Note
Go to the end to download the full example code.
Show dominating source
import os
from datetime import datetime
import xarray as xr
import opendrift
from opendrift.models.oceandrift import OceanDrift
of = 'test.nc'
Seed elements at 5 different locations/longitudes
lons = [4, 4.2, 4.3, 4.32, 4.6]
t = datetime.now()
o = OceanDrift(loglevel=20)
o.set_config('environment:constant:y_sea_water_velocity', .1)
for i, lon in enumerate(lons):
o.seed_elements(lon=lon, lat=60, radius=3000, number=2000, time=t, origin_marker_name='Lon %f' % lon)
o.run(steps=15, outfile=of)
09:35:39 INFO opendrift:507: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-21-g950aeec)
09:35:39 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
09:35:43 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
09:35:43 INFO opendrift.models.basemodel.environment:232: x_sea_water_velocity: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_height: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: x_wind: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: y_wind: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: upward_sea_water_velocity: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_to_direction: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_significant_height: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_to_direction: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_mean_period: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_significant_height: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: surface_downward_x_stress: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: surface_downward_y_stress: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: turbulent_kinetic_energy: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: turbulent_generic_length_scale: 0.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: ocean_mixed_layer_thickness: 50.000000
09:35:43 INFO opendrift.models.basemodel.environment:232: sea_floor_depth_below_sea_level: 10000.000000
09:35:43 INFO opendrift:911: Using existing reader for land_binary_mask
09:35:43 INFO opendrift:940: All points are in ocean
09:35:43 INFO opendrift:2102: 2025-05-28 09:35:38.952476 - step 1 of 15 - 10000 active elements (0 deactivated)
09:35:43 INFO opendrift:2102: 2025-05-28 10:35:38.952476 - step 2 of 15 - 10000 active elements (0 deactivated)
09:35:43 INFO opendrift:2102: 2025-05-28 11:35:38.952476 - step 3 of 15 - 10000 active elements (0 deactivated)
09:35:43 INFO opendrift:2102: 2025-05-28 12:35:38.952476 - step 4 of 15 - 10000 active elements (0 deactivated)
09:35:43 INFO opendrift:2102: 2025-05-28 13:35:38.952476 - step 5 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 14:35:38.952476 - step 6 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 15:35:38.952476 - step 7 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 16:35:38.952476 - step 8 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 17:35:38.952476 - step 9 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 18:35:38.952476 - step 10 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 19:35:38.952476 - step 11 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 20:35:38.952476 - step 12 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 21:35:38.952476 - step 13 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 22:35:38.952476 - step 14 of 15 - 10000 active elements (0 deactivated)
09:35:44 INFO opendrift:2102: 2025-05-28 23:35:38.952476 - step 15 of 15 - 10000 active elements (0 deactivated)
Calculate spatial density of elements at 1500m grid spacing
d = o.get_histogram(pixelsize_m=1500, weights=None)
dom = d.argmax(dim='origin_marker', skipna=True)
dom = dom.where(d.sum(dim='origin_marker')>0)
dom.name = 'Dominating source'
09:35:46 INFO opendrift:3896: calculating for origin_marker 0...
09:35:46 INFO opendrift:3896: calculating for origin_marker 1...
09:35:46 INFO opendrift:3896: calculating for origin_marker 2...
09:35:46 INFO opendrift:3896: calculating for origin_marker 3...
09:35:46 INFO opendrift:3896: calculating for origin_marker 4...
Show which of the 5 sources are dominating within each grid cell
o.animation(background=dom, show_elements=False, bgalpha=1,
legend=list(o.origin_marker.values()), colorbar=False, vmin=0, vmax=4)
/opt/conda/envs/opendrift/lib/python3.13/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'vmin', 'vmax' will be ignored
result = super().scatter(*args, **kwargs)
09:36:08 INFO opendrift:4609: Saving animation to /root/project/docs/source/gallery/animations/example_dominating_0.gif...
09:36:24 INFO opendrift:3062: Time to make animation: 0:00:37.931146
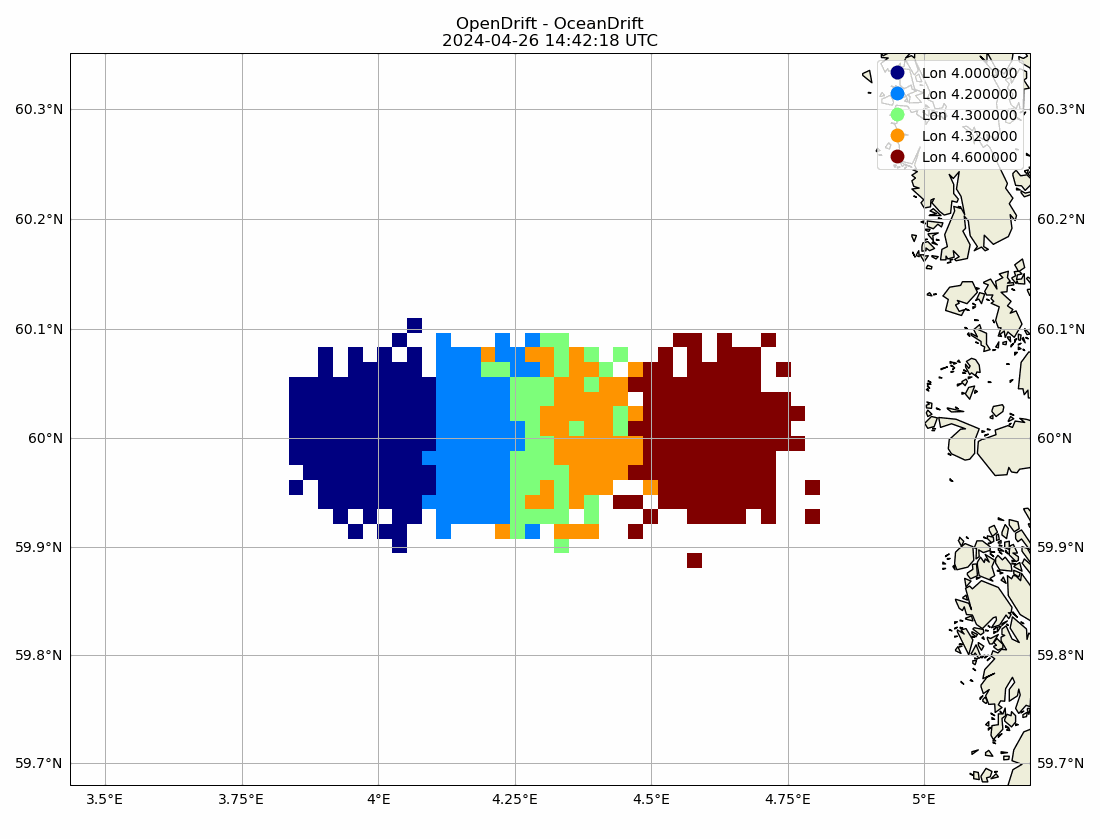
os.remove(of)
Total running time of the script: (0 minutes 51.740 seconds)