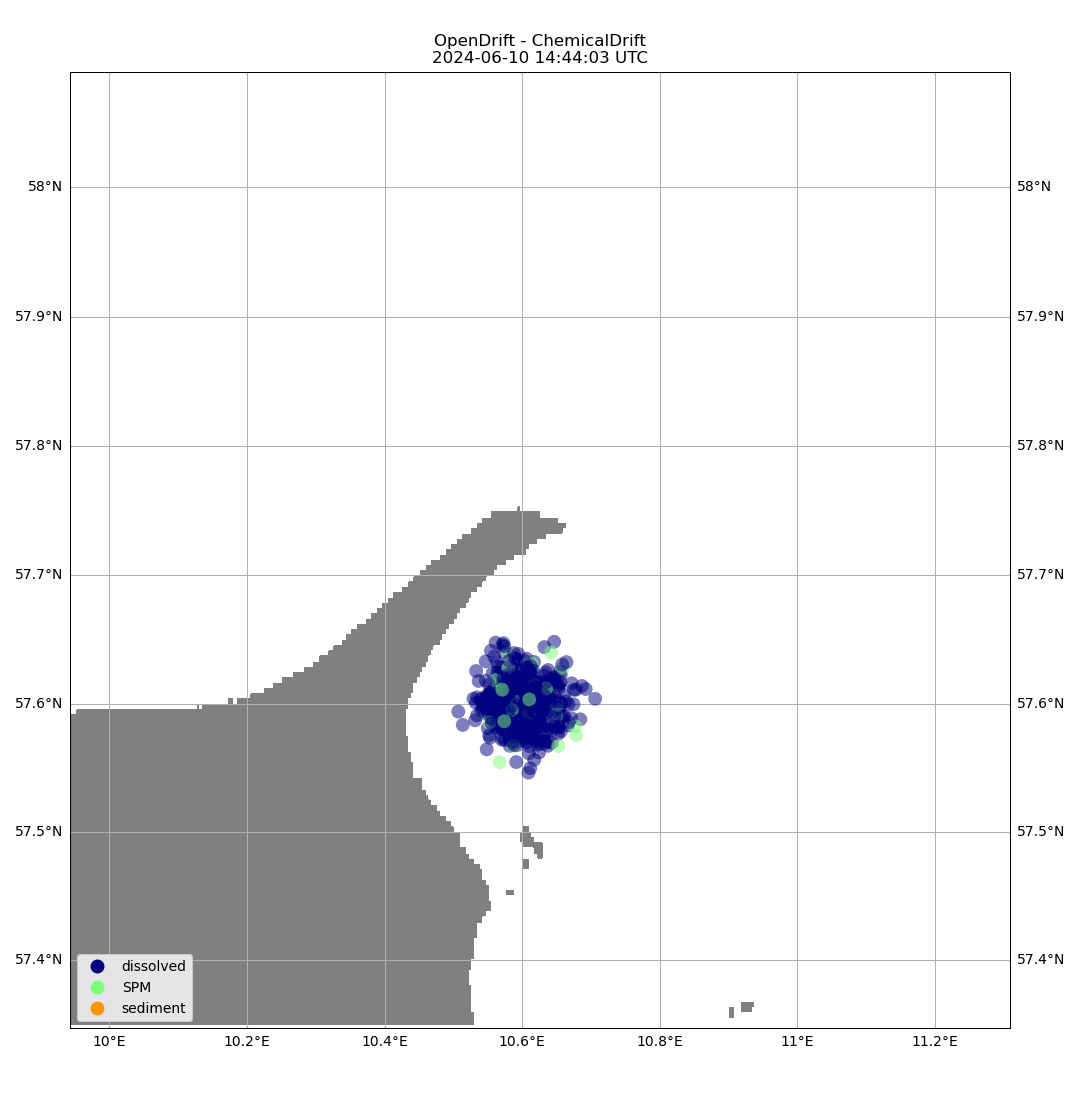
o.plot_mass(legend = legend,
time_unit = 'hours',
title = 'Chemical mass budget')
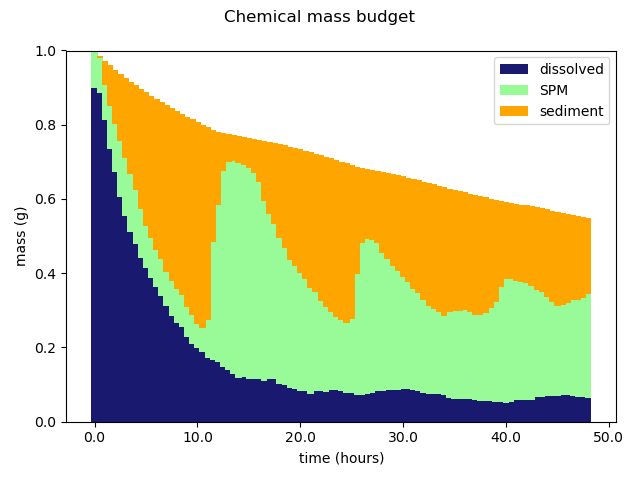
dissolved : 0.008826441131532192g (5.702615237704194%)
SPM : 0.0g (0.0%)
sediment : 0.14595238864421844g (94.2973847622958%)
/root/project/opendrift/models/chemicaldrift.py:3369: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.axes.get_xaxis().set_ticklabels(np.round(ax.axes.get_xticks() * time_conversion_factor))
Total running time of the script: (3 minutes 20.965 seconds)