Note
Go to the end to download the full example code.
Biodegradation of oil at depth
import numpy as np
from datetime import datetime, timedelta
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.openoil import OpenOil
o = OpenOil(loglevel=0) # Set loglevel to 0 for debug information
time = datetime.now()
09:35:52 DEBUG opendrift.config:168: Adding 18 config items from __init__
09:35:52 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
09:35:52 DEBUG opendrift.config:168: Adding 6 config items from __init__
09:35:52 INFO opendrift:507: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-21-g950aeec)
09:35:52 DEBUG opendrift.config:168: Adding 17 config items from oceandrift
09:35:52 DEBUG opendrift.config:178: Overwriting config item seed:z
09:35:52 DEBUG opendrift.config:168: Adding 15 config items from openoil
Current from HYCOM and wind from NCEP GFS
o.add_readers_from_list([
'https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd',
'https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd'])
o.set_config('environment:constant:ocean_mixed_layer_thickness', 20)
o.set_config('drift', {'current_uncertainty': 0, 'wind_uncertainty': 0, 'horizontal_diffusivity': 20})
09:35:52 DEBUG opendrift.readers.reader_lazy:37: Delaying initialisation of LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:52 DEBUG opendrift.readers.reader_lazy:37: Delaying initialisation of LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:35:52 DEBUG opendrift.models.basemodel.environment:312: Added reader LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:52 DEBUG opendrift.models.basemodel.environment:312: Added reader LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:35:52 INFO opendrift.config:68: set_config('drift:current_uncertainty', 0)
09:35:52 INFO opendrift.config:68: set_config('drift:wind_uncertainty', 0)
09:35:52 INFO opendrift.config:68: set_config('drift:horizontal_diffusivity', 20)
Configuration
o.set_config('drift:vertical_mixing', True)
o.set_config('drift:vertical_mixing', True)
o.set_config('processes:biodegradation', True)
o.set_config('processes:dispersion', False)
o.set_config('biodegradation:method', 'half_time')
Fast decay for droplets, and slow decay for slick
kwargs = {'biodegradation_half_time_slick': 3, # days
'biodegradation_half_time_droplet': 1, # days
'oil_type': 'GENERIC MEDIUM CRUDE', 'm3_per_hour': .5, 'diameter': 8e-5} # small droplets
Seed oil at surface and at 150m depth
time = datetime.today() - timedelta(days=5)
lon = 23.5
lat = 35.0
o.seed_elements(lon=lon, lat=lat, z=0, radius=100, number=3000, time=time, **kwargs)
o.seed_elements(lon=lon, lat=lat, z=-150, radius=100, number=3000, time=time, **kwargs)
09:35:52 INFO opendrift.models.openoil.openoil:1625: Droplet diameter is provided, and will be kept constant during simulation
09:35:52 INFO opendrift.models.openoil.adios.dirjs:86: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
09:35:52 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
09:35:52 INFO opendrift.models.openoil.openoil:1712: Using density 877.5726099999999 and viscosity 4.5431401718650355e-05 of oiltype GENERIC MEDIUM CRUDE
09:35:52 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
09:35:52 DEBUG opendrift.models.basemodel.environment:312: Added reader constant_reader
09:35:52 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
09:35:52 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
09:35:56 DEBUG opendrift.models.basemodel.environment:312: Added reader global_landmask
09:35:56 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_height: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: upward_sea_water_velocity: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_ice_area_fraction: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_ice_x_velocity: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_ice_y_velocity: 0.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_water_temperature: 10.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_water_salinity: 34.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: sea_floor_depth_below_sea_level: 10000.000000
09:35:56 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.020000
09:35:56 DEBUG opendrift:100: Changed mode from Mode.Config to Mode.Ready
09:35:56 INFO opendrift.models.openoil.openoil:1625: Droplet diameter is provided, and will be kept constant during simulation
09:35:56 INFO opendrift.models.openoil.adios.dirjs:86: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
09:35:56 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
09:35:56 INFO opendrift.models.openoil.openoil:1712: Using density 877.5726099999999 and viscosity 4.5431401718650355e-05 of oiltype GENERIC MEDIUM CRUDE
Running model
o.run(duration=timedelta(days=5), time_step=3600)
09:35:56 DEBUG opendrift:100: Changed mode from Mode.Ready to Mode.Run
09:35:56 DEBUG opendrift:1813:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.14.2
Platform: Linux, 6.8.0-1029-aws
4.0 GB memory
36 processors (x86_64)
NumPy version 2.2.6
SciPy version 1.15.2
Matplotlib version 3.10.3
NetCDF4 version 1.7.2
Xarray version 2025.4.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 2.1.1
Python version 3.13.3 | packaged by conda-forge | (main, Apr 14 2025, 20:44:03) [GCC 13.3.0]
------------------------------------------------------
09:35:56 DEBUG opendrift:1827: No output file is specified, neglecting export_buffer_length
09:35:56 DEBUG opendrift:1945: Finalizing environment and preparing readers for simulation coverage ([np.float64(17.320116865357402), np.float64(29.937168348157726), np.float64(29.680066240111348), np.float64(40.06288505760399)]) and time (2025-05-23 09:35:52.207946 to 2025-05-28 09:35:52.207946)
09:35:56 DEBUG opendrift.models.basemodel.environment:168: Preparing LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd for extent [np.float64(17.320116865357402), np.float64(29.937168348157726), np.float64(29.680066240111348), np.float64(40.06288505760399)]
09:35:56 DEBUG opendrift.models.basemodel.environment:168: Preparing LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd for extent [np.float64(17.320116865357402), np.float64(29.937168348157726), np.float64(29.680066240111348), np.float64(40.06288505760399)]
09:35:56 DEBUG opendrift.models.basemodel.environment:168: Preparing constant_reader for extent [np.float64(17.320116865357402), np.float64(29.937168348157726), np.float64(29.680066240111348), np.float64(40.06288505760399)]
09:35:56 DEBUG opendrift.readers.basereader.variables:553: Nothing more to prepare for constant_reader
09:35:56 DEBUG opendrift.models.basemodel.environment:168: Preparing global_landmask for extent [np.float64(17.320116865357402), np.float64(29.937168348157726), np.float64(29.680066240111348), np.float64(40.06288505760399)]
09:35:56 DEBUG opendrift.readers.basereader.variables:553: Nothing more to prepare for global_landmask
09:35:56 DEBUG opendrift:2032: Initial self.result, size Frozen({'trajectory': 6000, 'time': 121})
09:35:56 INFO opendrift:911: Using existing reader for land_binary_mask
09:35:56 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:35:56 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:35:56 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:35:56 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:35:56 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:35:56 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:35:56 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:35:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:35:56 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:35:56 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:35:56 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:35:56 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:35:56 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:35:56 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:35:56 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:35:56 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:35:56 INFO opendrift:940: All points are in ocean
09:35:56 INFO opendrift.models.openoil.openoil:687: Oil-water surface tension is 0.031369 Nm
09:35:56 INFO opendrift.models.openoil.openoil:700: Max water fraction not available for GENERIC MEDIUM CRUDE, using default
09:35:56 DEBUG opendrift:872: to be seeded: 6000, already seeded 0
09:35:56 DEBUG opendrift:890: Released 6000 new elements.
09:35:56 DEBUG opendrift:2101: ======================================================================
09:35:56 INFO opendrift:2102: 2025-05-23 09:35:52.207946 - step 1 of 120 - 6000 active elements (0 deactivated)
09:35:56 DEBUG opendrift:2108: 0 elements scheduled.
09:35:56 DEBUG opendrift:2110: ======================================================================
09:35:56 DEBUG opendrift:2121: 34.99662780761719 <- latitude -> 35.00342559814453
09:35:56 DEBUG opendrift:2121: 23.4965763092041 <- longitude -> 23.50360679626465
09:35:56 DEBUG opendrift:2121: -150.0 <- z -> 0.0
09:35:56 DEBUG opendrift:2122: ---------------------------------
09:35:56 DEBUG opendrift.models.basemodel.environment:565: Variables not covered by any reader: ['sea_water_temperature', 'sea_water_salinity', 'y_wind', 'y_sea_water_velocity', 'x_wind', 'x_sea_water_velocity', 'sea_floor_depth_below_sea_level', 'sea_surface_height']
09:35:56 DEBUG opendrift.readers.reader_lazy:56: Initialising: LazyReader: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:56 DEBUG opendrift.readers:148: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
09:35:56 INFO opendrift.readers:61: Opening file with xr.open_dataset
09:35:56 DEBUG gribapi.bindings:58: eccodes lib search: trying to find binary wheel
09:35:56 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes.libs
09:35:56 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes/.dylibs
09:35:56 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes
09:35:56 DEBUG gribapi.bindings:91: eccodes lib search: did not find library from wheel; try to find as separate lib
09:35:56 DEBUG gribapi.bindings:99: eccodes lib search: findlibs returned /opt/conda/envs/opendrift/lib/libeccodes.so
09:35:57 WARNING opendrift.readers:67: Removing variables that cannot be CF decoded: ['tau']
09:35:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:128: Finding coordinate variables.
09:35:57 INFO opendrift.readers.reader_netCDF_CF_generic:299: Grid coordinates are detected, but proj4 string not given: assuming latlong
09:35:57 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'z': 'depth', 'y': 'lat', 'x': 'lon', 'time': 'time'}
09:35:57 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 9 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 5 m/s and time span of 3:00:00
09:35:57 INFO opendrift.readers.basereader:176: Variable y_sea_water_velocity will be rotated from northward_sea_water_velocity
09:35:57 INFO opendrift.readers.basereader:176: Variable x_sea_water_velocity will be rotated from eastward_sea_water_velocity
09:35:57 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
09:35:57 DEBUG opendrift.readers.basereader.variables:567: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
09:35:57 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd before starting new simulation
09:35:57 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 4 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
09:35:57 DEBUG opendrift.readers.basereader.variables:553: Nothing more to prepare for https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:57 DEBUG opendrift.readers.reader_lazy:71: Reader initialised: https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:57 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 4 for reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
09:35:57 DEBUG opendrift.readers.reader_lazy:56: Initialising: LazyReader: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:35:57 DEBUG opendrift.readers:148: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
09:35:57 INFO opendrift.readers:61: Opening file with xr.open_dataset
09:35:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:128: Finding coordinate variables.
09:35:59 DEBUG opendrift.readers.reader_netCDF_CF_generic:262: Lon and lat are 1D arrays - using as projection coordinates
09:35:59 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'time': 'time', 'x': 'longitude', 'y': 'latitude'}
09:35:59 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 3 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 5 m/s and time span of 3:00:00
09:35:59 INFO opendrift.readers.basereader:176: Variable x_wind will be rotated from eastward_wind
09:35:59 INFO opendrift.readers.basereader:176: Variable y_wind will be rotated from northward_wind
09:35:59 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
09:35:59 DEBUG opendrift.readers.basereader.variables:567: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
09:35:59 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd before starting new simulation
09:35:59 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 3 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
09:35:59 DEBUG opendrift.readers.basereader.variables:553: Nothing more to prepare for https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:35:59 DEBUG opendrift.readers.reader_lazy:71: Reader initialised: https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:35:59 DEBUG opendrift.readers.basereader.variables:612: Setting buffer size 3 for reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd, assuming a maximum average speed of 1.3 m/s and time span of 3:00:00
09:35:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:35:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:35:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:35:59 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:35:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:35:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:35:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:35:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:35:59 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:35:59 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:35:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:35:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:35:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:35:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:35:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:35:59 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:35:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:35:59 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:00 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 9x9x23) for time before (2025-05-23 09:00:00)
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:00 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x9x23) for time after (2025-05-23 12:00:00)
09:36:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:00 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:00 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.80) and
after (2025-05-23 12:00:00, weight 0.20) in time
09:36:00 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:00 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:00 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:00 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:00 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:00 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:00 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:00 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:00 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:00 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:00 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 8x7x1) for time before (2025-05-23 09:00:00)
09:36:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:01 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:01 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:01 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:01 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:01 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-23 12:00:00)
09:36:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.80) and
after (2025-05-23 12:00:00, weight 0.20) in time
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:01 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.141866 (min) -0.133788 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.156775 (min) 0.208027 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: 1.72463 (min) 1.76839 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.95114 (min) -0.914857 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:01 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.094874, mean: 0.096469, max: 0.098429
09:36:01 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:01 DEBUG opendrift.models.physics_methods:917: min: 1.678043, mean: 1.692083, max: 1.709195
09:36:01 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.678043, mean: 1.692083, max: 1.709195
09:36:01 DEBUG opendrift:648: No elements hit coastline.
09:36:01 DEBUG opendrift:1745: No elements to deactivate
09:36:01 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:01 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:01 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:01 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:01 DEBUG opendrift.models.openoil.openoil:861: Emulsification not yet started
09:36:01 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:01 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:01 DEBUG opendrift.models.physics_methods:917: min: 1.678043, mean: 1.692083, max: 1.709195
09:36:01 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:01 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004149, dN_50: 0.000326
09:36:01 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:01 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.001447889127079235
09:36:01 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:01 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:01 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:01 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.058925 m/s - 0.060009 m/s)
09:36:01 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:01 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022460362237508094 and 0.48135814700051643 m/s
09:36:01 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:01 DEBUG opendrift:2101: ======================================================================
09:36:01 INFO opendrift:2102: 2025-05-23 10:35:52.207946 - step 2 of 120 - 6000 active elements (0 deactivated)
09:36:01 DEBUG opendrift:2108: 0 elements scheduled.
09:36:01 DEBUG opendrift:2110: ======================================================================
09:36:01 DEBUG opendrift:2121: 34.99147827762667 <- latitude -> 35.019811716579426
09:36:01 DEBUG opendrift:2121: 23.478881709517808 <- longitude -> 23.51448509300957
09:36:01 DEBUG opendrift:2121: -150.14817558138057 <- z -> 0.0
09:36:01 DEBUG opendrift:2122: ---------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:01 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:36:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.47) and
after (2025-05-23 12:00:00, weight 0.53) in time
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:36:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.47) and
after (2025-05-23 12:00:00, weight 0.53) in time
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:01 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.16366 (min) -0.112599 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.142842 (min) 0.196466 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.78652 (min) 0.964047 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.041582 (min) 0.192924 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:01 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.015304, mean: 0.018246, max: 0.023004
09:36:01 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:01 DEBUG opendrift.models.physics_methods:917: min: 0.673961, mean: 0.735643, max: 0.826294
09:36:01 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.673961, mean: 0.735643, max: 0.826294
09:36:01 DEBUG opendrift:648: No elements hit coastline.
09:36:01 DEBUG opendrift:1745: No elements to deactivate
09:36:01 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:01 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:01 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:01 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:01 DEBUG opendrift.models.openoil.openoil:861: Emulsification not yet started
09:36:01 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:01 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:01 DEBUG opendrift.models.physics_methods:917: min: 0.673961, mean: 0.735643, max: 0.826294
09:36:01 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:01 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.011278, dN_50: 0.000885
09:36:01 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:01 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.000341611196202579
09:36:01 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:01 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:01 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:01 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.023662 m/s - 0.029011 m/s)
09:36:01 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:01 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006187029221147531 and 0.45730975932548934 m/s
09:36:01 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:01 DEBUG opendrift:2101: ======================================================================
09:36:01 INFO opendrift:2102: 2025-05-23 11:35:52.207946 - step 3 of 120 - 6000 active elements (0 deactivated)
09:36:01 DEBUG opendrift:2108: 0 elements scheduled.
09:36:01 DEBUG opendrift:2110: ======================================================================
09:36:01 DEBUG opendrift:2121: 34.992244099940585 <- latitude -> 35.03021742879759
09:36:01 DEBUG opendrift:2121: 23.469641239368187 <- longitude -> 23.51695597919493
09:36:01 DEBUG opendrift:2121: -149.66531192119777 <- z -> 0.0
09:36:01 DEBUG opendrift:2122: ---------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:01 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:36:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.13) and
after (2025-05-23 12:00:00, weight 0.87) in time
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 09:00:00 (before)
2025-05-23 12:00:00 (after)
09:36:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 09:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 12:00:00) in space (linearNDFast)
09:36:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 09:00:00, weight 0.13) and
after (2025-05-23 12:00:00, weight 0.87) in time
09:36:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:01 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.185821 (min) -0.0968097 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.132239 (min) 0.184585 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.132903 (min) 0.167742 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.02725 (min) 1.20464 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:01 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.025961, mean: 0.029483, max: 0.036390
09:36:01 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:01 DEBUG opendrift.models.physics_methods:917: min: 0.877793, mean: 0.935116, max: 1.039258
09:36:01 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.877793, mean: 0.935116, max: 1.039258
09:36:01 DEBUG opendrift:648: No elements hit coastline.
09:36:01 DEBUG opendrift:1745: No elements to deactivate
09:36:01 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:01 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:01 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:02 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:02 DEBUG opendrift.models.openoil.openoil:861: Emulsification not yet started
09:36:02 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:02 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:02 DEBUG opendrift.models.physics_methods:917: min: 0.877793, mean: 0.935116, max: 1.039258
09:36:02 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:02 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008550, dN_50: 0.000671
09:36:02 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:02 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.000537948607421273
09:36:02 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:02 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:02 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:02 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.030819 m/s - 0.035496 m/s)
09:36:02 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:02 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017541404241848248 and 0.49065842250043995 m/s
09:36:02 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:02 DEBUG opendrift:2101: ======================================================================
09:36:02 INFO opendrift:2102: 2025-05-23 12:35:52.207946 - step 4 of 120 - 6000 active elements (0 deactivated)
09:36:02 DEBUG opendrift:2108: 0 elements scheduled.
09:36:02 DEBUG opendrift:2110: ======================================================================
09:36:02 DEBUG opendrift:2121: 34.991289645544555 <- latitude -> 35.039702430098764
09:36:02 DEBUG opendrift:2121: 23.45964195281027 <- longitude -> 23.512420897186285
09:36:02 DEBUG opendrift:2121: -149.0134531272703 <- z -> 0.0
09:36:02 DEBUG opendrift:2122: ---------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:02 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:02 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:02 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:02 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x23) for time after (2025-05-23 15:00:00)
09:36:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.80) and
after (2025-05-23 15:00:00, weight 0.20) in time
09:36:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:02 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:02 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:02 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:02 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-23 15:00:00)
09:36:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.80) and
after (2025-05-23 15:00:00, weight 0.20) in time
09:36:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:02 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:02 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:02 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:02 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:02 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.201439 (min) -0.0858987 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.137914 (min) 0.173295 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.686225 (min) -0.26469 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.62162 (min) 1.76876 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:02 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:02 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.070302, mean: 0.076305, max: 0.079629
09:36:02 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:02 DEBUG opendrift.models.physics_methods:917: min: 1.444481, mean: 1.504876, max: 1.537320
09:36:02 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.444481, mean: 1.504876, max: 1.537320
09:36:02 DEBUG opendrift:648: No elements hit coastline.
09:36:03 DEBUG opendrift:1745: No elements to deactivate
09:36:03 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:03 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:03 DEBUG opendrift.models.physics_methods:917: min: 1.444481, mean: 1.504876, max: 1.537320
09:36:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004931, dN_50: 0.000387
09:36:03 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:03 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0011721347086576326
09:36:03 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:03 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:03 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:03 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.050715 m/s - 0.053724 m/s)
09:36:03 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:03 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015934277674514868 and 0.4558445351294395 m/s
09:36:03 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:03 DEBUG opendrift:2101: ======================================================================
09:36:03 INFO opendrift:2102: 2025-05-23 13:35:52.207946 - step 5 of 120 - 6000 active elements (0 deactivated)
09:36:03 DEBUG opendrift:2108: 0 elements scheduled.
09:36:03 DEBUG opendrift:2110: ======================================================================
09:36:03 DEBUG opendrift:2121: 34.992090417217 <- latitude -> 35.05516098305346
09:36:03 DEBUG opendrift:2121: 23.44937165411941 <- longitude -> 23.51105053934565
09:36:03 DEBUG opendrift:2121: -148.0144108880677 <- z -> 0.0
09:36:03 DEBUG opendrift:2122: ---------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:03 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.47) and
after (2025-05-23 15:00:00, weight 0.53) in time
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.47) and
after (2025-05-23 15:00:00, weight 0.53) in time
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:03 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.215514 (min) -0.078829 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.113028 (min) 0.211062 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.965926 (min) -0.455568 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.94887 (min) 2.0455 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:03 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.106441, mean: 0.113658, max: 0.121422
09:36:03 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:03 DEBUG opendrift.models.physics_methods:917: min: 1.777399, mean: 1.836610, max: 1.898358
09:36:03 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.777399, mean: 1.836610, max: 1.898358
09:36:03 DEBUG opendrift:648: No elements hit coastline.
09:36:03 DEBUG opendrift:1745: No elements to deactivate
09:36:03 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:03 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:03 DEBUG opendrift.models.physics_methods:917: min: 1.777399, mean: 1.836610, max: 1.898358
09:36:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003950, dN_50: 0.000310
09:36:03 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:03 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0017851298267540306
09:36:03 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:03 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:03 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:03 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.062404 m/s - 0.066650 m/s)
09:36:03 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:03 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0036340290906254212 and 0.465993503464017 m/s
09:36:03 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:03 DEBUG opendrift:2101: ======================================================================
09:36:03 INFO opendrift:2102: 2025-05-23 14:35:52.207946 - step 6 of 120 - 6000 active elements (0 deactivated)
09:36:03 DEBUG opendrift:2108: 0 elements scheduled.
09:36:03 DEBUG opendrift:2110: ======================================================================
09:36:03 DEBUG opendrift:2121: 34.99877117371139 <- latitude -> 35.06639340860542
09:36:03 DEBUG opendrift:2121: 23.43948088120998 <- longitude -> 23.510570012877146
09:36:03 DEBUG opendrift:2121: -147.3759126099074 <- z -> 0.0
09:36:03 DEBUG opendrift:2122: ---------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:03 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.13) and
after (2025-05-23 15:00:00, weight 0.87) in time
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 12:00:00 (before)
2025-05-23 15:00:00 (after)
09:36:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 12:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 15:00:00) in space (linearNDFast)
09:36:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 12:00:00, weight 0.13) and
after (2025-05-23 15:00:00, weight 0.87) in time
09:36:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:03 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.222386 (min) -0.0755946 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0810558 (min) 0.250864 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.24231 (min) -0.671768 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.23589 (min) 2.39469 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:03 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.135224, mean: 0.156745, max: 0.176637
09:36:03 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:03 DEBUG opendrift.models.physics_methods:917: min: 2.003348, mean: 2.156636, max: 2.289656
09:36:03 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.003348, mean: 2.156636, max: 2.289656
09:36:03 DEBUG opendrift:648: No elements hit coastline.
09:36:03 DEBUG opendrift:1745: No elements to deactivate
09:36:03 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:03 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:03 DEBUG opendrift.models.physics_methods:917: min: 2.003348, mean: 2.156636, max: 2.289656
09:36:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003312, dN_50: 0.000260
09:36:03 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:03 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0025949810621452487
09:36:03 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:03 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:03 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:04 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.070336 m/s - 0.080181 m/s)
09:36:04 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:04 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.004161841768363598 and 0.445781632089122 m/s
09:36:04 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:04 DEBUG opendrift:2101: ======================================================================
09:36:04 INFO opendrift:2102: 2025-05-23 15:35:52.207946 - step 7 of 120 - 6000 active elements (0 deactivated)
09:36:04 DEBUG opendrift:2108: 0 elements scheduled.
09:36:04 DEBUG opendrift:2110: ======================================================================
09:36:04 DEBUG opendrift:2121: 34.99975999992668 <- latitude -> 35.07865198071498
09:36:04 DEBUG opendrift:2121: 23.427783528737116 <- longitude -> 23.507501262508804
09:36:04 DEBUG opendrift:2121: -146.3712181938332 <- z -> 0.0
09:36:04 DEBUG opendrift:2122: ---------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:04 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:06 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:06 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:06 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x10x23) for time after (2025-05-23 18:00:00)
09:36:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:06 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:06 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.80) and
after (2025-05-23 18:00:00, weight 0.20) in time
09:36:06 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:06 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:07 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:07 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:07 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:07 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:07 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-23 18:00:00)
09:36:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.80) and
after (2025-05-23 18:00:00, weight 0.20) in time
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:07 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.2213 (min) -0.0862907 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0666723 (min) 0.279872 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.24985 (min) -1.58661 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.09606 (min) 2.33078 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:07 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.170006, mean: 0.215126, max: 0.255380
09:36:07 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:07 DEBUG opendrift.models.physics_methods:917: min: 2.246267, mean: 2.526134, max: 2.753105
09:36:07 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.246267, mean: 2.526134, max: 2.753105
09:36:07 DEBUG opendrift:648: No elements hit coastline.
09:36:07 DEBUG opendrift:1745: No elements to deactivate
09:36:07 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:07 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:07 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:07 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:07 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:07 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:07 DEBUG opendrift.models.physics_methods:917: min: 2.246267, mean: 2.526134, max: 2.753104
09:36:07 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:07 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002790, dN_50: 0.000219
09:36:07 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:07 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0037499242108244325
09:36:07 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:07 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:07 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:07 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.078865 m/s - 0.096329 m/s)
09:36:07 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:07 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010419219817162152 and 0.4256272139196229 m/s
09:36:07 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:07 DEBUG opendrift:2101: ======================================================================
09:36:07 INFO opendrift:2102: 2025-05-23 16:35:52.207946 - step 8 of 120 - 6000 active elements (0 deactivated)
09:36:07 DEBUG opendrift:2108: 0 elements scheduled.
09:36:07 DEBUG opendrift:2110: ======================================================================
09:36:07 DEBUG opendrift:2121: 35.00241369874268 <- latitude -> 35.094127226359554
09:36:07 DEBUG opendrift:2121: 23.416199317162977 <- longitude -> 23.504281213975606
09:36:07 DEBUG opendrift:2121: -145.92395360634313 <- z -> 0.0
09:36:07 DEBUG opendrift:2122: ---------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:07 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.47) and
after (2025-05-23 18:00:00, weight 0.53) in time
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.47) and
after (2025-05-23 18:00:00, weight 0.53) in time
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:07 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.21538 (min) -0.107658 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0550143 (min) 0.301934 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.72608 (min) -2.9517 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.73277 (min) 1.98273 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:07 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.288190, mean: 0.366162, max: 0.432029
09:36:07 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:07 DEBUG opendrift.models.physics_methods:917: min: 2.924617, mean: 3.295227, max: 3.580851
09:36:07 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.924617, mean: 3.295227, max: 3.580851
09:36:07 DEBUG opendrift:648: No elements hit coastline.
09:36:07 DEBUG opendrift:1745: No elements to deactivate
09:36:07 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:07 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:07 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:07 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:07 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:07 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:07 DEBUG opendrift.models.physics_methods:917: min: 2.924617, mean: 3.295227, max: 3.580851
09:36:07 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:07 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002093, dN_50: 0.000164
09:36:07 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:07 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.00634089587651932
09:36:07 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:07 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:07 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:07 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.102682 m/s - 0.123691 m/s)
09:36:07 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:07 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018810156762602741 and 0.40454949578149074 m/s
09:36:07 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:07 DEBUG opendrift:2101: ======================================================================
09:36:07 INFO opendrift:2102: 2025-05-23 17:35:52.207946 - step 9 of 120 - 6000 active elements (0 deactivated)
09:36:07 DEBUG opendrift:2108: 0 elements scheduled.
09:36:07 DEBUG opendrift:2110: ======================================================================
09:36:07 DEBUG opendrift:2121: 35.00388212948274 <- latitude -> 35.10091434353616
09:36:07 DEBUG opendrift:2121: 23.399263684250137 <- longitude -> 23.50146344481887
09:36:07 DEBUG opendrift:2121: -145.02889915466807 <- z -> 0.0
09:36:07 DEBUG opendrift:2122: ---------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:07 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.13) and
after (2025-05-23 18:00:00, weight 0.87) in time
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 15:00:00 (before)
2025-05-23 18:00:00 (after)
09:36:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 15:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 18:00:00) in space (linearNDFast)
09:36:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 15:00:00, weight 0.13) and
after (2025-05-23 18:00:00, weight 0.87) in time
09:36:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:07 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.214035 (min) -0.127136 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0473845 (min) 0.333988 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.23799 (min) -4.38571 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.37753 (min) 1.6116 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:07 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.519849, mean: 0.622248, max: 0.736801
09:36:07 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:07 DEBUG opendrift.models.physics_methods:917: min: 3.927969, mean: 4.295388, max: 4.676326
09:36:07 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.927969, mean: 4.295388, max: 4.676326
09:36:07 DEBUG opendrift:648: No elements hit coastline.
09:36:07 DEBUG opendrift:1745: No elements to deactivate
09:36:07 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:07 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:07 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:08 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:08 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:08 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:08 DEBUG opendrift.models.physics_methods:917: min: 3.927969, mean: 4.295388, max: 4.676326
09:36:08 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:08 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001592, dN_50: 0.000125
09:36:08 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:08 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.010811070465828035
09:36:08 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:08 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:08 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:08 DEBUG opendrift.models.physics_methods:771: Advecting 3000 of 6000 elements above 0.100m with wind-sheared ocean current (0.137909 m/s - 0.158094 m/s)
09:36:08 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:08 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00030840357758222376 and 0.4484315123261148 m/s
09:36:08 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:08 DEBUG opendrift:2101: ======================================================================
09:36:08 INFO opendrift:2102: 2025-05-23 18:35:52.207946 - step 10 of 120 - 6000 active elements (0 deactivated)
09:36:08 DEBUG opendrift:2108: 0 elements scheduled.
09:36:08 DEBUG opendrift:2110: ======================================================================
09:36:08 DEBUG opendrift:2121: 35.00105052356092 <- latitude -> 35.111175533608694
09:36:08 DEBUG opendrift:2121: 23.38386058351853 <- longitude -> 23.49789539899677
09:36:08 DEBUG opendrift:2121: -143.88015790391924 <- z -> 0.0
09:36:08 DEBUG opendrift:2122: ---------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:08 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:08 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:08 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:08 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:08 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:08 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x11x23) for time after (2025-05-23 21:00:00)
09:36:08 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:08 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:08 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.80) and
after (2025-05-23 21:00:00, weight 0.20) in time
09:36:08 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:08 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:08 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:08 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:08 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-23 21:00:00)
09:36:08 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:08 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:08 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:08 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.80) and
after (2025-05-23 21:00:00, weight 0.20) in time
09:36:08 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:08 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:08 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:08 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:08 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.205104 (min) -0.144527 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0508157 (min) 0.374658 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.84717 (min) -4.98942 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.48876 (min) 1.81692 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:08 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.668704, mean: 0.791979, max: 0.920577
09:36:08 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:08 DEBUG opendrift.models.physics_methods:917: min: 4.454989, mean: 4.845759, max: 5.227086
09:36:08 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.454989, mean: 4.845759, max: 5.227086
09:36:08 DEBUG opendrift:648: No elements hit coastline.
09:36:09 DEBUG opendrift:1745: No elements to deactivate
09:36:09 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:09 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:09 DEBUG opendrift.models.physics_methods:917: min: 4.454989, mean: 4.845759, max: 5.227087
09:36:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001447, dN_50: 0.000114
09:36:09 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:09 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.013506560744809517
09:36:09 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:09 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:09 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 3000 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2999 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2998 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2997 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2994 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2993 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2992 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2991 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2988 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2987 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2986 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2985 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2984 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2983 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2982 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2981 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2980 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2979 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2977 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2975 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2974 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2971 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2969 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2969 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2969 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2966 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2966 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2965 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2963 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2962 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2961 surface elements
09:36:09 DEBUG opendrift.models.physics_methods:771: Advecting 2960 of 6000 elements above 0.100m with wind-sheared ocean current (0.156412 m/s - 0.174636 m/s)
09:36:09 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:09 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018802227484577855 and 0.4732995180827738 m/s
09:36:09 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:09 DEBUG opendrift:2101: ======================================================================
09:36:09 INFO opendrift:2102: 2025-05-23 19:35:52.207946 - step 11 of 120 - 6000 active elements (0 deactivated)
09:36:09 DEBUG opendrift:2108: 0 elements scheduled.
09:36:09 DEBUG opendrift:2110: ======================================================================
09:36:09 DEBUG opendrift:2121: 34.9980651997557 <- latitude -> 35.12290272138843
09:36:09 DEBUG opendrift:2121: 23.366624104319776 <- longitude -> 23.496788730748783
09:36:09 DEBUG opendrift:2121: -143.11508365828539 <- z -> 0.0
09:36:09 DEBUG opendrift:2122: ---------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:09 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.47) and
after (2025-05-23 21:00:00, weight 0.53) in time
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.47) and
after (2025-05-23 21:00:00, weight 0.53) in time
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:09 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.208571 (min) -0.101285 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0633589 (min) 0.421803 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.98776 (min) -5.09728 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.93488 (min) 2.40975 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:09 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.731261, mean: 0.870970, max: 1.024841
09:36:09 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:09 DEBUG opendrift.models.physics_methods:917: min: 4.658711, mean: 5.081725, max: 5.515157
09:36:09 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.658711, mean: 5.081725, max: 5.515157
09:36:09 DEBUG opendrift:648: No elements hit coastline.
09:36:09 DEBUG opendrift:1745: No elements to deactivate
09:36:09 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:09 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:09 DEBUG opendrift.models.physics_methods:917: min: 4.658711, mean: 5.081725, max: 5.515157
09:36:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001446, dN_50: 0.000113
09:36:09 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:09 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.015035827890938932
09:36:09 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:09 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:09 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2960 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2958 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2957 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2955 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2954 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2953 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2950 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2949 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2949 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2947 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2945 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2944 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2943 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2942 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2940 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2939 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2938 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2936 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2934 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2934 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2932 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2931 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2929 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2928 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2927 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2926 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2924 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2924 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2923 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2922 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2921 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2920 surface elements
09:36:09 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2918 surface elements
09:36:09 DEBUG opendrift.models.physics_methods:771: Advecting 2917 of 6000 elements above 0.100m with wind-sheared ocean current (0.163565 m/s - 0.182095 m/s)
09:36:09 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:09 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002081735626850855 and 0.4552286743614181 m/s
09:36:09 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:09 DEBUG opendrift:2101: ======================================================================
09:36:09 INFO opendrift:2102: 2025-05-23 20:35:52.207946 - step 12 of 120 - 6000 active elements (0 deactivated)
09:36:09 DEBUG opendrift:2108: 0 elements scheduled.
09:36:09 DEBUG opendrift:2110: ======================================================================
09:36:09 DEBUG opendrift:2121: 34.99591777083822 <- latitude -> 35.13815250460157
09:36:09 DEBUG opendrift:2121: 23.351434083233116 <- longitude -> 23.4922241119315
09:36:09 DEBUG opendrift:2121: -142.10978325615676 <- z -> 0.0
09:36:09 DEBUG opendrift:2122: ---------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:09 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.13) and
after (2025-05-23 21:00:00, weight 0.87) in time
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 18:00:00 (before)
2025-05-23 21:00:00 (after)
09:36:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 18:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-23 21:00:00) in space (linearNDFast)
09:36:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 18:00:00, weight 0.13) and
after (2025-05-23 21:00:00, weight 0.87) in time
09:36:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:09 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.229729 (min) -0.0498685 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.076489 (min) 0.464268 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.15102 (min) -5.21202 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.35609 (min) 3.03271 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:09 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.804822, mean: 0.970589, max: 1.156997
09:36:09 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:09 DEBUG opendrift.models.physics_methods:917: min: 4.887419, mean: 5.364605, max: 5.859973
09:36:09 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.887419, mean: 5.364605, max: 5.859973
09:36:09 DEBUG opendrift:648: No elements hit coastline.
09:36:09 DEBUG opendrift:1745: No elements to deactivate
09:36:09 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:09 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:09 DEBUG opendrift.models.physics_methods:917: min: 4.887419, mean: 5.364605, max: 5.859972
09:36:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001451, dN_50: 0.000114
09:36:13 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:13 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.016974189749342047
09:36:13 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:13 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:13 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2917 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2915 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2913 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2912 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2907 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2906 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2903 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2900 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2898 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2896 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2894 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2893 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2892 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2889 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2888 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2887 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2885 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2880 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2878 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2874 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2873 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2871 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2870 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2869 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2868 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2865 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2861 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2860 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2859 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2858 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2857 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2853 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2852 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2850 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2849 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2848 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2847 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2844 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2843 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2843 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2842 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2841 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2840 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2837 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2834 surface elements
09:36:13 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2833 surface elements
09:36:13 DEBUG opendrift.models.physics_methods:771: Advecting 2834 of 6000 elements above 0.100m with wind-sheared ocean current (0.043574 m/s - 0.193625 m/s)
09:36:13 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:13 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016644752455382337 and 0.45041203473427394 m/s
09:36:13 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:13 DEBUG opendrift:2101: ======================================================================
09:36:13 INFO opendrift:2102: 2025-05-23 21:35:52.207946 - step 13 of 120 - 6000 active elements (0 deactivated)
09:36:13 DEBUG opendrift:2108: 0 elements scheduled.
09:36:13 DEBUG opendrift:2110: ======================================================================
09:36:13 DEBUG opendrift:2121: 35.00015763977233 <- latitude -> 35.159040117321645
09:36:13 DEBUG opendrift:2121: 23.336034910217446 <- longitude -> 23.48860642937221
09:36:13 DEBUG opendrift:2121: -141.31508122260274 <- z -> 0.0
09:36:13 DEBUG opendrift:2122: ---------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:13 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:13 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:13 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:13 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:13 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:13 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:13 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 11x12x23) for time after (2025-05-24 00:00:00)
09:36:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.80) and
after (2025-05-24 00:00:00, weight 0.20) in time
09:36:13 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:13 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:13 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:13 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:13 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-24 00:00:00)
09:36:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.80) and
after (2025-05-24 00:00:00, weight 0.20) in time
09:36:13 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:13 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:13 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.234507 (min) 0.0077768 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0909396 (min) 0.47181 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.34636 (min) -5.31352 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.93242 (min) 3.51792 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.912705, mean: 1.089245, max: 1.285874
09:36:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 5.204689, mean: 5.683393, max: 6.177728
09:36:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.204689, mean: 5.683393, max: 6.177728
09:36:14 DEBUG opendrift:648: No elements hit coastline.
09:36:14 DEBUG opendrift:1745: No elements to deactivate
09:36:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 5.204689, mean: 5.683393, max: 6.177728
09:36:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001469, dN_50: 0.000115
09:36:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.018864472798840293
09:36:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2832 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2829 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2828 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2827 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2826 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2824 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2822 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2820 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2817 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2815 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2813 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2811 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2808 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2807 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2806 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2805 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2803 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2799 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2796 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2793 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2791 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2790 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2787 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2786 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2785 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2784 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2779 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2775 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2774 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2773 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2771 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2769 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2768 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2767 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2764 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2761 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2758 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2757 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2753 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2749 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2747 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2745 surface elements
09:36:14 DEBUG opendrift.models.physics_methods:771: Advecting 2744 of 6000 elements above 0.100m with wind-sheared ocean current (0.182734 m/s - 0.206359 m/s)
09:36:14 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:14 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002381379748994719 and 0.42621304252069175 m/s
09:36:14 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:14 DEBUG opendrift:2101: ======================================================================
09:36:14 INFO opendrift:2102: 2025-05-23 22:35:52.207946 - step 14 of 120 - 6000 active elements (0 deactivated)
09:36:14 DEBUG opendrift:2108: 0 elements scheduled.
09:36:14 DEBUG opendrift:2110: ======================================================================
09:36:14 DEBUG opendrift:2121: 35.00261956749863 <- latitude -> 35.18208801101191
09:36:14 DEBUG opendrift:2121: 23.32985847305003 <- longitude -> 23.48446435727254
09:36:14 DEBUG opendrift:2121: -140.5894324748975 <- z -> 0.0
09:36:14 DEBUG opendrift:2122: ---------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:14 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.47) and
after (2025-05-24 00:00:00, weight 0.53) in time
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.47) and
after (2025-05-24 00:00:00, weight 0.53) in time
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:14 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.236717 (min) 0.0377163 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.103002 (min) 0.464736 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.55138 (min) -5.35306 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.44805 (min) 4.17926 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.066298, mean: 1.230879, max: 1.417775
09:36:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 5.625600, mean: 6.042481, max: 6.486840
09:36:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.625600, mean: 6.042481, max: 6.486840
09:36:14 DEBUG opendrift:648: No elements hit coastline.
09:36:14 DEBUG opendrift:1745: No elements to deactivate
09:36:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 5.625600, mean: 6.042481, max: 6.486840
09:36:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001502, dN_50: 0.000118
09:36:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02079909574275755
09:36:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2744 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2738 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2734 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2733 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2730 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2726 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2723 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2721 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2718 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2716 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2715 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2712 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2709 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2708 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2706 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2705 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2701 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2699 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2697 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2696 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2695 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2693 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2691 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2691 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2690 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2687 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2686 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2684 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2683 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2681 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2679 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2676 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2673 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2671 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2669 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2666 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2660 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2655 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2653 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2648 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2647 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2645 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2643 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2640 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2640 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2639 surface elements
09:36:14 DEBUG opendrift.models.physics_methods:771: Advecting 2639 of 6000 elements above 0.100m with wind-sheared ocean current (0.027691 m/s - 0.218696 m/s)
09:36:14 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:14 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010137294541979057 and 0.46543462203736635 m/s
09:36:14 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:14 DEBUG opendrift:2101: ======================================================================
09:36:14 INFO opendrift:2102: 2025-05-23 23:35:52.207946 - step 15 of 120 - 6000 active elements (0 deactivated)
09:36:14 DEBUG opendrift:2108: 0 elements scheduled.
09:36:14 DEBUG opendrift:2110: ======================================================================
09:36:14 DEBUG opendrift:2121: 35.000235330316215 <- latitude -> 35.19945696858622
09:36:14 DEBUG opendrift:2121: 23.318887066929165 <- longitude -> 23.484755882978373
09:36:14 DEBUG opendrift:2121: -139.94721206193483 <- z -> 0.0
09:36:14 DEBUG opendrift:2122: ---------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:14 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.13) and
after (2025-05-24 00:00:00, weight 0.87) in time
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-23 21:00:00 (before)
2025-05-24 00:00:00 (after)
09:36:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-23 21:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 00:00:00) in space (linearNDFast)
09:36:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-23 21:00:00, weight 0.13) and
after (2025-05-24 00:00:00, weight 0.87) in time
09:36:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:14 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:14 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:14 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.236294 (min) 0.0819559 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.110486 (min) 0.459503 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.7913 (min) -5.33951 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.92167 (min) 5.08775 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.264009, mean: 1.402820, max: 1.560922
09:36:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 6.124981, mean: 6.451699, max: 6.806443
09:36:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.124981, mean: 6.451699, max: 6.806443
09:36:14 DEBUG opendrift:648: No elements hit coastline.
09:36:14 DEBUG opendrift:1745: No elements to deactivate
09:36:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:14 DEBUG opendrift.models.physics_methods:917: min: 6.124981, mean: 6.451699, max: 6.806443
09:36:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001554, dN_50: 0.000122
09:36:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.022898676914323516
09:36:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2638 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2634 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2630 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2629 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2628 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2625 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2624 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2622 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2619 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2617 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2614 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2612 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2610 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2607 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2603 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2601 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2598 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2596 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2594 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2590 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2585 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2580 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2578 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2576 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2571 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2567 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2565 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2563 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2561 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2559 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2556 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2555 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2553 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2549 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2547 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2544 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2541 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2540 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2539 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2537 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2536 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2535 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2530 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2529 surface elements
09:36:14 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2527 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2526 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2525 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2524 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2523 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2522 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2521 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2518 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2515 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2513 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2511 surface elements
09:36:15 DEBUG opendrift.models.physics_methods:771: Advecting 2512 of 6000 elements above 0.100m with wind-sheared ocean current (0.012808 m/s - 0.233351 m/s)
09:36:15 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:15 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005100047373104568 and 0.448816599910058 m/s
09:36:15 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:15 DEBUG opendrift:2101: ======================================================================
09:36:15 INFO opendrift:2102: 2025-05-24 00:35:52.207946 - step 16 of 120 - 6000 active elements (0 deactivated)
09:36:15 DEBUG opendrift:2108: 0 elements scheduled.
09:36:15 DEBUG opendrift:2110: ======================================================================
09:36:15 DEBUG opendrift:2121: 35.00391227388803 <- latitude -> 35.21967037298528
09:36:15 DEBUG opendrift:2121: 23.30869442314397 <- longitude -> 23.477518848023273
09:36:15 DEBUG opendrift:2121: -138.88837627027803 <- z -> 0.0
09:36:15 DEBUG opendrift:2122: ---------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:15 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 11x13x23) for time after (2025-05-24 03:00:00)
09:36:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.80) and
after (2025-05-24 03:00:00, weight 0.20) in time
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-24 03:00:00)
09:36:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.80) and
after (2025-05-24 03:00:00, weight 0.20) in time
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:15 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.225552 (min) 0.072551 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.120074 (min) 0.443255 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.53272 (min) -5.86503 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.90178 (min) 5.61098 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:15 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.481994, mean: 1.653562, max: 1.810727
09:36:15 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:15 DEBUG opendrift.models.physics_methods:917: min: 6.632127, mean: 7.004854, max: 7.330880
09:36:15 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.632127, mean: 7.004854, max: 7.330880
09:36:15 DEBUG opendrift:648: No elements hit coastline.
09:36:15 DEBUG opendrift:1745: No elements to deactivate
09:36:15 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:15 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:15 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:15 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:15 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:15 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:15 DEBUG opendrift.models.physics_methods:917: min: 6.632127, mean: 7.004854, max: 7.330880
09:36:15 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:15 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001616, dN_50: 0.000127
09:36:15 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:15 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.026562636829722472
09:36:15 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:15 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:15 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2510 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2508 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2507 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2505 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2504 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2498 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2495 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2491 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2489 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2484 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2479 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2473 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2470 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2468 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2467 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 2464 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2457 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2456 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2453 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2447 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2441 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2435 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2434 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2429 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2426 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2420 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2416 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2413 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2412 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2411 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2409 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2406 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2405 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2403 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2400 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2399 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2394 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2392 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2389 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2386 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2383 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2379 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2379 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2376 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2374 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2370 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2366 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2364 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2360 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2359 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2357 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2351 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2351 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2348 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2345 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2345 surface elements
09:36:15 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2342 surface elements
09:36:15 DEBUG opendrift.models.physics_methods:771: Advecting 2344 of 6000 elements above 0.100m with wind-sheared ocean current (0.066888 m/s - 0.253155 m/s)
09:36:15 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:15 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0004169399674894707 and 0.488578492022291 m/s
09:36:15 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:15 DEBUG opendrift:2101: ======================================================================
09:36:15 INFO opendrift:2102: 2025-05-24 01:35:52.207946 - step 17 of 120 - 6000 active elements (0 deactivated)
09:36:15 DEBUG opendrift:2108: 0 elements scheduled.
09:36:15 DEBUG opendrift:2110: ======================================================================
09:36:15 DEBUG opendrift:2121: 35.00441613943958 <- latitude -> 35.241840311946035
09:36:15 DEBUG opendrift:2121: 23.304846880040127 <- longitude -> 23.47178231049993
09:36:15 DEBUG opendrift:2121: -138.2377680345438 <- z -> 0.0
09:36:15 DEBUG opendrift:2122: ---------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:15 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:15 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.47) and
after (2025-05-24 03:00:00, weight 0.53) in time
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.47) and
after (2025-05-24 03:00:00, weight 0.53) in time
09:36:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:15 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.210001 (min) 0.0469293 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.099401 (min) 0.416948 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.64579 (min) -6.72682 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.53436 (min) 5.93413 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:15 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.781230, mean: 1.987804, max: 2.173502
09:36:15 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:15 DEBUG opendrift.models.physics_methods:917: min: 7.270923, mean: 7.680196, max: 8.031739
09:36:15 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 7.270923, mean: 7.680196, max: 8.031739
09:36:15 DEBUG opendrift:648: No elements hit coastline.
09:36:16 DEBUG opendrift:1745: No elements to deactivate
09:36:16 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:16 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:16 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:16 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:16 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:16 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:16 DEBUG opendrift.models.physics_methods:917: min: 7.270924, mean: 7.680196, max: 8.031739
09:36:16 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:16 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001707, dN_50: 0.000134
09:36:16 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:16 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03188355284494389
09:36:16 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:16 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:16 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2339 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2338 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2335 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2332 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2327 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2325 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2322 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2319 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2317 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2315 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2316 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 2314 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2308 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2306 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2304 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2299 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 2297 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2290 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2285 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2282 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2282 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2281 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2279 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2277 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2272 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2267 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2263 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2262 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2261 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2257 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2254 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2251 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2245 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2242 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2239 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2237 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2234 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2233 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2230 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2224 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2222 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2219 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2213 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2209 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2206 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2204 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2201 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2196 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2194 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2192 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2191 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2186 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2183 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2181 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2178 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2175 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2172 surface elements
09:36:16 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2169 surface elements
09:36:16 DEBUG opendrift.models.physics_methods:771: Advecting 2167 of 6000 elements above 0.100m with wind-sheared ocean current (0.007391 m/s - 0.276506 m/s)
09:36:16 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:16 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001536612797264656 and 0.45746952247171574 m/s
09:36:16 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:16 DEBUG opendrift:2101: ======================================================================
09:36:16 INFO opendrift:2102: 2025-05-24 02:35:52.207946 - step 18 of 120 - 6000 active elements (0 deactivated)
09:36:16 DEBUG opendrift:2108: 0 elements scheduled.
09:36:16 DEBUG opendrift:2110: ======================================================================
09:36:16 DEBUG opendrift:2121: 35.00809063453122 <- latitude -> 35.2665379793143
09:36:16 DEBUG opendrift:2121: 23.28891205550925 <- longitude -> 23.461721842827142
09:36:16 DEBUG opendrift:2121: -137.6022484891696 <- z -> 0.0
09:36:16 DEBUG opendrift:2122: ---------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:16 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:16 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 38 elements, expanding data 1
09:36:16 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:16 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.13) and
after (2025-05-24 03:00:00, weight 0.87) in time
09:36:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:16 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 00:00:00 (before)
2025-05-24 03:00:00 (after)
09:36:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 00:00:00) in space (linearNDFast)
09:36:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:16 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 03:00:00) in space (linearNDFast)
09:36:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:16 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 00:00:00, weight 0.13) and
after (2025-05-24 03:00:00, weight 0.87) in time
09:36:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:16 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:16 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:16 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:16 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:16 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.186487 (min) 0.035418 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.071447 (min) 0.381462 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.71908 (min) -7.55459 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.23267 (min) 6.30782 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:16 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:16 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.136059, mean: 2.381683, max: 2.601491
09:36:16 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:16 DEBUG opendrift.models.physics_methods:917: min: 7.962258, mean: 8.406733, max: 8.787003
09:36:16 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 7.962258, mean: 8.406733, max: 8.787003
09:36:16 DEBUG opendrift:648: No elements hit coastline.
09:36:16 DEBUG opendrift:1745: No elements to deactivate
09:36:16 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:16 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:16 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:16 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:16 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:16 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:16 DEBUG opendrift.models.physics_methods:917: min: 7.962258, mean: 8.406734, max: 8.787003
09:36:16 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:16 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.001848, dN_50: 0.000145
09:36:17 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:17 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03816099459507457
09:36:17 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:17 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:17 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2164 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2162 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2158 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2153 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2149 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2148 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2146 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2144 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2142 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2136 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2134 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2130 surface elements
09:36:17 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2128 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2126 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2124 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2123 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2119 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2115 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2114 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2111 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2108 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2103 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2101 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 2097 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2090 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2088 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2088 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2084 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2079 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2073 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2067 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2064 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 8 of 2062 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2054 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2049 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2048 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2043 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2040 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2038 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2036 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2034 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2029 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 2027 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2021 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2019 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2015 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 2011 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 2010 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 2008 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 2004 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 2000 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1997 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1994 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1992 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1989 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1984 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1982 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1975 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1974 surface elements
09:36:18 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1969 surface elements
09:36:18 DEBUG opendrift.models.physics_methods:771: Advecting 1973 of 6000 elements above 0.100m with wind-sheared ocean current (0.054278 m/s - 0.303159 m/s)
09:36:18 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:18 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013611490288181931 and 0.4236016440884586 m/s
09:36:18 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:18 DEBUG opendrift:2101: ======================================================================
09:36:18 INFO opendrift:2102: 2025-05-24 03:35:52.207946 - step 19 of 120 - 6000 active elements (0 deactivated)
09:36:18 DEBUG opendrift:2108: 0 elements scheduled.
09:36:18 DEBUG opendrift:2110: ======================================================================
09:36:18 DEBUG opendrift:2121: 35.012918986729794 <- latitude -> 35.2846043622897
09:36:18 DEBUG opendrift:2121: 23.27384745486226 <- longitude -> 23.453941291034035
09:36:18 DEBUG opendrift:2121: -137.0090954649914 <- z -> 0.0
09:36:18 DEBUG opendrift:2122: ---------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:18 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:19 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:19 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:19 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:19 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 11x15x23) for time after (2025-05-24 06:00:00)
09:36:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.80) and
after (2025-05-24 06:00:00, weight 0.20) in time
09:36:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:19 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:19 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:19 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:19 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-24 06:00:00)
09:36:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.80) and
after (2025-05-24 06:00:00, weight 0.20) in time
09:36:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:19 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.184971 (min) 0.00154964 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0652847 (min) 0.390224 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: -10.2356 (min) -8.0214 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.4174 (min) 7.12275 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:19 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.463856, mean: 2.728174, max: 2.959588
09:36:19 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:19 DEBUG opendrift.models.physics_methods:917: min: 8.551399, mean: 8.997758, max: 9.372279
09:36:19 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 8.551399, mean: 8.997758, max: 9.372279
09:36:19 DEBUG opendrift:648: No elements hit coastline.
09:36:20 DEBUG opendrift:1745: No elements to deactivate
09:36:20 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:20 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:20 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:20 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:20 DEBUG opendrift.models.physics_methods:917: min: 8.551399, mean: 8.997759, max: 9.372278
09:36:20 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:20 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002073, dN_50: 0.000163
09:36:20 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:20 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.043413296858040934
09:36:20 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:20 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:20 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1968 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1961 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1958 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1953 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1949 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1943 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1938 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1932 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1928 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1926 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1924 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1922 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1919 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1916 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1911 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1908 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1902 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1900 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1897 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1894 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1894 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1891 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1888 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1884 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1883 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1880 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1880 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1877 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1871 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1870 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1866 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 9 of 1860 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1852 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1847 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1843 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1840 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1834 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1832 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1827 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1826 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1823 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1820 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1819 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1814 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1809 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1805 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1800 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1796 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1794 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1792 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1791 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1785 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1781 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1777 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1774 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1772 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1766 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1759 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1756 surface elements
09:36:20 DEBUG opendrift.models.physics_methods:771: Advecting 1752 of 6000 elements above 0.100m with wind-sheared ocean current (0.145246 m/s - 0.329056 m/s)
09:36:20 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:20 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00132774449349926 and 0.43011199849577236 m/s
09:36:20 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:20 DEBUG opendrift:2101: ======================================================================
09:36:20 INFO opendrift:2102: 2025-05-24 04:35:52.207946 - step 20 of 120 - 6000 active elements (0 deactivated)
09:36:20 DEBUG opendrift:2108: 0 elements scheduled.
09:36:20 DEBUG opendrift:2110: ======================================================================
09:36:20 DEBUG opendrift:2121: 35.01374855409679 <- latitude -> 35.308202087386725
09:36:20 DEBUG opendrift:2121: 23.25733735641533 <- longitude -> 23.447217047136235
09:36:20 DEBUG opendrift:2121: -135.75096712380622 <- z -> 0.0
09:36:20 DEBUG opendrift:2122: ---------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.47) and
after (2025-05-24 06:00:00, weight 0.53) in time
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.47) and
after (2025-05-24 06:00:00, weight 0.53) in time
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:20 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.21417 (min) -0.0437833 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0787783 (min) 0.432535 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -10.4466 (min) -8.12982 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.89336 (min) 8.45393 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:20 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.795535, mean: 3.057160, max: 3.537499
09:36:20 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:20 DEBUG opendrift.models.physics_methods:917: min: 9.108818, mean: 9.522832, max: 10.246551
09:36:20 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 9.108818, mean: 9.522832, max: 10.246551
09:36:20 DEBUG opendrift:648: No elements hit coastline.
09:36:20 DEBUG opendrift:1745: No elements to deactivate
09:36:20 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:20 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:20 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:20 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:20 DEBUG opendrift.models.physics_methods:917: min: 9.108818, mean: 9.522832, max: 10.246552
09:36:20 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:20 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002372, dN_50: 0.000186
09:36:20 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:20 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.051889671604547966
09:36:20 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:20 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:20 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1749 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1742 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1740 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1733 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1731 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1729 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1725 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1721 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1716 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1714 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1709 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1707 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1703 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1701 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1695 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1692 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1690 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1687 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1686 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1684 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1683 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1681 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1676 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1673 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1669 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1665 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1663 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 8 of 1661 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1653 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1651 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1648 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1645 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1641 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1634 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1631 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1626 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1624 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1620 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1613 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1611 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 8 of 1610 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1602 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1595 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1590 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1589 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1585 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1582 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1577 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1575 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1573 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1570 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1567 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1566 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1562 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1557 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1555 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1553 surface elements
09:36:20 DEBUG opendrift.models.physics_methods:771: Advecting 1559 of 6000 elements above 0.100m with wind-sheared ocean current (0.029442 m/s - 0.359751 m/s)
09:36:20 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:20 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001962788596762018 and 0.4584055201733749 m/s
09:36:20 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:20 DEBUG opendrift:2101: ======================================================================
09:36:20 INFO opendrift:2102: 2025-05-24 05:35:52.207946 - step 21 of 120 - 6000 active elements (0 deactivated)
09:36:20 DEBUG opendrift:2108: 0 elements scheduled.
09:36:20 DEBUG opendrift:2110: ======================================================================
09:36:20 DEBUG opendrift:2121: 35.01675431101462 <- latitude -> 35.334867561821106
09:36:20 DEBUG opendrift:2121: 23.237213087383136 <- longitude -> 23.442160543452925
09:36:20 DEBUG opendrift:2121: -134.57584122080803 <- z -> 0.0
09:36:20 DEBUG opendrift:2122: ---------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:20 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.13) and
after (2025-05-24 06:00:00, weight 0.87) in time
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 03:00:00 (before)
2025-05-24 06:00:00 (after)
09:36:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 03:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 06:00:00) in space (linearNDFast)
09:36:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 03:00:00, weight 0.13) and
after (2025-05-24 06:00:00, weight 0.87) in time
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:20 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.254068 (min) -0.0485809 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0678589 (min) 0.478666 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -10.6439 (min) -8.24999 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: 4.31319 (min) 9.91576 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:20 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 3.081564, mean: 3.443398, max: 4.168484
09:36:20 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:20 DEBUG opendrift.models.physics_methods:917: min: 9.563463, mean: 10.101018, max: 11.122915
09:36:20 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 9.563463, mean: 10.101018, max: 11.122915
09:36:20 DEBUG opendrift:648: No elements hit coastline.
09:36:20 DEBUG opendrift:1745: No elements to deactivate
09:36:20 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:20 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:20 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:20 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:20 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:20 DEBUG opendrift.models.physics_methods:917: min: 9.563462, mean: 10.101018, max: 11.122915
09:36:20 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:20 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.002741, dN_50: 0.000215
09:36:20 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:20 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.06114450209690782
09:36:20 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:20 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:20 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1551 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1548 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1546 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1541 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1535 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1530 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1526 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1525 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1521 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1519 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1515 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1509 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1506 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1504 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1501 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1498 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1495 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1493 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1490 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1485 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1482 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1480 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1478 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1477 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1474 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1473 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1469 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1466 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1460 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1458 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1452 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1446 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1442 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1440 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1435 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1431 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1425 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1422 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1420 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1416 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1414 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1412 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1410 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1407 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1403 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1401 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1399 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 7 of 1396 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1389 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1386 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1380 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1379 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1375 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1374 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1372 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1372 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1368 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1367 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1364 surface elements
09:36:20 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1361 surface elements
09:36:20 DEBUG opendrift.models.physics_methods:771: Advecting 1369 of 6000 elements above 0.100m with wind-sheared ocean current (0.016383 m/s - 0.390520 m/s)
09:36:20 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:20 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0025886005479159218 and 0.4620561572740499 m/s
09:36:20 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:20 DEBUG opendrift:2101: ======================================================================
09:36:20 INFO opendrift:2102: 2025-05-24 06:35:52.207946 - step 22 of 120 - 6000 active elements (0 deactivated)
09:36:20 DEBUG opendrift:2108: 0 elements scheduled.
09:36:20 DEBUG opendrift:2110: ======================================================================
09:36:20 DEBUG opendrift:2121: 35.02564673389862 <- latitude -> 35.36300247494406
09:36:20 DEBUG opendrift:2121: 23.22438439253468 <- longitude -> 23.44239947450382
09:36:20 DEBUG opendrift:2121: -133.77166802727518 <- z -> 0.0
09:36:20 DEBUG opendrift:2122: ---------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:20 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:22 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:22 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:22 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:22 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 12x17x23) for time after (2025-05-24 09:00:00)
09:36:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.80) and
after (2025-05-24 09:00:00, weight 0.20) in time
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:22 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:22 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:22 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-24 09:00:00)
09:36:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.80) and
after (2025-05-24 09:00:00, weight 0.20) in time
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:22 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.333819 (min) -0.0364013 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0913737 (min) 0.525608 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -10.3647 (min) -7.65823 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: 4.46401 (min) 10.6709 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:22 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 3.011646, mean: 3.392781, max: 4.274530
09:36:22 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:22 DEBUG opendrift.models.physics_methods:917: min: 9.454347, mean: 10.023073, max: 11.263510
09:36:22 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 9.454347, mean: 10.023073, max: 11.263510
09:36:22 DEBUG opendrift:648: No elements hit coastline.
09:36:22 DEBUG opendrift:1745: No elements to deactivate
09:36:22 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:22 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:22 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:22 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:22 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:22 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:22 DEBUG opendrift.models.physics_methods:917: min: 9.454348, mean: 10.023073, max: 11.263510
09:36:22 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:22 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.003359, dN_50: 0.000264
09:36:22 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:22 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.06269991167671249
09:36:22 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:22 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:22 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1360 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1356 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1353 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1350 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1346 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1344 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1340 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1338 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1336 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1334 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1333 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1329 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1328 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1325 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1323 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1321 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1315 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1313 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1307 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1305 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1304 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1302 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1301 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1300 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1298 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1296 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1294 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1291 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1287 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1285 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1284 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1282 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1280 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1278 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1273 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1270 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1265 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1264 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1261 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1258 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1257 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1256 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1253 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1251 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1249 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 6 of 1245 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1239 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1235 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1231 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1229 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1225 surface elements
09:36:22 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1222 surface elements
09:36:22 DEBUG opendrift.models.physics_methods:771: Advecting 1228 of 6000 elements above 0.100m with wind-sheared ocean current (0.018982 m/s - 0.395456 m/s)
09:36:22 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:22 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005510257353160126 and 0.45918607891121627 m/s
09:36:22 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:22 DEBUG opendrift:2101: ======================================================================
09:36:22 INFO opendrift:2102: 2025-05-24 07:35:52.207946 - step 23 of 120 - 6000 active elements (0 deactivated)
09:36:22 DEBUG opendrift:2108: 0 elements scheduled.
09:36:22 DEBUG opendrift:2110: ======================================================================
09:36:22 DEBUG opendrift:2121: 35.03252525960711 <- latitude -> 35.39419578851733
09:36:22 DEBUG opendrift:2121: 23.203578034603087 <- longitude -> 23.43668217104183
09:36:22 DEBUG opendrift:2121: -132.82618029687725 <- z -> 0.0
09:36:22 DEBUG opendrift:2122: ---------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:22 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.47) and
after (2025-05-24 09:00:00, weight 0.53) in time
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.47) and
after (2025-05-24 09:00:00, weight 0.53) in time
09:36:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:22 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.333172 (min) -0.05432 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.10337 (min) 0.571851 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.8009 (min) -6.54969 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: 4.23868 (min) 10.8704 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:22 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.719710, mean: 3.029855, max: 3.962188
09:36:22 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:22 DEBUG opendrift.models.physics_methods:917: min: 8.984436, mean: 9.470743, max: 10.844190
09:36:22 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 8.984436, mean: 9.470743, max: 10.844190
09:36:22 DEBUG opendrift:648: No elements hit coastline.
09:36:23 DEBUG opendrift:1745: No elements to deactivate
09:36:23 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:23 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:23 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:23 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:23 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:23 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:23 DEBUG opendrift.models.physics_methods:917: min: 8.984436, mean: 9.470742, max: 10.844191
09:36:23 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:23 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.004210, dN_50: 0.000330
09:36:23 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:23 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.05811871724044258
09:36:23 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:23 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:23 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1221 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1219 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1217 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1215 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1212 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1208 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1206 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 4 of 1205 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1201 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1200 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1197 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1196 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1194 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1193 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1192 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1191 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1189 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1187 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 5 of 1185 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1180 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1179 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1176 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1175 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1173 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1173 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1170 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1168 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1166 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1164 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1162 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1159 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1158 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1155 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1152 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1151 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1150 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1150 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1149 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1147 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1147 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1147 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1145 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1144 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1143 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1143 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1142 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1141 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1140 surface elements
09:36:23 DEBUG opendrift.models.physics_methods:771: Advecting 1146 of 6000 elements above 0.100m with wind-sheared ocean current (0.040576 m/s - 0.380734 m/s)
09:36:23 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:23 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0019022567265625375 and 0.44246181450760974 m/s
09:36:23 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:23 DEBUG opendrift:2101: ======================================================================
09:36:23 INFO opendrift:2102: 2025-05-24 08:35:52.207946 - step 24 of 120 - 6000 active elements (0 deactivated)
09:36:23 DEBUG opendrift:2108: 0 elements scheduled.
09:36:23 DEBUG opendrift:2110: ======================================================================
09:36:23 DEBUG opendrift:2121: 35.03307357338659 <- latitude -> 35.421948941351694
09:36:23 DEBUG opendrift:2121: 23.189801764887566 <- longitude -> 23.431798323419653
09:36:23 DEBUG opendrift:2121: -132.20227255947594 <- z -> 0.0
09:36:23 DEBUG opendrift:2122: ---------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:23 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:23 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
09:36:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.13) and
after (2025-05-24 09:00:00, weight 0.87) in time
09:36:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 06:00:00 (before)
2025-05-24 09:00:00 (after)
09:36:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 06:00:00) in space (linearNDFast)
09:36:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 09:00:00) in space (linearNDFast)
09:36:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 06:00:00, weight 0.13) and
after (2025-05-24 09:00:00, weight 0.87) in time
09:36:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:23 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.375305 (min) -0.0444801 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0914931 (min) 0.625542 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.28995 (min) -5.40002 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.92869 (min) 10.9755 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:23 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.424469, mean: 2.684964, max: 3.680676
09:36:23 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:23 DEBUG opendrift.models.physics_methods:917: min: 8.482775, mean: 8.913265, max: 10.451855
09:36:23 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 8.482775, mean: 8.913265, max: 10.451855
09:36:23 DEBUG opendrift:648: No elements hit coastline.
09:36:23 DEBUG opendrift:1745: No elements to deactivate
09:36:23 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:23 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:23 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:23 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:23 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:23 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:23 DEBUG opendrift.models.physics_methods:917: min: 8.482775, mean: 8.913264, max: 10.451855
09:36:23 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:23 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.005170, dN_50: 0.000406
09:36:23 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:23 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0539896970035776
09:36:23 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:23 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:23 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1139 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1138 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1137 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1136 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1135 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1134 surface elements
09:36:23 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1132 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1130 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1128 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1127 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1125 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1123 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1124 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1122 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1120 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1119 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1119 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1118 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1116 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1116 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1116 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1115 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1116 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1115 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1114 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1113 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1111 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1110 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1108 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1107 surface elements
09:36:24 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1107 surface elements
09:36:24 DEBUG opendrift.models.physics_methods:771: Advecting 1114 of 6000 elements above 0.100m with wind-sheared ocean current (0.000963 m/s - 0.366959 m/s)
09:36:24 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:24 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016767608448967815 and 0.4674749268040271 m/s
09:36:24 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:24 DEBUG opendrift:2101: ======================================================================
09:36:24 INFO opendrift:2102: 2025-05-24 09:35:52.207946 - step 25 of 120 - 6000 active elements (0 deactivated)
09:36:24 DEBUG opendrift:2108: 0 elements scheduled.
09:36:24 DEBUG opendrift:2110: ======================================================================
09:36:24 DEBUG opendrift:2121: 35.03484546355348 <- latitude -> 35.4479321078702
09:36:24 DEBUG opendrift:2121: 23.174189603511984 <- longitude -> 23.425760543386147
09:36:24 DEBUG opendrift:2121: -131.30105269191117 <- z -> 0.0
09:36:24 DEBUG opendrift:2122: ---------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:24 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:24 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:24 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:24 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:24 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:24 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:24 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:24 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:24 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:24 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 12x19x23) for time after (2025-05-24 12:00:00)
09:36:24 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:24 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:24 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:24 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:24 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.80) and
after (2025-05-24 12:00:00, weight 0.20) in time
09:36:24 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:24 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:24 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:24 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:24 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:24 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:24 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:24 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:25 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:25 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:25 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x6x1) for time after (2025-05-24 12:00:00)
09:36:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.80) and
after (2025-05-24 12:00:00, weight 0.20) in time
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.358241 (min) 0.00319797 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.07879 (min) 0.691543 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.0223 (min) -4.55669 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.53246 (min) 10.9522 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 2.173337, mean: 2.434164, max: 3.461558
09:36:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 8.031435, mean: 8.484204, max: 10.135972
09:36:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 8.031435, mean: 8.484204, max: 10.135972
09:36:25 DEBUG opendrift:648: No elements hit coastline.
09:36:25 DEBUG opendrift:1745: No elements to deactivate
09:36:25 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:25 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 8.031435, mean: 8.484204, max: 10.135971
09:36:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.006187, dN_50: 0.000486
09:36:25 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:25 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0507758241236273
09:36:25 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:25 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:25 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1105 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1102 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1101 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1099 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1098 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1097 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1096 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1095 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1094 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1093 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1091 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1091 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1090 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1090 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1089 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1088 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1087 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1086 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1085 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1084 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1084 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1084 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1083 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1082 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1080 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1078 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1076 surface elements
09:36:25 DEBUG opendrift.models.physics_methods:771: Advecting 1089 of 6000 elements above 0.100m with wind-sheared ocean current (0.021206 m/s - 0.355868 m/s)
09:36:25 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:25 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0037736568903420273 and 0.46058579467491984 m/s
09:36:25 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:25 DEBUG opendrift:2101: ======================================================================
09:36:25 INFO opendrift:2102: 2025-05-24 10:35:52.207946 - step 26 of 120 - 6000 active elements (0 deactivated)
09:36:25 DEBUG opendrift:2108: 0 elements scheduled.
09:36:25 DEBUG opendrift:2110: ======================================================================
09:36:25 DEBUG opendrift:2121: 35.03686829598948 <- latitude -> 35.48371327834591
09:36:25 DEBUG opendrift:2121: 23.16660946638183 <- longitude -> 23.42392617043079
09:36:25 DEBUG opendrift:2121: -130.60212540731908 <- z -> 0.0
09:36:25 DEBUG opendrift:2122: ---------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:25 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:25 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.47) and
after (2025-05-24 12:00:00, weight 0.53) in time
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.47) and
after (2025-05-24 12:00:00, weight 0.53) in time
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.292995 (min) 0.0613526 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0600489 (min) 0.736027 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.84696 (min) -3.78171 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.19154 (min) 11.0054 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.960218, mean: 2.239804, max: 3.331336
09:36:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 7.627491, mean: 8.136319, max: 9.943489
09:36:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 7.627491, mean: 8.136319, max: 9.943489
09:36:25 DEBUG opendrift:648: No elements hit coastline.
09:36:25 DEBUG opendrift:1745: No elements to deactivate
09:36:25 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:25 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 7.627491, mean: 8.136319, max: 9.943489
09:36:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007193, dN_50: 0.000564
09:36:25 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:25 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.04886582231060299
09:36:25 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:25 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:25 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1075 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1074 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1073 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1072 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1071 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1068 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1067 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1066 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1065 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1064 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1061 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1060 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1054 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1052 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1051 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1050 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
09:36:25 DEBUG opendrift.models.physics_methods:771: Advecting 1051 of 6000 elements above 0.100m with wind-sheared ocean current (0.062094 m/s - 0.349110 m/s)
09:36:25 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:25 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0002914579376952525 and 0.431620491407613 m/s
09:36:25 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:25 DEBUG opendrift:2101: ======================================================================
09:36:25 INFO opendrift:2102: 2025-05-24 11:35:52.207946 - step 27 of 120 - 6000 active elements (0 deactivated)
09:36:25 DEBUG opendrift:2108: 0 elements scheduled.
09:36:25 DEBUG opendrift:2110: ======================================================================
09:36:25 DEBUG opendrift:2121: 35.03976327686126 <- latitude -> 35.51331521146365
09:36:25 DEBUG opendrift:2121: 23.159075620604504 <- longitude -> 23.423747644517654
09:36:25 DEBUG opendrift:2121: -129.39205372262592 <- z -> 0.0
09:36:25 DEBUG opendrift:2122: ---------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:25 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:25 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 8 elements, expanding data 1
09:36:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.13) and
after (2025-05-24 12:00:00, weight 0.87) in time
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 09:00:00 (before)
2025-05-24 12:00:00 (after)
09:36:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 09:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 12:00:00) in space (linearNDFast)
09:36:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 09:00:00, weight 0.13) and
after (2025-05-24 12:00:00, weight 0.87) in time
09:36:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.215543 (min) 0.115956 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0353729 (min) 0.779696 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.71142 (min) -2.99822 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.80083 (min) 10.6977 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.748713, mean: 2.050323, max: 3.044742
09:36:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 7.204250, mean: 7.782162, max: 9.506154
09:36:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 7.204250, mean: 7.782162, max: 9.506154
09:36:25 DEBUG opendrift:648: No elements hit coastline.
09:36:25 DEBUG opendrift:1745: No elements to deactivate
09:36:25 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:25 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:25 DEBUG opendrift.models.physics_methods:917: min: 7.204250, mean: 7.782161, max: 9.506154
09:36:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.007899, dN_50: 0.000620
09:36:25 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:25 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.04466228115390063
09:36:25 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:25 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:25 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1047 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1045 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1044 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1043 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1043 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1041 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1039 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1039 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1037 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1036 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1035 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1034 surface elements
09:36:25 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1033 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1032 surface elements
09:36:26 DEBUG opendrift.models.physics_methods:771: Advecting 1041 of 6000 elements above 0.100m with wind-sheared ocean current (0.004830 m/s - 0.333756 m/s)
09:36:26 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:26 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0022859984016221605 and 0.49811742001960796 m/s
09:36:26 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:26 DEBUG opendrift:2101: ======================================================================
09:36:26 INFO opendrift:2102: 2025-05-24 12:35:52.207946 - step 28 of 120 - 6000 active elements (0 deactivated)
09:36:26 DEBUG opendrift:2108: 0 elements scheduled.
09:36:26 DEBUG opendrift:2110: ======================================================================
09:36:26 DEBUG opendrift:2121: 35.03781998915691 <- latitude -> 35.543986862551165
09:36:26 DEBUG opendrift:2121: 23.15100259315346 <- longitude -> 23.427244085578216
09:36:26 DEBUG opendrift:2121: -128.39917635883475 <- z -> 0.0
09:36:26 DEBUG opendrift:2122: ---------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:26 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:26 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x21x23) for time after (2025-05-24 15:00:00)
09:36:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.80) and
after (2025-05-24 15:00:00, weight 0.20) in time
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:26 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-24 15:00:00)
09:36:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.80) and
after (2025-05-24 15:00:00, weight 0.20) in time
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:26 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:26 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.129124 (min) 0.160104 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0214521 (min) 0.761032 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.3611 (min) -2.21706 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.61822 (min) 10.0644 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:26 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.545364, mean: 1.843708, max: 2.681577
09:36:26 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:26 DEBUG opendrift.models.physics_methods:917: min: 6.772437, mean: 7.376281, max: 8.921230
09:36:26 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.772437, mean: 7.376281, max: 8.921230
09:36:26 DEBUG opendrift:648: No elements hit coastline.
09:36:26 DEBUG opendrift:1745: No elements to deactivate
09:36:26 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:26 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:26 DEBUG opendrift.models.physics_methods:917: min: 6.772437, mean: 7.376281, max: 8.921229
09:36:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.008545, dN_50: 0.000671
09:36:26 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:26 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03933563425183645
09:36:26 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:26 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:26 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1031 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1031 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1030 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1028 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1027 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1026 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1025 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1024 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1021 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1019 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1018 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1017 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 3 of 1016 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1013 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1013 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1012 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1011 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1010 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
09:36:26 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:26 DEBUG opendrift.models.physics_methods:771: Advecting 1019 of 6000 elements above 0.100m with wind-sheared ocean current (0.079620 m/s - 0.313220 m/s)
09:36:26 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:26 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002302269231106012 and 0.4283160208094611 m/s
09:36:26 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:26 DEBUG opendrift:2101: ======================================================================
09:36:26 INFO opendrift:2102: 2025-05-24 13:35:52.207946 - step 29 of 120 - 6000 active elements (0 deactivated)
09:36:26 DEBUG opendrift:2108: 0 elements scheduled.
09:36:26 DEBUG opendrift:2110: ======================================================================
09:36:26 DEBUG opendrift:2121: 35.03533494943184 <- latitude -> 35.576966678947876
09:36:26 DEBUG opendrift:2121: 23.151656018082903 <- longitude -> 23.42314834806402
09:36:26 DEBUG opendrift:2121: -127.69505938884895 <- z -> 0.0
09:36:26 DEBUG opendrift:2122: ---------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:26 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:26 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
09:36:26 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.47) and
after (2025-05-24 15:00:00, weight 0.53) in time
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:26 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.47) and
after (2025-05-24 15:00:00, weight 0.53) in time
09:36:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:26 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:26 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:26 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:26 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:26 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0888291 (min) 0.208973 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0142212 (min) 0.686623 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.83337 (min) -1.50558 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.62015 (min) 9.30879 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:26 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:26 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.334408, mean: 1.622783, max: 2.296009
09:36:26 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:26 DEBUG opendrift.models.physics_methods:917: min: 6.293234, mean: 6.918273, max: 8.254987
09:36:26 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.293234, mean: 6.918273, max: 8.254987
09:36:26 DEBUG opendrift:648: No elements hit coastline.
09:36:27 DEBUG opendrift:1745: No elements to deactivate
09:36:27 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:27 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:27 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:27 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:27 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:27 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:27 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:27 DEBUG opendrift.models.physics_methods:917: min: 6.293234, mean: 6.918273, max: 8.254987
09:36:27 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:27 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.009302, dN_50: 0.000730
09:36:27 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:27 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.033680403576865844
09:36:27 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:27 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:27 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:27 DEBUG opendrift.models.physics_methods:771: Advecting 1013 of 6000 elements above 0.100m with wind-sheared ocean current (0.047534 m/s - 0.289828 m/s)
09:36:27 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:27 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008084395709090957 and 0.4980713141225855 m/s
09:36:27 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:27 DEBUG opendrift:2101: ======================================================================
09:36:27 INFO opendrift:2102: 2025-05-24 14:35:52.207946 - step 30 of 120 - 6000 active elements (0 deactivated)
09:36:27 DEBUG opendrift:2108: 0 elements scheduled.
09:36:27 DEBUG opendrift:2110: ======================================================================
09:36:27 DEBUG opendrift:2121: 35.039457422005995 <- latitude -> 35.604014482115936
09:36:27 DEBUG opendrift:2121: 23.156970094313454 <- longitude -> 23.431278746629808
09:36:27 DEBUG opendrift:2121: -126.75057914021954 <- z -> 0.0
09:36:27 DEBUG opendrift:2122: ---------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:27 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 14 elements, expanding data 1
09:36:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.13) and
after (2025-05-24 15:00:00, weight 0.87) in time
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 12:00:00 (before)
2025-05-24 15:00:00 (after)
09:36:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 12:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 15:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 12:00:00, weight 0.13) and
after (2025-05-24 15:00:00, weight 0.87) in time
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:27 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.103498 (min) 0.287193 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0153934 (min) 0.659875 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.21146 (min) -0.785049 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.71903 (min) 8.55208 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:27 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.138011, mean: 1.398206, max: 1.945530
09:36:27 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:27 DEBUG opendrift.models.physics_methods:917: min: 5.811694, mean: 6.423381, max: 7.598862
09:36:27 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.811694, mean: 6.423381, max: 7.598862
09:36:27 DEBUG opendrift:648: No elements hit coastline.
09:36:27 DEBUG opendrift:1745: No elements to deactivate
09:36:27 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:27 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:27 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:27 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:27 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:27 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:27 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:27 DEBUG opendrift.models.physics_methods:917: min: 5.811694, mean: 6.423380, max: 7.598862
09:36:27 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:27 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.010220, dN_50: 0.000802
09:36:27 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:27 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.028539824521011475
09:36:27 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:27 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:27 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1006 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1010 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1009 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1009 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
09:36:27 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
09:36:27 DEBUG opendrift.models.physics_methods:771: Advecting 1010 of 6000 elements above 0.100m with wind-sheared ocean current (0.077532 m/s - 0.266792 m/s)
09:36:27 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:27 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0011796229060205793 and 0.4679299717518188 m/s
09:36:27 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:27 DEBUG opendrift:2101: ======================================================================
09:36:27 INFO opendrift:2102: 2025-05-24 15:35:52.207946 - step 31 of 120 - 6000 active elements (0 deactivated)
09:36:27 DEBUG opendrift:2108: 0 elements scheduled.
09:36:27 DEBUG opendrift:2110: ======================================================================
09:36:27 DEBUG opendrift:2121: 35.042935374787305 <- latitude -> 35.62633474974284
09:36:27 DEBUG opendrift:2121: 23.159713848290913 <- longitude -> 23.43066637910305
09:36:27 DEBUG opendrift:2121: -125.84432978088033 <- z -> 0.0
09:36:27 DEBUG opendrift:2122: ---------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:27 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:27 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x22x23) for time after (2025-05-24 18:00:00)
09:36:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.80) and
after (2025-05-24 18:00:00, weight 0.20) in time
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:27 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:27 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-24 18:00:00)
09:36:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.80) and
after (2025-05-24 18:00:00, weight 0.20) in time
09:36:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:27 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0995684 (min) 0.312839 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0223995 (min) 0.613924 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.91839 (min) -0.431633 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.69121 (min) 7.61752 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:27 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.022338, mean: 1.213921, max: 1.583962
09:36:27 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:27 DEBUG opendrift.models.physics_methods:917: min: 5.508418, mean: 5.995106, max: 6.856492
09:36:27 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.508418, mean: 5.995106, max: 6.856492
09:36:27 DEBUG opendrift:648: No elements hit coastline.
09:36:28 DEBUG opendrift:1745: No elements to deactivate
09:36:28 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:28 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:28 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:28 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:28 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:28 DEBUG opendrift.models.physics_methods:917: min: 5.508418, mean: 5.995105, max: 6.856492
09:36:28 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:28 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.011305, dN_50: 0.000887
09:36:28 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:28 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.023236607092040878
09:36:28 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:28 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:28 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1007 surface elements
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1008 surface elements
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1010 surface elements
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1013 surface elements
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1011 surface elements
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1010 surface elements
09:36:28 DEBUG opendrift.models.physics_methods:771: Advecting 1016 of 6000 elements above 0.100m with wind-sheared ocean current (0.000874 m/s - 0.240728 m/s)
09:36:28 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:28 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0028003437947522456 and 0.44123663925808704 m/s
09:36:28 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:28 DEBUG opendrift:2101: ======================================================================
09:36:28 INFO opendrift:2102: 2025-05-24 16:35:52.207946 - step 32 of 120 - 6000 active elements (0 deactivated)
09:36:28 DEBUG opendrift:2108: 0 elements scheduled.
09:36:28 DEBUG opendrift:2110: ======================================================================
09:36:28 DEBUG opendrift:2121: 35.04202355138668 <- latitude -> 35.651105452329666
09:36:28 DEBUG opendrift:2121: 23.167034589360195 <- longitude -> 23.42977453895889
09:36:28 DEBUG opendrift:2121: -124.63088588740492 <- z -> 0.0
09:36:28 DEBUG opendrift:2122: ---------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:28 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.47) and
after (2025-05-24 18:00:00, weight 0.53) in time
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.47) and
after (2025-05-24 18:00:00, weight 0.53) in time
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:28 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:28 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0837389 (min) 0.31596 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0352595 (min) 0.532214 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.88293 (min) -0.269201 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.49305 (min) 6.53848 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:28 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.707253, mean: 1.067835, max: 1.324843
09:36:28 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:28 DEBUG opendrift.models.physics_methods:917: min: 4.581598, mean: 5.626139, max: 6.270639
09:36:28 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.581598, mean: 5.626139, max: 6.270639
09:36:28 DEBUG opendrift:648: No elements hit coastline.
09:36:28 DEBUG opendrift:1745: No elements to deactivate
09:36:28 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:28 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:28 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:28 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:28 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:28 DEBUG opendrift.models.physics_methods:917: min: 4.581598, mean: 5.626139, max: 6.270639
09:36:28 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:28 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.012585, dN_50: 0.000988
09:36:28 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:28 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.019436036654720254
09:36:28 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:28 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:28 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:28 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1011 surface elements
09:36:28 DEBUG opendrift.models.physics_methods:771: Advecting 1013 of 6000 elements above 0.100m with wind-sheared ocean current (0.019203 m/s - 0.213023 m/s)
09:36:28 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:28 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001813417952798883 and 0.5117220625509399 m/s
09:36:28 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:28 DEBUG opendrift:2101: ======================================================================
09:36:28 INFO opendrift:2102: 2025-05-24 17:35:52.207946 - step 33 of 120 - 6000 active elements (0 deactivated)
09:36:28 DEBUG opendrift:2108: 0 elements scheduled.
09:36:28 DEBUG opendrift:2110: ======================================================================
09:36:28 DEBUG opendrift:2121: 35.041543827271035 <- latitude -> 35.66702304352362
09:36:28 DEBUG opendrift:2121: 23.170382528240346 <- longitude -> 23.43315954967957
09:36:28 DEBUG opendrift:2121: -123.81549375909756 <- z -> 0.0
09:36:28 DEBUG opendrift:2122: ---------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:28 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 4 elements, expanding data 1
09:36:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.13) and
after (2025-05-24 18:00:00, weight 0.87) in time
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 15:00:00 (before)
2025-05-24 18:00:00 (after)
09:36:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 15:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 18:00:00) in space (linearNDFast)
09:36:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 15:00:00, weight 0.13) and
after (2025-05-24 18:00:00, weight 0.87) in time
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:28 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:28 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:28 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0821036 (min) 0.316489 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0463577 (min) 0.455478 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.90473 (min) -0.129911 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.34047 (min) 5.46239 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:28 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:28 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.414738, mean: 0.942256, max: 1.307566
09:36:28 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:28 DEBUG opendrift.models.physics_methods:917: min: 3.508461, mean: 5.270400, max: 6.229617
09:36:28 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.508461, mean: 5.270400, max: 6.229617
09:36:28 DEBUG opendrift:648: No elements hit coastline.
09:36:28 DEBUG opendrift:1745: No elements to deactivate
09:36:28 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:28 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:28 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:28 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:28 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:28 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:28 DEBUG opendrift.models.physics_methods:917: min: 3.508461, mean: 5.270400, max: 6.229617
09:36:28 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:28 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.014103, dN_50: 0.001107
09:36:28 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:28 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.019182629566002328
09:36:28 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:28 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:28 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:28 DEBUG opendrift.models.physics_methods:771: Advecting 1022 of 6000 elements above 0.100m with wind-sheared ocean current (0.030079 m/s - 0.187883 m/s)
09:36:28 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:28 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018283509639090813 and 0.4238601891108635 m/s
09:36:28 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:28 DEBUG opendrift:2101: ======================================================================
09:36:28 INFO opendrift:2102: 2025-05-24 18:35:52.207946 - step 34 of 120 - 6000 active elements (0 deactivated)
09:36:28 DEBUG opendrift:2108: 0 elements scheduled.
09:36:28 DEBUG opendrift:2110: ======================================================================
09:36:28 DEBUG opendrift:2121: 35.04266575401423 <- latitude -> 35.68174137431303
09:36:28 DEBUG opendrift:2121: 23.166369971249576 <- longitude -> 23.438971827560703
09:36:28 DEBUG opendrift:2121: -122.6373280944179 <- z -> 0.0
09:36:28 DEBUG opendrift:2122: ---------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:28 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:30 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:33 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:33 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:33 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:33 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x23x22) for time after (2025-05-24 21:00:00)
09:36:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.80) and
after (2025-05-24 21:00:00, weight 0.20) in time
09:36:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:34 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:34 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:34 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-24 21:00:00)
09:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.80) and
after (2025-05-24 21:00:00, weight 0.20) in time
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:34 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.091555 (min) 0.272597 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0566446 (min) 0.410975 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.30746 (min) -0.275277 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.51311 (min) 4.95725 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:34 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.251188, mean: 0.946607, max: 1.468980
09:36:34 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:34 DEBUG opendrift.models.physics_methods:917: min: 2.730416, mean: 5.248153, max: 6.602943
09:36:34 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.730416, mean: 5.248153, max: 6.602943
09:36:34 DEBUG opendrift:648: No elements hit coastline.
09:36:34 DEBUG opendrift:1745: No elements to deactivate
09:36:34 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:34 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:34 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:34 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:34 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:34 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:34 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:34 DEBUG opendrift.models.physics_methods:917: min: 2.730416, mean: 5.248153, max: 6.602943
09:36:34 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:34 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.015321, dN_50: 0.001202
09:36:34 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:34 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.021550134545188164
09:36:34 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:34 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:34 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:34 DEBUG opendrift.models.physics_methods:771: Advecting 1027 of 6000 elements above 0.100m with wind-sheared ocean current (0.039564 m/s - 0.181449 m/s)
09:36:34 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:34 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0019520467603742014 and 0.46510201652138533 m/s
09:36:34 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:34 DEBUG opendrift:2101: ======================================================================
09:36:34 INFO opendrift:2102: 2025-05-24 19:35:52.207946 - step 35 of 120 - 6000 active elements (0 deactivated)
09:36:34 DEBUG opendrift:2108: 0 elements scheduled.
09:36:34 DEBUG opendrift:2110: ======================================================================
09:36:34 DEBUG opendrift:2121: 35.04139237303789 <- latitude -> 35.69283380910598
09:36:34 DEBUG opendrift:2121: 23.16309512500164 <- longitude -> 23.43994356223224
09:36:34 DEBUG opendrift:2121: -121.38403804210648 <- z -> 0.0
09:36:34 DEBUG opendrift:2122: ---------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:34 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.47) and
after (2025-05-24 21:00:00, weight 0.53) in time
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.47) and
after (2025-05-24 21:00:00, weight 0.53) in time
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:34 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0988705 (min) 0.209073 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0176062 (min) 0.405413 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.98286 (min) -0.693557 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.46907 (min) 4.87487 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:34 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.161802, mean: 1.034655, max: 1.793460
09:36:34 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:34 DEBUG opendrift.models.physics_methods:917: min: 2.191398, mean: 5.439725, max: 7.295842
09:36:34 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.191398, mean: 5.439725, max: 7.295842
09:36:34 DEBUG opendrift:648: No elements hit coastline.
09:36:34 DEBUG opendrift:1745: No elements to deactivate
09:36:34 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:34 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:34 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:34 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:34 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:34 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:34 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:34 DEBUG opendrift.models.physics_methods:917: min: 2.191398, mean: 5.439725, max: 7.295842
09:36:34 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:34 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.016258, dN_50: 0.001276
09:36:34 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:34 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.026309379579633165
09:36:34 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:34 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:34 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:34 DEBUG opendrift.models.physics_methods:771: Advecting 1033 of 6000 elements above 0.100m with wind-sheared ocean current (0.040176 m/s - 0.184227 m/s)
09:36:34 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:34 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0035582553352488783 and 0.4788246250206879 m/s
09:36:34 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:34 DEBUG opendrift:2101: ======================================================================
09:36:34 INFO opendrift:2102: 2025-05-24 20:35:52.207946 - step 36 of 120 - 6000 active elements (0 deactivated)
09:36:34 DEBUG opendrift:2108: 0 elements scheduled.
09:36:34 DEBUG opendrift:2110: ======================================================================
09:36:34 DEBUG opendrift:2121: 35.042350075747194 <- latitude -> 35.691721459083126
09:36:34 DEBUG opendrift:2121: 23.157800667570328 <- longitude -> 23.446570592248598
09:36:34 DEBUG opendrift:2121: -120.84367059491001 <- z -> 0.0
09:36:34 DEBUG opendrift:2122: ---------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:34 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.13) and
after (2025-05-24 21:00:00, weight 0.87) in time
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 18:00:00 (before)
2025-05-24 21:00:00 (after)
09:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 18:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-24 21:00:00) in space (linearNDFast)
09:36:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 18:00:00, weight 0.13) and
after (2025-05-24 21:00:00, weight 0.87) in time
09:36:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:34 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.110946 (min) 0.14368 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0356489 (min) 0.367483 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.66698 (min) -1.313 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.75489 (min) 4.89647 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:34 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.122452, mean: 1.136461, max: 2.164447
09:36:34 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:34 DEBUG opendrift.models.physics_methods:917: min: 1.906393, mean: 5.652075, max: 8.014992
09:36:34 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.906393, mean: 5.652075, max: 8.014992
09:36:34 DEBUG opendrift:648: No elements hit coastline.
09:36:35 DEBUG opendrift:1745: No elements to deactivate
09:36:35 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:35 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:35 DEBUG opendrift.models.physics_methods:917: min: 1.906393, mean: 5.652074, max: 8.014992
09:36:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.017156, dN_50: 0.001346
09:36:35 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:35 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.031750742935766914
09:36:35 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:35 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:35 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:35 DEBUG opendrift.models.physics_methods:771: Advecting 1032 of 6000 elements above 0.100m with wind-sheared ocean current (0.063093 m/s - 0.190285 m/s)
09:36:35 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:35 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010903346356779173 and 0.4498663622548493 m/s
09:36:35 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:35 DEBUG opendrift:2101: ======================================================================
09:36:35 INFO opendrift:2102: 2025-05-24 21:35:52.207946 - step 37 of 120 - 6000 active elements (0 deactivated)
09:36:35 DEBUG opendrift:2108: 0 elements scheduled.
09:36:35 DEBUG opendrift:2110: ======================================================================
09:36:35 DEBUG opendrift:2121: 35.04948363018304 <- latitude -> 35.69813836753363
09:36:35 DEBUG opendrift:2121: 23.151367262484648 <- longitude -> 23.44584572440973
09:36:35 DEBUG opendrift:2121: -120.16017052254601 <- z -> 0.0
09:36:35 DEBUG opendrift:2122: ---------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:35 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:36 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:36 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:36 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:36 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x24x22) for time after (2025-05-25 00:00:00)
09:36:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.80) and
after (2025-05-25 00:00:00, weight 0.20) in time
09:36:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:37 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:37 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:37 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 00:00:00)
09:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.80) and
after (2025-05-25 00:00:00, weight 0.20) in time
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:37 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.164486 (min) 0.087641 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0471035 (min) 0.392523 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.05738 (min) -1.72306 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.86902 (min) 5.6349 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:37 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.158969, mean: 1.263080, max: 2.338426
09:36:37 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 2.172131, mean: 5.986303, max: 8.330891
09:36:37 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.172131, mean: 5.986303, max: 8.330891
09:36:37 DEBUG opendrift:648: No elements hit coastline.
09:36:37 DEBUG opendrift:1745: No elements to deactivate
09:36:37 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:37 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:37 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:37 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:37 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 2.172131, mean: 5.986303, max: 8.330891
09:36:37 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:37 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.017116, dN_50: 0.001343
09:36:37 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:37 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.034302547665344886
09:36:37 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:37 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:37 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:37 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1029 surface elements
09:36:37 DEBUG opendrift.models.physics_methods:771: Advecting 1039 of 6000 elements above 0.100m with wind-sheared ocean current (0.033060 m/s - 0.219015 m/s)
09:36:37 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:37 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.00198047339323146 and 0.4253184457134359 m/s
09:36:37 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:37 DEBUG opendrift:2101: ======================================================================
09:36:37 INFO opendrift:2102: 2025-05-24 22:35:52.207946 - step 38 of 120 - 6000 active elements (0 deactivated)
09:36:37 DEBUG opendrift:2108: 0 elements scheduled.
09:36:37 DEBUG opendrift:2110: ======================================================================
09:36:37 DEBUG opendrift:2121: 35.05257098889072 <- latitude -> 35.7002878995094
09:36:37 DEBUG opendrift:2121: 23.14487366550622 <- longitude -> 23.44880410313658
09:36:37 DEBUG opendrift:2121: -119.08773467421567 <- z -> 0.0
09:36:37 DEBUG opendrift:2122: ---------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:37 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.47) and
after (2025-05-25 00:00:00, weight 0.53) in time
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.47) and
after (2025-05-25 00:00:00, weight 0.53) in time
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:37 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.209298 (min) 0.0456316 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0138052 (min) 0.428679 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.33367 (min) -2.11385 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.58767 (min) 6.75847 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:37 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.274644, mean: 1.425636, max: 2.419630
09:36:37 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 2.855057, mean: 6.422619, max: 8.474305
09:36:37 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.855057, mean: 6.422619, max: 8.474305
09:36:37 DEBUG opendrift:648: No elements hit coastline.
09:36:37 DEBUG opendrift:1745: No elements to deactivate
09:36:37 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:37 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:37 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:37 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:37 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 2.855057, mean: 6.422618, max: 8.474304
09:36:37 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:37 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.016578, dN_50: 0.001301
09:36:37 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:37 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03549357790527012
09:36:37 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:37 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:37 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:37 DEBUG opendrift.models.physics_methods:771: Advecting 1044 of 6000 elements above 0.100m with wind-sheared ocean current (0.005406 m/s - 0.260726 m/s)
09:36:37 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:37 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006931579280825805 and 0.4368256885859911 m/s
09:36:37 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:37 DEBUG opendrift:2101: ======================================================================
09:36:37 INFO opendrift:2102: 2025-05-24 23:35:52.207946 - step 39 of 120 - 6000 active elements (0 deactivated)
09:36:37 DEBUG opendrift:2108: 0 elements scheduled.
09:36:37 DEBUG opendrift:2110: ======================================================================
09:36:37 DEBUG opendrift:2121: 35.05972478392029 <- latitude -> 35.70239362852543
09:36:37 DEBUG opendrift:2121: 23.136827694624017 <- longitude -> 23.45059909296086
09:36:37 DEBUG opendrift:2121: -118.06993938001331 <- z -> 0.0
09:36:37 DEBUG opendrift:2122: ---------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:37 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.13) and
after (2025-05-25 00:00:00, weight 0.87) in time
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-24 21:00:00 (before)
2025-05-25 00:00:00 (after)
09:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-24 21:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 00:00:00) in space (linearNDFast)
09:36:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-24 21:00:00, weight 0.13) and
after (2025-05-25 00:00:00, weight 0.87) in time
09:36:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:37 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.258572 (min) 0.04051 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.026495 (min) 0.50326 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: -9.55937 (min) -2.54561 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: 2.78861 (min) 7.54309 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:37 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.431325, mean: 1.622768, max: 2.494472
09:36:37 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 3.577930, mean: 6.894454, max: 8.604366
09:36:37 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.577930, mean: 6.894454, max: 8.604366
09:36:37 DEBUG opendrift:648: No elements hit coastline.
09:36:37 DEBUG opendrift:1745: No elements to deactivate
09:36:37 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:37 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:37 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:37 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:37 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:37 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:37 DEBUG opendrift.models.physics_methods:917: min: 3.577930, mean: 6.894453, max: 8.604365
09:36:37 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:37 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.016130, dN_50: 0.001266
09:36:37 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:37 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03659130627536634
09:36:37 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:37 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:37 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:37 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
09:36:37 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
09:36:38 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
09:36:38 DEBUG opendrift.models.physics_methods:771: Advecting 1051 of 6000 elements above 0.100m with wind-sheared ocean current (0.028947 m/s - 0.292907 m/s)
09:36:38 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:38 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001894280241066813 and 0.4239115349360826 m/s
09:36:38 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:38 DEBUG opendrift:2101: ======================================================================
09:36:38 INFO opendrift:2102: 2025-05-25 00:35:52.207946 - step 40 of 120 - 6000 active elements (0 deactivated)
09:36:38 DEBUG opendrift:2108: 0 elements scheduled.
09:36:38 DEBUG opendrift:2110: ======================================================================
09:36:38 DEBUG opendrift:2121: 35.06447977034491 <- latitude -> 35.71417959212075
09:36:38 DEBUG opendrift:2121: 23.120744865172064 <- longitude -> 23.447848563482157
09:36:38 DEBUG opendrift:2121: -117.49913032987261 <- z -> 0.0
09:36:38 DEBUG opendrift:2122: ---------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:38 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:38 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:38 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:39 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:39 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:39 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:39 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x24x22) for time after (2025-05-25 03:00:00)
09:36:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.80) and
after (2025-05-25 03:00:00, weight 0.20) in time
09:36:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:39 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:40 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:40 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:40 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 03:00:00)
09:36:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.80) and
after (2025-05-25 03:00:00, weight 0.20) in time
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:40 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.274793 (min) 0.0465157 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0843576 (min) 0.568305 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_wind: -8.95532 (min) -2.68881 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.2321 (min) 8.54422 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:40 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.625536, mean: 1.799960, max: 2.649536
09:36:40 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 4.308794, mean: 7.288165, max: 8.867772
09:36:40 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.308794, mean: 7.288165, max: 8.867772
09:36:40 DEBUG opendrift:648: No elements hit coastline.
09:36:40 DEBUG opendrift:1745: No elements to deactivate
09:36:40 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:40 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:40 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:40 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:40 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 4.308794, mean: 7.288166, max: 8.867772
09:36:40 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:40 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.015738, dN_50: 0.001235
09:36:40 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:40 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03886568037704051
09:36:40 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:40 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:40 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1051 surface elements
09:36:40 DEBUG opendrift.models.physics_methods:771: Advecting 1058 of 6000 elements above 0.100m with wind-sheared ocean current (0.035427 m/s - 0.310529 m/s)
09:36:40 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:40 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0024842471784800603 and 0.419317696019614 m/s
09:36:40 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:40 DEBUG opendrift:2101: ======================================================================
09:36:40 INFO opendrift:2102: 2025-05-25 01:35:52.207946 - step 41 of 120 - 6000 active elements (0 deactivated)
09:36:40 DEBUG opendrift:2108: 0 elements scheduled.
09:36:40 DEBUG opendrift:2110: ======================================================================
09:36:40 DEBUG opendrift:2121: 35.068073491802814 <- latitude -> 35.71956043648575
09:36:40 DEBUG opendrift:2121: 23.10128580946216 <- longitude -> 23.44780527275281
09:36:40 DEBUG opendrift:2121: -116.45468941785886 <- z -> 0.0
09:36:40 DEBUG opendrift:2122: ---------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.47) and
after (2025-05-25 03:00:00, weight 0.53) in time
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.47) and
after (2025-05-25 03:00:00, weight 0.53) in time
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:40 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.26397 (min) 0.0630053 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.133076 (min) 0.629793 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.90375 (min) -2.70849 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_wind: 4.05964 (min) 10.0108 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:40 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.930272, mean: 2.044918, max: 3.086739
09:36:40 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 5.254539, mean: 7.761341, max: 9.571490
09:36:40 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.254539, mean: 7.761341, max: 9.571490
09:36:40 DEBUG opendrift:648: No elements hit coastline.
09:36:40 DEBUG opendrift:1745: No elements to deactivate
09:36:40 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:40 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:40 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:40 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:40 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 5.254539, mean: 7.761341, max: 9.571489
09:36:40 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:40 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.015331, dN_50: 0.001203
09:36:40 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:40 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.04527825189088064
09:36:40 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:40 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:40 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1051 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1050 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1050 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:40 DEBUG opendrift.models.physics_methods:771: Advecting 1065 of 6000 elements above 0.100m with wind-sheared ocean current (0.053376 m/s - 0.336050 m/s)
09:36:40 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:40 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007222447785961865 and 0.4220613910967817 m/s
09:36:40 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:40 DEBUG opendrift:2101: ======================================================================
09:36:40 INFO opendrift:2102: 2025-05-25 02:35:52.207946 - step 42 of 120 - 6000 active elements (0 deactivated)
09:36:40 DEBUG opendrift:2108: 0 elements scheduled.
09:36:40 DEBUG opendrift:2110: ======================================================================
09:36:40 DEBUG opendrift:2121: 35.077389760544804 <- latitude -> 35.732456844393184
09:36:40 DEBUG opendrift:2121: 23.081910809826056 <- longitude -> 23.44336715643245
09:36:40 DEBUG opendrift:2121: -115.5496783110231 <- z -> 0.0
09:36:40 DEBUG opendrift:2122: ---------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.13) and
after (2025-05-25 03:00:00, weight 0.87) in time
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 00:00:00 (before)
2025-05-25 03:00:00 (after)
09:36:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 00:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 03:00:00) in space (linearNDFast)
09:36:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 00:00:00, weight 0.13) and
after (2025-05-25 03:00:00, weight 0.87) in time
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:40 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:40 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.256339 (min) 0.0822247 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.130403 (min) 0.706872 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: x_wind: -7.02449 (min) -2.62979 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.03661 (min) 11.4874 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:40 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:40 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.225619, mean: 2.408730, max: 3.719501
09:36:40 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 6.031249, mean: 8.391439, max: 10.506835
09:36:40 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.031249, mean: 8.391439, max: 10.506835
09:36:40 DEBUG opendrift:648: No elements hit coastline.
09:36:40 DEBUG opendrift:1745: No elements to deactivate
09:36:40 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:40 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:40 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:40 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:40 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:40 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:40 DEBUG opendrift.models.physics_methods:917: min: 6.031250, mean: 8.391438, max: 10.506835
09:36:40 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:40 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.014983, dN_50: 0.001176
09:36:40 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:40 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.05455915430461994
09:36:40 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:40 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:40 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1054 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:40 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:40 DEBUG opendrift.models.physics_methods:771: Advecting 1062 of 6000 elements above 0.100m with wind-sheared ocean current (0.026217 m/s - 0.368889 m/s)
09:36:40 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:40 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014377826745921476 and 0.47618968222355185 m/s
09:36:40 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:40 DEBUG opendrift:2101: ======================================================================
09:36:40 INFO opendrift:2102: 2025-05-25 03:35:52.207946 - step 43 of 120 - 6000 active elements (0 deactivated)
09:36:40 DEBUG opendrift:2108: 0 elements scheduled.
09:36:40 DEBUG opendrift:2110: ======================================================================
09:36:40 DEBUG opendrift:2121: 35.083089140032364 <- latitude -> 35.749486285234774
09:36:40 DEBUG opendrift:2121: 23.06840448622576 <- longitude -> 23.44436263782441
09:36:40 DEBUG opendrift:2121: -114.45271544141903 <- z -> 0.0
09:36:40 DEBUG opendrift:2122: ---------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:40 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:40 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:42 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:42 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:42 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:42 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 14x24x22) for time after (2025-05-25 06:00:00)
09:36:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:42 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:42 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:42 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:42 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.80) and
after (2025-05-25 06:00:00, weight 0.20) in time
09:36:42 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:42 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:42 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:42 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:42 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:42 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:42 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:42 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:42 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:42 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:42 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:42 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:42 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:42 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 06:00:00)
09:36:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:42 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:42 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:42 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:42 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.80) and
after (2025-05-25 06:00:00, weight 0.20) in time
09:36:42 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:42 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:42 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:42 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:42 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:42 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:42 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:42 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:42 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:42 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.170723 (min) 0.0917602 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.120672 (min) 0.725722 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: x_wind: -6.10065 (min) -1.83197 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.50048 (min) 11.6752 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:42 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:42 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.392468, mean: 2.392985, max: 3.564004
09:36:42 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:42 DEBUG opendrift.models.physics_methods:917: min: 6.428687, mean: 8.357531, max: 10.284867
09:36:42 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.428687, mean: 8.357531, max: 10.284867
09:36:42 DEBUG opendrift:648: No elements hit coastline.
09:36:43 DEBUG opendrift:1745: No elements to deactivate
09:36:43 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:43 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:43 DEBUG opendrift.models.physics_methods:917: min: 6.428687, mean: 8.357532, max: 10.284867
09:36:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.015718, dN_50: 0.001234
09:36:43 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:43 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.052278437621936756
09:36:43 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:43 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:43 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
09:36:43 DEBUG opendrift.models.physics_methods:771: Advecting 1072 of 6000 elements above 0.100m with wind-sheared ocean current (0.003587 m/s - 0.360774 m/s)
09:36:43 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:43 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0024130773032864654 and 0.42155808443670395 m/s
09:36:43 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:43 DEBUG opendrift:2101: ======================================================================
09:36:43 INFO opendrift:2102: 2025-05-25 04:35:52.207946 - step 44 of 120 - 6000 active elements (0 deactivated)
09:36:43 DEBUG opendrift:2108: 0 elements scheduled.
09:36:43 DEBUG opendrift:2110: ======================================================================
09:36:43 DEBUG opendrift:2121: 35.08815795041045 <- latitude -> 35.77082611713271
09:36:43 DEBUG opendrift:2121: 23.062258404653534 <- longitude -> 23.44859558543592
09:36:43 DEBUG opendrift:2121: -113.4532925995056 <- z -> 0.0
09:36:43 DEBUG opendrift:2122: ---------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.47) and
after (2025-05-25 06:00:00, weight 0.53) in time
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.47) and
after (2025-05-25 06:00:00, weight 0.53) in time
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:43 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:43 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0946119 (min) 0.110118 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0977326 (min) 0.725323 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: x_wind: -5.2596 (min) -0.463367 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.69782 (min) 10.8506 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:43 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.387478, mean: 2.119969, max: 3.029664
09:36:43 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:43 DEBUG opendrift.models.physics_methods:917: min: 6.417156, mean: 7.883461, max: 9.482586
09:36:43 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.417156, mean: 7.883461, max: 9.482586
09:36:43 DEBUG opendrift:648: No elements hit coastline.
09:36:43 DEBUG opendrift:1745: No elements to deactivate
09:36:43 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:43 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:43 DEBUG opendrift.models.physics_methods:917: min: 6.417156, mean: 7.883461, max: 9.482586
09:36:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.017203, dN_50: 0.001350
09:36:43 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:43 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.044441115812446405
09:36:43 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:43 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:43 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
09:36:43 DEBUG opendrift.models.physics_methods:771: Advecting 1059 of 6000 elements above 0.100m with wind-sheared ocean current (0.068673 m/s - 0.329810 m/s)
09:36:43 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:43 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.004101206223366215 and 0.4414615296049425 m/s
09:36:43 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:43 DEBUG opendrift:2101: ======================================================================
09:36:43 INFO opendrift:2102: 2025-05-25 05:35:52.207946 - step 45 of 120 - 6000 active elements (0 deactivated)
09:36:43 DEBUG opendrift:2108: 0 elements scheduled.
09:36:43 DEBUG opendrift:2110: ======================================================================
09:36:43 DEBUG opendrift:2121: 35.08974323798381 <- latitude -> 35.78773776593284
09:36:43 DEBUG opendrift:2121: 23.060675085660254 <- longitude -> 23.44677774411137
09:36:43 DEBUG opendrift:2121: -112.23626852403285 <- z -> 0.0
09:36:43 DEBUG opendrift:2122: ---------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.13) and
after (2025-05-25 06:00:00, weight 0.87) in time
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 03:00:00 (before)
2025-05-25 06:00:00 (after)
09:36:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 03:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 06:00:00) in space (linearNDFast)
09:36:43 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:43 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 03:00:00, weight 0.13) and
after (2025-05-25 06:00:00, weight 0.87) in time
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:43 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:43 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:43 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0816812 (min) 0.246915 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0665036 (min) 0.743962 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: x_wind: -4.53174 (min) 0.944523 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.89089 (min) 10.0228 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:43 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:43 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.221091, mean: 1.932521, max: 2.550883
09:36:43 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:43 DEBUG opendrift.models.physics_methods:917: min: 6.020099, mean: 7.534499, max: 8.701114
09:36:43 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.020099, mean: 7.534499, max: 8.701114
09:36:43 DEBUG opendrift:648: No elements hit coastline.
09:36:43 DEBUG opendrift:1745: No elements to deactivate
09:36:43 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:43 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:43 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:43 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:43 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:43 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:43 DEBUG opendrift.models.physics_methods:917: min: 6.020099, mean: 7.534499, max: 8.701115
09:36:43 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:43 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.018703, dN_50: 0.001468
09:36:43 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:43 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0374187151825922
09:36:43 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:43 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:43 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:43 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
09:36:43 DEBUG opendrift.models.physics_methods:771: Advecting 1064 of 6000 elements above 0.100m with wind-sheared ocean current (0.106821 m/s - 0.303883 m/s)
09:36:43 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:43 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0017908188912365063 and 0.41383519308694083 m/s
09:36:43 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:43 DEBUG opendrift:2101: ======================================================================
09:36:43 INFO opendrift:2102: 2025-05-25 06:35:52.207946 - step 46 of 120 - 6000 active elements (0 deactivated)
09:36:43 DEBUG opendrift:2108: 0 elements scheduled.
09:36:43 DEBUG opendrift:2110: ======================================================================
09:36:43 DEBUG opendrift:2121: 35.092950045529946 <- latitude -> 35.81588049722957
09:36:43 DEBUG opendrift:2121: 23.06729558496365 <- longitude -> 23.451896188594315
09:36:43 DEBUG opendrift:2121: -111.43951776035291 <- z -> 0.0
09:36:43 DEBUG opendrift:2122: ---------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:43 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:43 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:43 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:43 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:43 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:43 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:43 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:43 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:43 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:45 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:47 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:47 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:47 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:47 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 14x26x22) for time after (2025-05-25 09:00:00)
09:36:47 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:47 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:47 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:47 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:47 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.80) and
after (2025-05-25 09:00:00, weight 0.20) in time
09:36:47 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:47 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:47 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:47 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:47 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:47 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:47 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:47 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:47 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:47 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:47 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:48 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:48 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:48 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:48 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:48 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 09:00:00)
09:36:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.80) and
after (2025-05-25 09:00:00, weight 0.20) in time
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:48 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0844094 (min) 0.302455 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0332011 (min) 0.706132 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.69179 (min) 2.29492 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.59442 (min) 9.95592 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:48 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.007521, mean: 1.850874, max: 2.489868
09:36:48 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:48 DEBUG opendrift.models.physics_methods:917: min: 5.468355, mean: 7.344551, max: 8.596423
09:36:48 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.468355, mean: 7.344551, max: 8.596423
09:36:48 DEBUG opendrift:648: No elements hit coastline.
09:36:48 DEBUG opendrift:1745: No elements to deactivate
09:36:48 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:48 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:48 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:48 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:48 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:48 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:48 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:48 DEBUG opendrift.models.physics_methods:917: min: 5.468355, mean: 7.344552, max: 8.596423
09:36:48 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:48 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.019799, dN_50: 0.001554
09:36:48 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:48 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.036523787226503894
09:36:48 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:48 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:48 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1060 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1061 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1058 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1060 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1059 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:48 DEBUG opendrift.models.physics_methods:771: Advecting 1068 of 6000 elements above 0.100m with wind-sheared ocean current (0.004587 m/s - 0.301816 m/s)
09:36:48 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:48 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002401565935798247 and 0.4285563959528655 m/s
09:36:48 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:48 DEBUG opendrift:2101: ======================================================================
09:36:48 INFO opendrift:2102: 2025-05-25 07:35:52.207946 - step 47 of 120 - 6000 active elements (0 deactivated)
09:36:48 DEBUG opendrift:2108: 0 elements scheduled.
09:36:48 DEBUG opendrift:2110: ======================================================================
09:36:48 DEBUG opendrift:2121: 35.099850455892344 <- latitude -> 35.84045398698472
09:36:48 DEBUG opendrift:2121: 23.072889539108925 <- longitude -> 23.452378513706588
09:36:48 DEBUG opendrift:2121: -110.55833317407189 <- z -> 0.0
09:36:48 DEBUG opendrift:2122: ---------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:48 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:48 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.47) and
after (2025-05-25 09:00:00, weight 0.53) in time
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.47) and
after (2025-05-25 09:00:00, weight 0.53) in time
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:48 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.0979497 (min) 0.353717 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.000914167 (min) 0.591883 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.78574 (min) 3.4724 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 5.09316 (min) 10.3836 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:48 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.762781, mean: 1.847584, max: 2.731523
09:36:48 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:48 DEBUG opendrift.models.physics_methods:917: min: 4.758055, mean: 7.287172, max: 9.003928
09:36:48 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.758055, mean: 7.287172, max: 9.003928
09:36:48 DEBUG opendrift:648: No elements hit coastline.
09:36:48 DEBUG opendrift:1745: No elements to deactivate
09:36:48 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:48 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:48 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:48 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:48 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:48 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:48 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:48 DEBUG opendrift.models.physics_methods:917: min: 4.758055, mean: 7.287171, max: 9.003928
09:36:48 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:48 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.020510, dN_50: 0.001610
09:36:48 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:48 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0400682076467992
09:36:48 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:48 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:48 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1058 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1057 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1056 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1055 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1054 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1053 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1052 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1051 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1050 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1049 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1046 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1046 surface elements
09:36:48 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:48 DEBUG opendrift.models.physics_methods:771: Advecting 1061 of 6000 elements above 0.100m with wind-sheared ocean current (0.051399 m/s - 0.316123 m/s)
09:36:48 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:48 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.003153510652613518 and 0.47529544875288304 m/s
09:36:48 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:48 DEBUG opendrift:2101: ======================================================================
09:36:48 INFO opendrift:2102: 2025-05-25 08:35:52.207946 - step 48 of 120 - 6000 active elements (0 deactivated)
09:36:48 DEBUG opendrift:2108: 0 elements scheduled.
09:36:48 DEBUG opendrift:2110: ======================================================================
09:36:48 DEBUG opendrift:2121: 35.099122463272366 <- latitude -> 35.861101836330995
09:36:48 DEBUG opendrift:2121: 23.082021164593755 <- longitude -> 23.45145345290458
09:36:48 DEBUG opendrift:2121: -109.70592754479289 <- z -> 0.0
09:36:48 DEBUG opendrift:2122: ---------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:48 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:48 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:48 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:48 WARNING opendrift.readers.basereader.structured:326: Data block from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd not large enough to cover element positions within timestep. Buffer size (4) must be increased. See `Variables.set_buffer_size`.
09:36:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:48 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:48 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 75 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:49 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:49 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.13) and
after (2025-05-25 09:00:00, weight 0.87) in time
09:36:49 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 06:00:00 (before)
2025-05-25 09:00:00 (after)
09:36:49 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 06:00:00) in space (linearNDFast)
09:36:49 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:49 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 09:00:00) in space (linearNDFast)
09:36:49 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:49 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 06:00:00, weight 0.13) and
after (2025-05-25 09:00:00, weight 0.87) in time
09:36:49 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:49 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:49 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:49 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:49 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.110836 (min) 0.466119 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0294472 (min) 0.530492 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.9155 (min) 4.5558 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 4.52174 (min) 10.8607 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:49 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.557053, mean: 1.915889, max: 3.111945
09:36:49 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:49 DEBUG opendrift.models.physics_methods:917: min: 4.066098, mean: 7.357812, max: 9.610490
09:36:49 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.066098, mean: 7.357812, max: 9.610490
09:36:49 DEBUG opendrift:648: No elements hit coastline.
09:36:49 DEBUG opendrift:1745: No elements to deactivate
09:36:49 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:49 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:49 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:49 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:49 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:49 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:49 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:49 DEBUG opendrift.models.physics_methods:917: min: 4.066098, mean: 7.357812, max: 9.610490
09:36:49 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:49 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.021051, dN_50: 0.001652
09:36:49 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:49 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.045647958434709285
09:36:49 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:49 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:49 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1048 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1047 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1046 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1045 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1044 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1044 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1043 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 1044 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1043 surface elements
09:36:49 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1042 surface elements
09:36:49 DEBUG opendrift.models.physics_methods:771: Advecting 1050 of 6000 elements above 0.100m with wind-sheared ocean current (0.022887 m/s - 0.337419 m/s)
09:36:49 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:49 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0003291284235230825 and 0.4203852057432633 m/s
09:36:49 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:49 DEBUG opendrift:2101: ======================================================================
09:36:49 INFO opendrift:2102: 2025-05-25 09:35:52.207946 - step 49 of 120 - 6000 active elements (0 deactivated)
09:36:49 DEBUG opendrift:2108: 0 elements scheduled.
09:36:49 DEBUG opendrift:2110: ======================================================================
09:36:49 DEBUG opendrift:2121: 35.100219037821134 <- latitude -> 35.87774928751696
09:36:49 DEBUG opendrift:2121: 23.093596759660468 <- longitude -> 23.450382972297042
09:36:49 DEBUG opendrift:2121: -108.71791673370937 <- z -> 0.0
09:36:49 DEBUG opendrift:2122: ---------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:49 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:49 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:49 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:49 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:49 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:49 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:49 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 14x27x22) for time after (2025-05-25 12:00:00)
09:36:49 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:49 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:49 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:49 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:36:49 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.80) and
after (2025-05-25 12:00:00, weight 0.20) in time
09:36:49 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:49 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:49 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:50 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:50 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:50 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:50 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 12:00:00)
09:36:50 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:50 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:50 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:50 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:50 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.80) and
after (2025-05-25 12:00:00, weight 0.20) in time
09:36:50 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:50 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:50 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:50 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:50 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.125446 (min) 0.565578 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0457734 (min) 0.450246 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.170084 (min) 5.21796 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.94206 (min) 9.64126 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:50 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.383648, mean: 1.566166, max: 2.613001
09:36:50 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:50 DEBUG opendrift.models.physics_methods:917: min: 3.374395, mean: 6.647848, max: 8.806420
09:36:50 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.374395, mean: 6.647848, max: 8.806420
09:36:50 DEBUG opendrift:648: No elements hit coastline.
09:36:50 DEBUG opendrift:1745: No elements to deactivate
09:36:50 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:50 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:50 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:50 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:50 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:50 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:50 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:50 DEBUG opendrift.models.physics_methods:917: min: 3.374395, mean: 6.647848, max: 8.806420
09:36:50 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:50 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.023530, dN_50: 0.001847
09:36:50 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:50 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03832981513372682
09:36:50 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:50 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:50 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1041 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1040 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1039 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:50 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1038 surface elements
09:36:50 DEBUG opendrift.models.physics_methods:771: Advecting 1045 of 6000 elements above 0.100m with wind-sheared ocean current (0.108420 m/s - 0.309189 m/s)
09:36:50 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:50 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001606695166277498 and 0.422320037252356 m/s
09:36:50 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:50 DEBUG opendrift:2101: ======================================================================
09:36:50 INFO opendrift:2102: 2025-05-25 10:35:52.207946 - step 50 of 120 - 6000 active elements (0 deactivated)
09:36:50 DEBUG opendrift:2108: 0 elements scheduled.
09:36:50 DEBUG opendrift:2110: ======================================================================
09:36:50 DEBUG opendrift:2121: 35.10091890838902 <- latitude -> 35.89690845392535
09:36:50 DEBUG opendrift:2121: 23.10608917085655 <- longitude -> 23.45051047527792
09:36:50 DEBUG opendrift:2121: -108.13266423649473 <- z -> 0.0
09:36:50 DEBUG opendrift:2122: ---------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:50 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 6000 elements
09:36:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:50 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:50 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 6000 elements
09:36:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:52 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:52 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:52 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 6000 elements
09:36:52 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:52 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:52 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:52 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:52 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:52 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.47) and
after (2025-05-25 12:00:00, weight 0.53) in time
09:36:52 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 6000 elements
09:36:52 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 6000 elements
09:36:52 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:52 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:52 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:52 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:52 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:52 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.47) and
after (2025-05-25 12:00:00, weight 0.53) in time
09:36:52 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:52 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:52 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:52 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:52 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.139588 (min) 0.628288 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0415533 (min) 0.38512 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.95826 (min) 5.59244 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 3.36152 (min) 7.30647 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:52 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.496244, mean: 1.189121, max: 1.927084
09:36:52 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:52 DEBUG opendrift.models.physics_methods:917: min: 3.837756, mean: 5.873788, max: 7.562753
09:36:52 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.837756, mean: 5.873788, max: 7.562753
09:36:52 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:36:52 DEBUG opendrift:1718: Added status stranded
09:36:52 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:36:52 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:53 DEBUG opendrift:1749: Removed 4 elements.
09:36:53 DEBUG opendrift:1752: Removed 4 values from environment.
09:36:53 DEBUG opendrift:1757: remove items from profile for z
09:36:53 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:53 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:53 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:53 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:36:53 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:53 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:53 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:53 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:53 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:53 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:53 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:53 DEBUG opendrift.models.physics_methods:917: min: 3.837756, mean: 5.873096, max: 7.562753
09:36:53 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:53 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.027552, dN_50: 0.002162
09:36:53 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:53 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.028269272367564918
09:36:53 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:53 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:53 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:53 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 1036 surface elements
09:36:53 DEBUG opendrift.models.physics_methods:771: Advecting 1046 of 5996 elements above 0.100m with wind-sheared ocean current (0.026520 m/s - 0.265524 m/s)
09:36:53 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:53 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005407970296167011 and 0.47388255321221384 m/s
09:36:53 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:53 DEBUG opendrift:2101: ======================================================================
09:36:53 INFO opendrift:2102: 2025-05-25 11:35:52.207946 - step 51 of 120 - 5996 active elements (4 deactivated)
09:36:53 DEBUG opendrift:2108: 0 elements scheduled.
09:36:53 DEBUG opendrift:2110: ======================================================================
09:36:53 DEBUG opendrift:2121: 35.09977686907338 <- latitude -> 35.90936267431479
09:36:53 DEBUG opendrift:2121: 23.11354980532546 <- longitude -> 23.457240200490588
09:36:53 DEBUG opendrift:2121: -106.94498645752586 <- z -> 0.0
09:36:53 DEBUG opendrift:2122: ---------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:53 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:53 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:53 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:53 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:53 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.13) and
after (2025-05-25 12:00:00, weight 0.87) in time
09:36:53 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5996 elements
09:36:53 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 09:00:00 (before)
2025-05-25 12:00:00 (after)
09:36:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 09:00:00) in space (linearNDFast)
09:36:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:53 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 12:00:00) in space (linearNDFast)
09:36:53 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:53 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 09:00:00, weight 0.13) and
after (2025-05-25 12:00:00, weight 0.87) in time
09:36:53 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:53 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:53 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.156487 (min) 0.7066 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.111749 (min) 0.364545 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 5.44937 (min) 6.95921 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 1.31742 (min) 5.00635 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:53 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.798618, mean: 1.177106, max: 1.807954
09:36:53 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:53 DEBUG opendrift.models.physics_methods:917: min: 4.868544, mean: 5.895710, max: 7.325263
09:36:53 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.868544, mean: 5.895710, max: 7.325263
09:36:53 DEBUG opendrift:651: 16 elements hit land, moving them to the coastline.
09:36:53 DEBUG opendrift:1729: 16 elements scheduled for deactivation (stranded)
09:36:53 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:53 DEBUG opendrift:1749: Removed 16 elements.
09:36:53 DEBUG opendrift:1752: Removed 16 values from environment.
09:36:53 DEBUG opendrift:1757: remove items from profile for z
09:36:53 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:53 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:53 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:53 DEBUG opendrift:1761: Removed 16 values from environment_profiles.
09:36:53 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:53 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:53 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:53 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:53 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:53 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:53 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:53 DEBUG opendrift.models.physics_methods:917: min: 4.868545, mean: 5.896580, max: 7.325263
09:36:53 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:53 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.030567, dN_50: 0.002399
09:36:54 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:54 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02652195828626391
09:36:54 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:54 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:54 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:54 DEBUG opendrift.models.physics_methods:771: Advecting 1032 of 5980 elements above 0.100m with wind-sheared ocean current (0.006647 m/s - 0.244040 m/s)
09:36:54 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:54 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018052701820559132 and 0.4572373966516414 m/s
09:36:54 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:54 DEBUG opendrift:2101: ======================================================================
09:36:54 INFO opendrift:2102: 2025-05-25 12:35:52.207946 - step 52 of 120 - 5980 active elements (20 deactivated)
09:36:54 DEBUG opendrift:2108: 0 elements scheduled.
09:36:54 DEBUG opendrift:2110: ======================================================================
09:36:54 DEBUG opendrift:2121: 35.10187724512265 <- latitude -> 35.919598827079746
09:36:54 DEBUG opendrift:2121: 23.12158161264207 <- longitude -> 23.493951664376606
09:36:54 DEBUG opendrift:2121: -106.12908461024696 <- z -> 0.0
09:36:54 DEBUG opendrift:2122: ---------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:54 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:54 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:54 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:54 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:54 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:54 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:54 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 13x28x22) for time after (2025-05-25 15:00:00)
09:36:54 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:54 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:54 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:54 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 669 elements, expanding data 1
09:36:54 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.80) and
after (2025-05-25 15:00:00, weight 0.20) in time
09:36:54 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5980 elements
09:36:54 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:54 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:54 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:54 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:54 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 7x7x1) for time after (2025-05-25 15:00:00)
09:36:54 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:54 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:54 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:54 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:54 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.80) and
after (2025-05-25 15:00:00, weight 0.20) in time
09:36:54 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:54 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:54 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:54 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:54 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:54 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:54 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:54 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:54 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.16514 (min) 0.697466 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.176462 (min) 0.329319 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: x_wind: 5.85751 (min) 7.72297 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.383021 (min) 3.37734 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:54 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:54 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.847644, mean: 1.237952, max: 1.747846
09:36:54 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:54 DEBUG opendrift.models.physics_methods:917: min: 5.015756, mean: 6.048412, max: 7.202466
09:36:54 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.015756, mean: 6.048412, max: 7.202466
09:36:54 DEBUG opendrift:651: 54 elements hit land, moving them to the coastline.
09:36:54 DEBUG opendrift:1729: 54 elements scheduled for deactivation (stranded)
09:36:54 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:54 DEBUG opendrift:1749: Removed 54 elements.
09:36:54 DEBUG opendrift:1752: Removed 54 values from environment.
09:36:54 DEBUG opendrift:1757: remove items from profile for z
09:36:54 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:54 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:54 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:54 DEBUG opendrift:1761: Removed 54 values from environment_profiles.
09:36:54 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:54 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:54 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:54 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:54 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:54 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:54 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:54 DEBUG opendrift.models.physics_methods:917: min: 5.015756, mean: 6.054668, max: 7.202466
09:36:54 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:54 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.032349, dN_50: 0.002539
09:36:54 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:54 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.025640350102856008
09:36:54 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:54 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:54 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:55 DEBUG opendrift.models.physics_methods:771: Advecting 982 of 5926 elements above 0.100m with wind-sheared ocean current (0.076378 m/s - 0.239659 m/s)
09:36:55 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:55 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012497955501245346 and 0.40828767925583687 m/s
09:36:55 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:55 DEBUG opendrift:2101: ======================================================================
09:36:55 INFO opendrift:2102: 2025-05-25 13:35:52.207946 - step 53 of 120 - 5926 active elements (74 deactivated)
09:36:55 DEBUG opendrift:2108: 0 elements scheduled.
09:36:55 DEBUG opendrift:2110: ======================================================================
09:36:55 DEBUG opendrift:2121: 35.10173094235753 <- latitude -> 35.92419561712722
09:36:55 DEBUG opendrift:2121: 23.122282354574317 <- longitude -> 23.532494258505235
09:36:55 DEBUG opendrift:2121: -105.53536981867826 <- z -> 0.0
09:36:55 DEBUG opendrift:2122: ---------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.47) and
after (2025-05-25 15:00:00, weight 0.53) in time
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5926 elements
09:36:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.47) and
after (2025-05-25 15:00:00, weight 0.53) in time
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:55 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.16631 (min) 0.687711 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.195838 (min) 0.339494 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: 6.06732 (min) 7.4793 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0.473254 (min) 2.21677 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:55 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.923070, mean: 1.202212, max: 1.491594
09:36:55 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:55 DEBUG opendrift.models.physics_methods:917: min: 5.234159, mean: 5.967187, max: 6.653572
09:36:55 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.234159, mean: 5.967187, max: 6.653572
09:36:55 DEBUG opendrift:651: 72 elements hit land, moving them to the coastline.
09:36:55 DEBUG opendrift:1729: 72 elements scheduled for deactivation (stranded)
09:36:55 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:55 DEBUG opendrift:1749: Removed 72 elements.
09:36:55 DEBUG opendrift:1752: Removed 72 values from environment.
09:36:55 DEBUG opendrift:1757: remove items from profile for z
09:36:55 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:55 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:55 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:55 DEBUG opendrift:1761: Removed 72 values from environment_profiles.
09:36:55 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:55 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:55 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:55 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:55 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:55 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:55 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:55 DEBUG opendrift.models.physics_methods:917: min: 5.234159, mean: 5.973654, max: 6.653573
09:36:55 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:55 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.034610, dN_50: 0.002716
09:36:55 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:55 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.021881819096303268
09:36:55 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:55 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:55 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:55 DEBUG opendrift.models.physics_methods:771: Advecting 914 of 5854 elements above 0.100m with wind-sheared ocean current (0.038044 m/s - 0.226206 m/s)
09:36:55 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:55 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0023156931708418985 and 0.4189364287550683 m/s
09:36:55 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:55 DEBUG opendrift:2101: ======================================================================
09:36:55 INFO opendrift:2102: 2025-05-25 14:35:52.207946 - step 54 of 120 - 5854 active elements (146 deactivated)
09:36:55 DEBUG opendrift:2108: 0 elements scheduled.
09:36:55 DEBUG opendrift:2110: ======================================================================
09:36:55 DEBUG opendrift:2121: 35.10208438053952 <- latitude -> 35.928953444466735
09:36:55 DEBUG opendrift:2121: 23.12391294266054 <- longitude -> 23.563970147258548
09:36:55 DEBUG opendrift:2121: -104.8193043357689 <- z -> 0.0
09:36:55 DEBUG opendrift:2122: ---------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.13) and
after (2025-05-25 15:00:00, weight 0.87) in time
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5854 elements
09:36:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 12:00:00 (before)
2025-05-25 15:00:00 (after)
09:36:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 12:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 15:00:00) in space (linearNDFast)
09:36:55 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:55 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 12:00:00, weight 0.13) and
after (2025-05-25 15:00:00, weight 0.87) in time
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:55 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.251129 (min) 0.687925 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.241184 (min) 0.352297 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: 5.97137 (min) 7.24458 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: -0.749355 (min) 1.29562 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:55 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.889460, mean: 1.203314, max: 1.302027
09:36:55 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:55 DEBUG opendrift.models.physics_methods:917: min: 5.137984, mean: 5.972172, max: 6.216407
09:36:55 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.137984, mean: 5.972172, max: 6.216407
09:36:55 DEBUG opendrift:651: 93 elements hit land, moving them to the coastline.
09:36:55 DEBUG opendrift:1729: 93 elements scheduled for deactivation (stranded)
09:36:55 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:55 DEBUG opendrift:1749: Removed 93 elements.
09:36:55 DEBUG opendrift:1752: Removed 93 values from environment.
09:36:55 DEBUG opendrift:1757: remove items from profile for z
09:36:55 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:55 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:55 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:55 DEBUG opendrift:1761: Removed 93 values from environment_profiles.
09:36:55 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:55 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:55 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:55 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:55 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:55 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:55 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:55 DEBUG opendrift.models.physics_methods:917: min: 5.137984, mean: 5.979547, max: 6.216407
09:36:55 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:55 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.037010, dN_50: 0.002905
09:36:55 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:55 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.019101385298758523
09:36:55 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:55 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:55 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:55 DEBUG opendrift.models.physics_methods:771: Advecting 825 of 5761 elements above 0.100m with wind-sheared ocean current (0.016231 m/s - 0.215093 m/s)
09:36:55 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:55 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014951097912537536 and 0.5197341499778997 m/s
09:36:55 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:55 DEBUG opendrift:2101: ======================================================================
09:36:55 INFO opendrift:2102: 2025-05-25 15:35:52.207946 - step 55 of 120 - 5761 active elements (239 deactivated)
09:36:55 DEBUG opendrift:2108: 0 elements scheduled.
09:36:55 DEBUG opendrift:2110: ======================================================================
09:36:55 DEBUG opendrift:2121: 35.0969262502125 <- latitude -> 35.92892787819432
09:36:55 DEBUG opendrift:2121: 23.114939991264833 <- longitude -> 23.592283857660867
09:36:55 DEBUG opendrift:2121: -104.12826867723327 <- z -> 0.0
09:36:55 DEBUG opendrift:2122: ---------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:55 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:55 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5761 elements
09:36:55 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:55 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:36:57 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:57 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:36:57 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:36:57 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:36:57 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 15x29x22) for time after (2025-05-25 18:00:00)
09:36:57 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:57 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:57 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:57 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 549 elements, expanding data 1
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.80) and
after (2025-05-25 18:00:00, weight 0.20) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5761 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5761 elements
09:36:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:36:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:36:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:36:58 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:36:58 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:36:58 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-25 18:00:00)
09:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.80) and
after (2025-05-25 18:00:00, weight 0.20) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:58 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.317181 (min) 0.596809 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.278715 (min) 0.358103 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_wind: 5.81115 (min) 7.48212 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.25213 (min) 0.641013 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:58 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.830819, mean: 1.301029, max: 1.501389
09:36:58 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:58 DEBUG opendrift.models.physics_methods:917: min: 4.965727, mean: 6.211009, max: 6.675383
09:36:58 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.965727, mean: 6.211009, max: 6.675383
09:36:58 DEBUG opendrift:651: 104 elements hit land, moving them to the coastline.
09:36:58 DEBUG opendrift:1729: 104 elements scheduled for deactivation (stranded)
09:36:58 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:36:58 DEBUG opendrift:1749: Removed 104 elements.
09:36:58 DEBUG opendrift:1752: Removed 104 values from environment.
09:36:58 DEBUG opendrift:1757: remove items from profile for z
09:36:58 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:58 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:58 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:58 DEBUG opendrift:1761: Removed 104 values from environment_profiles.
09:36:58 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:58 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:58 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:58 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:58 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:58 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:58 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:58 DEBUG opendrift.models.physics_methods:917: min: 4.965727, mean: 6.215230, max: 6.675384
09:36:58 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:58 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.038643, dN_50: 0.003033
09:36:58 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:58 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.022025489661843915
09:36:58 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:58 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:58 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:58 DEBUG opendrift.models.physics_methods:771: Advecting 728 of 5657 elements above 0.100m with wind-sheared ocean current (0.108354 m/s - 0.214685 m/s)
09:36:58 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:58 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010015980594053826 and 0.4234651429247644 m/s
09:36:58 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:58 DEBUG opendrift:2101: ======================================================================
09:36:58 INFO opendrift:2102: 2025-05-25 16:35:52.207946 - step 56 of 120 - 5657 active elements (343 deactivated)
09:36:58 DEBUG opendrift:2108: 0 elements scheduled.
09:36:58 DEBUG opendrift:2110: ======================================================================
09:36:58 DEBUG opendrift:2121: 35.09808848644971 <- latitude -> 35.930975447106796
09:36:58 DEBUG opendrift:2121: 23.095065371120835 <- longitude -> 23.619609107297713
09:36:58 DEBUG opendrift:2121: -103.38065098351858 <- z -> 0.0
09:36:58 DEBUG opendrift:2122: ---------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:58 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.47) and
after (2025-05-25 18:00:00, weight 0.53) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5657 elements
09:36:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.47) and
after (2025-05-25 18:00:00, weight 0.53) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:58 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.332051 (min) 0.501819 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.317654 (min) 0.360603 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_wind: 6.21585 (min) 8.28507 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.93247 (min) 0.0504066 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:58 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.954643, mean: 1.498931, max: 1.856590
09:36:58 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:58 DEBUG opendrift.models.physics_methods:917: min: 5.322922, mean: 6.663785, max: 7.423139
09:36:58 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.322922, mean: 6.663785, max: 7.423139
09:36:58 DEBUG opendrift:651: 90 elements hit land, moving them to the coastline.
09:36:58 DEBUG opendrift:1729: 90 elements scheduled for deactivation (stranded)
09:36:58 DEBUG opendrift:1731: (z: -17.909875 to 0.000000)
09:36:58 DEBUG opendrift:1749: Removed 90 elements.
09:36:58 DEBUG opendrift:1752: Removed 90 values from environment.
09:36:58 DEBUG opendrift:1757: remove items from profile for z
09:36:58 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:58 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:58 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:58 DEBUG opendrift:1761: Removed 90 values from environment_profiles.
09:36:58 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:58 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:58 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:58 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:58 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:58 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:58 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:58 DEBUG opendrift.models.physics_methods:917: min: 5.322922, mean: 6.661368, max: 7.423139
09:36:58 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:58 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.039593, dN_50: 0.003107
09:36:58 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:58 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.027235315317808314
09:36:58 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:58 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:58 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:58 DEBUG opendrift.models.physics_methods:771: Advecting 647 of 5567 elements above 0.100m with wind-sheared ocean current (0.001323 m/s - 0.245250 m/s)
09:36:58 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:58 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0023240329698029517 and 0.44402604666056006 m/s
09:36:58 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:58 DEBUG opendrift:2101: ======================================================================
09:36:58 INFO opendrift:2102: 2025-05-25 17:35:52.207946 - step 57 of 120 - 5567 active elements (433 deactivated)
09:36:58 DEBUG opendrift:2108: 0 elements scheduled.
09:36:58 DEBUG opendrift:2110: ======================================================================
09:36:58 DEBUG opendrift:2121: 35.09531995020383 <- latitude -> 35.923635222611814
09:36:58 DEBUG opendrift:2121: 23.079944827559242 <- longitude -> 23.64255405936523
09:36:58 DEBUG opendrift:2121: -102.21850588382542 <- z -> 0.0
09:36:58 DEBUG opendrift:2122: ---------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:58 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.13) and
after (2025-05-25 18:00:00, weight 0.87) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:36:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:36:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5567 elements
09:36:58 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 15:00:00 (before)
2025-05-25 18:00:00 (after)
09:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 15:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 18:00:00) in space (linearNDFast)
09:36:58 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:36:58 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 15:00:00, weight 0.13) and
after (2025-05-25 18:00:00, weight 0.87) in time
09:36:58 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:58 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:36:58 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:36:58 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:36:58 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.359984 (min) 0.478193 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.407861 (min) 0.358901 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: x_wind: 6.76225 (min) 9.58517 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.63807 (min) -0.360285 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:36:58 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:36:58 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.140131, mean: 1.760450, max: 2.512728
09:36:58 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:36:58 DEBUG opendrift.models.physics_methods:917: min: 5.817106, mean: 7.213406, max: 8.635796
09:36:58 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.817106, mean: 7.213406, max: 8.635796
09:36:58 DEBUG opendrift:651: 60 elements hit land, moving them to the coastline.
09:36:58 DEBUG opendrift:1729: 60 elements scheduled for deactivation (stranded)
09:36:58 DEBUG opendrift:1731: (z: -13.976623 to 0.000000)
09:36:59 DEBUG opendrift:1749: Removed 60 elements.
09:36:59 DEBUG opendrift:1752: Removed 60 values from environment.
09:36:59 DEBUG opendrift:1757: remove items from profile for z
09:36:59 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:36:59 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:36:59 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:36:59 DEBUG opendrift:1761: Removed 60 values from environment_profiles.
09:36:59 DEBUG opendrift:2157: Calling OpenOil.update()
09:36:59 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:36:59 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:36:59 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:36:59 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:36:59 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:36:59 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:36:59 DEBUG opendrift.models.physics_methods:917: min: 5.817106, mean: 7.208502, max: 8.635797
09:36:59 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:36:59 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.040315, dN_50: 0.003164
09:36:59 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:36:59 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.036859087295587165
09:36:59 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:36:59 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:36:59 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:36:59 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 586 surface elements
09:36:59 DEBUG opendrift.models.physics_methods:771: Advecting 593 of 5507 elements above 0.100m with wind-sheared ocean current (0.092382 m/s - 0.283154 m/s)
09:36:59 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:36:59 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016274266253301847 and 0.4706752778982453 m/s
09:36:59 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:36:59 DEBUG opendrift:2101: ======================================================================
09:36:59 INFO opendrift:2102: 2025-05-25 18:35:52.207946 - step 58 of 120 - 5507 active elements (493 deactivated)
09:36:59 DEBUG opendrift:2108: 0 elements scheduled.
09:36:59 DEBUG opendrift:2110: ======================================================================
09:36:59 DEBUG opendrift:2121: 35.096132116472205 <- latitude -> 35.91471191952025
09:36:59 DEBUG opendrift:2121: 23.069250237866157 <- longitude -> 23.666809130888645
09:36:59 DEBUG opendrift:2121: -101.42563273025681 <- z -> 0.0
09:36:59 DEBUG opendrift:2122: ---------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:36:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:36:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:59 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:36:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:36:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:36:59 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:36:59 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:36:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:36:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:36:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:36:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:36:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5507 elements
09:36:59 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:36:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:36:59 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:36:59 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:00 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:00 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:00 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:00 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 16x28x22) for time after (2025-05-25 21:00:00)
09:37:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:00 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 143 elements, expanding data 1
09:37:00 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.80) and
after (2025-05-25 21:00:00, weight 0.20) in time
09:37:00 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:00 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:00 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:00 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:00 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:00 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:00 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:00 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5507 elements
09:37:00 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5507 elements
09:37:00 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:37:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:00 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:00 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:00 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:00 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-25 21:00:00)
09:37:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:00 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:00 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:00 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.80) and
after (2025-05-25 21:00:00, weight 0.20) in time
09:37:00 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:00 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:00 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:00 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:00 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:00 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:00 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:00 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:00 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:00 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.346655 (min) 0.363023 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.452959 (min) 0.361956 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: x_wind: 6.96541 (min) 10.4284 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.39581 (min) -0.648528 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:00 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:00 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.280326, mean: 1.972488, max: 3.074312
09:37:00 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:00 DEBUG opendrift.models.physics_methods:917: min: 6.164388, mean: 7.633275, max: 9.552203
09:37:00 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.164388, mean: 7.633275, max: 9.552203
09:37:00 DEBUG opendrift:651: 35 elements hit land, moving them to the coastline.
09:37:00 DEBUG opendrift:1729: 35 elements scheduled for deactivation (stranded)
09:37:00 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:00 DEBUG opendrift:1749: Removed 35 elements.
09:37:00 DEBUG opendrift:1752: Removed 35 values from environment.
09:37:00 DEBUG opendrift:1757: remove items from profile for z
09:37:00 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:00 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:00 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:00 DEBUG opendrift:1761: Removed 35 values from environment_profiles.
09:37:00 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:00 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:00 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:00 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:00 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:00 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:00 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:00 DEBUG opendrift.models.physics_methods:917: min: 6.164388, mean: 7.628699, max: 9.552203
09:37:00 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:00 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.041073, dN_50: 0.003223
09:37:00 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:00 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.04509598113180072
09:37:00 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:00 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:00 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:00 DEBUG opendrift.models.physics_methods:771: Advecting 561 of 5472 elements above 0.100m with wind-sheared ocean current (0.002826 m/s - 0.307173 m/s)
09:37:00 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:00 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0026905489751987053 and 0.4611572176524359 m/s
09:37:01 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:01 DEBUG opendrift:2101: ======================================================================
09:37:01 INFO opendrift:2102: 2025-05-25 19:35:52.207946 - step 59 of 120 - 5472 active elements (528 deactivated)
09:37:01 DEBUG opendrift:2108: 0 elements scheduled.
09:37:01 DEBUG opendrift:2110: ======================================================================
09:37:01 DEBUG opendrift:2121: 35.09283203571372 <- latitude -> 35.89870393168019
09:37:01 DEBUG opendrift:2121: 23.05915481181977 <- longitude -> 23.68048738954989
09:37:01 DEBUG opendrift:2121: -100.40886656680998 <- z -> 0.0
09:37:01 DEBUG opendrift:2122: ---------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:37:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.47) and
after (2025-05-25 21:00:00, weight 0.53) in time
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5472 elements
09:37:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:37:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.47) and
after (2025-05-25 21:00:00, weight 0.53) in time
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:01 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.290814 (min) 0.256806 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.461991 (min) 0.364749 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: 7.26128 (min) 10.8584 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.59918 (min) -0.491026 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:01 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.348248, mean: 2.128144, max: 3.377990
09:37:01 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:01 DEBUG opendrift.models.physics_methods:917: min: 6.325786, mean: 7.931614, max: 10.012874
09:37:01 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.325786, mean: 7.931614, max: 10.012874
09:37:01 DEBUG opendrift:651: 19 elements hit land, moving them to the coastline.
09:37:01 DEBUG opendrift:1729: 19 elements scheduled for deactivation (stranded)
09:37:01 DEBUG opendrift:1731: (z: -7.148153 to 0.000000)
09:37:01 DEBUG opendrift:1749: Removed 19 elements.
09:37:01 DEBUG opendrift:1752: Removed 19 values from environment.
09:37:01 DEBUG opendrift:1757: remove items from profile for z
09:37:01 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:01 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:01 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:01 DEBUG opendrift:1761: Removed 19 values from environment_profiles.
09:37:01 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:01 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:01 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:01 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:01 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:01 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:01 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:01 DEBUG opendrift.models.physics_methods:917: min: 6.325786, mean: 7.928531, max: 10.012874
09:37:01 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:01 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.041916, dN_50: 0.003290
09:37:01 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:01 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.049550119462232575
09:37:01 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:01 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:01 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:01 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 542 surface elements
09:37:01 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 543 surface elements
09:37:01 DEBUG opendrift.models.physics_methods:771: Advecting 553 of 5453 elements above 0.100m with wind-sheared ocean current (0.003627 m/s - 0.320331 m/s)
09:37:01 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:01 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.003177972502095813 and 0.44016259088398607 m/s
09:37:01 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:01 DEBUG opendrift:2101: ======================================================================
09:37:01 INFO opendrift:2102: 2025-05-25 20:35:52.207946 - step 60 of 120 - 5453 active elements (547 deactivated)
09:37:01 DEBUG opendrift:2108: 0 elements scheduled.
09:37:01 DEBUG opendrift:2110: ======================================================================
09:37:01 DEBUG opendrift:2121: 35.09942416786722 <- latitude -> 35.895425454459506
09:37:01 DEBUG opendrift:2121: 23.050299621315254 <- longitude -> 23.699228423458038
09:37:01 DEBUG opendrift:2121: -99.31833672307782 <- z -> 0.0
09:37:01 DEBUG opendrift:2122: ---------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:37:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.13) and
after (2025-05-25 21:00:00, weight 0.87) in time
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5453 elements
09:37:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 18:00:00 (before)
2025-05-25 21:00:00 (after)
09:37:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 18:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-25 21:00:00) in space (linearNDFast)
09:37:01 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:01 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 18:00:00, weight 0.13) and
after (2025-05-25 21:00:00, weight 0.87) in time
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:01 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.308438 (min) 0.175855 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.47779 (min) 0.372497 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: 7.42938 (min) 11.3089 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.73277 (min) -0.21401 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:01 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.412819, mean: 2.295090, max: 3.681510
09:37:01 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:01 DEBUG opendrift.models.physics_methods:917: min: 6.475492, mean: 8.238940, max: 10.453039
09:37:01 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.475492, mean: 8.238940, max: 10.453039
09:37:01 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:01 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:01 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:01 DEBUG opendrift:1749: Removed 5 elements.
09:37:01 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:01 DEBUG opendrift:1757: remove items from profile for z
09:37:01 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:01 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:01 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:01 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:01 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:01 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:01 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:01 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:01 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:01 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:01 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:01 DEBUG opendrift.models.physics_methods:917: min: 6.475492, mean: 8.238520, max: 10.453038
09:37:01 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:01 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.042710, dN_50: 0.003352
09:37:01 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:01 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.054001927361287574
09:37:01 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:01 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:01 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:01 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 544 surface elements
09:37:01 DEBUG opendrift.models.openoil.openoil:1036: Entraining 2 of 547 surface elements
09:37:01 DEBUG opendrift.models.physics_methods:771: Advecting 555 of 5448 elements above 0.100m with wind-sheared ocean current (0.014704 m/s - 0.332377 m/s)
09:37:01 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:01 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0016424862134437066 and 0.469532679141593 m/s
09:37:01 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:01 DEBUG opendrift:2101: ======================================================================
09:37:01 INFO opendrift:2102: 2025-05-25 21:35:52.207946 - step 61 of 120 - 5448 active elements (552 deactivated)
09:37:01 DEBUG opendrift:2108: 0 elements scheduled.
09:37:01 DEBUG opendrift:2110: ======================================================================
09:37:01 DEBUG opendrift:2121: 35.098853305246394 <- latitude -> 35.90394590796968
09:37:01 DEBUG opendrift:2121: 23.04005612490871 <- longitude -> 23.712274412626453
09:37:01 DEBUG opendrift:2121: -98.47818594410553 <- z -> 0.0
09:37:01 DEBUG opendrift:2122: ---------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:01 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:01 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5448 elements
09:37:01 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:01 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:01 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:02 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:02 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:02 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:02 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 17x28x21) for time after (2025-05-26 00:00:00)
09:37:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 51 elements, expanding data 1
09:37:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.80) and
after (2025-05-26 00:00:00, weight 0.20) in time
09:37:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:02 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:02 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:02 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:02 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:02 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:02 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5448 elements
09:37:02 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5448 elements
09:37:02 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:02 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:02 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:02 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:02 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-26 00:00:00)
09:37:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:02 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:02 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:02 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.80) and
after (2025-05-26 00:00:00, weight 0.20) in time
09:37:02 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:02 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:02 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:02 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:02 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:02 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:02 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:02 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.302987 (min) 0.233596 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.469525 (min) 0.372315 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: x_wind: 7.67631 (min) 11.4666 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.7728 (min) -0.46472 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:02 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:02 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.483965, mean: 2.466370, max: 3.927590
09:37:02 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:02 DEBUG opendrift.models.physics_methods:917: min: 6.636534, mean: 8.543516, max: 10.796740
09:37:02 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.636534, mean: 8.543516, max: 10.796740
09:37:02 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:02 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:02 DEBUG opendrift:1731: (z: -17.200928 to 0.000000)
09:37:02 DEBUG opendrift:1749: Removed 5 elements.
09:37:02 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:02 DEBUG opendrift:1757: remove items from profile for z
09:37:02 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:02 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:02 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:02 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:02 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:02 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:02 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:02 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:02 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:02 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:02 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:02 DEBUG opendrift.models.physics_methods:917: min: 6.636534, mean: 8.542924, max: 10.796740
09:37:02 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:02 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.043584, dN_50: 0.003420
09:37:02 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:02 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.05761124729865561
09:37:02 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:02 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:02 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:02 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 548 surface elements
09:37:03 DEBUG opendrift.models.physics_methods:771: Advecting 557 of 5443 elements above 0.100m with wind-sheared ocean current (0.021060 m/s - 0.339439 m/s)
09:37:03 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:03 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0025235234662183002 and 0.429015506107453 m/s
09:37:03 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:03 DEBUG opendrift:2101: ======================================================================
09:37:03 INFO opendrift:2102: 2025-05-25 22:35:52.207946 - step 62 of 120 - 5443 active elements (557 deactivated)
09:37:03 DEBUG opendrift:2108: 0 elements scheduled.
09:37:03 DEBUG opendrift:2110: ======================================================================
09:37:03 DEBUG opendrift:2121: 35.10340755272164 <- latitude -> 35.900634205999985
09:37:03 DEBUG opendrift:2121: 23.034631041590167 <- longitude -> 23.73145030319727
09:37:03 DEBUG opendrift:2121: -97.02682869889648 <- z -> 0.0
09:37:03 DEBUG opendrift:2122: ---------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.47) and
after (2025-05-26 00:00:00, weight 0.53) in time
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5443 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.47) and
after (2025-05-26 00:00:00, weight 0.53) in time
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:03 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.259482 (min) 0.315397 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.42696 (min) 0.36215 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: 7.89743 (min) 11.4478 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.73192 (min) -1.05141 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:03 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.564750, mean: 2.648619, max: 4.112360
09:37:03 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:03 DEBUG opendrift.models.physics_methods:917: min: 6.814783, mean: 8.856068, max: 11.047784
09:37:03 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.814783, mean: 8.856068, max: 11.047784
09:37:03 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:03 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:03 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:03 DEBUG opendrift:1749: Removed 3 elements.
09:37:03 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:03 DEBUG opendrift:1757: remove items from profile for z
09:37:03 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:03 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:03 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:03 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:03 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:03 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:03 DEBUG opendrift.models.physics_methods:917: min: 6.960552, mean: 8.856602, max: 11.047785
09:37:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.044488, dN_50: 0.003491
09:37:03 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:03 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.06032133450427553
09:37:03 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:03 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:03 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:03 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 546 surface elements
09:37:03 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 549 surface elements
09:37:03 DEBUG opendrift.models.physics_methods:771: Advecting 561 of 5440 elements above 0.100m with wind-sheared ocean current (0.024207 m/s - 0.344926 m/s)
09:37:03 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:03 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007353379018262754 and 0.45069557750157885 m/s
09:37:03 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:03 DEBUG opendrift:2101: ======================================================================
09:37:03 INFO opendrift:2102: 2025-05-25 23:35:52.207946 - step 63 of 120 - 5440 active elements (560 deactivated)
09:37:03 DEBUG opendrift:2108: 0 elements scheduled.
09:37:03 DEBUG opendrift:2110: ======================================================================
09:37:03 DEBUG opendrift:2121: 35.10416399311379 <- latitude -> 35.89473398038759
09:37:03 DEBUG opendrift:2121: 23.02612056831979 <- longitude -> 23.751157551127996
09:37:03 DEBUG opendrift:2121: -95.87718356146758 <- z -> 0.0
09:37:03 DEBUG opendrift:2122: ---------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.13) and
after (2025-05-26 00:00:00, weight 0.87) in time
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5440 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-25 21:00:00 (before)
2025-05-26 00:00:00 (after)
09:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-25 21:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 00:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-25 21:00:00, weight 0.13) and
after (2025-05-26 00:00:00, weight 0.87) in time
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:03 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:03 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:03 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.22903 (min) 0.380898 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.389466 (min) 0.38933 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: x_wind: 8.18288 (min) 11.416 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.7412 (min) -1.63036 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:03 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:03 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.763643, mean: 2.848012, max: 4.323895
09:37:03 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:03 DEBUG opendrift.models.physics_methods:917: min: 7.234940, mean: 9.184949, max: 11.328361
09:37:03 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 7.234940, mean: 9.184949, max: 11.328361
09:37:03 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:03 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:03 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:03 DEBUG opendrift:1749: Removed 3 elements.
09:37:03 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:03 DEBUG opendrift:1757: remove items from profile for z
09:37:03 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:03 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:03 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:03 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:03 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:03 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:03 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:03 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:03 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:03 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:03 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:03 DEBUG opendrift.models.physics_methods:917: min: 7.234940, mean: 9.185768, max: 11.328362
09:37:03 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:03 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.045385, dN_50: 0.003562
09:37:03 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:03 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0634239601351106
09:37:03 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:03 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:03 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:03 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 554 surface elements
09:37:03 DEBUG opendrift.models.physics_methods:771: Advecting 564 of 5437 elements above 0.100m with wind-sheared ocean current (0.060485 m/s - 0.355677 m/s)
09:37:03 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:03 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0014264654094926972 and 0.4453373203608711 m/s
09:37:03 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:03 DEBUG opendrift:2101: ======================================================================
09:37:03 INFO opendrift:2102: 2025-05-26 00:35:52.207946 - step 64 of 120 - 5437 active elements (563 deactivated)
09:37:03 DEBUG opendrift:2108: 0 elements scheduled.
09:37:03 DEBUG opendrift:2110: ======================================================================
09:37:03 DEBUG opendrift:2121: 35.104978828863 <- latitude -> 35.89242110676342
09:37:03 DEBUG opendrift:2121: 23.024652784305808 <- longitude -> 23.778835503796653
09:37:03 DEBUG opendrift:2121: -94.63770926562447 <- z -> 0.0
09:37:03 DEBUG opendrift:2122: ---------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:03 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:03 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:03 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:03 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:03 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 19x28x21) for time after (2025-05-26 03:00:00)
09:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:03 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 65 elements, expanding data 1
09:37:03 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.80) and
after (2025-05-26 03:00:00, weight 0.20) in time
09:37:03 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:03 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:03 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:03 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:03 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:03 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5437 elements
09:37:03 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:03 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:04 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-26 03:00:00)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.80) and
after (2025-05-26 03:00:00, weight 0.20) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:04 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.253084 (min) 0.404927 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.354964 (min) 0.39067 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: 7.1941 (min) 9.93792 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.06177 (min) -2.29435 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:04 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 1.402671, mean: 2.756059, max: 3.540524
09:37:04 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 6.452196, mean: 9.028823, max: 10.250932
09:37:04 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 6.452196, mean: 9.028823, max: 10.250932
09:37:04 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:04 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:04 DEBUG opendrift:1731: (z: -12.699781 to 0.000000)
09:37:04 DEBUG opendrift:1749: Removed 3 elements.
09:37:04 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:04 DEBUG opendrift:1757: remove items from profile for z
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:04 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:04 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:04 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:04 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 6.452196, mean: 9.029600, max: 10.250933
09:37:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.048743, dN_50: 0.003825
09:37:04 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:04 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.05193405204353707
09:37:04 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:04 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:04 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:04 DEBUG opendrift.models.openoil.openoil:1036: Entraining 1 of 555 surface elements
09:37:04 DEBUG opendrift.models.physics_methods:771: Advecting 563 of 5434 elements above 0.100m with wind-sheared ocean current (0.062582 m/s - 0.336679 m/s)
09:37:04 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:04 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0010231411508476174 and 0.4519422994638825 m/s
09:37:04 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:04 DEBUG opendrift:2101: ======================================================================
09:37:04 INFO opendrift:2102: 2025-05-26 01:35:52.207946 - step 65 of 120 - 5434 active elements (566 deactivated)
09:37:04 DEBUG opendrift:2108: 0 elements scheduled.
09:37:04 DEBUG opendrift:2110: ======================================================================
09:37:04 DEBUG opendrift:2121: 35.09842613107247 <- latitude -> 35.890201576294196
09:37:04 DEBUG opendrift:2121: 23.023592705492003 <- longitude -> 23.799662819352772
09:37:04 DEBUG opendrift:2121: -93.7214015368206 <- z -> 0.0
09:37:04 DEBUG opendrift:2122: ---------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.47) and
after (2025-05-26 03:00:00, weight 0.53) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5434 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.47) and
after (2025-05-26 03:00:00, weight 0.53) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:04 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.190239 (min) 0.415481 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.290376 (min) 0.375847 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: 4.73721 (min) 9.12216 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.68536 (min) -3.14261 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:04 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.915734, mean: 2.525606, max: 3.486404
09:37:04 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 5.213320, mean: 8.607407, max: 10.172284
09:37:04 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 5.213320, mean: 8.607407, max: 10.172284
09:37:04 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:04 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:04 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:04 DEBUG opendrift:1749: Removed 4 elements.
09:37:04 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:04 DEBUG opendrift:1757: remove items from profile for z
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:04 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:04 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:04 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:04 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 5.213320, mean: 8.609280, max: 10.172283
09:37:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.054130, dN_50: 0.004248
09:37:04 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:04 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.05114025634772861
09:37:04 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:04 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:04 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:04 DEBUG opendrift.models.physics_methods:771: Advecting 570 of 5430 elements above 0.100m with wind-sheared ocean current (0.016306 m/s - 0.306643 m/s)
09:37:04 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:04 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.003053810780012524 and 0.42893573648957173 m/s
09:37:04 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:04 DEBUG opendrift:2101: ======================================================================
09:37:04 INFO opendrift:2102: 2025-05-26 02:35:52.207946 - step 66 of 120 - 5430 active elements (570 deactivated)
09:37:04 DEBUG opendrift:2108: 0 elements scheduled.
09:37:04 DEBUG opendrift:2110: ======================================================================
09:37:04 DEBUG opendrift:2121: 35.092771301340974 <- latitude -> 35.88522631308056
09:37:04 DEBUG opendrift:2121: 23.026469051882266 <- longitude -> 23.82783829368973
09:37:04 DEBUG opendrift:2121: -92.8619936684491 <- z -> 0.0
09:37:04 DEBUG opendrift:2122: ---------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.13) and
after (2025-05-26 03:00:00, weight 0.87) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5430 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 00:00:00 (before)
2025-05-26 03:00:00 (after)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 00:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 00:00:00, weight 0.13) and
after (2025-05-26 03:00:00, weight 0.87) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:04 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.213112 (min) 0.434447 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.24238 (min) 0.36176 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: 1.81043 (min) 9.06234 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.29836 (min) -3.90464 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:04 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.650587, mean: 2.382026, max: 3.714323
09:37:04 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 4.394227, mean: 8.310635, max: 10.499520
09:37:04 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.394227, mean: 8.310635, max: 10.499520
09:37:04 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:04 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:04 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:04 DEBUG opendrift:1749: Removed 4 elements.
09:37:04 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:04 DEBUG opendrift:1757: remove items from profile for z
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:04 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:04 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:04 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:04 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:04 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:04 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:04 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:04 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:04 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:04 DEBUG opendrift.models.physics_methods:917: min: 4.394227, mean: 8.312402, max: 10.499519
09:37:04 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:04 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.059655, dN_50: 0.004682
09:37:04 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:04 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.054483205510792214
09:37:04 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:04 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:04 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:04 DEBUG opendrift.models.physics_methods:771: Advecting 571 of 5426 elements above 0.100m with wind-sheared ocean current (0.017162 m/s - 0.297674 m/s)
09:37:04 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:04 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0007531899172985478 and 0.4196183043802186 m/s
09:37:04 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:04 DEBUG opendrift:2101: ======================================================================
09:37:04 INFO opendrift:2102: 2025-05-26 03:35:52.207946 - step 67 of 120 - 5426 active elements (574 deactivated)
09:37:04 DEBUG opendrift:2108: 0 elements scheduled.
09:37:04 DEBUG opendrift:2110: ======================================================================
09:37:04 DEBUG opendrift:2121: 35.0926534908177 <- latitude -> 35.88059452528255
09:37:04 DEBUG opendrift:2121: 23.0288129594728 <- longitude -> 23.83870635777055
09:37:04 DEBUG opendrift:2121: -91.7449495101002 <- z -> 0.0
09:37:04 DEBUG opendrift:2122: ---------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:04 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:04 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:04 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 19x27x21) for time after (2025-05-26 06:00:00)
09:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:04 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 82 elements, expanding data 1
09:37:04 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.80) and
after (2025-05-26 06:00:00, weight 0.20) in time
09:37:04 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5426 elements
09:37:04 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:04 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:05 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:05 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:05 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-26 06:00:00)
09:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.80) and
after (2025-05-26 06:00:00, weight 0.20) in time
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:05 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.20274 (min) 0.46023 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.2507 (min) 0.331869 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.077361 (min) 8.31648 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.51202 (min) -4.41419 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:05 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.569521, mean: 1.871274, max: 3.483808
09:37:05 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 4.111351, mean: 7.354073, max: 10.168495
09:37:05 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 4.111351, mean: 7.354073, max: 10.168495
09:37:05 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:05 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:05 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:05 DEBUG opendrift:1749: Removed 3 elements.
09:37:05 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:05 DEBUG opendrift:1757: remove items from profile for z
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:05 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:05 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:05 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:05 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:05 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:05 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:05 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 4.111351, mean: 7.354928, max: 10.168495
09:37:05 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:05 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.068013, dN_50: 0.005338
09:37:05 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:05 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.051102179707985806
09:37:05 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:05 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:05 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:05 DEBUG opendrift.models.physics_methods:771: Advecting 570 of 5423 elements above 0.100m with wind-sheared ocean current (0.001323 m/s - 0.261396 m/s)
09:37:05 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:05 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002317864615410018 and 0.45676005822942606 m/s
09:37:05 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:05 DEBUG opendrift:2101: ======================================================================
09:37:05 INFO opendrift:2102: 2025-05-26 04:35:52.207946 - step 68 of 120 - 5423 active elements (577 deactivated)
09:37:05 DEBUG opendrift:2108: 0 elements scheduled.
09:37:05 DEBUG opendrift:2110: ======================================================================
09:37:05 DEBUG opendrift:2121: 35.09019860566106 <- latitude -> 35.87630911632708
09:37:05 DEBUG opendrift:2121: 23.030374390476698 <- longitude -> 23.848037701088757
09:37:05 DEBUG opendrift:2121: -90.69049279979897 <- z -> 0.0
09:37:05 DEBUG opendrift:2122: ---------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.47) and
after (2025-05-26 06:00:00, weight 0.53) in time
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5423 elements
09:37:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.47) and
after (2025-05-26 06:00:00, weight 0.53) in time
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:05 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.184963 (min) 0.471402 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.260869 (min) 0.303139 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.3743 (min) 7.172 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.47763 (min) -4.03948 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:05 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.404629, mean: 1.320430, max: 3.033371
09:37:05 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 3.465436, mean: 6.194426, max: 9.488386
09:37:05 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.465436, mean: 6.194426, max: 9.488386
09:37:05 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:05 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:05 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:05 DEBUG opendrift:1749: Removed 4 elements.
09:37:05 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:05 DEBUG opendrift:1757: remove items from profile for z
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:05 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:05 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:05 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:05 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:05 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:05 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:05 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 3.465436, mean: 6.195259, max: 9.488386
09:37:05 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:05 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.077648, dN_50: 0.006094
09:37:05 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:05 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.044495498420597586
09:37:05 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:05 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:05 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:05 DEBUG opendrift.models.physics_methods:771: Advecting 571 of 5419 elements above 0.100m with wind-sheared ocean current (0.017839 m/s - 0.214019 m/s)
09:37:05 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:05 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0024900824531007533 and 0.44300805314806124 m/s
09:37:05 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:05 DEBUG opendrift:2101: ======================================================================
09:37:05 INFO opendrift:2102: 2025-05-26 05:35:52.207946 - step 69 of 120 - 5419 active elements (581 deactivated)
09:37:05 DEBUG opendrift:2108: 0 elements scheduled.
09:37:05 DEBUG opendrift:2110: ======================================================================
09:37:05 DEBUG opendrift:2121: 35.084269210218764 <- latitude -> 35.88343323250308
09:37:05 DEBUG opendrift:2121: 23.024534942237548 <- longitude -> 23.85937571359508
09:37:05 DEBUG opendrift:2121: -89.97767577682203 <- z -> 0.0
09:37:05 DEBUG opendrift:2122: ---------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.13) and
after (2025-05-26 06:00:00, weight 0.87) in time
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5419 elements
09:37:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 03:00:00 (before)
2025-05-26 06:00:00 (after)
09:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 03:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 06:00:00) in space (linearNDFast)
09:37:05 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:05 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 03:00:00, weight 0.13) and
after (2025-05-26 06:00:00, weight 0.87) in time
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:05 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:05 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.206831 (min) 0.477224 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.292388 (min) 0.299815 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.81792 (min) 6.06762 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.46346 (min) -3.11561 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:05 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:05 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.267667, mean: 1.085301, max: 2.667777
09:37:05 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 2.818556, mean: 5.641311, max: 8.898244
09:37:05 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.818556, mean: 5.641311, max: 8.898244
09:37:05 DEBUG opendrift:651: 1 elements hit land, moving them to the coastline.
09:37:05 DEBUG opendrift:1729: 1 elements scheduled for deactivation (stranded)
09:37:05 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:05 DEBUG opendrift:1749: Removed 1 elements.
09:37:05 DEBUG opendrift:1752: Removed 1 values from environment.
09:37:05 DEBUG opendrift:1757: remove items from profile for z
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:05 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:05 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:05 DEBUG opendrift:1761: Removed 1 values from environment_profiles.
09:37:05 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:05 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:05 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:05 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:05 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:05 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:05 DEBUG opendrift.models.physics_methods:917: min: 2.818556, mean: 5.641395, max: 8.898244
09:37:05 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:05 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.082118, dN_50: 0.006445
09:37:05 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:05 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03913321812984076
09:37:05 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:05 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:05 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:05 DEBUG opendrift.models.physics_methods:771: Advecting 572 of 5418 elements above 0.100m with wind-sheared ocean current (0.114007 m/s - 0.218877 m/s)
09:37:05 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:05 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0003901387409806751 and 0.45492316701378227 m/s
09:37:05 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:05 DEBUG opendrift:2101: ======================================================================
09:37:05 INFO opendrift:2102: 2025-05-26 06:35:52.207946 - step 70 of 120 - 5418 active elements (582 deactivated)
09:37:05 DEBUG opendrift:2108: 0 elements scheduled.
09:37:05 DEBUG opendrift:2110: ======================================================================
09:37:05 DEBUG opendrift:2121: 35.07941386521847 <- latitude -> 35.88745365240191
09:37:05 DEBUG opendrift:2121: 23.016941858354368 <- longitude -> 23.8742374289493
09:37:05 DEBUG opendrift:2121: -88.75179423135262 <- z -> 0.0
09:37:05 DEBUG opendrift:2122: ---------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:05 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:05 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:05 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:05 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:05 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:05 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:05 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5418 elements
09:37:05 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:05 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:06 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:06 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:06 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:06 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 20x29x20) for time after (2025-05-26 09:00:00)
09:37:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:06 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 110 elements, expanding data 1
09:37:06 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.80) and
after (2025-05-26 09:00:00, weight 0.20) in time
09:37:06 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5418 elements
09:37:06 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5418 elements
09:37:06 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:06 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:06 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:06 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:06 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 8x7x1) for time after (2025-05-26 09:00:00)
09:37:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:06 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:06 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:06 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.80) and
after (2025-05-26 09:00:00, weight 0.20) in time
09:37:06 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:06 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:06 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:06 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:06 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:06 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:06 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.22845 (min) 0.443141 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.307822 (min) 0.298016 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.55551 (min) 5.46945 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.44975 (min) -2.36196 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:06 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:06 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.197576, mean: 0.997336, max: 2.492301
09:37:06 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:06 DEBUG opendrift.models.physics_methods:917: min: 2.421570, mean: 5.411535, max: 8.600621
09:37:06 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.421570, mean: 5.411535, max: 8.600621
09:37:06 DEBUG opendrift:651: 1 elements hit land, moving them to the coastline.
09:37:06 DEBUG opendrift:1729: 1 elements scheduled for deactivation (stranded)
09:37:06 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:06 DEBUG opendrift:1749: Removed 1 elements.
09:37:06 DEBUG opendrift:1752: Removed 1 values from environment.
09:37:06 DEBUG opendrift:1757: remove items from profile for z
09:37:06 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:06 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:06 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:06 DEBUG opendrift:1761: Removed 1 values from environment_profiles.
09:37:06 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:06 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:06 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:06 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:06 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:06 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:06 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:06 DEBUG opendrift.models.physics_methods:917: min: 2.421570, mean: 5.411511, max: 8.600621
09:37:07 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:07 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.086674, dN_50: 0.006802
09:37:07 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:07 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03655946644818881
09:37:07 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:07 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:07 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:07 DEBUG opendrift.models.physics_methods:771: Advecting 583 of 5417 elements above 0.100m with wind-sheared ocean current (0.083475 m/s - 0.210768 m/s)
09:37:07 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:07 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0006251229321380102 and 0.42629605028143425 m/s
09:37:07 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:07 DEBUG opendrift:2101: ======================================================================
09:37:07 INFO opendrift:2102: 2025-05-26 07:35:52.207946 - step 71 of 120 - 5417 active elements (583 deactivated)
09:37:07 DEBUG opendrift:2108: 0 elements scheduled.
09:37:07 DEBUG opendrift:2110: ======================================================================
09:37:07 DEBUG opendrift:2121: 35.07396116843355 <- latitude -> 35.893094987757465
09:37:07 DEBUG opendrift:2121: 23.007806193725358 <- longitude -> 23.87833580825886
09:37:07 DEBUG opendrift:2121: -87.70631877733068 <- z -> 0.0
09:37:07 DEBUG opendrift:2122: ---------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.47) and
after (2025-05-26 09:00:00, weight 0.53) in time
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5417 elements
09:37:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.47) and
after (2025-05-26 09:00:00, weight 0.53) in time
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:07 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.246495 (min) 0.376589 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.337016 (min) 0.301378 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.43346 (min) 5.22369 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.45092 (min) -1.79209 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:07 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.126281, mean: 0.868262, max: 2.428145
09:37:07 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:07 DEBUG opendrift.models.physics_methods:917: min: 1.935970, mean: 5.038818, max: 8.489202
09:37:07 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.935970, mean: 5.038818, max: 8.489202
09:37:07 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:07 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:07 DEBUG opendrift:1731: (z: -10.757754 to 0.000000)
09:37:07 DEBUG opendrift:1749: Removed 3 elements.
09:37:07 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:07 DEBUG opendrift:1757: remove items from profile for z
09:37:07 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:07 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:07 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:07 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:07 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:07 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:07 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:07 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:07 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:07 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:07 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:07 DEBUG opendrift.models.physics_methods:917: min: 1.935970, mean: 5.039064, max: 8.489202
09:37:07 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:07 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.095741, dN_50: 0.007514
09:37:07 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:07 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.035618472809241304
09:37:07 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:07 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:07 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:07 DEBUG opendrift.models.physics_methods:771: Advecting 580 of 5414 elements above 0.100m with wind-sheared ocean current (0.097382 m/s - 0.200673 m/s)
09:37:07 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:07 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0011575202497575536 and 0.42381405987049126 m/s
09:37:07 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:07 DEBUG opendrift:2101: ======================================================================
09:37:07 INFO opendrift:2102: 2025-05-26 08:35:52.207946 - step 72 of 120 - 5414 active elements (586 deactivated)
09:37:07 DEBUG opendrift:2108: 0 elements scheduled.
09:37:07 DEBUG opendrift:2110: ======================================================================
09:37:07 DEBUG opendrift:2121: 35.07299350686126 <- latitude -> 35.89707161907188
09:37:07 DEBUG opendrift:2121: 23.004549200113114 <- longitude -> 23.877127722355134
09:37:07 DEBUG opendrift:2121: -86.8263938759837 <- z -> 0.0
09:37:07 DEBUG opendrift:2122: ---------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.13) and
after (2025-05-26 09:00:00, weight 0.87) in time
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5414 elements
09:37:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 06:00:00 (before)
2025-05-26 09:00:00 (after)
09:37:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 06:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 09:00:00) in space (linearNDFast)
09:37:07 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:07 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 06:00:00, weight 0.13) and
after (2025-05-26 09:00:00, weight 0.87) in time
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:07 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.294822 (min) 0.31657 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.359888 (min) 0.305721 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: -3.28405 (min) 4.87562 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: -8.42349 (min) -1.29368 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:07 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.078473, mean: 0.751973, max: 2.330280
09:37:07 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:07 DEBUG opendrift.models.physics_methods:917: min: 1.526128, mean: 4.672863, max: 8.316367
09:37:07 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.526128, mean: 4.672863, max: 8.316367
09:37:07 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:07 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:07 DEBUG opendrift:1731: (z: -4.459518 to 0.000000)
09:37:07 DEBUG opendrift:1749: Removed 4 elements.
09:37:07 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:07 DEBUG opendrift:1757: remove items from profile for z
09:37:07 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:07 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:07 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:07 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:07 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:07 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:07 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:07 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:07 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:07 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:07 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:07 DEBUG opendrift.models.physics_methods:917: min: 1.526128, mean: 4.672764, max: 8.316367
09:37:07 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:07 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.106389, dN_50: 0.008349
09:37:07 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:07 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0341830610544631
09:37:07 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:07 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:07 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:07 DEBUG opendrift.models.physics_methods:771: Advecting 580 of 5410 elements above 0.100m with wind-sheared ocean current (0.002240 m/s - 0.192515 m/s)
09:37:07 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:07 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018711510719575492 and 0.40857685187025483 m/s
09:37:07 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:07 DEBUG opendrift:2101: ======================================================================
09:37:07 INFO opendrift:2102: 2025-05-26 09:35:52.207946 - step 73 of 120 - 5410 active elements (590 deactivated)
09:37:07 DEBUG opendrift:2108: 0 elements scheduled.
09:37:07 DEBUG opendrift:2110: ======================================================================
09:37:07 DEBUG opendrift:2121: 35.068287776490145 <- latitude -> 35.90597865158191
09:37:07 DEBUG opendrift:2121: 22.988390041143333 <- longitude -> 23.87483758136602
09:37:07 DEBUG opendrift:2121: -85.94366995088328 <- z -> 0.0
09:37:07 DEBUG opendrift:2122: ---------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:07 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:07 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5410 elements
09:37:07 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:07 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:08 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:09 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:09 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:09 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:09 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 20x29x20) for time after (2025-05-26 12:00:00)
09:37:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 113 elements, expanding data 1
09:37:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.80) and
after (2025-05-26 12:00:00, weight 0.20) in time
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5410 elements
09:37:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5410 elements
09:37:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:09 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:09 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:09 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:09 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-26 12:00:00)
09:37:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.80) and
after (2025-05-26 12:00:00, weight 0.20) in time
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:09 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.326589 (min) 0.254962 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.351216 (min) 0.354395 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.82627 (min) 4.86448 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.99529 (min) -0.827268 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:09 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.038450, mean: 0.607661, max: 2.154663
09:37:09 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:09 DEBUG opendrift.models.physics_methods:917: min: 1.068261, mean: 4.173019, max: 7.996856
09:37:09 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.068261, mean: 4.173019, max: 7.996856
09:37:09 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:09 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:09 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:09 DEBUG opendrift:1749: Removed 4 elements.
09:37:09 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:09 DEBUG opendrift:1757: remove items from profile for z
09:37:09 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:09 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:09 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:09 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:09 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:09 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:09 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:09 DEBUG opendrift.models.physics_methods:917: min: 1.068261, mean: 4.173165, max: 7.996856
09:37:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.121993, dN_50: 0.009574
09:37:09 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:09 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03160724336254363
09:37:09 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:09 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:09 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:09 DEBUG opendrift.models.physics_methods:771: Advecting 575 of 5406 elements above 0.100m with wind-sheared ocean current (0.076020 m/s - 0.175470 m/s)
09:37:09 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:09 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0020811690078840293 and 0.5432010536035915 m/s
09:37:09 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:09 DEBUG opendrift:2101: ======================================================================
09:37:09 INFO opendrift:2102: 2025-05-26 10:35:52.207946 - step 74 of 120 - 5406 active elements (594 deactivated)
09:37:09 DEBUG opendrift:2108: 0 elements scheduled.
09:37:09 DEBUG opendrift:2110: ======================================================================
09:37:09 DEBUG opendrift:2121: 35.068544479129606 <- latitude -> 35.911655162077075
09:37:09 DEBUG opendrift:2121: 22.979332687297624 <- longitude -> 23.86725134666648
09:37:09 DEBUG opendrift:2121: -85.15182040387705 <- z -> 0.0
09:37:09 DEBUG opendrift:2122: ---------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:09 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.47) and
after (2025-05-26 12:00:00, weight 0.53) in time
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5406 elements
09:37:09 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:09 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:09 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.47) and
after (2025-05-26 12:00:00, weight 0.53) in time
09:37:09 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:09 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.346478 (min) 0.188245 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.334885 (min) 0.348655 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: -2.11956 (min) 5.06016 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: -7.298 (min) -0.346114 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:09 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.013563, mean: 0.472288, max: 1.940105
09:37:09 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:09 DEBUG opendrift.models.physics_methods:917: min: 0.634463, mean: 3.633825, max: 7.588261
09:37:09 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.634463, mean: 3.633825, max: 7.588261
09:37:09 DEBUG opendrift:648: No elements hit coastline.
09:37:09 DEBUG opendrift:1745: No elements to deactivate
09:37:09 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:09 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:09 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:09 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:09 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:09 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:09 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:09 DEBUG opendrift.models.physics_methods:917: min: 0.634463, mean: 3.633825, max: 7.588261
09:37:09 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:09 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.144756, dN_50: 0.011360
09:37:09 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:10 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.028460261972022973
09:37:10 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:10 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:10 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:10 DEBUG opendrift.models.physics_methods:771: Advecting 580 of 5406 elements above 0.100m with wind-sheared ocean current (0.005065 m/s - 0.159945 m/s)
09:37:10 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:10 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008425820205955976 and 0.46097528599955123 m/s
09:37:10 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:10 DEBUG opendrift:2101: ======================================================================
09:37:10 INFO opendrift:2102: 2025-05-26 11:35:52.207946 - step 75 of 120 - 5406 active elements (594 deactivated)
09:37:10 DEBUG opendrift:2108: 0 elements scheduled.
09:37:10 DEBUG opendrift:2110: ======================================================================
09:37:10 DEBUG opendrift:2121: 35.06697467949731 <- latitude -> 35.921607785946584
09:37:10 DEBUG opendrift:2121: 22.970124587927742 <- longitude -> 23.864976462011892
09:37:10 DEBUG opendrift:2121: -84.33221645634589 <- z -> 0.0
09:37:10 DEBUG opendrift:2122: ---------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:10 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:10 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:10 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:10 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:10 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:10 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.13) and
after (2025-05-26 12:00:00, weight 0.87) in time
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5406 elements
09:37:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 09:00:00 (before)
2025-05-26 12:00:00 (after)
09:37:10 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 09:00:00) in space (linearNDFast)
09:37:10 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:10 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 12:00:00) in space (linearNDFast)
09:37:10 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:10 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 09:00:00, weight 0.13) and
after (2025-05-26 12:00:00, weight 0.87) in time
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:10 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:10 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:10 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:10 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:10 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:10 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.387454 (min) 0.129457 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.328997 (min) 0.378262 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.44507 (min) 5.31061 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.62536 (min) 0.232717 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:10 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:10 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.002370, mean: 0.380104, max: 1.773611
09:37:10 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:10 DEBUG opendrift.models.physics_methods:917: min: 0.265212, mean: 3.194482, max: 7.255356
09:37:10 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.265212, mean: 3.194482, max: 7.255356
09:37:10 DEBUG opendrift:651: 2 elements hit land, moving them to the coastline.
09:37:10 DEBUG opendrift:1729: 2 elements scheduled for deactivation (stranded)
09:37:10 DEBUG opendrift:1731: (z: -16.996040 to -8.019144)
09:37:10 DEBUG opendrift:1749: Removed 2 elements.
09:37:10 DEBUG opendrift:1752: Removed 2 values from environment.
09:37:10 DEBUG opendrift:1757: remove items from profile for z
09:37:10 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:10 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:10 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:10 DEBUG opendrift:1761: Removed 2 values from environment_profiles.
09:37:10 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:10 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:10 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:10 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:10 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:10 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:10 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:10 DEBUG opendrift.models.physics_methods:917: min: 0.265212, mean: 3.194579, max: 7.255356
09:37:10 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:10 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.176076, dN_50: 0.013818
09:37:10 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:10 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.026018243124229352
09:37:10 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:10 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:10 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:10 DEBUG opendrift.models.physics_methods:771: Advecting 580 of 5404 elements above 0.100m with wind-sheared ocean current (0.040293 m/s - 0.147110 m/s)
09:37:10 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:10 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0028421261752021214 and 0.45785304501258667 m/s
09:37:10 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:10 DEBUG opendrift:2101: ======================================================================
09:37:10 INFO opendrift:2102: 2025-05-26 12:35:52.207946 - step 76 of 120 - 5404 active elements (596 deactivated)
09:37:10 DEBUG opendrift:2108: 0 elements scheduled.
09:37:10 DEBUG opendrift:2110: ======================================================================
09:37:10 DEBUG opendrift:2121: 35.07018302646961 <- latitude -> 35.921768533328034
09:37:10 DEBUG opendrift:2121: 22.954958109280323 <- longitude -> 23.861020223068543
09:37:10 DEBUG opendrift:2121: -83.22065516229098 <- z -> 0.0
09:37:10 DEBUG opendrift:2122: ---------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:10 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:10 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5404 elements
09:37:10 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:10 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:11 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:11 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:11 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:11 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 20x29x20) for time after (2025-05-26 15:00:00)
09:37:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 112 elements, expanding data 1
09:37:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.80) and
after (2025-05-26 15:00:00, weight 0.20) in time
09:37:11 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5404 elements
09:37:11 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5404 elements
09:37:11 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:11 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:11 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:11 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:11 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-26 15:00:00)
09:37:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:11 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:11 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:11 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.80) and
after (2025-05-26 15:00:00, weight 0.20) in time
09:37:11 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:11 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:11 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:11 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:11 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:11 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:11 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.38072 (min) 0.13844 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.321511 (min) 0.350865 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.897349 (min) 5.41563 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.14708 (min) 0.350939 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:11 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:11 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.000311, mean: 0.335357, max: 1.651044
09:37:11 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:11 DEBUG opendrift.models.physics_methods:917: min: 0.096073, mean: 2.949609, max: 7.000176
09:37:11 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.096073, mean: 2.949609, max: 7.000176
09:37:11 DEBUG opendrift:651: 6 elements hit land, moving them to the coastline.
09:37:11 DEBUG opendrift:1729: 6 elements scheduled for deactivation (stranded)
09:37:11 DEBUG opendrift:1731: (z: -12.332908 to 0.000000)
09:37:11 DEBUG opendrift:1749: Removed 6 elements.
09:37:11 DEBUG opendrift:1752: Removed 6 values from environment.
09:37:11 DEBUG opendrift:1757: remove items from profile for z
09:37:11 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:11 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:11 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:11 DEBUG opendrift:1761: Removed 6 values from environment_profiles.
09:37:11 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:11 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:11 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:11 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:11 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:11 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:11 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:11 DEBUG opendrift.models.physics_methods:917: min: 0.096073, mean: 2.950237, max: 7.000175
09:37:11 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:11 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.202948, dN_50: 0.015927
09:37:11 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:11 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.024220525091068815
09:37:11 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:11 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:11 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:12 DEBUG opendrift.models.physics_methods:771: Advecting 574 of 5398 elements above 0.100m with wind-sheared ocean current (0.039234 m/s - 0.140960 m/s)
09:37:12 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:12 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018992919334936194 and 0.4556755061330886 m/s
09:37:12 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:12 DEBUG opendrift:2101: ======================================================================
09:37:12 INFO opendrift:2102: 2025-05-26 13:35:52.207946 - step 77 of 120 - 5398 active elements (602 deactivated)
09:37:12 DEBUG opendrift:2108: 0 elements scheduled.
09:37:12 DEBUG opendrift:2110: ======================================================================
09:37:12 DEBUG opendrift:2121: 35.071646671473616 <- latitude -> 35.92349909102805
09:37:12 DEBUG opendrift:2121: 22.94851619869231 <- longitude -> 23.85700493728062
09:37:12 DEBUG opendrift:2121: -82.4430052600377 <- z -> 0.0
09:37:12 DEBUG opendrift:2122: ---------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.47) and
after (2025-05-26 15:00:00, weight 0.53) in time
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5398 elements
09:37:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.47) and
after (2025-05-26 15:00:00, weight 0.53) in time
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:12 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:12 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.321298 (min) 0.191462 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.311584 (min) 0.382896 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.416658 (min) 5.48826 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.82309 (min) 0.341243 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:12 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.006084, mean: 0.327095, max: 1.575124
09:37:12 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:12 DEBUG opendrift.models.physics_methods:917: min: 0.424920, mean: 2.900949, max: 6.837338
09:37:12 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.424920, mean: 2.900949, max: 6.837338
09:37:12 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:12 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:12 DEBUG opendrift:1731: (z: -0.423285 to 0.000000)
09:37:12 DEBUG opendrift:1749: Removed 5 elements.
09:37:12 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:12 DEBUG opendrift:1757: remove items from profile for z
09:37:12 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:12 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:12 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:12 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:12 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:12 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:12 DEBUG opendrift.models.physics_methods:917: min: 0.424920, mean: 2.902153, max: 6.837337
09:37:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.214867, dN_50: 0.016863
09:37:12 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:12 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.023106986101442364
09:37:12 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:12 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:12 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:12 DEBUG opendrift.models.physics_methods:771: Advecting 571 of 5393 elements above 0.100m with wind-sheared ocean current (0.014919 m/s - 0.135831 m/s)
09:37:12 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:12 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005123758321433694 and 0.4779792038558424 m/s
09:37:12 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:12 DEBUG opendrift:2101: ======================================================================
09:37:12 INFO opendrift:2102: 2025-05-26 14:35:52.207946 - step 78 of 120 - 5393 active elements (607 deactivated)
09:37:12 DEBUG opendrift:2108: 0 elements scheduled.
09:37:12 DEBUG opendrift:2110: ======================================================================
09:37:12 DEBUG opendrift:2121: 35.07094835100608 <- latitude -> 35.93542056483117
09:37:12 DEBUG opendrift:2121: 22.93488020560274 <- longitude -> 23.8524154504919
09:37:12 DEBUG opendrift:2121: -81.98447489152427 <- z -> 0.0
09:37:12 DEBUG opendrift:2122: ---------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.13) and
after (2025-05-26 15:00:00, weight 0.87) in time
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5393 elements
09:37:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 12:00:00 (before)
2025-05-26 15:00:00 (after)
09:37:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 12:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 15:00:00) in space (linearNDFast)
09:37:12 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:12 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 12:00:00, weight 0.13) and
after (2025-05-26 15:00:00, weight 0.87) in time
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:12 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:12 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:12 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.306059 (min) 0.243785 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.278297 (min) 0.439836 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.0264192 (min) 5.58238 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.50513 (min) 0.350381 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:12 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:12 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.009432, mean: 0.350851, max: 1.512147
09:37:12 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:12 DEBUG opendrift.models.physics_methods:917: min: 0.529099, mean: 3.015367, max: 6.699257
09:37:12 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.529099, mean: 3.015367, max: 6.699257
09:37:12 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:12 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:12 DEBUG opendrift:1731: (z: -19.092807 to 0.000000)
09:37:12 DEBUG opendrift:1749: Removed 3 elements.
09:37:12 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:12 DEBUG opendrift:1757: remove items from profile for z
09:37:12 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:12 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:12 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:12 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:12 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:12 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:12 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:12 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:12 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:12 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:12 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:12 DEBUG opendrift.models.physics_methods:917: min: 0.529099, mean: 3.016428, max: 6.699257
09:37:12 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:12 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.218742, dN_50: 0.017167
09:37:12 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:12 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.022183283840237312
09:37:12 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:12 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:12 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:12 DEBUG opendrift.models.physics_methods:771: Advecting 574 of 5390 elements above 0.100m with wind-sheared ocean current (0.008987 m/s - 0.142348 m/s)
09:37:12 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:12 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0027279883024029982 and 0.47831254201915446 m/s
09:37:12 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:12 DEBUG opendrift:2101: ======================================================================
09:37:12 INFO opendrift:2102: 2025-05-26 15:35:52.207946 - step 79 of 120 - 5390 active elements (610 deactivated)
09:37:12 DEBUG opendrift:2108: 0 elements scheduled.
09:37:12 DEBUG opendrift:2110: ======================================================================
09:37:12 DEBUG opendrift:2121: 35.073714782244316 <- latitude -> 35.94574949057447
09:37:12 DEBUG opendrift:2121: 22.931651163538998 <- longitude -> 23.860058519982125
09:37:12 DEBUG opendrift:2121: -81.48902975600176 <- z -> 0.0
09:37:12 DEBUG opendrift:2122: ---------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:12 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:12 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:12 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:12 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:12 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:12 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5390 elements
09:37:12 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:12 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:13 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:13 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:13 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:13 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 21x30x20) for time after (2025-05-26 18:00:00)
09:37:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:13 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:13 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:13 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.80) and
after (2025-05-26 18:00:00, weight 0.20) in time
09:37:13 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5390 elements
09:37:13 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5390 elements
09:37:13 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:13 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:14 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:14 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:14 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:14 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:14 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-26 18:00:00)
09:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.80) and
after (2025-05-26 18:00:00, weight 0.20) in time
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:14 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.270623 (min) 0.251761 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.258209 (min) 0.425424 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.650703 (min) 6.14967 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.53008 (min) 0.502848 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.026451, mean: 0.471868, max: 1.589402
09:37:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 0.886026, mean: 3.576629, max: 6.868255
09:37:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.886026, mean: 3.576629, max: 6.868255
09:37:14 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:14 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:14 DEBUG opendrift:1731: (z: -16.247734 to -9.715922)
09:37:14 DEBUG opendrift:1749: Removed 3 elements.
09:37:14 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:14 DEBUG opendrift:1757: remove items from profile for z
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:14 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:14 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 0.886026, mean: 3.577770, max: 6.868255
09:37:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.186302, dN_50: 0.014621
09:37:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.023316397043183397
09:37:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:14 DEBUG opendrift.models.physics_methods:771: Advecting 577 of 5387 elements above 0.100m with wind-sheared ocean current (0.031108 m/s - 0.169641 m/s)
09:37:14 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:14 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002148749085913589 and 0.42734852520725214 m/s
09:37:14 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:14 DEBUG opendrift:2101: ======================================================================
09:37:14 INFO opendrift:2102: 2025-05-26 16:35:52.207946 - step 80 of 120 - 5387 active elements (613 deactivated)
09:37:14 DEBUG opendrift:2108: 0 elements scheduled.
09:37:14 DEBUG opendrift:2110: ======================================================================
09:37:14 DEBUG opendrift:2121: 35.07275072959549 <- latitude -> 35.959573825426375
09:37:14 DEBUG opendrift:2121: 22.936508611796825 <- longitude -> 23.866867511920656
09:37:14 DEBUG opendrift:2121: -80.6287344917421 <- z -> 0.0
09:37:14 DEBUG opendrift:2122: ---------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:14 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.47) and
after (2025-05-26 18:00:00, weight 0.53) in time
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5387 elements
09:37:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.47) and
after (2025-05-26 18:00:00, weight 0.53) in time
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:14 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.2146 (min) 0.249918 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.234693 (min) 0.407838 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: 1.47129 (min) 7.33871 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.84978 (min) 0.72 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.053282, mean: 0.690620, max: 1.805985
09:37:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 1.257534, mean: 4.407850, max: 7.321274
09:37:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.257534, mean: 4.407850, max: 7.321274
09:37:14 DEBUG opendrift:651: 2 elements hit land, moving them to the coastline.
09:37:14 DEBUG opendrift:1729: 2 elements scheduled for deactivation (stranded)
09:37:14 DEBUG opendrift:1731: (z: -1.186929 to 0.000000)
09:37:14 DEBUG opendrift:1749: Removed 2 elements.
09:37:14 DEBUG opendrift:1752: Removed 2 values from environment.
09:37:14 DEBUG opendrift:1757: remove items from profile for z
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:14 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:14 DEBUG opendrift:1761: Removed 2 values from environment_profiles.
09:37:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 1.257534, mean: 4.408606, max: 7.321274
09:37:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.156912, dN_50: 0.012314
09:37:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02649308702674041
09:37:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:14 DEBUG opendrift.models.physics_methods:771: Advecting 578 of 5385 elements above 0.100m with wind-sheared ocean current (0.044151 m/s - 0.210894 m/s)
09:37:14 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:14 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008178870129438819 and 0.4721411244915887 m/s
09:37:14 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:14 DEBUG opendrift:2101: ======================================================================
09:37:14 INFO opendrift:2102: 2025-05-26 17:35:52.207946 - step 81 of 120 - 5385 active elements (615 deactivated)
09:37:14 DEBUG opendrift:2108: 0 elements scheduled.
09:37:14 DEBUG opendrift:2110: ======================================================================
09:37:14 DEBUG opendrift:2121: 35.07133623182429 <- latitude -> 35.96475087821516
09:37:14 DEBUG opendrift:2121: 22.92808349694261 <- longitude -> 23.878787908851017
09:37:14 DEBUG opendrift:2121: -79.18571755269801 <- z -> 0.0
09:37:14 DEBUG opendrift:2122: ---------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:14 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.13) and
after (2025-05-26 18:00:00, weight 0.87) in time
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:14 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:14 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5385 elements
09:37:14 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 15:00:00 (before)
2025-05-26 18:00:00 (after)
09:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 15:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 18:00:00) in space (linearNDFast)
09:37:14 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:14 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 15:00:00, weight 0.13) and
after (2025-05-26 18:00:00, weight 0.87) in time
09:37:14 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:14 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:14 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:14 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:14 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:14 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.161359 (min) 0.241605 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.236131 (min) 0.404826 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.13034 (min) 8.70029 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.18436 (min) 1.07763 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:14 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:14 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.130117, mean: 0.968669, max: 2.298962
09:37:14 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 1.965153, mean: 5.271231, max: 8.260294
09:37:14 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.965153, mean: 5.271231, max: 8.260294
09:37:14 DEBUG opendrift:651: 1 elements hit land, moving them to the coastline.
09:37:14 DEBUG opendrift:1729: 1 elements scheduled for deactivation (stranded)
09:37:14 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:14 DEBUG opendrift:1749: Removed 1 elements.
09:37:14 DEBUG opendrift:1752: Removed 1 values from environment.
09:37:14 DEBUG opendrift:1757: remove items from profile for z
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:14 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:14 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:14 DEBUG opendrift:1761: Removed 1 values from environment_profiles.
09:37:14 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:14 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:14 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:14 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:14 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:14 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:14 DEBUG opendrift.models.physics_methods:917: min: 1.965153, mean: 5.271636, max: 8.260294
09:37:14 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:14 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.139260, dN_50: 0.010929
09:37:14 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:14 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03372371222895849
09:37:14 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:14 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:14 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:15 DEBUG opendrift.models.physics_methods:771: Advecting 582 of 5384 elements above 0.100m with wind-sheared ocean current (0.008810 m/s - 0.248949 m/s)
09:37:15 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:15 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012431884139629146 and 0.5160716508631948 m/s
09:37:15 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:15 DEBUG opendrift:2101: ======================================================================
09:37:15 INFO opendrift:2102: 2025-05-26 18:35:52.207946 - step 82 of 120 - 5384 active elements (616 deactivated)
09:37:15 DEBUG opendrift:2108: 0 elements scheduled.
09:37:15 DEBUG opendrift:2110: ======================================================================
09:37:15 DEBUG opendrift:2121: 35.072188206284224 <- latitude -> 35.97037223853754
09:37:15 DEBUG opendrift:2121: 22.931657259348547 <- longitude -> 23.880604770384426
09:37:15 DEBUG opendrift:2121: -78.5747215588378 <- z -> 0.0
09:37:15 DEBUG opendrift:2122: ---------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:15 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 21x31x19) for time after (2025-05-26 21:00:00)
09:37:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.80) and
after (2025-05-26 21:00:00, weight 0.20) in time
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5384 elements
09:37:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:15 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:15 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-26 21:00:00)
09:37:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.80) and
after (2025-05-26 21:00:00, weight 0.20) in time
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:15 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.142655 (min) 0.237804 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.231289 (min) 0.43433 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.54328 (min) 9.04399 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.25889 (min) 1.43752 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:15 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.199089, mean: 1.118894, max: 2.536818
09:37:15 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:15 DEBUG opendrift.models.physics_methods:917: min: 2.430822, mean: 5.680499, max: 8.677092
09:37:15 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.430822, mean: 5.680499, max: 8.677092
09:37:15 DEBUG opendrift:651: 2 elements hit land, moving them to the coastline.
09:37:15 DEBUG opendrift:1729: 2 elements scheduled for deactivation (stranded)
09:37:15 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:15 DEBUG opendrift:1749: Removed 2 elements.
09:37:15 DEBUG opendrift:1752: Removed 2 values from environment.
09:37:15 DEBUG opendrift:1757: remove items from profile for z
09:37:15 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:15 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:15 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:15 DEBUG opendrift:1761: Removed 2 values from environment_profiles.
09:37:15 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:15 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:15 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:15 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:15 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:15 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:15 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:15 DEBUG opendrift.models.physics_methods:917: min: 2.430822, mean: 5.680991, max: 8.677093
09:37:15 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:15 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.135578, dN_50: 0.010640
09:37:15 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:15 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03721240678186724
09:37:15 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:15 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:15 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:15 DEBUG opendrift.models.physics_methods:771: Advecting 583 of 5382 elements above 0.100m with wind-sheared ocean current (0.003838 m/s - 0.270602 m/s)
09:37:15 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:15 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0002444475013202762 and 0.4427043825078108 m/s
09:37:15 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:15 DEBUG opendrift:2101: ======================================================================
09:37:15 INFO opendrift:2102: 2025-05-26 19:35:52.207946 - step 83 of 120 - 5382 active elements (618 deactivated)
09:37:15 DEBUG opendrift:2108: 0 elements scheduled.
09:37:15 DEBUG opendrift:2110: ======================================================================
09:37:15 DEBUG opendrift:2121: 35.07088529341273 <- latitude -> 35.977817429922375
09:37:15 DEBUG opendrift:2121: 22.92545509544718 <- longitude -> 23.888289111177258
09:37:15 DEBUG opendrift:2121: -77.78695038588894 <- z -> 0.0
09:37:15 DEBUG opendrift:2122: ---------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:15 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.47) and
after (2025-05-26 21:00:00, weight 0.53) in time
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5382 elements
09:37:15 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:15 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:15 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.47) and
after (2025-05-26 21:00:00, weight 0.53) in time
09:37:15 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:15 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.133506 (min) 0.262356 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.22925 (min) 0.35027 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.74271 (min) 8.67507 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.17939 (min) 1.52635 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:15 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.237300, mean: 1.157953, max: 2.504432
09:37:15 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:15 DEBUG opendrift.models.physics_methods:917: min: 2.653863, mean: 5.780634, max: 8.621528
09:37:15 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.653863, mean: 5.780634, max: 8.621528
09:37:15 DEBUG opendrift:651: 2 elements hit land, moving them to the coastline.
09:37:15 DEBUG opendrift:1729: 2 elements scheduled for deactivation (stranded)
09:37:15 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:15 DEBUG opendrift:1749: Removed 2 elements.
09:37:15 DEBUG opendrift:1752: Removed 2 values from environment.
09:37:15 DEBUG opendrift:1757: remove items from profile for z
09:37:15 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:15 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:15 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:15 DEBUG opendrift:1761: Removed 2 values from environment_profiles.
09:37:15 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:15 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:15 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:15 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:15 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:15 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:15 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:15 DEBUG opendrift.models.physics_methods:917: min: 2.653863, mean: 5.780758, max: 8.621528
09:37:15 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:15 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.138085, dN_50: 0.010837
09:37:16 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:16 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03673740422669059
09:37:16 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:16 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:16 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:16 DEBUG opendrift.models.physics_methods:771: Advecting 586 of 5380 elements above 0.100m with wind-sheared ocean current (0.039450 m/s - 0.263658 m/s)
09:37:16 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:16 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005317651037398495 and 0.44619789090442247 m/s
09:37:16 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:16 DEBUG opendrift:2101: ======================================================================
09:37:16 INFO opendrift:2102: 2025-05-26 20:35:52.207946 - step 84 of 120 - 5380 active elements (620 deactivated)
09:37:16 DEBUG opendrift:2108: 0 elements scheduled.
09:37:16 DEBUG opendrift:2110: ======================================================================
09:37:16 DEBUG opendrift:2121: 35.06712457073461 <- latitude -> 35.98011303465165
09:37:16 DEBUG opendrift:2121: 22.93060107425241 <- longitude -> 23.894374958097327
09:37:16 DEBUG opendrift:2121: -76.44855191066978 <- z -> 0.0
09:37:16 DEBUG opendrift:2122: ---------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:16 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:16 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:16 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.13) and
after (2025-05-26 21:00:00, weight 0.87) in time
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 18:00:00 (before)
2025-05-26 21:00:00 (after)
09:37:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 18:00:00) in space (linearNDFast)
09:37:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:16 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-26 21:00:00) in space (linearNDFast)
09:37:16 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:16 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 18:00:00, weight 0.13) and
after (2025-05-26 21:00:00, weight 0.87) in time
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:16 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:16 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:16 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:16 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.116131 (min) 0.303733 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.229115 (min) 0.320439 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.95439 (min) 8.35068 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.12222 (min) 1.72897 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:16 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:16 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.277330, mean: 1.201017, max: 2.452681
09:37:16 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:16 DEBUG opendrift.models.physics_methods:917: min: 2.868983, mean: 5.889304, max: 8.531986
09:37:16 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.868983, mean: 5.889304, max: 8.531986
09:37:16 DEBUG opendrift:648: No elements hit coastline.
09:37:16 DEBUG opendrift:1745: No elements to deactivate
09:37:16 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:16 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:16 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:16 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:16 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:16 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:16 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:16 DEBUG opendrift.models.physics_methods:917: min: 2.868983, mean: 5.889304, max: 8.531986
09:37:16 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:16 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.140393, dN_50: 0.011018
09:37:16 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:16 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03597835346464743
09:37:16 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:16 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:16 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:16 DEBUG opendrift.models.physics_methods:771: Advecting 592 of 5380 elements above 0.100m with wind-sheared ocean current (0.014206 m/s - 0.257702 m/s)
09:37:16 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:16 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001901362929314405 and 0.4035045247963953 m/s
09:37:16 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:16 DEBUG opendrift:2101: ======================================================================
09:37:16 INFO opendrift:2102: 2025-05-26 21:35:52.207946 - step 85 of 120 - 5380 active elements (620 deactivated)
09:37:16 DEBUG opendrift:2108: 0 elements scheduled.
09:37:16 DEBUG opendrift:2110: ======================================================================
09:37:16 DEBUG opendrift:2121: 35.057571524911296 <- latitude -> 35.984737689086266
09:37:16 DEBUG opendrift:2121: 22.939530428135907 <- longitude -> 23.907142999685608
09:37:16 DEBUG opendrift:2121: -75.4469697957927 <- z -> 0.0
09:37:16 DEBUG opendrift:2122: ---------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:16 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:16 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5380 elements
09:37:16 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:16 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:16 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:17 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:17 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:17 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:17 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 22x31x19) for time after (2025-05-27 00:00:00)
09:37:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 111 elements, expanding data 1
09:37:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.80) and
after (2025-05-27 00:00:00, weight 0.20) in time
09:37:17 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5380 elements
09:37:17 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5380 elements
09:37:17 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:17 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:17 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:17 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:17 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-27 00:00:00)
09:37:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:17 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:17 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:17 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.80) and
after (2025-05-27 00:00:00, weight 0.20) in time
09:37:17 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:17 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:17 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.110022 (min) 0.327071 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.233951 (min) 0.265413 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.49977 (min) 7.44152 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.20876 (min) 1.71398 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:17 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.211012, mean: 1.152459, max: 2.119561
09:37:17 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:17 DEBUG opendrift.models.physics_methods:917: min: 2.502550, mean: 5.754680, max: 7.931450
09:37:17 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.502550, mean: 5.754680, max: 7.931450
09:37:17 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:17 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:17 DEBUG opendrift:1731: (z: -2.718099 to 0.000000)
09:37:17 DEBUG opendrift:1749: Removed 5 elements.
09:37:17 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:17 DEBUG opendrift:1757: remove items from profile for z
09:37:17 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:17 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:17 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:17 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:17 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:17 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:17 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:17 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:17 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:17 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:17 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:17 DEBUG opendrift.models.physics_methods:917: min: 2.502550, mean: 5.756457, max: 7.931450
09:37:17 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:17 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.148232, dN_50: 0.011633
09:37:17 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:17 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.031092397834613882
09:37:17 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:17 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:17 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:18 DEBUG opendrift.models.physics_methods:771: Advecting 587 of 5375 elements above 0.100m with wind-sheared ocean current (0.024761 m/s - 0.236180 m/s)
09:37:18 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:18 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0011690331060154715 and 0.44343180891575984 m/s
09:37:18 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:18 DEBUG opendrift:2101: ======================================================================
09:37:18 INFO opendrift:2102: 2025-05-26 22:35:52.207946 - step 86 of 120 - 5375 active elements (625 deactivated)
09:37:18 DEBUG opendrift:2108: 0 elements scheduled.
09:37:18 DEBUG opendrift:2110: ======================================================================
09:37:18 DEBUG opendrift:2121: 35.05860100621936 <- latitude -> 35.98607256682296
09:37:18 DEBUG opendrift:2121: 22.94649673572966 <- longitude -> 23.91130737626998
09:37:18 DEBUG opendrift:2121: -74.68235917750184 <- z -> 0.0
09:37:18 DEBUG opendrift:2122: ---------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.47) and
after (2025-05-27 00:00:00, weight 0.53) in time
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5375 elements
09:37:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.47) and
after (2025-05-27 00:00:00, weight 0.53) in time
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:18 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:18 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.120074 (min) 0.359532 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.228444 (min) 0.259634 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: x_wind: 1.55784 (min) 6.75098 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.25283 (min) 1.55518 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:18 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.085040, mean: 1.057184, max: 2.081642
09:37:18 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:18 DEBUG opendrift.models.physics_methods:917: min: 1.588696, mean: 5.482783, max: 7.860182
09:37:18 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.588696, mean: 5.482783, max: 7.860182
09:37:18 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:18 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:18 DEBUG opendrift:1731: (z: -3.779078 to 0.000000)
09:37:18 DEBUG opendrift:1749: Removed 3 elements.
09:37:18 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:18 DEBUG opendrift:1757: remove items from profile for z
09:37:18 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:18 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:18 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:18 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:18 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:18 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:18 DEBUG opendrift.models.physics_methods:917: min: 1.588696, mean: 5.483665, max: 7.860182
09:37:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.160408, dN_50: 0.012589
09:37:18 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:18 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03053621799887445
09:37:18 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:18 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:18 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:18 DEBUG opendrift.models.physics_methods:771: Advecting 595 of 5372 elements above 0.100m with wind-sheared ocean current (0.033650 m/s - 0.217469 m/s)
09:37:18 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:18 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018486757826086922 and 0.42200864385571774 m/s
09:37:18 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:18 DEBUG opendrift:2101: ======================================================================
09:37:18 INFO opendrift:2102: 2025-05-26 23:35:52.207946 - step 87 of 120 - 5372 active elements (628 deactivated)
09:37:18 DEBUG opendrift:2108: 0 elements scheduled.
09:37:18 DEBUG opendrift:2110: ======================================================================
09:37:18 DEBUG opendrift:2121: 35.05361765173927 <- latitude -> 35.98787192873449
09:37:18 DEBUG opendrift:2121: 22.948574473977626 <- longitude -> 23.923482315779538
09:37:18 DEBUG opendrift:2121: -73.73660834209768 <- z -> 0.0
09:37:18 DEBUG opendrift:2122: ---------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.13) and
after (2025-05-27 00:00:00, weight 0.87) in time
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5372 elements
09:37:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-26 21:00:00 (before)
2025-05-27 00:00:00 (after)
09:37:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-26 21:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 00:00:00) in space (linearNDFast)
09:37:18 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:18 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-26 21:00:00, weight 0.13) and
after (2025-05-27 00:00:00, weight 0.87) in time
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:18 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:18 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:18 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.130007 (min) 0.373909 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.2259 (min) 0.258187 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.532083 (min) 6.74175 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.35118 (min) 1.47833 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:18 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:18 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.017764, mean: 0.984000, max: 2.110401
09:37:18 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:18 DEBUG opendrift.models.physics_methods:917: min: 0.726112, mean: 5.260151, max: 7.914292
09:37:18 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.726112, mean: 5.260151, max: 7.914292
09:37:18 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:18 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:18 DEBUG opendrift:1731: (z: -17.862214 to 0.000000)
09:37:18 DEBUG opendrift:1749: Removed 3 elements.
09:37:18 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:18 DEBUG opendrift:1757: remove items from profile for z
09:37:18 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:18 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:18 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:18 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:18 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:18 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:18 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:18 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:18 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:18 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:18 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:18 DEBUG opendrift.models.physics_methods:917: min: 0.726112, mean: 5.261043, max: 7.914292
09:37:18 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:18 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.172715, dN_50: 0.013555
09:37:18 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:18 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.030958033129314076
09:37:18 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:18 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:18 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:18 DEBUG opendrift.models.physics_methods:771: Advecting 602 of 5369 elements above 0.100m with wind-sheared ocean current (0.025493 m/s - 0.207592 m/s)
09:37:18 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:18 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0038443776359773207 and 0.4764031945564811 m/s
09:37:18 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:18 DEBUG opendrift:2101: ======================================================================
09:37:18 INFO opendrift:2102: 2025-05-27 00:35:52.207946 - step 88 of 120 - 5369 active elements (631 deactivated)
09:37:18 DEBUG opendrift:2108: 0 elements scheduled.
09:37:18 DEBUG opendrift:2110: ======================================================================
09:37:18 DEBUG opendrift:2121: 35.048561757971584 <- latitude -> 35.986683617388444
09:37:18 DEBUG opendrift:2121: 22.946867807421217 <- longitude -> 23.935429663162925
09:37:18 DEBUG opendrift:2121: -73.04962856147088 <- z -> 0.0
09:37:18 DEBUG opendrift:2122: ---------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:18 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:18 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5369 elements
09:37:18 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:18 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:19 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:19 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:19 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:19 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 22x31x19) for time after (2025-05-27 03:00:00)
09:37:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 124 elements, expanding data 1
09:37:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.80) and
after (2025-05-27 03:00:00, weight 0.20) in time
09:37:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5369 elements
09:37:19 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5369 elements
09:37:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:19 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:19 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:19 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:19 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-27 03:00:00)
09:37:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:19 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.80) and
after (2025-05-27 03:00:00, weight 0.20) in time
09:37:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:19 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:19 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.132516 (min) 0.38206 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.217742 (min) 0.259568 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.0126378 (min) 6.45533 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.49122 (min) 1.51511 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:19 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.001428, mean: 0.834903, max: 2.061659
09:37:19 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:19 DEBUG opendrift.models.physics_methods:917: min: 0.205875, mean: 4.826084, max: 7.822363
09:37:19 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.205875, mean: 4.826084, max: 7.822363
09:37:19 DEBUG opendrift:651: 2 elements hit land, moving them to the coastline.
09:37:19 DEBUG opendrift:1729: 2 elements scheduled for deactivation (stranded)
09:37:19 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:19 DEBUG opendrift:1749: Removed 2 elements.
09:37:19 DEBUG opendrift:1752: Removed 2 values from environment.
09:37:19 DEBUG opendrift:1757: remove items from profile for z
09:37:19 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:19 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:19 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:19 DEBUG opendrift:1761: Removed 2 values from environment_profiles.
09:37:19 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:19 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:19 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:19 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:19 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:19 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:19 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:19 DEBUG opendrift.models.physics_methods:917: min: 0.205875, mean: 4.827015, max: 7.822363
09:37:19 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:19 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.190028, dN_50: 0.014913
09:37:19 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:19 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.030243123064454237
09:37:19 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:19 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:19 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:19 DEBUG opendrift.models.physics_methods:771: Advecting 596 of 5367 elements above 0.100m with wind-sheared ocean current (0.007228 m/s - 0.197050 m/s)
09:37:19 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:19 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015682182788624524 and 0.4216964811958547 m/s
09:37:19 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:19 DEBUG opendrift:2101: ======================================================================
09:37:19 INFO opendrift:2102: 2025-05-27 01:35:52.207946 - step 89 of 120 - 5367 active elements (633 deactivated)
09:37:19 DEBUG opendrift:2108: 0 elements scheduled.
09:37:19 DEBUG opendrift:2110: ======================================================================
09:37:19 DEBUG opendrift:2121: 35.04392644515048 <- latitude -> 35.98293022272651
09:37:19 DEBUG opendrift:2121: 22.93981145338045 <- longitude -> 23.94702502029512
09:37:19 DEBUG opendrift:2121: -72.24942518811986 <- z -> 0.0
09:37:19 DEBUG opendrift:2122: ---------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:19 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:19 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5367 elements
09:37:19 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:19 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:19 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.47) and
after (2025-05-27 03:00:00, weight 0.53) in time
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5367 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5367 elements
09:37:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.47) and
after (2025-05-27 03:00:00, weight 0.53) in time
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:20 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.171646 (min) 0.371313 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.222847 (min) 0.257571 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.177184 (min) 5.9914 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.66143 (min) 1.66101 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:20 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.000041, mean: 0.666065, max: 1.974679
09:37:20 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:20 DEBUG opendrift.models.physics_methods:917: min: 0.034857, mean: 4.302087, max: 7.655575
09:37:20 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.034857, mean: 4.302087, max: 7.655575
09:37:20 DEBUG opendrift:651: 8 elements hit land, moving them to the coastline.
09:37:20 DEBUG opendrift:1729: 8 elements scheduled for deactivation (stranded)
09:37:20 DEBUG opendrift:1731: (z: -1.909433 to 0.000000)
09:37:20 DEBUG opendrift:1749: Removed 8 elements.
09:37:20 DEBUG opendrift:1752: Removed 8 values from environment.
09:37:20 DEBUG opendrift:1757: remove items from profile for z
09:37:20 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:20 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:20 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:20 DEBUG opendrift:1761: Removed 8 values from environment_profiles.
09:37:20 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:20 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:20 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:20 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:20 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:20 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:20 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:20 DEBUG opendrift.models.physics_methods:917: min: 0.034857, mean: 4.304347, max: 7.655575
09:37:20 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:20 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.211145, dN_50: 0.016571
09:37:20 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:20 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02896736701267908
09:37:20 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:20 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:20 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:20 DEBUG opendrift.models.physics_methods:771: Advecting 592 of 5359 elements above 0.100m with wind-sheared ocean current (0.001224 m/s - 0.181967 m/s)
09:37:20 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:20 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001122187852409315 and 0.45890582871618757 m/s
09:37:20 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:20 DEBUG opendrift:2101: ======================================================================
09:37:20 INFO opendrift:2102: 2025-05-27 02:35:52.207946 - step 90 of 120 - 5359 active elements (641 deactivated)
09:37:20 DEBUG opendrift:2108: 0 elements scheduled.
09:37:20 DEBUG opendrift:2110: ======================================================================
09:37:20 DEBUG opendrift:2121: 35.0406464188565 <- latitude -> 35.984853941854176
09:37:20 DEBUG opendrift:2121: 22.93760049748941 <- longitude -> 23.956956947918975
09:37:20 DEBUG opendrift:2121: -71.13099093877335 <- z -> 0.0
09:37:20 DEBUG opendrift:2122: ---------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:20 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.13) and
after (2025-05-27 03:00:00, weight 0.87) in time
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5359 elements
09:37:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 00:00:00 (before)
2025-05-27 03:00:00 (after)
09:37:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 00:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 03:00:00) in space (linearNDFast)
09:37:20 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:20 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 00:00:00, weight 0.13) and
after (2025-05-27 03:00:00, weight 0.87) in time
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:20 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:20 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:20 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.264615 (min) 0.369959 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.220349 (min) 0.260017 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.548998 (min) 5.52022 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.80922 (min) 1.85436 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:20 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:20 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.002277, mean: 0.549852, max: 1.890224
09:37:20 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:20 DEBUG opendrift.models.physics_methods:917: min: 0.259979, mean: 3.915446, max: 7.490075
09:37:20 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.259979, mean: 3.915446, max: 7.490075
09:37:20 DEBUG opendrift:651: 6 elements hit land, moving them to the coastline.
09:37:20 DEBUG opendrift:1729: 6 elements scheduled for deactivation (stranded)
09:37:20 DEBUG opendrift:1731: (z: -8.595292 to 0.000000)
09:37:20 DEBUG opendrift:1749: Removed 6 elements.
09:37:20 DEBUG opendrift:1752: Removed 6 values from environment.
09:37:20 DEBUG opendrift:1757: remove items from profile for z
09:37:20 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:20 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:20 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:20 DEBUG opendrift:1761: Removed 6 values from environment_profiles.
09:37:20 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:20 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:20 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:20 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:20 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:20 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:20 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:20 DEBUG opendrift.models.physics_methods:917: min: 0.259979, mean: 3.917081, max: 7.490075
09:37:20 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:20 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.225244, dN_50: 0.017677
09:37:20 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:20 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.027728633441824215
09:37:20 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:20 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:20 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:20 DEBUG opendrift.models.physics_methods:771: Advecting 593 of 5353 elements above 0.100m with wind-sheared ocean current (0.009128 m/s - 0.173550 m/s)
09:37:20 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:20 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002271555033194497 and 0.4750519508547653 m/s
09:37:20 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:20 DEBUG opendrift:2101: ======================================================================
09:37:20 INFO opendrift:2102: 2025-05-27 03:35:52.207946 - step 91 of 120 - 5353 active elements (647 deactivated)
09:37:20 DEBUG opendrift:2108: 0 elements scheduled.
09:37:20 DEBUG opendrift:2110: ======================================================================
09:37:20 DEBUG opendrift:2121: 35.03500689517691 <- latitude -> 35.986417872260304
09:37:20 DEBUG opendrift:2121: 22.9284583365059 <- longitude -> 23.975181596357466
09:37:20 DEBUG opendrift:2121: -69.8043963959516 <- z -> 0.0
09:37:20 DEBUG opendrift:2122: ---------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:20 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:20 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:20 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:20 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:20 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:20 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:20 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5353 elements
09:37:20 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:20 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:21 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:21 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:21 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:21 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 22x32x18) for time after (2025-05-27 06:00:00)
09:37:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:21 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 147 elements, expanding data 1
09:37:21 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.80) and
after (2025-05-27 06:00:00, weight 0.20) in time
09:37:21 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:21 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:21 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:21 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:21 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:21 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:21 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5353 elements
09:37:21 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5353 elements
09:37:21 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:21 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:21 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:21 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:21 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 9x7x1) for time after (2025-05-27 06:00:00)
09:37:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:21 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:21 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:21 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.80) and
after (2025-05-27 06:00:00, weight 0.20) in time
09:37:21 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:21 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:21 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:21 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:21 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:21 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:21 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:21 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.338677 (min) 0.359489 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.226877 (min) 0.261039 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.759847 (min) 4.93106 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.59099 (min) 1.59593 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:21 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:21 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.005294, mean: 0.466887, max: 1.666810
09:37:21 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:21 DEBUG opendrift.models.physics_methods:917: min: 0.396404, mean: 3.620249, max: 7.033519
09:37:21 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.396404, mean: 3.620249, max: 7.033519
09:37:21 DEBUG opendrift:651: 10 elements hit land, moving them to the coastline.
09:37:21 DEBUG opendrift:1729: 10 elements scheduled for deactivation (stranded)
09:37:21 DEBUG opendrift:1731: (z: -16.067544 to 0.000000)
09:37:21 DEBUG opendrift:1749: Removed 10 elements.
09:37:21 DEBUG opendrift:1752: Removed 10 values from environment.
09:37:21 DEBUG opendrift:1757: remove items from profile for z
09:37:21 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:21 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:21 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:21 DEBUG opendrift:1761: Removed 10 values from environment_profiles.
09:37:21 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:21 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:21 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:21 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:21 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:21 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:21 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:21 DEBUG opendrift.models.physics_methods:917: min: 0.396404, mean: 3.622812, max: 7.033519
09:37:21 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:21 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.238607, dN_50: 0.018726
09:37:21 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:21 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.024451768402403116
09:37:21 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:21 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:21 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:22 DEBUG opendrift.models.physics_methods:771: Advecting 588 of 5343 elements above 0.100m with wind-sheared ocean current (0.013918 m/s - 0.160241 m/s)
09:37:22 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:22 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002041474614903433 and 0.4413566182556876 m/s
09:37:22 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:22 DEBUG opendrift:2101: ======================================================================
09:37:22 INFO opendrift:2102: 2025-05-27 04:35:52.207946 - step 92 of 120 - 5343 active elements (657 deactivated)
09:37:22 DEBUG opendrift:2108: 0 elements scheduled.
09:37:22 DEBUG opendrift:2110: ======================================================================
09:37:22 DEBUG opendrift:2121: 35.021103070578064 <- latitude -> 35.986415275851016
09:37:22 DEBUG opendrift:2121: 22.914024471931874 <- longitude -> 23.990632270304037
09:37:22 DEBUG opendrift:2121: -68.59484741210139 <- z -> 0.0
09:37:22 DEBUG opendrift:2122: ---------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.47) and
after (2025-05-27 06:00:00, weight 0.53) in time
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5343 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.47) and
after (2025-05-27 06:00:00, weight 0.53) in time
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:22 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.407043 (min) 0.344145 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.22712 (min) 0.263556 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.753461 (min) 4.30427 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: -6.15272 (min) 1.03363 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:22 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.003566, mean: 0.392506, max: 1.387015
09:37:22 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:22 DEBUG opendrift.models.physics_methods:917: min: 0.325339, mean: 3.328543, max: 6.416085
09:37:22 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.325339, mean: 3.328543, max: 6.416085
09:37:22 DEBUG opendrift:651: 9 elements hit land, moving them to the coastline.
09:37:22 DEBUG opendrift:1729: 9 elements scheduled for deactivation (stranded)
09:37:22 DEBUG opendrift:1731: (z: -14.659629 to 0.000000)
09:37:22 DEBUG opendrift:1749: Removed 9 elements.
09:37:22 DEBUG opendrift:1752: Removed 9 values from environment.
09:37:22 DEBUG opendrift:1757: remove items from profile for z
09:37:22 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:22 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:22 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:22 DEBUG opendrift:1761: Removed 9 values from environment_profiles.
09:37:22 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:22 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:22 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:22 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:22 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:22 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:22 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:22 DEBUG opendrift.models.physics_methods:917: min: 0.325339, mean: 3.330328, max: 6.416085
09:37:22 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:22 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.254835, dN_50: 0.019999
09:37:22 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:22 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.020347928106375455
09:37:22 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:22 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:22 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:22 DEBUG opendrift.models.physics_methods:771: Advecting 581 of 5334 elements above 0.100m with wind-sheared ocean current (0.011422 m/s - 0.144268 m/s)
09:37:22 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:22 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018718398077601347 and 0.4272781374950061 m/s
09:37:22 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:22 DEBUG opendrift:2101: ======================================================================
09:37:22 INFO opendrift:2102: 2025-05-27 05:35:52.207946 - step 93 of 120 - 5334 active elements (666 deactivated)
09:37:22 DEBUG opendrift:2108: 0 elements scheduled.
09:37:22 DEBUG opendrift:2110: ======================================================================
09:37:22 DEBUG opendrift:2121: 35.01404847068441 <- latitude -> 35.991909857745846
09:37:22 DEBUG opendrift:2121: 22.8877666268711 <- longitude -> 24.008651479584845
09:37:22 DEBUG opendrift:2121: -68.01843412646741 <- z -> 0.0
09:37:22 DEBUG opendrift:2122: ---------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.13) and
after (2025-05-27 06:00:00, weight 0.87) in time
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5334 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 03:00:00 (before)
2025-05-27 06:00:00 (after)
09:37:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 03:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 06:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 03:00:00, weight 0.13) and
after (2025-05-27 06:00:00, weight 0.87) in time
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:22 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:22 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:22 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.473575 (min) 0.322896 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.228824 (min) 0.269615 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.840815 (min) 3.60765 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.6441 (min) 0.35421 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:22 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:22 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.001797, mean: 0.330194, max: 1.103827
09:37:22 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:22 DEBUG opendrift.models.physics_methods:917: min: 0.230923, mean: 3.063852, max: 5.723742
09:37:22 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.230923, mean: 3.063852, max: 5.723742
09:37:22 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:22 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:22 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:22 DEBUG opendrift:1749: Removed 3 elements.
09:37:22 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:22 DEBUG opendrift:1757: remove items from profile for z
09:37:22 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:22 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:22 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:22 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:22 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:22 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:22 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:22 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:22 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:22 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:22 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:22 DEBUG opendrift.models.physics_methods:917: min: 0.230923, mean: 3.064481, max: 5.723742
09:37:22 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:22 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.270907, dN_50: 0.021261
09:37:22 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:22 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.016194330808802403
09:37:22 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:22 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:22 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:22 DEBUG opendrift.models.physics_methods:771: Advecting 580 of 5331 elements above 0.100m with wind-sheared ocean current (0.008108 m/s - 0.129426 m/s)
09:37:22 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:22 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013604375778454793 and 0.4706650168857665 m/s
09:37:22 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:22 DEBUG opendrift:2101: ======================================================================
09:37:22 INFO opendrift:2102: 2025-05-27 06:35:52.207946 - step 94 of 120 - 5331 active elements (669 deactivated)
09:37:22 DEBUG opendrift:2108: 0 elements scheduled.
09:37:22 DEBUG opendrift:2110: ======================================================================
09:37:22 DEBUG opendrift:2121: 35.00939345352416 <- latitude -> 35.988789857572684
09:37:22 DEBUG opendrift:2121: 22.872877369059328 <- longitude -> 24.017513512576542
09:37:22 DEBUG opendrift:2121: -67.04850586066436 <- z -> 0.0
09:37:22 DEBUG opendrift:2122: ---------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:22 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:22 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:22 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:22 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:22 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 23x32x18) for time after (2025-05-27 09:00:00)
09:37:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:22 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 163 elements, expanding data 1
09:37:22 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.80) and
after (2025-05-27 09:00:00, weight 0.20) in time
09:37:22 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:22 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:22 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:22 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:22 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:22 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5331 elements
09:37:22 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:22 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:23 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:23 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:23 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:23 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x7x1) for time after (2025-05-27 09:00:00)
09:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.80) and
after (2025-05-27 09:00:00, weight 0.20) in time
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:23 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.464594 (min) 0.322177 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.229853 (min) 0.271515 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.900922 (min) 3.41077 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.30084 (min) -0.421654 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:23 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.008822, mean: 0.343710, max: 0.977414
09:37:23 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 0.511706, mean: 3.144380, max: 5.386030
09:37:23 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 0.511706, mean: 3.144380, max: 5.386030
09:37:23 DEBUG opendrift:651: 4 elements hit land, moving them to the coastline.
09:37:23 DEBUG opendrift:1729: 4 elements scheduled for deactivation (stranded)
09:37:23 DEBUG opendrift:1731: (z: -10.704532 to 0.000000)
09:37:23 DEBUG opendrift:1749: Removed 4 elements.
09:37:23 DEBUG opendrift:1752: Removed 4 values from environment.
09:37:23 DEBUG opendrift:1757: remove items from profile for z
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:23 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:23 DEBUG opendrift:1761: Removed 4 values from environment_profiles.
09:37:23 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:23 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:23 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:23 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:23 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 0.511706, mean: 3.144795, max: 5.386030
09:37:23 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:23 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.264271, dN_50: 0.020740
09:37:23 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:23 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.014340195006352585
09:37:23 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:23 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:23 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:23 DEBUG opendrift.models.physics_methods:771: Advecting 579 of 5327 elements above 0.100m with wind-sheared ocean current (0.011244 m/s - 0.134572 m/s)
09:37:23 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:23 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0031476420918518755 and 0.4624396716073938 m/s
09:37:23 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:23 DEBUG opendrift:2101: ======================================================================
09:37:23 INFO opendrift:2102: 2025-05-27 07:35:52.207946 - step 95 of 120 - 5327 active elements (673 deactivated)
09:37:23 DEBUG opendrift:2108: 0 elements scheduled.
09:37:23 DEBUG opendrift:2110: ======================================================================
09:37:23 DEBUG opendrift:2121: 35.00847561853624 <- latitude -> 35.9912099408677
09:37:23 DEBUG opendrift:2121: 22.861252467881787 <- longitude -> 24.02775165403963
09:37:23 DEBUG opendrift:2121: -66.52559705905733 <- z -> 0.0
09:37:23 DEBUG opendrift:2122: ---------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.47) and
after (2025-05-27 09:00:00, weight 0.53) in time
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5327 elements
09:37:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.47) and
after (2025-05-27 09:00:00, weight 0.53) in time
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:23 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.39561 (min) 0.31585 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.2279 (min) 0.292466 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.958091 (min) 3.50099 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.0476 (min) -1.21933 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:23 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.040189, mean: 0.417105, max: 0.921477
09:37:23 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 1.092149, mean: 3.472468, max: 5.229640
09:37:23 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.092149, mean: 3.472468, max: 5.229640
09:37:23 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:23 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:23 DEBUG opendrift:1731: (z: -18.677307 to 0.000000)
09:37:23 DEBUG opendrift:1749: Removed 5 elements.
09:37:23 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:23 DEBUG opendrift:1757: remove items from profile for z
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:23 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:23 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:23 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:23 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:23 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:23 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:23 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 1.092149, mean: 3.472526, max: 5.229640
09:37:23 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:23 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.247040, dN_50: 0.019388
09:37:23 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:23 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.013519758002375927
09:37:23 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:23 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:23 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:23 DEBUG opendrift.models.physics_methods:771: Advecting 577 of 5322 elements above 0.100m with wind-sheared ocean current (0.022432 m/s - 0.151965 m/s)
09:37:23 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:23 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008856872714453859 and 0.47155966519299547 m/s
09:37:23 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:23 DEBUG opendrift:2101: ======================================================================
09:37:23 INFO opendrift:2102: 2025-05-27 08:35:52.207946 - step 96 of 120 - 5322 active elements (678 deactivated)
09:37:23 DEBUG opendrift:2108: 0 elements scheduled.
09:37:23 DEBUG opendrift:2110: ======================================================================
09:37:23 DEBUG opendrift:2121: 34.99969026287345 <- latitude -> 35.99548192256715
09:37:23 DEBUG opendrift:2121: 22.851916504001043 <- longitude -> 24.03833822469055
09:37:23 DEBUG opendrift:2121: -66.06273505793897 <- z -> 0.0
09:37:23 DEBUG opendrift:2122: ---------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.13) and
after (2025-05-27 09:00:00, weight 0.87) in time
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5322 elements
09:37:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 06:00:00 (before)
2025-05-27 09:00:00 (after)
09:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 06:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 09:00:00) in space (linearNDFast)
09:37:23 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:23 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 06:00:00, weight 0.13) and
after (2025-05-27 09:00:00, weight 0.87) in time
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:23 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:23 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.369802 (min) 0.32082 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.270805 (min) 0.428345 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: x_wind: -1.03189 (min) 3.68598 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.76229 (min) -0.703397 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:23 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:23 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.104780, mean: 0.508583, max: 0.890242
09:37:23 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 1.763469, mean: 3.829003, max: 5.140242
09:37:23 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 1.763469, mean: 3.829003, max: 5.140242
09:37:23 DEBUG opendrift:651: 6 elements hit land, moving them to the coastline.
09:37:23 DEBUG opendrift:1729: 6 elements scheduled for deactivation (stranded)
09:37:23 DEBUG opendrift:1731: (z: -9.516941 to 0.000000)
09:37:23 DEBUG opendrift:1749: Removed 6 elements.
09:37:23 DEBUG opendrift:1752: Removed 6 values from environment.
09:37:23 DEBUG opendrift:1757: remove items from profile for z
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:23 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:23 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:23 DEBUG opendrift:1761: Removed 6 values from environment_profiles.
09:37:23 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:23 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:23 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:23 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:23 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:23 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:23 DEBUG opendrift.models.physics_methods:917: min: 1.763469, mean: 3.829209, max: 5.140242
09:37:23 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:23 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.232849, dN_50: 0.018274
09:37:23 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:23 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.013061625513974209
09:37:23 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:23 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:23 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:23 DEBUG opendrift.models.physics_methods:771: Advecting 581 of 5316 elements above 0.100m with wind-sheared ocean current (0.005640 m/s - 0.172431 m/s)
09:37:23 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:23 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001403324371886806 and 0.4332440828543239 m/s
09:37:23 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:23 DEBUG opendrift:2101: ======================================================================
09:37:23 INFO opendrift:2102: 2025-05-27 09:35:52.207946 - step 97 of 120 - 5316 active elements (684 deactivated)
09:37:23 DEBUG opendrift:2108: 0 elements scheduled.
09:37:23 DEBUG opendrift:2110: ======================================================================
09:37:23 DEBUG opendrift:2121: 34.9915737169225 <- latitude -> 36.00016528479255
09:37:23 DEBUG opendrift:2121: 22.839023221909635 <- longitude -> 24.048609797271514
09:37:23 DEBUG opendrift:2121: -64.8793704597122 <- z -> 0.0
09:37:23 DEBUG opendrift:2122: ---------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:23 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:23 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:23 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:23 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:23 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:23 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:23 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5316 elements
09:37:23 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:23 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:23 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:25 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:25 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 24x33x18) for time after (2025-05-27 12:00:00)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 186 elements, expanding data 1
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.80) and
after (2025-05-27 12:00:00, weight 0.20) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5316 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5316 elements
09:37:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:25 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:25 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-27 12:00:00)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.80) and
after (2025-05-27 12:00:00, weight 0.20) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.274138 (min) 0.305646 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.300427 (min) 0.48662 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.689839 (min) 4.23667 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.6638 (min) -0.426888 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.140625, mean: 0.531617, max: 0.948254
09:37:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:25 DEBUG opendrift.models.physics_methods:917: min: 2.042964, mean: 3.929180, max: 5.305080
09:37:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.042964, mean: 3.929180, max: 5.305080
09:37:25 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:25 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:25 DEBUG opendrift:1731: (z: -17.015179 to 0.000000)
09:37:25 DEBUG opendrift:1749: Removed 5 elements.
09:37:25 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:25 DEBUG opendrift:1757: remove items from profile for z
09:37:25 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:25 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:25 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:25 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:25 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:25 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:25 DEBUG opendrift.models.physics_methods:917: min: 2.042964, mean: 3.929386, max: 5.305080
09:37:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.232665, dN_50: 0.018260
09:37:25 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:25 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.01391250708307485
09:37:25 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:25 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:25 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:25 DEBUG opendrift.models.physics_methods:771: Advecting 574 of 5311 elements above 0.100m with wind-sheared ocean current (0.071727 m/s - 0.171924 m/s)
09:37:25 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:25 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013110905721524526 and 0.47119044800853577 m/s
09:37:25 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:25 DEBUG opendrift:2101: ======================================================================
09:37:25 INFO opendrift:2102: 2025-05-27 10:35:52.207946 - step 98 of 120 - 5311 active elements (689 deactivated)
09:37:25 DEBUG opendrift:2108: 0 elements scheduled.
09:37:25 DEBUG opendrift:2110: ======================================================================
09:37:25 DEBUG opendrift:2121: 34.98410106575645 <- latitude -> 36.007226589410116
09:37:25 DEBUG opendrift:2121: 22.83061107025651 <- longitude -> 24.06158525489273
09:37:25 DEBUG opendrift:2121: -63.844921661245394 <- z -> 0.0
09:37:25 DEBUG opendrift:2122: ---------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.47) and
after (2025-05-27 12:00:00, weight 0.53) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5311 elements
09:37:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.47) and
after (2025-05-27 12:00:00, weight 0.53) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.183023 (min) 0.292504 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.320349 (min) 0.506372 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: -0.0388668 (min) 5.05534 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.68675 (min) -0.35791 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.160256, mean: 0.571358, max: 1.100528
09:37:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:25 DEBUG opendrift.models.physics_methods:917: min: 2.180904, mean: 4.095119, max: 5.715182
09:37:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.180904, mean: 4.095119, max: 5.715182
09:37:25 DEBUG opendrift:651: 3 elements hit land, moving them to the coastline.
09:37:25 DEBUG opendrift:1729: 3 elements scheduled for deactivation (stranded)
09:37:25 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:25 DEBUG opendrift:1749: Removed 3 elements.
09:37:25 DEBUG opendrift:1752: Removed 3 values from environment.
09:37:25 DEBUG opendrift:1757: remove items from profile for z
09:37:25 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:25 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:25 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:25 DEBUG opendrift:1761: Removed 3 values from environment_profiles.
09:37:25 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:25 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:25 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:25 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:25 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:25 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:25 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:25 DEBUG opendrift.models.physics_methods:917: min: 2.180904, mean: 4.095124, max: 5.715182
09:37:25 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:25 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.231261, dN_50: 0.018149
09:37:25 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:25 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.016145947034218115
09:37:25 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:25 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:25 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:25 DEBUG opendrift.models.physics_methods:771: Advecting 579 of 5308 elements above 0.100m with wind-sheared ocean current (0.006312 m/s - 0.170024 m/s)
09:37:25 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:25 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0029764247380397735 and 0.4152108809342185 m/s
09:37:25 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:25 DEBUG opendrift:2101: ======================================================================
09:37:25 INFO opendrift:2102: 2025-05-27 11:35:52.207946 - step 99 of 120 - 5308 active elements (692 deactivated)
09:37:25 DEBUG opendrift:2108: 0 elements scheduled.
09:37:25 DEBUG opendrift:2110: ======================================================================
09:37:25 DEBUG opendrift:2121: 34.96965494432186 <- latitude -> 36.01266522384936
09:37:25 DEBUG opendrift:2121: 22.82761666642967 <- longitude -> 24.070197695092013
09:37:25 DEBUG opendrift:2121: -63.120432020828694 <- z -> 0.0
09:37:25 DEBUG opendrift:2122: ---------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:25 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.13) and
after (2025-05-27 12:00:00, weight 0.87) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:25 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:25 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5308 elements
09:37:25 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 09:00:00 (before)
2025-05-27 12:00:00 (after)
09:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 09:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 12:00:00) in space (linearNDFast)
09:37:25 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:25 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 09:00:00, weight 0.13) and
after (2025-05-27 12:00:00, weight 0.87) in time
09:37:25 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:25 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:25 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:25 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:25 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:25 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.158722 (min) 0.271621 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.347809 (min) 0.53469 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0.64215 (min) 6.02492 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.24567 (min) -0.114357 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:25 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:25 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.201530, mean: 0.700128, max: 1.283895
09:37:25 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:25 DEBUG opendrift.models.physics_methods:917: min: 2.445677, mean: 4.540781, max: 6.172973
09:37:25 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.445677, mean: 4.540781, max: 6.172973
09:37:25 DEBUG opendrift:651: 9 elements hit land, moving them to the coastline.
09:37:25 DEBUG opendrift:1729: 9 elements scheduled for deactivation (stranded)
09:37:25 DEBUG opendrift:1731: (z: -19.592112 to 0.000000)
09:37:26 DEBUG opendrift:1749: Removed 9 elements.
09:37:26 DEBUG opendrift:1752: Removed 9 values from environment.
09:37:26 DEBUG opendrift:1757: remove items from profile for z
09:37:26 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:26 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:26 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:26 DEBUG opendrift:1761: Removed 9 values from environment_profiles.
09:37:26 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:26 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:26 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:26 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:26 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:26 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:26 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:26 DEBUG opendrift.models.physics_methods:917: min: 2.445677, mean: 4.541520, max: 6.172973
09:37:26 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:26 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.219913, dN_50: 0.017259
09:37:26 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:26 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.01883544993788078
09:37:26 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:26 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:26 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:26 DEBUG opendrift.models.physics_methods:771: Advecting 576 of 5299 elements above 0.100m with wind-sheared ocean current (0.019031 m/s - 0.180979 m/s)
09:37:26 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:26 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0019954920772322134 and 0.4204500958088935 m/s
09:37:26 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:26 DEBUG opendrift:2101: ======================================================================
09:37:26 INFO opendrift:2102: 2025-05-27 12:35:52.207946 - step 100 of 120 - 5299 active elements (701 deactivated)
09:37:26 DEBUG opendrift:2108: 0 elements scheduled.
09:37:26 DEBUG opendrift:2110: ======================================================================
09:37:26 DEBUG opendrift:2121: 34.95589828800167 <- latitude -> 36.02430389253352
09:37:26 DEBUG opendrift:2121: 22.82639348525931 <- longitude -> 24.074427826101704
09:37:26 DEBUG opendrift:2121: -61.9125453349064 <- z -> 0.0
09:37:26 DEBUG opendrift:2122: ---------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:26 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:26 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:26 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:26 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:26 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:26 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:26 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5299 elements
09:37:26 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:26 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:26 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:26 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:26 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:26 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 25x35x18) for time after (2025-05-27 15:00:00)
09:37:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:26 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:26 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:26 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 203 elements, expanding data 1
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.80) and
after (2025-05-27 15:00:00, weight 0.20) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5299 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5299 elements
09:37:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:27 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:27 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:27 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:27 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-27 15:00:00)
09:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.80) and
after (2025-05-27 15:00:00, weight 0.20) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:27 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.155802 (min) 0.270275 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.355242 (min) 0.474743 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: 1.38126 (min) 6.53763 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.45552 (min) 0.0438741 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:27 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.223334, mean: 0.794492, max: 1.436689
09:37:27 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.574586, mean: 4.837766, max: 6.529966
09:37:27 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.574586, mean: 4.837766, max: 6.529966
09:37:27 DEBUG opendrift:651: 10 elements hit land, moving them to the coastline.
09:37:27 DEBUG opendrift:1729: 10 elements scheduled for deactivation (stranded)
09:37:27 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:27 DEBUG opendrift:1749: Removed 10 elements.
09:37:27 DEBUG opendrift:1752: Removed 10 values from environment.
09:37:27 DEBUG opendrift:1757: remove items from profile for z
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:27 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:27 DEBUG opendrift:1761: Removed 10 values from environment_profiles.
09:37:27 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:27 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:27 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:27 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:27 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.574586, mean: 4.838995, max: 6.529966
09:37:27 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:27 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.214388, dN_50: 0.016825
09:37:27 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:27 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02107651403100958
09:37:27 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:27 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:27 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:27 DEBUG opendrift.models.physics_methods:771: Advecting 566 of 5289 elements above 0.100m with wind-sheared ocean current (0.048610 m/s - 0.195328 m/s)
09:37:27 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:27 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0032725594992848175 and 0.45701062251391206 m/s
09:37:27 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:27 DEBUG opendrift:2101: ======================================================================
09:37:27 INFO opendrift:2102: 2025-05-27 13:35:52.207946 - step 101 of 120 - 5289 active elements (711 deactivated)
09:37:27 DEBUG opendrift:2108: 0 elements scheduled.
09:37:27 DEBUG opendrift:2110: ======================================================================
09:37:27 DEBUG opendrift:2121: 34.943314234679015 <- latitude -> 36.037029813300705
09:37:27 DEBUG opendrift:2121: 22.82463720553049 <- longitude -> 24.083846274396326
09:37:27 DEBUG opendrift:2121: -60.8367915766447 <- z -> 0.0
09:37:27 DEBUG opendrift:2122: ---------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:27 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.47) and
after (2025-05-27 15:00:00, weight 0.53) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5289 elements
09:37:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.47) and
after (2025-05-27 15:00:00, weight 0.53) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:27 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.143701 (min) 0.290261 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.348684 (min) 0.373317 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.14276 (min) 6.69213 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.91608 (min) 0.0540869 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:27 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.244234, mean: 0.828821, max: 1.569901
09:37:27 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.692359, mean: 4.941047, max: 6.825990
09:37:27 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.692359, mean: 4.941047, max: 6.825990
09:37:27 DEBUG opendrift:651: 7 elements hit land, moving them to the coastline.
09:37:27 DEBUG opendrift:1729: 7 elements scheduled for deactivation (stranded)
09:37:27 DEBUG opendrift:1731: (z: -5.996414 to 0.000000)
09:37:27 DEBUG opendrift:1749: Removed 7 elements.
09:37:27 DEBUG opendrift:1752: Removed 7 values from environment.
09:37:27 DEBUG opendrift:1757: remove items from profile for z
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:27 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:27 DEBUG opendrift:1761: Removed 7 values from environment_profiles.
09:37:27 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:27 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:27 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:27 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:27 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.692358, mean: 4.942254, max: 6.825990
09:37:27 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:27 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.214654, dN_50: 0.016846
09:37:27 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:27 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02303037096641914
09:37:27 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:27 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:27 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:27 DEBUG opendrift.models.physics_methods:771: Advecting 563 of 5282 elements above 0.100m with wind-sheared ocean current (0.021691 m/s - 0.198789 m/s)
09:37:27 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:27 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002505714060011058 and 0.46855239202298615 m/s
09:37:27 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:27 DEBUG opendrift:2101: ======================================================================
09:37:27 INFO opendrift:2102: 2025-05-27 14:35:52.207946 - step 102 of 120 - 5282 active elements (718 deactivated)
09:37:27 DEBUG opendrift:2108: 0 elements scheduled.
09:37:27 DEBUG opendrift:2110: ======================================================================
09:37:27 DEBUG opendrift:2121: 34.93129477855615 <- latitude -> 36.04920009110083
09:37:27 DEBUG opendrift:2121: 22.835519487894555 <- longitude -> 24.099799105884916
09:37:27 DEBUG opendrift:2121: -59.741732923402814 <- z -> 0.0
09:37:27 DEBUG opendrift:2122: ---------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:27 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.13) and
after (2025-05-27 15:00:00, weight 0.87) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:27 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:27 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5282 elements
09:37:27 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 12:00:00 (before)
2025-05-27 15:00:00 (after)
09:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 12:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 15:00:00) in space (linearNDFast)
09:37:27 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:27 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 12:00:00, weight 0.13) and
after (2025-05-27 15:00:00, weight 0.87) in time
09:37:27 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:27 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:27 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:27 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:27 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:27 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.148916 (min) 0.312704 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.351969 (min) 0.338721 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.92037 (min) 6.97607 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.36828 (min) 0.0663407 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:27 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:27 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.299120, mean: 0.869762, max: 1.722175
09:37:27 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.979560, mean: 5.061789, max: 7.149378
09:37:27 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.979560, mean: 5.061789, max: 7.149378
09:37:27 DEBUG opendrift:651: 12 elements hit land, moving them to the coastline.
09:37:27 DEBUG opendrift:1729: 12 elements scheduled for deactivation (stranded)
09:37:27 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:27 DEBUG opendrift:1749: Removed 12 elements.
09:37:27 DEBUG opendrift:1752: Removed 12 values from environment.
09:37:27 DEBUG opendrift:1757: remove items from profile for z
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:27 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:27 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:27 DEBUG opendrift:1761: Removed 12 values from environment_profiles.
09:37:27 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:27 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:27 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:27 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:27 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:27 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:27 DEBUG opendrift.models.physics_methods:917: min: 2.979560, mean: 5.063694, max: 7.149378
09:37:27 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:27 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.214619, dN_50: 0.016843
09:37:27 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:27 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02526381973159938
09:37:27 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:27 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:27 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:27 DEBUG opendrift.models.physics_methods:771: Advecting 552 of 5270 elements above 0.100m with wind-sheared ocean current (0.029806 m/s - 0.201377 m/s)
09:37:27 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:27 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0008457954175027621 and 0.42881109190455613 m/s
09:37:28 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:28 DEBUG opendrift:2101: ======================================================================
09:37:28 INFO opendrift:2102: 2025-05-27 15:35:52.207946 - step 103 of 120 - 5270 active elements (730 deactivated)
09:37:28 DEBUG opendrift:2108: 0 elements scheduled.
09:37:28 DEBUG opendrift:2110: ======================================================================
09:37:28 DEBUG opendrift:2121: 34.92234101421437 <- latitude -> 36.056655600278596
09:37:28 DEBUG opendrift:2121: 22.84275597180659 <- longitude -> 24.112961840746156
09:37:28 DEBUG opendrift:2121: -59.07962677204589 <- z -> 0.0
09:37:28 DEBUG opendrift:2122: ---------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:28 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:28 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:28 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:28 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:28 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 25x37x17) for time after (2025-05-27 18:00:00)
09:37:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:28 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:28 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 235 elements, expanding data 1
09:37:28 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.80) and
after (2025-05-27 18:00:00, weight 0.20) in time
09:37:28 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:28 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:28 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:28 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:28 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:28 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5270 elements
09:37:28 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:29 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:29 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:29 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-27 18:00:00)
09:37:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.80) and
after (2025-05-27 18:00:00, weight 0.20) in time
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:29 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.163996 (min) 0.327902 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.36177 (min) 0.280923 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.27826 (min) 7.18047 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.48728 (min) 0.0339254 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:29 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.295496, mean: 0.857216, max: 1.731125
09:37:29 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.961456, mean: 5.016427, max: 7.167931
09:37:29 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.961456, mean: 5.016427, max: 7.167931
09:37:29 DEBUG opendrift:651: 7 elements hit land, moving them to the coastline.
09:37:29 DEBUG opendrift:1729: 7 elements scheduled for deactivation (stranded)
09:37:29 DEBUG opendrift:1731: (z: 0.000000 to 0.000000)
09:37:29 DEBUG opendrift:1749: Removed 7 elements.
09:37:29 DEBUG opendrift:1752: Removed 7 values from environment.
09:37:29 DEBUG opendrift:1757: remove items from profile for z
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:29 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:29 DEBUG opendrift:1761: Removed 7 values from environment_profiles.
09:37:29 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:29 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:29 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:29 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:29 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.961457, mean: 5.017691, max: 7.167931
09:37:29 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:29 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.220322, dN_50: 0.017291
09:37:29 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:29 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.025395094235534876
09:37:29 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:29 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:29 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:29 DEBUG opendrift.models.physics_methods:771: Advecting 550 of 5263 elements above 0.100m with wind-sheared ocean current (0.039672 m/s - 0.211819 m/s)
09:37:29 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:29 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0025497119969691384 and 0.488136998666484 m/s
09:37:29 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:29 DEBUG opendrift:2101: ======================================================================
09:37:29 INFO opendrift:2102: 2025-05-27 16:35:52.207946 - step 104 of 120 - 5263 active elements (737 deactivated)
09:37:29 DEBUG opendrift:2108: 0 elements scheduled.
09:37:29 DEBUG opendrift:2110: ======================================================================
09:37:29 DEBUG opendrift:2121: 34.907689989480524 <- latitude -> 36.06251313418457
09:37:29 DEBUG opendrift:2121: 22.850008756146693 <- longitude -> 24.136347579036403
09:37:29 DEBUG opendrift:2121: -58.66674356082458 <- z -> 0.0
09:37:29 DEBUG opendrift:2122: ---------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.47) and
after (2025-05-27 18:00:00, weight 0.53) in time
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5263 elements
09:37:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.47) and
after (2025-05-27 18:00:00, weight 0.53) in time
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:29 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.169857 (min) 0.352408 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.38342 (min) 0.26121 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.36599 (min) 7.76344 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.36888 (min) 0.747521 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:29 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.278746, mean: 0.813852, max: 1.637134
09:37:29 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.876299, mean: 4.868871, max: 6.970625
09:37:29 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.876299, mean: 4.868871, max: 6.970625
09:37:29 DEBUG opendrift:651: 5 elements hit land, moving them to the coastline.
09:37:29 DEBUG opendrift:1729: 5 elements scheduled for deactivation (stranded)
09:37:29 DEBUG opendrift:1731: (z: -12.338924 to 0.000000)
09:37:29 DEBUG opendrift:1749: Removed 5 elements.
09:37:29 DEBUG opendrift:1752: Removed 5 values from environment.
09:37:29 DEBUG opendrift:1757: remove items from profile for z
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:29 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:29 DEBUG opendrift:1761: Removed 5 values from environment_profiles.
09:37:29 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:29 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:29 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:29 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:29 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.876299, mean: 4.869405, max: 6.970624
09:37:29 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:29 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.230324, dN_50: 0.018076
09:37:29 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:29 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.024016496000306243
09:37:29 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:29 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:29 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:29 DEBUG opendrift.models.physics_methods:771: Advecting 546 of 5258 elements above 0.100m with wind-sheared ocean current (0.000077 m/s - 0.226941 m/s)
09:37:29 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:29 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005442594041168019 and 0.40152343365022763 m/s
09:37:29 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:29 DEBUG opendrift:2101: ======================================================================
09:37:29 INFO opendrift:2102: 2025-05-27 17:35:52.207946 - step 105 of 120 - 5258 active elements (742 deactivated)
09:37:29 DEBUG opendrift:2108: 0 elements scheduled.
09:37:29 DEBUG opendrift:2110: ======================================================================
09:37:29 DEBUG opendrift:2121: 34.8947235072653 <- latitude -> 36.069229280608575
09:37:29 DEBUG opendrift:2121: 22.862798139179986 <- longitude -> 24.151998332724485
09:37:29 DEBUG opendrift:2121: -57.41053704286352 <- z -> 0.0
09:37:29 DEBUG opendrift:2122: ---------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.13) and
after (2025-05-27 18:00:00, weight 0.87) in time
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5258 elements
09:37:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 15:00:00 (before)
2025-05-27 18:00:00 (after)
09:37:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 15:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 18:00:00) in space (linearNDFast)
09:37:29 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:29 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 15:00:00, weight 0.13) and
after (2025-05-27 18:00:00, weight 0.87) in time
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:29 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:29 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.17693 (min) 0.371441 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.404501 (min) 0.250236 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.35565 (min) 8.34154 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.29543 (min) 1.57377 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:29 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:29 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.277438, mean: 0.780776, max: 1.879901
09:37:29 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.869543, mean: 4.745404, max: 7.469594
09:37:29 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.869543, mean: 4.745404, max: 7.469594
09:37:29 DEBUG opendrift:651: 21 elements hit land, moving them to the coastline.
09:37:29 DEBUG opendrift:1729: 21 elements scheduled for deactivation (stranded)
09:37:29 DEBUG opendrift:1731: (z: -7.361727 to 0.000000)
09:37:29 DEBUG opendrift:1749: Removed 21 elements.
09:37:29 DEBUG opendrift:1752: Removed 21 values from environment.
09:37:29 DEBUG opendrift:1757: remove items from profile for z
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:29 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:29 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:29 DEBUG opendrift:1761: Removed 21 values from environment_profiles.
09:37:29 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:29 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:29 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:29 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:29 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:29 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:29 DEBUG opendrift.models.physics_methods:917: min: 2.879092, mean: 4.750426, max: 7.469594
09:37:29 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:29 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.240889, dN_50: 0.018905
09:37:29 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:29 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.027577222389009923
09:37:29 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:29 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:29 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:29 DEBUG opendrift.models.physics_methods:771: Advecting 531 of 5237 elements above 0.100m with wind-sheared ocean current (0.002753 m/s - 0.239303 m/s)
09:37:29 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:29 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0005569322362461206 and 0.4436721917306487 m/s
09:37:29 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:29 DEBUG opendrift:2101: ======================================================================
09:37:29 INFO opendrift:2102: 2025-05-27 18:35:52.207946 - step 106 of 120 - 5237 active elements (763 deactivated)
09:37:29 DEBUG opendrift:2108: 0 elements scheduled.
09:37:29 DEBUG opendrift:2110: ======================================================================
09:37:29 DEBUG opendrift:2121: 34.8798295320407 <- latitude -> 36.06192261368508
09:37:29 DEBUG opendrift:2121: 22.870477813959344 <- longitude -> 24.166697052693355
09:37:29 DEBUG opendrift:2121: -56.69922040473868 <- z -> 0.0
09:37:29 DEBUG opendrift:2122: ---------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:29 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:29 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:29 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:29 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:29 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:29 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:29 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5237 elements
09:37:29 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:29 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:31 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:33 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:33 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 25x38x17) for time after (2025-05-27 21:00:00)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 285 elements, expanding data 1
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.80) and
after (2025-05-27 21:00:00, weight 0.20) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5237 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5237 elements
09:37:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:33 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:33 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-27 21:00:00)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.80) and
after (2025-05-27 21:00:00, weight 0.20) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:33 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.163115 (min) 0.395965 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.42767 (min) 0.237918 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.29665 (min) 8.744 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_wind: -5.01054 (min) 2.22578 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:33 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.278071, mean: 0.827625, max: 2.128182
09:37:33 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:33 DEBUG opendrift.models.physics_methods:917: min: 2.872813, mean: 4.866514, max: 7.947562
09:37:33 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.872813, mean: 4.866514, max: 7.947562
09:37:33 DEBUG opendrift:651: 24 elements hit land, moving them to the coastline.
09:37:33 DEBUG opendrift:1729: 24 elements scheduled for deactivation (stranded)
09:37:33 DEBUG opendrift:1731: (z: -2.256683 to 0.000000)
09:37:33 DEBUG opendrift:1749: Removed 24 elements.
09:37:33 DEBUG opendrift:1752: Removed 24 values from environment.
09:37:33 DEBUG opendrift:1757: remove items from profile for z
09:37:33 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:33 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:33 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:33 DEBUG opendrift:1761: Removed 24 values from environment_profiles.
09:37:33 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:33 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:33 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:33 DEBUG opendrift.models.physics_methods:917: min: 2.938349, mean: 4.873999, max: 7.947562
09:37:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.244598, dN_50: 0.019196
09:37:33 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:33 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.031218829906633935
09:37:33 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:33 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:33 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:33 DEBUG opendrift.models.physics_methods:771: Advecting 505 of 5213 elements above 0.100m with wind-sheared ocean current (0.037442 m/s - 0.252757 m/s)
09:37:33 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:33 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002354082211346359 and 0.4261582670508275 m/s
09:37:33 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:33 DEBUG opendrift:2101: ======================================================================
09:37:33 INFO opendrift:2102: 2025-05-27 19:35:52.207946 - step 107 of 120 - 5213 active elements (787 deactivated)
09:37:33 DEBUG opendrift:2108: 0 elements scheduled.
09:37:33 DEBUG opendrift:2110: ======================================================================
09:37:33 DEBUG opendrift:2121: 34.86494906658373 <- latitude -> 36.063126918948164
09:37:33 DEBUG opendrift:2121: 22.8774927511097 <- longitude -> 24.185482483966364
09:37:33 DEBUG opendrift:2121: -55.91128437228222 <- z -> 0.0
09:37:33 DEBUG opendrift:2122: ---------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.47) and
after (2025-05-27 21:00:00, weight 0.53) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5213 elements
09:37:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.47) and
after (2025-05-27 21:00:00, weight 0.53) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:33 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.157453 (min) 0.393649 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.433794 (min) 0.255253 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.28477 (min) 9.08581 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.60611 (min) 2.68897 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:33 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.306589, mean: 0.932018, max: 2.399305
09:37:33 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:33 DEBUG opendrift.models.physics_methods:917: min: 3.016529, mean: 5.147358, max: 8.438638
09:37:33 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.016529, mean: 5.147358, max: 8.438638
09:37:33 DEBUG opendrift:651: 16 elements hit land, moving them to the coastline.
09:37:33 DEBUG opendrift:1729: 16 elements scheduled for deactivation (stranded)
09:37:33 DEBUG opendrift:1731: (z: -5.219466 to 0.000000)
09:37:33 DEBUG opendrift:1749: Removed 16 elements.
09:37:33 DEBUG opendrift:1752: Removed 16 values from environment.
09:37:33 DEBUG opendrift:1757: remove items from profile for z
09:37:33 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:33 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:33 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:33 DEBUG opendrift:1761: Removed 16 values from environment_profiles.
09:37:33 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:33 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:33 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:33 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:33 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:33 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:33 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:33 DEBUG opendrift.models.physics_methods:917: min: 3.016529, mean: 5.152354, max: 8.438638
09:37:33 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:33 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.243695, dN_50: 0.019125
09:37:33 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:33 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03519547659784669
09:37:33 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:33 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:33 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:33 DEBUG opendrift.models.physics_methods:771: Advecting 499 of 5197 elements above 0.100m with wind-sheared ocean current (0.002739 m/s - 0.268646 m/s)
09:37:33 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:33 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0028282717859398876 and 0.48222736866845706 m/s
09:37:33 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:33 DEBUG opendrift:2101: ======================================================================
09:37:33 INFO opendrift:2102: 2025-05-27 20:35:52.207946 - step 108 of 120 - 5197 active elements (803 deactivated)
09:37:33 DEBUG opendrift:2108: 0 elements scheduled.
09:37:33 DEBUG opendrift:2110: ======================================================================
09:37:33 DEBUG opendrift:2121: 34.851200067146934 <- latitude -> 36.0590130187264
09:37:33 DEBUG opendrift:2121: 22.876574481102836 <- longitude -> 24.201157395839104
09:37:33 DEBUG opendrift:2121: -55.199151971686675 <- z -> 0.0
09:37:33 DEBUG opendrift:2122: ---------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:33 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.13) and
after (2025-05-27 21:00:00, weight 0.87) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:33 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:33 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5197 elements
09:37:33 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 18:00:00 (before)
2025-05-27 21:00:00 (after)
09:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 18:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-27 21:00:00) in space (linearNDFast)
09:37:33 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:33 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 18:00:00, weight 0.13) and
after (2025-05-27 21:00:00, weight 0.87) in time
09:37:33 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:33 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:33 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:33 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:33 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:33 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.189092 (min) 0.387538 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.431788 (min) 0.2806 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.97611 (min) 9.5577 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.60253 (min) 3.0628 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:33 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:33 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.322396, mean: 1.048077, max: 2.760350
09:37:33 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:33 DEBUG opendrift.models.physics_methods:917: min: 3.093318, mean: 5.438003, max: 9.051316
09:37:33 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.093318, mean: 5.438003, max: 9.051316
09:37:33 DEBUG opendrift:651: 18 elements hit land, moving them to the coastline.
09:37:33 DEBUG opendrift:1729: 18 elements scheduled for deactivation (stranded)
09:37:33 DEBUG opendrift:1731: (z: -10.487958 to 0.000000)
09:37:34 DEBUG opendrift:1749: Removed 18 elements.
09:37:34 DEBUG opendrift:1752: Removed 18 values from environment.
09:37:34 DEBUG opendrift:1757: remove items from profile for z
09:37:34 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:34 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:34 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:34 DEBUG opendrift:1761: Removed 18 values from environment_profiles.
09:37:34 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:34 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:34 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:34 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:34 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:34 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:34 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:34 DEBUG opendrift.models.physics_methods:917: min: 3.093318, mean: 5.444289, max: 9.051315
09:37:34 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:34 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.242774, dN_50: 0.019053
09:37:34 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:34 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.04049102405183947
09:37:34 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:34 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:34 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:34 DEBUG opendrift.models.physics_methods:771: Advecting 493 of 5179 elements above 0.100m with wind-sheared ocean current (0.014887 m/s - 0.296585 m/s)
09:37:34 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:34 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002046118237478586 and 0.4956673412090573 m/s
09:37:34 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:34 DEBUG opendrift:2101: ======================================================================
09:37:34 INFO opendrift:2102: 2025-05-27 21:35:52.207946 - step 109 of 120 - 5179 active elements (821 deactivated)
09:37:34 DEBUG opendrift:2108: 0 elements scheduled.
09:37:34 DEBUG opendrift:2110: ======================================================================
09:37:34 DEBUG opendrift:2121: 34.83814786868275 <- latitude -> 36.050182043916976
09:37:34 DEBUG opendrift:2121: 22.87172787292356 <- longitude -> 24.218273816109456
09:37:34 DEBUG opendrift:2121: -54.0906496321792 <- z -> 0.0
09:37:34 DEBUG opendrift:2122: ---------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:34 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:34 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:34 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:34 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:34 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 25x39x17) for time after (2025-05-28 00:00:00)
09:37:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 306 elements, expanding data 1
09:37:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.80) and
after (2025-05-28 00:00:00, weight 0.20) in time
09:37:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:34 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:34 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:34 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5179 elements
09:37:34 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:34 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:34 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:34 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:34 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-28 00:00:00)
09:37:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:34 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:34 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:34 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.80) and
after (2025-05-28 00:00:00, weight 0.20) in time
09:37:34 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:34 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:34 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:34 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:34 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:34 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:34 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:34 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.303508 (min) 0.386039 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.442234 (min) 0.283022 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.7753 (min) 9.51939 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.80413 (min) 3.07898 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:34 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:34 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.296812, mean: 1.087888, max: 2.796980
09:37:34 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:34 DEBUG opendrift.models.physics_methods:917: min: 2.968043, mean: 5.535510, max: 9.111172
09:37:34 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.968043, mean: 5.535510, max: 9.111172
09:37:34 DEBUG opendrift:651: 15 elements hit land, moving them to the coastline.
09:37:34 DEBUG opendrift:1729: 15 elements scheduled for deactivation (stranded)
09:37:34 DEBUG opendrift:1731: (z: -9.003619 to 0.000000)
09:37:34 DEBUG opendrift:1749: Removed 15 elements.
09:37:34 DEBUG opendrift:1752: Removed 15 values from environment.
09:37:34 DEBUG opendrift:1757: remove items from profile for z
09:37:34 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:34 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:34 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:34 DEBUG opendrift:1761: Removed 15 values from environment_profiles.
09:37:34 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:34 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:34 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:34 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:34 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:34 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:34 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:34 DEBUG opendrift.models.physics_methods:917: min: 2.968043, mean: 5.540174, max: 9.111172
09:37:34 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:34 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.245283, dN_50: 0.019250
09:37:34 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:34 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0410282819129956
09:37:34 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:34 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:34 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:35 DEBUG opendrift.models.physics_methods:771: Advecting 484 of 5164 elements above 0.100m with wind-sheared ocean current (0.001019 m/s - 0.294897 m/s)
09:37:35 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:35 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0013999542476860775 and 0.41205494808943066 m/s
09:37:35 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:35 DEBUG opendrift:2101: ======================================================================
09:37:35 INFO opendrift:2102: 2025-05-27 22:35:52.207946 - step 110 of 120 - 5164 active elements (836 deactivated)
09:37:35 DEBUG opendrift:2108: 0 elements scheduled.
09:37:35 DEBUG opendrift:2110: ======================================================================
09:37:35 DEBUG opendrift:2121: 34.82531502967947 <- latitude -> 36.03479652785417
09:37:35 DEBUG opendrift:2121: 22.85690491042299 <- longitude -> 24.2342447666497
09:37:35 DEBUG opendrift:2121: -52.92587981161915 <- z -> 0.0
09:37:35 DEBUG opendrift:2122: ---------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.47) and
after (2025-05-28 00:00:00, weight 0.53) in time
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5164 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.47) and
after (2025-05-28 00:00:00, weight 0.53) in time
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:35 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:35 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.379136 (min) 0.37194 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.453996 (min) 0.276592 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.63753 (min) 9.10487 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.62473 (min) 2.73142 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:35 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.259078, mean: 1.070308, max: 2.564937
09:37:35 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:35 DEBUG opendrift.models.physics_methods:917: min: 2.772969, mean: 5.496940, max: 8.725050
09:37:35 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.772969, mean: 5.496940, max: 8.725050
09:37:35 DEBUG opendrift:651: 24 elements hit land, moving them to the coastline.
09:37:35 DEBUG opendrift:1729: 24 elements scheduled for deactivation (stranded)
09:37:35 DEBUG opendrift:1731: (z: -18.505822 to 0.000000)
09:37:35 DEBUG opendrift:1749: Removed 24 elements.
09:37:35 DEBUG opendrift:1752: Removed 24 values from environment.
09:37:35 DEBUG opendrift:1757: remove items from profile for z
09:37:35 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:35 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:35 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:35 DEBUG opendrift:1761: Removed 24 values from environment_profiles.
09:37:35 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:35 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:35 DEBUG opendrift.models.physics_methods:917: min: 2.772969, mean: 5.505768, max: 8.725050
09:37:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.249580, dN_50: 0.019587
09:37:35 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:35 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03762484377696281
09:37:35 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:35 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:35 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:35 DEBUG opendrift.models.physics_methods:771: Advecting 462 of 5140 elements above 0.100m with wind-sheared ocean current (0.009815 m/s - 0.286043 m/s)
09:37:35 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:35 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0012010952116902113 and 0.4382491443784128 m/s
09:37:35 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:35 DEBUG opendrift:2101: ======================================================================
09:37:35 INFO opendrift:2102: 2025-05-27 23:35:52.207946 - step 111 of 120 - 5140 active elements (860 deactivated)
09:37:35 DEBUG opendrift:2108: 0 elements scheduled.
09:37:35 DEBUG opendrift:2110: ======================================================================
09:37:35 DEBUG opendrift:2121: 34.806428657184995 <- latitude -> 36.02740561729336
09:37:35 DEBUG opendrift:2121: 22.847522971764956 <- longitude -> 24.249741696974798
09:37:35 DEBUG opendrift:2121: -51.81508139925767 <- z -> 0.0
09:37:35 DEBUG opendrift:2122: ---------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.13) and
after (2025-05-28 00:00:00, weight 0.87) in time
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5140 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-27 21:00:00 (before)
2025-05-28 00:00:00 (after)
09:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-27 21:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 00:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-27 21:00:00, weight 0.13) and
after (2025-05-28 00:00:00, weight 0.87) in time
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:35 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:35 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:35 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.48439 (min) 0.363216 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.465791 (min) 0.270024 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.52487 (min) 8.72716 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.47605 (min) 2.61203 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:35 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:35 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.228733, mean: 1.055350, max: 2.361518
09:37:35 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:35 DEBUG opendrift.models.physics_methods:917: min: 2.605519, mean: 5.465904, max: 8.371922
09:37:35 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.605519, mean: 5.465904, max: 8.371922
09:37:35 DEBUG opendrift:651: 17 elements hit land, moving them to the coastline.
09:37:35 DEBUG opendrift:1729: 17 elements scheduled for deactivation (stranded)
09:37:35 DEBUG opendrift:1731: (z: -13.702884 to 0.000000)
09:37:35 DEBUG opendrift:1749: Removed 17 elements.
09:37:35 DEBUG opendrift:1752: Removed 17 values from environment.
09:37:35 DEBUG opendrift:1757: remove items from profile for z
09:37:35 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:35 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:35 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:35 DEBUG opendrift:1761: Removed 17 values from environment_profiles.
09:37:35 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:35 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:35 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:35 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:35 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:35 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:35 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:35 DEBUG opendrift.models.physics_methods:917: min: 2.605519, mean: 5.471782, max: 8.371922
09:37:35 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:35 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.253671, dN_50: 0.019908
09:37:35 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:35 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03464123232462752
09:37:35 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:35 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:35 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:35 DEBUG opendrift.models.physics_methods:771: Advecting 453 of 5123 elements above 0.100m with wind-sheared ocean current (0.031327 m/s - 0.276521 m/s)
09:37:35 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:35 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0018752125698434147 and 0.5187697890623044 m/s
09:37:35 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:35 DEBUG opendrift:2101: ======================================================================
09:37:35 INFO opendrift:2102: 2025-05-28 00:35:52.207946 - step 112 of 120 - 5123 active elements (877 deactivated)
09:37:35 DEBUG opendrift:2108: 0 elements scheduled.
09:37:35 DEBUG opendrift:2110: ======================================================================
09:37:35 DEBUG opendrift:2121: 34.79284254568114 <- latitude -> 36.031058562302
09:37:35 DEBUG opendrift:2121: 22.828294047977533 <- longitude -> 24.266535942936898
09:37:35 DEBUG opendrift:2121: -50.86461107505637 <- z -> 0.0
09:37:35 DEBUG opendrift:2122: ---------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:35 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:35 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:35 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:35 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:35 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 27x39x17) for time after (2025-05-28 03:00:00)
09:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:35 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 309 elements, expanding data 1
09:37:35 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.80) and
after (2025-05-28 03:00:00, weight 0.20) in time
09:37:35 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:35 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:35 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:35 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:35 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:35 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5123 elements
09:37:35 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:35 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:36 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:36 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:36 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-28 03:00:00)
09:37:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.80) and
after (2025-05-28 03:00:00, weight 0.20) in time
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:36 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.511576 (min) 0.358848 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.4866 (min) 0.263495 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.52744 (min) 8.41801 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_wind: -4.17908 (min) 2.52398 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:36 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.214768, mean: 0.997415, max: 2.157269
09:37:36 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.524730, mean: 5.318576, max: 8.001690
09:37:36 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.524730, mean: 5.318576, max: 8.001690
09:37:36 DEBUG opendrift:651: 17 elements hit land, moving them to the coastline.
09:37:36 DEBUG opendrift:1729: 17 elements scheduled for deactivation (stranded)
09:37:36 DEBUG opendrift:1731: (z: -13.331647 to 0.000000)
09:37:36 DEBUG opendrift:1749: Removed 17 elements.
09:37:36 DEBUG opendrift:1752: Removed 17 values from environment.
09:37:36 DEBUG opendrift:1757: remove items from profile for z
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:36 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:36 DEBUG opendrift:1761: Removed 17 values from environment_profiles.
09:37:36 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:36 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:36 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:36 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:36 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.524729, mean: 5.323717, max: 8.001690
09:37:36 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:36 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.260730, dN_50: 0.020462
09:37:36 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:36 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.03164546102687807
09:37:36 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:36 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:36 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:36 DEBUG opendrift.models.physics_methods:771: Advecting 439 of 5106 elements above 0.100m with wind-sheared ocean current (0.088642 m/s - 0.263675 m/s)
09:37:36 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:36 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002207139625252141 and 0.43741866769045074 m/s
09:37:36 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:36 DEBUG opendrift:2101: ======================================================================
09:37:36 INFO opendrift:2102: 2025-05-28 01:35:52.207946 - step 113 of 120 - 5106 active elements (894 deactivated)
09:37:36 DEBUG opendrift:2108: 0 elements scheduled.
09:37:36 DEBUG opendrift:2110: ======================================================================
09:37:36 DEBUG opendrift:2121: 34.77170380331912 <- latitude -> 36.03899038460366
09:37:36 DEBUG opendrift:2121: 22.80566497664875 <- longitude -> 24.271014257513663
09:37:36 DEBUG opendrift:2121: -50.029687403427765 <- z -> 0.0
09:37:36 DEBUG opendrift:2122: ---------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.47) and
after (2025-05-28 03:00:00, weight 0.53) in time
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5106 elements
09:37:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.47) and
after (2025-05-28 03:00:00, weight 0.53) in time
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:36 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.4943 (min) 0.370122 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.528192 (min) 0.317672 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.66497 (min) 8.09071 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.83173 (min) 2.44852 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:36 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.217275, mean: 0.913512, max: 1.941772
09:37:36 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.539421, mean: 5.093778, max: 7.591519
09:37:36 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.539421, mean: 5.093778, max: 7.591519
09:37:36 DEBUG opendrift:651: 23 elements hit land, moving them to the coastline.
09:37:36 DEBUG opendrift:1729: 23 elements scheduled for deactivation (stranded)
09:37:36 DEBUG opendrift:1731: (z: -17.820446 to 0.000000)
09:37:36 DEBUG opendrift:1749: Removed 23 elements.
09:37:36 DEBUG opendrift:1752: Removed 23 values from environment.
09:37:36 DEBUG opendrift:1757: remove items from profile for z
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:36 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:36 DEBUG opendrift:1761: Removed 23 values from environment_profiles.
09:37:36 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:36 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:36 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:36 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:36 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.539421, mean: 5.101484, max: 7.591519
09:37:36 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:36 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.270532, dN_50: 0.021231
09:37:36 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:36 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02848470858498377
09:37:36 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:36 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:36 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:36 DEBUG opendrift.models.physics_methods:771: Advecting 428 of 5083 elements above 0.100m with wind-sheared ocean current (0.035305 m/s - 0.249344 m/s)
09:37:36 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:36 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0021311237687104577 and 0.41814980737826907 m/s
09:37:36 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:36 DEBUG opendrift:2101: ======================================================================
09:37:36 INFO opendrift:2102: 2025-05-28 02:35:52.207946 - step 114 of 120 - 5083 active elements (917 deactivated)
09:37:36 DEBUG opendrift:2108: 0 elements scheduled.
09:37:36 DEBUG opendrift:2110: ======================================================================
09:37:36 DEBUG opendrift:2121: 34.75697602921212 <- latitude -> 36.044825133412964
09:37:36 DEBUG opendrift:2121: 22.787768743291483 <- longitude -> 24.27983945442614
09:37:36 DEBUG opendrift:2121: -48.93313165111796 <- z -> 0.0
09:37:36 DEBUG opendrift:2122: ---------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.13) and
after (2025-05-28 03:00:00, weight 0.87) in time
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5083 elements
09:37:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 00:00:00 (before)
2025-05-28 03:00:00 (after)
09:37:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 00:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 03:00:00) in space (linearNDFast)
09:37:36 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:36 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 00:00:00, weight 0.13) and
after (2025-05-28 03:00:00, weight 0.87) in time
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:36 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:36 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.459497 (min) 0.378845 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.561194 (min) 0.295604 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: x_wind: 2.77061 (min) 7.81288 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.8111 (min) 2.37229 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:36 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:36 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.217086, mean: 0.833924, max: 1.748753
09:37:36 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.538316, mean: 4.871793, max: 7.204333
09:37:36 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.538316, mean: 4.871793, max: 7.204333
09:37:36 DEBUG opendrift:651: 18 elements hit land, moving them to the coastline.
09:37:36 DEBUG opendrift:1729: 18 elements scheduled for deactivation (stranded)
09:37:36 DEBUG opendrift:1731: (z: -9.157052 to 0.000000)
09:37:36 DEBUG opendrift:1749: Removed 18 elements.
09:37:36 DEBUG opendrift:1752: Removed 18 values from environment.
09:37:36 DEBUG opendrift:1757: remove items from profile for z
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:36 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:36 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:36 DEBUG opendrift:1761: Removed 18 values from environment_profiles.
09:37:36 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:36 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:36 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:36 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:36 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:36 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:36 DEBUG opendrift.models.physics_methods:917: min: 2.538316, mean: 4.877319, max: 7.204333
09:37:36 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:36 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.280875, dN_50: 0.022043
09:37:36 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:36 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.025653643433425473
09:37:36 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:36 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:36 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:36 DEBUG opendrift.models.physics_methods:771: Advecting 413 of 5065 elements above 0.100m with wind-sheared ocean current (0.013560 m/s - 0.236588 m/s)
09:37:36 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:36 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0023640187606317215 and 0.44205971755489615 m/s
09:37:36 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:36 DEBUG opendrift:2101: ======================================================================
09:37:36 INFO opendrift:2102: 2025-05-28 03:35:52.207946 - step 115 of 120 - 5065 active elements (935 deactivated)
09:37:36 DEBUG opendrift:2108: 0 elements scheduled.
09:37:36 DEBUG opendrift:2110: ======================================================================
09:37:36 DEBUG opendrift:2121: 34.73211130475206 <- latitude -> 36.04517352398928
09:37:36 DEBUG opendrift:2121: 22.768638960191748 <- longitude -> 24.29037963627042
09:37:36 DEBUG opendrift:2121: -47.778175774938994 <- z -> 0.0
09:37:36 DEBUG opendrift:2122: ---------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:36 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:36 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:36 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:36 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:36 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:36 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:36 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5065 elements
09:37:36 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:36 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:37 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:37 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:37 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:37 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 28x41x16) for time after (2025-05-28 06:00:00)
09:37:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 290 elements, expanding data 1
09:37:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.80) and
after (2025-05-28 06:00:00, weight 0.20) in time
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5065 elements
09:37:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5065 elements
09:37:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:37 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:37 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:37 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:37 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-28 06:00:00)
09:37:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.80) and
after (2025-05-28 06:00:00, weight 0.20) in time
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:37 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.39179 (min) 0.391364 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.574191 (min) 0.440809 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.02678 (min) 7.50741 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.66953 (min) 1.87116 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:37 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.235368, mean: 0.778904, max: 1.585842
09:37:37 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:37 DEBUG opendrift.models.physics_methods:917: min: 2.643039, mean: 4.719631, max: 6.860560
09:37:37 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.643039, mean: 4.719631, max: 6.860560
09:37:37 DEBUG opendrift:651: 18 elements hit land, moving them to the coastline.
09:37:37 DEBUG opendrift:1729: 18 elements scheduled for deactivation (stranded)
09:37:37 DEBUG opendrift:1731: (z: -17.139676 to 0.000000)
09:37:37 DEBUG opendrift:1749: Removed 18 elements.
09:37:37 DEBUG opendrift:1752: Removed 18 values from environment.
09:37:37 DEBUG opendrift:1757: remove items from profile for z
09:37:37 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:37 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:37 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:37 DEBUG opendrift:1761: Removed 18 values from environment_profiles.
09:37:37 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:37 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:37 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:37 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:37 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:37 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:37 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:37 DEBUG opendrift.models.physics_methods:917: min: 2.643039, mean: 4.724386, max: 6.860560
09:37:37 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:37 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.287516, dN_50: 0.022564
09:37:37 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:37 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.023264186213743477
09:37:37 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:37 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:37 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:37 DEBUG opendrift.models.physics_methods:771: Advecting 403 of 5047 elements above 0.100m with wind-sheared ocean current (0.064617 m/s - 0.225910 m/s)
09:37:37 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:37 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 3.612533488255077e-05 and 0.4807411837130033 m/s
09:37:37 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:37 DEBUG opendrift:2101: ======================================================================
09:37:37 INFO opendrift:2102: 2025-05-28 04:35:52.207946 - step 116 of 120 - 5047 active elements (953 deactivated)
09:37:37 DEBUG opendrift:2108: 0 elements scheduled.
09:37:37 DEBUG opendrift:2110: ======================================================================
09:37:37 DEBUG opendrift:2121: 34.71704256111963 <- latitude -> 36.042498239263644
09:37:37 DEBUG opendrift:2121: 22.753815777789992 <- longitude -> 24.31391768435703
09:37:37 DEBUG opendrift:2121: -47.007385222375945 <- z -> 0.0
09:37:37 DEBUG opendrift:2122: ---------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:37 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.47) and
after (2025-05-28 06:00:00, weight 0.53) in time
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:37 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:37 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5047 elements
09:37:37 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:37 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:37 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.47) and
after (2025-05-28 06:00:00, weight 0.53) in time
09:37:37 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:37 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:37 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:37 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:37 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:37 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:37 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.314095 (min) 0.415977 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.601227 (min) 0.464301 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.31415 (min) 7.13035 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.44847 (min) 1.10675 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:37 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:37 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.270443, mean: 0.739920, max: 1.439540
09:37:37 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:37 DEBUG opendrift.models.physics_methods:917: min: 2.833139, mean: 4.615167, max: 6.536443
09:37:37 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 2.833139, mean: 4.615167, max: 6.536443
09:37:37 DEBUG opendrift:651: 13 elements hit land, moving them to the coastline.
09:37:37 DEBUG opendrift:1729: 13 elements scheduled for deactivation (stranded)
09:37:37 DEBUG opendrift:1731: (z: -14.585148 to 0.000000)
09:37:37 DEBUG opendrift:1749: Removed 13 elements.
09:37:37 DEBUG opendrift:1752: Removed 13 values from environment.
09:37:37 DEBUG opendrift:1757: remove items from profile for z
09:37:37 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:37 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:37 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:37 DEBUG opendrift:1761: Removed 13 values from environment_profiles.
09:37:37 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:37 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:37 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:37 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:37 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:37 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:37 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:37 DEBUG opendrift.models.physics_methods:917: min: 2.833139, mean: 4.617258, max: 6.536443
09:37:37 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:37 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.291175, dN_50: 0.022851
09:37:37 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:37 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.021118333110853028
09:37:37 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:37 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:37 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:38 DEBUG opendrift.models.physics_methods:771: Advecting 393 of 5034 elements above 0.100m with wind-sheared ocean current (0.023594 m/s - 0.215898 m/s)
09:37:38 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:38 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.001976039338872585 and 0.43092504069097476 m/s
09:37:38 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:38 DEBUG opendrift:2101: ======================================================================
09:37:38 INFO opendrift:2102: 2025-05-28 05:35:52.207946 - step 117 of 120 - 5034 active elements (966 deactivated)
09:37:38 DEBUG opendrift:2108: 0 elements scheduled.
09:37:38 DEBUG opendrift:2110: ======================================================================
09:37:38 DEBUG opendrift:2121: 34.69997630917067 <- latitude -> 36.0486378561852
09:37:38 DEBUG opendrift:2121: 22.749878161607796 <- longitude -> 24.33230750792
09:37:38 DEBUG opendrift:2121: -46.562037113526664 <- z -> 0.0
09:37:38 DEBUG opendrift:2122: ---------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:38 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:38 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:38 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:38 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.13) and
after (2025-05-28 06:00:00, weight 0.87) in time
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5034 elements
09:37:38 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 03:00:00 (before)
2025-05-28 06:00:00 (after)
09:37:38 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 03:00:00) in space (linearNDFast)
09:37:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:38 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 06:00:00) in space (linearNDFast)
09:37:38 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:38 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 03:00:00, weight 0.13) and
after (2025-05-28 06:00:00, weight 0.87) in time
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:38 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:38 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:38 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:38 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:38 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:38 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.245302 (min) 0.449385 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.622685 (min) 0.468079 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.53896 (min) 6.77665 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.24693 (min) 0.526704 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:38 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:38 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.311497, mean: 0.701165, max: 1.310516
09:37:38 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:38 DEBUG opendrift.models.physics_methods:917: min: 3.040578, mean: 4.507104, max: 6.236640
09:37:38 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.040578, mean: 4.507104, max: 6.236640
09:37:38 DEBUG opendrift:651: 16 elements hit land, moving them to the coastline.
09:37:38 DEBUG opendrift:1729: 16 elements scheduled for deactivation (stranded)
09:37:38 DEBUG opendrift:1731: (z: -3.106800 to 0.000000)
09:37:38 DEBUG opendrift:1749: Removed 16 elements.
09:37:38 DEBUG opendrift:1752: Removed 16 values from environment.
09:37:38 DEBUG opendrift:1757: remove items from profile for z
09:37:38 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:38 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:38 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:38 DEBUG opendrift:1761: Removed 16 values from environment_profiles.
09:37:38 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:38 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:38 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:38 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:38 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:38 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:38 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:38 DEBUG opendrift.models.physics_methods:917: min: 3.040578, mean: 4.510136, max: 6.236640
09:37:38 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:38 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.295196, dN_50: 0.023167
09:37:38 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:38 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.01922589591071748
09:37:38 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:38 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:38 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:38 DEBUG opendrift.models.physics_methods:771: Advecting 384 of 5018 elements above 0.100m with wind-sheared ocean current (0.019162 m/s - 0.203766 m/s)
09:37:38 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:38 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0011721243491213272 and 0.4330924311867694 m/s
09:37:38 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:38 DEBUG opendrift:2101: ======================================================================
09:37:38 INFO opendrift:2102: 2025-05-28 06:35:52.207946 - step 118 of 120 - 5018 active elements (982 deactivated)
09:37:38 DEBUG opendrift:2108: 0 elements scheduled.
09:37:38 DEBUG opendrift:2110: ======================================================================
09:37:38 DEBUG opendrift:2121: 34.68355546778845 <- latitude -> 36.06102609657447
09:37:38 DEBUG opendrift:2121: 22.74266011669697 <- longitude -> 24.35588809823576
09:37:38 DEBUG opendrift:2121: -45.55156837576067 <- z -> 0.0
09:37:38 DEBUG opendrift:2122: ---------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:38 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:38 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:38 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:38 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:38 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:38 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:38 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5018 elements
09:37:38 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:38 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_sea_water_velocity to retrieve y_sea_water_velocity
09:37:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_sea_water_velocity to retrieve x_sea_water_velocity
09:37:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking y_sea_water_velocity for invalid values
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking x_sea_water_velocity for invalid values
09:37:39 DEBUG opendrift.readers.interpolation.structured:70: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
09:37:39 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 29x42x16) for time after (2025-05-28 09:00:00)
09:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.interpolation.interpolators:131: Linear2DInterpolator informational: NaN values for 266 elements, expanding data 1
09:37:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.80) and
after (2025-05-28 09:00:00, weight 0.20) in time
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5018 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5018 elements
09:37:39 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using eastward_wind to retrieve x_wind
09:37:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:450: Using northward_wind to retrieve y_wind
09:37:39 DEBUG opendrift.readers.reader_netCDF_CF_generic:572: North is up, no rotation necessary
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking x_wind for invalid values
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking y_wind for invalid values
09:37:39 DEBUG opendrift.readers.basereader.structured:315: Fetched env-block (size 10x9x1) for time after (2025-05-28 09:00:00)
09:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.80) and
after (2025-05-28 09:00:00, weight 0.20) in time
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:39 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.256713 (min) 0.472362 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.632935 (min) 0.449669 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: 3.83168 (min) 6.81504 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: -3.02205 (min) 0.458121 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:39 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.382733, mean: 0.679666, max: 1.300241
09:37:39 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:39 DEBUG opendrift.models.physics_methods:917: min: 3.370370, mean: 4.449430, max: 6.212145
09:37:39 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.370370, mean: 4.449430, max: 6.212145
09:37:39 DEBUG opendrift:651: 16 elements hit land, moving them to the coastline.
09:37:39 DEBUG opendrift:1729: 16 elements scheduled for deactivation (stranded)
09:37:39 DEBUG opendrift:1731: (z: -18.957345 to 0.000000)
09:37:39 DEBUG opendrift:1749: Removed 16 elements.
09:37:39 DEBUG opendrift:1752: Removed 16 values from environment.
09:37:39 DEBUG opendrift:1757: remove items from profile for z
09:37:39 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:39 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:39 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:39 DEBUG opendrift:1761: Removed 16 values from environment_profiles.
09:37:39 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:39 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:39 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:39 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:39 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:39 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:39 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:39 DEBUG opendrift.models.physics_methods:917: min: 3.370370, mean: 4.451663, max: 6.212145
09:37:39 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:39 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.297097, dN_50: 0.023316
09:37:39 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:39 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.019075198794898367
09:37:39 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:39 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:39 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:39 DEBUG opendrift.models.physics_methods:771: Advecting 375 of 5002 elements above 0.100m with wind-sheared ocean current (0.040016 m/s - 0.194948 m/s)
09:37:39 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:39 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.002453358047009446 and 0.45214053123299003 m/s
09:37:39 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:39 DEBUG opendrift:2101: ======================================================================
09:37:39 INFO opendrift:2102: 2025-05-28 07:35:52.207946 - step 119 of 120 - 5002 active elements (998 deactivated)
09:37:39 DEBUG opendrift:2108: 0 elements scheduled.
09:37:39 DEBUG opendrift:2110: ======================================================================
09:37:39 DEBUG opendrift:2121: 34.6716379391911 <- latitude -> 36.0821383922498
09:37:39 DEBUG opendrift:2121: 22.742979687899215 <- longitude -> 24.377477434383884
09:37:39 DEBUG opendrift:2121: -44.15509869180218 <- z -> 0.0
09:37:39 DEBUG opendrift:2122: ---------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.47) and
after (2025-05-28 09:00:00, weight 0.53) in time
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 5002 elements
09:37:39 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:39 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.47) and
after (2025-05-28 09:00:00, weight 0.53) in time
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:39 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:39 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:39 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.292541 (min) 0.486332 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.625006 (min) 0.39547 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: x_wind: 4.1125 (min) 6.9829 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.78486 (min) 0.580742 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:39 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:39 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.423877, mean: 0.671971, max: 1.332397
09:37:39 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:39 DEBUG opendrift.models.physics_methods:917: min: 3.546905, mean: 4.433383, max: 6.288491
09:37:39 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.546905, mean: 4.433383, max: 6.288491
09:37:39 DEBUG opendrift:651: 23 elements hit land, moving them to the coastline.
09:37:39 DEBUG opendrift:1729: 23 elements scheduled for deactivation (stranded)
09:37:39 DEBUG opendrift:1731: (z: -40.233948 to 0.000000)
09:37:39 DEBUG opendrift:1749: Removed 23 elements.
09:37:39 DEBUG opendrift:1752: Removed 23 values from environment.
09:37:39 DEBUG opendrift:1757: remove items from profile for z
09:37:39 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:39 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:39 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:39 DEBUG opendrift:1761: Removed 23 values from environment_profiles.
09:37:39 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:39 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:39 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:39 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:39 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:39 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:39 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:39 DEBUG opendrift.models.physics_methods:917: min: 3.546905, mean: 4.435481, max: 6.288491
09:37:39 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:39 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.297879, dN_50: 0.023378
09:37:39 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:39 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.0195468414391792
09:37:39 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:39 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:39 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:39 DEBUG opendrift.models.physics_methods:771: Advecting 353 of 4979 elements above 0.100m with wind-sheared ocean current (0.124530 m/s - 0.190468 m/s)
09:37:39 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:39 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0015448310732722103 and 0.431388093647129 m/s
09:37:39 DEBUG opendrift:872: to be seeded: 0, already seeded 6000
09:37:39 DEBUG opendrift:2101: ======================================================================
09:37:39 INFO opendrift:2102: 2025-05-28 08:35:52.207946 - step 120 of 120 - 4979 active elements (1021 deactivated)
09:37:39 DEBUG opendrift:2108: 0 elements scheduled.
09:37:39 DEBUG opendrift:2110: ======================================================================
09:37:39 DEBUG opendrift:2121: 34.651844536887424 <- latitude -> 36.09316155168524
09:37:39 DEBUG opendrift:2121: 22.74602581140618 <- longitude -> 24.390091449527265
09:37:39 DEBUG opendrift:2121: -42.89650823052565 <- z -> 0.0
09:37:39 DEBUG opendrift:2122: ---------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['ocean_mixed_layer_thickness']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader constant_reader
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from constant_reader covering 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:39 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:39 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:39 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:39 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity', 'x_sea_water_velocity']
09:37:39 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd
09:37:39 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:39 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://tds.hycom.org/thredds/dodsC/FMRC_ESPC-D-V02_uv3z/FMRC_ESPC-D-V02_uv3z_best.ncd covering 4979 elements
09:37:39 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:39 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.13) and
after (2025-05-28 09:00:00, weight 0.87) in time
09:37:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_wind', 'y_wind']
09:37:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd
09:37:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4979 elements
09:37:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from https://pae-paha.pacioos.hawaii.edu/thredds/dodsC/ncep_global/NCEP_Global_Atmospheric_Model_best.ncd covering 4979 elements
09:37:40 DEBUG opendrift.readers.basereader.structured:213: Shifting coordinates to 0-360
09:37:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-05-28 06:00:00 (before)
2025-05-28 09:00:00 (after)
09:37:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-05-28 06:00:00) in space (linearNDFast)
09:37:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:40 DEBUG opendrift.readers.basereader.structured:342: Interpolating after (2025-05-28 09:00:00) in space (linearNDFast)
09:37:40 DEBUG opendrift.readers.interpolation.structured:97: Initialising interpolator.
09:37:40 DEBUG opendrift.readers.basereader.structured:357: Interpolating before (2025-05-28 06:00:00, weight 0.13) and
after (2025-05-28 09:00:00, weight 0.87) in time
09:37:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:40 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:40 DEBUG opendrift.models.basemodel.environment:782: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
09:37:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 10 for sea_water_temperature for all profiles
09:37:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 34 for sea_water_salinity for all profiles
09:37:40 DEBUG opendrift.models.basemodel.environment:787: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
09:37:40 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:40 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: -0.330113 (min) 0.51048 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.637671 (min) 0.379377 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: x_wind: 4.29215 (min) 7.15506 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: y_wind: -2.59053 (min) 0.705118 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_area_fraction: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_x_velocity: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_ice_y_velocity: 0 (min) 0 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_temperature: 10 (min) 10 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_water_salinity: 34 (min) 34 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 20 (min) 20 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:40 DEBUG opendrift.models.physics_methods:849: Calculating Hs from wind, min: 0.464311, mean: 0.668270, max: 1.368932
09:37:40 DEBUG opendrift.models.physics_methods:907: Calculating wave period Tm02 from wind
09:37:40 DEBUG opendrift.models.physics_methods:917: min: 3.712223, mean: 4.428341, max: 6.374123
09:37:40 DEBUG opendrift.models.physics_methods:857: Calculating wave period from wind, min: 3.712223, mean: 4.428341, max: 6.374123
09:37:40 DEBUG opendrift:651: 22 elements hit land, moving them to the coastline.
09:37:40 DEBUG opendrift:1729: 22 elements scheduled for deactivation (stranded)
09:37:40 DEBUG opendrift:1731: (z: -19.549943 to 0.000000)
09:37:40 DEBUG opendrift:1749: Removed 22 elements.
09:37:40 DEBUG opendrift:1752: Removed 22 values from environment.
09:37:40 DEBUG opendrift:1757: remove items from profile for z
09:37:40 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:40 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:40 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:40 DEBUG opendrift:1761: Removed 22 values from environment_profiles.
09:37:40 DEBUG opendrift:2157: Calling OpenOil.update()
09:37:40 DEBUG opendrift.models.openoil.openoil:711: NOAA oil weathering
09:37:40 DEBUG opendrift.models.openoil.openoil:816: Calculating evaporation - NOAA
09:37:40 DEBUG opendrift.models.openoil.openoil:822: All surface oil elements older than 24 hours, skipping further evaporation.
09:37:40 DEBUG opendrift.models.openoil.openoil:849: Calculating emulsification - NOAA
09:37:40 DEBUG opendrift.models.openoil.openoil:552: Calculating: biodegradation (half_time)
09:37:40 DEBUG opendrift.models.physics_methods:899: Using mean period Tm02 as wave period
09:37:40 DEBUG opendrift.models.physics_methods:917: min: 3.712223, mean: 4.430739, max: 6.374123
09:37:40 DEBUG opendrift.models.openoil.openoil:1121: Generating wave breaking droplet size spectrum
09:37:40 DEBUG opendrift.models.openoil.openoil:1148: Droplet distribution median diameter dV_50: 0.298099, dN_50: 0.023395
09:37:40 DEBUG opendrift.models.oceandrift:427: Using diffusivity from Large1994 since model diffusivities not available
09:37:40 DEBUG opendrift.models.oceandrift:441: Diffusivities are in range 0.0 to 0.02008269786845591
09:37:40 DEBUG opendrift.models.oceandrift:460: TSprofiles deactivated for vertical mixing
09:37:40 DEBUG opendrift.models.oceandrift:474: Vertical mixing module:environment
09:37:40 DEBUG opendrift.models.oceandrift:477: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
09:37:40 DEBUG opendrift.models.physics_methods:771: Advecting 344 of 4957 elements above 0.100m with wind-sheared ocean current (0.083406 m/s - 0.206296 m/s)
09:37:40 DEBUG opendrift.models.physics_methods:789: No Stokes drift velocity available
09:37:40 DEBUG opendrift:1707: Moving elements according to horizontal diffusivity of 20.0, with speeds between 0.0009393975541036834 and 0.44541656513458244 m/s
09:37:40 DEBUG opendrift:2195: Cleaning up
09:37:40 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
09:37:40 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
09:37:40 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4957 elements
09:37:40 DEBUG opendrift.readers.basereader.variables:759: Fetching variables from global_landmask covering 4957 elements
09:37:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
09:37:40 DEBUG opendrift.readers.basereader.variables:637: Checking land_binary_mask for invalid values
09:37:40 DEBUG opendrift.readers.basereader.variables:795: Reader projection is latlon - rotation of vectors is not needed.
09:37:40 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
09:37:40 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
09:37:40 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
09:37:40 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
09:37:40 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
09:37:40 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
09:37:40 DEBUG opendrift:651: 19 elements hit land, moving them to the coastline.
09:37:40 DEBUG opendrift:1729: 19 elements scheduled for deactivation (stranded)
09:37:40 DEBUG opendrift:1731: (z: -9.710895 to 0.000000)
09:37:40 DEBUG opendrift:2281: Updating minval and maxval
09:37:40 DEBUG opendrift:2361: Writing to file
09:37:40 DEBUG opendrift:1749: Removed 19 elements.
09:37:40 DEBUG opendrift:1752: Removed 19 values from environment.
09:37:40 DEBUG opendrift:1757: remove items from profile for z
09:37:40 DEBUG opendrift:1757: remove items from profile for sea_water_temperature
09:37:40 DEBUG opendrift:1757: remove items from profile for sea_water_salinity
09:37:40 DEBUG opendrift:1757: remove items from profile for ocean_vertical_diffusivity
09:37:40 DEBUG opendrift:1761: Removed 19 values from environment_profiles.
09:37:40 DEBUG opendrift:100: Changed mode from Mode.Run to Mode.Result
Plot and animate results
o.animation(color='z', markersize='mass_oil', markersize_scaling=80)
09:37:40 DEBUG opendrift:2428: Setting up map: corners=None, fast=False, lscale=None
09:37:41 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 1) with Cartopy shapereader...
09:38:00 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 5) with Cartopy shapereader...
09:38:00 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 6) with Cartopy shapereader...
09:38:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:01 DEBUG opendrift:3069: Saving animation..
09:38:01 INFO opendrift:4609: Saving animation to /root/project/docs/source/gallery/animations/example_oilspill_seafloor_biodegradation_0.gif...
09:38:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:06 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:06 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:38 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:38 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:44 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:44 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:50 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:50 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:38:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:06 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:06 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:06 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (22.34266090393066, 24.806360244750973, 34.43127822875975, 36.306350708007805)..
09:39:52 DEBUG opendrift:4647: MPLBACKEND = agg
09:39:52 DEBUG opendrift:4648: DISPLAY = None
09:39:52 DEBUG opendrift:4649: Time to save animation: 0:01:51.067173
09:39:52 INFO opendrift:3062: Time to make animation: 0:02:11.757244
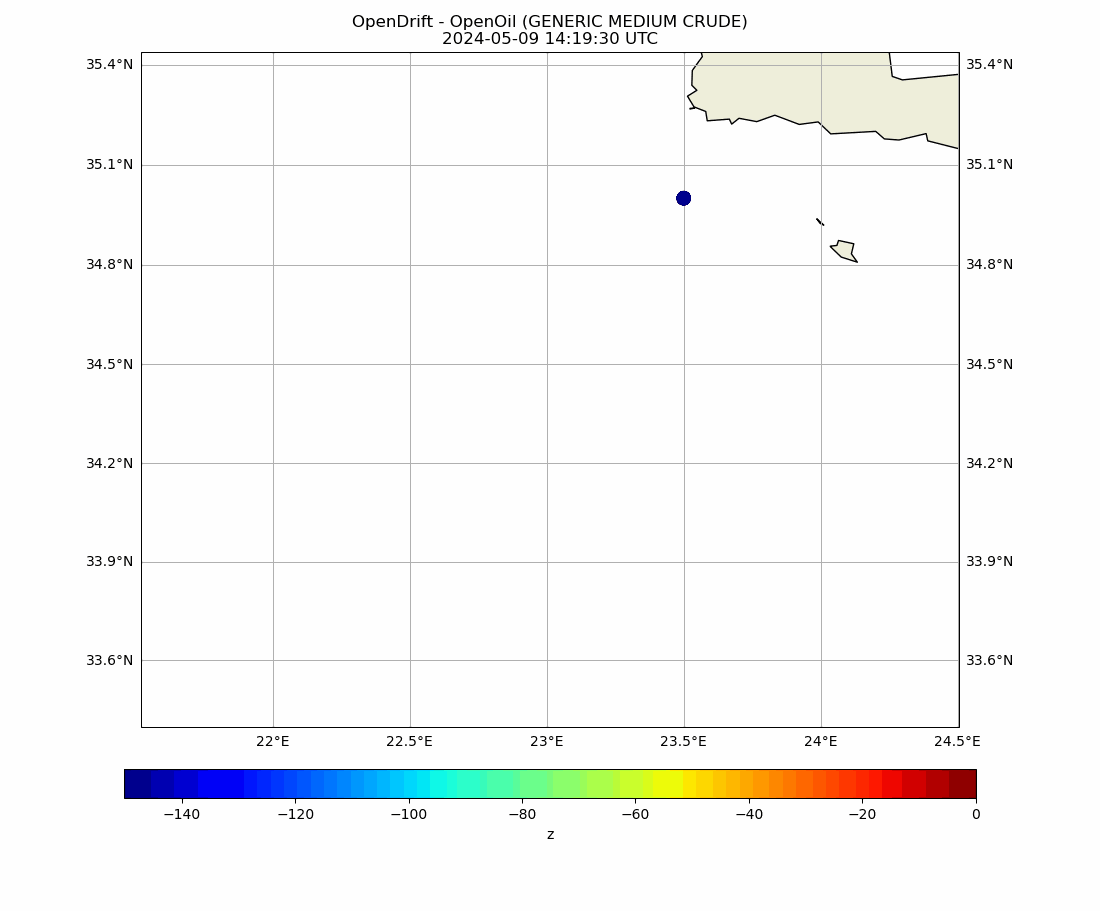
o.plot_oil_budget(show_watercontent_and_viscosity=False, show_wind_and_current=False)
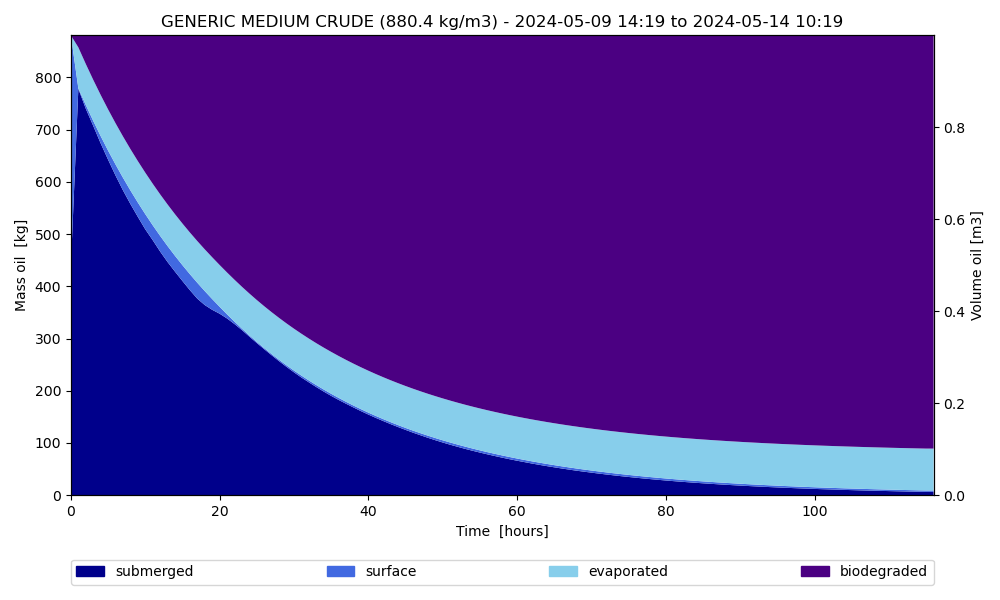
Custom oil budget plot
b = o.get_oil_budget()
import matplotlib.pyplot as plt
time = (o.result.time-o.result.time[0]).dt.total_seconds()/3600 # Hours since start
fig, ax = plt.subplots()
ax.plot(time, b['mass_submerged'], label='Submerged oil mass')
ax.plot(time, b['mass_surface'], label='Surface oil mass')
ax.plot(time, b['mass_biodegraded'], label='Biodegraded oil mass')
ax.set_title(f'{o.get_oil_name()}, {b["oil_density"].max():.2f} kg/m3')
plt.legend()
plt.xlabel('Time [hours]')
plt.ylabel('Mass oil [kg]')
plt.show()
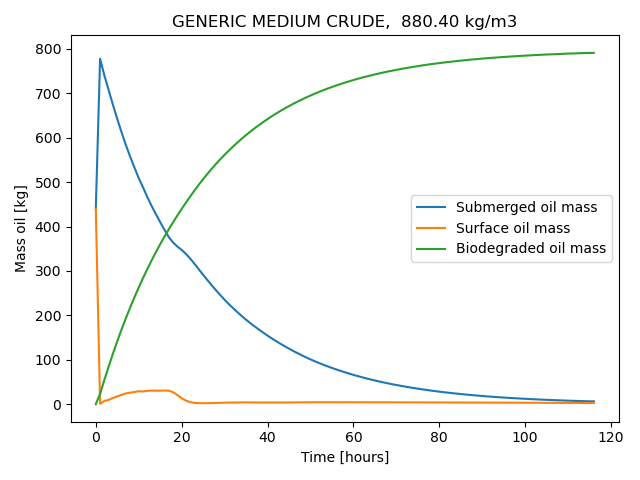
Animation of vertical behaviour
o.animation_profile(markersize='mass_oil', markersize_scaling=80, color='z', alpha=.5)
09:39:52 DEBUG opendrift:3069: Saving animation..
09:39:52 INFO opendrift:4609: Saving animation to /root/project/docs/source/gallery/animations/example_oilspill_seafloor_biodegradation_1.gif...
09:40:14 DEBUG opendrift:4647: MPLBACKEND = agg
09:40:14 DEBUG opendrift:4648: DISPLAY = None
09:40:14 DEBUG opendrift:4649: Time to save animation: 0:00:21.229158
09:40:14 INFO opendrift:3272: Time to make animation: 0:00:21.360983
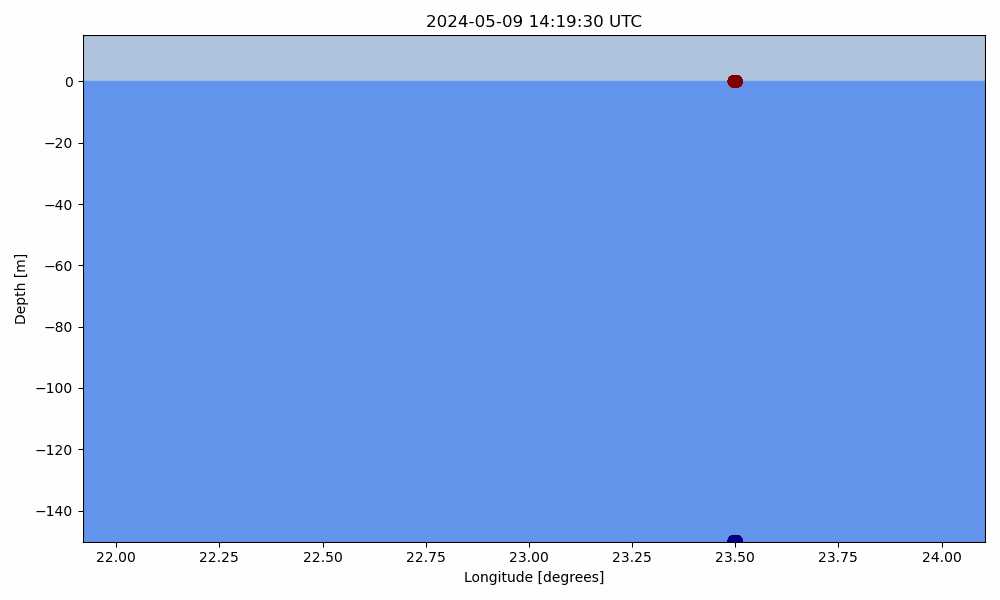
Total running time of the script: (4 minutes 28.987 seconds)