Note
Go to the end to download the full example code.
Manual aggregate
from datetime import datetime, timedelta
import pandas as pd
import matplotlib.pyplot as plt
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.readers import open_mfdataset_overlap
from opendrift.models.oceandrift import OceanDrift
#%
# Create manual aggregate from individual URLs, for NorKyst ocean model initialized at 00 hours every day
start_time = datetime.now().date()-timedelta(days=3)
end_time = datetime.now().date()-timedelta(days=1)
ds = open_mfdataset_overlap(
'https://thredds.met.no/thredds/dodsC/fou-hi/norkyst800m-1h/NorKyst-800m_ZDEPTHS_his.an.%Y%m%d%H.nc',
time_series=pd.date_range(start_time, end_time, freq='1D'))
#%
# Create reader from Xarray dataset
rm = reader_netCDF_CF_generic.Reader(ds, name='NorKyst manual aggregate')
print(rm)
om = OceanDrift()
om.add_reader(rm)
om.seed_elements(lon=4.5, lat=60.0, number=1000, radius=100, time=rm.start_time)
om.run(end_time=rm.end_time)
#%
# Second simulation using ready made aggregate from thredds
ot = OceanDrift()
ot.add_readers_from_list(['https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be'])
ot.seed_elements(lon=4.5, lat=60.0, number=1000, radius=100, time=rm.start_time)
ot.run(end_time=rm.end_time)
#%
# Simulation should be identical, but we see that manual aggregate is significantly slower than using thredds aggregate
om.animation(compare=ot,
legend=[f'NorKyst manual aggregate {om.timing["total time"]}',
f'NorKyst thredds aggregate {ot.timing["total time"]}'])
Opening individual URLs...
Concatenating...
===========================
Reader: NorKyst manual aggregate
Projection:
+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs
Coverage: [degrees]
xmin: 0.000000 xmax: 2080800.000000 step: 800 numx: 2602
ymin: 0.000000 ymax: 720800.000000 step: 800 numy: 902
Corners (lon, lat):
( -1.58, 58.50) ( 23.71, 75.32)
( 9.19, 55.91) ( 38.06, 70.03)
Vertical levels [m]:
[ -0. -3. -10. -15. -25. -50. -75. -100. -150. -200.
-250. -300. -500. -1000. -2000. -3000.]
Available time range:
start: 2025-01-11 00:00:00 end: 2025-01-13 23:00:00 step: 1:00:00
72 times (0 missing)
Variables:
ocean_vertical_diffusivity
x_wind
y_wind
sea_floor_depth_below_sea_level
sea_water_salinity
sea_water_temperature
x_sea_water_velocity
eastward_sea_water_velocity
y_sea_water_velocity
northward_sea_water_velocity
upward_sea_water_velocity
sea_surface_height
latitude
longitude
wind_speed - derived from ['x_wind', 'y_wind']
sea_water_speed - derived from ['x_sea_water_velocity', 'y_sea_water_velocity']
===========================
17:19:12 DEBUG opendrift.config:168: Adding 18 config items from __init__
17:19:12 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
17:19:12 DEBUG opendrift.config:168: Adding 5 config items from __init__
17:19:12 INFO opendrift.models.basemodel:512: OpenDriftSimulation initialised (version 1.12.0 / v1.12.0-55-g4faa301)
17:19:12 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
17:19:12 DEBUG opendrift.config:178: Overwriting config item seed:z
17:19:12 DEBUG opendrift.models.basemodel.environment:328: Added reader NorKyst manual aggregate
17:19:12 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
17:19:12 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
17:19:15 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
17:19:15 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_to_direction: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_significant_height: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_to_direction: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_mean_period: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_significant_height: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: surface_downward_x_stress: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: surface_downward_y_stress: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: turbulent_kinetic_energy: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: turbulent_generic_length_scale: 0.000000
17:19:15 INFO opendrift.models.basemodel.environment:248: ocean_mixed_layer_thickness: 50.000000
17:19:15 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Config to Mode.Ready
17:19:15 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Ready to Mode.Run
17:19:16 DEBUG numcodecs:71: Registering codec 'zlib'
17:19:16 DEBUG numcodecs:71: Registering codec 'gzip'
17:19:16 DEBUG numcodecs:71: Registering codec 'bz2'
17:19:16 DEBUG numcodecs:71: Registering codec 'lzma'
17:19:16 DEBUG numcodecs:71: Registering codec 'blosc'
17:19:16 DEBUG numcodecs:71: Registering codec 'zstd'
17:19:16 DEBUG numcodecs:71: Registering codec 'lz4'
17:19:16 DEBUG numcodecs:71: Registering codec 'astype'
17:19:16 DEBUG numcodecs:71: Registering codec 'delta'
17:19:16 DEBUG numcodecs:71: Registering codec 'quantize'
17:19:16 DEBUG numcodecs:71: Registering codec 'fixedscaleoffset'
17:19:16 DEBUG numcodecs:71: Registering codec 'packbits'
17:19:16 DEBUG numcodecs:71: Registering codec 'categorize'
17:19:16 DEBUG numcodecs:71: Registering codec 'pickle'
17:19:16 DEBUG numcodecs:71: Registering codec 'base64'
17:19:16 DEBUG numcodecs:71: Registering codec 'shuffle'
17:19:16 DEBUG numcodecs:71: Registering codec 'bitround'
17:19:16 DEBUG numcodecs:71: Registering codec 'msgpack2'
17:19:16 DEBUG numcodecs:71: Registering codec 'crc32'
17:19:16 DEBUG numcodecs:71: Registering codec 'adler32'
17:19:16 DEBUG numcodecs:71: Registering codec 'jenkins_lookup3'
17:19:16 DEBUG numcodecs:71: Registering codec 'json2'
17:19:16 DEBUG numcodecs:71: Registering codec 'vlen-utf8'
17:19:16 DEBUG numcodecs:71: Registering codec 'vlen-bytes'
17:19:16 DEBUG numcodecs:71: Registering codec 'vlen-array'
17:19:16 DEBUG numcodecs:71: Registering codec 'fletcher32'
17:19:16 DEBUG numcodecs:71: Registering codec 'pcodec'
17:19:16 DEBUG numcodecs:71: Registering codec 'n5_wrapper'
17:19:16 DEBUG opendrift.models.basemodel:1774:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.12.0
Platform: Linux, 5.15.0-1057-aws
68.56774139404297 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.11.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 1.3.5
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
17:19:16 DEBUG opendrift.models.basemodel:1788: No output file is specified, neglecting export_buffer_length
17:19:16 DEBUG opendrift.models.basemodel:1906: Finalizing environment and preparing readers for simulation coverage ([-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]) and time (2025-01-11 00:00:00 to 2025-01-13 23:00:00)
17:19:16 DEBUG opendrift.models.basemodel.environment:180: Preparing NorKyst manual aggregate for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
17:19:16 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader NorKyst manual aggregate before starting new simulation
17:19:16 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader NorKyst manual aggregate, assuming a maximum average speed of 2 m/s and time span of 1:00:00
17:19:16 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for NorKyst manual aggregate
17:19:16 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
17:19:16 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
17:19:16 INFO opendrift.models.basemodel:932: Using existing reader for land_binary_mask
17:19:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:19:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:19:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:19:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:19:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:19:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:19:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:19:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:19:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:19:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:19:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:19:16 INFO opendrift.models.basemodel:943: All points are in ocean
17:19:16 DEBUG opendrift.models.basemodel:887: to be seeded: 1000, already seeded 0
17:19:16 DEBUG opendrift.models.basemodel:905: Released 1000 new elements.
17:19:16 WARNING opendrift.models.basemodel:726: Seafloor check not being run because environment is missing. This will happen the first time the function is run but if it happens subsequently there is probably a problem.
17:19:16 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:19:16 INFO opendrift.models.basemodel:2032: 2025-01-11 00:00:00 - step 1 of 71 - 1000 active elements (0 deactivated)
17:19:16 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:19:16 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:19:16 DEBUG opendrift.models.basemodel:2051: 59.99731 <- latitude -> 60.002846
17:19:16 DEBUG opendrift.models.basemodel:2056: 4.4945407 <- longitude -> 4.504945
17:19:16 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:19:16 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:19:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:19:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:19:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 00:00:00 (before)
2025-01-11 01:00:00 (after)
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:19:28 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-11 00:00:00)
17:19:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 00:00:00) in space (linearNDFast)
17:19:28 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:19:28 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:19:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
17:19:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
17:19:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:19:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:19:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:19:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:19:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:19:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:19:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:19:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:19:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:19:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:19:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:19:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0299121 (min) 0.0385718 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.123546 (min) -0.101854 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.721338 (min) -0.71924 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.37718 (min) -1.35714 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.06815 (min) -7.94024 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.73794e-07 (min) 3.18015e-07 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.369 (min) 298.632 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:19:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:19:28 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.597613, mean: 1.622316, max: 1.646645
17:19:28 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:19:28 DEBUG opendrift.models.physics_methods:1061: min: 6.885974, mean: 6.938986, max: 6.990844
17:19:28 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 6.885974, mean: 6.938986, max: 6.990844
17:19:28 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:19:28 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:19:28 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:19:28 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:19:28 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.161175 m/s - 0.163630 m/s)
17:19:28 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:19:28 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:19:28 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:19:28 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:19:28 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:19:28 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:19:28 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:19:28 INFO opendrift.models.basemodel:2032: 2025-01-11 01:00:00 - step 2 of 71 - 1000 active elements (0 deactivated)
17:19:28 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:19:28 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:19:28 DEBUG opendrift.models.basemodel:2051: 59.988504092561726 <- latitude -> 59.99409196331214
17:19:28 DEBUG opendrift.models.basemodel:2056: 4.495099233223983 <- longitude -> 4.5054428283887855
17:19:28 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:19:28 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:19:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:19:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:19:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 01:00:00 (before)
2025-01-11 02:00:00 (after)
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:19:45 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 01:00:00)
17:19:45 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 01:00:00) in space (linearNDFast)
17:19:45 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:19:45 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:19:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50491168847081 and -65.49456810576453 degrees.
17:19:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50491168847081 and -65.49456810576453 degrees.
17:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:19:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:19:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:19:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:19:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:19:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:19:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:19:45 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:19:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:19:45 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0302097 (min) 0.0410515 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0639426 (min) -0.04447 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.752453 (min) -0.750927 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.41837 (min) -1.39774 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.54686 (min) -8.48849 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.2857e-06 (min) 6.45347e-06 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.962 (min) 298.291 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:19:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:19:45 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.820749, mean: 1.834391, max: 1.846378
17:19:45 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:19:45 DEBUG opendrift.models.physics_methods:1061: min: 7.351138, mean: 7.378622, max: 7.402696
17:19:45 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.351138, mean: 7.378622, max: 7.402696
17:19:45 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:19:45 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:19:45 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:19:45 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:19:45 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.172063 m/s - 0.173270 m/s)
17:19:45 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:19:45 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:19:45 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:19:45 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:19:45 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:19:45 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:19:45 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:19:45 INFO opendrift.models.basemodel:2032: 2025-01-11 02:00:00 - step 3 of 71 - 1000 active elements (0 deactivated)
17:19:45 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:19:45 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:19:45 DEBUG opendrift.models.basemodel:2051: 59.981084562800206 <- latitude -> 59.98691162512811
17:19:45 DEBUG opendrift.models.basemodel:2056: 4.495231268758131 <- longitude -> 4.506287767226153
17:19:45 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:19:45 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:19:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:19:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:19:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:19:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:19:45 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 02:00:00 (before)
2025-01-11 03:00:00 (after)
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:20:02 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-11 02:00:00)
17:20:02 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 02:00:00) in space (linearNDFast)
17:20:02 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:20:02 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:20:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50477965678654 and -65.49372315370067 degrees.
17:20:02 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50477965678654 and -65.49372315370067 degrees.
17:20:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:20:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:20:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:20:02 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:20:02 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:20:02 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:20:02 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:02 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:20:02 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:20:02 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:20:02 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.00333729 (min) 0.0240672 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0263263 (min) -0.00841098 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.714738 (min) -0.713182 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.61837 (min) -1.6064 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.44506 (min) -8.4241 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.29824e-05 (min) -1.91683e-05 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.537 (min) 297.947 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:20:02 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:20:02 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.810182, mean: 1.814181, max: 1.817931
17:20:02 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:20:02 DEBUG opendrift.models.physics_methods:1061: min: 7.329776, mean: 7.337867, max: 7.345447
17:20:02 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.329776, mean: 7.337867, max: 7.345447
17:20:02 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:20:02 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:02 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:20:02 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:20:02 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.171563 m/s - 0.171930 m/s)
17:20:02 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:20:02 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:20:02 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:20:02 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:20:02 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:20:02 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:02 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:20:02 INFO opendrift.models.basemodel:2032: 2025-01-11 03:00:00 - step 4 of 71 - 1000 active elements (0 deactivated)
17:20:02 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:20:02 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:20:02 DEBUG opendrift.models.basemodel:2051: 59.97481112515269 <- latitude -> 59.98118303989345
17:20:02 DEBUG opendrift.models.basemodel:2056: 4.493372331996275 <- longitude -> 4.505640901495267
17:20:02 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:20:02 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:20:02 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:20:02 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:02 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:02 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:20:02 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 03:00:00 (before)
2025-01-11 04:00:00 (after)
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:20:18 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-11 03:00:00)
17:20:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 03:00:00) in space (linearNDFast)
17:20:18 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:20:18 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:20:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5066385931251 and -65.49437003092132 degrees.
17:20:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5066385931251 and -65.49437003092132 degrees.
17:20:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:20:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:20:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:20:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:20:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:20:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:20:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:20:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:20:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:20:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0261764 (min) -0.00948368 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0550451 (min) 0.0657477 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.597457 (min) -0.595651 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.11173 (min) -2.07221 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.0639 (min) -8.02445 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.39918e-05 (min) 3.45642e-05 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.089 (min) 297.598 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:20:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:20:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.689824, mean: 1.699174, max: 1.709352
17:20:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:20:18 DEBUG opendrift.models.physics_methods:1061: min: 7.081909, mean: 7.101472, max: 7.122711
17:20:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.081909, mean: 7.101472, max: 7.122711
17:20:18 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:20:18 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:18 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:20:18 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:20:18 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.165761 m/s - 0.166716 m/s)
17:20:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:20:18 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:20:18 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:20:18 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:20:18 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:20:18 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:18 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:20:18 INFO opendrift.models.basemodel:2032: 2025-01-11 04:00:00 - step 5 of 71 - 1000 active elements (0 deactivated)
17:20:18 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:20:18 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:20:18 DEBUG opendrift.models.basemodel:2051: 59.97171660524069 <- latitude -> 59.977769454970186
17:20:18 DEBUG opendrift.models.basemodel:2056: 4.489012839664575 <- longitude -> 4.5022646199067236
17:20:18 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:20:18 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:20:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:20:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:20:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 04:00:00 (before)
2025-01-11 05:00:00 (after)
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:20:41 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-11 04:00:00)
17:20:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 04:00:00) in space (linearNDFast)
17:20:41 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:20:41 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:20:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51099809612052 and -65.49774630536227 degrees.
17:20:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51099809612052 and -65.49774630536227 degrees.
17:20:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:20:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:20:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:20:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:20:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:20:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:20:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:20:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:20:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:20:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:20:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0261125 (min) -0.013582 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.141867 (min) 0.153997 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.456874 (min) -0.454275 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.43844 (min) -1.39596 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.50383 (min) -7.42503 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.07132e-05 (min) 4.08934e-05 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.803 (min) 297.322 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:20:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:20:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.404348, mean: 1.419107, max: 1.435742
17:20:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:20:41 DEBUG opendrift.models.physics_methods:1061: min: 6.456050, mean: 6.489877, max: 6.527815
17:20:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 6.456050, mean: 6.489877, max: 6.527815
17:20:41 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:20:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:41 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:20:41 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:20:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.151112 m/s - 0.152792 m/s)
17:20:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:20:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:20:41 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:20:41 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:20:41 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:20:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:20:41 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:20:41 INFO opendrift.models.basemodel:2032: 2025-01-11 05:00:00 - step 6 of 71 - 1000 active elements (0 deactivated)
17:20:41 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:20:41 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:20:41 DEBUG opendrift.models.basemodel:2051: 59.97184576044411 <- latitude -> 59.97754037006435
17:20:41 DEBUG opendrift.models.basemodel:2056: 4.485640289103096 <- longitude -> 4.499480755734126
17:20:41 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:20:41 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:20:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:20:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:20:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:20:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:20:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 05:00:00 (before)
2025-01-11 06:00:00 (after)
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:21:00 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 05:00:00)
17:21:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 05:00:00) in space (linearNDFast)
17:21:00 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:21:00 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:21:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51437063902036 and -65.50053017995474 degrees.
17:21:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51437063902036 and -65.50053017995474 degrees.
17:21:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:21:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:21:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:21:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:21:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:21:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:21:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:21:00 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:21:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:21:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0189633 (min) 0.051294 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.200789 (min) 0.214313 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.309297 (min) -0.307518 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.28004 (min) -1.26432 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.98006 (min) -6.87668 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.26599e-05 (min) 5.29343e-05 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.772 (min) 297.264 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:21:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:21:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.202722, mean: 1.220645, max: 1.238534
17:21:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:21:00 DEBUG opendrift.models.physics_methods:1061: min: 5.974646, mean: 6.018980, max: 6.062945
17:21:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.974646, mean: 6.018980, max: 6.062945
17:21:00 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:21:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:00 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:21:00 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:21:00 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.139844 m/s - 0.141911 m/s)
17:21:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:21:00 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:21:00 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:21:00 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:21:00 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:21:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:00 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:21:00 INFO opendrift.models.basemodel:2032: 2025-01-11 06:00:00 - step 7 of 71 - 1000 active elements (0 deactivated)
17:21:00 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:21:00 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:21:00 DEBUG opendrift.models.basemodel:2051: 59.974273132816045 <- latitude -> 59.97955381863777
17:21:00 DEBUG opendrift.models.basemodel:2056: 4.485978370070531 <- longitude -> 4.500641428280635
17:21:00 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:21:00 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:21:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:21:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:21:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 06:00:00 (before)
2025-01-11 07:00:00 (after)
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:21:16 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 06:00:00)
17:21:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 06:00:00) in space (linearNDFast)
17:21:16 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:21:16 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:21:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51403256771981 and -65.4993694977281 degrees.
17:21:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51403256771981 and -65.4993694977281 degrees.
17:21:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:21:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:21:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:21:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:21:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:21:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:21:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:21:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:21:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:21:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0989847 (min) 0.137595 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.192913 (min) 0.197805 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.139302 (min) -0.137866 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.715419 (min) -0.702794 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.99927 (min) -6.8861 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.33699e-05 (min) 4.35014e-05 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.966 (min) 297.401 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:21:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:21:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.178840, mean: 1.198107, max: 1.217565
17:21:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:21:16 DEBUG opendrift.models.physics_methods:1061: min: 5.915030, mean: 5.963153, max: 6.011401
17:21:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.915030, mean: 5.963153, max: 6.011401
17:21:16 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:21:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:16 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:21:16 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:21:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.138449 m/s - 0.140705 m/s)
17:21:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:21:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:21:16 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:21:16 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:21:16 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:21:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:16 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:21:16 INFO opendrift.models.basemodel:2032: 2025-01-11 07:00:00 - step 8 of 71 - 1000 active elements (0 deactivated)
17:21:16 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:21:16 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:21:16 DEBUG opendrift.models.basemodel:2051: 59.97606461732485 <- latitude -> 59.98140923647237
17:21:16 DEBUG opendrift.models.basemodel:2056: 4.492278344625498 <- longitude -> 4.5081702350132185
17:21:16 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:21:16 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:21:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:21:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:21:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 07:00:00 (before)
2025-01-11 08:00:00 (after)
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:21:32 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-11 07:00:00)
17:21:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 07:00:00) in space (linearNDFast)
17:21:32 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:21:32 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:21:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50773258391567 and -65.4918406948722 degrees.
17:21:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50773258391567 and -65.4918406948722 degrees.
17:21:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:21:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:21:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:21:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:21:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:21:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:21:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:21:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:21:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:21:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.1733 (min) 0.201065 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.124665 (min) 0.133417 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.00981673 (min) -0.00865051 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.866055 (min) -0.852133 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.24313 (min) -6.18181 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.89429e-05 (min) 1.94251e-05 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.202 (min) 297.611 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:21:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:21:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.958524, mean: 0.966320, max: 0.976689
17:21:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:21:32 DEBUG opendrift.models.physics_methods:1061: min: 5.333729, mean: 5.355372, max: 5.384034
17:21:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.333729, mean: 5.355372, max: 5.384034
17:21:32 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:21:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:32 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:21:32 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:21:32 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.124843 m/s - 0.126020 m/s)
17:21:32 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:21:32 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:21:32 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:21:32 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:21:32 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:21:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:32 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:21:32 INFO opendrift.models.basemodel:2032: 2025-01-11 08:00:00 - step 9 of 71 - 1000 active elements (0 deactivated)
17:21:32 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:21:32 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:21:32 DEBUG opendrift.models.basemodel:2051: 59.9760981711631 <- latitude -> 59.98171213866539
17:21:32 DEBUG opendrift.models.basemodel:2056: 4.502967176756443 <- longitude -> 4.51975313257609
17:21:32 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:21:32 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:21:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:21:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:21:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 08:00:00 (before)
2025-01-11 09:00:00 (after)
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:21:50 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 08:00:00)
17:21:50 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 08:00:00) in space (linearNDFast)
17:21:50 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:21:50 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:21:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49704375029656 and -65.4802578031726 degrees.
17:21:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49704375029656 and -65.4802578031726 degrees.
17:21:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:21:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:21:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:21:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:21:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:21:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:21:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:21:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:21:50 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:21:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:21:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.223672 (min) 0.243852 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0505621 (min) 0.0594983 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0314569 (min) 0.0329843 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.635929 (min) -0.509189 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.60515 (min) -6.43301 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.00466e-05 (min) -9.16418e-06 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.397 (min) 297.796 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:21:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:21:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.027987, mean: 1.053371, max: 1.079629
17:21:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:21:50 DEBUG opendrift.models.physics_methods:1061: min: 5.523614, mean: 5.591359, max: 5.660657
17:21:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.523614, mean: 5.591359, max: 5.660657
17:21:50 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:21:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:50 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:21:50 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:21:50 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.129287 m/s - 0.132495 m/s)
17:21:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:21:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:21:50 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:21:50 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:21:50 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:21:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:21:50 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:21:50 INFO opendrift.models.basemodel:2032: 2025-01-11 09:00:00 - step 10 of 71 - 1000 active elements (0 deactivated)
17:21:50 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:21:50 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:21:50 DEBUG opendrift.models.basemodel:2051: 59.973805375529466 <- latitude -> 59.979152527849955
17:21:50 DEBUG opendrift.models.basemodel:2056: 4.517400589720703 <- longitude -> 4.534324787047799
17:21:50 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:21:50 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:21:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:21:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:21:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:21:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:21:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 09:00:00 (before)
2025-01-11 10:00:00 (after)
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:22:07 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 09:00:00)
17:22:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 09:00:00) in space (linearNDFast)
17:22:07 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:22:07 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:22:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48261034472999 and -65.46568615368999 degrees.
17:22:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48261034472999 and -65.46568615368999 degrees.
17:22:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:22:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:22:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:22:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:22:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:22:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:22:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:22:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:22:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:22:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.265701 (min) 0.291542 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0241131 (min) 0.0553339 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0380497 (min) -0.0355991 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.16879 (min) -1.06231 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.37064 (min) -5.25735 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.89164e-05 (min) -3.86085e-05 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.497 (min) 297.877 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:22:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:22:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.713543, mean: 0.725478, max: 0.737438
17:22:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:22:07 DEBUG opendrift.models.physics_methods:1061: min: 4.601926, mean: 4.640237, max: 4.678347
17:22:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.601926, mean: 4.640237, max: 4.678347
17:22:07 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:22:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:07 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:22:07 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:22:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.107714 m/s - 0.109503 m/s)
17:22:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:22:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:22:07 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:22:07 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:22:07 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:22:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:07 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:22:07 INFO opendrift.models.basemodel:2032: 2025-01-11 10:00:00 - step 11 of 71 - 1000 active elements (0 deactivated)
17:22:07 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:22:07 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:22:07 DEBUG opendrift.models.basemodel:2051: 59.97214753548138 <- latitude -> 59.97648991008711
17:22:07 DEBUG opendrift.models.basemodel:2056: 4.534082412807478 <- longitude -> 4.5510874568765045
17:22:07 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:22:07 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:22:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:22:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:22:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 10:00:00 (before)
2025-01-11 11:00:00 (after)
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:22:24 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x24x7) for time before (2025-01-11 10:00:00)
17:22:24 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 10:00:00) in space (linearNDFast)
17:22:24 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:22:24 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:22:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46592850522856 and -65.4489234731135 degrees.
17:22:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46592850522856 and -65.4489234731135 degrees.
17:22:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:22:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:22:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:22:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:22:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:22:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:22:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:22:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:22:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:22:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.333282 (min) 0.356602 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0241131 (min) 0.0342193 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.224206 (min) -0.222843 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.28282 (min) -1.26417 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.94131 (min) -3.85228 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.05388e-05 (min) -6.04199e-05 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.691 (min) 297.993 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:22:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:22:24 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.405345, mean: 0.412519, max: 0.421789
17:22:24 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:22:24 DEBUG opendrift.models.physics_methods:1061: min: 3.468501, mean: 3.499044, max: 3.538157
17:22:24 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.468501, mean: 3.499044, max: 3.538157
17:22:24 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:22:24 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:24 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:22:24 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:22:24 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.081185 m/s - 0.082815 m/s)
17:22:24 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:22:24 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:22:24 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:22:24 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:22:24 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:22:24 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:24 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:22:24 INFO opendrift.models.basemodel:2032: 2025-01-11 11:00:00 - step 12 of 71 - 1000 active elements (0 deactivated)
17:22:24 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:22:24 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:22:24 DEBUG opendrift.models.basemodel:2051: 59.97059127811301 <- latitude -> 59.97483841471826
17:22:24 DEBUG opendrift.models.basemodel:2056: 4.554688800136138 <- longitude -> 4.5719565289625095
17:22:24 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:22:24 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:22:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:22:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:22:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 11:00:00 (before)
2025-01-11 12:00:00 (after)
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:22:36 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 11:00:00)
17:22:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 11:00:00) in space (linearNDFast)
17:22:36 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:22:36 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:22:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44532213429143 and -65.42805440645367 degrees.
17:22:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44532213429143 and -65.42805440645367 degrees.
17:22:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:22:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:22:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:22:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:22:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:22:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:22:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:22:36 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:22:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:22:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.381591 (min) 0.387685 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0304098 (min) -0.0219725 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.454384 (min) -0.452452 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.32134 (min) -1.31099 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.65683 (min) -3.55804 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.10819e-05 (min) -6.06417e-05 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.998 (min) 298.271 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:22:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:22:36 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.353943, mean: 0.362260, max: 0.371849
17:22:36 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:22:36 DEBUG opendrift.models.physics_methods:1061: min: 3.241131, mean: 3.278962, max: 3.322103
17:22:36 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.241131, mean: 3.278962, max: 3.322103
17:22:36 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:22:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:36 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:22:36 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:22:36 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.075863 m/s - 0.077758 m/s)
17:22:36 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:22:36 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:22:36 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:22:36 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:22:36 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:22:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:36 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:22:36 INFO opendrift.models.basemodel:2032: 2025-01-11 12:00:00 - step 13 of 71 - 1000 active elements (0 deactivated)
17:22:36 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:22:36 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:22:36 DEBUG opendrift.models.basemodel:2051: 59.96728562327035 <- latitude -> 59.97182252266313
17:22:36 DEBUG opendrift.models.basemodel:2056: 4.577809141577973 <- longitude -> 4.594851384344319
17:22:36 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:22:36 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:22:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:22:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:22:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 12:00:00 (before)
2025-01-11 13:00:00 (after)
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:22:49 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-11 12:00:00)
17:22:49 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 12:00:00) in space (linearNDFast)
17:22:49 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:22:49 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:22:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42220178758515 and -65.40515953315897 degrees.
17:22:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42220178758515 and -65.40515953315897 degrees.
17:22:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:22:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:22:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:22:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:22:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:22:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:22:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:22:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:22:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:22:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:22:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.36091 (min) 0.387693 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0605161 (min) -0.0317066 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.64083 (min) -0.637864 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.565515 (min) -0.545974 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.50118 (min) -3.3926 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.50632e-05 (min) -3.48112e-05 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.242 (min) 298.504 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:22:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:22:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.291007, mean: 0.299629, max: 0.308889
17:22:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:22:49 DEBUG opendrift.models.physics_methods:1061: min: 2.938875, mean: 2.982060, max: 3.027824
17:22:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.938875, mean: 2.982060, max: 3.027824
17:22:49 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:22:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:49 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:22:49 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:22:49 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.068788 m/s - 0.070870 m/s)
17:22:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:22:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:22:49 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:22:49 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:22:49 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:22:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:22:49 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:22:49 INFO opendrift.models.basemodel:2032: 2025-01-11 13:00:00 - step 14 of 71 - 1000 active elements (0 deactivated)
17:22:49 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:22:49 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:22:49 DEBUG opendrift.models.basemodel:2051: 59.96374961591877 <- latitude -> 59.967727424462225
17:22:49 DEBUG opendrift.models.basemodel:2056: 4.602048476625936 <- longitude -> 4.617409005657026
17:22:49 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:22:49 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:22:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:22:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:22:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:22:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:22:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 13:00:00 (before)
2025-01-11 14:00:00 (after)
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:23:01 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-11 13:00:00)
17:23:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 13:00:00) in space (linearNDFast)
17:23:01 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:23:01 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:23:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39796244561853 and -65.3826019134292 degrees.
17:23:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39796244561853 and -65.3826019134292 degrees.
17:23:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:23:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:23:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:23:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:23:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:23:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:23:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:23:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:23:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:23:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.30618 (min) 0.333856 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00778391 (min) 0.027319 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.720306 (min) -0.716689 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.266892 (min) -0.2419 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.39797 (min) -3.31302 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.82697e-06 (min) -8.75554e-06 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.475 (min) 298.746 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:23:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:23:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.271764, mean: 0.278342, max: 0.285482
17:23:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:23:01 DEBUG opendrift.models.physics_methods:1061: min: 2.840046, mean: 2.874190, max: 2.910846
17:23:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.840046, mean: 2.874190, max: 2.910846
17:23:01 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:23:01 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:01 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:23:01 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:23:01 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.066475 m/s - 0.068132 m/s)
17:23:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:23:01 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:23:01 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:23:01 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:23:01 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:23:01 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:01 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:23:01 INFO opendrift.models.basemodel:2032: 2025-01-11 14:00:00 - step 15 of 71 - 1000 active elements (0 deactivated)
17:23:01 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:23:01 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:23:01 DEBUG opendrift.models.basemodel:2051: 59.96203440601768 <- latitude -> 59.965499428065996
17:23:01 DEBUG opendrift.models.basemodel:2056: 4.623223124764574 <- longitude -> 4.63683042187158
17:23:01 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:23:01 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:23:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:23:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:23:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 14:00:00 (before)
2025-01-11 15:00:00 (after)
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:23:13 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-11 14:00:00)
17:23:13 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 14:00:00) in space (linearNDFast)
17:23:13 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:23:13 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:23:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37678780679435 and -65.3631804984475 degrees.
17:23:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37678780679435 and -65.3631804984475 degrees.
17:23:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:23:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:23:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:23:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:23:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:23:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:23:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:23:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:23:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:23:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.26272 (min) 0.284156 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0546003 (min) 0.0738397 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.696098 (min) -0.693168 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.112227 (min) 0.114737 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.29217 (min) -3.22439 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.64901e-05 (min) 1.66141e-05 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.763 (min) 299.042 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:23:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:23:13 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.256074, mean: 0.261343, max: 0.266937
17:23:13 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:23:13 DEBUG opendrift.models.physics_methods:1061: min: 2.756842, mean: 2.785046, max: 2.814713
17:23:13 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.756842, mean: 2.785046, max: 2.814713
17:23:13 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:23:13 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:13 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:23:13 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:23:13 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064527 m/s - 0.065882 m/s)
17:23:13 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:23:13 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:23:13 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:23:13 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:23:13 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:23:13 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:13 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:23:13 INFO opendrift.models.basemodel:2032: 2025-01-11 15:00:00 - step 16 of 71 - 1000 active elements (0 deactivated)
17:23:13 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:23:13 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:23:13 DEBUG opendrift.models.basemodel:2051: 59.961764950458985 <- latitude -> 59.96565439126498
17:23:13 DEBUG opendrift.models.basemodel:2056: 4.641576936713147 <- longitude -> 4.653917638514869
17:23:13 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:23:13 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:23:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:23:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:23:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 15:00:00 (before)
2025-01-11 16:00:00 (after)
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:23:29 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 15:00:00)
17:23:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 15:00:00) in space (linearNDFast)
17:23:29 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:23:29 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:23:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.35843398896895 and -65.34609328843915 degrees.
17:23:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.35843398896895 and -65.34609328843915 degrees.
17:23:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:23:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:23:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:23:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:23:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:23:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:23:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:23:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:23:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:23:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.196506 (min) 0.222549 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0813321 (min) 0.106421 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.606261 (min) -0.60362 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.628972 (min) -0.591594 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.23468 (min) -3.18811 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.01619e-05 (min) 3.02817e-05 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.03 (min) 299.272 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:23:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:23:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.259028, mean: 0.262906, max: 0.266690
17:23:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:23:29 DEBUG opendrift.models.physics_methods:1061: min: 2.772702, mean: 2.793372, max: 2.813411
17:23:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.772702, mean: 2.793372, max: 2.813411
17:23:29 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:23:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:29 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:23:29 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:23:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064899 m/s - 0.065852 m/s)
17:23:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:23:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:23:29 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:23:29 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:23:29 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:23:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:29 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:23:29 INFO opendrift.models.basemodel:2032: 2025-01-11 16:00:00 - step 17 of 71 - 1000 active elements (0 deactivated)
17:23:29 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:23:29 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:23:29 DEBUG opendrift.models.basemodel:2051: 59.962303623269875 <- latitude -> 59.96702646456477
17:23:29 DEBUG opendrift.models.basemodel:2056: 4.6550067533238355 <- longitude -> 4.665772007423337
17:23:29 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:23:29 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:23:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:23:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:23:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 16:00:00 (before)
2025-01-11 17:00:00 (after)
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:23:41 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x22x7) for time before (2025-01-11 16:00:00)
17:23:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 16:00:00) in space (linearNDFast)
17:23:41 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:23:41 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:23:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34500417931328 and -65.33423891859279 degrees.
17:23:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34500417931328 and -65.33423891859279 degrees.
17:23:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:23:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:23:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:23:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:23:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:23:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:23:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:23:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:23:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:23:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.114543 (min) 0.14608 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.114092 (min) 0.142509 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.479382 (min) -0.477031 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.21381 (min) -1.19888 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.61875 (min) -2.56611 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.62635e-05 (min) 3.65343e-05 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.049 (min) 299.385 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:23:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:23:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.197983, mean: 0.201130, max: 0.204362
17:23:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:23:41 DEBUG opendrift.models.physics_methods:1061: min: 2.424063, mean: 2.443243, max: 2.462804
17:23:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.424063, mean: 2.443243, max: 2.462804
17:23:41 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:23:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:41 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:23:41 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:23:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.056738 m/s - 0.057645 m/s)
17:23:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:23:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:23:41 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:23:41 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:23:41 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:23:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:41 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:23:41 INFO opendrift.models.basemodel:2032: 2025-01-11 17:00:00 - step 18 of 71 - 1000 active elements (0 deactivated)
17:23:41 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:23:41 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:23:41 DEBUG opendrift.models.basemodel:2051: 59.96432257321534 <- latitude -> 59.969954368568494
17:23:41 DEBUG opendrift.models.basemodel:2056: 4.662399308146423 <- longitude -> 4.671951678524563
17:23:41 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:23:41 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:23:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:23:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:23:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 17:00:00 (before)
2025-01-11 18:00:00 (after)
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:23:53 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-11 17:00:00)
17:23:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 17:00:00) in space (linearNDFast)
17:23:53 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:23:53 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:23:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33761161618891 and -65.32805923702662 degrees.
17:23:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33761161618891 and -65.32805923702662 degrees.
17:23:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:23:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:23:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:23:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:23:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:23:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:23:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:23:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:23:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:23:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:23:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.051936 (min) 0.0802498 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.173557 (min) 0.233623 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.336758 (min) -0.334729 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.170251 (min) -0.154842 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.71734 (min) -2.67086 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.04552e-05 (min) 4.07391e-05 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.153 (min) 299.529 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:23:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:23:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.176085, mean: 0.179136, max: 0.182325
17:23:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:23:53 DEBUG opendrift.models.physics_methods:1061: min: 2.286078, mean: 2.305786, max: 2.326232
17:23:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.286078, mean: 2.305786, max: 2.326232
17:23:53 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:23:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:53 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:23:53 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:23:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.053509 m/s - 0.054449 m/s)
17:23:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:23:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:23:53 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:23:53 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:23:53 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:23:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:23:53 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:23:53 INFO opendrift.models.basemodel:2032: 2025-01-11 18:00:00 - step 19 of 71 - 1000 active elements (0 deactivated)
17:23:53 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:23:53 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:23:53 DEBUG opendrift.models.basemodel:2051: 59.968506969286615 <- latitude -> 59.9752314598785
17:23:53 DEBUG opendrift.models.basemodel:2056: 4.666925985765665 <- longitude -> 4.675445129461778
17:23:53 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:23:53 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:23:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:23:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:23:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:23:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:23:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 18:00:00 (before)
2025-01-11 19:00:00 (after)
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:24:05 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-11 18:00:00)
17:24:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 18:00:00) in space (linearNDFast)
17:24:05 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:24:05 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:24:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33308493772962 and -65.32456578661767 degrees.
17:24:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33308493772962 and -65.32456578661767 degrees.
17:24:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:24:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:24:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:24:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:24:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:24:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:24:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:24:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:24:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:24:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.034289 (min) 0.0569343 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.211075 (min) 0.276108 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.181109 (min) -0.179942 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.54601 (min) -0.493504 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.95241 (min) -1.91174 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.42954e-05 (min) 4.4467e-05 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.417 (min) 299.843 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:24:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:24:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.096137, mean: 0.098408, max: 0.100790
17:24:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:24:05 DEBUG opendrift.models.physics_methods:1061: min: 1.689180, mean: 1.708995, max: 1.729569
17:24:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.689180, mean: 1.708995, max: 1.729569
17:24:05 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:24:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:05 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:24:05 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:24:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039537 m/s - 0.040483 m/s)
17:24:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:24:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:24:05 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:24:05 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:24:05 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:24:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:05 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:24:05 INFO opendrift.models.basemodel:2032: 2025-01-11 19:00:00 - step 20 of 71 - 1000 active elements (0 deactivated)
17:24:05 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:24:05 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:24:05 DEBUG opendrift.models.basemodel:2051: 59.97432830036097 <- latitude -> 59.98246725762972
17:24:05 DEBUG opendrift.models.basemodel:2056: 4.668966521861544 <- longitude -> 4.677812510366006
17:24:05 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:24:05 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:24:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:24:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:24:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 19:00:00 (before)
2025-01-11 20:00:00 (after)
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:24:17 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-11 19:00:00)
17:24:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 19:00:00) in space (linearNDFast)
17:24:17 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:24:17 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:24:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33104439255906 and -65.32219840932183 degrees.
17:24:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33104439255906 and -65.32219840932183 degrees.
17:24:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:24:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:24:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:24:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:24:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:24:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:24:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:24:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:24:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:24:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0513345 (min) 0.0705025 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.194708 (min) 0.261313 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0272315 (min) -0.0255531 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.273366 (min) -0.251497 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.889371 (min) -0.80812 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.82119e-05 (min) 3.82878e-05 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.802 (min) 300.247 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:24:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:24:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.017902, mean: 0.019362, max: 0.021034
17:24:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:24:17 DEBUG opendrift.models.physics_methods:1061: min: 0.728925, mean: 0.758005, max: 0.790120
17:24:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.728925, mean: 0.758005, max: 0.790120
17:24:17 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:24:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:17 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:24:17 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:24:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.017061 m/s - 0.018494 m/s)
17:24:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:24:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:24:17 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:24:17 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:24:17 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:24:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:17 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:24:17 INFO opendrift.models.basemodel:2032: 2025-01-11 20:00:00 - step 21 of 71 - 1000 active elements (0 deactivated)
17:24:17 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:24:17 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:24:17 DEBUG opendrift.models.basemodel:2051: 59.98021453604092 <- latitude -> 59.99007399864844
17:24:17 DEBUG opendrift.models.basemodel:2056: 4.672183920907031 <- longitude -> 4.681631187493185
17:24:17 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:24:17 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:24:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:24:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:24:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 20:00:00 (before)
2025-01-11 21:00:00 (after)
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:24:29 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x7) for time before (2025-01-11 20:00:00)
17:24:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 20:00:00) in space (linearNDFast)
17:24:29 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:24:29 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:24:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32782699202842 and -65.31837972369411 degrees.
17:24:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32782699202842 and -65.31837972369411 degrees.
17:24:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:24:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:24:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:24:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:24:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:24:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:24:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:24:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:24:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:24:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0687269 (min) 0.0815171 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.166984 (min) 0.242912 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0653243 (min) 0.0666338 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.855037 (min) -0.799074 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.467746 (min) -0.427694 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.2891e-06 (min) 9.56563e-06 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.149 (min) 300.54 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:24:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:24:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020207, mean: 0.021697, max: 0.023291
17:24:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:24:29 DEBUG opendrift.models.physics_methods:1061: min: 0.774436, mean: 0.802418, max: 0.831435
17:24:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.774436, mean: 0.802418, max: 0.831435
17:24:29 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:24:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:29 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:24:29 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:24:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.018127 m/s - 0.019461 m/s)
17:24:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:24:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:24:29 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:24:29 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:24:29 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:24:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:29 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:24:29 INFO opendrift.models.basemodel:2032: 2025-01-11 21:00:00 - step 22 of 71 - 1000 active elements (0 deactivated)
17:24:29 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:24:29 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:24:29 DEBUG opendrift.models.basemodel:2051: 59.98548955125115 <- latitude -> 59.99762337450098
17:24:29 DEBUG opendrift.models.basemodel:2056: 4.675919618704093 <- longitude -> 4.685430293816793
17:24:29 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:24:29 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:24:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:24:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:24:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 21:00:00 (before)
2025-01-11 22:00:00 (after)
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:24:42 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x7) for time before (2025-01-11 21:00:00)
17:24:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 21:00:00) in space (linearNDFast)
17:24:42 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:24:42 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:24:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32409128805713 and -65.31458061540204 degrees.
17:24:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32409128805713 and -65.31458061540204 degrees.
17:24:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:24:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:24:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:24:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:24:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:24:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:24:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:24:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:24:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:24:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0856775 (min) 0.0959271 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.157196 (min) 0.238832 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.031382 (min) 0.0325927 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.959363 (min) -0.910001 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0752877 (min) 0.102242 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.75712e-05 (min) -2.70766e-05 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.395 (min) 300.656 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:24:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:24:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020517, mean: 0.021775, max: 0.022893
17:24:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:24:42 DEBUG opendrift.models.physics_methods:1061: min: 0.780339, mean: 0.803877, max: 0.824291
17:24:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.780339, mean: 0.803877, max: 0.824291
17:24:42 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:24:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:42 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:24:42 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:24:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.018265 m/s - 0.019294 m/s)
17:24:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:24:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:24:42 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:24:42 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:24:42 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:24:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:42 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:24:42 INFO opendrift.models.basemodel:2032: 2025-01-11 22:00:00 - step 23 of 71 - 1000 active elements (0 deactivated)
17:24:42 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:24:42 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:24:42 DEBUG opendrift.models.basemodel:2051: 59.99084183543532 <- latitude -> 60.00540584470957
17:24:42 DEBUG opendrift.models.basemodel:2056: 4.680668241039926 <- longitude -> 4.6901155438343025
17:24:42 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:24:42 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:24:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:24:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:24:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 22:00:00 (before)
2025-01-11 23:00:00 (after)
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:24:53 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x23x7) for time before (2025-01-11 22:00:00)
17:24:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 22:00:00) in space (linearNDFast)
17:24:53 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:24:53 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:24:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31934267317374 and -65.30989536433579 degrees.
17:24:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31934267317374 and -65.30989536433579 degrees.
17:24:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:24:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:24:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:24:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:24:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:24:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:24:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:24:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:24:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:24:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:24:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.122055 (min) 0.129515 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.167113 (min) 0.24861 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.130006 (min) -0.129099 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.252262 (min) -0.197244 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.30355 (min) 0.355878 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.80889e-05 (min) -5.76524e-05 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.506 (min) 300.652 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:24:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:24:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.003224, mean: 0.003875, max: 0.004681
17:24:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:24:53 DEBUG opendrift.models.physics_methods:1061: min: 0.309323, mean: 0.338952, max: 0.372734
17:24:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.309323, mean: 0.338952, max: 0.372734
17:24:53 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:24:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:53 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:24:53 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:24:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.007240 m/s - 0.008724 m/s)
17:24:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:24:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:24:53 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:24:53 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:24:53 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:24:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:24:53 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:24:53 INFO opendrift.models.basemodel:2032: 2025-01-11 23:00:00 - step 24 of 71 - 1000 active elements (0 deactivated)
17:24:53 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:24:53 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:24:53 DEBUG opendrift.models.basemodel:2051: 59.9966153363131 <- latitude -> 60.013668753571196
17:24:53 DEBUG opendrift.models.basemodel:2056: 4.68859463250398 <- longitude -> 4.697765192965255
17:24:53 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:24:53 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:24:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:24:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:24:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:24:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:24:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 23:00:00 (before)
2025-01-12 00:00:00 (after)
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:25:07 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x7) for time before (2025-01-11 23:00:00)
17:25:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 23:00:00) in space (linearNDFast)
17:25:07 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:25:07 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:25:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31141627500779 and -65.30224570523316 degrees.
17:25:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31141627500779 and -65.30224570523316 degrees.
17:25:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:25:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:25:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:25:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:25:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:25:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:25:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:25:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:25:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:25:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.137863 (min) 0.162363 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.196234 (min) 0.293395 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.375924 (min) -0.374117 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.00722 (min) 1.01658 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0317464 (min) 0.123029 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.92679e-05 (min) -6.89609e-05 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.048 (min) 300.549 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:25:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:25:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.024981, mean: 0.025302, max: 0.025795
17:25:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:25:07 DEBUG opendrift.models.physics_methods:1061: min: 0.861069, mean: 0.866568, max: 0.874974
17:25:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.861069, mean: 0.866568, max: 0.874974
17:25:07 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:25:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:07 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:25:07 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:25:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.020154 m/s - 0.020480 m/s)
17:25:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:25:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:25:07 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:25:07 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:25:07 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:25:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:07 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:25:07 INFO opendrift.models.basemodel:2032: 2025-01-12 00:00:00 - step 25 of 71 - 1000 active elements (0 deactivated)
17:25:07 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:25:07 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:25:07 DEBUG opendrift.models.basemodel:2051: 60.00306365215231 <- latitude -> 60.02322822246655
17:25:07 DEBUG opendrift.models.basemodel:2056: 4.70037116092166 <- longitude -> 4.707978403457643
17:25:07 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:25:07 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:25:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:25:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:25:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 00:00:00 (before)
2025-01-12 01:00:00 (after)
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:25:21 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x22x7) for time before (2025-01-12 00:00:00)
17:25:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 00:00:00) in space (linearNDFast)
17:25:21 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:25:21 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:25:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29963974331434 and -65.29203249680828 degrees.
17:25:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29963974331434 and -65.29203249680828 degrees.
17:25:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:25:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:25:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:25:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:25:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:25:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:25:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:25:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:25:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:25:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0983149 (min) 0.155663 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.267038 (min) 0.381604 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.600406 (min) -0.596715 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0625515 (min) 0.00858001 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.34036 (min) 1.43305 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.00362e-07 (min) -1.80002e-07 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.255 (min) 300.041 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:25:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:25:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.044197, mean: 0.046961, max: 0.050616
17:25:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:25:21 DEBUG opendrift.models.physics_methods:1061: min: 1.145319, mean: 1.180514, max: 1.225666
17:25:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.145319, mean: 1.180514, max: 1.225666
17:25:21 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:25:21 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:21 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:25:21 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:25:21 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026808 m/s - 0.028688 m/s)
17:25:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:25:21 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:25:21 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:25:21 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:25:21 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:25:21 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:21 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:25:21 INFO opendrift.models.basemodel:2032: 2025-01-12 01:00:00 - step 26 of 71 - 1000 active elements (0 deactivated)
17:25:21 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:25:21 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:25:21 DEBUG opendrift.models.basemodel:2051: 60.01256170158717 <- latitude -> 60.036484651703674
17:25:21 DEBUG opendrift.models.basemodel:2056: 4.71042627553912 <- longitude -> 4.714409056040815
17:25:21 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:25:21 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:25:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:25:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:25:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 01:00:00 (before)
2025-01-12 02:00:00 (after)
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:25:35 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x23x7) for time before (2025-01-12 01:00:00)
17:25:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 01:00:00) in space (linearNDFast)
17:25:35 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:25:35 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:25:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28958462387472 and -65.28560182347294 degrees.
17:25:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28958462387472 and -65.28560182347294 degrees.
17:25:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:25:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:25:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:25:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:25:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:25:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:25:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:25:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:25:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:25:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0353638 (min) 0.114789 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.354211 (min) 0.482208 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.739307 (min) -0.732583 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.825676 (min) -0.779431 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.64432 (min) 1.75175 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.90393e-05 (min) -1.78814e-05 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.722 (min) 299.839 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:25:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:25:35 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.081458, mean: 0.086062, max: 0.092259
17:25:35 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:25:35 DEBUG opendrift.models.physics_methods:1061: min: 1.554876, mean: 1.598130, max: 1.654759
17:25:35 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.554876, mean: 1.598130, max: 1.654759
17:25:35 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:25:35 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:35 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:25:35 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:25:35 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.036394 m/s - 0.038732 m/s)
17:25:35 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:25:35 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:25:35 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:25:35 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:25:35 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:25:35 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:35 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:25:35 INFO opendrift.models.basemodel:2032: 2025-01-12 02:00:00 - step 27 of 71 - 1000 active elements (0 deactivated)
17:25:35 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:25:35 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:25:35 DEBUG opendrift.models.basemodel:2051: 60.02506950462927 <- latitude -> 60.05316249904092
17:25:35 DEBUG opendrift.models.basemodel:2056: 4.715465338465818 <- longitude -> 4.717538864599493
17:25:35 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:25:35 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:25:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:25:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:25:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 02:00:00 (before)
2025-01-12 03:00:00 (after)
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:25:48 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x24x7) for time before (2025-01-12 02:00:00)
17:25:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 02:00:00) in space (linearNDFast)
17:25:48 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:25:48 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:25:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28454554624648 and -65.28247203176903 degrees.
17:25:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28454554624648 and -65.28247203176903 degrees.
17:25:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:25:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:25:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:25:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:25:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:25:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:25:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:25:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:25:48 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:25:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:25:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0225504 (min) 0.0598388 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.461391 (min) 0.591543 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.761775 (min) -0.752015 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.41852 (min) -1.39042 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.20558 (min) 2.30746 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.27559e-06 (min) 6.3233e-06 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.474 (min) 301.514 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:25:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:25:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.167273, mean: 0.172669, max: 0.180480
17:25:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:25:48 DEBUG opendrift.models.physics_methods:1061: min: 2.228138, mean: 2.263743, max: 2.314429
17:25:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.228138, mean: 2.263743, max: 2.314429
17:25:48 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:25:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:48 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:25:48 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:25:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052152 m/s - 0.054172 m/s)
17:25:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:25:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:25:48 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:25:48 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:25:48 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:25:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:25:48 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:25:48 INFO opendrift.models.basemodel:2032: 2025-01-12 03:00:00 - step 28 of 71 - 1000 active elements (0 deactivated)
17:25:48 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:25:48 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:25:48 DEBUG opendrift.models.basemodel:2051: 60.04140335274356 <- latitude -> 60.07374125342362
17:25:48 DEBUG opendrift.models.basemodel:2056: 4.712172860813302 <- longitude -> 4.7189229551326335
17:25:48 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:25:48 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:25:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:25:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:25:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:25:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:25:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 03:00:00 (before)
2025-01-12 04:00:00 (after)
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:26:03 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x24x7) for time before (2025-01-12 03:00:00)
17:26:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 03:00:00) in space (linearNDFast)
17:26:03 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:26:03 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:26:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28783801593059 and -65.28108793369832 degrees.
17:26:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28783801593059 and -65.28108793369832 degrees.
17:26:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:26:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:26:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:26:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:26:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:26:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:26:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:26:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:26:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:26:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0750173 (min) -6.32956e-05 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.577903 (min) 0.692958 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.707647 (min) -0.696004 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.87429 (min) -1.59851 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.06374 (min) 3.29558 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.27182e-05 (min) 3.39482e-05 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.179 (min) 302.611 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:26:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:26:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.293766, mean: 0.324112, max: 0.353596
17:26:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:26:03 DEBUG opendrift.models.physics_methods:1061: min: 2.952777, mean: 3.101134, max: 3.239538
17:26:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.952777, mean: 3.101134, max: 3.239538
17:26:03 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:26:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:03 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:26:03 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:26:03 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.069114 m/s - 0.075826 m/s)
17:26:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:26:03 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:26:03 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:26:03 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:26:03 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:26:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:03 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:26:03 INFO opendrift.models.basemodel:2032: 2025-01-12 04:00:00 - step 29 of 71 - 1000 active elements (0 deactivated)
17:26:03 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:26:03 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:26:03 DEBUG opendrift.models.basemodel:2051: 60.06205653871415 <- latitude -> 60.09826172719717
17:26:03 DEBUG opendrift.models.basemodel:2056: 4.704893313180323 <- longitude -> 4.716852401475014
17:26:03 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:26:03 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:26:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:26:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:26:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 04:00:00 (before)
2025-01-12 05:00:00 (after)
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:26:17 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x7) for time before (2025-01-12 04:00:00)
17:26:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 04:00:00) in space (linearNDFast)
17:26:17 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:26:17 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:26:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.2951175556008 and -65.28315847698772 degrees.
17:26:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.2951175556008 and -65.28315847698772 degrees.
17:26:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:26:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:26:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:26:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:26:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:26:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:26:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:26:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:26:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:26:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0825795 (min) -0.0588259 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.696508 (min) 0.788064 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.572588 (min) -0.560278 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.47053 (min) -1.21054 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: 4.7619 (min) 4.8641 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.16506e-05 (min) 4.31121e-05 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 302.246 (min) 303.099 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:26:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:26:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.598855, mean: 0.605279, max: 0.635219
17:26:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:26:17 DEBUG opendrift.models.physics_methods:1061: min: 4.215900, mean: 4.238379, max: 4.342014
17:26:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.215900, mean: 4.238379, max: 4.342014
17:26:17 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:26:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:17 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:26:17 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:26:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.098679 m/s - 0.101630 m/s)
17:26:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:26:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:26:17 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:26:17 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:26:17 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:26:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:17 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:26:17 INFO opendrift.models.basemodel:2032: 2025-01-12 05:00:00 - step 30 of 71 - 1000 active elements (0 deactivated)
17:26:17 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:26:17 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:26:17 DEBUG opendrift.models.basemodel:2051: 60.08770544005195 <- latitude -> 60.12673174754035
17:26:17 DEBUG opendrift.models.basemodel:2056: 4.698349143946271 <- longitude -> 4.711144966687878
17:26:17 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:26:17 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:26:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:26:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:26:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 05:00:00 (before)
2025-01-12 06:00:00 (after)
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:26:29 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 28x25x7) for time before (2025-01-12 05:00:00)
17:26:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 05:00:00) in space (linearNDFast)
17:26:29 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:26:29 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:26:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30166172694715 and -65.2888659050958 degrees.
17:26:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30166172694715 and -65.2888659050958 degrees.
17:26:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:26:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:26:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:26:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:26:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:26:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:26:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:26:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:26:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:26:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0669709 (min) -0.0567031 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.800462 (min) 0.840117 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.411636 (min) -0.405704 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.6175 (min) -1.53761 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: 5.65841 (min) 5.8498 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.1563e-05 (min) 5.34094e-05 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 303.389 (min) 304.093 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:26:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:26:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.845793, mean: 0.884081, max: 0.906124
17:26:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:26:29 DEBUG opendrift.models.physics_methods:1061: min: 5.010277, mean: 5.122309, max: 5.185890
17:26:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.010277, mean: 5.122309, max: 5.185890
17:26:29 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:26:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:29 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:26:29 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:26:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.117272 m/s - 0.121383 m/s)
17:26:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:26:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:26:29 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:26:29 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:26:29 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:26:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:29 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:26:29 INFO opendrift.models.basemodel:2032: 2025-01-12 06:00:00 - step 31 of 71 - 1000 active elements (0 deactivated)
17:26:29 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:26:29 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:26:29 DEBUG opendrift.models.basemodel:2051: 60.117350160523884 <- latitude -> 60.15739543957562
17:26:29 DEBUG opendrift.models.basemodel:2056: 4.692657592176683 <- longitude -> 4.704756849414703
17:26:29 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:26:29 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:26:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:26:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:26:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 06:00:00 (before)
2025-01-12 07:00:00 (after)
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:26:47 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x7) for time before (2025-01-12 06:00:00)
17:26:47 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 06:00:00) in space (linearNDFast)
17:26:47 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:26:47 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:26:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30735326905285 and -65.29525402217521 degrees.
17:26:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30735326905285 and -65.29525402217521 degrees.
17:26:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:26:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:26:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:26:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:26:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:26:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:26:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:26:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:26:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:26:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:26:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0379247 (min) -0.0323833 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.827463 (min) 0.849614 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.218842 (min) -0.217434 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.51695 (min) -1.38097 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: 6.01337 (min) 6.72191 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.28168e-05 (min) 5.52006e-05 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 304.562 (min) 305.202 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:26:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:26:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.946159, mean: 1.060727, max: 1.158452
17:26:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:26:47 DEBUG opendrift.models.physics_methods:1061: min: 5.299217, mean: 5.610017, max: 5.863659
17:26:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.299217, mean: 5.610017, max: 5.863659
17:26:47 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:26:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:47 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:26:47 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:26:47 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.124035 m/s - 0.137247 m/s)
17:26:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:26:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:26:47 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:26:47 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:26:47 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:26:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:26:47 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:26:47 INFO opendrift.models.basemodel:2032: 2025-01-12 07:00:00 - step 32 of 71 - 1000 active elements (0 deactivated)
17:26:47 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:26:47 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:26:47 DEBUG opendrift.models.basemodel:2051: 60.14843085320513 <- latitude -> 60.18856655532913
17:26:47 DEBUG opendrift.models.basemodel:2056: 4.688228537427647 <- longitude -> 4.700773135182342
17:26:47 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:26:47 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:26:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:26:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:26:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:26:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:26:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 07:00:00 (before)
2025-01-12 08:00:00 (after)
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:27:05 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x7) for time before (2025-01-12 07:00:00)
17:27:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 07:00:00) in space (linearNDFast)
17:27:05 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:27:05 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:27:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31178230224711 and -65.299237726361 degrees.
17:27:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31178230224711 and -65.299237726361 degrees.
17:27:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:27:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:27:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:27:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:27:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:27:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:27:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:27:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:27:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:27:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00990526 (min) -0.00489199 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.790768 (min) 0.82119 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0275823 (min) -0.0248983 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.51146 (min) -2.00146 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: 6.52107 (min) 7.00142 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.17743e-05 (min) 5.24669e-05 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 301.208 (min) 306.141 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:27:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:27:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.201261, mean: 1.240514, max: 1.304434
17:27:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:27:05 DEBUG opendrift.models.physics_methods:1061: min: 5.971017, mean: 6.067621, max: 6.222151
17:27:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.971017, mean: 6.067621, max: 6.222151
17:27:05 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:27:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:05 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:27:05 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:27:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.139759 m/s - 0.145638 m/s)
17:27:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:27:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:27:05 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:27:05 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:27:05 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:27:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:05 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:27:05 INFO opendrift.models.basemodel:2032: 2025-01-12 08:00:00 - step 33 of 71 - 1000 active elements (0 deactivated)
17:27:05 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:27:05 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:27:05 DEBUG opendrift.models.basemodel:2051: 60.179260639132096 <- latitude -> 60.21833144611796
17:27:05 DEBUG opendrift.models.basemodel:2056: 4.684442308677881 <- longitude -> 4.697839157335178
17:27:05 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:27:05 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:27:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:27:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:27:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 08:00:00 (before)
2025-01-12 09:00:00 (after)
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:27:21 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 28x25x7) for time before (2025-01-12 08:00:00)
17:27:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 08:00:00) in space (linearNDFast)
17:27:21 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:27:21 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:27:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31556852728805 and -65.30217168929084 degrees.
17:27:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31556852728805 and -65.30217168929084 degrees.
17:27:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:27:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:27:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:27:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:27:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:27:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:27:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:27:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:27:21 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:27:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:27:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0306295 (min) 0.0400336 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.670798 (min) 0.760654 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.120944 (min) 0.127892 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.95904 (min) -1.7432 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.40493 (min) 8.48849 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.46046e-05 (min) 2.53108e-05 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:27:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.335 (min) 302.462 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:27:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:27:22 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.812566, mean: 1.851070, max: 1.858539
17:27:22 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:27:22 DEBUG opendrift.models.physics_methods:1061: min: 7.334602, mean: 7.412083, max: 7.427033
17:27:22 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.334602, mean: 7.412083, max: 7.427033
17:27:22 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:27:22 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:22 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:27:22 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:27:22 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.171676 m/s - 0.173839 m/s)
17:27:22 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:27:22 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:27:22 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:27:22 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:27:22 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:27:22 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:22 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:27:22 INFO opendrift.models.basemodel:2032: 2025-01-12 09:00:00 - step 34 of 71 - 1000 active elements (0 deactivated)
17:27:22 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:27:22 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:27:22 DEBUG opendrift.models.basemodel:2051: 60.209245131495535 <- latitude -> 60.24547081332363
17:27:22 DEBUG opendrift.models.basemodel:2056: 4.684497309264781 <- longitude -> 4.697619070428
17:27:22 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:27:22 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:27:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:27:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:27:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:27:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 09:00:00 (before)
2025-01-12 10:00:00 (after)
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:27:34 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['x_sea_water_velocity', 'y_sea_water_velocity']
17:27:34 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x7) for time before (2025-01-12 09:00:00)
17:27:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 09:00:00) in space (linearNDFast)
17:27:34 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:27:34 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:27:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31551351370236 and -65.30239177181204 degrees.
17:27:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31551351370236 and -65.30239177181204 degrees.
17:27:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:27:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:27:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:27:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:27:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:27:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:27:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:27:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:27:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:27:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0580166 (min) 0.0616742 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.53561 (min) 0.642914 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.147769 (min) 0.152507 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.29525 (min) -2.25824 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.42585 (min) 8.49115 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.30492e-05 (min) -1.2805e-05 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.135 (min) 304.615 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:27:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:27:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.874159, mean: 1.881681, max: 1.901230
17:27:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:27:34 DEBUG opendrift.models.physics_methods:1061: min: 7.458180, mean: 7.473126, max: 7.511851
17:27:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.458180, mean: 7.473126, max: 7.511851
17:27:34 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:27:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:34 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:27:34 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:27:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.174568 m/s - 0.175825 m/s)
17:27:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:27:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:27:34 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:27:34 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:27:34 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:27:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:34 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:27:34 INFO opendrift.models.basemodel:2032: 2025-01-12 10:00:00 - step 35 of 71 - 1000 active elements (0 deactivated)
17:27:34 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:27:34 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:27:34 DEBUG opendrift.models.basemodel:2051: 60.23546898683253 <- latitude -> 60.268264073007195
17:27:34 DEBUG opendrift.models.basemodel:2056: 4.685432030069987 <- longitude -> 4.69869115623599
17:27:34 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:27:34 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:27:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:27:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:27:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 10:00:00 (before)
2025-01-12 11:00:00 (after)
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:27:51 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:27:51 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x7) for time before (2025-01-12 10:00:00)
17:27:51 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 10:00:00) in space (linearNDFast)
17:27:51 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:27:51 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:27:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31457879297741 and -65.30131967274284 degrees.
17:27:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31457879297741 and -65.30131967274284 degrees.
17:27:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:27:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:27:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:27:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:27:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:27:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:27:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:27:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:27:51 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:27:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:27:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0540407 (min) 0.0673612 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.428113 (min) 0.548044 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0258713 (min) 0.0277232 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.56553 (min) -2.55642 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.75261 (min) 8.81605 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.58344e-05 (min) -5.50427e-05 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 303.518 (min) 310.828 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:27:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:27:51 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.045329, mean: 2.061391, max: 2.072946
17:27:51 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:27:51 DEBUG opendrift.models.physics_methods:1061: min: 7.791321, mean: 7.821852, max: 7.843747
17:27:51 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.791321, mean: 7.821852, max: 7.843747
17:27:51 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:27:51 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:51 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:27:51 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:27:51 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.182366 m/s - 0.183593 m/s)
17:27:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:27:51 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:27:51 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:27:51 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:27:51 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:27:51 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:27:51 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:27:51 INFO opendrift.models.basemodel:2032: 2025-01-12 11:00:00 - step 36 of 71 - 1000 active elements (0 deactivated)
17:27:51 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:27:51 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:27:51 DEBUG opendrift.models.basemodel:2051: 60.258874011198756 <- latitude -> 60.2877528554113
17:27:51 DEBUG opendrift.models.basemodel:2056: 4.686487535697327 <- longitude -> 4.698877849332712
17:27:51 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:27:51 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:27:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:27:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:27:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:27:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:27:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 11:00:00 (before)
2025-01-12 12:00:00 (after)
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:28:04 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:28:04 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x24x7) for time before (2025-01-12 11:00:00)
17:28:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 11:00:00) in space (linearNDFast)
17:28:04 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:28:04 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:28:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31352328059126 and -65.30113297013638 degrees.
17:28:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31352328059126 and -65.30113297013638 degrees.
17:28:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:28:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:28:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:28:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:28:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:28:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:28:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:28:04 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:28:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:28:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0695205 (min) 0.0795583 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.351909 (min) 0.493998 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.222474 (min) -0.220803 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.34666 (min) -1.83547 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.21562 (min) 9.48396 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.56074e-05 (min) -7.46898e-05 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 309.81 (min) 317.96 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:28:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:28:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.224690, mean: 2.254206, max: 2.295536
17:28:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:28:04 DEBUG opendrift.models.physics_methods:1061: min: 8.125766, mean: 8.179460, max: 8.254136
17:28:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.125766, mean: 8.179460, max: 8.254136
17:28:04 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:28:04 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:04 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:28:04 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:28:04 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.190194 m/s - 0.193199 m/s)
17:28:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:28:04 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:28:04 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:28:04 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:28:04 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:28:04 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:04 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:28:04 INFO opendrift.models.basemodel:2032: 2025-01-12 12:00:00 - step 37 of 71 - 1000 active elements (0 deactivated)
17:28:04 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:28:04 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:28:04 DEBUG opendrift.models.basemodel:2051: 60.28096426341709 <- latitude -> 60.305078547343925
17:28:04 DEBUG opendrift.models.basemodel:2056: 4.688264555863092 <- longitude -> 4.701011976051709
17:28:04 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:28:04 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:28:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:28:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:28:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 12:00:00 (before)
2025-01-12 13:00:00 (after)
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:28:17 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:28:17 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x24x7) for time before (2025-01-12 12:00:00)
17:28:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 12:00:00) in space (linearNDFast)
17:28:17 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:28:17 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:28:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31174624939825 and -65.29899884231736 degrees.
17:28:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31174624939825 and -65.29899884231736 degrees.
17:28:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:28:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:28:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:28:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:28:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:28:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:28:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:28:17 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:28:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:28:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0678737 (min) 0.0823219 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.342259 (min) 0.452552 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.490318 (min) -0.487601 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.89121 (min) -1.73269 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.68609 (min) 9.70518 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.80371e-05 (min) -6.74822e-05 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 317.754 (min) 324.444 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:28:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:28:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.390556, mean: 2.393769, max: 2.402895
17:28:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:28:17 DEBUG opendrift.models.physics_methods:1061: min: 8.423237, mean: 8.428895, max: 8.444947
17:28:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.423237, mean: 8.428895, max: 8.444947
17:28:17 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:28:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:17 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:28:17 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:28:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.197157 m/s - 0.197665 m/s)
17:28:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:28:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:28:17 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:28:17 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:28:17 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:28:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:17 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:28:17 INFO opendrift.models.basemodel:2032: 2025-01-12 13:00:00 - step 38 of 71 - 1000 active elements (0 deactivated)
17:28:17 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:28:17 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:28:17 DEBUG opendrift.models.basemodel:2051: 60.30185818931325 <- latitude -> 60.32240584166583
17:28:17 DEBUG opendrift.models.basemodel:2056: 4.6902214659585395 <- longitude -> 4.704019560333252
17:28:17 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:28:17 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:28:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:28:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:28:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 13:00:00 (before)
2025-01-12 14:00:00 (after)
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:28:30 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:28:30 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x7) for time before (2025-01-12 13:00:00)
17:28:30 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 13:00:00) in space (linearNDFast)
17:28:30 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:28:30 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:28:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30978932672696 and -65.29599124515289 degrees.
17:28:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30978932672696 and -65.29599124515289 degrees.
17:28:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:28:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:28:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:28:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:28:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:28:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:28:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:28:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:28:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:28:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0547786 (min) 0.0651769 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.366115 (min) 0.445125 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.699744 (min) -0.695497 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.44172 (min) -1.43014 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.97719 (min) 10.0373 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.27324e-05 (min) -4.23868e-05 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 325.422 (min) 328.497 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:28:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:28:30 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.499593, mean: 2.513249, max: 2.529262
17:28:30 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:28:30 DEBUG opendrift.models.physics_methods:1061: min: 8.613195, mean: 8.636685, max: 8.664161
17:28:30 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.613195, mean: 8.636685, max: 8.664161
17:28:30 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:28:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:30 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:28:30 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:28:30 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.201603 m/s - 0.202796 m/s)
17:28:30 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:28:30 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:28:30 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:28:30 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:28:30 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:28:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:30 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:28:30 INFO opendrift.models.basemodel:2032: 2025-01-12 14:00:00 - step 39 of 71 - 1000 active elements (0 deactivated)
17:28:30 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:28:30 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:28:30 DEBUG opendrift.models.basemodel:2051: 60.32272680032973 <- latitude -> 60.34068265722416
17:28:30 DEBUG opendrift.models.basemodel:2056: 4.692027110652659 <- longitude -> 4.706391478220369
17:28:30 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:28:30 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:28:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:28:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:28:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 14:00:00 (before)
2025-01-12 15:00:00 (after)
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:28:43 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:28:43 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x7) for time before (2025-01-12 14:00:00)
17:28:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 14:00:00) in space (linearNDFast)
17:28:43 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:28:43 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:28:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3079836830224 and -65.29361932331219 degrees.
17:28:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3079836830224 and -65.29361932331219 degrees.
17:28:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:28:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:28:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:28:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:28:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:28:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:28:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:28:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:28:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:28:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0129283 (min) 0.0194898 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.429737 (min) 0.49114 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.794319 (min) -0.788812 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.70262 (min) -2.60698 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.344 (min) 10.4032 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.75402e-06 (min) -5.39987e-06 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 327.426 (min) 329.465 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:28:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:28:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.811841, mean: 2.825762, max: 2.830028
17:28:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:28:43 DEBUG opendrift.models.physics_methods:1061: min: 9.135345, mean: 9.157930, max: 9.164842
17:28:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.135345, mean: 9.157930, max: 9.164842
17:28:43 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:28:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:43 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:28:43 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:28:43 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.213825 m/s - 0.214515 m/s)
17:28:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:28:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:28:43 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:28:43 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:28:43 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:28:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:43 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:28:43 INFO opendrift.models.basemodel:2032: 2025-01-12 15:00:00 - step 40 of 71 - 1000 active elements (0 deactivated)
17:28:43 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:28:43 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:28:43 DEBUG opendrift.models.basemodel:2051: 60.34528031042049 <- latitude -> 60.36132617043075
17:28:43 DEBUG opendrift.models.basemodel:2056: 4.689897139530516 <- longitude -> 4.703981743313033
17:28:43 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:28:43 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:28:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:28:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:28:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 15:00:00 (before)
2025-01-12 16:00:00 (after)
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:28:57 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:28:57 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-12 15:00:00)
17:28:57 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 15:00:00) in space (linearNDFast)
17:28:57 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:28:57 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:28:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31011365058285 and -65.29602904434346 degrees.
17:28:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31011365058285 and -65.29602904434346 degrees.
17:28:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:28:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:28:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:28:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:28:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:28:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:28:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:28:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:28:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:28:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:28:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0169856 (min) -0.00639939 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.553708 (min) 0.578767 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.769788 (min) -0.763338 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.79361 (min) -2.75361 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.908 (min) 11.0199 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.89164e-05 (min) 1.91106e-05 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 323.96 (min) 326.375 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:28:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:28:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.117072, mean: 3.151479, max: 3.176685
17:28:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:28:57 DEBUG opendrift.models.physics_methods:1061: min: 9.618403, mean: 9.671326, max: 9.709942
17:28:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.618403, mean: 9.671326, max: 9.709942
17:28:57 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:28:57 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:57 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:28:57 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:28:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.225131 m/s - 0.227274 m/s)
17:28:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:28:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:28:57 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:28:57 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:28:57 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:28:57 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:28:57 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:28:57 INFO opendrift.models.basemodel:2032: 2025-01-12 16:00:00 - step 41 of 71 - 1000 active elements (0 deactivated)
17:28:57 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:28:57 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:28:57 DEBUG opendrift.models.basemodel:2051: 60.37102950620877 <- latitude -> 60.38648075439158
17:28:57 DEBUG opendrift.models.basemodel:2056: 4.685857965025721 <- longitude -> 4.6992880814919955
17:28:57 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:28:57 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:28:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:28:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:28:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:28:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:28:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 16:00:00 (before)
2025-01-12 17:00:00 (after)
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:29:10 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:29:10 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-12 16:00:00)
17:29:10 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 16:00:00) in space (linearNDFast)
17:29:10 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:29:10 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:29:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31415280902613 and -65.30072270525523 degrees.
17:29:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31415280902613 and -65.30072270525523 degrees.
17:29:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:29:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:29:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:29:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:29:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:29:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:29:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:29:10 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:29:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:29:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.011506 (min) 0.00978421 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.684894 (min) 0.694123 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.663693 (min) -0.657033 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.26035 (min) -2.18542 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1279 (min) 11.1891 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.79649e-05 (min) 3.8007e-05 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 323.086 (min) 327.954 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:29:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:29:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.171904, mean: 3.184964, max: 3.198747
17:29:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:29:10 DEBUG opendrift.models.physics_methods:1061: min: 9.702633, mean: 9.722584, max: 9.743602
17:29:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.702633, mean: 9.722584, max: 9.743602
17:29:10 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:29:10 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:10 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:29:10 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:29:10 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.227103 m/s - 0.228062 m/s)
17:29:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:29:10 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:29:10 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:29:10 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:29:10 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:29:10 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:10 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:29:10 INFO opendrift.models.basemodel:2032: 2025-01-12 17:00:00 - step 42 of 71 - 1000 active elements (0 deactivated)
17:29:10 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:29:10 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:29:10 DEBUG opendrift.models.basemodel:2051: 60.4005418510947 <- latitude -> 60.41613865240457
17:29:10 DEBUG opendrift.models.basemodel:2056: 4.683624148588222 <- longitude -> 4.695594596685294
17:29:10 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:29:10 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:29:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:29:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:29:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 17:00:00 (before)
2025-01-12 18:00:00 (after)
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:29:27 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:29:27 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x7) for time before (2025-01-12 17:00:00)
17:29:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 17:00:00) in space (linearNDFast)
17:29:27 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:29:27 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:29:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31638662577456 and -65.30441618289754 degrees.
17:29:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31638662577456 and -65.30441618289754 degrees.
17:29:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:29:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:29:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:29:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:29:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:29:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:29:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:29:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:29:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:29:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0259912 (min) 0.0708196 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.786532 (min) 0.791573 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.506792 (min) -0.500516 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.45644 (min) -3.37378 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.2261 (min) 11.3253 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.15472e-05 (min) 5.19408e-05 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 330.781 (min) 338.268 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:29:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:29:27 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.394120, mean: 3.415139, max: 3.435241
17:29:27 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:29:27 DEBUG opendrift.models.physics_methods:1061: min: 10.036752, mean: 10.067776, max: 10.097369
17:29:27 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.036752, mean: 10.067776, max: 10.097369
17:29:27 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:29:27 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:27 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:29:27 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:29:27 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.234923 m/s - 0.236342 m/s)
17:29:27 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:29:27 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:29:27 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:29:27 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:29:27 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:29:27 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:27 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:29:27 INFO opendrift.models.basemodel:2032: 2025-01-12 18:00:00 - step 43 of 71 - 1000 active elements (0 deactivated)
17:29:27 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:29:27 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:29:27 DEBUG opendrift.models.basemodel:2051: 60.4332828899768 <- latitude -> 60.44903291797765
17:29:27 DEBUG opendrift.models.basemodel:2056: 4.683841814782847 <- longitude -> 4.692774190587349
17:29:27 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:29:27 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:29:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:29:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:29:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 18:00:00 (before)
2025-01-12 19:00:00 (after)
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:29:46 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:29:46 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x7) for time before (2025-01-12 18:00:00)
17:29:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 18:00:00) in space (linearNDFast)
17:29:46 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:29:46 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:29:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31616894444883 and -65.30723657483256 degrees.
17:29:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31616894444883 and -65.30723657483256 degrees.
17:29:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:29:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:29:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:29:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:29:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:29:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:29:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:29:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:29:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:29:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:29:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0884128 (min) 0.134991 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.805271 (min) 0.816179 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.292394 (min) -0.287724 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.1627 (min) -4.14472 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.5964 (min) 11.6828 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.54535e-05 (min) 6.58894e-05 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 332.655 (min) 334.239 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:29:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:29:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.734393, mean: 3.756398, max: 3.780199
17:29:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:29:46 DEBUG opendrift.models.physics_methods:1061: min: 10.527848, mean: 10.558814, max: 10.592218
17:29:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.527848, mean: 10.558814, max: 10.592218
17:29:46 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:29:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:46 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:29:46 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:29:46 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.246418 m/s - 0.247925 m/s)
17:29:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:29:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:29:46 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:29:46 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:29:46 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:29:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:29:46 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:29:46 INFO opendrift.models.basemodel:2032: 2025-01-12 19:00:00 - step 44 of 71 - 1000 active elements (0 deactivated)
17:29:46 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:29:46 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:29:46 DEBUG opendrift.models.basemodel:2051: 60.467007123762 <- latitude -> 60.482600380860006
17:29:46 DEBUG opendrift.models.basemodel:2056: 4.68711058504122 <- longitude -> 4.693110443546115
17:29:46 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:29:46 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:29:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:29:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:29:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:29:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:29:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 19:00:00 (before)
2025-01-12 20:00:00 (after)
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:30:03 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:30:03 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-12 19:00:00)
17:30:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 19:00:00) in space (linearNDFast)
17:30:03 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:30:03 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:30:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3129001637181 and -65.30690030776138 degrees.
17:30:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3129001637181 and -65.30690030776138 degrees.
17:30:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:30:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:30:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:30:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:30:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:30:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:30:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:30:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:30:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:30:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.146278 (min) 0.170875 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.66489 (min) 0.810602 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0593768 (min) -0.0545353 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.52484 (min) -3.52127 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: 12.9889 (min) 13.0328 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.43664e-05 (min) 6.44971e-05 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 314.592 (min) 317.778 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:30:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:30:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.455751, mean: 4.473482, max: 4.483967
17:30:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:30:03 DEBUG opendrift.models.physics_methods:1061: min: 11.499794, mean: 11.522648, max: 11.536147
17:30:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.499794, mean: 11.522648, max: 11.536147
17:30:03 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:30:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:03 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:30:03 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:30:03 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.269168 m/s - 0.270019 m/s)
17:30:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:30:03 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:30:03 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:30:03 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:30:03 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:30:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:03 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:30:03 INFO opendrift.models.basemodel:2032: 2025-01-12 20:00:00 - step 45 of 71 - 1000 active elements (0 deactivated)
17:30:03 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:30:03 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:30:03 DEBUG opendrift.models.basemodel:2051: 60.50159061840708 <- latitude -> 60.51250404518839
17:30:03 DEBUG opendrift.models.basemodel:2056: 4.692215959174207 <- longitude -> 4.6988568981532435
17:30:03 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:30:03 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:30:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:30:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:30:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 20:00:00 (before)
2025-01-12 21:00:00 (after)
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:30:16 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:30:16 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-12 20:00:00)
17:30:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 20:00:00) in space (linearNDFast)
17:30:16 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:30:16 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:30:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30779477623194 and -65.30115384172868 degrees.
17:30:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30779477623194 and -65.30115384172868 degrees.
17:30:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:30:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:30:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:30:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:30:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:30:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:30:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:30:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:30:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:30:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0329776 (min) 0.106547 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.593508 (min) 0.669557 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.137107 (min) 0.138657 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.68949 (min) -3.65646 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: 13.3315 (min) 13.4299 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.94848e-05 (min) 3.97599e-05 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 294.212 (min) 296.529 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:30:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:30:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.702021, mean: 4.737369, max: 4.771760
17:30:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:30:16 DEBUG opendrift.models.physics_methods:1061: min: 11.813316, mean: 11.857628, max: 11.900600
17:30:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.813316, mean: 11.857628, max: 11.900600
17:30:16 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:30:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:16 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:30:16 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:30:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.276506 m/s - 0.278549 m/s)
17:30:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:30:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:30:16 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:30:16 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:30:16 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:30:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:16 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:30:16 INFO opendrift.models.basemodel:2032: 2025-01-12 21:00:00 - step 46 of 71 - 1000 active elements (0 deactivated)
17:30:16 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:30:16 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:30:16 DEBUG opendrift.models.basemodel:2051: 60.53183845836314 <- latitude -> 60.54038594965458
17:30:16 DEBUG opendrift.models.basemodel:2056: 4.685213803725041 <- longitude -> 4.701039244835985
17:30:16 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:30:16 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:30:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:30:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:30:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 21:00:00 (before)
2025-01-12 22:00:00 (after)
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:30:29 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:30:29 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-12 21:00:00)
17:30:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 21:00:00) in space (linearNDFast)
17:30:29 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:30:29 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:30:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31479693242214 and -65.29897148538589 degrees.
17:30:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31479693242214 and -65.29897148538589 degrees.
17:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:30:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:30:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:30:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:30:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:30:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:30:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:30:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:30:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:30:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.265168 (min) -0.22391 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.614575 (min) 0.646819 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.220053 (min) 0.223261 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.41488 (min) -4.28743 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: 14.7839 (min) 14.9114 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.36052e-07 (min) 1.17957e-06 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 271.513 (min) 287.475 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:30:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:30:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 5.856120, mean: 5.895848, max: 5.922023
17:30:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:30:29 DEBUG opendrift.models.physics_methods:1061: min: 13.183617, mean: 13.228252, max: 13.257592
17:30:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.183617, mean: 13.228252, max: 13.257592
17:30:29 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:30:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:29 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:30:29 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:30:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.308580 m/s - 0.310311 m/s)
17:30:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:30:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:30:29 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:30:29 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:30:29 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:30:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:29 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:30:29 INFO opendrift.models.basemodel:2032: 2025-01-12 22:00:00 - step 47 of 71 - 1000 active elements (0 deactivated)
17:30:29 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:30:29 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:30:29 DEBUG opendrift.models.basemodel:2051: 60.562289315800115 <- latitude -> 60.56998268415134
17:30:29 DEBUG opendrift.models.basemodel:2056: 4.6621817774144265 <- longitude -> 4.680552409016256
17:30:29 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:30:29 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:30:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:30:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:30:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 22:00:00 (before)
2025-01-12 23:00:00 (after)
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:30:42 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:30:42 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x7) for time before (2025-01-12 22:00:00)
17:30:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 22:00:00) in space (linearNDFast)
17:30:42 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:30:42 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:30:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33782894272488 and -65.31945831639092 degrees.
17:30:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33782894272488 and -65.31945831639092 degrees.
17:30:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:30:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:30:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:30:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:30:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:30:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:30:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:30:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:30:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:30:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.440736 (min) -0.37417 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.811005 (min) 0.822173 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.133596 (min) 0.138673 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.94814 (min) -2.82614 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: 15.5926 (min) 15.6332 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.08977e-05 (min) -5.03071e-05 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 277.054 (min) 297.588 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:30:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:30:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.194805, mean: 6.203357, max: 6.211655
17:30:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:30:42 DEBUG opendrift.models.physics_methods:1061: min: 13.559492, mean: 13.568848, max: 13.577919
17:30:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.559492, mean: 13.568848, max: 13.577919
17:30:42 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:30:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:42 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:30:42 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:30:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.317378 m/s - 0.317809 m/s)
17:30:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:30:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:30:42 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:30:42 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:30:42 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:30:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:42 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:30:42 INFO opendrift.models.basemodel:2032: 2025-01-12 23:00:00 - step 48 of 71 - 1000 active elements (0 deactivated)
17:30:42 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:30:42 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:30:42 DEBUG opendrift.models.basemodel:2051: 60.59871237065026 <- latitude -> 60.606569218970236
17:30:42 DEBUG opendrift.models.basemodel:2056: 4.633855094756503 <- longitude -> 4.647727704845753
17:30:42 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:30:42 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:30:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:30:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:30:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 23:00:00 (before)
2025-01-13 00:00:00 (after)
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:30:54 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:30:54 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-12 23:00:00)
17:30:54 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 23:00:00) in space (linearNDFast)
17:30:54 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:30:54 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:30:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36615561218524 and -65.35228300242211 degrees.
17:30:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36615561218524 and -65.35228300242211 degrees.
17:30:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:30:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:30:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:30:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:30:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:30:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:30:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:30:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:30:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:30:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:30:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.303677 (min) -0.248865 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.0102 (min) 1.0424 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.107974 (min) -0.104342 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.27042 (min) -2.26192 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: 15.998 (min) 16.099 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.73232e-05 (min) -7.72554e-05 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.503 (min) 313.377 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:30:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:30:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.421869, mean: 6.449458, max: 6.502606
17:30:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:30:54 DEBUG opendrift.models.physics_methods:1061: min: 13.805759, mean: 13.835374, max: 13.892272
17:30:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.805759, mean: 13.835374, max: 13.892272
17:30:54 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:30:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:54 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:30:54 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:30:54 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.323142 m/s - 0.325167 m/s)
17:30:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:30:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:30:54 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:30:54 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:30:54 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:30:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:30:54 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:30:54 INFO opendrift.models.basemodel:2032: 2025-01-13 00:00:00 - step 49 of 71 - 1000 active elements (0 deactivated)
17:30:54 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:30:54 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:30:54 DEBUG opendrift.models.basemodel:2051: 60.64279313499647 <- latitude -> 60.64968471518813
17:30:54 DEBUG opendrift.models.basemodel:2056: 4.6145051758327815 <- longitude -> 4.624765494848029
17:30:54 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:30:54 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:30:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:30:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:30:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:30:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:30:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 00:00:00 (before)
2025-01-13 01:00:00 (after)
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:31:12 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:31:12 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 00:00:00)
17:31:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 00:00:00) in space (linearNDFast)
17:31:12 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:31:12 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:31:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38550552394587 and -65.37524519658372 degrees.
17:31:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38550552394587 and -65.37524519658372 degrees.
17:31:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:31:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:31:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:31:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:31:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:31:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:31:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:31:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:31:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:31:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0968727 (min) -0.0817094 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.00641 (min) 1.05338 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.397135 (min) -0.393726 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.10438 (min) -2.96592 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 16.9365 (min) 16.9928 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.72643e-06 (min) 1.93772e-06 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 304.303 (min) 315.434 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:31:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:31:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.273295, mean: 7.294432, max: 7.340438
17:31:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:31:12 DEBUG opendrift.models.physics_methods:1061: min: 14.692484, mean: 14.713809, max: 14.760143
17:31:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 14.692484, mean: 14.713809, max: 14.760143
17:31:12 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:31:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:12 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:31:12 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:31:12 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.343897 m/s - 0.345480 m/s)
17:31:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:31:12 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:31:12 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:31:12 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:31:12 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:31:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:12 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:31:12 INFO opendrift.models.basemodel:2032: 2025-01-13 01:00:00 - step 50 of 71 - 1000 active elements (0 deactivated)
17:31:12 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:31:12 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:31:12 DEBUG opendrift.models.basemodel:2051: 60.68780705476017 <- latitude -> 60.69314750225147
17:31:12 DEBUG opendrift.models.basemodel:2056: 4.605211228713572 <- longitude -> 4.614294000360602
17:31:12 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:31:12 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:31:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:31:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:31:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 01:00:00 (before)
2025-01-13 02:00:00 (after)
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:31:31 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:31:31 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 01:00:00)
17:31:31 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 01:00:00) in space (linearNDFast)
17:31:31 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:31:31 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:31:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39479945760225 and -65.38571668623231 degrees.
17:31:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39479945760225 and -65.38571668623231 degrees.
17:31:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:31:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:31:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:31:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:31:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:31:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:31:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:31:31 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:31:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:31:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0989551 (min) -0.0852099 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.01143 (min) 1.03385 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.651666 (min) -0.646849 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.04677 (min) -3.74806 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.8254 (min) 18.064 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.11622e-05 (min) -6.09723e-05 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 272.506 (min) 283.541 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:31:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:31:31 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.219414, mean: 8.321775, max: 8.372796
17:31:31 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:31:31 DEBUG opendrift.models.physics_methods:1061: min: 15.618883, mean: 15.715813, max: 15.763941
17:31:31 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.618883, mean: 15.715813, max: 15.763941
17:31:31 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:31:31 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:31 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:31:31 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:31:31 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.365580 m/s - 0.368976 m/s)
17:31:31 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:31:31 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:31:31 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:31:31 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:31:31 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:31:31 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:31 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:31:31 INFO opendrift.models.basemodel:2032: 2025-01-13 02:00:00 - step 51 of 71 - 1000 active elements (0 deactivated)
17:31:31 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:31:31 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:31:31 DEBUG opendrift.models.basemodel:2051: 60.73271994991171 <- latitude -> 60.73749815055514
17:31:31 DEBUG opendrift.models.basemodel:2056: 4.593736803339148 <- longitude -> 4.603333647937261
17:31:31 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:31:31 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:31:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:31:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:31:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 02:00:00 (before)
2025-01-13 03:00:00 (after)
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:31:44 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:31:44 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 02:00:00)
17:31:44 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 02:00:00) in space (linearNDFast)
17:31:44 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:31:44 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:31:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40627386609707 and -65.39667702680653 degrees.
17:31:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40627386609707 and -65.39667702680653 degrees.
17:31:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:31:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:31:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:31:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:31:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:31:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:31:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:31:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:31:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:31:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.14941 (min) -0.139835 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.03762 (min) 1.05716 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.805039 (min) -0.799412 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.77013 (min) -2.55859 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.82 (min) 18.8687 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.87524e-05 (min) -2.81655e-05 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 236.906 (min) 250.091 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:31:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:31:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.901714, mean: 8.907675, max: 8.919294
17:31:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:31:44 DEBUG opendrift.models.physics_methods:1061: min: 16.254230, mean: 16.259671, max: 16.270273
17:31:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.254230, mean: 16.259671, max: 16.270273
17:31:44 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:31:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:44 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:31:44 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:31:44 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.380451 m/s - 0.380827 m/s)
17:31:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:31:44 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:31:44 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:31:44 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:31:44 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:31:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:44 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:31:44 INFO opendrift.models.basemodel:2032: 2025-01-13 03:00:00 - step 52 of 71 - 1000 active elements (0 deactivated)
17:31:44 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:31:44 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:31:44 DEBUG opendrift.models.basemodel:2051: 60.779003243015794 <- latitude -> 60.78329901955462
17:31:44 DEBUG opendrift.models.basemodel:2056: 4.580752601217427 <- longitude -> 4.590436698790125
17:31:44 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:31:44 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:31:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:31:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:31:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 03:00:00 (before)
2025-01-13 04:00:00 (after)
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:31:57 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:31:57 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x22x7) for time before (2025-01-13 03:00:00)
17:31:57 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 03:00:00) in space (linearNDFast)
17:31:57 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:31:57 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:31:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.41925806432593 and -65.40957395347968 degrees.
17:31:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.41925806432593 and -65.40957395347968 degrees.
17:31:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:31:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:31:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:31:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:31:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:31:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:31:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:31:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:31:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:31:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:31:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.131739 (min) -0.113563 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.06834 (min) 1.08924 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.836289 (min) -0.830223 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.35274 (min) -2.03291 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.1729 (min) 19.3923 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.00623e-05 (min) 1.0156e-05 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 223.178 (min) 237.097 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:31:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:31:57 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.179108, mean: 9.288103, max: 9.352777
17:31:57 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:31:57 DEBUG opendrift.models.physics_methods:1061: min: 16.505543, mean: 16.603226, max: 16.660954
17:31:57 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.505543, mean: 16.603226, max: 16.660954
17:31:57 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:31:57 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:57 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:31:57 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:31:57 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.386334 m/s - 0.389971 m/s)
17:31:57 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:31:57 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:31:57 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:31:57 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:31:57 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:31:57 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:31:57 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:31:57 INFO opendrift.models.basemodel:2032: 2025-01-13 04:00:00 - step 53 of 71 - 1000 active elements (0 deactivated)
17:31:57 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:31:57 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:31:57 DEBUG opendrift.models.basemodel:2051: 60.826583812854274 <- latitude -> 60.830345794781245
17:31:57 DEBUG opendrift.models.basemodel:2056: 4.569346470183393 <- longitude -> 4.5798108892139835
17:31:57 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:31:57 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:31:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:31:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:31:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:31:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:31:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 04:00:00 (before)
2025-01-13 05:00:00 (after)
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:32:15 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:32:15 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 04:00:00)
17:32:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 04:00:00) in space (linearNDFast)
17:32:15 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:32:15 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:32:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.43066417368505 and -65.42019975177351 degrees.
17:32:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.43066417368505 and -65.42019975177351 degrees.
17:32:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:32:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:32:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:32:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:32:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:32:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:32:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:32:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:32:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:32:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.16717 (min) -0.152046 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.02356 (min) 1.04048 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.743954 (min) -0.738293 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.158378 (min) -0.018767 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.5643 (min) 19.6341 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.71418e-05 (min) 3.75734e-05 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 202.348 (min) 218.425 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:32:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:32:15 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.416516, mean: 9.459757, max: 9.483252
17:32:15 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:32:15 DEBUG opendrift.models.physics_methods:1061: min: 16.717631, mean: 16.755966, max: 16.776764
17:32:15 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.717631, mean: 16.755966, max: 16.776764
17:32:15 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:32:15 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:15 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:32:15 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:32:15 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.391298 m/s - 0.392682 m/s)
17:32:15 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:32:15 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:32:15 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:32:15 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:32:15 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:32:15 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:15 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:32:15 INFO opendrift.models.basemodel:2032: 2025-01-13 05:00:00 - step 54 of 71 - 1000 active elements (0 deactivated)
17:32:15 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:32:15 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:32:15 DEBUG opendrift.models.basemodel:2051: 60.872836014712234 <- latitude -> 60.876101773797885
17:32:15 DEBUG opendrift.models.basemodel:2056: 4.55844013685757 <- longitude -> 4.569528944643601
17:32:15 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:32:15 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:32:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:32:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:32:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 05:00:00 (before)
2025-01-13 06:00:00 (after)
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:32:33 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:32:33 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 05:00:00)
17:32:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 05:00:00) in space (linearNDFast)
17:32:33 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:32:33 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:32:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44157048883676 and -65.43048168702565 degrees.
17:32:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44157048883676 and -65.43048168702565 degrees.
17:32:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:32:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:32:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:32:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:32:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:32:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:32:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:32:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:32:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:32:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.106719 (min) -0.0855766 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.915748 (min) 0.932907 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.552062 (min) -0.546914 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.680923 (min) 0.737103 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.2139 (min) 19.2285 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.63686e-05 (min) 6.66064e-05 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 194.084 (min) 201.776 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:32:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:32:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.093185, mean: 9.098926, max: 9.108802
17:32:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:32:33 DEBUG opendrift.models.physics_methods:1061: min: 16.428111, mean: 16.433294, max: 16.442212
17:32:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.428111, mean: 16.433294, max: 16.442212
17:32:33 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:32:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:33 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:32:33 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:32:33 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.384521 m/s - 0.384851 m/s)
17:32:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:32:33 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:32:33 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:32:33 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:32:33 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:32:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:33 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:32:33 INFO opendrift.models.basemodel:2032: 2025-01-13 06:00:00 - step 55 of 71 - 1000 active elements (0 deactivated)
17:32:33 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:32:33 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:32:33 DEBUG opendrift.models.basemodel:2051: 60.91539489197538 <- latitude -> 60.91811229625833
17:32:33 DEBUG opendrift.models.basemodel:2056: 4.552337219350506 <- longitude -> 4.5647631133847915
17:32:33 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:32:33 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:32:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:32:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:32:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 06:00:00 (before)
2025-01-13 07:00:00 (after)
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:32:53 WARNING opendrift.readers.basereader.variables:650: Invalid values (1.0245 to 1.2277) found for ocean_vertical_diffusivity, replacing with NaN
17:32:53 WARNING opendrift.readers.basereader.variables:653: (allowed range: [0, 1])
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:32:53 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:32:53 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 06:00:00)
17:32:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 06:00:00) in space (linearNDFast)
17:32:53 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:32:53 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:32:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44767339646955 and -65.43524750148678 degrees.
17:32:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44767339646955 and -65.43524750148678 degrees.
17:32:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:32:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:32:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:32:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:32:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:32:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:32:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:32:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:32:53 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:32:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:32:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.140778 (min) -0.113187 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.735877 (min) 0.744694 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.270951 (min) -0.26686 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.06296 (min) 1.09459 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.2349 (min) 19.2555 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.08716e-05 (min) 8.11544e-05 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 171.639 (min) 178.483 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:32:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:32:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.129863, mean: 9.138782, max: 9.150541
17:32:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:32:53 DEBUG opendrift.models.physics_methods:1061: min: 16.461208, mean: 16.469247, max: 16.479840
17:32:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.461208, mean: 16.469247, max: 16.479840
17:32:53 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:32:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:53 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:32:53 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:32:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.385296 m/s - 0.385732 m/s)
17:32:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:32:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:32:53 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:32:53 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:32:53 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:32:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:32:53 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:32:53 INFO opendrift.models.basemodel:2032: 2025-01-13 07:00:00 - step 56 of 71 - 1000 active elements (0 deactivated)
17:32:53 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:32:53 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:32:53 DEBUG opendrift.models.basemodel:2051: 60.951889029595804 <- latitude -> 60.95432864273909
17:32:53 DEBUG opendrift.models.basemodel:2056: 4.544442220739111 <- longitude -> 4.558671211720065
17:32:53 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:32:53 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:32:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:32:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:32:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:32:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:32:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 07:00:00 (before)
2025-01-13 08:00:00 (after)
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:33:06 WARNING opendrift.readers.basereader.variables:650: Invalid values (1.0071 to 1.2427) found for ocean_vertical_diffusivity, replacing with NaN
17:33:06 WARNING opendrift.readers.basereader.variables:653: (allowed range: [0, 1])
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:33:06 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:33:06 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 07:00:00)
17:33:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 07:00:00) in space (linearNDFast)
17:33:06 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:33:06 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:33:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45556837986891 and -65.44133939881016 degrees.
17:33:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45556837986891 and -65.44133939881016 degrees.
17:33:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:33:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:33:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:33:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:33:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:33:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:33:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:33:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:33:06 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:33:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:33:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.107084 (min) -0.0786616 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.65561 (min) 0.689893 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0497727 (min) 0.0543797 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.32789 (min) 1.35807 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.9419 (min) 19.0254 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.90708e-05 (min) 8.96678e-05 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 136.768 (min) 147.865 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:33:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:33:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.871545, mean: 8.896519, max: 8.947733
17:33:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:33:06 DEBUG opendrift.models.physics_methods:1061: min: 16.226662, mean: 16.249480, max: 16.296191
17:33:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.226662, mean: 16.249480, max: 16.296191
17:33:06 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:33:06 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:06 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:33:06 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:33:06 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.379806 m/s - 0.381434 m/s)
17:33:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:33:06 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:33:06 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:33:06 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:33:06 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:33:06 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:06 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:33:06 INFO opendrift.models.basemodel:2032: 2025-01-13 08:00:00 - step 57 of 71 - 1000 active elements (0 deactivated)
17:33:06 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:33:06 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:33:06 DEBUG opendrift.models.basemodel:2051: 60.98538003804933 <- latitude -> 60.98885699944495
17:33:06 DEBUG opendrift.models.basemodel:2056: 4.539154732157406 <- longitude -> 4.555208128702169
17:33:06 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:33:06 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:33:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:33:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:33:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:33:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 08:00:00 (before)
2025-01-13 09:00:00 (after)
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:33:18 WARNING opendrift.readers.basereader.variables:650: Invalid values (1.0035 to 1.2534) found for ocean_vertical_diffusivity, replacing with NaN
17:33:18 WARNING opendrift.readers.basereader.variables:653: (allowed range: [0, 1])
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:33:18 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:33:18 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 08:00:00)
17:33:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 08:00:00) in space (linearNDFast)
17:33:18 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:33:18 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:33:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46085585349245 and -65.44480245801333 degrees.
17:33:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46085585349245 and -65.44480245801333 degrees.
17:33:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:33:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:33:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:33:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:33:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:33:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:33:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:33:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:33:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:33:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:33:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.053847 (min) -0.0469289 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.655499 (min) 0.671449 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.325603 (min) 0.329573 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.21399 (min) 1.23219 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.4909 (min) 18.5869 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.00884e-05 (min) 6.06871e-05 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 82.0022 (min) 92.583 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:33:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:33:18 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.448426, mean: 8.475944, max: 8.534888
17:33:18 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:33:18 DEBUG opendrift.models.physics_methods:1061: min: 15.834979, mean: 15.860737, max: 15.915802
17:33:18 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.834979, mean: 15.860737, max: 15.915802
17:33:18 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:33:18 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:18 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:33:18 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:33:18 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.370638 m/s - 0.372530 m/s)
17:33:18 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:33:18 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:33:18 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:33:18 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:33:18 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:33:18 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:18 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:33:18 INFO opendrift.models.basemodel:2032: 2025-01-13 09:00:00 - step 58 of 71 - 1000 active elements (0 deactivated)
17:33:18 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:33:18 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:33:18 DEBUG opendrift.models.basemodel:2051: 61.018580291409535 <- latitude -> 61.02249771627346
17:33:18 DEBUG opendrift.models.basemodel:2056: 4.537672135626022 <- longitude -> 4.553316118932647
17:33:18 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:33:18 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:33:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:33:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:33:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 1000 elements
17:33:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 09:00:00 (before)
2025-01-13 10:00:00 (after)
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:33:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:33:31 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:33:31 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 09:00:00)
17:33:31 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 09:00:00) in space (linearNDFast)
17:33:31 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:33:31 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:33:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46233844295647 and -65.44669446166746 degrees.
17:33:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46233844295647 and -65.44669446166746 degrees.
17:33:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:33:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:33:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:33:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:33:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:33:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:33:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:33:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:33:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:33:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:33:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:33:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0983017 (min) 0.118032 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.612657 (min) 0.625859 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.471167 (min) 0.473588 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.835904 (min) 0.922526 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.2532 (min) 18.3052 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.46012e-05 (min) 2.58839e-05 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0248925 (min) 0.0255589 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 49.6354 (min) 58.245 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 1 (max)
17:33:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:33:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.216937, mean: 8.230499, max: 8.260162
17:33:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:33:33 DEBUG opendrift.models.physics_methods:1061: min: 15.616530, mean: 15.629410, max: 15.657552
17:33:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.616530, mean: 15.629410, max: 15.657552
17:33:33 DEBUG opendrift.models.basemodel:659: 5 elements hit land, moving them to the coastline.
17:33:33 DEBUG opendrift.models.basemodel:1679: Added status stranded
17:33:33 DEBUG opendrift.models.basemodel:1690: 5 elements scheduled for deactivation (stranded)
17:33:33 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:33:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:34 DEBUG opendrift.models.basemodel:1710: Removed 5 elements.
17:33:34 DEBUG opendrift.models.basemodel:1713: Removed 5 values from environment.
17:33:34 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:33:34 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:33:34 DEBUG opendrift.models.basemodel:1722: Removed 5 values from environment_profiles.
17:33:34 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:33:34 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.365525 m/s - 0.366357 m/s)
17:33:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:33:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:33:34 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:33:34 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:33:34 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:33:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:34 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:33:34 INFO opendrift.models.basemodel:2032: 2025-01-13 10:00:00 - step 59 of 71 - 995 active elements (5 deactivated)
17:33:34 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:33:34 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:33:34 DEBUG opendrift.models.basemodel:2051: 61.05079298224237 <- latitude -> 61.05409060163903
17:33:34 DEBUG opendrift.models.basemodel:2056: 4.546353991269659 <- longitude -> 4.5603398843709
17:33:34 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:33:34 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:33:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:33:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:33:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:33:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:33:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 10:00:00 (before)
2025-01-13 11:00:00 (after)
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:33:46 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:33:46 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 10:00:00)
17:33:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 10:00:00) in space (linearNDFast)
17:33:46 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:33:46 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:33:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45365658778225 and -65.43967069069781 degrees.
17:33:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45365658778225 and -65.43967069069781 degrees.
17:33:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:33:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:33:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:33:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:33:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:33:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:33:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:33:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:33:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:33:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:33:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:33:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0752263 (min) 0.215476 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.34559 (min) 0.412746 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.429169 (min) 0.431357 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.33089 (min) 1.46276 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.5447 (min) 17.5871 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.085e-05 (min) -3.99261e-05 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 72.1476 (min) 75.7989 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:33:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:33:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.623437, mean: 7.628584, max: 7.652464
17:33:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:33:46 DEBUG opendrift.models.physics_methods:1061: min: 15.041979, mean: 15.047056, max: 15.070588
17:33:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.041979, mean: 15.047056, max: 15.070588
17:33:46 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:33:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:46 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:33:46 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:33:46 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.352077 m/s - 0.352747 m/s)
17:33:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:33:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:33:46 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:33:46 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:33:46 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:33:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:33:46 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:33:46 INFO opendrift.models.basemodel:2032: 2025-01-13 11:00:00 - step 60 of 71 - 995 active elements (5 deactivated)
17:33:46 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:33:46 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:33:46 DEBUG opendrift.models.basemodel:2051: 61.07549069042528 <- latitude -> 61.07660249787362
17:33:46 DEBUG opendrift.models.basemodel:2056: 4.553574967894127 <- longitude -> 4.576304525945804
17:33:46 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:33:46 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:33:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:33:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:33:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:33:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:33:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 11:00:00 (before)
2025-01-13 12:00:00 (after)
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:34:00 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:34:00 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 11:00:00)
17:34:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 11:00:00) in space (linearNDFast)
17:34:00 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:34:00 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:34:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4464355924388 and -65.42370603295416 degrees.
17:34:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4464355924388 and -65.42370603295416 degrees.
17:34:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:34:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:34:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:34:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:34:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:34:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:34:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:34:00 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:34:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:34:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.104923 (min) 0.04208 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.218205 (min) 0.381126 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.209107 (min) 0.213023 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.73375 (min) 1.80823 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.6159 (min) 17.7495 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.49863e-05 (min) -8.46565e-05 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 124.926 (min) 136.249 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:34:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:34:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.714276, mean: 7.764357, max: 7.824037
17:34:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:34:00 DEBUG opendrift.models.physics_methods:1061: min: 15.131331, mean: 15.180345, max: 15.238599
17:34:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.131331, mean: 15.180345, max: 15.238599
17:34:00 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:34:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:00 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:34:00 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:34:00 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.354169 m/s - 0.356679 m/s)
17:34:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:34:00 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:34:00 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:34:00 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:34:00 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:34:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:00 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:34:00 INFO opendrift.models.basemodel:2032: 2025-01-13 12:00:00 - step 61 of 71 - 995 active elements (5 deactivated)
17:34:00 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:34:00 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:34:00 DEBUG opendrift.models.basemodel:2051: 61.094008767235024 <- latitude -> 61.10029786874647
17:34:00 DEBUG opendrift.models.basemodel:2056: 4.558721608327842 <- longitude -> 4.571619323419489
17:34:00 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:34:00 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:34:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:34:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:34:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 12:00:00 (before)
2025-01-13 13:00:00 (after)
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:34:12 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:34:12 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 12:00:00)
17:34:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 12:00:00) in space (linearNDFast)
17:34:12 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:34:12 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:34:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44128894711767 and -65.42839123238758 degrees.
17:34:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44128894711767 and -65.42839123238758 degrees.
17:34:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:34:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:34:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:34:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:34:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:34:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:34:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:34:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:34:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:34:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.204801 (min) 0.29567 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.135794 (min) 0.316379 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.122359 (min) -0.119693 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.75638 (min) 4.83749 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 14.9343 (min) 14.982 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.61159e-05 (min) -9.57015e-05 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 149.228 (min) 150.179 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:34:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:34:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.061986, mean: 6.070524, max: 6.078305
17:34:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:34:12 DEBUG opendrift.models.physics_methods:1061: min: 13.413344, mean: 13.422785, max: 13.431386
17:34:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.413344, mean: 13.422785, max: 13.431386
17:34:12 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:34:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:12 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:34:12 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:34:12 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.313957 m/s - 0.314379 m/s)
17:34:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:34:12 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:34:12 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:34:12 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:34:12 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:34:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:12 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:34:12 INFO opendrift.models.basemodel:2032: 2025-01-13 13:00:00 - step 62 of 71 - 995 active elements (5 deactivated)
17:34:12 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:34:12 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:34:12 DEBUG opendrift.models.basemodel:2051: 61.11364055777683 <- latitude -> 61.11438018090328
17:34:12 DEBUG opendrift.models.basemodel:2056: 4.578927820469323 <- longitude -> 4.593554267868056
17:34:12 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:34:12 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:34:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:34:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:34:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 13:00:00 (before)
2025-01-13 14:00:00 (after)
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:34:25 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:34:25 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 13:00:00)
17:34:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 13:00:00) in space (linearNDFast)
17:34:25 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:34:25 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:34:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42108273495978 and -65.40645626868675 degrees.
17:34:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42108273495978 and -65.40645626868675 degrees.
17:34:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:34:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:34:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:34:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:34:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:34:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:34:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:34:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:34:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:34:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.118059 (min) 0.153643 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.196611 (min) 0.319815 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.461518 (min) -0.458729 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.64482 (min) 4.90533 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 13.5779 (min) 13.6543 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.71299e-05 (min) -8.69454e-05 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 140.058 (min) 146.769 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:34:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:34:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 5.116197, mean: 5.121492, max: 5.128545
17:34:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:34:25 DEBUG opendrift.models.physics_methods:1061: min: 12.322624, mean: 12.328998, max: 12.337486
17:34:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 12.322624, mean: 12.328998, max: 12.337486
17:34:25 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:34:25 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:25 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:34:25 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:34:25 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.288427 m/s - 0.288775 m/s)
17:34:25 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:34:25 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:34:25 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:34:25 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:34:25 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:34:25 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:25 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:34:25 INFO opendrift.models.basemodel:2032: 2025-01-13 14:00:00 - step 63 of 71 - 995 active elements (5 deactivated)
17:34:25 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:34:25 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:34:25 DEBUG opendrift.models.basemodel:2051: 61.129027978144805 <- latitude -> 61.133494405005976
17:34:25 DEBUG opendrift.models.basemodel:2056: 4.5948593474010195 <- longitude -> 4.60776051202794
17:34:25 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:34:25 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:34:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:34:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:34:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 14:00:00 (before)
2025-01-13 15:00:00 (after)
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:34:39 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:34:39 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 14:00:00)
17:34:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 14:00:00) in space (linearNDFast)
17:34:39 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:34:39 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:34:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40515119595682 and -65.39225003860156 degrees.
17:34:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40515119595682 and -65.39225003860156 degrees.
17:34:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:34:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:34:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:34:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:34:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:34:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:34:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:34:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:34:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:34:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0808814 (min) 0.128526 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.297175 (min) 0.313455 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.714277 (min) -0.711477 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.07432 (min) 5.29554 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 12.1545 (min) 12.2506 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.49653e-05 (min) -5.48913e-05 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 138.01 (min) 145.848 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:34:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:34:39 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.324073, mean: 4.324843, max: 4.325512
17:34:39 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:34:39 DEBUG opendrift.models.physics_methods:1061: min: 11.328596, mean: 11.329605, max: 11.330480
17:34:39 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.328596, mean: 11.329605, max: 11.330480
17:34:39 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:34:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:39 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:34:39 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:34:39 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.265161 m/s - 0.265205 m/s)
17:34:39 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:34:39 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:34:39 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:34:39 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:34:39 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:34:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:39 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:34:39 INFO opendrift.models.basemodel:2032: 2025-01-13 15:00:00 - step 64 of 71 - 995 active elements (5 deactivated)
17:34:39 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:34:39 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:34:39 DEBUG opendrift.models.basemodel:2051: 61.14685858984823 <- latitude -> 61.15100956962716
17:34:39 DEBUG opendrift.models.basemodel:2056: 4.610514988163163 <- longitude -> 4.619958860451343
17:34:39 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:34:39 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:34:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:34:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:34:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 15:00:00 (before)
2025-01-13 16:00:00 (after)
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:34:54 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:34:54 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 15:00:00)
17:34:54 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 15:00:00) in space (linearNDFast)
17:34:54 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:34:54 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:34:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38949554319242 and -65.38005166990533 degrees.
17:34:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38949554319242 and -65.38005166990533 degrees.
17:34:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:34:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:34:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:34:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:34:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:34:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:34:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:34:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:34:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:34:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:34:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.105286 (min) 0.123069 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.229568 (min) 0.253406 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.844076 (min) -0.841915 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.01343 (min) 5.1299 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1744 (min) 11.2275 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.86117e-05 (min) -1.83844e-05 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 147.94 (min) 155.442 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:34:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:34:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.717685, mean: 3.719486, max: 3.720359
17:34:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:34:54 DEBUG opendrift.models.physics_methods:1061: min: 10.504271, mean: 10.506814, max: 10.508046
17:34:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.504271, mean: 10.506814, max: 10.508046
17:34:54 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:34:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:54 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:34:54 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:34:54 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.245866 m/s - 0.245955 m/s)
17:34:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:34:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:34:54 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:34:54 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:34:54 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:34:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:34:54 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:34:54 INFO opendrift.models.basemodel:2032: 2025-01-13 16:00:00 - step 65 of 71 - 995 active elements (5 deactivated)
17:34:54 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:34:54 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:34:54 DEBUG opendrift.models.basemodel:2051: 61.16221698110228 <- latitude -> 61.165732734867035
17:34:54 DEBUG opendrift.models.basemodel:2056: 4.625513190148678 <- longitude -> 4.633745539730111
17:34:54 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:34:54 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:34:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:34:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:34:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:34:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:34:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 16:00:00 (before)
2025-01-13 17:00:00 (after)
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:35:06 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:35:06 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 16:00:00)
17:35:06 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 16:00:00) in space (linearNDFast)
17:35:06 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:35:06 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:35:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37449734454444 and -65.36626498444872 degrees.
17:35:06 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37449734454444 and -65.36626498444872 degrees.
17:35:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:35:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:35:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:35:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:35:06 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:35:06 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:35:06 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:06 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:35:06 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:35:06 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:35:06 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0712329 (min) 0.0906685 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.218823 (min) 0.229953 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.83223 (min) -0.830189 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.66275 (min) 5.747 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1687 (min) 11.1852 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.53316e-05 (min) 2.56699e-05 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 151.181 (min) 159.767 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:35:06 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:35:06 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.866520, mean: 3.871316, max: 3.881100
17:35:06 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:35:06 DEBUG opendrift.models.physics_methods:1061: min: 10.712471, mean: 10.719114, max: 10.732651
17:35:06 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.712471, mean: 10.719114, max: 10.732651
17:35:06 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:35:06 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:06 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:35:06 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:35:06 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.250739 m/s - 0.251212 m/s)
17:35:06 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:35:06 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:35:06 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:35:06 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:35:06 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:35:06 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:06 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:35:06 INFO opendrift.models.basemodel:2032: 2025-01-13 17:00:00 - step 66 of 71 - 995 active elements (5 deactivated)
17:35:06 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:35:06 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:35:06 DEBUG opendrift.models.basemodel:2051: 61.1766915681053 <- latitude -> 61.18029484660008
17:35:06 DEBUG opendrift.models.basemodel:2056: 4.639215866920429 <- longitude -> 4.64610150885358
17:35:06 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:35:06 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:35:06 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:35:06 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:06 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:06 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:35:06 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 17:00:00 (before)
2025-01-13 18:00:00 (after)
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:35:19 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:35:19 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x7) for time before (2025-01-13 17:00:00)
17:35:19 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 17:00:00) in space (linearNDFast)
17:35:19 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:35:19 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:35:19 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36079464926206 and -65.35390901331881 degrees.
17:35:19 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36079464926206 and -65.35390901331881 degrees.
17:35:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:35:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:35:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:35:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:35:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:35:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:35:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:19 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:35:19 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:35:19 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:35:19 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -5.65159e-06 (min) 0.0775311 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.258764 (min) 0.384047 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.684984 (min) -0.682977 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.13602 (min) 7.1979 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.7673 (min) 10.7688 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.47726e-05 (min) 5.54861e-05 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 165.022 (min) 168.884 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:35:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:35:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.105314, mean: 4.112370, max: 4.126541
17:35:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:35:19 DEBUG opendrift.models.physics_methods:1061: min: 11.038314, mean: 11.047793, max: 11.066815
17:35:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.038314, mean: 11.047793, max: 11.066815
17:35:19 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:35:19 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:19 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:35:19 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:35:19 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.258366 m/s - 0.259033 m/s)
17:35:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:35:19 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:35:19 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:35:19 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:35:19 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:35:19 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:19 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:35:19 INFO opendrift.models.basemodel:2032: 2025-01-13 18:00:00 - step 67 of 71 - 995 active elements (5 deactivated)
17:35:19 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:35:19 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:35:19 DEBUG opendrift.models.basemodel:2051: 61.192008701888184 <- latitude -> 61.199659527382124
17:35:19 DEBUG opendrift.models.basemodel:2056: 4.653790224734166 <- longitude -> 4.656089059363346
17:35:19 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:35:19 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:35:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:35:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:35:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 18:00:00 (before)
2025-01-13 19:00:00 (after)
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:35:32 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:35:32 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x7) for time before (2025-01-13 18:00:00)
17:35:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 18:00:00) in space (linearNDFast)
17:35:32 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:35:32 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:35:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34622028664398 and -65.34392145482107 degrees.
17:35:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34622028664398 and -65.34392145482107 degrees.
17:35:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:35:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:35:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:35:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:35:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:35:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:35:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:35:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:35:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:35:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.101424 (min) -0.0818482 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.545717 (min) 0.558793 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.409503 (min) -0.40726 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.53951 (min) 7.55674 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.1274 (min) 10.1419 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.63702e-05 (min) 8.66948e-05 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 191.752 (min) 219.573 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:35:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:35:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.923539, mean: 3.930242, max: 3.934696
17:35:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:35:32 DEBUG opendrift.models.physics_methods:1061: min: 10.791171, mean: 10.800384, max: 10.806503
17:35:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.791171, mean: 10.800384, max: 10.806503
17:35:32 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:35:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:32 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:35:32 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:35:32 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.252581 m/s - 0.252940 m/s)
17:35:32 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:35:32 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:35:32 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:35:32 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:35:32 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:35:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:32 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:35:32 INFO opendrift.models.basemodel:2032: 2025-01-13 19:00:00 - step 68 of 71 - 995 active elements (5 deactivated)
17:35:32 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:35:32 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:35:32 DEBUG opendrift.models.basemodel:2051: 61.21643325784643 <- latitude -> 61.22385227098118
17:35:32 DEBUG opendrift.models.basemodel:2056: 4.658427108866105 <- longitude -> 4.65977422922173
17:35:32 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:35:32 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:35:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:35:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:35:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 19:00:00 (before)
2025-01-13 20:00:00 (after)
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:35:43 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:35:43 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-13 19:00:00)
17:35:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 19:00:00) in space (linearNDFast)
17:35:43 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:35:43 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:35:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34158340277892 and -65.3402362706677 degrees.
17:35:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34158340277892 and -65.3402362706677 degrees.
17:35:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:35:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:35:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:35:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:35:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:35:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:35:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:35:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:35:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:35:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0562139 (min) -0.0298352 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.531358 (min) 0.568422 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0690207 (min) -0.067707 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.68632 (min) 6.71038 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.15822 (min) 9.17242 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.54842e-05 (min) 9.59871e-05 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 263.823 (min) 273.186 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:35:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:35:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.163696, mean: 3.172084, max: 3.177386
17:35:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:35:43 DEBUG opendrift.models.physics_methods:1061: min: 9.690070, mean: 9.702906, max: 9.711013
17:35:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.690070, mean: 9.702906, max: 9.711013
17:35:43 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:35:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:43 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:35:43 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:35:43 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.226809 m/s - 0.227299 m/s)
17:35:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:35:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:35:43 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:35:43 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:35:43 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:35:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:43 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:35:43 INFO opendrift.models.basemodel:2032: 2025-01-13 20:00:00 - step 69 of 71 - 995 active elements (5 deactivated)
17:35:43 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:35:43 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:35:43 DEBUG opendrift.models.basemodel:2051: 61.23951828052264 <- latitude -> 61.24814217727128
17:35:43 DEBUG opendrift.models.basemodel:2056: 4.663744082206798 <- longitude -> 4.666483356441154
17:35:43 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:35:43 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:35:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:35:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:35:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 20:00:00 (before)
2025-01-13 21:00:00 (after)
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:35:56 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:35:56 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-13 20:00:00)
17:35:56 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 20:00:00) in space (linearNDFast)
17:35:56 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:35:56 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:35:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3362664154098 and -65.33352714289013 degrees.
17:35:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3362664154098 and -65.33352714289013 degrees.
17:35:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:35:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:35:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:35:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:35:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:35:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:35:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:35:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:35:56 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:35:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:35:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0465059 (min) 0.131652 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.587119 (min) 0.618893 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.25832 (min) 0.259568 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.26158 (min) 7.28146 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.53585 (min) 9.55067 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.09529e-05 (min) 8.12121e-05 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 231.937 (min) 258.478 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:35:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:35:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.534735, mean: 3.543152, max: 3.547611
17:35:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:35:56 DEBUG opendrift.models.physics_methods:1061: min: 10.242549, mean: 10.254734, max: 10.261185
17:35:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.242549, mean: 10.254734, max: 10.261185
17:35:56 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:35:56 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:56 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:35:56 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:35:56 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.239740 m/s - 0.240176 m/s)
17:35:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:35:56 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:35:56 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:35:56 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:35:56 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:35:56 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:35:56 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:35:56 INFO opendrift.models.basemodel:2032: 2025-01-13 21:00:00 - step 70 of 71 - 995 active elements (5 deactivated)
17:35:56 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:35:56 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:35:56 DEBUG opendrift.models.basemodel:2051: 61.26464766312757 <- latitude -> 61.27430660074056
17:35:56 DEBUG opendrift.models.basemodel:2056: 4.677042178899647 <- longitude -> 4.685088864852748
17:35:56 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:35:56 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:35:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:35:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:35:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:35:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:35:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 21:00:00 (before)
2025-01-13 22:00:00 (after)
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:09 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:36:09 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x7) for time before (2025-01-13 21:00:00)
17:36:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 21:00:00) in space (linearNDFast)
17:36:09 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:09 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32296830878197 and -65.31492161067312 degrees.
17:36:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32296830878197 and -65.31492161067312 degrees.
17:36:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:36:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:36:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:36:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.278389 (min) 0.337377 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.448456 (min) 0.536179 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.456594 (min) 0.457127 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: 9.2821 (min) 9.50116 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.10679 (min) 8.34221 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.30363e-05 (min) 3.38542e-05 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 113.819 (min) 156.299 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.736187, mean: 3.810842, max: 3.932667
17:36:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:09 DEBUG opendrift.models.physics_methods:1061: min: 10.530377, mean: 10.634678, max: 10.803718
17:36:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.530377, mean: 10.634678, max: 10.803718
17:36:09 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:09 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:09 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:09 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:09 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.246477 m/s - 0.252875 m/s)
17:36:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:09 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:09 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:36:09 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:09 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:09 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:09 INFO opendrift.models.basemodel:2032: 2025-01-13 22:00:00 - step 71 of 71 - 995 active elements (5 deactivated)
17:36:09 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:09 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:09 DEBUG opendrift.models.basemodel:2051: 61.287357787351915 <- latitude -> 61.29403001734691
17:36:09 DEBUG opendrift.models.basemodel:2056: 4.708705954519134 <- longitude -> 4.720208914566266
17:36:09 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:09 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_vertical_diffusivity', 'x_wind', 'y_wind', 'sea_floor_depth_below_sea_level', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader NorKyst manual aggregate
17:36:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:36:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from NorKyst manual aggregate covering 995 elements
17:36:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 22:00:00 (before)
2025-01-13 23:00:00 (after)
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking ocean_vertical_diffusivity for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:22 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity', 'ocean_vertical_diffusivity']
17:36:22 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x23x7) for time before (2025-01-13 22:00:00)
17:36:22 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 22:00:00) in space (linearNDFast)
17:36:22 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:36:22 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:36:22 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29130451845404 and -65.27980155655742 degrees.
17:36:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29130451845404 and -65.27980155655742 degrees.
17:36:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:36:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:36:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 0 for ocean_vertical_diffusivity for 0 profiles
17:36:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.171202 (min) 0.329056 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.36504 (min) 0.4947 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.472662 (min) 0.475 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.86679 (min) 7.94436 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: 7.80532 (min) 8.0425 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.62901e-05 (min) -2.09798e-05 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 3.39161e-06 (min) 0.00869782 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 5 (min) 11.8083 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 1 (max)
17:36:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:22 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.021112, mean: 3.080790, max: 3.143526
17:36:22 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:22 DEBUG opendrift.models.physics_methods:1061: min: 9.469193, mean: 9.562074, max: 9.659133
17:36:22 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.469193, mean: 9.562074, max: 9.659133
17:36:22 DEBUG opendrift.models.basemodel:659: 958 elements hit land, moving them to the coastline.
17:36:22 DEBUG opendrift.models.basemodel:1690: 958 elements scheduled for deactivation (stranded)
17:36:22 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:36:23 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:23 DEBUG opendrift.models.basemodel:1710: Removed 958 elements.
17:36:23 DEBUG opendrift.models.basemodel:1713: Removed 958 values from environment.
17:36:23 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:36:23 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:36:23 DEBUG opendrift.models.basemodel:1722: Removed 958 values from environment_profiles.
17:36:23 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:23 DEBUG opendrift.models.physics_methods:915: Advecting 37 of 37 elements above 0.100m with wind-sheared ocean current (0.225990 m/s - 0.226085 m/s)
17:36:23 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:23 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:23 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:23 DEBUG opendrift.models.basemodel:2120: 37 active elements (963 deactivated)
17:36:23 DEBUG opendrift.models.basemodel:2149: Cleaning up
17:36:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 37 elements
17:36:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 37 elements
17:36:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 1 (min) 1 (max)
17:36:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:23 DEBUG opendrift.models.basemodel:659: 37 elements hit land, moving them to the coastline.
17:36:23 DEBUG opendrift.models.basemodel:1690: 37 elements scheduled for deactivation (stranded)
17:36:23 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:36:23 DEBUG opendrift.models.basemodel:1710: Removed 37 elements.
17:36:23 DEBUG opendrift.models.basemodel:1713: Removed 37 values from environment.
17:36:23 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:36:23 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:36:23 DEBUG opendrift.models.basemodel:1722: Removed 37 values from environment_profiles.
17:36:23 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Run to Mode.Result
17:36:23 DEBUG opendrift.models.oceandrift:115: No machine learning correction available.
17:36:23 DEBUG opendrift.config:168: Adding 50 config items from environment
17:36:23 DEBUG opendrift.config:168: Adding 5 config items from environment
17:36:23 DEBUG opendrift.config:168: Adding 18 config items from __init__
17:36:23 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
17:36:23 DEBUG opendrift.config:168: Adding 5 config items from __init__
17:36:23 INFO opendrift.models.basemodel:512: OpenDriftSimulation initialised (version 1.12.0 / v1.12.0-55-g4faa301)
17:36:23 DEBUG opendrift.config:168: Adding 15 config items from oceandrift
17:36:23 DEBUG opendrift.config:178: Overwriting config item seed:z
17:36:23 DEBUG opendrift.readers.reader_lazy:38: Delaying initialisation of LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:23 DEBUG opendrift.models.basemodel.environment:328: Added reader LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:23 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
17:36:23 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
17:36:23 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
17:36:23 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
17:36:23 INFO opendrift.models.basemodel.environment:248: x_sea_water_velocity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: y_sea_water_velocity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_height: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: x_wind: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: y_wind: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: upward_sea_water_velocity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_significant_height: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_x_velocity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_stokes_drift_y_velocity: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_to_direction: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_swell_wave_significant_height: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_to_direction: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_mean_period: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_surface_wind_wave_significant_height: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: surface_downward_x_stress: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: surface_downward_y_stress: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: turbulent_kinetic_energy: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: turbulent_generic_length_scale: 0.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: ocean_mixed_layer_thickness: 50.000000
17:36:23 INFO opendrift.models.basemodel.environment:248: sea_floor_depth_below_sea_level: 10000.000000
17:36:23 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Config to Mode.Ready
17:36:23 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Ready to Mode.Run
17:36:23 DEBUG opendrift.models.basemodel:1774:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.12.0
Platform: Linux, 5.15.0-1057-aws
68.56774139404297 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.14.1
Matplotlib version 3.9.1
NetCDF4 version 1.6.1
Xarray version 2024.11.0
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 1.3.5
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
17:36:23 DEBUG opendrift.models.basemodel:1788: No output file is specified, neglecting export_buffer_length
17:36:23 DEBUG opendrift.models.basemodel:1906: Finalizing environment and preparing readers for simulation coverage ([-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]) and time (2025-01-11 00:00:00 to 2025-01-13 23:00:00)
17:36:23 DEBUG opendrift.models.basemodel.environment:180: Preparing LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
17:36:23 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [-4.71627286447143, 55.39190523302233, 13.715758357177728, 64.60825116956556]
17:36:23 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
17:36:23 INFO opendrift.models.basemodel:932: Using existing reader for land_binary_mask
17:36:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:23 INFO opendrift.models.basemodel:943: All points are in ocean
17:36:23 DEBUG opendrift.models.basemodel:887: to be seeded: 1000, already seeded 0
17:36:23 DEBUG opendrift.models.basemodel:905: Released 1000 new elements.
17:36:23 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:23 INFO opendrift.models.basemodel:2032: 2025-01-11 00:00:00 - step 1 of 71 - 1000 active elements (0 deactivated)
17:36:23 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:23 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:23 DEBUG opendrift.models.basemodel:2051: 59.99731 <- latitude -> 60.002846
17:36:23 DEBUG opendrift.models.basemodel:2056: 4.4945407 <- longitude -> 4.504945
17:36:23 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:23 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:23 DEBUG opendrift.models.basemodel.environment:581: Variables not covered by any reader: ['sea_surface_wave_stokes_drift_x_velocity', 'turbulent_generic_length_scale', 'x_sea_water_velocity', 'surface_downward_x_stress', 'ocean_mixed_layer_thickness', 'sea_surface_wave_stokes_drift_y_velocity', 'sea_surface_wave_significant_height', 'sea_surface_height', 'y_sea_water_velocity', 'sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment', 'sea_surface_wave_period_at_variance_spectral_density_maximum', 'upward_sea_water_velocity', 'x_wind', 'surface_downward_y_stress', 'turbulent_kinetic_energy', 'sea_floor_depth_below_sea_level', 'ocean_vertical_diffusivity', 'y_wind']
17:36:23 DEBUG opendrift.readers.reader_lazy:57: Initialising: LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:23 DEBUG opendrift.readers:136: Testing reader <module 'opendrift.readers.reader_netCDF_CF_generic' from '/root/project/opendrift/readers/reader_netCDF_CF_generic.py'>
17:36:23 INFO opendrift.readers:58: Opening file with xr.open_dataset
17:36:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:128: Finding coordinate variables.
17:36:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:143: Parsing CF grid mapping dictionary: {'grid_mapping_name': 'polar_stereographic', 'straight_vertical_longitude_from_pole': 70.0, 'latitude_of_projection_origin': 90.0, 'standard_parallel': 60.0, 'false_easting': 3192800.0, 'false_northing': 1784000.0, 'semi_major_axis': 6378137.0, 'semi_minor_axis': 6356752.3142, 'proj4': '+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs'}
17:36:28 INFO opendrift.readers.reader_netCDF_CF_generic:338: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
17:36:28 DEBUG opendrift.readers.reader_netCDF_CF_generic:374: Skipped variables without standard_name: ['angle', 'tke', 'ubar', 'vbar']
17:36:28 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 25 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 5 m/s and time span of 1:00:00
17:36:28 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
17:36:28 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
17:36:28 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
17:36:28 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be before starting new simulation
17:36:28 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
17:36:28 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:28 DEBUG opendrift.readers.reader_lazy:72: Reader initialised: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:28 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
17:36:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 00:00:00 (before)
2025-01-11 01:00:00 (after)
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:29 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-11 00:00:00)
17:36:29 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 00:00:00) in space (linearNDFast)
17:36:29 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:29 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
17:36:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50547356643384 and -65.49506707409589 degrees.
17:36:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:29 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0299121 (min) 0.0385718 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.123546 (min) -0.101854 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.721338 (min) -0.71924 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.37718 (min) -1.35714 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.06815 (min) -7.94024 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.73794e-07 (min) 3.18015e-07 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.369 (min) 298.632 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:29 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.597613, mean: 1.622316, max: 1.646645
17:36:29 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:29 DEBUG opendrift.models.physics_methods:1061: min: 6.885974, mean: 6.938986, max: 6.990844
17:36:29 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 6.885974, mean: 6.938986, max: 6.990844
17:36:29 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:29 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:29 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:29 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.161175 m/s - 0.163630 m/s)
17:36:29 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:29 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:29 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:29 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:29 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:29 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:29 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:29 INFO opendrift.models.basemodel:2032: 2025-01-11 01:00:00 - step 2 of 71 - 1000 active elements (0 deactivated)
17:36:29 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:29 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:29 DEBUG opendrift.models.basemodel:2051: 59.988504092561726 <- latitude -> 59.99409196331214
17:36:29 DEBUG opendrift.models.basemodel:2056: 4.495099233223983 <- longitude -> 4.5054428283887855
17:36:29 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:29 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 01:00:00 (before)
2025-01-11 02:00:00 (after)
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:30 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 01:00:00)
17:36:30 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 01:00:00) in space (linearNDFast)
17:36:30 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:30 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50491168847081 and -65.49456810576453 degrees.
17:36:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50491168847081 and -65.49456810576453 degrees.
17:36:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:30 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0302097 (min) 0.0410515 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0639426 (min) -0.04447 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.752453 (min) -0.750927 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.41837 (min) -1.39774 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.54686 (min) -8.48849 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.2857e-06 (min) 6.45347e-06 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.962 (min) 298.291 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:30 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.820749, mean: 1.834391, max: 1.846378
17:36:30 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:30 DEBUG opendrift.models.physics_methods:1061: min: 7.351138, mean: 7.378622, max: 7.402696
17:36:30 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.351138, mean: 7.378622, max: 7.402696
17:36:30 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:30 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:30 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:30 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.172063 m/s - 0.173270 m/s)
17:36:30 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:30 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:30 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:30 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:30 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:30 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:30 INFO opendrift.models.basemodel:2032: 2025-01-11 02:00:00 - step 3 of 71 - 1000 active elements (0 deactivated)
17:36:30 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:30 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:30 DEBUG opendrift.models.basemodel:2051: 59.981084562800206 <- latitude -> 59.98691162512811
17:36:30 DEBUG opendrift.models.basemodel:2056: 4.495231268758131 <- longitude -> 4.506287767226153
17:36:30 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:30 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 02:00:00 (before)
2025-01-11 03:00:00 (after)
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:32 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-11 02:00:00)
17:36:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 02:00:00) in space (linearNDFast)
17:36:32 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:32 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50477965678654 and -65.49372315370067 degrees.
17:36:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50477965678654 and -65.49372315370067 degrees.
17:36:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:32 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.00333729 (min) 0.0240672 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0263263 (min) -0.00841098 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.714738 (min) -0.713182 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.61837 (min) -1.6064 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.44506 (min) -8.4241 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.29824e-05 (min) -1.91683e-05 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.537 (min) 297.947 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.810182, mean: 1.814181, max: 1.817931
17:36:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:32 DEBUG opendrift.models.physics_methods:1061: min: 7.329776, mean: 7.337867, max: 7.345447
17:36:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.329776, mean: 7.337867, max: 7.345447
17:36:32 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:32 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:32 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:32 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.171563 m/s - 0.171930 m/s)
17:36:32 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:32 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:32 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:32 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:32 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:32 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:32 INFO opendrift.models.basemodel:2032: 2025-01-11 03:00:00 - step 4 of 71 - 1000 active elements (0 deactivated)
17:36:32 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:32 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:32 DEBUG opendrift.models.basemodel:2051: 59.97481112515269 <- latitude -> 59.98118303989345
17:36:32 DEBUG opendrift.models.basemodel:2056: 4.493372331996275 <- longitude -> 4.505640901495267
17:36:32 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:32 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 03:00:00 (before)
2025-01-11 04:00:00 (after)
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:33 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-11 03:00:00)
17:36:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 03:00:00) in space (linearNDFast)
17:36:33 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:33 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5066385931251 and -65.49437003092132 degrees.
17:36:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.5066385931251 and -65.49437003092132 degrees.
17:36:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0261764 (min) -0.00948368 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0550451 (min) 0.0657477 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.597457 (min) -0.595651 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.11173 (min) -2.07221 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.0639 (min) -8.02445 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.39918e-05 (min) 3.45642e-05 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.089 (min) 297.598 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.689824, mean: 1.699174, max: 1.709352
17:36:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:33 DEBUG opendrift.models.physics_methods:1061: min: 7.081909, mean: 7.101472, max: 7.122711
17:36:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.081909, mean: 7.101472, max: 7.122711
17:36:33 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:33 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:33 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:33 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.165761 m/s - 0.166716 m/s)
17:36:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:33 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:33 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:33 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:33 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:33 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:33 INFO opendrift.models.basemodel:2032: 2025-01-11 04:00:00 - step 5 of 71 - 1000 active elements (0 deactivated)
17:36:33 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:33 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:33 DEBUG opendrift.models.basemodel:2051: 59.97171660524069 <- latitude -> 59.977769454970186
17:36:33 DEBUG opendrift.models.basemodel:2056: 4.489012839664575 <- longitude -> 4.5022646199067236
17:36:33 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:33 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 04:00:00 (before)
2025-01-11 05:00:00 (after)
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:34 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-11 04:00:00)
17:36:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 04:00:00) in space (linearNDFast)
17:36:34 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:34 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51099809612052 and -65.49774630536227 degrees.
17:36:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51099809612052 and -65.49774630536227 degrees.
17:36:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:34 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0261125 (min) -0.013582 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.141867 (min) 0.153997 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.456874 (min) -0.454275 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.43844 (min) -1.39596 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.50383 (min) -7.42503 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.07132e-05 (min) 4.08934e-05 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.803 (min) 297.322 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.404348, mean: 1.419107, max: 1.435742
17:36:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:34 DEBUG opendrift.models.physics_methods:1061: min: 6.456050, mean: 6.489877, max: 6.527815
17:36:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 6.456050, mean: 6.489877, max: 6.527815
17:36:34 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:34 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:34 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.151112 m/s - 0.152792 m/s)
17:36:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:34 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:34 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:34 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:34 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:34 INFO opendrift.models.basemodel:2032: 2025-01-11 05:00:00 - step 6 of 71 - 1000 active elements (0 deactivated)
17:36:34 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:34 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:34 DEBUG opendrift.models.basemodel:2051: 59.97184576044411 <- latitude -> 59.97754037006435
17:36:34 DEBUG opendrift.models.basemodel:2056: 4.485640289103096 <- longitude -> 4.499480755734126
17:36:34 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:34 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 05:00:00 (before)
2025-01-11 06:00:00 (after)
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:36 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 05:00:00)
17:36:36 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 05:00:00) in space (linearNDFast)
17:36:36 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:36 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51437063902036 and -65.50053017995474 degrees.
17:36:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51437063902036 and -65.50053017995474 degrees.
17:36:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:36 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0189633 (min) 0.051294 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.200789 (min) 0.214313 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.309297 (min) -0.307518 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.28004 (min) -1.26432 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.98006 (min) -6.87668 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.26599e-05 (min) 5.29343e-05 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.772 (min) 297.264 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:36 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.202722, mean: 1.220645, max: 1.238534
17:36:36 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:36 DEBUG opendrift.models.physics_methods:1061: min: 5.974646, mean: 6.018980, max: 6.062945
17:36:36 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.974646, mean: 6.018980, max: 6.062945
17:36:36 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:36 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:36 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:36 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.139844 m/s - 0.141911 m/s)
17:36:36 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:36 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:36 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:36 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:36 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:36 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:36 INFO opendrift.models.basemodel:2032: 2025-01-11 06:00:00 - step 7 of 71 - 1000 active elements (0 deactivated)
17:36:36 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:36 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:36 DEBUG opendrift.models.basemodel:2051: 59.974273132816045 <- latitude -> 59.97955381863777
17:36:36 DEBUG opendrift.models.basemodel:2056: 4.485978370070531 <- longitude -> 4.500641428280635
17:36:36 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:36 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 06:00:00 (before)
2025-01-11 07:00:00 (after)
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:37 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 06:00:00)
17:36:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 06:00:00) in space (linearNDFast)
17:36:37 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:37 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51403256771981 and -65.4993694977281 degrees.
17:36:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.51403256771981 and -65.4993694977281 degrees.
17:36:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:37 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0989847 (min) 0.137595 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.192913 (min) 0.197805 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.139302 (min) -0.137866 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.715419 (min) -0.702794 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.99927 (min) -6.8861 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.33699e-05 (min) 4.35014e-05 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 296.966 (min) 297.401 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.178840, mean: 1.198107, max: 1.217565
17:36:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:37 DEBUG opendrift.models.physics_methods:1061: min: 5.915030, mean: 5.963153, max: 6.011401
17:36:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.915030, mean: 5.963153, max: 6.011401
17:36:37 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:37 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:37 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:37 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.138449 m/s - 0.140705 m/s)
17:36:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:37 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:37 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:37 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:37 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:37 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:37 INFO opendrift.models.basemodel:2032: 2025-01-11 07:00:00 - step 8 of 71 - 1000 active elements (0 deactivated)
17:36:37 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:37 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:37 DEBUG opendrift.models.basemodel:2051: 59.97606461732485 <- latitude -> 59.98140923647237
17:36:37 DEBUG opendrift.models.basemodel:2056: 4.492278344625498 <- longitude -> 4.5081702350132185
17:36:37 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:37 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 07:00:00 (before)
2025-01-11 08:00:00 (after)
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:38 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-11 07:00:00)
17:36:38 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 07:00:00) in space (linearNDFast)
17:36:38 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:38 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50773258391567 and -65.4918406948722 degrees.
17:36:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.50773258391567 and -65.4918406948722 degrees.
17:36:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:38 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.1733 (min) 0.201065 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.124665 (min) 0.133417 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.00981673 (min) -0.00865051 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.866055 (min) -0.852133 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.24313 (min) -6.18181 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.89429e-05 (min) 1.94251e-05 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.202 (min) 297.611 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:38 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.958524, mean: 0.966320, max: 0.976689
17:36:38 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:38 DEBUG opendrift.models.physics_methods:1061: min: 5.333729, mean: 5.355372, max: 5.384034
17:36:38 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.333729, mean: 5.355372, max: 5.384034
17:36:38 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:38 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:38 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:38 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:38 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.124843 m/s - 0.126020 m/s)
17:36:38 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:38 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:38 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:38 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:38 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:38 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:38 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:38 INFO opendrift.models.basemodel:2032: 2025-01-11 08:00:00 - step 9 of 71 - 1000 active elements (0 deactivated)
17:36:38 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:38 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:38 DEBUG opendrift.models.basemodel:2051: 59.9760981711631 <- latitude -> 59.98171213866539
17:36:38 DEBUG opendrift.models.basemodel:2056: 4.502967176756443 <- longitude -> 4.51975313257609
17:36:38 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:38 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 08:00:00 (before)
2025-01-11 09:00:00 (after)
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:39 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 08:00:00)
17:36:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 08:00:00) in space (linearNDFast)
17:36:39 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:39 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49704375029656 and -65.4802578031726 degrees.
17:36:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.49704375029656 and -65.4802578031726 degrees.
17:36:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:39 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.223672 (min) 0.243852 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0505621 (min) 0.0594983 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0314569 (min) 0.0329843 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.635929 (min) -0.509189 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.60515 (min) -6.43301 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.00466e-05 (min) -9.16418e-06 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.397 (min) 297.796 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:39 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.027987, mean: 1.053371, max: 1.079629
17:36:39 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:39 DEBUG opendrift.models.physics_methods:1061: min: 5.523614, mean: 5.591359, max: 5.660657
17:36:39 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.523614, mean: 5.591359, max: 5.660657
17:36:39 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:39 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:39 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:39 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.129287 m/s - 0.132495 m/s)
17:36:39 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:39 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:39 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:39 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:39 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:39 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:39 INFO opendrift.models.basemodel:2032: 2025-01-11 09:00:00 - step 10 of 71 - 1000 active elements (0 deactivated)
17:36:39 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:39 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:39 DEBUG opendrift.models.basemodel:2051: 59.973805375529466 <- latitude -> 59.979152527849955
17:36:39 DEBUG opendrift.models.basemodel:2056: 4.517400589720703 <- longitude -> 4.534324787047799
17:36:39 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:39 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 09:00:00 (before)
2025-01-11 10:00:00 (after)
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:41 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 09:00:00)
17:36:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 09:00:00) in space (linearNDFast)
17:36:41 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:41 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48261034472999 and -65.46568615368999 degrees.
17:36:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.48261034472999 and -65.46568615368999 degrees.
17:36:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.265701 (min) 0.291542 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0241131 (min) 0.0553339 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0380497 (min) -0.0355991 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.16879 (min) -1.06231 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.37064 (min) -5.25735 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.89164e-05 (min) -3.86085e-05 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.497 (min) 297.877 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.713543, mean: 0.725478, max: 0.737438
17:36:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:41 DEBUG opendrift.models.physics_methods:1061: min: 4.601926, mean: 4.640237, max: 4.678347
17:36:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.601926, mean: 4.640237, max: 4.678347
17:36:41 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:41 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:41 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:41 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.107714 m/s - 0.109503 m/s)
17:36:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:41 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:41 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:41 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:41 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:41 INFO opendrift.models.basemodel:2032: 2025-01-11 10:00:00 - step 11 of 71 - 1000 active elements (0 deactivated)
17:36:41 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:41 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:41 DEBUG opendrift.models.basemodel:2051: 59.97214753548138 <- latitude -> 59.97648991008711
17:36:41 DEBUG opendrift.models.basemodel:2056: 4.534082412807478 <- longitude -> 4.5510874568765045
17:36:41 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:41 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 10:00:00 (before)
2025-01-11 11:00:00 (after)
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:42 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x24x2) for time before (2025-01-11 10:00:00)
17:36:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 10:00:00) in space (linearNDFast)
17:36:42 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:42 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46592850522856 and -65.4489234731135 degrees.
17:36:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46592850522856 and -65.4489234731135 degrees.
17:36:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.333282 (min) 0.356602 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0241131 (min) 0.0342193 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.224206 (min) -0.222843 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.28282 (min) -1.26417 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.94131 (min) -3.85228 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.05388e-05 (min) -6.04199e-05 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.691 (min) 297.993 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.405345, mean: 0.412519, max: 0.421789
17:36:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:42 DEBUG opendrift.models.physics_methods:1061: min: 3.468501, mean: 3.499044, max: 3.538157
17:36:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.468501, mean: 3.499044, max: 3.538157
17:36:42 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:42 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:42 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:42 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.081185 m/s - 0.082815 m/s)
17:36:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:42 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:42 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:42 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:42 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:42 INFO opendrift.models.basemodel:2032: 2025-01-11 11:00:00 - step 12 of 71 - 1000 active elements (0 deactivated)
17:36:42 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:42 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:42 DEBUG opendrift.models.basemodel:2051: 59.97059127811301 <- latitude -> 59.97483841471826
17:36:42 DEBUG opendrift.models.basemodel:2056: 4.554688800136138 <- longitude -> 4.5719565289625095
17:36:42 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:42 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 11:00:00 (before)
2025-01-11 12:00:00 (after)
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:43 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 11:00:00)
17:36:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 11:00:00) in space (linearNDFast)
17:36:43 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:43 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44532213429143 and -65.42805440645367 degrees.
17:36:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44532213429143 and -65.42805440645367 degrees.
17:36:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.381591 (min) 0.387685 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0304098 (min) -0.0219725 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.454384 (min) -0.452452 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.32134 (min) -1.31099 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.65683 (min) -3.55804 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.10819e-05 (min) -6.06417e-05 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 297.998 (min) 298.271 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.353943, mean: 0.362260, max: 0.371849
17:36:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:43 DEBUG opendrift.models.physics_methods:1061: min: 3.241131, mean: 3.278962, max: 3.322103
17:36:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 3.241131, mean: 3.278962, max: 3.322103
17:36:43 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:43 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:43 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:43 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.075863 m/s - 0.077758 m/s)
17:36:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:43 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:43 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:43 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:43 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:43 INFO opendrift.models.basemodel:2032: 2025-01-11 12:00:00 - step 13 of 71 - 1000 active elements (0 deactivated)
17:36:43 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:43 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:43 DEBUG opendrift.models.basemodel:2051: 59.96728562327035 <- latitude -> 59.97182252266313
17:36:43 DEBUG opendrift.models.basemodel:2056: 4.577809141577973 <- longitude -> 4.594851384344319
17:36:43 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:43 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 12:00:00 (before)
2025-01-11 13:00:00 (after)
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:44 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-11 12:00:00)
17:36:44 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 12:00:00) in space (linearNDFast)
17:36:44 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:44 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42220178758515 and -65.40515953315897 degrees.
17:36:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42220178758515 and -65.40515953315897 degrees.
17:36:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:44 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.36091 (min) 0.387693 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0605161 (min) -0.0317066 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.64083 (min) -0.637864 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.565515 (min) -0.545974 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.50118 (min) -3.3926 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.50632e-05 (min) -3.48112e-05 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.242 (min) 298.504 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.291007, mean: 0.299629, max: 0.308889
17:36:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:44 DEBUG opendrift.models.physics_methods:1061: min: 2.938875, mean: 2.982060, max: 3.027824
17:36:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.938875, mean: 2.982060, max: 3.027824
17:36:44 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:44 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:44 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:44 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.068788 m/s - 0.070870 m/s)
17:36:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:44 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:44 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:44 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:44 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:44 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:44 INFO opendrift.models.basemodel:2032: 2025-01-11 13:00:00 - step 14 of 71 - 1000 active elements (0 deactivated)
17:36:44 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:44 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:44 DEBUG opendrift.models.basemodel:2051: 59.96374961591877 <- latitude -> 59.967727424462225
17:36:44 DEBUG opendrift.models.basemodel:2056: 4.602048476625936 <- longitude -> 4.617409005657026
17:36:44 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:44 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 13:00:00 (before)
2025-01-11 14:00:00 (after)
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:46 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-11 13:00:00)
17:36:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 13:00:00) in space (linearNDFast)
17:36:46 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:46 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39796244561853 and -65.3826019134292 degrees.
17:36:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39796244561853 and -65.3826019134292 degrees.
17:36:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.30618 (min) 0.333856 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00778391 (min) 0.027319 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.720306 (min) -0.716689 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.266892 (min) -0.2419 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.39797 (min) -3.31302 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.82697e-06 (min) -8.75554e-06 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.475 (min) 298.746 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.271764, mean: 0.278342, max: 0.285482
17:36:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:46 DEBUG opendrift.models.physics_methods:1061: min: 2.840046, mean: 2.874190, max: 2.910846
17:36:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.840046, mean: 2.874190, max: 2.910846
17:36:46 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:46 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:46 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:46 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.066475 m/s - 0.068132 m/s)
17:36:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:46 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:46 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:46 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:46 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:46 INFO opendrift.models.basemodel:2032: 2025-01-11 14:00:00 - step 15 of 71 - 1000 active elements (0 deactivated)
17:36:46 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:46 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:46 DEBUG opendrift.models.basemodel:2051: 59.96203440601768 <- latitude -> 59.965499428065996
17:36:46 DEBUG opendrift.models.basemodel:2056: 4.623223124764574 <- longitude -> 4.63683042187158
17:36:46 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:46 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 14:00:00 (before)
2025-01-11 15:00:00 (after)
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:47 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-11 14:00:00)
17:36:47 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 14:00:00) in space (linearNDFast)
17:36:47 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:47 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37678780679435 and -65.3631804984475 degrees.
17:36:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37678780679435 and -65.3631804984475 degrees.
17:36:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:47 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.26272 (min) 0.284156 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0546003 (min) 0.0738397 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.696098 (min) -0.693168 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.112227 (min) 0.114737 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.29217 (min) -3.22439 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.64901e-05 (min) 1.66141e-05 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.763 (min) 299.042 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.256074, mean: 0.261343, max: 0.266937
17:36:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:47 DEBUG opendrift.models.physics_methods:1061: min: 2.756842, mean: 2.785046, max: 2.814713
17:36:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.756842, mean: 2.785046, max: 2.814713
17:36:47 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:47 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:47 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:47 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064527 m/s - 0.065882 m/s)
17:36:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:47 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:47 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:47 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:47 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:47 INFO opendrift.models.basemodel:2032: 2025-01-11 15:00:00 - step 16 of 71 - 1000 active elements (0 deactivated)
17:36:47 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:47 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:47 DEBUG opendrift.models.basemodel:2051: 59.961764950458985 <- latitude -> 59.96565439126498
17:36:47 DEBUG opendrift.models.basemodel:2056: 4.641576936713147 <- longitude -> 4.653917638514869
17:36:47 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:47 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 15:00:00 (before)
2025-01-11 16:00:00 (after)
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:48 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 15:00:00)
17:36:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 15:00:00) in space (linearNDFast)
17:36:48 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:48 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.35843398896895 and -65.34609328843915 degrees.
17:36:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.35843398896895 and -65.34609328843915 degrees.
17:36:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.196506 (min) 0.222549 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0813321 (min) 0.106421 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.606261 (min) -0.60362 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.628972 (min) -0.591594 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.23468 (min) -3.18811 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.01619e-05 (min) 3.02817e-05 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.03 (min) 299.272 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.259028, mean: 0.262906, max: 0.266690
17:36:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:48 DEBUG opendrift.models.physics_methods:1061: min: 2.772702, mean: 2.793372, max: 2.813411
17:36:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.772702, mean: 2.793372, max: 2.813411
17:36:48 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:48 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:48 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:48 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.064899 m/s - 0.065852 m/s)
17:36:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:48 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:48 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:48 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:48 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:48 INFO opendrift.models.basemodel:2032: 2025-01-11 16:00:00 - step 17 of 71 - 1000 active elements (0 deactivated)
17:36:48 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:48 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:48 DEBUG opendrift.models.basemodel:2051: 59.962303623269875 <- latitude -> 59.96702646456477
17:36:48 DEBUG opendrift.models.basemodel:2056: 4.6550067533238355 <- longitude -> 4.665772007423337
17:36:48 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:48 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 16:00:00 (before)
2025-01-11 17:00:00 (after)
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:49 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x22x2) for time before (2025-01-11 16:00:00)
17:36:49 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 16:00:00) in space (linearNDFast)
17:36:49 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:49 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34500417931328 and -65.33423891859279 degrees.
17:36:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34500417931328 and -65.33423891859279 degrees.
17:36:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.114543 (min) 0.14608 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.114092 (min) 0.142509 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.479382 (min) -0.477031 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.21381 (min) -1.19888 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.61875 (min) -2.56611 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.62635e-05 (min) 3.65343e-05 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.049 (min) 299.385 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.197983, mean: 0.201130, max: 0.204362
17:36:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:49 DEBUG opendrift.models.physics_methods:1061: min: 2.424063, mean: 2.443243, max: 2.462804
17:36:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.424063, mean: 2.443243, max: 2.462804
17:36:49 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:49 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:49 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:49 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.056738 m/s - 0.057645 m/s)
17:36:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:49 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:49 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:49 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:49 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:49 INFO opendrift.models.basemodel:2032: 2025-01-11 17:00:00 - step 18 of 71 - 1000 active elements (0 deactivated)
17:36:49 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:49 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:49 DEBUG opendrift.models.basemodel:2051: 59.96432257321534 <- latitude -> 59.969954368568494
17:36:49 DEBUG opendrift.models.basemodel:2056: 4.662399308146423 <- longitude -> 4.671951678524563
17:36:49 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:49 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 17:00:00 (before)
2025-01-11 18:00:00 (after)
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:50 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-11 17:00:00)
17:36:50 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 17:00:00) in space (linearNDFast)
17:36:50 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:50 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33761161618891 and -65.32805923702662 degrees.
17:36:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33761161618891 and -65.32805923702662 degrees.
17:36:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.051936 (min) 0.0802498 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.173557 (min) 0.233623 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.336758 (min) -0.334729 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.170251 (min) -0.154842 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.71734 (min) -2.67086 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.04552e-05 (min) 4.07391e-05 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.153 (min) 299.529 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.176085, mean: 0.179136, max: 0.182325
17:36:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:50 DEBUG opendrift.models.physics_methods:1061: min: 2.286078, mean: 2.305786, max: 2.326232
17:36:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.286078, mean: 2.305786, max: 2.326232
17:36:50 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:50 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:50 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:50 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.053509 m/s - 0.054449 m/s)
17:36:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:50 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:50 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:50 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:50 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:50 INFO opendrift.models.basemodel:2032: 2025-01-11 18:00:00 - step 19 of 71 - 1000 active elements (0 deactivated)
17:36:50 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:50 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:50 DEBUG opendrift.models.basemodel:2051: 59.968506969286615 <- latitude -> 59.9752314598785
17:36:50 DEBUG opendrift.models.basemodel:2056: 4.666925985765665 <- longitude -> 4.675445129461778
17:36:50 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:50 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 18:00:00 (before)
2025-01-11 19:00:00 (after)
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:52 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-11 18:00:00)
17:36:52 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 18:00:00) in space (linearNDFast)
17:36:52 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:52 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33308493772962 and -65.32456578661767 degrees.
17:36:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33308493772962 and -65.32456578661767 degrees.
17:36:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:52 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.034289 (min) 0.0569343 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.211075 (min) 0.276108 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.181109 (min) -0.179942 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.54601 (min) -0.493504 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.95241 (min) -1.91174 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.42954e-05 (min) 4.4467e-05 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.417 (min) 299.843 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:52 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.096137, mean: 0.098408, max: 0.100790
17:36:52 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:52 DEBUG opendrift.models.physics_methods:1061: min: 1.689180, mean: 1.708995, max: 1.729569
17:36:52 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.689180, mean: 1.708995, max: 1.729569
17:36:52 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:52 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:52 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:52 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:52 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.039537 m/s - 0.040483 m/s)
17:36:52 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:52 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:52 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:52 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:52 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:52 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:52 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:52 INFO opendrift.models.basemodel:2032: 2025-01-11 19:00:00 - step 20 of 71 - 1000 active elements (0 deactivated)
17:36:52 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:52 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:52 DEBUG opendrift.models.basemodel:2051: 59.97432830036097 <- latitude -> 59.98246725762972
17:36:52 DEBUG opendrift.models.basemodel:2056: 4.668966521861544 <- longitude -> 4.677812510366006
17:36:52 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:52 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 19:00:00 (before)
2025-01-11 20:00:00 (after)
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:53 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-11 19:00:00)
17:36:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 19:00:00) in space (linearNDFast)
17:36:53 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:53 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33104439255906 and -65.32219840932183 degrees.
17:36:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33104439255906 and -65.32219840932183 degrees.
17:36:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0513345 (min) 0.0705025 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.194708 (min) 0.261313 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0272315 (min) -0.0255531 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.273366 (min) -0.251497 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.889371 (min) -0.80812 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.82119e-05 (min) 3.82878e-05 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.802 (min) 300.247 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.017902, mean: 0.019362, max: 0.021034
17:36:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:53 DEBUG opendrift.models.physics_methods:1061: min: 0.728925, mean: 0.758005, max: 0.790120
17:36:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.728925, mean: 0.758005, max: 0.790120
17:36:53 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:53 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:53 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:53 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.017061 m/s - 0.018494 m/s)
17:36:53 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:53 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:53 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:53 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:53 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:53 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:53 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:53 INFO opendrift.models.basemodel:2032: 2025-01-11 20:00:00 - step 21 of 71 - 1000 active elements (0 deactivated)
17:36:53 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:53 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:53 DEBUG opendrift.models.basemodel:2051: 59.98021453604092 <- latitude -> 59.99007399864844
17:36:53 DEBUG opendrift.models.basemodel:2056: 4.672183920907031 <- longitude -> 4.681631187493185
17:36:53 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:53 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:53 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:53 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:53 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:53 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:53 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:53 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:53 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:53 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 20:00:00 (before)
2025-01-11 21:00:00 (after)
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:54 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x2) for time before (2025-01-11 20:00:00)
17:36:54 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 20:00:00) in space (linearNDFast)
17:36:54 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:54 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32782699202842 and -65.31837972369411 degrees.
17:36:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32782699202842 and -65.31837972369411 degrees.
17:36:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:54 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0687269 (min) 0.0815171 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.166984 (min) 0.242912 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0653243 (min) 0.0666338 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.855037 (min) -0.799074 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.467746 (min) -0.427694 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.2891e-06 (min) 9.56563e-06 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.149 (min) 300.54 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:54 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020207, mean: 0.021697, max: 0.023291
17:36:54 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:54 DEBUG opendrift.models.physics_methods:1061: min: 0.774436, mean: 0.802418, max: 0.831435
17:36:54 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.774436, mean: 0.802418, max: 0.831435
17:36:54 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:54 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:54 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:54 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.018127 m/s - 0.019461 m/s)
17:36:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:54 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:54 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:54 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:54 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:54 INFO opendrift.models.basemodel:2032: 2025-01-11 21:00:00 - step 22 of 71 - 1000 active elements (0 deactivated)
17:36:54 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:54 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:54 DEBUG opendrift.models.basemodel:2051: 59.98548955125115 <- latitude -> 59.99762337450098
17:36:54 DEBUG opendrift.models.basemodel:2056: 4.675919618704093 <- longitude -> 4.685430293816793
17:36:54 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:54 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 21:00:00 (before)
2025-01-11 22:00:00 (after)
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:55 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x2) for time before (2025-01-11 21:00:00)
17:36:55 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 21:00:00) in space (linearNDFast)
17:36:55 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:55 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32409128805713 and -65.31458061540204 degrees.
17:36:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32409128805713 and -65.31458061540204 degrees.
17:36:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:55 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0856775 (min) 0.0959271 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.157196 (min) 0.238832 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.031382 (min) 0.0325927 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.959363 (min) -0.910001 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0752877 (min) 0.102242 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.75712e-05 (min) -2.70766e-05 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.395 (min) 300.656 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:55 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.020517, mean: 0.021775, max: 0.022893
17:36:55 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:55 DEBUG opendrift.models.physics_methods:1061: min: 0.780339, mean: 0.803877, max: 0.824291
17:36:55 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.780339, mean: 0.803877, max: 0.824291
17:36:55 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:55 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:55 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:55 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:55 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.018265 m/s - 0.019294 m/s)
17:36:55 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:55 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:55 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:55 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:55 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:55 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:55 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:55 INFO opendrift.models.basemodel:2032: 2025-01-11 22:00:00 - step 23 of 71 - 1000 active elements (0 deactivated)
17:36:55 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:55 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:55 DEBUG opendrift.models.basemodel:2051: 59.99084183543532 <- latitude -> 60.00540584470957
17:36:55 DEBUG opendrift.models.basemodel:2056: 4.680668241039926 <- longitude -> 4.6901155438343025
17:36:55 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:55 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 22:00:00 (before)
2025-01-11 23:00:00 (after)
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:56 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x23x2) for time before (2025-01-11 22:00:00)
17:36:56 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 22:00:00) in space (linearNDFast)
17:36:56 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:56 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31934267317374 and -65.30989536433579 degrees.
17:36:56 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31934267317374 and -65.30989536433579 degrees.
17:36:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:56 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:56 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:56 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:56 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:56 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.122055 (min) 0.129515 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.167113 (min) 0.24861 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.130006 (min) -0.129099 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.252262 (min) -0.197244 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.30355 (min) 0.355878 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.80889e-05 (min) -5.76524e-05 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.506 (min) 300.652 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:56 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:56 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.003224, mean: 0.003875, max: 0.004681
17:36:56 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:56 DEBUG opendrift.models.physics_methods:1061: min: 0.309323, mean: 0.338952, max: 0.372734
17:36:56 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.309323, mean: 0.338952, max: 0.372734
17:36:56 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:56 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:56 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:56 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:56 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.007240 m/s - 0.008724 m/s)
17:36:56 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:56 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:56 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:56 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:56 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:56 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:56 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:56 INFO opendrift.models.basemodel:2032: 2025-01-11 23:00:00 - step 24 of 71 - 1000 active elements (0 deactivated)
17:36:56 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:56 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:56 DEBUG opendrift.models.basemodel:2051: 59.9966153363131 <- latitude -> 60.013668753571196
17:36:56 DEBUG opendrift.models.basemodel:2056: 4.68859463250398 <- longitude -> 4.697765192965255
17:36:56 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:56 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:56 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:56 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:56 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:56 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-11 23:00:00 (before)
2025-01-12 00:00:00 (after)
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:58 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x22x2) for time before (2025-01-11 23:00:00)
17:36:58 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-11 23:00:00) in space (linearNDFast)
17:36:58 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:58 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31141627500779 and -65.30224570523316 degrees.
17:36:58 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31141627500779 and -65.30224570523316 degrees.
17:36:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:58 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:58 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:58 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:58 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:58 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.137863 (min) 0.162363 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.196234 (min) 0.293395 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.375924 (min) -0.374117 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.00722 (min) 1.01658 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0317464 (min) 0.123029 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.92679e-05 (min) -6.89609e-05 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.048 (min) 300.549 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:58 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:58 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.024981, mean: 0.025302, max: 0.025795
17:36:58 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:58 DEBUG opendrift.models.physics_methods:1061: min: 0.861069, mean: 0.866568, max: 0.874974
17:36:58 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 0.861069, mean: 0.866568, max: 0.874974
17:36:58 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:58 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:58 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:58 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:58 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.020154 m/s - 0.020480 m/s)
17:36:58 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:58 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:58 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:58 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:58 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:58 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:58 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:58 INFO opendrift.models.basemodel:2032: 2025-01-12 00:00:00 - step 25 of 71 - 1000 active elements (0 deactivated)
17:36:58 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:58 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:58 DEBUG opendrift.models.basemodel:2051: 60.00306365215231 <- latitude -> 60.02322822246655
17:36:58 DEBUG opendrift.models.basemodel:2056: 4.70037116092166 <- longitude -> 4.707978403457643
17:36:58 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:58 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:58 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:58 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:58 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:58 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:58 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:58 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:58 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:58 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:58 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 00:00:00 (before)
2025-01-12 01:00:00 (after)
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:36:59 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x22x2) for time before (2025-01-12 00:00:00)
17:36:59 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 00:00:00) in space (linearNDFast)
17:36:59 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:36:59 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:36:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29963974331434 and -65.29203249680828 degrees.
17:36:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29963974331434 and -65.29203249680828 degrees.
17:36:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:36:59 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:36:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:36:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:36:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0983149 (min) 0.155663 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.267038 (min) 0.381604 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.600406 (min) -0.596715 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0625515 (min) 0.00858001 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.34036 (min) 1.43305 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.00362e-07 (min) -1.80002e-07 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 299.255 (min) 300.041 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:36:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:36:59 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.044197, mean: 0.046961, max: 0.050616
17:36:59 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:36:59 DEBUG opendrift.models.physics_methods:1061: min: 1.145319, mean: 1.180514, max: 1.225666
17:36:59 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.145319, mean: 1.180514, max: 1.225666
17:36:59 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:36:59 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:59 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:36:59 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:36:59 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.026808 m/s - 0.028688 m/s)
17:36:59 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:36:59 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:36:59 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:36:59 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:36:59 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:36:59 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:36:59 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:36:59 INFO opendrift.models.basemodel:2032: 2025-01-12 01:00:00 - step 26 of 71 - 1000 active elements (0 deactivated)
17:36:59 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:36:59 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:36:59 DEBUG opendrift.models.basemodel:2051: 60.01256170158717 <- latitude -> 60.036484651703674
17:36:59 DEBUG opendrift.models.basemodel:2056: 4.71042627553912 <- longitude -> 4.714409056040815
17:36:59 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:36:59 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:36:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:36:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:36:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:36:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:36:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:36:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:36:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:36:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:36:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:36:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:36:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:36:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 01:00:00 (before)
2025-01-12 02:00:00 (after)
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:00 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x23x2) for time before (2025-01-12 01:00:00)
17:37:00 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 01:00:00) in space (linearNDFast)
17:37:00 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:00 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28958462387472 and -65.28560182347294 degrees.
17:37:00 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28958462387472 and -65.28560182347294 degrees.
17:37:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:00 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:00 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:00 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:00 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:00 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0353638 (min) 0.114789 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.354211 (min) 0.482208 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.739307 (min) -0.732583 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.825676 (min) -0.779431 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.64432 (min) 1.75175 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.90393e-05 (min) -1.78814e-05 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.722 (min) 299.839 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:00 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:00 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.081458, mean: 0.086062, max: 0.092259
17:37:00 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:00 DEBUG opendrift.models.physics_methods:1061: min: 1.554876, mean: 1.598130, max: 1.654759
17:37:00 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 1.554876, mean: 1.598130, max: 1.654759
17:37:00 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:00 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:00 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:00 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.036394 m/s - 0.038732 m/s)
17:37:00 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:00 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:00 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:00 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:00 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:00 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:00 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:00 INFO opendrift.models.basemodel:2032: 2025-01-12 02:00:00 - step 27 of 71 - 1000 active elements (0 deactivated)
17:37:00 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:00 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:00 DEBUG opendrift.models.basemodel:2051: 60.02506950462927 <- latitude -> 60.05316249904092
17:37:00 DEBUG opendrift.models.basemodel:2056: 4.715465338465818 <- longitude -> 4.717538864599493
17:37:00 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:00 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:00 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:00 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:00 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:00 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:00 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:00 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:00 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:00 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:00 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:00 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 02:00:00 (before)
2025-01-12 03:00:00 (after)
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:01 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x24x2) for time before (2025-01-12 02:00:00)
17:37:01 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 02:00:00) in space (linearNDFast)
17:37:01 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:01 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28454554624648 and -65.28247203176903 degrees.
17:37:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28454554624648 and -65.28247203176903 degrees.
17:37:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:01 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0225504 (min) 0.0598388 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.461391 (min) 0.591543 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.761775 (min) -0.752015 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.41852 (min) -1.39042 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.20558 (min) 2.30746 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.27559e-06 (min) 6.3233e-06 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.474 (min) 301.514 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:01 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.167273, mean: 0.172669, max: 0.180480
17:37:01 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:01 DEBUG opendrift.models.physics_methods:1061: min: 2.228138, mean: 2.263743, max: 2.314429
17:37:01 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.228138, mean: 2.263743, max: 2.314429
17:37:01 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:01 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:01 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:01 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:01 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.052152 m/s - 0.054172 m/s)
17:37:01 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:01 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:01 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:01 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:01 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:01 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:01 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:01 INFO opendrift.models.basemodel:2032: 2025-01-12 03:00:00 - step 28 of 71 - 1000 active elements (0 deactivated)
17:37:01 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:01 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:01 DEBUG opendrift.models.basemodel:2051: 60.04140335274356 <- latitude -> 60.07374125342362
17:37:01 DEBUG opendrift.models.basemodel:2056: 4.712172860813302 <- longitude -> 4.7189229551326335
17:37:01 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:01 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 03:00:00 (before)
2025-01-12 04:00:00 (after)
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:03 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x24x2) for time before (2025-01-12 03:00:00)
17:37:03 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 03:00:00) in space (linearNDFast)
17:37:03 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:03 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28783801593059 and -65.28108793369832 degrees.
17:37:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.28783801593059 and -65.28108793369832 degrees.
17:37:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:03 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0750173 (min) -6.32956e-05 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.577903 (min) 0.692958 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.707647 (min) -0.696004 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.87429 (min) -1.59851 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: 3.06374 (min) 3.29558 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.27182e-05 (min) 3.39482e-05 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.179 (min) 302.611 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:03 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.293766, mean: 0.324112, max: 0.353596
17:37:03 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:03 DEBUG opendrift.models.physics_methods:1061: min: 2.952777, mean: 3.101134, max: 3.239538
17:37:03 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 2.952777, mean: 3.101134, max: 3.239538
17:37:03 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:03 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:03 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:03 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.069114 m/s - 0.075826 m/s)
17:37:03 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:03 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:03 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:03 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:03 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:03 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:03 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:03 INFO opendrift.models.basemodel:2032: 2025-01-12 04:00:00 - step 29 of 71 - 1000 active elements (0 deactivated)
17:37:03 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:03 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:03 DEBUG opendrift.models.basemodel:2051: 60.06205653871415 <- latitude -> 60.09826172719717
17:37:03 DEBUG opendrift.models.basemodel:2056: 4.704893313180323 <- longitude -> 4.716852401475014
17:37:03 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:03 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 04:00:00 (before)
2025-01-12 05:00:00 (after)
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:04 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x2) for time before (2025-01-12 04:00:00)
17:37:04 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 04:00:00) in space (linearNDFast)
17:37:04 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:04 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.2951175556008 and -65.28315847698772 degrees.
17:37:04 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.2951175556008 and -65.28315847698772 degrees.
17:37:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:04 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:04 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:04 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0825795 (min) -0.0588259 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.696508 (min) 0.788064 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.572588 (min) -0.560278 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.47053 (min) -1.21054 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: y_wind: 4.7619 (min) 4.8641 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 4.16506e-05 (min) 4.31121e-05 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 302.246 (min) 303.099 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:04 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.598855, mean: 0.605279, max: 0.635219
17:37:04 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:04 DEBUG opendrift.models.physics_methods:1061: min: 4.215900, mean: 4.238379, max: 4.342014
17:37:04 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 4.215900, mean: 4.238379, max: 4.342014
17:37:04 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:04 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:04 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:04 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:04 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.098679 m/s - 0.101630 m/s)
17:37:04 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:04 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:04 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:04 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:04 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:04 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:04 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:04 INFO opendrift.models.basemodel:2032: 2025-01-12 05:00:00 - step 30 of 71 - 1000 active elements (0 deactivated)
17:37:04 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:04 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:04 DEBUG opendrift.models.basemodel:2051: 60.08770544005195 <- latitude -> 60.12673174754035
17:37:04 DEBUG opendrift.models.basemodel:2056: 4.698349143946271 <- longitude -> 4.711144966687878
17:37:04 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:04 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 05:00:00 (before)
2025-01-12 06:00:00 (after)
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:05 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 28x25x2) for time before (2025-01-12 05:00:00)
17:37:05 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 05:00:00) in space (linearNDFast)
17:37:05 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:05 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30166172694715 and -65.2888659050958 degrees.
17:37:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30166172694715 and -65.2888659050958 degrees.
17:37:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:05 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0669709 (min) -0.0567031 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.800462 (min) 0.840117 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.411636 (min) -0.405704 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.6175 (min) -1.53761 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: 5.65841 (min) 5.8498 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.1563e-05 (min) 5.34094e-05 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 303.389 (min) 304.093 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:05 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.845793, mean: 0.884081, max: 0.906124
17:37:05 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:05 DEBUG opendrift.models.physics_methods:1061: min: 5.010277, mean: 5.122309, max: 5.185890
17:37:05 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.010277, mean: 5.122309, max: 5.185890
17:37:05 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:05 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:05 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:05 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.117272 m/s - 0.121383 m/s)
17:37:05 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:05 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:05 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:05 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:05 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:05 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:05 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:05 INFO opendrift.models.basemodel:2032: 2025-01-12 06:00:00 - step 31 of 71 - 1000 active elements (0 deactivated)
17:37:05 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:05 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:05 DEBUG opendrift.models.basemodel:2051: 60.117350160523884 <- latitude -> 60.15739543957562
17:37:05 DEBUG opendrift.models.basemodel:2056: 4.692657592176683 <- longitude -> 4.704756849414703
17:37:05 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:05 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 06:00:00 (before)
2025-01-12 07:00:00 (after)
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:07 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x2) for time before (2025-01-12 06:00:00)
17:37:07 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 06:00:00) in space (linearNDFast)
17:37:07 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:07 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30735326905285 and -65.29525402217521 degrees.
17:37:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30735326905285 and -65.29525402217521 degrees.
17:37:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:07 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0379247 (min) -0.0323833 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.827463 (min) 0.849614 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.218842 (min) -0.217434 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.51695 (min) -1.38097 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: 6.01337 (min) 6.72191 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.28168e-05 (min) 5.52006e-05 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 304.562 (min) 305.202 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:07 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 0.946159, mean: 1.060727, max: 1.158452
17:37:07 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:07 DEBUG opendrift.models.physics_methods:1061: min: 5.299217, mean: 5.610017, max: 5.863659
17:37:07 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.299217, mean: 5.610017, max: 5.863659
17:37:07 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:07 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:07 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:07 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.124035 m/s - 0.137247 m/s)
17:37:07 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:07 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:07 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:07 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:07 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:07 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:07 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:07 INFO opendrift.models.basemodel:2032: 2025-01-12 07:00:00 - step 32 of 71 - 1000 active elements (0 deactivated)
17:37:07 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:07 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:07 DEBUG opendrift.models.basemodel:2051: 60.14843085320513 <- latitude -> 60.18856655532913
17:37:07 DEBUG opendrift.models.basemodel:2056: 4.688228537427647 <- longitude -> 4.700773135182342
17:37:07 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:07 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 07:00:00 (before)
2025-01-12 08:00:00 (after)
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:09 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x2) for time before (2025-01-12 07:00:00)
17:37:09 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 07:00:00) in space (linearNDFast)
17:37:09 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:09 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31178230224711 and -65.299237726361 degrees.
17:37:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31178230224711 and -65.299237726361 degrees.
17:37:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:09 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.00990526 (min) -0.00489199 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.790768 (min) 0.82119 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0275823 (min) -0.0248983 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.51146 (min) -2.00146 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: 6.52107 (min) 7.00142 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.17743e-05 (min) 5.24669e-05 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 301.208 (min) 306.141 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:09 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.201261, mean: 1.240514, max: 1.304434
17:37:09 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:09 DEBUG opendrift.models.physics_methods:1061: min: 5.971017, mean: 6.067621, max: 6.222151
17:37:09 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 5.971017, mean: 6.067621, max: 6.222151
17:37:09 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:09 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:09 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:09 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:09 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.139759 m/s - 0.145638 m/s)
17:37:09 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:09 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:09 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:09 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:09 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:09 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:09 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:09 INFO opendrift.models.basemodel:2032: 2025-01-12 08:00:00 - step 33 of 71 - 1000 active elements (0 deactivated)
17:37:09 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:09 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:09 DEBUG opendrift.models.basemodel:2051: 60.179260639132096 <- latitude -> 60.21833144611796
17:37:09 DEBUG opendrift.models.basemodel:2056: 4.684442308677881 <- longitude -> 4.697839157335178
17:37:09 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:09 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 08:00:00 (before)
2025-01-12 09:00:00 (after)
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:10 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 28x25x2) for time before (2025-01-12 08:00:00)
17:37:10 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 08:00:00) in space (linearNDFast)
17:37:10 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:10 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31556852728805 and -65.30217168929084 degrees.
17:37:10 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31556852728805 and -65.30217168929084 degrees.
17:37:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:10 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:10 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:10 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:10 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:10 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0306295 (min) 0.0400336 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.670798 (min) 0.760654 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.120944 (min) 0.127892 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.95904 (min) -1.7432 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.40493 (min) 8.48849 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.46046e-05 (min) 2.53108e-05 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.335 (min) 302.462 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:10 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:10 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.812566, mean: 1.851070, max: 1.858539
17:37:10 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:10 DEBUG opendrift.models.physics_methods:1061: min: 7.334602, mean: 7.412083, max: 7.427033
17:37:10 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.334602, mean: 7.412083, max: 7.427033
17:37:10 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:10 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:10 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:10 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:10 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.171676 m/s - 0.173839 m/s)
17:37:10 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:10 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:10 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:10 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:10 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:10 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:10 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:10 INFO opendrift.models.basemodel:2032: 2025-01-12 09:00:00 - step 34 of 71 - 1000 active elements (0 deactivated)
17:37:10 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:10 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:10 DEBUG opendrift.models.basemodel:2051: 60.209245131495535 <- latitude -> 60.24547081332363
17:37:10 DEBUG opendrift.models.basemodel:2056: 4.684497309264781 <- longitude -> 4.697619070428
17:37:10 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:10 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:10 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:10 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:10 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:10 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:10 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:10 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:10 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:10 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:10 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 09:00:00 (before)
2025-01-12 10:00:00 (after)
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:11 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x2) for time before (2025-01-12 09:00:00)
17:37:11 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 09:00:00) in space (linearNDFast)
17:37:11 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:11 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31551351370236 and -65.30239177181204 degrees.
17:37:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31551351370236 and -65.30239177181204 degrees.
17:37:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:11 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0580166 (min) 0.0616742 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.53561 (min) 0.642914 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.147769 (min) 0.152507 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.29525 (min) -2.25824 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.42585 (min) 8.49115 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.30492e-05 (min) -1.2805e-05 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 300.135 (min) 304.615 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:11 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 1.874159, mean: 1.881681, max: 1.901230
17:37:11 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:11 DEBUG opendrift.models.physics_methods:1061: min: 7.458180, mean: 7.473126, max: 7.511851
17:37:11 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.458180, mean: 7.473126, max: 7.511851
17:37:11 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:11 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:11 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:11 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:11 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.174568 m/s - 0.175825 m/s)
17:37:11 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:11 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:11 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:11 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:11 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:11 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:11 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:11 INFO opendrift.models.basemodel:2032: 2025-01-12 10:00:00 - step 35 of 71 - 1000 active elements (0 deactivated)
17:37:11 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:11 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:11 DEBUG opendrift.models.basemodel:2051: 60.23546898683253 <- latitude -> 60.268264073007195
17:37:11 DEBUG opendrift.models.basemodel:2056: 4.685432030069987 <- longitude -> 4.69869115623599
17:37:11 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:11 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 10:00:00 (before)
2025-01-12 11:00:00 (after)
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:12 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x25x2) for time before (2025-01-12 10:00:00)
17:37:12 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 10:00:00) in space (linearNDFast)
17:37:12 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:12 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31457879297741 and -65.30131967274284 degrees.
17:37:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31457879297741 and -65.30131967274284 degrees.
17:37:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:12 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0540407 (min) 0.0673612 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.428113 (min) 0.548044 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0258713 (min) 0.0277232 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.56553 (min) -2.55642 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.75261 (min) 8.81605 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.58344e-05 (min) -5.50427e-05 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 303.518 (min) 310.828 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:12 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.045329, mean: 2.061391, max: 2.072946
17:37:12 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:12 DEBUG opendrift.models.physics_methods:1061: min: 7.791321, mean: 7.821852, max: 7.843747
17:37:12 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 7.791321, mean: 7.821852, max: 7.843747
17:37:12 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:12 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:12 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:12 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.182366 m/s - 0.183593 m/s)
17:37:12 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:12 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:12 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:12 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:12 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:12 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:12 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:12 INFO opendrift.models.basemodel:2032: 2025-01-12 11:00:00 - step 36 of 71 - 1000 active elements (0 deactivated)
17:37:12 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:12 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:12 DEBUG opendrift.models.basemodel:2051: 60.258874011198756 <- latitude -> 60.2877528554113
17:37:12 DEBUG opendrift.models.basemodel:2056: 4.686487535697327 <- longitude -> 4.698877849332712
17:37:12 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:12 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 11:00:00 (before)
2025-01-12 12:00:00 (after)
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:14 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['x_sea_water_velocity']
17:37:14 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 27x24x2) for time before (2025-01-12 11:00:00)
17:37:14 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 11:00:00) in space (linearNDFast)
17:37:14 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:14 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31352328059126 and -65.30113297013638 degrees.
17:37:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31352328059126 and -65.30113297013638 degrees.
17:37:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:14 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0695205 (min) 0.0795583 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.351909 (min) 0.493998 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.222474 (min) -0.220803 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.34666 (min) -1.83547 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.21562 (min) 9.48396 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.56074e-05 (min) -7.46898e-05 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 309.81 (min) 317.96 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:14 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.224690, mean: 2.254206, max: 2.295536
17:37:14 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:14 DEBUG opendrift.models.physics_methods:1061: min: 8.125766, mean: 8.179460, max: 8.254136
17:37:14 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.125766, mean: 8.179460, max: 8.254136
17:37:14 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:14 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:14 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:14 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:14 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.190194 m/s - 0.193199 m/s)
17:37:14 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:14 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:14 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:14 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:14 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:14 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:14 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:14 INFO opendrift.models.basemodel:2032: 2025-01-12 12:00:00 - step 37 of 71 - 1000 active elements (0 deactivated)
17:37:14 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:14 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:14 DEBUG opendrift.models.basemodel:2051: 60.28096426341709 <- latitude -> 60.305078547343925
17:37:14 DEBUG opendrift.models.basemodel:2056: 4.688264555863092 <- longitude -> 4.701011976051709
17:37:14 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:14 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 12:00:00 (before)
2025-01-12 13:00:00 (after)
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:15 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:15 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 26x24x2) for time before (2025-01-12 12:00:00)
17:37:15 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 12:00:00) in space (linearNDFast)
17:37:15 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:15 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31174624939825 and -65.29899884231736 degrees.
17:37:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31174624939825 and -65.29899884231736 degrees.
17:37:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:15 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0678737 (min) 0.0823219 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.342259 (min) 0.452552 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.490318 (min) -0.487601 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.89121 (min) -1.73269 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.68609 (min) 9.70518 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.80371e-05 (min) -6.74822e-05 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 317.754 (min) 324.444 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:15 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.390556, mean: 2.393769, max: 2.402895
17:37:15 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:15 DEBUG opendrift.models.physics_methods:1061: min: 8.423237, mean: 8.428895, max: 8.444947
17:37:15 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.423237, mean: 8.428895, max: 8.444947
17:37:15 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:15 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:15 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:15 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:15 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.197157 m/s - 0.197665 m/s)
17:37:15 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:15 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:15 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:15 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:15 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:15 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:15 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:15 INFO opendrift.models.basemodel:2032: 2025-01-12 13:00:00 - step 38 of 71 - 1000 active elements (0 deactivated)
17:37:15 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:15 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:15 DEBUG opendrift.models.basemodel:2051: 60.30185818931325 <- latitude -> 60.32240584166583
17:37:15 DEBUG opendrift.models.basemodel:2056: 4.6902214659585395 <- longitude -> 4.704019560333252
17:37:15 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:15 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 13:00:00 (before)
2025-01-12 14:00:00 (after)
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:16 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:16 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x2) for time before (2025-01-12 13:00:00)
17:37:16 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 13:00:00) in space (linearNDFast)
17:37:16 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:16 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30978932672696 and -65.29599124515289 degrees.
17:37:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30978932672696 and -65.29599124515289 degrees.
17:37:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:16 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0547786 (min) 0.0651769 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.366115 (min) 0.445125 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.699744 (min) -0.695497 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.44172 (min) -1.43014 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.97719 (min) 10.0373 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.27324e-05 (min) -4.23868e-05 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 325.422 (min) 328.497 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:16 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.499593, mean: 2.513249, max: 2.529262
17:37:16 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:16 DEBUG opendrift.models.physics_methods:1061: min: 8.613195, mean: 8.636685, max: 8.664161
17:37:16 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 8.613195, mean: 8.636685, max: 8.664161
17:37:16 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:16 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:16 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:16 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.201603 m/s - 0.202796 m/s)
17:37:16 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:16 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:16 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:16 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:16 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:16 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:16 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:16 INFO opendrift.models.basemodel:2032: 2025-01-12 14:00:00 - step 39 of 71 - 1000 active elements (0 deactivated)
17:37:16 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:16 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:16 DEBUG opendrift.models.basemodel:2051: 60.32272680032973 <- latitude -> 60.34068265722416
17:37:16 DEBUG opendrift.models.basemodel:2056: 4.692027110652659 <- longitude -> 4.706391478220369
17:37:16 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:16 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 14:00:00 (before)
2025-01-12 15:00:00 (after)
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:17 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:17 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x2) for time before (2025-01-12 14:00:00)
17:37:17 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 14:00:00) in space (linearNDFast)
17:37:17 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:17 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3079836830224 and -65.29361932331219 degrees.
17:37:17 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3079836830224 and -65.29361932331219 degrees.
17:37:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:17 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:17 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:17 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:17 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:17 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0129283 (min) 0.0194898 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.429737 (min) 0.49114 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.794319 (min) -0.788812 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.70262 (min) -2.60698 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.344 (min) 10.4032 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.75402e-06 (min) -5.39987e-06 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 327.426 (min) 329.465 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:17 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:17 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 2.811841, mean: 2.825762, max: 2.830028
17:37:17 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:17 DEBUG opendrift.models.physics_methods:1061: min: 9.135345, mean: 9.157930, max: 9.164842
17:37:17 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.135345, mean: 9.157930, max: 9.164842
17:37:17 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:17 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:17 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:17 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.213825 m/s - 0.214515 m/s)
17:37:17 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:17 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:17 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:17 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:17 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:17 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:17 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:17 INFO opendrift.models.basemodel:2032: 2025-01-12 15:00:00 - step 40 of 71 - 1000 active elements (0 deactivated)
17:37:17 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:17 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:17 DEBUG opendrift.models.basemodel:2051: 60.34528031042049 <- latitude -> 60.36132617043075
17:37:17 DEBUG opendrift.models.basemodel:2056: 4.689897139530516 <- longitude -> 4.703981743313033
17:37:17 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:17 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:17 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:17 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:17 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:17 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:17 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:17 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:17 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:17 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:17 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 15:00:00 (before)
2025-01-12 16:00:00 (after)
17:37:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:18 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-12 15:00:00)
17:37:18 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 15:00:00) in space (linearNDFast)
17:37:18 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:18 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31011365058285 and -65.29602904434346 degrees.
17:37:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31011365058285 and -65.29602904434346 degrees.
17:37:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:18 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0169856 (min) -0.00639939 (max)
17:37:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.553708 (min) 0.578767 (max)
17:37:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.769788 (min) -0.763338 (max)
17:37:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.79361 (min) -2.75361 (max)
17:37:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.908 (min) 11.0199 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.89164e-05 (min) 1.91106e-05 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 323.96 (min) 326.375 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:19 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:19 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.117072, mean: 3.151479, max: 3.176685
17:37:19 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:19 DEBUG opendrift.models.physics_methods:1061: min: 9.618403, mean: 9.671326, max: 9.709942
17:37:19 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.618403, mean: 9.671326, max: 9.709942
17:37:19 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:19 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:19 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:19 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:19 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.225131 m/s - 0.227274 m/s)
17:37:19 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:19 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:19 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:19 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:19 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:19 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:19 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:19 INFO opendrift.models.basemodel:2032: 2025-01-12 16:00:00 - step 41 of 71 - 1000 active elements (0 deactivated)
17:37:19 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:19 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:19 DEBUG opendrift.models.basemodel:2051: 60.37102950620877 <- latitude -> 60.38648075439158
17:37:19 DEBUG opendrift.models.basemodel:2056: 4.685857965025721 <- longitude -> 4.6992880814919955
17:37:19 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:19 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:19 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:19 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:19 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:19 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:19 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:19 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:19 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:19 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:19 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 16:00:00 (before)
2025-01-12 17:00:00 (after)
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:20 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:20 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-12 16:00:00)
17:37:20 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 16:00:00) in space (linearNDFast)
17:37:20 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:20 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31415280902613 and -65.30072270525523 degrees.
17:37:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31415280902613 and -65.30072270525523 degrees.
17:37:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:20 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.011506 (min) 0.00978421 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.684894 (min) 0.694123 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.663693 (min) -0.657033 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.26035 (min) -2.18542 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1279 (min) 11.1891 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.79649e-05 (min) 3.8007e-05 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 323.086 (min) 327.954 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:20 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.171904, mean: 3.184964, max: 3.198747
17:37:20 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:20 DEBUG opendrift.models.physics_methods:1061: min: 9.702633, mean: 9.722584, max: 9.743602
17:37:20 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.702633, mean: 9.722584, max: 9.743602
17:37:20 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:20 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:20 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:20 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:20 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.227103 m/s - 0.228062 m/s)
17:37:20 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:20 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:20 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:20 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:20 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:20 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:20 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:20 INFO opendrift.models.basemodel:2032: 2025-01-12 17:00:00 - step 42 of 71 - 1000 active elements (0 deactivated)
17:37:20 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:20 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:20 DEBUG opendrift.models.basemodel:2051: 60.4005418510947 <- latitude -> 60.41613865240457
17:37:20 DEBUG opendrift.models.basemodel:2056: 4.683624148588222 <- longitude -> 4.695594596685294
17:37:20 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:20 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 17:00:00 (before)
2025-01-12 18:00:00 (after)
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:21 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:21 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x2) for time before (2025-01-12 17:00:00)
17:37:21 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 17:00:00) in space (linearNDFast)
17:37:21 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:21 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31638662577456 and -65.30441618289754 degrees.
17:37:21 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31638662577456 and -65.30441618289754 degrees.
17:37:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:21 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:21 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:21 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:21 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:21 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0259912 (min) 0.0708196 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.786532 (min) 0.791573 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.506792 (min) -0.500516 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.45644 (min) -3.37378 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.2261 (min) 11.3253 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.15472e-05 (min) 5.19408e-05 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 330.781 (min) 338.268 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:21 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:21 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.394120, mean: 3.415139, max: 3.435241
17:37:21 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:21 DEBUG opendrift.models.physics_methods:1061: min: 10.036752, mean: 10.067776, max: 10.097369
17:37:21 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.036752, mean: 10.067776, max: 10.097369
17:37:21 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:21 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:21 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:21 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:21 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.234923 m/s - 0.236342 m/s)
17:37:21 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:21 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:21 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:21 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:21 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:21 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:21 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:21 INFO opendrift.models.basemodel:2032: 2025-01-12 18:00:00 - step 43 of 71 - 1000 active elements (0 deactivated)
17:37:21 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:21 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:21 DEBUG opendrift.models.basemodel:2051: 60.4332828899768 <- latitude -> 60.44903291797765
17:37:21 DEBUG opendrift.models.basemodel:2056: 4.683841814782847 <- longitude -> 4.692774190587349
17:37:21 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:21 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:21 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:21 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:21 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:21 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:21 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:21 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:21 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:21 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:21 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:21 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 18:00:00 (before)
2025-01-12 19:00:00 (after)
17:37:22 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:23 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:23 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:23 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['x_sea_water_velocity']
17:37:23 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x24x2) for time before (2025-01-12 18:00:00)
17:37:23 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 18:00:00) in space (linearNDFast)
17:37:23 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:23 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31616894444883 and -65.30723657483256 degrees.
17:37:23 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31616894444883 and -65.30723657483256 degrees.
17:37:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:23 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:23 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:23 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:23 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:23 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0884128 (min) 0.134991 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.805271 (min) 0.816179 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.292394 (min) -0.287724 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.1627 (min) -4.14472 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.5964 (min) 11.6828 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.54535e-05 (min) 6.58894e-05 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 332.655 (min) 334.239 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:23 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:23 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.734393, mean: 3.756398, max: 3.780199
17:37:23 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:23 DEBUG opendrift.models.physics_methods:1061: min: 10.527848, mean: 10.558814, max: 10.592218
17:37:23 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.527848, mean: 10.558814, max: 10.592218
17:37:23 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:23 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:23 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:23 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:23 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.246418 m/s - 0.247925 m/s)
17:37:23 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:23 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:23 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:23 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:23 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:23 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:23 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:23 INFO opendrift.models.basemodel:2032: 2025-01-12 19:00:00 - step 44 of 71 - 1000 active elements (0 deactivated)
17:37:23 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:23 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:23 DEBUG opendrift.models.basemodel:2051: 60.467007123762 <- latitude -> 60.482600380860006
17:37:23 DEBUG opendrift.models.basemodel:2056: 4.68711058504122 <- longitude -> 4.693110443546115
17:37:23 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:23 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:23 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:23 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:23 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:23 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:23 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:23 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:23 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:23 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:23 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:23 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 19:00:00 (before)
2025-01-12 20:00:00 (after)
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:24 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:24 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-12 19:00:00)
17:37:24 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 19:00:00) in space (linearNDFast)
17:37:24 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:24 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3129001637181 and -65.30690030776138 degrees.
17:37:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3129001637181 and -65.30690030776138 degrees.
17:37:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:24 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.146278 (min) 0.170875 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.66489 (min) 0.810602 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0593768 (min) -0.0545353 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.52484 (min) -3.52127 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: 12.9889 (min) 13.0328 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.43664e-05 (min) 6.44971e-05 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 314.592 (min) 317.778 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:24 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.455751, mean: 4.473482, max: 4.483967
17:37:24 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:24 DEBUG opendrift.models.physics_methods:1061: min: 11.499794, mean: 11.522648, max: 11.536147
17:37:24 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.499794, mean: 11.522648, max: 11.536147
17:37:24 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:24 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:24 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:24 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:24 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.269168 m/s - 0.270019 m/s)
17:37:24 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:24 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:24 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:24 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:24 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:24 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:24 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:24 INFO opendrift.models.basemodel:2032: 2025-01-12 20:00:00 - step 45 of 71 - 1000 active elements (0 deactivated)
17:37:24 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:24 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:24 DEBUG opendrift.models.basemodel:2051: 60.50159061840708 <- latitude -> 60.51250404518839
17:37:24 DEBUG opendrift.models.basemodel:2056: 4.692215959174207 <- longitude -> 4.6988568981532435
17:37:24 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:24 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 20:00:00 (before)
2025-01-12 21:00:00 (after)
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:25 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:25 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-12 20:00:00)
17:37:25 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 20:00:00) in space (linearNDFast)
17:37:25 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:25 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30779477623194 and -65.30115384172868 degrees.
17:37:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.30779477623194 and -65.30115384172868 degrees.
17:37:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:25 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0329776 (min) 0.106547 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.593508 (min) 0.669557 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.137107 (min) 0.138657 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.68949 (min) -3.65646 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 13.3315 (min) 13.4299 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.94848e-05 (min) 3.97599e-05 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 294.212 (min) 296.529 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:25 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.702021, mean: 4.737369, max: 4.771760
17:37:25 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:25 DEBUG opendrift.models.physics_methods:1061: min: 11.813316, mean: 11.857628, max: 11.900600
17:37:25 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.813316, mean: 11.857628, max: 11.900600
17:37:25 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:25 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:25 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:25 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:25 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.276506 m/s - 0.278549 m/s)
17:37:25 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:25 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:25 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:25 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:25 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:25 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:25 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:25 INFO opendrift.models.basemodel:2032: 2025-01-12 21:00:00 - step 46 of 71 - 1000 active elements (0 deactivated)
17:37:25 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:25 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:25 DEBUG opendrift.models.basemodel:2051: 60.53183845836314 <- latitude -> 60.54038594965458
17:37:25 DEBUG opendrift.models.basemodel:2056: 4.685213803725041 <- longitude -> 4.701039244835985
17:37:25 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:25 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 21:00:00 (before)
2025-01-12 22:00:00 (after)
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:26 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:26 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-12 21:00:00)
17:37:26 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 21:00:00) in space (linearNDFast)
17:37:26 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:26 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31479693242214 and -65.29897148538589 degrees.
17:37:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.31479693242214 and -65.29897148538589 degrees.
17:37:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:26 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.265168 (min) -0.22391 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.614575 (min) 0.646819 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.220053 (min) 0.223261 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.41488 (min) -4.28743 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: 14.7839 (min) 14.9114 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.36052e-07 (min) 1.17957e-06 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 271.513 (min) 287.475 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:26 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 5.856120, mean: 5.895848, max: 5.922023
17:37:26 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:26 DEBUG opendrift.models.physics_methods:1061: min: 13.183617, mean: 13.228252, max: 13.257592
17:37:26 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.183617, mean: 13.228252, max: 13.257592
17:37:26 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:26 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:26 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:26 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:26 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.308580 m/s - 0.310311 m/s)
17:37:26 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:26 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:26 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:26 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:26 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:26 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:26 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:26 INFO opendrift.models.basemodel:2032: 2025-01-12 22:00:00 - step 47 of 71 - 1000 active elements (0 deactivated)
17:37:26 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:26 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:26 DEBUG opendrift.models.basemodel:2051: 60.562289315800115 <- latitude -> 60.56998268415134
17:37:26 DEBUG opendrift.models.basemodel:2056: 4.6621817774144265 <- longitude -> 4.680552409016256
17:37:26 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:26 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 22:00:00 (before)
2025-01-12 23:00:00 (after)
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:27 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:27 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x24x2) for time before (2025-01-12 22:00:00)
17:37:27 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 22:00:00) in space (linearNDFast)
17:37:27 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:27 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33782894272488 and -65.31945831639092 degrees.
17:37:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.33782894272488 and -65.31945831639092 degrees.
17:37:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:27 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.440736 (min) -0.37417 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.811005 (min) 0.822173 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.133596 (min) 0.138673 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.94814 (min) -2.82614 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: 15.5926 (min) 15.6332 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.08977e-05 (min) -5.03071e-05 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 277.054 (min) 297.588 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:27 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.194805, mean: 6.203357, max: 6.211655
17:37:27 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:27 DEBUG opendrift.models.physics_methods:1061: min: 13.559492, mean: 13.568848, max: 13.577919
17:37:27 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.559492, mean: 13.568848, max: 13.577919
17:37:27 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:27 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:27 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:27 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:27 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.317378 m/s - 0.317809 m/s)
17:37:27 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:27 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:27 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:27 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:27 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:27 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:27 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:27 INFO opendrift.models.basemodel:2032: 2025-01-12 23:00:00 - step 48 of 71 - 1000 active elements (0 deactivated)
17:37:27 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:27 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:27 DEBUG opendrift.models.basemodel:2051: 60.59871237065026 <- latitude -> 60.606569218970236
17:37:27 DEBUG opendrift.models.basemodel:2056: 4.633855094756503 <- longitude -> 4.647727704845753
17:37:27 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:27 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-12 23:00:00 (before)
2025-01-13 00:00:00 (after)
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:28 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:28 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-12 23:00:00)
17:37:28 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-12 23:00:00) in space (linearNDFast)
17:37:28 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:28 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36615561218524 and -65.35228300242211 degrees.
17:37:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36615561218524 and -65.35228300242211 degrees.
17:37:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:28 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.303677 (min) -0.248865 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.0102 (min) 1.0424 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.107974 (min) -0.104342 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.27042 (min) -2.26192 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: 15.998 (min) 16.099 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.73232e-05 (min) -7.72554e-05 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 298.503 (min) 313.377 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:28 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.421869, mean: 6.449458, max: 6.502606
17:37:28 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:28 DEBUG opendrift.models.physics_methods:1061: min: 13.805759, mean: 13.835374, max: 13.892272
17:37:28 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.805759, mean: 13.835374, max: 13.892272
17:37:28 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:28 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:28 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:28 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:28 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.323142 m/s - 0.325167 m/s)
17:37:28 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:28 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:28 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:28 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:28 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:28 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:28 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:28 INFO opendrift.models.basemodel:2032: 2025-01-13 00:00:00 - step 49 of 71 - 1000 active elements (0 deactivated)
17:37:28 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:28 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:28 DEBUG opendrift.models.basemodel:2051: 60.64279313499647 <- latitude -> 60.64968471518813
17:37:28 DEBUG opendrift.models.basemodel:2056: 4.6145051758327815 <- longitude -> 4.624765494848029
17:37:28 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:28 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 00:00:00 (before)
2025-01-13 01:00:00 (after)
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:30 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:30 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 00:00:00)
17:37:30 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 00:00:00) in space (linearNDFast)
17:37:30 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:30 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38550552394587 and -65.37524519658372 degrees.
17:37:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38550552394587 and -65.37524519658372 degrees.
17:37:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:30 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0968727 (min) -0.0817094 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.00641 (min) 1.05338 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.397135 (min) -0.393726 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.10438 (min) -2.96592 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 16.9365 (min) 16.9928 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.72643e-06 (min) 1.93772e-06 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 304.303 (min) 315.434 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:30 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.273295, mean: 7.294432, max: 7.340438
17:37:30 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:30 DEBUG opendrift.models.physics_methods:1061: min: 14.692484, mean: 14.713809, max: 14.760143
17:37:30 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 14.692484, mean: 14.713809, max: 14.760143
17:37:30 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:30 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:30 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:30 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.343897 m/s - 0.345480 m/s)
17:37:30 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:30 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:30 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:30 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:30 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:30 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:30 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:30 INFO opendrift.models.basemodel:2032: 2025-01-13 01:00:00 - step 50 of 71 - 1000 active elements (0 deactivated)
17:37:30 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:30 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:30 DEBUG opendrift.models.basemodel:2051: 60.68780705476017 <- latitude -> 60.69314750225147
17:37:30 DEBUG opendrift.models.basemodel:2056: 4.605211228713572 <- longitude -> 4.614294000360602
17:37:30 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:30 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 01:00:00 (before)
2025-01-13 02:00:00 (after)
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:31 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:31 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 01:00:00)
17:37:31 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 01:00:00) in space (linearNDFast)
17:37:31 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:31 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39479945760225 and -65.38571668623231 degrees.
17:37:31 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.39479945760225 and -65.38571668623231 degrees.
17:37:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:31 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:31 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:31 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:31 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:31 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0989551 (min) -0.0852099 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.01143 (min) 1.03385 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.651666 (min) -0.646849 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.04677 (min) -3.74806 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.8254 (min) 18.064 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.11622e-05 (min) -6.09723e-05 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 272.506 (min) 283.541 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:31 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:31 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.219414, mean: 8.321775, max: 8.372796
17:37:31 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:31 DEBUG opendrift.models.physics_methods:1061: min: 15.618883, mean: 15.715813, max: 15.763941
17:37:31 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.618883, mean: 15.715813, max: 15.763941
17:37:31 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:31 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:31 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:31 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:31 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.365580 m/s - 0.368976 m/s)
17:37:31 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:31 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:31 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:31 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:31 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:31 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:31 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:31 INFO opendrift.models.basemodel:2032: 2025-01-13 02:00:00 - step 51 of 71 - 1000 active elements (0 deactivated)
17:37:31 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:31 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:31 DEBUG opendrift.models.basemodel:2051: 60.73271994991171 <- latitude -> 60.73749815055514
17:37:31 DEBUG opendrift.models.basemodel:2056: 4.593736803339148 <- longitude -> 4.603333647937261
17:37:31 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:31 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:31 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:31 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:31 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:31 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:31 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:31 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:31 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:31 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:31 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:31 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 02:00:00 (before)
2025-01-13 03:00:00 (after)
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:32 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:32 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 02:00:00)
17:37:32 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 02:00:00) in space (linearNDFast)
17:37:32 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:32 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40627386609707 and -65.39667702680653 degrees.
17:37:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40627386609707 and -65.39667702680653 degrees.
17:37:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:32 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.14941 (min) -0.139835 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.03762 (min) 1.05716 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.805039 (min) -0.799412 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.77013 (min) -2.55859 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.82 (min) 18.8687 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.87524e-05 (min) -2.81655e-05 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 236.906 (min) 250.091 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:32 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.901714, mean: 8.907675, max: 8.919294
17:37:32 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:32 DEBUG opendrift.models.physics_methods:1061: min: 16.254230, mean: 16.259671, max: 16.270273
17:37:32 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.254230, mean: 16.259671, max: 16.270273
17:37:32 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:32 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:32 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:32 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.380451 m/s - 0.380827 m/s)
17:37:32 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:32 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:32 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:32 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:32 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:32 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:32 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:32 INFO opendrift.models.basemodel:2032: 2025-01-13 03:00:00 - step 52 of 71 - 1000 active elements (0 deactivated)
17:37:32 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:32 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:32 DEBUG opendrift.models.basemodel:2051: 60.779003243015794 <- latitude -> 60.78329901955462
17:37:32 DEBUG opendrift.models.basemodel:2056: 4.580752601217427 <- longitude -> 4.590436698790125
17:37:32 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:32 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 03:00:00 (before)
2025-01-13 04:00:00 (after)
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:33 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:33 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x22x2) for time before (2025-01-13 03:00:00)
17:37:33 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 03:00:00) in space (linearNDFast)
17:37:33 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:33 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.41925806432593 and -65.40957395347968 degrees.
17:37:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.41925806432593 and -65.40957395347968 degrees.
17:37:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:33 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.131739 (min) -0.113563 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.06834 (min) 1.08924 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.836289 (min) -0.830223 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.35274 (min) -2.03291 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.1729 (min) 19.3923 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.00623e-05 (min) 1.0156e-05 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 223.178 (min) 237.097 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:33 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.179108, mean: 9.288103, max: 9.352777
17:37:33 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:33 DEBUG opendrift.models.physics_methods:1061: min: 16.505543, mean: 16.603226, max: 16.660954
17:37:33 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.505543, mean: 16.603226, max: 16.660954
17:37:33 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:33 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:33 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:33 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.386334 m/s - 0.389971 m/s)
17:37:33 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:33 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:33 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:33 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:33 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:33 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:33 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:33 INFO opendrift.models.basemodel:2032: 2025-01-13 04:00:00 - step 53 of 71 - 1000 active elements (0 deactivated)
17:37:33 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:33 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:33 DEBUG opendrift.models.basemodel:2051: 60.826583812854274 <- latitude -> 60.830345794781245
17:37:33 DEBUG opendrift.models.basemodel:2056: 4.569346470183393 <- longitude -> 4.5798108892139835
17:37:33 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:33 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 04:00:00 (before)
2025-01-13 05:00:00 (after)
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:34 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:34 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 04:00:00)
17:37:34 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 04:00:00) in space (linearNDFast)
17:37:34 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:34 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.43066417368505 and -65.42019975177351 degrees.
17:37:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.43066417368505 and -65.42019975177351 degrees.
17:37:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:34 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.16717 (min) -0.152046 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 1.02356 (min) 1.04048 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.743954 (min) -0.738293 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.158378 (min) -0.018767 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.5643 (min) 19.6341 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.71418e-05 (min) 3.75734e-05 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 202.348 (min) 218.425 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:34 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.416516, mean: 9.459757, max: 9.483252
17:37:34 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:34 DEBUG opendrift.models.physics_methods:1061: min: 16.717631, mean: 16.755966, max: 16.776764
17:37:34 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.717631, mean: 16.755966, max: 16.776764
17:37:34 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:34 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:34 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:34 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.391298 m/s - 0.392682 m/s)
17:37:34 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:34 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:34 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:34 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:34 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:34 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:34 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:34 INFO opendrift.models.basemodel:2032: 2025-01-13 05:00:00 - step 54 of 71 - 1000 active elements (0 deactivated)
17:37:34 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:34 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:34 DEBUG opendrift.models.basemodel:2051: 60.872836014712234 <- latitude -> 60.876101773797885
17:37:34 DEBUG opendrift.models.basemodel:2056: 4.55844013685757 <- longitude -> 4.569528944643601
17:37:34 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:34 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 05:00:00 (before)
2025-01-13 06:00:00 (after)
17:37:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:35 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:35 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 05:00:00)
17:37:35 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 05:00:00) in space (linearNDFast)
17:37:35 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:35 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44157048883676 and -65.43048168702565 degrees.
17:37:36 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44157048883676 and -65.43048168702565 degrees.
17:37:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:36 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:36 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:36 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:36 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:36 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.106719 (min) -0.0855766 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.915748 (min) 0.932907 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.552062 (min) -0.546914 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.680923 (min) 0.737103 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.2139 (min) 19.2285 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.63686e-05 (min) 6.66064e-05 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 194.084 (min) 201.776 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:36 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:36 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.093185, mean: 9.098926, max: 9.108802
17:37:36 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:36 DEBUG opendrift.models.physics_methods:1061: min: 16.428111, mean: 16.433294, max: 16.442212
17:37:36 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.428111, mean: 16.433294, max: 16.442212
17:37:36 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:36 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:36 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:36 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.384521 m/s - 0.384851 m/s)
17:37:36 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:36 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:36 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:36 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:36 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:36 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:36 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:36 INFO opendrift.models.basemodel:2032: 2025-01-13 06:00:00 - step 55 of 71 - 1000 active elements (0 deactivated)
17:37:36 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:36 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:36 DEBUG opendrift.models.basemodel:2051: 60.91539489197538 <- latitude -> 60.91811229625833
17:37:36 DEBUG opendrift.models.basemodel:2056: 4.552337219350506 <- longitude -> 4.5647631133847915
17:37:36 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:36 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:36 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:36 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:36 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:36 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 06:00:00 (before)
2025-01-13 07:00:00 (after)
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:37 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:37 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 06:00:00)
17:37:37 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 06:00:00) in space (linearNDFast)
17:37:37 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:37 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44767339646955 and -65.43524750148678 degrees.
17:37:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44767339646955 and -65.43524750148678 degrees.
17:37:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:37 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.140778 (min) -0.113187 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.735877 (min) 0.744694 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.270951 (min) -0.26686 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.06296 (min) 1.09459 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: 19.2349 (min) 19.2555 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.08716e-05 (min) 8.11544e-05 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 171.639 (min) 178.483 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:37 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 9.129863, mean: 9.138782, max: 9.150541
17:37:37 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:37 DEBUG opendrift.models.physics_methods:1061: min: 16.461208, mean: 16.469247, max: 16.479840
17:37:37 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.461208, mean: 16.469247, max: 16.479840
17:37:37 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:37 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:37 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:37 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:37 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.385296 m/s - 0.385732 m/s)
17:37:37 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:37 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:37 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:37 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:37 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:37 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:37 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:37 INFO opendrift.models.basemodel:2032: 2025-01-13 07:00:00 - step 56 of 71 - 1000 active elements (0 deactivated)
17:37:37 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:37 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:37 DEBUG opendrift.models.basemodel:2051: 60.951889029595804 <- latitude -> 60.95432864273909
17:37:37 DEBUG opendrift.models.basemodel:2056: 4.544442220739111 <- longitude -> 4.558671211720065
17:37:37 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:37 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 07:00:00 (before)
2025-01-13 08:00:00 (after)
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:38 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:38 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 07:00:00)
17:37:38 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 07:00:00) in space (linearNDFast)
17:37:38 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:38 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45556837986891 and -65.44133939881016 degrees.
17:37:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45556837986891 and -65.44133939881016 degrees.
17:37:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:38 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.107084 (min) -0.0786616 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.65561 (min) 0.689893 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.0497727 (min) 0.0543797 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.32789 (min) 1.35807 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.9419 (min) 19.0254 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.90708e-05 (min) 8.96678e-05 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 136.768 (min) 147.865 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:38 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.871545, mean: 8.896519, max: 8.947733
17:37:38 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:38 DEBUG opendrift.models.physics_methods:1061: min: 16.226662, mean: 16.249480, max: 16.296191
17:37:38 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 16.226662, mean: 16.249480, max: 16.296191
17:37:38 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:38 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:38 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:38 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:38 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.379806 m/s - 0.381434 m/s)
17:37:38 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:38 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:38 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:38 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:38 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:38 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:38 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:38 INFO opendrift.models.basemodel:2032: 2025-01-13 08:00:00 - step 57 of 71 - 1000 active elements (0 deactivated)
17:37:38 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:38 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:38 DEBUG opendrift.models.basemodel:2051: 60.98538003804933 <- latitude -> 60.98885699944495
17:37:38 DEBUG opendrift.models.basemodel:2056: 4.539154732157406 <- longitude -> 4.555208128702169
17:37:38 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:38 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 08:00:00 (before)
2025-01-13 09:00:00 (after)
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:39 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:39 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 08:00:00)
17:37:39 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 08:00:00) in space (linearNDFast)
17:37:39 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:39 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46085585349245 and -65.44480245801333 degrees.
17:37:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46085585349245 and -65.44480245801333 degrees.
17:37:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:39 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.053847 (min) -0.0469289 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.655499 (min) 0.671449 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.325603 (min) 0.329573 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.21399 (min) 1.23219 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.4909 (min) 18.5869 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 6.00884e-05 (min) 6.06871e-05 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 82.0022 (min) 92.583 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:39 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.448426, mean: 8.475944, max: 8.534888
17:37:39 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:39 DEBUG opendrift.models.physics_methods:1061: min: 15.834979, mean: 15.860737, max: 15.915802
17:37:39 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.834979, mean: 15.860737, max: 15.915802
17:37:39 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:39 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:39 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:39 DEBUG opendrift.models.physics_methods:915: Advecting 1000 of 1000 elements above 0.100m with wind-sheared ocean current (0.370638 m/s - 0.372530 m/s)
17:37:39 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:39 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:39 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:39 DEBUG opendrift.models.basemodel:2120: 1000 active elements (0 deactivated)
17:37:39 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:39 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:39 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:39 INFO opendrift.models.basemodel:2032: 2025-01-13 09:00:00 - step 58 of 71 - 1000 active elements (0 deactivated)
17:37:39 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:39 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:39 DEBUG opendrift.models.basemodel:2051: 61.018580291409535 <- latitude -> 61.02249771627346
17:37:39 DEBUG opendrift.models.basemodel:2056: 4.537672135626022 <- longitude -> 4.553316118932647
17:37:39 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:39 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 1000 elements
17:37:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 1000 elements
17:37:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 1000 elements
17:37:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 09:00:00 (before)
2025-01-13 10:00:00 (after)
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:40 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:40 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 09:00:00)
17:37:40 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 09:00:00) in space (linearNDFast)
17:37:40 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:40 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46233844295647 and -65.44669446166746 degrees.
17:37:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.46233844295647 and -65.44669446166746 degrees.
17:37:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:40 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0983017 (min) 0.118032 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.612657 (min) 0.625859 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.471167 (min) 0.473588 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.835904 (min) 0.922526 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: 18.2532 (min) 18.3052 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.46012e-05 (min) 2.58839e-05 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 49.6354 (min) 58.245 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 1 (max)
17:37:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:40 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 8.216937, mean: 8.230499, max: 8.260162
17:37:40 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:40 DEBUG opendrift.models.physics_methods:1061: min: 15.616530, mean: 15.629410, max: 15.657552
17:37:40 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.616530, mean: 15.629410, max: 15.657552
17:37:40 DEBUG opendrift.models.basemodel:659: 5 elements hit land, moving them to the coastline.
17:37:40 DEBUG opendrift.models.basemodel:1679: Added status stranded
17:37:40 DEBUG opendrift.models.basemodel:1690: 5 elements scheduled for deactivation (stranded)
17:37:40 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:37:40 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:40 DEBUG opendrift.models.basemodel:1710: Removed 5 elements.
17:37:40 DEBUG opendrift.models.basemodel:1713: Removed 5 values from environment.
17:37:40 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:37:40 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:37:40 DEBUG opendrift.models.basemodel:1722: Removed 5 values from environment_profiles.
17:37:40 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:40 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.365525 m/s - 0.366357 m/s)
17:37:40 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:40 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:40 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:40 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:40 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:40 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:40 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:40 INFO opendrift.models.basemodel:2032: 2025-01-13 10:00:00 - step 59 of 71 - 995 active elements (5 deactivated)
17:37:40 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:40 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:40 DEBUG opendrift.models.basemodel:2051: 61.05079298224237 <- latitude -> 61.05409060163903
17:37:40 DEBUG opendrift.models.basemodel:2056: 4.546353991269659 <- longitude -> 4.5603398843709
17:37:40 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:40 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 10:00:00 (before)
2025-01-13 11:00:00 (after)
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:41 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:41 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 10:00:00)
17:37:41 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 10:00:00) in space (linearNDFast)
17:37:41 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:41 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45365658778225 and -65.43967069069781 degrees.
17:37:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.45365658778225 and -65.43967069069781 degrees.
17:37:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:41 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0752263 (min) 0.215476 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.34559 (min) 0.412746 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.429169 (min) 0.431357 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.33089 (min) 1.46276 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.5447 (min) 17.5871 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.085e-05 (min) -3.99261e-05 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 72.1476 (min) 75.7989 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:41 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.623437, mean: 7.628584, max: 7.652464
17:37:41 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:41 DEBUG opendrift.models.physics_methods:1061: min: 15.041979, mean: 15.047056, max: 15.070588
17:37:41 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.041979, mean: 15.047056, max: 15.070588
17:37:41 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:41 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:41 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:41 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.352077 m/s - 0.352747 m/s)
17:37:41 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:41 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:41 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:41 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:41 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:41 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:41 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:41 INFO opendrift.models.basemodel:2032: 2025-01-13 11:00:00 - step 60 of 71 - 995 active elements (5 deactivated)
17:37:41 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:41 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:41 DEBUG opendrift.models.basemodel:2051: 61.07549069042528 <- latitude -> 61.07660249787362
17:37:41 DEBUG opendrift.models.basemodel:2056: 4.553574967894127 <- longitude -> 4.576304525945804
17:37:41 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:41 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 11:00:00 (before)
2025-01-13 12:00:00 (after)
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:42 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:42 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 11:00:00)
17:37:42 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 11:00:00) in space (linearNDFast)
17:37:42 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:42 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4464355924388 and -65.42370603295416 degrees.
17:37:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.4464355924388 and -65.42370603295416 degrees.
17:37:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:42 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.104923 (min) 0.04208 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.218205 (min) 0.381126 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.209107 (min) 0.213023 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.73375 (min) 1.80823 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: 17.6159 (min) 17.7495 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.49863e-05 (min) -8.46565e-05 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 124.926 (min) 136.249 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:42 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 7.714276, mean: 7.764357, max: 7.824037
17:37:42 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:42 DEBUG opendrift.models.physics_methods:1061: min: 15.131331, mean: 15.180345, max: 15.238599
17:37:42 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 15.131331, mean: 15.180345, max: 15.238599
17:37:42 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:42 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:42 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:42 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.354169 m/s - 0.356679 m/s)
17:37:42 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:42 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:42 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:42 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:42 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:42 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:42 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:42 INFO opendrift.models.basemodel:2032: 2025-01-13 12:00:00 - step 61 of 71 - 995 active elements (5 deactivated)
17:37:42 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:42 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:42 DEBUG opendrift.models.basemodel:2051: 61.094008767235024 <- latitude -> 61.10029786874647
17:37:42 DEBUG opendrift.models.basemodel:2056: 4.558721608327842 <- longitude -> 4.571619323419489
17:37:42 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:42 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 12:00:00 (before)
2025-01-13 13:00:00 (after)
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:43 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:43 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 12:00:00)
17:37:43 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 12:00:00) in space (linearNDFast)
17:37:43 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:43 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44128894711767 and -65.42839123238758 degrees.
17:37:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.44128894711767 and -65.42839123238758 degrees.
17:37:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:43 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.204801 (min) 0.29567 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.135794 (min) 0.316379 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.122359 (min) -0.119693 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.75638 (min) 4.83749 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: 14.9343 (min) 14.982 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.61159e-05 (min) -9.57015e-05 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 149.228 (min) 150.179 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:43 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 6.061986, mean: 6.070524, max: 6.078305
17:37:43 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:43 DEBUG opendrift.models.physics_methods:1061: min: 13.413344, mean: 13.422785, max: 13.431386
17:37:43 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 13.413344, mean: 13.422785, max: 13.431386
17:37:43 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:43 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:43 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:43 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.313957 m/s - 0.314379 m/s)
17:37:43 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:43 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:43 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:43 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:43 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:43 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:43 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:43 INFO opendrift.models.basemodel:2032: 2025-01-13 13:00:00 - step 62 of 71 - 995 active elements (5 deactivated)
17:37:43 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:43 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:43 DEBUG opendrift.models.basemodel:2051: 61.11364055777683 <- latitude -> 61.11438018090328
17:37:43 DEBUG opendrift.models.basemodel:2056: 4.578927820469323 <- longitude -> 4.593554267868056
17:37:43 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:43 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 13:00:00 (before)
2025-01-13 14:00:00 (after)
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:44 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:44 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 13:00:00)
17:37:44 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 13:00:00) in space (linearNDFast)
17:37:44 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:44 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42108273495978 and -65.40645626868675 degrees.
17:37:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.42108273495978 and -65.40645626868675 degrees.
17:37:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:44 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.118059 (min) 0.153643 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.196611 (min) 0.319815 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.461518 (min) -0.458729 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.64482 (min) 4.90533 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: 13.5779 (min) 13.6543 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.71299e-05 (min) -8.69454e-05 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 140.058 (min) 146.769 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:44 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 5.116197, mean: 5.121492, max: 5.128545
17:37:44 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:44 DEBUG opendrift.models.physics_methods:1061: min: 12.322624, mean: 12.328998, max: 12.337486
17:37:44 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 12.322624, mean: 12.328998, max: 12.337486
17:37:44 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:44 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:44 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:44 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.288427 m/s - 0.288775 m/s)
17:37:44 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:44 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:44 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:44 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:44 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:44 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:44 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:44 INFO opendrift.models.basemodel:2032: 2025-01-13 14:00:00 - step 63 of 71 - 995 active elements (5 deactivated)
17:37:44 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:44 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:44 DEBUG opendrift.models.basemodel:2051: 61.129027978144805 <- latitude -> 61.133494405005976
17:37:44 DEBUG opendrift.models.basemodel:2056: 4.5948593474010195 <- longitude -> 4.60776051202794
17:37:44 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:44 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 14:00:00 (before)
2025-01-13 15:00:00 (after)
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:45 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:45 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 14:00:00)
17:37:45 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 14:00:00) in space (linearNDFast)
17:37:45 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:45 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40515119595682 and -65.39225003860156 degrees.
17:37:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.40515119595682 and -65.39225003860156 degrees.
17:37:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:45 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:45 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0808814 (min) 0.128526 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.297175 (min) 0.313455 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.714277 (min) -0.711477 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.07432 (min) 5.29554 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: y_wind: 12.1545 (min) 12.2506 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.49653e-05 (min) -5.48913e-05 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 138.01 (min) 145.848 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:45 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.324073, mean: 4.324843, max: 4.325512
17:37:45 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:45 DEBUG opendrift.models.physics_methods:1061: min: 11.328596, mean: 11.329605, max: 11.330480
17:37:45 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.328596, mean: 11.329605, max: 11.330480
17:37:45 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:45 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:45 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:45 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:45 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.265161 m/s - 0.265205 m/s)
17:37:45 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:45 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:45 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:45 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:45 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:45 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:45 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:45 INFO opendrift.models.basemodel:2032: 2025-01-13 15:00:00 - step 64 of 71 - 995 active elements (5 deactivated)
17:37:45 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:45 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:45 DEBUG opendrift.models.basemodel:2051: 61.14685858984823 <- latitude -> 61.15100956962716
17:37:45 DEBUG opendrift.models.basemodel:2056: 4.610514988163163 <- longitude -> 4.619958860451343
17:37:45 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:45 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:45 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:45 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 15:00:00 (before)
2025-01-13 16:00:00 (after)
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:46 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:46 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 15:00:00)
17:37:46 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 15:00:00) in space (linearNDFast)
17:37:46 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:46 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38949554319242 and -65.38005166990533 degrees.
17:37:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.38949554319242 and -65.38005166990533 degrees.
17:37:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:46 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.105286 (min) 0.123069 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.229568 (min) 0.253406 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.844076 (min) -0.841915 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.01343 (min) 5.1299 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1744 (min) 11.2275 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.86117e-05 (min) -1.83844e-05 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 147.94 (min) 155.442 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:46 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.717685, mean: 3.719486, max: 3.720359
17:37:46 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:46 DEBUG opendrift.models.physics_methods:1061: min: 10.504271, mean: 10.506814, max: 10.508046
17:37:46 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.504271, mean: 10.506814, max: 10.508046
17:37:46 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:46 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:46 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:46 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.245866 m/s - 0.245955 m/s)
17:37:46 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:46 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:46 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:46 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:46 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:46 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:46 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:46 INFO opendrift.models.basemodel:2032: 2025-01-13 16:00:00 - step 65 of 71 - 995 active elements (5 deactivated)
17:37:46 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:46 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:46 DEBUG opendrift.models.basemodel:2051: 61.16221698110228 <- latitude -> 61.165732734867035
17:37:46 DEBUG opendrift.models.basemodel:2056: 4.625513190148678 <- longitude -> 4.633745539730111
17:37:46 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:46 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 16:00:00 (before)
2025-01-13 17:00:00 (after)
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:47 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:47 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 16:00:00)
17:37:47 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 16:00:00) in space (linearNDFast)
17:37:47 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:47 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37449734454444 and -65.36626498444872 degrees.
17:37:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.37449734454444 and -65.36626498444872 degrees.
17:37:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:47 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0712329 (min) 0.0906685 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.218823 (min) 0.229953 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.83223 (min) -0.830189 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.66275 (min) 5.747 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: 11.1687 (min) 11.1852 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.53316e-05 (min) 2.56699e-05 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 151.181 (min) 159.767 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:47 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.866520, mean: 3.871316, max: 3.881100
17:37:47 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:47 DEBUG opendrift.models.physics_methods:1061: min: 10.712471, mean: 10.719114, max: 10.732651
17:37:47 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.712471, mean: 10.719114, max: 10.732651
17:37:47 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:47 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:47 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:47 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.250739 m/s - 0.251212 m/s)
17:37:47 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:47 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:47 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:47 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:47 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:47 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:47 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:47 INFO opendrift.models.basemodel:2032: 2025-01-13 17:00:00 - step 66 of 71 - 995 active elements (5 deactivated)
17:37:47 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:47 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:47 DEBUG opendrift.models.basemodel:2051: 61.1766915681053 <- latitude -> 61.18029484660008
17:37:47 DEBUG opendrift.models.basemodel:2056: 4.639215866920429 <- longitude -> 4.64610150885358
17:37:47 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:47 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:47 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 17:00:00 (before)
2025-01-13 18:00:00 (after)
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:48 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:48 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 23x23x2) for time before (2025-01-13 17:00:00)
17:37:48 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 17:00:00) in space (linearNDFast)
17:37:48 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:48 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36079464926206 and -65.35390901331881 degrees.
17:37:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.36079464926206 and -65.35390901331881 degrees.
17:37:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:48 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -5.65159e-06 (min) 0.0775311 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.258764 (min) 0.384047 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.684984 (min) -0.682977 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.13602 (min) 7.1979 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.7673 (min) 10.7688 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 5.47726e-05 (min) 5.54861e-05 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 165.022 (min) 168.884 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:48 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 4.105314, mean: 4.112370, max: 4.126541
17:37:48 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:48 DEBUG opendrift.models.physics_methods:1061: min: 11.038314, mean: 11.047793, max: 11.066815
17:37:48 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 11.038314, mean: 11.047793, max: 11.066815
17:37:48 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:48 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:48 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:48 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.258366 m/s - 0.259033 m/s)
17:37:48 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:48 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:48 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:48 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:48 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:48 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:48 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:48 INFO opendrift.models.basemodel:2032: 2025-01-13 18:00:00 - step 67 of 71 - 995 active elements (5 deactivated)
17:37:48 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:48 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:48 DEBUG opendrift.models.basemodel:2051: 61.192008701888184 <- latitude -> 61.199659527382124
17:37:48 DEBUG opendrift.models.basemodel:2056: 4.653790224734166 <- longitude -> 4.656089059363346
17:37:48 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:48 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 18:00:00 (before)
2025-01-13 19:00:00 (after)
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:49 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:49 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x23x2) for time before (2025-01-13 18:00:00)
17:37:49 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 18:00:00) in space (linearNDFast)
17:37:49 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:49 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34622028664398 and -65.34392145482107 degrees.
17:37:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34622028664398 and -65.34392145482107 degrees.
17:37:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:49 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.101424 (min) -0.0818482 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.545717 (min) 0.558793 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.409503 (min) -0.40726 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.53951 (min) 7.55674 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: 10.1274 (min) 10.1419 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.63702e-05 (min) 8.66948e-05 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 191.752 (min) 219.573 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:49 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.923539, mean: 3.930242, max: 3.934696
17:37:49 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:49 DEBUG opendrift.models.physics_methods:1061: min: 10.791171, mean: 10.800384, max: 10.806503
17:37:49 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.791171, mean: 10.800384, max: 10.806503
17:37:49 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:49 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:49 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:49 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.252581 m/s - 0.252940 m/s)
17:37:49 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:49 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:49 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:49 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:49 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:49 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:49 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:49 INFO opendrift.models.basemodel:2032: 2025-01-13 19:00:00 - step 68 of 71 - 995 active elements (5 deactivated)
17:37:49 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:49 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:49 DEBUG opendrift.models.basemodel:2051: 61.21643325784643 <- latitude -> 61.22385227098118
17:37:49 DEBUG opendrift.models.basemodel:2056: 4.658427108866105 <- longitude -> 4.65977422922173
17:37:49 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:49 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 19:00:00 (before)
2025-01-13 20:00:00 (after)
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:50 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:50 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-13 19:00:00)
17:37:50 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 19:00:00) in space (linearNDFast)
17:37:50 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:50 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34158340277892 and -65.3402362706677 degrees.
17:37:50 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.34158340277892 and -65.3402362706677 degrees.
17:37:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:50 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:50 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:50 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:50 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:50 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0562139 (min) -0.0298352 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.531358 (min) 0.568422 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: -0.0690207 (min) -0.067707 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: x_wind: 6.68632 (min) 6.71038 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.15822 (min) 9.17242 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.54842e-05 (min) 9.59871e-05 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 263.823 (min) 273.186 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:50 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:50 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.163696, mean: 3.172084, max: 3.177386
17:37:50 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:50 DEBUG opendrift.models.physics_methods:1061: min: 9.690070, mean: 9.702906, max: 9.711013
17:37:50 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.690070, mean: 9.702906, max: 9.711013
17:37:50 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:50 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:50 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:50 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.226809 m/s - 0.227299 m/s)
17:37:50 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:50 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:50 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:50 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:50 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:50 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:50 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:50 INFO opendrift.models.basemodel:2032: 2025-01-13 20:00:00 - step 69 of 71 - 995 active elements (5 deactivated)
17:37:50 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:50 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:50 DEBUG opendrift.models.basemodel:2051: 61.23951828052264 <- latitude -> 61.24814217727128
17:37:50 DEBUG opendrift.models.basemodel:2056: 4.663744082206798 <- longitude -> 4.666483356441154
17:37:50 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:50 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:50 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:50 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:50 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:50 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:50 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:50 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:50 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:50 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:50 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 20:00:00 (before)
2025-01-13 21:00:00 (after)
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:51 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:51 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-13 20:00:00)
17:37:51 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 20:00:00) in space (linearNDFast)
17:37:51 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:51 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3362664154098 and -65.33352714289013 degrees.
17:37:51 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.3362664154098 and -65.33352714289013 degrees.
17:37:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:51 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:51 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:51 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:51 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:51 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.0465059 (min) 0.131652 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.587119 (min) 0.618893 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.25832 (min) 0.259568 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.26158 (min) 7.28146 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: y_wind: 9.53585 (min) 9.55067 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 8.09529e-05 (min) 8.12121e-05 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 231.937 (min) 258.478 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:51 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:51 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.534735, mean: 3.543152, max: 3.547611
17:37:51 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:51 DEBUG opendrift.models.physics_methods:1061: min: 10.242549, mean: 10.254734, max: 10.261185
17:37:51 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.242549, mean: 10.254734, max: 10.261185
17:37:51 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:51 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:51 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:51 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:51 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.239740 m/s - 0.240176 m/s)
17:37:51 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:51 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:51 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:51 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:51 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:51 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:51 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:51 INFO opendrift.models.basemodel:2032: 2025-01-13 21:00:00 - step 70 of 71 - 995 active elements (5 deactivated)
17:37:51 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:51 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:51 DEBUG opendrift.models.basemodel:2051: 61.26464766312757 <- latitude -> 61.27430660074056
17:37:51 DEBUG opendrift.models.basemodel:2056: 4.677042178899647 <- longitude -> 4.685088864852748
17:37:51 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:51 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:51 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:51 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:51 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:51 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:51 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:51 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:51 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:51 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:51 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 21:00:00 (before)
2025-01-13 22:00:00 (after)
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:52 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:52 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 24x22x2) for time before (2025-01-13 21:00:00)
17:37:52 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 21:00:00) in space (linearNDFast)
17:37:52 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:52 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32296830878197 and -65.31492161067312 degrees.
17:37:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.32296830878197 and -65.31492161067312 degrees.
17:37:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:52 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.278389 (min) 0.337377 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.448456 (min) 0.536179 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.456594 (min) 0.457127 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: 9.2821 (min) 9.50116 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: 8.10679 (min) 8.34221 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 3.30363e-05 (min) 3.38542e-05 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 113.819 (min) 156.299 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
17:37:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:52 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.736187, mean: 3.810842, max: 3.932667
17:37:52 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:52 DEBUG opendrift.models.physics_methods:1061: min: 10.530377, mean: 10.634678, max: 10.803718
17:37:52 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 10.530377, mean: 10.634678, max: 10.803718
17:37:52 DEBUG opendrift.models.basemodel:656: No elements hit coastline.
17:37:52 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:52 DEBUG opendrift.models.basemodel:1706: No elements to deactivate
17:37:52 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:52 DEBUG opendrift.models.physics_methods:915: Advecting 995 of 995 elements above 0.100m with wind-sheared ocean current (0.246477 m/s - 0.252875 m/s)
17:37:52 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:52 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:52 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:52 DEBUG opendrift.models.basemodel:2120: 995 active elements (5 deactivated)
17:37:52 DEBUG opendrift.models.basemodel:887: to be seeded: 0, already seeded 1000
17:37:52 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:52 DEBUG opendrift.models.basemodel:2031: ======================================================================
17:37:52 INFO opendrift.models.basemodel:2032: 2025-01-13 22:00:00 - step 71 of 71 - 995 active elements (5 deactivated)
17:37:52 DEBUG opendrift.models.basemodel:2038: 0 elements scheduled.
17:37:52 DEBUG opendrift.models.basemodel:2040: ======================================================================
17:37:52 DEBUG opendrift.models.basemodel:2051: 61.287357787351915 <- latitude -> 61.29403001734691
17:37:52 DEBUG opendrift.models.basemodel:2056: 4.708705954519134 <- longitude -> 4.720208914566266
17:37:52 DEBUG opendrift.models.basemodel:2059: z = 0.0
17:37:52 DEBUG opendrift.models.basemodel:2062: ---------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 995 elements
17:37:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity', 'sea_surface_height']
17:37:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
17:37:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 995 elements
17:37:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 995 elements
17:37:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2025-01-13 22:00:00 (before)
2025-01-13 23:00:00 (after)
17:37:53 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
17:37:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
17:37:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
17:37:53 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
17:37:53 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
17:37:53 DEBUG opendrift.readers.interpolation.structured:62: Filled NaN-values toward seafloor for :['upward_sea_water_velocity', 'x_sea_water_velocity', 'y_sea_water_velocity']
17:37:53 DEBUG opendrift.readers.basereader.structured:292: Fetched env-block (size 25x23x2) for time before (2025-01-13 22:00:00)
17:37:53 DEBUG opendrift.readers.basereader.structured:336: Interpolating before (2025-01-13 22:00:00) in space (linearNDFast)
17:37:53 DEBUG opendrift.readers.interpolation.structured:89: Initialising interpolator.
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 921 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 995 elements, expanding data 1
17:37:53 DEBUG opendrift:136: Linear2DInterpolator informational: NaN values for 480 elements, expanding data 2
17:37:53 DEBUG opendrift.readers.basereader.structured:390: No time interpolation needed - right on time.
17:37:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29130451845404 and -65.27980155655742 degrees.
17:37:53 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -65.29130451845404 and -65.27980155655742 degrees.
17:37:53 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:53 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:53 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:53 DEBUG opendrift.models.basemodel.environment:798: Creating empty dictionary for profiles not profided by any reader: ['ocean_vertical_diffusivity']
17:37:53 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0 for ocean_vertical_diffusivity for all profiles
17:37:53 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:53 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: 0.171202 (min) 0.329056 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.36504 (min) 0.4947 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0.472662 (min) 0.475 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: x_wind: 7.86679 (min) 7.94436 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: y_wind: 7.80532 (min) 8.0425 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.62901e-05 (min) -2.09798e-05 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_significant_height: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_x_stress: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: surface_downward_y_stress: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_kinetic_energy: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: turbulent_generic_length_scale: 0 (min) 0 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 50 (min) 50 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 5 (min) 11.8083 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 1 (max)
17:37:53 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:53 DEBUG opendrift.models.physics_methods:993: Calculating Hs from wind, min: 3.021112, mean: 3.080790, max: 3.143526
17:37:53 DEBUG opendrift.models.physics_methods:1051: Calculating wave period Tm02 from wind
17:37:53 DEBUG opendrift.models.physics_methods:1061: min: 9.469193, mean: 9.562074, max: 9.659133
17:37:53 DEBUG opendrift.models.physics_methods:1001: Calculating wave period from wind, min: 9.469193, mean: 9.562074, max: 9.659133
17:37:53 DEBUG opendrift.models.basemodel:659: 958 elements hit land, moving them to the coastline.
17:37:53 DEBUG opendrift.models.basemodel:1690: 958 elements scheduled for deactivation (stranded)
17:37:53 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:37:54 DEBUG opendrift.models.basemodel:746: No elements hit seafloor.
17:37:54 DEBUG opendrift.models.basemodel:1710: Removed 958 elements.
17:37:54 DEBUG opendrift.models.basemodel:1713: Removed 958 values from environment.
17:37:54 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:37:54 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:37:54 DEBUG opendrift.models.basemodel:1722: Removed 958 values from environment_profiles.
17:37:54 DEBUG opendrift.models.basemodel:2105: Calling OceanDrift.update()
17:37:54 DEBUG opendrift.models.physics_methods:915: Advecting 37 of 37 elements above 0.100m with wind-sheared ocean current (0.225990 m/s - 0.226085 m/s)
17:37:54 DEBUG opendrift.models.physics_methods:933: No Stokes drift velocity available
17:37:54 DEBUG opendrift.models.oceandrift:401: No vertical advection for elements at surface
17:37:54 DEBUG opendrift.models.basemodel:1657: Horizontal diffusivity is 0, no random walk.
17:37:54 DEBUG opendrift.models.basemodel:2120: 37 active elements (963 deactivated)
17:37:54 DEBUG opendrift.models.basemodel:2149: Cleaning up
17:37:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
17:37:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
17:37:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
17:37:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
17:37:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
17:37:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 37 elements
17:37:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 37 elements
17:37:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
17:37:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
17:37:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
17:37:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
17:37:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
17:37:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
17:37:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
17:37:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 1 (min) 1 (max)
17:37:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
17:37:54 DEBUG opendrift.models.basemodel:659: 37 elements hit land, moving them to the coastline.
17:37:54 DEBUG opendrift.models.basemodel:1690: 37 elements scheduled for deactivation (stranded)
17:37:54 DEBUG opendrift.models.basemodel:1692: (z: 0.000000 to 0.000000)
17:37:54 DEBUG opendrift.models.basemodel:1710: Removed 37 elements.
17:37:54 DEBUG opendrift.models.basemodel:1713: Removed 37 values from environment.
17:37:54 DEBUG opendrift.models.basemodel:1718: remove items from profile for z
17:37:54 DEBUG opendrift.models.basemodel:1718: remove items from profile for ocean_vertical_diffusivity
17:37:54 DEBUG opendrift.models.basemodel:1722: Removed 37 values from environment_profiles.
17:37:54 DEBUG opendrift.models.basemodel:96: Changed mode from Mode.Run to Mode.Result
17:37:54 DEBUG opendrift.models.basemodel:2361: Setting up map: corners=None, fast=False, lscale=None
17:37:54 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes with Cartopy shapereader...
17:38:07 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes with Cartopy shapereader...
17:38:07 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes with Cartopy shapereader...
17:38:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/opt/conda/envs/opendrift/lib/python3.11/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'cmap' will be ignored
result = super().scatter(*args, **kwargs)
17:38:07 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:07 DEBUG opendrift.models.basemodel:3041: Saving animation..
17:38:07 INFO opendrift.models.basemodel:4606: Saving animation to /root/project/docs/source/gallery/animations/example_manual_aggregate_0.gif...
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:08 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
/root/project/opendrift/models/basemodel/__init__.py:4642: UserWarning: Tight layout not applied. The left and right margins cannot be made large enough to accommodate all Axes decorations.
writer.grab_frame()
17:38:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (4.085640525817871, 5.118923091888429, 59.76176528930663, 61.48791427612306)..
17:38:37 DEBUG opendrift.models.basemodel:4644: MPLBACKEND = agg
17:38:37 DEBUG opendrift.models.basemodel:4645: DISPLAY = None
17:38:37 DEBUG opendrift.models.basemodel:4646: Time to save animation: 0:00:29.834487
17:38:37 INFO opendrift.models.basemodel:3034: Time to make animation: 0:00:43.676995
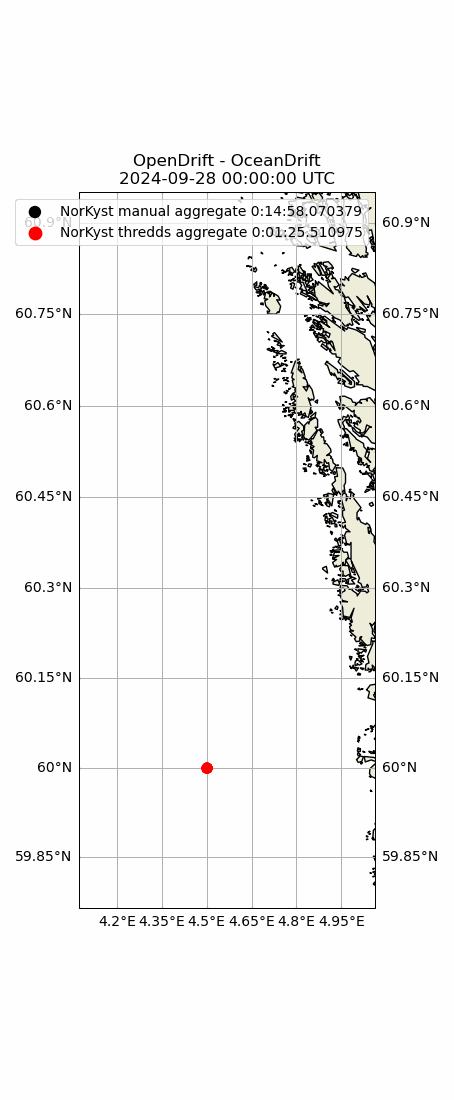
Total running time of the script: (19 minutes 33.246 seconds)