Note
Go to the end to download the full example code.
Biodegradation of oil
import numpy as np
from datetime import datetime, timedelta
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.openoil import OpenOil
o = OpenOil(loglevel=0) # Set loglevel to 0 for debug information
time = datetime.now()
# No motion is needed for this test
o.set_config('environment:constant', {k: 0 for k in
['x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity']})
o.set_config('drift', {'current_uncertainty': 0, 'wind_uncertainty': 0, 'horizontal_diffusivity': 10})
20:13:51 DEBUG opendrift.config:168: Adding 18 config items from __init__
20:13:51 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
20:13:51 DEBUG opendrift.config:168: Adding 6 config items from __init__
20:13:51 INFO opendrift.models.basemodel:515: OpenDriftSimulation initialised (version 1.12.0 / v1.12.0-26-g390e945)
20:13:51 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
20:13:51 DEBUG opendrift.config:178: Overwriting config item seed:z
20:13:51 DEBUG opendrift.config:168: Adding 15 config items from openoil
20:13:51 INFO opendrift.config:68: set_config('environment:constant:x_wind', 0)
20:13:51 INFO opendrift.config:68: set_config('environment:constant:y_wind', 0)
20:13:51 INFO opendrift.config:68: set_config('environment:constant:x_sea_water_velocity', 0)
20:13:51 INFO opendrift.config:68: set_config('environment:constant:y_sea_water_velocity', 0)
20:13:51 INFO opendrift.config:68: set_config('drift:current_uncertainty', 0)
20:13:51 INFO opendrift.config:68: set_config('drift:wind_uncertainty', 0)
20:13:51 INFO opendrift.config:68: set_config('drift:horizontal_diffusivity', 10)
Seeding some particles
o.set_config('drift:vertical_mixing', True)
o.set_config('processes:biodegradation', True)
o.set_config('biodegradation:method', 'half_time')
Fast decay for droplets, and slow decay for slick
kwargs = {'biodegradation_half_time_slick': 3, # days
'biodegradation_half_time_droplet': 1, # days
'oil_type': 'GENERIC MEDIUM CRUDE', 'm3_per_hour': .5, 'diameter': 1e-5} # small droplets
Seed 500 oil elements at surface, and 500 elements at 50m depth
o.seed_elements(lon=4, lat=60.0, z=0, number=500, time=datetime.now(), **kwargs)
o.seed_elements(lon=4, lat=60.0, z=-50, number=500, time=datetime.now(), **kwargs)
20:13:51 INFO opendrift.models.openoil.openoil:1634: Droplet diameter is provided, and will be kept constant during simulation
20:13:51 INFO opendrift.models.openoil.adios.dirjs:86: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
20:13:51 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
20:13:51 INFO opendrift.models.openoil.openoil:1715: Using density 877.5726099999999 and viscosity 4.5431401718650355e-05 of oiltype GENERIC MEDIUM CRUDE
20:13:51 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
20:13:51 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
20:13:51 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
20:13:51 DEBUG opendrift.models.basemodel.environment:328: Added reader constant_reader
20:13:51 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 1.3 m/s. Adding a customised landmask may be faster...
20:13:51 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
20:13:54 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
20:13:54 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_height: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: upward_sea_water_velocity: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_ice_area_fraction: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_ice_x_velocity: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_ice_y_velocity: 0.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_water_temperature: 10.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_water_salinity: 34.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: sea_floor_depth_below_sea_level: 10000.000000
20:13:54 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.020000
20:13:54 INFO opendrift.models.basemodel.environment:248: ocean_mixed_layer_thickness: 50.000000
20:13:54 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Config to Mode.Ready
20:13:54 INFO opendrift.models.openoil.openoil:1634: Droplet diameter is provided, and will be kept constant during simulation
20:13:54 INFO opendrift.models.openoil.adios.dirjs:86: Querying ADIOS database for oil: GENERIC MEDIUM CRUDE
20:13:54 DEBUG opendrift.models.openoil.adios.oil:76: Parsing Oil: AD04001 / GENERIC MEDIUM CRUDE
20:13:54 INFO opendrift.models.openoil.openoil:1715: Using density 877.5726099999999 and viscosity 4.5431401718650355e-05 of oiltype GENERIC MEDIUM CRUDE
Running model
o.run(duration=timedelta(hours=72), time_step=3600, outfile='oil.nc')
20:13:54 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Ready to Mode.Run
20:13:55 DEBUG opendrift.models.basemodel:1777:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.12.0
Platform: Linux, 5.15.0-1057-aws
69.05992889404297 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.11.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 1.3.5
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
20:13:55 DEBUG opendrift.models.basemodel:1909: Finalizing environment and preparing readers for simulation coverage ([-2.071351713232114, 56.96432432432432, 10.071351713232115, 63.03567567567568]) and time (2024-12-12 20:13:51.671343 to 2024-12-15 20:13:51.671343)
20:13:55 DEBUG opendrift.models.basemodel.environment:180: Preparing constant_reader for extent [-2.071351713232114, 56.96432432432432, 10.071351713232115, 63.03567567567568]
20:13:55 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [-2.071351713232114, 56.96432432432432, 10.071351713232115, 63.03567567567568]
20:13:55 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
20:13:55 INFO opendrift.models.basemodel:935: Using existing reader for land_binary_mask
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 INFO opendrift.models.basemodel:946: All points are in ocean
20:13:55 INFO opendrift.models.openoil.openoil:684: Oil-water surface tension is 0.031369 Nm
20:13:55 INFO opendrift.models.openoil.openoil:697: Max water fraction not available for GENERIC MEDIUM CRUDE, using default
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 1000, already seeded 0
20:13:55 DEBUG opendrift.models.basemodel:908: Released 1000 new elements.
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-12 20:13:51.671343 - step 1 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2052: latitude = 60.0
20:13:55 DEBUG opendrift.models.basemodel:2057: longitude = 4.0
20:13:55 DEBUG opendrift.models.basemodel:2064: -50.0 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:55 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:55 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:55 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:55 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
/root/project/opendrift/models/openoil/openoil.py:1134: RuntimeWarning: divide by zero encountered in power
dN_50 = (A * self.elements.oil_film_thickness * we**-0.6) + (
/root/project/opendrift/models/openoil/openoil.py:1135: RuntimeWarning: divide by zero encountered in power
B * self.elements.oil_film_thickness * re**-0.6)
20:13:55 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
/root/project/opendrift/models/openoil/openoil.py:1152: RuntimeWarning: invalid value encountered in divide
self.droplet_spectrum_pdf = spectrum / np.sum(spectrum)
20:13:55 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:55 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:55 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:55 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:55 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:55 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:55 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:55 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.005230950457444111 and 0.2866440990165205 m/s
20:13:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-12 21:13:51.671343 - step 2 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2054: 59.99300559059122 <- latitude -> 60.00925996553464
20:13:55 DEBUG opendrift.models.basemodel:2059: 3.982689631663062 <- longitude -> 4.016277231133427
20:13:55 DEBUG opendrift.models.basemodel:2064: -51.00964196365002 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:55 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:55 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:55 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:55 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:55 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:55 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:55 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:55 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:55 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:55 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:55 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:55 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:55 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036361161308154795 and 0.28366168985017537 m/s
20:13:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-12 22:13:51.671343 - step 3 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2054: 59.990898010763814 <- latitude -> 60.010070873438494
20:13:55 DEBUG opendrift.models.basemodel:2059: 3.9753494955027637 <- longitude -> 4.02853504361713
20:13:55 DEBUG opendrift.models.basemodel:2064: -51.04946722829375 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:55 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:55 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:55 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:55 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:55 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:55 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:55 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:55 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:55 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:55 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:55 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:55 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:55 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0034401185184955384 and 0.3102105653225473 m/s
20:13:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-12 23:13:51.671343 - step 4 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2054: 59.98600372795558 <- latitude -> 60.01479617288673
20:13:55 DEBUG opendrift.models.basemodel:2059: 3.9753853523805014 <- longitude -> 4.028573218792662
20:13:55 DEBUG opendrift.models.basemodel:2064: -51.434883603088664 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:55 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:55 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:55 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:55 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:55 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:55 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:55 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:55 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:55 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:55 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:55 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:55 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:55 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004201980796857228 and 0.27556120226147685 m/s
20:13:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-13 00:13:51.671343 - step 5 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2054: 59.9844101374394 <- latitude -> 60.014066158114424
20:13:55 DEBUG opendrift.models.basemodel:2059: 3.9722295759831976 <- longitude -> 4.03186780557294
20:13:55 DEBUG opendrift.models.basemodel:2064: -51.6836299797262 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:55 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:55 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:55 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:55 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:55 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:55 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:55 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:55 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:55 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:55 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:55 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:55 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:55 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:55 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:55 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:55 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0025847043089351094 and 0.28448278396433224 m/s
20:13:55 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:55 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:55 INFO opendrift.models.basemodel:2035: 2024-12-13 01:13:51.671343 - step 6 of 72 - 1000 active elements (0 deactivated)
20:13:55 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:55 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:55 DEBUG opendrift.models.basemodel:2054: 59.981783784070856 <- latitude -> 60.0182670614452
20:13:55 DEBUG opendrift.models.basemodel:2059: 3.9704418854766645 <- longitude -> 4.035712451412111
20:13:55 DEBUG opendrift.models.basemodel:2064: -52.04347041355175 <- z -> 0.0
20:13:55 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:55 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:55 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004774221774378705 and 0.2689882721199039 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 02:13:51.671343 - step 7 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.98110651443229 <- latitude -> 60.01881467423323
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9677675573782962 <- longitude -> 4.038942193959054
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.42404289079638 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0035847303639862144 and 0.3056118354589426 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 03:13:51.671343 - step 8 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.978852265964186 <- latitude -> 60.01970878895446
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.963080250971123 <- longitude -> 4.039595307039366
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.442220302016246 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011964156096968845 and 0.26977947087144943 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 04:13:51.671343 - step 9 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97779113982597 <- latitude -> 60.0236382934134
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.961622425653881 <- longitude -> 4.0418680044316
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.43935828453126 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004006411553231905 and 0.3276092985581127 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 05:13:51.671343 - step 10 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97645366690813 <- latitude -> 60.02659329100814
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.956886605939512 <- longitude -> 4.048665160174358
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.37346668006803 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019485125713325482 and 0.2860428608074356 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 06:13:51.671343 - step 11 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97544309889227 <- latitude -> 60.02583876391965
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9556931052810542 <- longitude -> 4.049234918758788
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.739235905328904 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020135251405121496 and 0.3089059368070698 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 07:13:51.671343 - step 12 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97478695596433 <- latitude -> 60.02603812606495
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9475855739402905 <- longitude -> 4.04766487128353
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.57059540085608 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012765399476683675 and 0.30559783681668884 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 08:13:51.671343 - step 13 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97517996339138 <- latitude -> 60.029461011924965
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9420558179709992 <- longitude -> 4.047986971562519
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.27159809115201 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002059811685033994 and 0.2982353539985148 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 09:13:51.671343 - step 14 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.972607663708374 <- latitude -> 60.029101942076664
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.945470664649419 <- longitude -> 4.051718678999692
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.583567846979335 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.005937776690819647 and 0.257183021365017 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 10:13:51.671343 - step 15 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.96939250992013 <- latitude -> 60.03234450871624
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.935415707861256 <- longitude -> 4.052607292686077
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.825560779586084 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0015273460078467074 and 0.27244834032549003 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 11:13:51.671343 - step 16 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.97072506965472 <- latitude -> 60.03325698026822
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9300446658540147 <- longitude -> 4.05404998175444
20:13:56 DEBUG opendrift.models.basemodel:2064: -53.01267150779023 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004114681201002062 and 0.33516775031858936 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 12:13:51.671343 - step 17 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.970155432489335 <- latitude -> 60.031068240014434
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.9274576488982302 <- longitude -> 4.055552672612285
20:13:56 DEBUG opendrift.models.basemodel:2064: -53.272470953345255 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:56 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:56 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:56 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:56 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:56 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:56 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:56 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:56 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011444925579211515 and 0.2956755822676069 m/s
20:13:56 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:56 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:56 INFO opendrift.models.basemodel:2035: 2024-12-13 13:13:51.671343 - step 18 of 72 - 1000 active elements (0 deactivated)
20:13:56 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:56 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:56 DEBUG opendrift.models.basemodel:2054: 59.96793764654285 <- latitude -> 60.034098714178874
20:13:56 DEBUG opendrift.models.basemodel:2059: 3.93639364255031 <- longitude -> 4.064747047667295
20:13:56 DEBUG opendrift.models.basemodel:2064: -52.899755583201134 <- z -> 0.0
20:13:56 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:56 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:56 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:56 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:56 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:56 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:56 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:56 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:56 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:56 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:56 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.006649856567931005 and 0.3229478526317954 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 14:13:51.671343 - step 19 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.9672947453065 <- latitude -> 60.038931846150845
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.9285066458051388 <- longitude -> 4.0698569955974735
20:13:57 DEBUG opendrift.models.basemodel:2064: -53.04017757995296 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0035649539960791207 and 0.2595320001259624 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 15:13:51.671343 - step 20 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.96695777075216 <- latitude -> 60.0390749759093
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.9279796752273906 <- longitude -> 4.072198423061733
20:13:57 DEBUG opendrift.models.basemodel:2064: -53.3265079005366 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00236571905300566 and 0.2630974789449512 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 16:13:51.671343 - step 21 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.9661355377691 <- latitude -> 60.03724203472615
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.9279848392960206 <- longitude -> 4.0793186538859905
20:13:57 DEBUG opendrift.models.basemodel:2064: -53.361635210246504 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012743434507719463 and 0.28589506826988104 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 17:13:51.671343 - step 22 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.966626996337595 <- latitude -> 60.035613960764564
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.929373727176326 <- longitude -> 4.082307019210956
20:13:57 DEBUG opendrift.models.basemodel:2064: -53.53051172610319 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003727588349098927 and 0.24873814711621203 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 18:13:51.671343 - step 23 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.96460253144221 <- latitude -> 60.035699506322416
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.92638366893989 <- longitude -> 4.078496511394482
20:13:57 DEBUG opendrift.models.basemodel:2064: -53.76093859201668 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0022342131125827696 and 0.3281579705479143 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 19:13:51.671343 - step 24 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.963810337160545 <- latitude -> 60.03410114183104
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.9298088266988556 <- longitude -> 4.080750498688261
20:13:57 DEBUG opendrift.models.basemodel:2064: -54.1178551172757 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004604736111769178 and 0.25753481033194786 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 20:13:51.671343 - step 25 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.959897726536 <- latitude -> 60.036116888865784
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.9262512642683727 <- longitude -> 4.082343136234759
20:13:57 DEBUG opendrift.models.basemodel:2064: -54.700899921319696 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003488462737987466 and 0.2305449379154643 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 21:13:51.671343 - step 26 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.962031422365634 <- latitude -> 60.03438190231284
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.929111574398975 <- longitude -> 4.088440478247428
20:13:57 DEBUG opendrift.models.basemodel:2064: -54.66348835964327 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020961820415778525 and 0.34616158110438694 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 22:13:51.671343 - step 27 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.961386335310834 <- latitude -> 60.0340709889864
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.928137560550945 <- longitude -> 4.094337845668902
20:13:57 DEBUG opendrift.models.basemodel:2064: -54.81680482210812 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0030229535139614993 and 0.2944213874904817 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-13 23:13:51.671343 - step 28 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.960056820518 <- latitude -> 60.03524187155291
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.923007024880261 <- longitude -> 4.090091246809056
20:13:57 DEBUG opendrift.models.basemodel:2064: -55.100302365783726 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003842990509628418 and 0.2616604311767797 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-14 00:13:51.671343 - step 29 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.95740804758019 <- latitude -> 60.03539962168136
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.918372279772955 <- longitude -> 4.088506944840047
20:13:57 DEBUG opendrift.models.basemodel:2064: -55.6833239553789 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0029360090514733775 and 0.3095914832428939 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-14 01:13:51.671343 - step 30 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.95525223588288 <- latitude -> 60.03750564643475
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.919218151405371 <- longitude -> 4.087904210061142
20:13:57 DEBUG opendrift.models.basemodel:2064: -55.1960905985658 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:57 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:57 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:57 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:57 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:57 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:57 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:57 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:57 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004151034838430559 and 0.28651972101405737 m/s
20:13:57 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:57 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:57 INFO opendrift.models.basemodel:2035: 2024-12-14 02:13:51.671343 - step 31 of 72 - 1000 active elements (0 deactivated)
20:13:57 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:57 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:57 DEBUG opendrift.models.basemodel:2054: 59.950471399768794 <- latitude -> 60.036961146801914
20:13:57 DEBUG opendrift.models.basemodel:2059: 3.920428492873537 <- longitude -> 4.086329553300678
20:13:57 DEBUG opendrift.models.basemodel:2064: -55.068766597345174 <- z -> 0.0
20:13:57 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:57 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:57 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:57 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:57 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:57 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:57 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:57 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:57 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:57 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:57 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:57 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:57 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.007153335429492487 and 0.30057830421343756 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 03:13:51.671343 - step 32 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.952218306194624 <- latitude -> 60.03812913679167
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.91621180072712 <- longitude -> 4.094181329906333
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.421502579988264 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018532850472968458 and 0.26099714995507445 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 04:13:51.671343 - step 33 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.950083614633215 <- latitude -> 60.04142914865003
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.913027602258684 <- longitude -> 4.104302218384725
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.535435871336034 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002727888887553324 and 0.3174852355538081 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 05:13:51.671343 - step 34 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.948130373473504 <- latitude -> 60.04110296195665
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9086170538112994 <- longitude -> 4.097883842996593
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.506615609738894 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00256401965931058 and 0.26326231414048723 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 06:13:51.671343 - step 35 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.95253465166398 <- latitude -> 60.04088531223651
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9144989014047527 <- longitude -> 4.09671739450217
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.50180724518627 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0028884253438362655 and 0.3715165954838363 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 07:13:51.671343 - step 36 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94771808045831 <- latitude -> 60.0400585579502
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9123987822437982 <- longitude -> 4.098979455006863
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.738074915557476 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0014790240697702172 and 0.2861933180158605 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 08:13:51.671343 - step 37 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.945644360149174 <- latitude -> 60.04052369836959
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.919965352905072 <- longitude -> 4.105180729777046
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.81740445900707 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002256607881934403 and 0.2815146703693781 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 09:13:51.671343 - step 38 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94346559629129 <- latitude -> 60.04292417713012
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9216192494914113 <- longitude -> 4.10823571261794
20:13:58 DEBUG opendrift.models.basemodel:2064: -56.026191696515475 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004301153980563228 and 0.2640843019497672 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 10:13:51.671343 - step 39 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.942004971800756 <- latitude -> 60.04322730019343
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9184612867947894 <- longitude -> 4.110144249434244
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.83318868929813 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036562243431624624 and 0.29216894384377906 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 11:13:51.671343 - step 40 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94481163029247 <- latitude -> 60.04517090017265
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9154846949642255 <- longitude -> 4.116897942609766
20:13:58 DEBUG opendrift.models.basemodel:2064: -55.96130069308527 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020428633670991963 and 0.2764383763645849 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 12:13:51.671343 - step 41 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94732990428363 <- latitude -> 60.045454264846285
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.913011806222297 <- longitude -> 4.12849541175674
20:13:58 DEBUG opendrift.models.basemodel:2064: -56.49542500399992 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00328709556680579 and 0.2959985814367729 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 13:13:51.671343 - step 42 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94551108983491 <- latitude -> 60.04488019526633
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.917697197409926 <- longitude -> 4.135588208739135
20:13:58 DEBUG opendrift.models.basemodel:2064: -56.44677114324868 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0035557575117498193 and 0.31795621567325416 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 14:13:51.671343 - step 43 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.94608472307493 <- latitude -> 60.04578196088521
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.914929537821032 <- longitude -> 4.133370062318652
20:13:58 DEBUG opendrift.models.basemodel:2064: -56.11034916124547 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:58 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:58 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001991822914842502 and 0.28683880335506023 m/s
20:13:58 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:58 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:58 INFO opendrift.models.basemodel:2035: 2024-12-14 15:13:51.671343 - step 44 of 72 - 1000 active elements (0 deactivated)
20:13:58 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:58 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:58 DEBUG opendrift.models.basemodel:2054: 59.949461445469794 <- latitude -> 60.0444480183521
20:13:58 DEBUG opendrift.models.basemodel:2059: 3.9151350308945405 <- longitude -> 4.128939823842959
20:13:58 DEBUG opendrift.models.basemodel:2064: -56.17525673295478 <- z -> 0.0
20:13:58 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:58 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:58 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:58 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:58 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:58 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:58 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:58 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:58 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:58 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:58 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:58 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:58 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:58 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:58 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:58 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:58 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:58 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004917165419061945 and 0.25159650008116047 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 16:13:51.671343 - step 45 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.951198405020165 <- latitude -> 60.045376774138546
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.915885331129562 <- longitude -> 4.128035087883264
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.92063328349611 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018538313404115592 and 0.28773947227876795 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 17:13:51.671343 - step 46 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.95097449163339 <- latitude -> 60.04643173223404
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.9096927721811077 <- longitude -> 4.129453060724575
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.093964108069706 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0029300486850991087 and 0.25681762616474624 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 18:13:51.671343 - step 47 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.95170192066117 <- latitude -> 60.04552358509092
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.903704492493362 <- longitude -> 4.1311007806549505
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.10553965879445 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012124780607103785 and 0.2315688691386235 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 19:13:51.671343 - step 48 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.95224417680786 <- latitude -> 60.04992175988092
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.9003474769956505 <- longitude -> 4.126903099511586
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.26195402883567 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004828536801733015 and 0.2754519518330615 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 20:13:51.671343 - step 49 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.95067029549343 <- latitude -> 60.04858906537044
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.897464989597345 <- longitude -> 4.126479585013719
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.946413881547066 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004925027855710996 and 0.2994884349519409 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 21:13:51.671343 - step 50 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.95207160594187 <- latitude -> 60.048105483417075
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.8937425913721064 <- longitude -> 4.131269195819437
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.33209629377252 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004804355228721794 and 0.26770718739517746 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 22:13:51.671343 - step 51 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.94906065140323 <- latitude -> 60.04638213759712
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.896763672660848 <- longitude -> 4.1293241105112495
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.91383475680791 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003450828734166133 and 0.2502093530992484 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-14 23:13:51.671343 - step 52 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.94680925903316 <- latitude -> 60.04775397432766
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.893686824895136 <- longitude -> 4.122584977736881
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.70863348263968 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018308891472327967 and 0.25443065306797136 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 00:13:51.671343 - step 53 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.947691667339626 <- latitude -> 60.04970383577723
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.888200896350141 <- longitude -> 4.12711426460727
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.021641703528026 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.005352573730319705 and 0.2745514794709536 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 01:13:51.671343 - step 54 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.948862190316106 <- latitude -> 60.049137470121366
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.895695358982515 <- longitude -> 4.129241850624524
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.69567926001158 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0035909213407049998 and 0.25877219503842386 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 02:13:51.671343 - step 55 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.94916318194835 <- latitude -> 60.04685232130481
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.8922013121835164 <- longitude -> 4.131603333437765
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.67437743630292 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0029312124265568123 and 0.31761704412750213 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 03:13:51.671343 - step 56 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.948935025243784 <- latitude -> 60.04885377634836
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.8922160703282334 <- longitude -> 4.132373750741957
20:13:59 DEBUG opendrift.models.basemodel:2064: -55.92310177544925 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003174531945168303 and 0.26511110847956676 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 04:13:51.671343 - step 57 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.949481453543854 <- latitude -> 60.047613400417944
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.8817841687922243 <- longitude -> 4.131086452259178
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.113276705163344 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:13:59 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036475179711561515 and 0.3105620923694763 m/s
20:13:59 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:13:59 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:13:59 INFO opendrift.models.basemodel:2035: 2024-12-15 05:13:51.671343 - step 58 of 72 - 1000 active elements (0 deactivated)
20:13:59 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:13:59 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:13:59 DEBUG opendrift.models.basemodel:2054: 59.94919325448255 <- latitude -> 60.05016756633955
20:13:59 DEBUG opendrift.models.basemodel:2059: 3.879186036374204 <- longitude -> 4.132363518940143
20:13:59 DEBUG opendrift.models.basemodel:2064: -56.456090293645765 <- z -> 0.0
20:13:59 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:13:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:13:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:13:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:13:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:13:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:13:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:13:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:13:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:13:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:13:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:13:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:13:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:13:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:13:59 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:13:59 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:13:59 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:13:59 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:13:59 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:13:59 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:13:59 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:13:59 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:13:59 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:13:59 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:13:59 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:13:59 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:13:59 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:13:59 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:13:59 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:13:59 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:13:59 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:13:59 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:13:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002946543054548501 and 0.3542742290295432 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 06:13:51.671343 - step 59 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.94828032028701 <- latitude -> 60.05061098814609
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8816356249250474 <- longitude -> 4.1399544947787925
20:14:00 DEBUG opendrift.models.basemodel:2064: -56.241443739962506 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.005611414469381687 and 0.33202694609320665 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 07:13:51.671343 - step 60 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.946289508136815 <- latitude -> 60.05065753842932
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8861641338414143 <- longitude -> 4.140795204641966
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.798827220092036 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0017543458465601324 and 0.2725048787199033 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 08:13:51.671343 - step 61 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.94425125596885 <- latitude -> 60.05192238352293
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8830886572005054 <- longitude -> 4.138350219566734
20:14:00 DEBUG opendrift.models.basemodel:2064: -56.039250349439335 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0034568580903300275 and 0.3183000735653997 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 09:13:51.671343 - step 62 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.94073358522347 <- latitude -> 60.05238322115588
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8832107048288496 <- longitude -> 4.140412486727274
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.92468643921042 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.004471727007293897 and 0.25717382889182455 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 10:13:51.671343 - step 63 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.93959685502028 <- latitude -> 60.0530503372714
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8769636528324214 <- longitude -> 4.136913286265729
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.96802880196617 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0008122463795154521 and 0.3390488213336253 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 11:13:51.671343 - step 64 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.942805375067486 <- latitude -> 60.05396151594777
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.87541960630896 <- longitude -> 4.14049950069255
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.845680459260215 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001858112780260192 and 0.2966621066566791 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 12:13:51.671343 - step 65 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.93931696674423 <- latitude -> 60.05961886624401
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8748863948368597 <- longitude -> 4.138701275243619
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.997440924459575 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0052621564138150105 and 0.3506362914195378 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 13:13:51.671343 - step 66 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.93331288324317 <- latitude -> 60.06117929934075
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8737532073966157 <- longitude -> 4.142852313255104
20:14:00 DEBUG opendrift.models.basemodel:2064: -56.18453801858221 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003212913582357832 and 0.2509205667444445 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 14:13:51.671343 - step 67 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.9356094741595 <- latitude -> 60.061961780080104
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.873996713779828 <- longitude -> 4.1397976097744404
20:14:00 DEBUG opendrift.models.basemodel:2064: -56.06655409247274 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0062745678240689684 and 0.29606592790166275 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 15:13:51.671343 - step 68 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.935971689124166 <- latitude -> 60.057843307700104
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8728564436767687 <- longitude -> 4.1392306875437
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.677279776468346 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003551649019788776 and 0.2855261245147178 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 16:13:51.671343 - step 69 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.93580861701865 <- latitude -> 60.05690567211515
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8649850952904212 <- longitude -> 4.148407351421344
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.731104446874575 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.007942977859339033 and 0.290253471472653 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 17:13:51.671343 - step 70 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.93142214962182 <- latitude -> 60.055687416727864
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8561075156101032 <- longitude -> 4.1441925419966354
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.585150435848774 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:00 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:00 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:00 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:00 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:00 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:00 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0009084255812054989 and 0.24832514731044186 m/s
20:14:00 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:00 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:00 INFO opendrift.models.basemodel:2035: 2024-12-15 18:13:51.671343 - step 71 of 72 - 1000 active elements (0 deactivated)
20:14:00 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:00 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:00 DEBUG opendrift.models.basemodel:2054: 59.932621524134476 <- latitude -> 60.058379537997936
20:14:00 DEBUG opendrift.models.basemodel:2059: 3.8608397455519143 <- longitude -> 4.152738755257064
20:14:00 DEBUG opendrift.models.basemodel:2064: -55.94488350503279 <- z -> 0.0
20:14:00 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:00 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:00 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:00 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:00 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:00 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:00 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:00 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:00 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:00 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:00 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:00 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:00 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:00 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:01 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:01 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:01 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:01 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:01 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:01 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003734647863041959 and 0.25379930575195786 m/s
20:14:01 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:01 DEBUG opendrift.models.basemodel:890: to be seeded: 0, already seeded 1000
20:14:01 DEBUG opendrift.models.basemodel:2034: ======================================================================
20:14:01 INFO opendrift.models.basemodel:2035: 2024-12-15 19:13:51.671343 - step 72 of 72 - 1000 active elements (0 deactivated)
20:14:01 DEBUG opendrift.models.basemodel:2041: 0 elements scheduled.
20:14:01 DEBUG opendrift.models.basemodel:2043: ======================================================================
20:14:01 DEBUG opendrift.models.basemodel:2054: 59.93033644036061 <- latitude -> 60.05580588768059
20:14:01 DEBUG opendrift.models.basemodel:2059: 3.8512436388661357 <- longitude -> 4.151503364713611
20:14:01 DEBUG opendrift.models.basemodel:2064: -56.19892765103577 <- z -> 0.0
20:14:01 DEBUG opendrift.models.basemodel:2065: ---------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['x_sea_water_velocity', 'y_sea_water_velocity', 'x_wind', 'y_wind']
20:14:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
20:14:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:01 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['sea_water_temperature', 'sea_water_salinity', 'ocean_vertical_diffusivity']
20:14:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 10 for sea_water_temperature for all profiles
20:14:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 34 for sea_water_salinity for all profiles
20:14:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.02 for ocean_vertical_diffusivity for all profiles
20:14:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_ice_area_fraction: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_ice_x_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_ice_y_velocity: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 10 (min) 10 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 34 (min) 34 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.02 (min) 0.02 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
20:14:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
20:14:01 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
20:14:01 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:01 DEBUG opendrift.models.basemodel:2108: Calling OpenOil.update()
20:14:01 DEBUG opendrift.models.openoil.openoil:708: NOAA oil weathering
20:14:01 DEBUG opendrift.models.openoil.openoil:813: Calculating evaporation - NOAA
20:14:01 DEBUG opendrift.models.openoil.openoil:819: All surface oil elements older than 24 hours, skipping further evaporation.
20:14:01 DEBUG opendrift.models.openoil.openoil:846: Calculating emulsification - NOAA
20:14:01 DEBUG opendrift.models.openoil.openoil:858: Emulsification not yet started
20:14:01 DEBUG opendrift.models.openoil.openoil:780: Calculating: dispersion - NOAA
20:14:01 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:01 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:01 DEBUG opendrift.models.openoil.openoil:549: Calculating: biodegradation (half_time)
20:14:01 DEBUG opendrift.models.physics_methods:1043: Using mean period Tm02 as wave period
20:14:01 DEBUG opendrift.models.physics_methods:1061: min: 1.256637, mean: 1.256637, max: 1.256637
20:14:01 DEBUG opendrift.models.openoil.openoil:1118: Generating wave breaking droplet size spectrum
20:14:01 DEBUG opendrift.models.openoil.openoil:1145: Droplet distribution median diameter dV_50: inf, dN_50: inf
20:14:01 WARNING opendrift.models.openoil.openoil:1155: Could not update droplet diameters.
20:14:01 DEBUG opendrift.models.oceandrift:512: Using diffusivity from Large1994 since model diffusivities not available
20:14:01 DEBUG opendrift.models.oceandrift:526: Diffusivities are in range 0.0 to 1.2e-05
20:14:01 DEBUG opendrift.models.oceandrift:545: TSprofiles deactivated for vertical mixing
20:14:01 DEBUG opendrift.models.oceandrift:559: Vertical mixing module:environment
20:14:01 DEBUG opendrift.models.oceandrift:562: Turbulent diffusion with random walk scheme using 60 fast time steps of dt=60s
20:14:01 DEBUG opendrift.models.physics_methods:905: No wind for wind-sheared ocean drift
20:14:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
20:14:01 DEBUG opendrift.models.basemodel:1671: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0013743073228135803 and 0.2509683781370698 m/s
20:14:01 DEBUG opendrift.models.basemodel:2123: 1000 active elements (0 deactivated)
20:14:01 DEBUG opendrift.models.basemodel:2152: Cleaning up
20:14:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
20:14:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
20:14:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
20:14:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
20:14:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
20:14:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
20:14:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
20:14:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
20:14:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
20:14:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
20:14:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
20:14:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
20:14:01 DEBUG opendrift.models.basemodel:659: No elements hit coastline.
20:14:01 DEBUG opendrift.models.basemodel:2182: Writing and closing output file: oil.nc
20:14:01 INFO opendrift.export.io_netcdf:121: Wrote 73 steps to file oil.nc
20:14:01 DEBUG opendrift.export.io_netcdf:189: Making netCDF file CDM compliant with fixed dimensions
20:14:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:01 DEBUG opendrift.export.io_netcdf:294: Importing from oil.nc
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/ma/core.py:467: RuntimeWarning: invalid value encountered in cast
fill_value = np.array(fill_value, copy=False, dtype=ndtype)
20:14:01 DEBUG opendrift.models.basemodel:1709: No elements to deactivate
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:x_sea_water_velocity -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:x_sea_water_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:y_sea_water_velocity -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:y_sea_water_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:x_wind -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:x_wind -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:y_wind -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:y_wind -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_height -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_height -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:upward_sea_water_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:upward_sea_water_velocity -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_significant_height -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_significant_height -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_x_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_x_velocity -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_y_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_y_velocity -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_area_fraction -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_area_fraction -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_x_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_x_velocity -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_ice_y_velocity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_ice_y_velocity -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_water_temperature -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_water_temperature -> 10
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_water_salinity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_water_salinity -> 34
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:sea_floor_depth_below_sea_level -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:sea_floor_depth_below_sea_level -> 10000
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:ocean_vertical_diffusivity -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:ocean_vertical_diffusivity -> 0.02
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:land_binary_mask -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:land_binary_mask -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:constant:ocean_mixed_layer_thickness -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: environment:fallback:ocean_mixed_layer_thickness -> 50
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:use_auto_landmask -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:current_uncertainty -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:current_uncertainty_uniform -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:max_speed -> 1.3
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: readers:max_number_of_fails -> 1
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:simulation_name ->
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:coastline_action -> stranding
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:coastline_approximation_precision -> 0.001
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:time_step_minutes -> 60
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:time_step_output_minutes -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:ocean_only -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:number -> 1
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:max_age_seconds -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:advection_scheme -> euler
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:horizontal_diffusivity -> 10.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:profiles_depth -> 50
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:wind_uncertainty -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:relative_wind -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_north_of -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_south_of -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_east_of -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:deactivate_west_of -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:origin_marker -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:z -> 0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:wind_drift_factor -> 0.03
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:current_drift_factor -> 1
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:terminal_velocity -> 0.0
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:oil_film_thickness -> 0.001
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:vertical_advection -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:vertical_mixing -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:timestep -> 60
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:diffusivitymodel -> environment
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:background_diffusivity -> 1.2e-05
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: vertical_mixing:TSprofiles -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:wind_drift_depth -> 0.1
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:stokes_drift -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:stokes_drift_profile -> Phillips
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:use_tabularised_stokes_drift -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:tabularised_stokes_drift_fetch -> 25000
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: general:seafloor_action -> lift_to_seafloor
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: drift:truncate_ocean_model_below_m -> None
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:seafloor -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:m3_per_hour -> 1
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_size_distribution -> uniform
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_mu -> 0.001
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_sigma -> 0.0005
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_min_subsea -> 0.0005
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:droplet_diameter_max_subsea -> 0.005
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:dispersion -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:evaporation -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:emulsification -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:biodegradation -> True
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: biodegradation:method -> half_time
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: processes:update_oilfilm_thickness -> False
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: wave_entrainment:droplet_size_distribution -> Johansen et al. (2015)
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: wave_entrainment:entrainment_rate -> Li et al. (2017)
20:14:01 DEBUG opendrift.export.io_netcdf:393: Setting imported config: seed:oil_type -> GENERIC MEDIUM CRUDE
20:14:01 DEBUG opendrift.models.basemodel:95: Changed mode from Mode.Result to Mode.Result
Plot results
o.plot_oil_budget(show_watercontent_and_viscosity=False, show_wind_and_current=False)
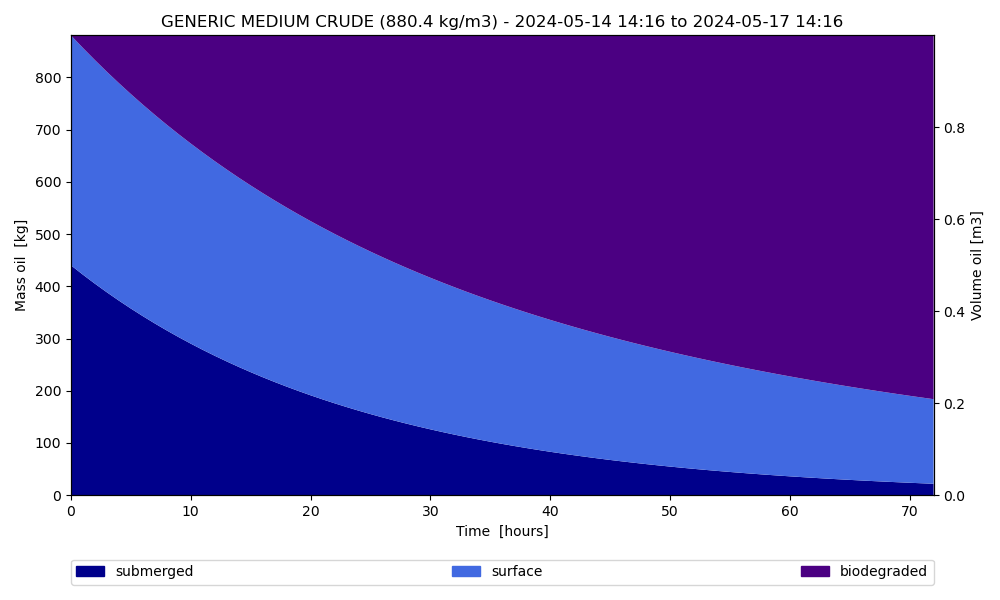
Custom oil budget plot
b = o.get_oil_budget()
import matplotlib.pyplot as plt
time, time_relative = o.get_time_array()
time = np.array([t.total_seconds() / 3600. for t in time_relative])
fig, ax = plt.subplots()
ax.plot(time, b['mass_submerged'], label='Submerged oil mass')
ax.plot(time, b['mass_surface'], label='Surface oil mass')
ax.plot(time, b['mass_biodegraded'], label='Biodegraded oil mass')
ax.set_title(f'{o.get_oil_name()}, {b["oil_density"].max():.2f} kg/m3')
plt.legend()
plt.xlabel('Time [hours]')
plt.ylabel('Mass oil [kg]')
plt.show()
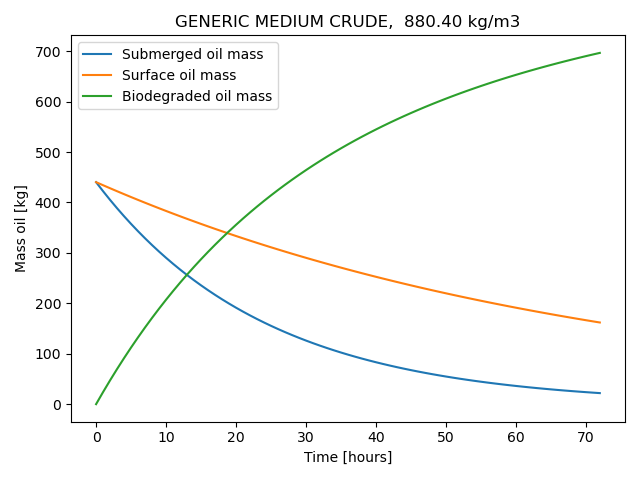
Animation of vertical behaviour
o.animation_profile(markersize='mass_oil', markersize_scaling=50, color='z', alpha=.5)
20:14:02 DEBUG opendrift.models.basemodel:3044: Saving animation..
20:14:02 INFO opendrift.models.basemodel:4613: Saving animation to /root/project/docs/source/gallery/animations/example_biodegradation_0.gif...
20:14:09 DEBUG opendrift.models.basemodel:4651: MPLBACKEND = agg
20:14:09 DEBUG opendrift.models.basemodel:4652: DISPLAY = None
20:14:09 DEBUG opendrift.models.basemodel:4653: Time to save animation: 0:00:07.431016
20:14:09 INFO opendrift.models.basemodel:3251: Time to make animation: 0:00:07.696989
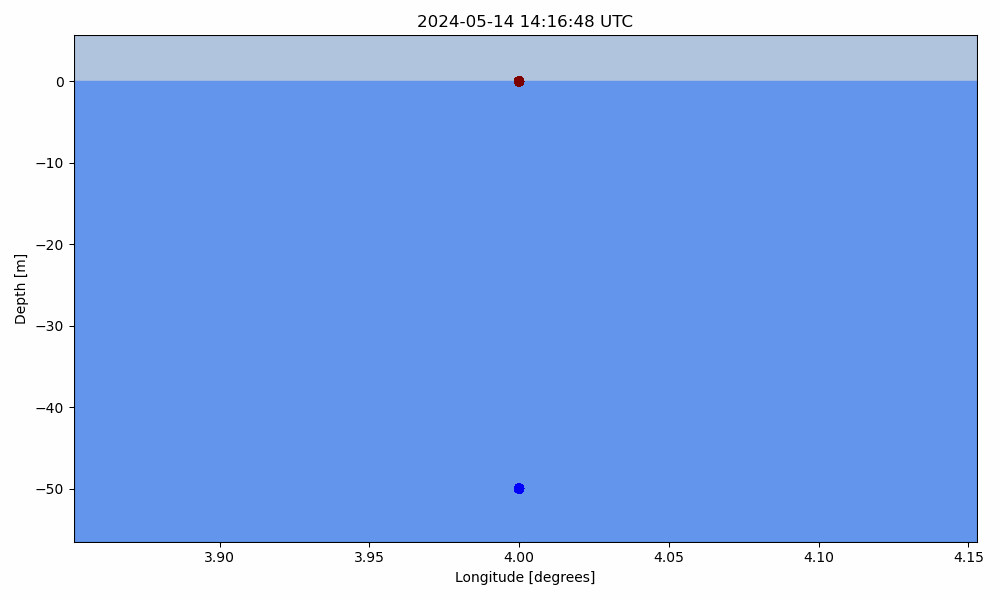
Total running time of the script: (0 minutes 22.652 seconds)