Note
Go to the end to download the full example code.
Retrieving wind drift factor from trajectory
import trajan as _
from datetime import datetime, timedelta
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
import cmocean
from opendrift import test_data_folder as tdf
from opendrift.models.oceandrift import OceanDrift
from opendrift.models.physics_methods import wind_drift_factor_from_trajectory
A very simple drift model is: current + wind_drift_factor*wind where wind_drift_factor for surface drift is typically 0.033 if Stokes drift included, and 0.02 in addition to Stokes drift. This example illustrates how a best-fit wind_drift_factor can be calculated from an observed trajectory, using two different methods.
First we simulate a synthetic drifter trajectory
ot = OceanDrift(loglevel=50)
ot.add_readers_from_list([tdf +
'16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc',
tdf + '16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc'], lazy=False)
Adding some horizontal diffusivity as “noise”
ot.set_config('drift:horizontal_diffusivity', 10)
Using a wind_drift_factor of 0.33 i.e. drift is current + 3.3% of wind speed
ot.seed_elements(lon=4, lat=60, number=1, time=ot.env.readers[list(ot.env.readers)[0]].start_time,
wind_drift_factor=0.033)
ot.run(duration=timedelta(hours=12), time_step=600)
<xarray.Dataset> Size: 11kB Dimensions: ( trajectory: 1, time: 73) Coordinates: * trajectory (trajectory) int64 8B ... * time (time) datetime64[ns] 584B ... Data variables: (12/35) status (trajectory, time) float32 292B ... moving (trajectory, time) float32 292B ... age_seconds (trajectory, time) float32 292B ... origin_marker (trajectory, time) float32 292B ... lon (trajectory, time) float32 292B ... lat (trajectory, time) float32 292B ... ... ... surface_downward_y_stress (trajectory, time) float32 292B ... turbulent_kinetic_energy (trajectory, time) float32 292B ... turbulent_generic_length_scale (trajectory, time) float32 292B ... ocean_mixed_layer_thickness (trajectory, time) float32 292B ... sea_floor_depth_below_sea_level (trajectory, time) float32 292B ... land_binary_mask (trajectory, time) float32 292B ... Attributes: (12/155) Conventions: ... standard_name_vocabulary: ... featureType: ... title: ... summary: ... keywords: ... ... ... geospatial_lon_units: ... geospatial_lon_resolution: ... runtime: ... geospatial_vertical_min: ... geospatial_vertical_max: ... geospatial_vertical_positive: ...
- trajectory: 1
- time: 73
- trajectory(trajectory)int640
- cf_role :
- trajectory_id
- dtype :
- <class 'numpy.int32'>
array([0])
- time(time)datetime64[ns]2015-11-16 ... 2015-11-16T12:00:00
- standard_name :
- time
- long_name :
- time
- dtype :
- <class 'numpy.float64'>
array(['2015-11-16T00:00:00.000000000', '2015-11-16T00:10:00.000000000', '2015-11-16T00:20:00.000000000', '2015-11-16T00:30:00.000000000', '2015-11-16T00:40:00.000000000', '2015-11-16T00:50:00.000000000', '2015-11-16T01:00:00.000000000', '2015-11-16T01:10:00.000000000', '2015-11-16T01:20:00.000000000', '2015-11-16T01:30:00.000000000', '2015-11-16T01:40:00.000000000', '2015-11-16T01:50:00.000000000', '2015-11-16T02:00:00.000000000', '2015-11-16T02:10:00.000000000', '2015-11-16T02:20:00.000000000', '2015-11-16T02:30:00.000000000', '2015-11-16T02:40:00.000000000', '2015-11-16T02:50:00.000000000', '2015-11-16T03:00:00.000000000', '2015-11-16T03:10:00.000000000', '2015-11-16T03:20:00.000000000', '2015-11-16T03:30:00.000000000', '2015-11-16T03:40:00.000000000', '2015-11-16T03:50:00.000000000', '2015-11-16T04:00:00.000000000', '2015-11-16T04:10:00.000000000', '2015-11-16T04:20:00.000000000', '2015-11-16T04:30:00.000000000', '2015-11-16T04:40:00.000000000', '2015-11-16T04:50:00.000000000', '2015-11-16T05:00:00.000000000', '2015-11-16T05:10:00.000000000', '2015-11-16T05:20:00.000000000', '2015-11-16T05:30:00.000000000', '2015-11-16T05:40:00.000000000', '2015-11-16T05:50:00.000000000', '2015-11-16T06:00:00.000000000', '2015-11-16T06:10:00.000000000', '2015-11-16T06:20:00.000000000', '2015-11-16T06:30:00.000000000', '2015-11-16T06:40:00.000000000', '2015-11-16T06:50:00.000000000', '2015-11-16T07:00:00.000000000', '2015-11-16T07:10:00.000000000', '2015-11-16T07:20:00.000000000', '2015-11-16T07:30:00.000000000', '2015-11-16T07:40:00.000000000', '2015-11-16T07:50:00.000000000', '2015-11-16T08:00:00.000000000', '2015-11-16T08:10:00.000000000', '2015-11-16T08:20:00.000000000', '2015-11-16T08:30:00.000000000', '2015-11-16T08:40:00.000000000', '2015-11-16T08:50:00.000000000', '2015-11-16T09:00:00.000000000', '2015-11-16T09:10:00.000000000', '2015-11-16T09:20:00.000000000', '2015-11-16T09:30:00.000000000', '2015-11-16T09:40:00.000000000', '2015-11-16T09:50:00.000000000', '2015-11-16T10:00:00.000000000', '2015-11-16T10:10:00.000000000', '2015-11-16T10:20:00.000000000', '2015-11-16T10:30:00.000000000', '2015-11-16T10:40:00.000000000', '2015-11-16T10:50:00.000000000', '2015-11-16T11:00:00.000000000', '2015-11-16T11:10:00.000000000', '2015-11-16T11:20:00.000000000', '2015-11-16T11:30:00.000000000', '2015-11-16T11:40:00.000000000', '2015-11-16T11:50:00.000000000', '2015-11-16T12:00:00.000000000'], dtype='datetime64[ns]')
- status(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- valid_range :
- [0 0]
- flag_values :
- [0]
- flag_meanings :
- active
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- moving(trajectory, time)float321.0 1.0 1.0 1.0 ... 1.0 1.0 1.0 1.0
- dtype :
- <class 'numpy.int32'>
- minval :
- 1
- maxval :
- 1
array([[1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.]], dtype=float32)
- age_seconds(trajectory, time)float320.0 600.0 ... 4.26e+04 4.32e+04
- dtype :
- <class 'numpy.float32'>
- units :
- s
- minval :
- 0.0
- maxval :
- 43200.0
array([[ 0., 600., 1200., 1800., 2400., 3000., 3600., 4200., 4800., 5400., 6000., 6600., 7200., 7800., 8400., 9000., 9600., 10200., 10800., 11400., 12000., 12600., 13200., 13800., 14400., 15000., 15600., 16200., 16800., 17400., 18000., 18600., 19200., 19800., 20400., 21000., 21600., 22200., 22800., 23400., 24000., 24600., 25200., 25800., 26400., 27000., 27600., 28200., 28800., 29400., 30000., 30600., 31200., 31800., 32400., 33000., 33600., 34200., 34800., 35400., 36000., 36600., 37200., 37800., 38400., 39000., 39600., 40200., 40800., 41400., 42000., 42600., 43200.]], dtype=float32)
- origin_marker(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- unit :
- description :
- An integer kept constant during the simulation. Different values may be used for different seedings, to separate elements during analysis. With GUI, only a single seeding is possible.
- flag_values :
- [0]
- flag_meanings :
- Seed_0
- minval :
- 0
- maxval :
- 0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- lon(trajectory, time)float324.0 4.0 3.997 ... 3.949 3.956 3.965
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_east
- standard_name :
- longitude
- long_name :
- longitude
- axis :
- X
- minval :
- 3.7812707
- maxval :
- 4.0
array([[4. , 3.9995713, 3.9974113, 3.9967334, 3.994082 , 3.9891586, 3.9845006, 3.9807963, 3.9764132, 3.9740367, 3.969281 , 3.9588764, 3.9552114, 3.9542491, 3.9487095, 3.9458885, 3.9401712, 3.9322417, 3.9252079, 3.9212203, 3.9142256, 3.905988 , 3.896412 , 3.8890455, 3.8801296, 3.870589 , 3.8628652, 3.8562696, 3.8506286, 3.844982 , 3.8376832, 3.8302822, 3.8228402, 3.817619 , 3.8091147, 3.8023343, 3.7993033, 3.7974896, 3.794635 , 3.7897365, 3.7864304, 3.781942 , 3.7812707, 3.7830985, 3.7847705, 3.7823513, 3.7816179, 3.7824059, 3.7837405, 3.7846553, 3.7866426, 3.7929158, 3.793678 , 3.7955437, 3.7998276, 3.8095036, 3.8196545, 3.8251717, 3.8328094, 3.843457 , 3.8538837, 3.863878 , 3.8736463, 3.8813987, 3.889888 , 3.9005349, 3.9088438, 3.9179878, 3.927132 , 3.938496 , 3.9492276, 3.9558058, 3.9651551]], dtype=float32)
- lat(trajectory, time)float3260.0 60.0 60.0 ... 60.25 60.25
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_north
- standard_name :
- latitude
- long_name :
- latitude
- axis :
- Y
- minval :
- 60.0
- maxval :
- 60.24799
array([[60. , 60.0015 , 60.004833, 60.004986, 60.006004, 60.00763 , 60.01036 , 60.011864, 60.013756, 60.015285, 60.016335, 60.019054, 60.020695, 60.02189 , 60.024456, 60.028908, 60.032528, 60.034096, 60.037945, 60.042862, 60.046173, 60.04823 , 60.05348 , 60.056313, 60.060493, 60.063946, 60.06835 , 60.071587, 60.076298, 60.080612, 60.08385 , 60.08695 , 60.088783, 60.0921 , 60.09646 , 60.100567, 60.104927, 60.10813 , 60.11202 , 60.11619 , 60.121258, 60.127438, 60.131508, 60.139164, 60.144844, 60.1517 , 60.15878 , 60.165646, 60.172226, 60.179813, 60.185787, 60.18984 , 60.195953, 60.202816, 60.20687 , 60.21296 , 60.218018, 60.223515, 60.22742 , 60.229843, 60.23289 , 60.23347 , 60.236168, 60.237328, 60.240444, 60.242104, 60.243813, 60.244762, 60.246296, 60.246906, 60.24645 , 60.247234, 60.24799 ]], dtype=float32)
- z(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.float32'>
- units :
- m
- standard_name :
- z
- long_name :
- vertical position
- axis :
- Z
- positive :
- up
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- wind_drift_factor(trajectory, time)float320.033 0.033 0.033 ... 0.033 0.033
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements at surface are moved with this fraction of the vind vector, in addition to currents and Stokes drift
- minval :
- 0.033
- maxval :
- 0.033
array([[0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033, 0.033]], dtype=float32)
- current_drift_factor(trajectory, time)float321.0 1.0 1.0 1.0 ... 1.0 1.0 1.0 1.0
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements are moved with this fraction of the current vector, in addition to currents and Stokes drift
- minval :
- 1.0
- maxval :
- 1.0
array([[1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.]], dtype=float32)
- terminal_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.float32'>
- units :
- m/s
- description :
- Terminal rise/sinking velocity (buoyancy) in the ocean column
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- x_sea_water_velocity(trajectory, time)float32-0.0694 -0.0808 ... 0.3853 0.3853
- minval :
- -0.17572045
- maxval :
- 0.48428676
array([[-0.06940369, -0.08079931, -0.09907474, -0.1087418 , -0.12145107, -0.13579927, -0.15334266, -0.15768693, -0.16202113, -0.16697715, -0.16762277, -0.16318424, -0.16764109, -0.17113927, -0.17363237, -0.17572045, -0.1746465 , -0.17312557, -0.16243301, -0.14157137, -0.13117425, -0.13021033, -0.1380909 , -0.14077573, -0.12717442, -0.0922602 , -0.05987609, -0.06150946, -0.08102374, -0.10800688, -0.1123608 , -0.10289865, -0.09041336, -0.07682064, -0.06062235, -0.02348054, 0.00266362, 0.02603225, 0.05429186, 0.08482854, 0.11631771, 0.15723197, 0.18663357, 0.21646436, 0.23576508, 0.2567211 , 0.27265233, 0.28311706, 0.29419103, 0.3175749 , 0.33924294, 0.35323748, 0.38464317, 0.4177318 , 0.43786478, 0.43498176, 0.4487033 , 0.4804256 , 0.48428676, 0.47630677, 0.43296927, 0.40996107, 0.38894713, 0.38488236, 0.35389122, 0.36675474, 0.3845193 , 0.40474233, 0.41547248, 0.41697416, 0.4049568 , 0.38528034, 0.38528034]], dtype=float32)
- y_sea_water_velocity(trajectory, time)float32-0.1531 -0.1545 ... -0.01154
- minval :
- -0.16403046
- maxval :
- 0.5000382
array([[-0.15314175, -0.1544842 , -0.16403046, -0.16013859, -0.15692389, -0.14878488, -0.13903847, -0.11981671, -0.10147452, -0.086386 , -0.06357147, -0.02182256, 0.01211555, 0.03346429, 0.07802533, 0.1197357 , 0.16662098, 0.1979991 , 0.20187677, 0.17936194, 0.1494911 , 0.1265126 , 0.11320075, 0.14024793, 0.18414232, 0.24419579, 0.3063169 , 0.28019422, 0.22210383, 0.1373224 , 0.10412746, 0.10525415, 0.12623842, 0.1505359 , 0.1692803 , 0.19107911, 0.21690413, 0.24471875, 0.28016895, 0.33054236, 0.3829015 , 0.43544176, 0.47376212, 0.48693386, 0.4815288 , 0.49392298, 0.5000382 , 0.4974812 , 0.48866913, 0.46239388, 0.4456654 , 0.42704147, 0.40963328, 0.40082383, 0.3933413 , 0.36415395, 0.321257 , 0.2891778 , 0.2452588 , 0.21399657, 0.16868414, 0.12740783, 0.11402417, 0.12541895, 0.1345488 , 0.14423983, 0.12840967, 0.11900291, 0.10892824, 0.07302611, 0.02722109, -0.01153968, -0.01153968]], dtype=float32)
- sea_surface_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- x_wind(trajectory, time)float32-8.865 -9.054 -9.24 ... 13.55 13.55
- minval :
- -14.052733
- maxval :
- 17.658354
array([[ -8.864629 , -9.054137 , -9.239969 , -9.432019 , -9.620788 , -9.805811 , -9.999821 , -10.025045 , -10.050981 , -10.068057 , -10.101467 , -10.160105 , -10.186928 , -10.668076 , -11.162569 , -11.629163 , -12.119576 , -12.629008 , -13.108743 , -13.252151 , -13.404994 , -13.558749 , -13.720855 , -13.884402 , -14.052733 , -13.972402 , -13.906605 , -13.840812 , -13.788364 , -13.734763 , -13.676967 , -13.299236 , -12.915143 , -12.550205 , -12.194173 , -11.8371105 , -11.478739 , -11.031828 , -10.583696 , -10.130024 , -9.679073 , -9.224238 , -8.759862 , -8.388627 , -8.006632 , -7.604668 , -7.204419 , -6.808045 , -6.4077125 , -4.755812 , -3.120537 , -1.5387678 , 0.06162483, 1.6282946 , 3.1873326 , 4.90657 , 6.6133375 , 8.302223 , 9.882646 , 11.3480215 , 12.763072 , 13.260946 , 13.747283 , 14.49689 , 15.342211 , 16.389708 , 17.658354 , 16.831219 , 16.007013 , 15.191186 , 14.379017 , 13.554495 , 13.554495 ]], dtype=float32)
- y_wind(trajectory, time)float3210.85 11.05 11.24 ... 1.086 1.086
- minval :
- 1.0860494
- maxval :
- 18.29276
array([[10.85413 , 11.045767 , 11.238269 , 11.4319515, 11.627093 , 11.822563 , 12.012818 , 12.420832 , 12.825455 , 13.230564 , 13.625249 , 13.999638 , 14.389209 , 14.476291 , 14.556144 , 14.646544 , 14.726218 , 14.8109865, 14.882517 , 14.877244 , 14.890252 , 14.914092 , 14.940205 , 14.976655 , 15.031316 , 15.241013 , 15.449901 , 15.657325 , 15.865482 , 16.070488 , 16.282312 , 16.676336 , 17.056427 , 17.401564 , 17.7191 , 18.017683 , 18.29276 , 18.242086 , 18.199823 , 18.178612 , 18.184385 , 18.21007 , 18.24416 , 18.190613 , 18.149199 , 18.110067 , 18.075796 , 18.044144 , 18.020256 , 17.381456 , 16.735487 , 16.106676 , 15.454015 , 14.81367 , 14.151513 , 12.416919 , 10.622425 , 8.785438 , 7.058875 , 5.54775 , 4.240399 , 3.972507 , 3.9031198, 3.5029144, 3.0063515, 2.2742426, 1.2697871, 1.293173 , 1.2901855, 1.2495993, 1.1787533, 1.0860494, 1.0860494]], dtype=float32)
- upward_sea_water_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- ocean_vertical_diffusivity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_significant_height(trajectory, time)float324.831 5.018 5.207 ... 4.549 4.549
- minval :
- 3.5943263
- maxval :
- 11.473107
array([[ 4.8312864, 5.018064 , 5.2072225, 5.4034514, 5.6026216, 5.803802 , 6.0098834, 6.2675533, 6.531656 , 6.7997737, 7.077101 , 7.3607526, 7.64624 , 7.9549212, 8.2775135, 8.604064 , 8.948142 , 9.319887 , 9.6758795, 9.765016 , 9.87477 , 9.994237 , 10.1222 , 10.260089 , 10.416125 , 10.516905 , 10.629489 , 10.743309 , 10.869079 , 10.993846 , 11.123458 , 11.192259 , 11.259976 , 11.323923 , 11.381544 , 11.432949 , 11.473107 , 11.180084 , 10.903906 , 10.653749 , 10.439165 , 10.250652 , 10.07578 , 9.87118 , 9.68009 , 9.490816 , 9.314495 , 9.149739 , 8.998394 , 7.988426 , 7.1294317, 6.440104 , 5.875227 , 5.4635653, 5.1764402, 4.385056 , 3.8516746, 3.5943263, 3.6283624, 3.9250557, 4.449574 , 4.714184 , 5.0238643, 5.471783 , 6.0127707, 6.7353497, 7.710373 , 7.01007 , 6.344072 , 5.715407 , 5.1203814, 4.548634 , 4.548634 ]], dtype=float32)
- sea_surface_wave_stokes_drift_x_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_stokes_drift_y_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_period_at_variance_spectral_density_maximum(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment(trajectory, time)float3211.97 12.2 12.43 ... 11.62 11.62
- minval :
- 10.328526
- maxval :
- 18.453135
array([[11.974599, 12.203873, 12.431761, 12.663835, 12.895115, 13.124595, 13.355575, 13.638875, 13.92327 , 14.206163, 14.492966, 14.780553, 15.064459, 15.365529, 15.673988, 15.980171, 16.296564, 16.631632, 16.946297, 17.024174, 17.11958 , 17.222824, 17.332731, 17.450392, 17.582584, 17.667439, 17.761751, 17.856594, 17.96081 , 18.063604, 18.169771, 18.225878, 18.280931, 18.332767, 18.37935 , 18.420809, 18.453135, 18.215961, 17.989565, 17.78201 , 17.602018, 17.442364, 17.292944, 17.116467, 16.949984, 16.783455, 16.626822, 16.479118, 16.342258, 15.397854, 14.546451, 13.825346, 13.205107, 12.734082, 12.394962, 11.408201, 10.691888, 10.328526, 10.377313, 10.793257, 11.491819, 11.828586, 12.210924, 12.743655, 13.358783, 14.138706, 15.127504, 14.424168, 13.72188 , 13.024263, 12.327663, 11.619035, 11.619035]], dtype=float32)
- sea_surface_swell_wave_to_direction(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_swell_wave_peak_period_from_variance_spectral_density(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_swell_wave_significant_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_to_direction(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_mean_period(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_significant_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- surface_downward_x_stress(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- surface_downward_y_stress(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- turbulent_kinetic_energy(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- turbulent_generic_length_scale(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- ocean_mixed_layer_thickness(trajectory, time)float3250.0 50.0 50.0 ... 50.0 50.0 50.0
- minval :
- 50.0
- maxval :
- 50.0
array([[50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.]], dtype=float32)
- sea_floor_depth_below_sea_level(trajectory, time)float321e+04 1e+04 1e+04 ... 1e+04 1e+04
- minval :
- 10000.0
- maxval :
- 10000.0
array([[10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000., 10000.]], dtype=float32)
- land_binary_mask(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- trajectoryPandasIndex
PandasIndex(Index([0], dtype='int64', name='trajectory'))
- timePandasIndex
PandasIndex(DatetimeIndex(['2015-11-16 00:00:00', '2015-11-16 00:10:00', '2015-11-16 00:20:00', '2015-11-16 00:30:00', '2015-11-16 00:40:00', '2015-11-16 00:50:00', '2015-11-16 01:00:00', '2015-11-16 01:10:00', '2015-11-16 01:20:00', '2015-11-16 01:30:00', '2015-11-16 01:40:00', '2015-11-16 01:50:00', '2015-11-16 02:00:00', '2015-11-16 02:10:00', '2015-11-16 02:20:00', '2015-11-16 02:30:00', '2015-11-16 02:40:00', '2015-11-16 02:50:00', '2015-11-16 03:00:00', '2015-11-16 03:10:00', '2015-11-16 03:20:00', '2015-11-16 03:30:00', '2015-11-16 03:40:00', '2015-11-16 03:50:00', '2015-11-16 04:00:00', '2015-11-16 04:10:00', '2015-11-16 04:20:00', '2015-11-16 04:30:00', '2015-11-16 04:40:00', '2015-11-16 04:50:00', '2015-11-16 05:00:00', '2015-11-16 05:10:00', '2015-11-16 05:20:00', '2015-11-16 05:30:00', '2015-11-16 05:40:00', '2015-11-16 05:50:00', '2015-11-16 06:00:00', '2015-11-16 06:10:00', '2015-11-16 06:20:00', '2015-11-16 06:30:00', '2015-11-16 06:40:00', '2015-11-16 06:50:00', '2015-11-16 07:00:00', '2015-11-16 07:10:00', '2015-11-16 07:20:00', '2015-11-16 07:30:00', '2015-11-16 07:40:00', '2015-11-16 07:50:00', '2015-11-16 08:00:00', '2015-11-16 08:10:00', '2015-11-16 08:20:00', '2015-11-16 08:30:00', '2015-11-16 08:40:00', '2015-11-16 08:50:00', '2015-11-16 09:00:00', '2015-11-16 09:10:00', '2015-11-16 09:20:00', '2015-11-16 09:30:00', '2015-11-16 09:40:00', '2015-11-16 09:50:00', '2015-11-16 10:00:00', '2015-11-16 10:10:00', '2015-11-16 10:20:00', '2015-11-16 10:30:00', '2015-11-16 10:40:00', '2015-11-16 10:50:00', '2015-11-16 11:00:00', '2015-11-16 11:10:00', '2015-11-16 11:20:00', '2015-11-16 11:30:00', '2015-11-16 11:40:00', '2015-11-16 11:50:00', '2015-11-16 12:00:00'], dtype='datetime64[ns]', name='time', freq='10min'))
- Conventions :
- CF-1.11, ACDD-1.3
- standard_name_vocabulary :
- CF Standard Name Table v85
- featureType :
- trajectory
- title :
- OpenDrift trajectory simulation
- summary :
- Output from simulation with OpenDrift framework
- keywords :
- trajectory, drift, lagrangian, simulation
- history :
- Created 2025-06-26 09:55:27.485427
- date_created :
- 2025-06-26T09:55:27.485433
- source :
- Output from simulation with OpenDrift
- model_url :
- https://github.com/OpenDrift/opendrift
- opendrift_class :
- OceanDrift
- opendrift_module :
- opendrift.models.oceandrift
- readers :
- odict_keys(['/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc', '/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc', 'global_landmask'])
- time_coverage_start :
- 2015-11-16 00:00:00
- time_step_calculation :
- 0:10:00
- time_step_output :
- 0:10:00
- config_environment:constant:x_sea_water_velocity :
- None
- config_environment:fallback:x_sea_water_velocity :
- 0
- config_environment:constant:y_sea_water_velocity :
- None
- config_environment:fallback:y_sea_water_velocity :
- 0
- config_environment:constant:sea_surface_height :
- None
- config_environment:fallback:sea_surface_height :
- 0
- config_environment:constant:x_wind :
- None
- config_environment:fallback:x_wind :
- 0
- config_environment:constant:y_wind :
- None
- config_environment:fallback:y_wind :
- 0
- config_environment:constant:upward_sea_water_velocity :
- None
- config_environment:fallback:upward_sea_water_velocity :
- 0
- config_environment:constant:ocean_vertical_diffusivity :
- None
- config_environment:fallback:ocean_vertical_diffusivity :
- 0
- config_environment:constant:sea_surface_wave_significant_height :
- None
- config_environment:fallback:sea_surface_wave_significant_height :
- 0
- config_environment:constant:sea_surface_wave_stokes_drift_x_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_x_velocity :
- 0
- config_environment:constant:sea_surface_wave_stokes_drift_y_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_y_velocity :
- 0
- config_environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum :
- None
- config_environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- config_environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- None
- config_environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- config_environment:constant:sea_surface_swell_wave_to_direction :
- None
- config_environment:fallback:sea_surface_swell_wave_to_direction :
- 0
- config_environment:constant:sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- None
- config_environment:fallback:sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- 0
- config_environment:constant:sea_surface_swell_wave_significant_height :
- None
- config_environment:fallback:sea_surface_swell_wave_significant_height :
- 0
- config_environment:constant:sea_surface_wind_wave_to_direction :
- None
- config_environment:fallback:sea_surface_wind_wave_to_direction :
- 0
- config_environment:constant:sea_surface_wind_wave_mean_period :
- None
- config_environment:fallback:sea_surface_wind_wave_mean_period :
- 0
- config_environment:constant:sea_surface_wind_wave_significant_height :
- None
- config_environment:fallback:sea_surface_wind_wave_significant_height :
- 0
- config_environment:constant:surface_downward_x_stress :
- None
- config_environment:fallback:surface_downward_x_stress :
- 0
- config_environment:constant:surface_downward_y_stress :
- None
- config_environment:fallback:surface_downward_y_stress :
- 0
- config_environment:constant:turbulent_kinetic_energy :
- None
- config_environment:fallback:turbulent_kinetic_energy :
- 0
- config_environment:constant:turbulent_generic_length_scale :
- None
- config_environment:fallback:turbulent_generic_length_scale :
- 0
- config_environment:constant:ocean_mixed_layer_thickness :
- None
- config_environment:fallback:ocean_mixed_layer_thickness :
- 50
- config_environment:constant:sea_floor_depth_below_sea_level :
- None
- config_environment:fallback:sea_floor_depth_below_sea_level :
- 10000
- config_environment:constant:land_binary_mask :
- None
- config_environment:fallback:land_binary_mask :
- None
- config_general:use_auto_landmask :
- True
- config_drift:current_uncertainty :
- 0
- config_drift:current_uncertainty_uniform :
- 0
- config_drift:max_speed :
- 2.0
- config_readers:max_number_of_fails :
- 1
- config_general:simulation_name :
- config_general:coastline_action :
- stranding
- config_general:coastline_approximation_precision :
- 0.001
- config_general:time_step_minutes :
- 60
- config_general:time_step_output_minutes :
- None
- config_seed:ocean_only :
- True
- config_seed:number :
- 1
- config_drift:max_age_seconds :
- None
- config_drift:advection_scheme :
- euler
- config_drift:horizontal_diffusivity :
- 10.0
- config_drift:profiles_depth :
- 50
- config_drift:wind_uncertainty :
- 0
- config_drift:relative_wind :
- False
- config_drift:deactivate_north_of :
- None
- config_drift:deactivate_south_of :
- None
- config_drift:deactivate_east_of :
- None
- config_drift:deactivate_west_of :
- None
- config_seed:origin_marker :
- 0
- config_seed:z :
- 0
- config_seed:wind_drift_factor :
- 0.02
- config_seed:current_drift_factor :
- 1
- config_seed:terminal_velocity :
- 0.0
- config_drift:vertical_advection :
- True
- config_drift:vertical_advection_at_surface :
- False
- config_drift:vertical_mixing :
- False
- config_drift:vertical_mixing_at_surface :
- False
- config_vertical_mixing:timestep :
- 60
- config_vertical_mixing:diffusivitymodel :
- environment
- config_vertical_mixing:background_diffusivity :
- 1.2e-05
- config_vertical_mixing:TSprofiles :
- False
- config_drift:wind_drift_depth :
- 0.1
- config_drift:stokes_drift :
- True
- config_drift:stokes_drift_profile :
- Phillips
- config_drift:use_tabularised_stokes_drift :
- False
- config_drift:tabularised_stokes_drift_fetch :
- 25000
- config_general:seafloor_action :
- lift_to_seafloor
- config_drift:truncate_ocean_model_below_m :
- None
- config_seed:seafloor :
- False
- opendrift_version :
- 1.14.2
- seed_geojson :
- {"features": [], "type": "FeatureCollection"}
- simulation_time :
- 2025-06-26 09:55:27.495558
- reader_x_sea_water_velocity :
- ['/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc']
- reader_y_sea_water_velocity :
- ['/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc']
- reader_sea_surface_height :
- 0
- reader_x_wind :
- ['/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc']
- reader_y_wind :
- ['/root/project/tests/test_data/16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc']
- reader_upward_sea_water_velocity :
- 0
- reader_ocean_vertical_diffusivity :
- 0
- reader_sea_surface_wave_significant_height :
- 0
- reader_sea_surface_wave_stokes_drift_x_velocity :
- 0
- reader_sea_surface_wave_stokes_drift_y_velocity :
- 0
- reader_sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- reader_sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- reader_sea_surface_swell_wave_to_direction :
- 0
- reader_sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- 0
- reader_sea_surface_swell_wave_significant_height :
- 0
- reader_sea_surface_wind_wave_to_direction :
- 0
- reader_sea_surface_wind_wave_mean_period :
- 0
- reader_sea_surface_wind_wave_significant_height :
- 0
- reader_surface_downward_x_stress :
- 0
- reader_surface_downward_y_stress :
- 0
- reader_turbulent_kinetic_energy :
- 0
- reader_turbulent_generic_length_scale :
- 0
- reader_ocean_mixed_layer_thickness :
- 50
- reader_sea_floor_depth_below_sea_level :
- 10000
- reader_land_binary_mask :
- ['global_landmask']
- time_coverage_end :
- 2015-11-16 12:00:00
- time_coverage_duration :
- P0DT12H0M0S
- time_coverage_resolution :
- P0DT0H10M0S
- performance :
- -------------------- Reader performance: -------------------- /root/project/tests/test_data/16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc 0:00:00.1 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 interpolation 0:00:00.0 interpolation_time 0:00:00.0 rotating vectors 0:00:00.0 masking -------------------- /root/project/tests/test_data/16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc 0:00:00.0 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 interpolation 0:00:00.0 interpolation_time 0:00:00.0 rotating vectors 0:00:00.0 masking -------------------- global_landmask 0:00:00.0 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 masking -------------------- Performance: 6.8 total time 4.7 configuration 0.0 preparing main loop 0.0 moving elements to ocean 2.0 main loop 0.0 updating elements 0.0 cleaning up --------------------
- geospatial_bounds_crs :
- EPSG:4326
- geospatial_bounds_vertical_crs :
- EPSG:5831
- geospatial_lat_min :
- 60.0
- geospatial_lat_max :
- 60.24799
- geospatial_lat_units :
- degrees_north
- geospatial_lat_resolution :
- point
- geospatial_lon_min :
- 3.7812707
- geospatial_lon_max :
- 4.0
- geospatial_lon_units :
- degrees_east
- geospatial_lon_resolution :
- point
- runtime :
- 0:00:06.813685
- geospatial_vertical_min :
- 0.0
- geospatial_vertical_max :
- 0.0
- geospatial_vertical_positive :
- up
Secondly, calculating the wind_drift_factor which reproduces the “observed” trajectory with minimal difference
drifter_lons = ot.result.lon.squeeze()
drifter_lats = ot.result.lat.squeeze()
drifter_times = pd.to_datetime(ot.result.time).to_pydatetime()
drifter={'lon': drifter_lons, 'lat': drifter_lats,
'time': drifter_times, 'linewidth': 2, 'color': 'b', 'label': 'Synthetic drifter'}
o = OceanDrift(loglevel=50)
o.add_readers_from_list([tdf +
'16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc',
tdf + '16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc'], lazy=False)
t = o.env.get_variables_along_trajectory(variables=['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind'],
lons=drifter_lons, lats=drifter_lats, times=drifter_times)
wind_drift_factor, azimuth = wind_drift_factor_from_trajectory(t)
o.seed_elements(lon=4, lat=60, number=1, time=ot.start_time,
wind_drift_factor=0.033)
New simulation, this time without diffusivity/noise
o.run(duration=timedelta(hours=12), time_step=600)
o.plot(fast=True, legend=True, drifter=drifter)
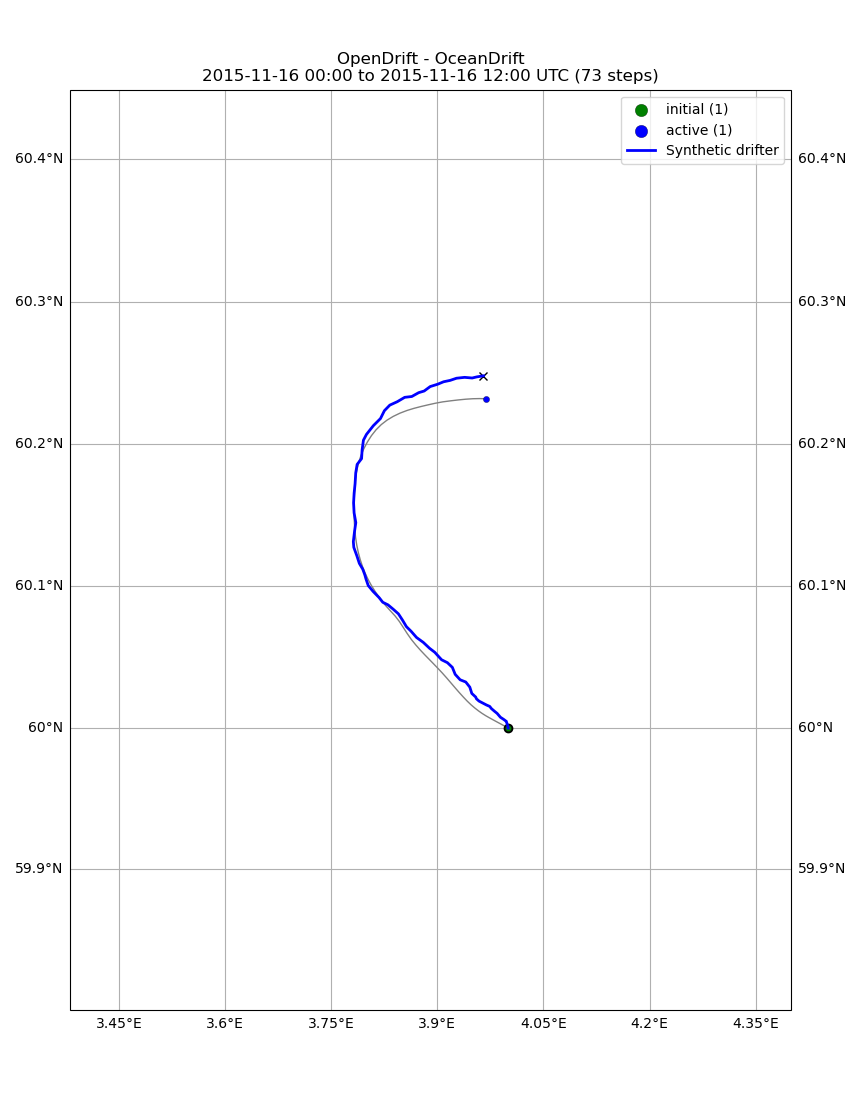
(<GeoAxes: title={'center': 'OpenDrift - OceanDrift\n2015-11-16 00:00 to 2015-11-16 12:00 UTC (73 steps)'}>, <Figure size 861.431x1100 with 1 Axes>)
The mean retrieved wind_drift_factor is 0.036, slichtly higher than the value 0.033 used for the simulation. The difference is due to the “noise” added by horizontal diffusion. Note that the retieved wind_drift_factor is linked to the wind and current used for the retrieval, other forcing datasets will yeld different value of the wind_drift_factor.
print(wind_drift_factor.mean())
plt.hist(wind_drift_factor, label='Retrieved wind_drift_factor')
plt.axvline(x=0.033, label='Actual wind_drift_factor of 0.033', color='k')
plt.axvline(x=wind_drift_factor.mean(), label='Mean retieved wind_drift_factor of %.3f' % wind_drift_factor.mean(), color='r')
plt.ylabel('Number')
plt.xlabel('Wind drift factor [fraction]')
plt.legend(loc='lower left')
plt.show()
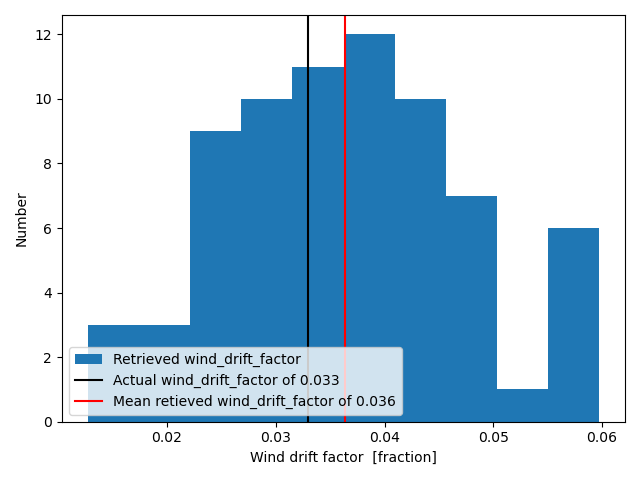
0.03639823255600984
A polar 2D histogram showing also the azimuth offset direction of the retrieved wind drift factor. See Sutherland et al. (2020), https://doi.org/10.1175/JTECH-D-20-0013.1
wmax = wind_drift_factor.max()
wbins = np.arange(0, wmax+.005, .005)
abins = np.linspace(-180, 180, 30)
hist, _, _ = np.histogram2d(azimuth, wind_drift_factor, bins=(abins, wbins))
A, W = np.meshgrid(abins, wbins)
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
ax.set_theta_zero_location('N', offset=0)
ax.set_theta_direction(-1)
pc = ax.pcolormesh(np.radians(A), W, hist.T, cmap=cmocean.cm.dense)
plt.arrow(np.pi, wmax, 0, -wmax, width=.015, facecolor='k', zorder=100,
head_width=.8, lw=2, head_length=.005, length_includes_head=True)
plt.text(np.pi, wmax*.5, ' Wind direction', fontsize=18)
plt.grid()
plt.show()
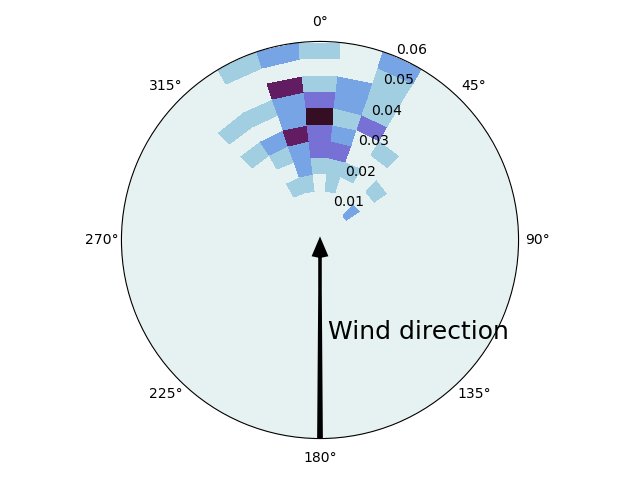
Alternative method, using skillscore
Here we calculate trajectories for a range of wind_drift_factors, and calculate the Liu-Weissberg skillscore for each. The optimized wind_drift_factor corresponds to the maximum skillscore.
o = OceanDrift(loglevel=50)
o.add_readers_from_list([tdf +
'16Nov2015_NorKyst_z_surface/norkyst800_subset_16Nov2015.nc',
tdf + '16Nov2015_NorKyst_z_surface/arome_subset_16Nov2015.nc'], lazy=False)
Calulating trajectories for 100 different wind_drift_factors between 0 and 0.05
wdf = np.linspace(0.0, 0.05, 100)
o.seed_elements(lon=4, lat=60, time=ot.start_time,
wind_drift_factor=wdf, number=len(wdf))
o.run(duration=timedelta(hours=12), time_step=600)
o.plot(linecolor='wind_drift_factor', drifter=drifter)
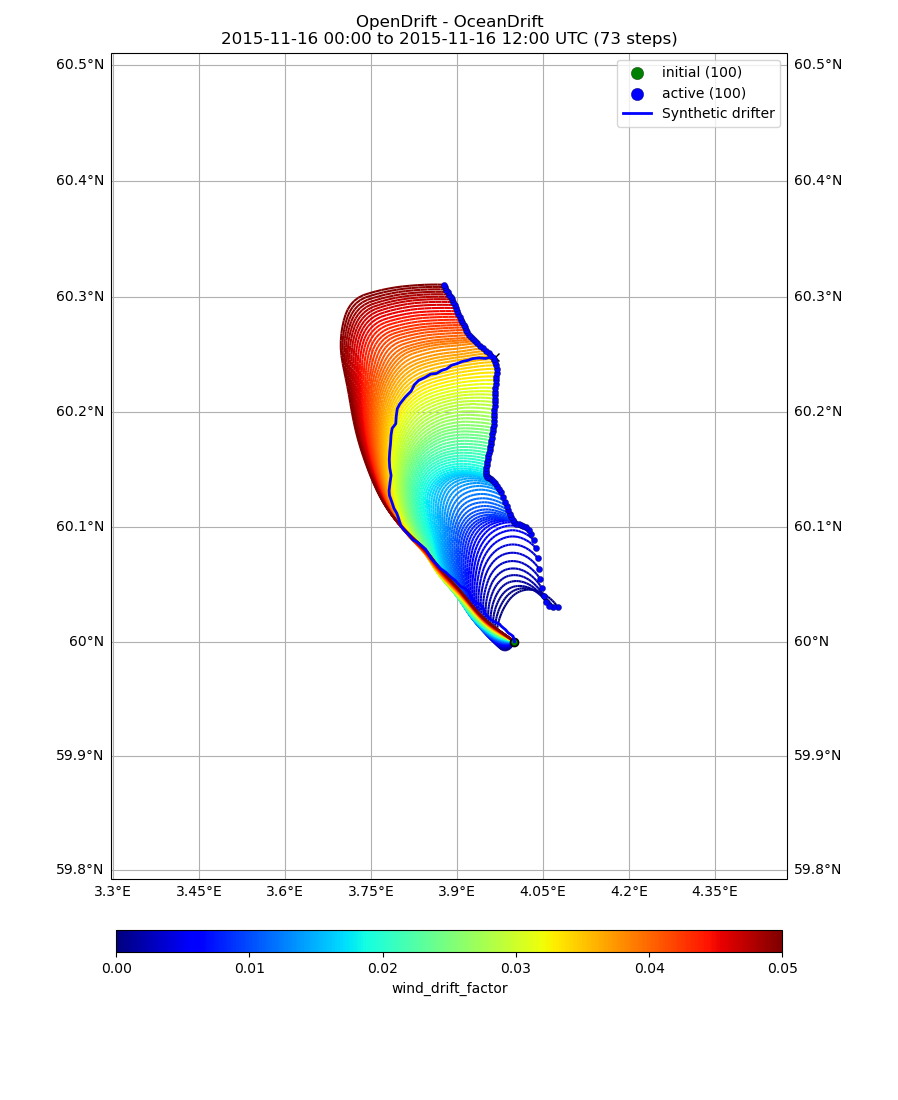
(<GeoAxes: title={'center': 'OpenDrift - OceanDrift\n2015-11-16 00:00 to 2015-11-16 12:00 UTC (73 steps)'}>, <Figure size 898.667x1100 with 2 Axes>)
Using TrajAn to calculate skillscore
skillscore = o.result.traj.skill(ot.result, tolerance_threshold=1)
Plotting and finding the wind_drift_factor which maximises the skillscore
ind = skillscore.argmax()
plt.plot(wdf, skillscore)
plt.xlabel('Wind drift factor [fraction]')
plt.ylabel('Liu-Weissberg skillscore')
plt.title('Maximum skillscore %.3f for wdf=%.3f' % (skillscore[ind], wdf[ind]))
plt.show()
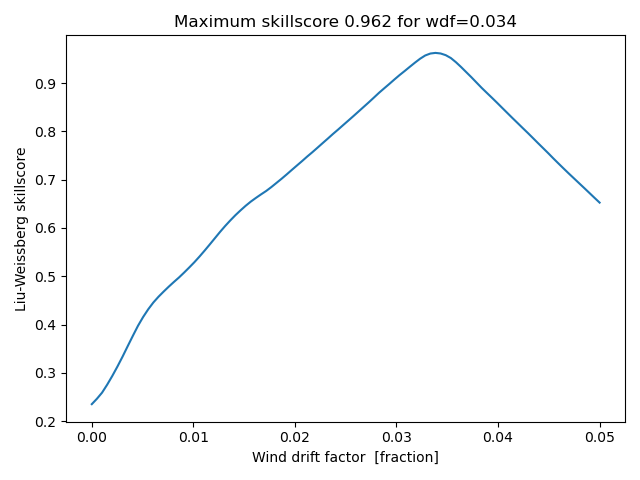
We see that using skillscore from the full trajectories gives a better estimate than calculating the average of the wind_drift_factor from one position to the next (i.e. polar histogram above). This is even more clear if increasing the diffusivity (i.e. noise) above from 10 m2/s to 200 m2/s: The histogram method then gives 0.071, which is much to high (true is 0.033), and the histogram is noisy. The skillscore method is still robust, and gives a wind_drift_factor of 0.034, only slightly too high.
Total running time of the script: (0 minutes 25.498 seconds)