Note
Go to the end to download the full example code.
Ship drift
from datetime import datetime
from opendrift.models.shipdrift import ShipDrift
o = ShipDrift(loglevel=20)
o.add_readers_from_list([
'https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml',
'https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc',
'https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml'
])
13:24:08 INFO opendrift:513: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-79-ga5f3ce3)
Seed ship elements at defined position and time Note: beam/length ratio is larger than allowed, but is then clipped internally
o.seed_elements(lon=5.0, lat=63.0, radius=1000, number=1000,
time=datetime.now(),
length=80.0, beam=20.0, height=9.0, draft=4.0)
13:24:08 WARNING opendrift.models.shipdrift:181: Ratio of beam to length should be in range 0.12 to 0.18, given range is 0.25-0.25. Using border value.
13:24:08 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
13:24:12 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
13:24:12 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
13:24:12 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
13:24:12 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
13:24:12 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
Running model
o.run(steps=24, stop_on_error=True)
13:24:12 INFO opendrift:889: Using existing reader for land_binary_mask
13:24:12 INFO opendrift:918: All points are in ocean
13:24:12 INFO opendrift:1948: Storing previous position of elements for coastline interaction
13:24:12 INFO opendrift:2009: 2025-07-01 13:24:08.109680 - step 1 of 24 - 1000 active elements (0 deactivated)
13:24:12 INFO opendrift.readers:61: Opening file with xr.open_dataset
13:24:14 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'x': 'x', 'y': 'y', 'time': 'time'}
13:24:14 INFO opendrift.readers:61: Opening file with xr.open_dataset
13:24:15 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'time': 'time', 'x': 'x', 'y': 'y'}
13:24:15 INFO opendrift.readers:61: Opening file with xr.open_dataset
13:24:17 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'y': 'rlat', 'x': 'rlon', 'time': 'time'}
13:24:19 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:19 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:19 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:19 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:21 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:21 INFO opendrift:2009: 2025-07-01 14:24:08.109680 - step 2 of 24 - 1000 active elements (0 deactivated)
13:24:22 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:22 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:23 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:23 INFO opendrift:2009: 2025-07-01 15:24:08.109680 - step 3 of 24 - 1000 active elements (0 deactivated)
13:24:24 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:24 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:25 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:25 INFO opendrift:2009: 2025-07-01 16:24:08.109680 - step 4 of 24 - 1000 active elements (0 deactivated)
13:24:26 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:26 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:27 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:27 INFO opendrift:2009: 2025-07-01 17:24:08.109680 - step 5 of 24 - 1000 active elements (0 deactivated)
13:24:28 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:28 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:29 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:29 INFO opendrift:2009: 2025-07-01 18:24:08.109680 - step 6 of 24 - 1000 active elements (0 deactivated)
13:24:30 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:30 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:31 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:31 INFO opendrift:2009: 2025-07-01 19:24:08.109680 - step 7 of 24 - 1000 active elements (0 deactivated)
13:24:32 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:32 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:33 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:33 INFO opendrift:2009: 2025-07-01 20:24:08.109680 - step 8 of 24 - 1000 active elements (0 deactivated)
13:24:35 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:35 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:35 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:35 INFO opendrift:2009: 2025-07-01 21:24:08.109680 - step 9 of 24 - 1000 active elements (0 deactivated)
13:24:37 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:37 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:38 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:38 INFO opendrift:2009: 2025-07-01 22:24:08.109680 - step 10 of 24 - 1000 active elements (0 deactivated)
13:24:39 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:39 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:40 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:40 INFO opendrift:2009: 2025-07-01 23:24:08.109680 - step 11 of 24 - 1000 active elements (0 deactivated)
13:24:42 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:42 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:43 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:43 INFO opendrift:2009: 2025-07-02 00:24:08.109680 - step 12 of 24 - 1000 active elements (0 deactivated)
13:24:44 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:44 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:45 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:45 INFO opendrift:2009: 2025-07-02 01:24:08.109680 - step 13 of 24 - 1000 active elements (0 deactivated)
13:24:47 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:47 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:47 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:47 INFO opendrift:2009: 2025-07-02 02:24:08.109680 - step 14 of 24 - 1000 active elements (0 deactivated)
13:24:49 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:49 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:50 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:50 INFO opendrift:2009: 2025-07-02 03:24:08.109680 - step 15 of 24 - 1000 active elements (0 deactivated)
13:24:51 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:51 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:52 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:52 INFO opendrift:2009: 2025-07-02 04:24:08.109680 - step 16 of 24 - 1000 active elements (0 deactivated)
13:24:53 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:53 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:54 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:54 INFO opendrift:2009: 2025-07-02 05:24:08.109680 - step 17 of 24 - 1000 active elements (0 deactivated)
13:24:56 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:56 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:57 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:57 INFO opendrift:2009: 2025-07-02 06:24:08.109680 - step 18 of 24 - 1000 active elements (0 deactivated)
13:24:59 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:59 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:24:59 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:24:59 INFO opendrift:2009: 2025-07-02 07:24:08.109680 - step 19 of 24 - 1000 active elements (0 deactivated)
13:25:01 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:01 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:02 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:25:02 INFO opendrift:2009: 2025-07-02 08:24:08.109680 - step 20 of 24 - 1000 active elements (0 deactivated)
13:25:03 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:03 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:04 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:25:04 INFO opendrift:2009: 2025-07-02 09:24:08.109680 - step 21 of 24 - 1000 active elements (0 deactivated)
13:25:06 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:06 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:07 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:25:07 INFO opendrift:2009: 2025-07-02 10:24:08.109680 - step 22 of 24 - 1000 active elements (0 deactivated)
13:25:08 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:08 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:09 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:25:09 INFO opendrift:2009: 2025-07-02 11:24:08.109680 - step 23 of 24 - 1000 active elements (0 deactivated)
13:25:11 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:11 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:12 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
13:25:12 INFO opendrift:2009: 2025-07-02 12:24:08.109680 - step 24 of 24 - 1000 active elements (0 deactivated)
13:25:14 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:14 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
13:25:15 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
Print and plot results
print(o)
o.plot(linecolor='orientation')
#o.animation(color='orientation', markersize=20, filename="orientation.gif", legend=['left','right'],colorbar=False,cmap='bwr')
o.animation(color='orientation', legend=['left','right'], markersize=20, colorbar=False, cmap='bwr')
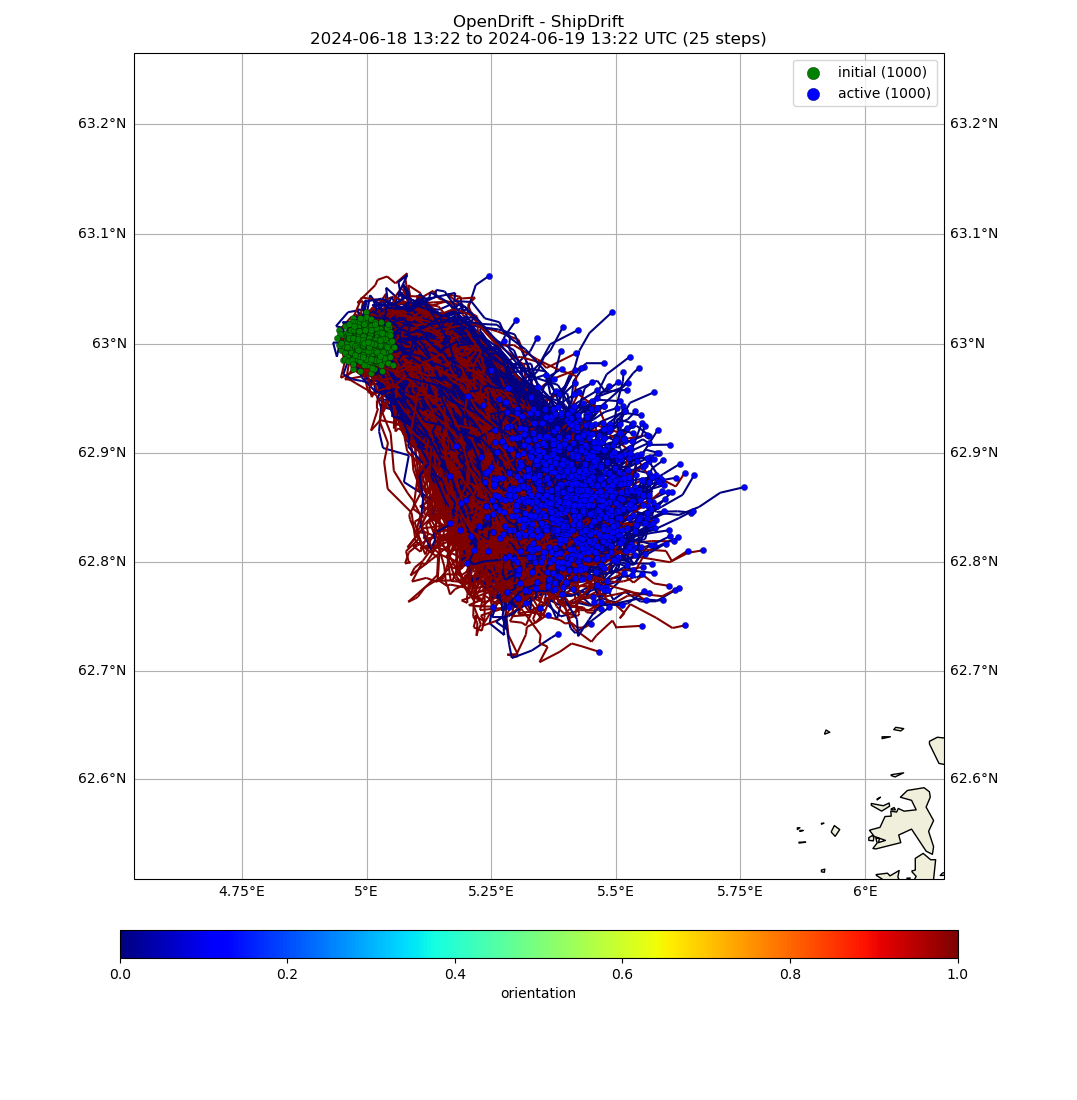
===========================
--------------------
Reader performance:
--------------------
global_landmask
0:00:00.0 total
0:00:00.0 preparing
0:00:00.0 reading
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml
0:00:21.7 total
0:00:00.0 preparing
0:00:21.6 reading
0:00:00.0 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc
0:00:16.5 total
0:00:00.0 preparing
0:00:16.4 reading
0:00:00.3 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
0:00:17.6 total
0:00:00.0 preparing
0:00:17.6 reading
0:00:00.0 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
Performance:
1:07.0 total time
4.4 configuration
0.0 preparing main loop
0.0 moving elements to ocean
1:02.4 main loop
0.5 updating elements
0.0 cleaning up
--------------------
===========================
Model: ShipDrift (OpenDrift version 1.14.2)
1000 active ShipObject particles (0 deactivated, 0 scheduled)
-------------------
Environment variables:
-----
land_binary_mask
1) global_landmask
-----
x_sea_water_velocity
y_sea_water_velocity
1) https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml
2) https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
-----
x_wind
y_wind
1) https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc
-----
sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment
sea_surface_wave_significant_height
sea_surface_wave_stokes_drift_x_velocity
sea_surface_wave_stokes_drift_y_velocity
1) https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
Discarded readers:
Time:
Start: 2025-07-01 13:24:08.109680 UTC
Present: 2025-07-02 13:24:08.109680 UTC
Calculation steps: 24 * 1:00:00 - total time: 1 day, 0:00:00
Output steps: 25 * 1:00:00
===========================
13:25:32 INFO opendrift:4527: Saving animation to /root/project/docs/source/gallery/animations/example_shipdrift_0.gif...
13:25:47 INFO opendrift:2968: Time to make animation: 0:00:16.464501
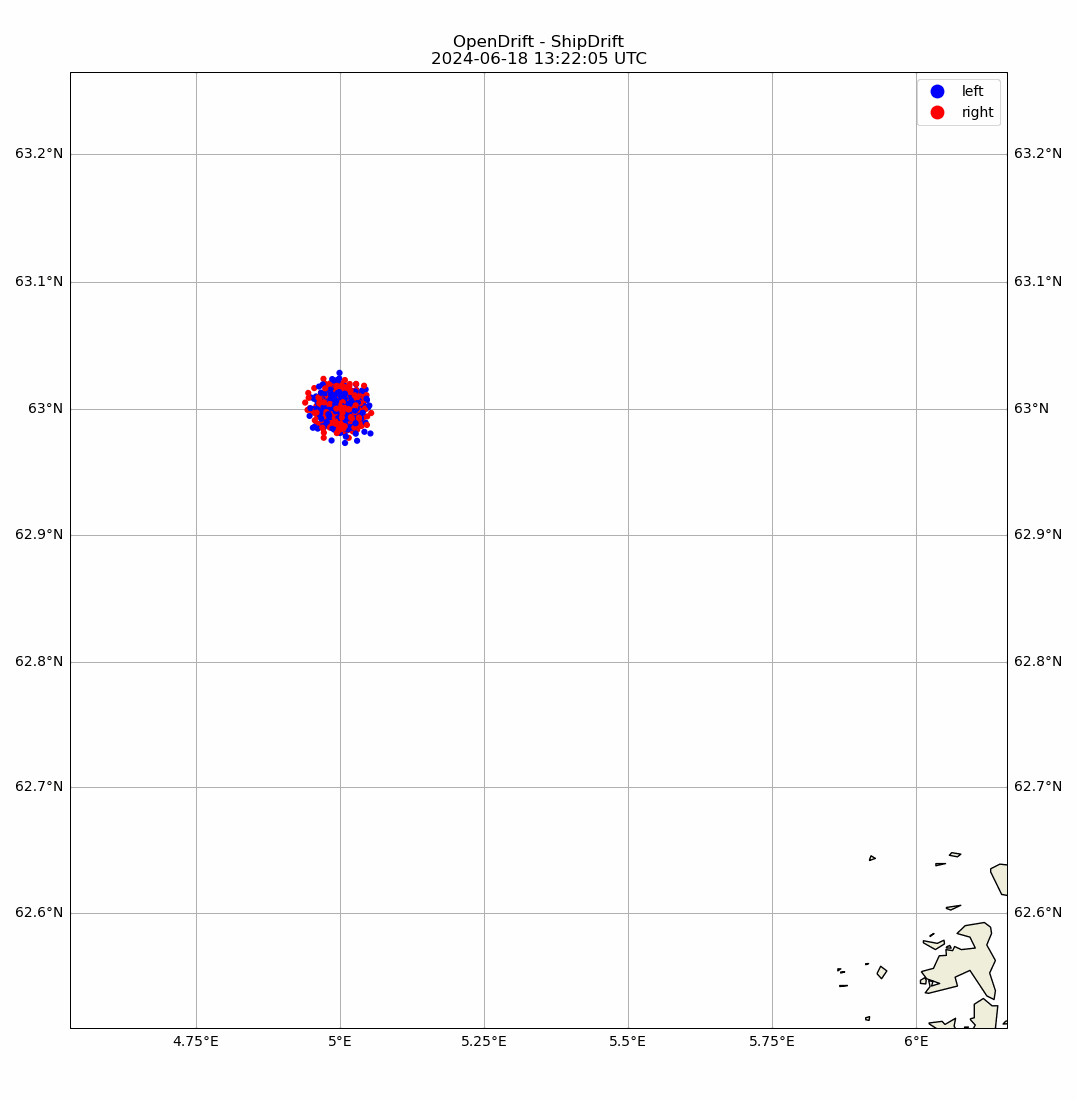
Total running time of the script: (1 minutes 47.629 seconds)