Note
Go to the end to download the full example code.
ROMS native reader
import numpy as np
from opendrift import test_data_folder
from opendrift.readers import reader_ROMS_native
from opendrift.models.oceandrift import OceanDrift
o = OceanDrift(loglevel=20) # Set loglevel to 0 for debug information
09:59:03 INFO opendrift:509: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-59-g60ee835)
Creating and adding reader for Nordic 4km current dataset
nordic_native = reader_ROMS_native.Reader(test_data_folder +
'2Feb2016_Nordic_sigma_3d/Nordic-4km_SLEVELS_avg_00_subset2Feb2016.nc')
o.add_reader(nordic_native)
09:59:03 INFO opendrift.readers:61: Opening file with xr.open_dataset
09:59:04 INFO opendrift.readers.reader_ROMS_native:240: Read GLS parameters from file.
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for latitude, selecting lat_psi, and discarding lat_rho
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for latitude, selecting lat_psi, and discarding lat_u
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for latitude, selecting lat_psi, and discarding lat_v
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for longitude, selecting lon_psi, and discarding lon_rho
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for longitude, selecting lon_psi, and discarding lon_u
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for longitude, selecting lon_psi, and discarding lon_v
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for land_binary_mask, selecting mask_psi, and discarding mask_rho
09:59:04 WARNING opendrift.readers.reader_ROMS_native:294: Duplicate variables for ocean_s_coordinate_g2, selecting s_rho, and discarding s_w
09:59:04 INFO opendrift.readers.reader_ROMS_native:301: The following variables without standard_name are discarded: ['AICEnudass', 'Akk_bak', 'Akp_bak', 'Akt_bak', 'Akv_bak', 'Charnok_alpha', 'CrgBan_cw', 'Cs_r', 'Cs_w', 'FSobc_in', 'FSobc_out', 'Falpha', 'Fbeta', 'Fgamma', 'LtracerSrc', 'M2nudg', 'M2obc_in', 'M2obc_out', 'M3nudg', 'M3obc_in', 'M3obc_out', 'Tcline', 'Tnudg', 'Tobc_in', 'Tobc_out', 'Vstretching', 'Vtransform', 'Znudg', 'Zob', 'Zos', 'Zos_hsig_alpha', 'angle', 'dstart', 'dt', 'dtfast', 'el', 'f', 'gamma2', 'gls_Kmin', 'gls_Pmin', 'gls_c1', 'gls_c2', 'gls_c3m', 'gls_c3p', 'gls_cmu0', 'gls_m', 'gls_n', 'gls_p', 'gls_sigk', 'gls_sigp', 'hc', 'mask_u', 'mask_v', 'nAVG', 'nHIS', 'nRST', 'nSTA', 'ndefAVG', 'ndefHIS', 'ndtfast', 'ntimes', 'ntsAVG', 'ocean_time', 'pm', 'pn', 'rdrg', 'rdrg2', 'rho0', 'spherical', 'swrad', 'sz_alpha', 'theta_b', 'theta_s', 'ubar', 'vbar', 'xl', 'zeta_detided']
09:59:04 WARNING opendrift.readers.basereader.structured:50: No proj string or projection could be derived, using 'fakeproj'. This assumes that the variables are structured and gridded approximately equidistantly on the surface (i.e. in meters). This must be guaranteed by the user. You can get rid of this warning by supplying a valid projection to the reader.
09:59:04 INFO opendrift.readers.basereader.structured:90: Making interpolator for lon,lat to x,y conversion...
Seed elements at defined positions, depth and time
o.seed_elements(lon=12.0, lat=68.3, radius=0, number=10,
z=np.linspace(0, -150, 10), time=nordic_native.start_time)
09:59:04 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
09:59:09 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
09:59:09 INFO opendrift.models.basemodel.environment:232: x_wind: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: y_wind: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: upward_sea_water_velocity: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_to_direction: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_significant_height: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_to_direction: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_mean_period: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_significant_height: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: surface_downward_x_stress: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: surface_downward_y_stress: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: turbulent_kinetic_energy: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: turbulent_generic_length_scale: 0.000000
09:59:09 INFO opendrift.models.basemodel.environment:232: ocean_mixed_layer_thickness: 50.000000
Running model
o.run(time_step=3600)
09:59:09 INFO opendrift:1866: Duration, steps or end time not specified, running until end of first reader: 2016-02-04 12:00:00
09:59:09 INFO opendrift:1866: Duration, steps or end time not specified, running until end of first reader: 2016-02-04 12:00:00
09:59:09 INFO opendrift:885: Using existing reader for land_binary_mask
09:59:09 INFO opendrift:914: All points are in ocean
09:59:09 INFO opendrift:2035: Storing previous position of elements for coastline interaction
09:59:09 INFO opendrift:2096: 2016-02-02 12:00:00 - step 1 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift.readers.reader_ROMS_native:351: Using mask_rho for mask_rho
09:59:09 INFO opendrift.readers.reader_ROMS_native:402: Using zeta for sea surface height
09:59:09 INFO opendrift.readers.reader_ROMS_native:372: Using mask_u for mask_u
09:59:09 INFO opendrift.readers.reader_ROMS_native:623: Time: 0:00:00.068339
09:59:09 INFO opendrift.readers.reader_ROMS_native:393: Using mask_v for mask_v
09:59:09 INFO opendrift.readers.reader_ROMS_native:416: Using angle from Dataset.
09:59:09 INFO opendrift:2096: 2016-02-02 13:00:00 - step 2 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 14:00:00 - step 3 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 15:00:00 - step 4 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 16:00:00 - step 5 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 17:00:00 - step 6 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 18:00:00 - step 7 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 19:00:00 - step 8 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 20:00:00 - step 9 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 21:00:00 - step 10 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 22:00:00 - step 11 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-02 23:00:00 - step 12 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 00:00:00 - step 13 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 01:00:00 - step 14 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 02:00:00 - step 15 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 03:00:00 - step 16 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 04:00:00 - step 17 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 05:00:00 - step 18 of 48 - 10 active elements (0 deactivated)
09:59:09 INFO opendrift:2096: 2016-02-03 06:00:00 - step 19 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 07:00:00 - step 20 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 08:00:00 - step 21 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 09:00:00 - step 22 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 10:00:00 - step 23 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 11:00:00 - step 24 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 12:00:00 - step 25 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 13:00:00 - step 26 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 14:00:00 - step 27 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 15:00:00 - step 28 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 16:00:00 - step 29 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 17:00:00 - step 30 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 18:00:00 - step 31 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 19:00:00 - step 32 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 20:00:00 - step 33 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 21:00:00 - step 34 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 22:00:00 - step 35 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-03 23:00:00 - step 36 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 00:00:00 - step 37 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 01:00:00 - step 38 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 02:00:00 - step 39 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 03:00:00 - step 40 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 04:00:00 - step 41 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 05:00:00 - step 42 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 06:00:00 - step 43 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 07:00:00 - step 44 of 48 - 10 active elements (0 deactivated)
09:59:10 INFO opendrift:2096: 2016-02-04 08:00:00 - step 45 of 48 - 10 active elements (0 deactivated)
09:59:11 INFO opendrift:2096: 2016-02-04 09:00:00 - step 46 of 48 - 10 active elements (0 deactivated)
09:59:11 INFO opendrift:2096: 2016-02-04 10:00:00 - step 47 of 48 - 10 active elements (0 deactivated)
09:59:11 INFO opendrift:2096: 2016-02-04 11:00:00 - step 48 of 48 - 10 active elements (0 deactivated)
<xarray.Dataset> Size: 69kB Dimensions: ( trajectory: 10, time: 49) Coordinates: * trajectory (trajectory) int64 80B ... * time (time) datetime64[ns] 392B ... Data variables: (12/35) status (trajectory, time) float32 2kB ... moving (trajectory, time) float32 2kB ... age_seconds (trajectory, time) float32 2kB ... origin_marker (trajectory, time) float32 2kB ... lon (trajectory, time) float32 2kB ... lat (trajectory, time) float32 2kB ... ... ... surface_downward_y_stress (trajectory, time) float32 2kB ... turbulent_kinetic_energy (trajectory, time) float32 2kB ... turbulent_generic_length_scale (trajectory, time) float32 2kB ... ocean_mixed_layer_thickness (trajectory, time) float32 2kB ... sea_floor_depth_below_sea_level (trajectory, time) float32 2kB ... land_binary_mask (trajectory, time) float32 2kB ... Attributes: (12/155) Conventions: ... standard_name_vocabulary: ... featureType: ... title: ... summary: ... keywords: ... ... ... geospatial_lon_units: ... geospatial_lon_resolution: ... runtime: ... geospatial_vertical_min: ... geospatial_vertical_max: ... geospatial_vertical_positive: ...
xarray.Dataset
- trajectory: 10
- time: 49
- trajectory(trajectory)int640 1 2 3 4 5 6 7 8 9
- cf_role :
- trajectory_id
- dtype :
- <class 'numpy.int32'>
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
- time(time)datetime64[ns]2016-02-02T12:00:00 ... 2016-02-...
- standard_name :
- time
- long_name :
- time
- dtype :
- <class 'numpy.float64'>
array(['2016-02-02T12:00:00.000000000', '2016-02-02T13:00:00.000000000', '2016-02-02T14:00:00.000000000', '2016-02-02T15:00:00.000000000', '2016-02-02T16:00:00.000000000', '2016-02-02T17:00:00.000000000', '2016-02-02T18:00:00.000000000', '2016-02-02T19:00:00.000000000', '2016-02-02T20:00:00.000000000', '2016-02-02T21:00:00.000000000', '2016-02-02T22:00:00.000000000', '2016-02-02T23:00:00.000000000', '2016-02-03T00:00:00.000000000', '2016-02-03T01:00:00.000000000', '2016-02-03T02:00:00.000000000', '2016-02-03T03:00:00.000000000', '2016-02-03T04:00:00.000000000', '2016-02-03T05:00:00.000000000', '2016-02-03T06:00:00.000000000', '2016-02-03T07:00:00.000000000', '2016-02-03T08:00:00.000000000', '2016-02-03T09:00:00.000000000', '2016-02-03T10:00:00.000000000', '2016-02-03T11:00:00.000000000', '2016-02-03T12:00:00.000000000', '2016-02-03T13:00:00.000000000', '2016-02-03T14:00:00.000000000', '2016-02-03T15:00:00.000000000', '2016-02-03T16:00:00.000000000', '2016-02-03T17:00:00.000000000', '2016-02-03T18:00:00.000000000', '2016-02-03T19:00:00.000000000', '2016-02-03T20:00:00.000000000', '2016-02-03T21:00:00.000000000', '2016-02-03T22:00:00.000000000', '2016-02-03T23:00:00.000000000', '2016-02-04T00:00:00.000000000', '2016-02-04T01:00:00.000000000', '2016-02-04T02:00:00.000000000', '2016-02-04T03:00:00.000000000', '2016-02-04T04:00:00.000000000', '2016-02-04T05:00:00.000000000', '2016-02-04T06:00:00.000000000', '2016-02-04T07:00:00.000000000', '2016-02-04T08:00:00.000000000', '2016-02-04T09:00:00.000000000', '2016-02-04T10:00:00.000000000', '2016-02-04T11:00:00.000000000', '2016-02-04T12:00:00.000000000'], dtype='datetime64[ns]')
- status(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- valid_range :
- [0 0]
- flag_values :
- [0]
- flag_meanings :
- active
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- moving(trajectory, time)float321.0 1.0 1.0 1.0 ... 1.0 1.0 1.0 1.0
- dtype :
- <class 'numpy.int32'>
- minval :
- 1
- maxval :
- 1
array([[1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.]], dtype=float32)
- age_seconds(trajectory, time)float320.0 3.6e+03 ... 1.692e+05 1.728e+05
- dtype :
- <class 'numpy.float32'>
- units :
- s
- minval :
- 0.0
- maxval :
- 172800.0
array([[ 0., 3600., 7200., 10800., 14400., 18000., 21600., 25200., 28800., 32400., 36000., 39600., 43200., 46800., 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., 151200., 154800., 158400., 162000., 165600., 169200., 172800.], [ 0., 3600., 7200., 10800., 14400., 18000., 21600., 25200., 28800., 32400., 36000., 39600., 43200., 46800., 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., 151200., 154800., 158400., 162000., 165600., 169200., 172800.], [ 0., 3600., 7200., 10800., 14400., 18000., 21600., 25200., 28800., 32400., 36000., 39600., 43200., 46800., 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., ... 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., 151200., 154800., 158400., 162000., 165600., 169200., 172800.], [ 0., 3600., 7200., 10800., 14400., 18000., 21600., 25200., 28800., 32400., 36000., 39600., 43200., 46800., 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., 151200., 154800., 158400., 162000., 165600., 169200., 172800.], [ 0., 3600., 7200., 10800., 14400., 18000., 21600., 25200., 28800., 32400., 36000., 39600., 43200., 46800., 50400., 54000., 57600., 61200., 64800., 68400., 72000., 75600., 79200., 82800., 86400., 90000., 93600., 97200., 100800., 104400., 108000., 111600., 115200., 118800., 122400., 126000., 129600., 133200., 136800., 140400., 144000., 147600., 151200., 154800., 158400., 162000., 165600., 169200., 172800.]], dtype=float32)
- origin_marker(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- unit :
- description :
- An integer kept constant during the simulation. Different values may be used for different seedings, to separate elements during analysis. With GUI, only a single seeding is possible.
- flag_values :
- [0]
- flag_meanings :
- Seed_0
- minval :
- 0
- maxval :
- 0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- lon(trajectory, time)float3212.0 12.02 12.04 ... 12.93 12.95
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_east
- standard_name :
- longitude
- long_name :
- longitude
- axis :
- X
- minval :
- 12.0
- maxval :
- 12.947239
array([[12. , 12.018996 , 12.037794 , 12.056405 , 12.074805 , 12.092995 , 12.110989 , 12.1288 , 12.14647 , 12.164095 , 12.181681 , 12.199236 , 12.216766 , 12.234496 , 12.2525015, 12.270767 , 12.289283 , 12.308141 , 12.327544 , 12.347472 , 12.367882 , 12.38874 , 12.410128 , 12.431969 , 12.454182 , 12.47668 , 12.498998 , 12.521021 , 12.542655 , 12.5638075, 12.584392 , 12.604235 , 12.623463 , 12.642068 , 12.660049 , 12.677409 , 12.694152 , 12.710288 , 12.725729 , 12.740456 , 12.754704 , 12.768488 , 12.781808 , 12.794662 , 12.807049 , 12.818962 , 12.830394 , 12.841333 , 12.851771 ], [12. , 12.019123 , 12.038081 , 12.056887 , 12.075509 , 12.093954 , 12.112238 , 12.130374 , 12.148438 , 12.166499 , 12.184567 , 12.20265 , 12.220836 , 12.239367 , 12.258238 , 12.277439 , 12.297006 , 12.317229 , 12.338075 , 12.359502 , 12.381498 , 12.404141 , 12.427357 , 12.451058 , 12.475115 , 12.499354 , 12.523364 , 12.547039 , 12.570239 , 12.59265 , 12.614255 , 12.635036 , 12.655204 , 12.674754 , 12.693566 , 12.711586 , 12.728848 , 12.745386 , 12.761231 , 12.776416 , 12.791061 , 12.805298 , 12.819078 , 12.8323345, 12.845056 , 12.857226 , 12.868831 , 12.879849 , 12.890262 ], ... [12. , 12.015296 , 12.030646 , 12.046055 , 12.061481 , 12.076913 , 12.092358 , 12.1078205, 12.123359 , 12.139044 , 12.154882 , 12.170883 , 12.187079 , 12.2035265, 12.22023 , 12.237424 , 12.255258 , 12.273761 , 12.2928505, 12.312506 , 12.333019 , 12.354284 , 12.376255 , 12.399273 , 12.423236 , 12.448054 , 12.473335 , 12.498938 , 12.524768 , 12.5505495, 12.576006 , 12.601143 , 12.625963 , 12.650182 , 12.673749 , 12.696687 , 12.719008 , 12.740639 , 12.761575 , 12.781869 , 12.801531 , 12.820568 , 12.839152 , 12.857318 , 12.875081 , 12.892455 , 12.909456 , 12.926235 , 12.942861 ], [12. , 12.0131235, 12.027008 , 12.040999 , 12.055061 , 12.069217 , 12.083493 , 12.097902 , 12.112496 , 12.127411 , 12.142722 , 12.158438 , 12.174586 , 12.191264 , 12.208548 , 12.226383 , 12.2448435, 12.264011 , 12.283847 , 12.304377 , 12.325862 , 12.348154 , 12.371212 , 12.395239 , 12.420104 , 12.445736 , 12.471702 , 12.497941 , 12.524366 , 12.550559 , 12.576383 , 12.601848 , 12.62691 , 12.651287 , 12.675008 , 12.698093 , 12.7205515, 12.742285 , 12.763309 , 12.783663 , 12.803401 , 12.822614 , 12.8414135, 12.859816 , 12.877835 , 12.895488 , 12.912873 , 12.930121 , 12.947239 ]], dtype=float32)
- lat(trajectory, time)float3268.3 68.31 68.31 ... 68.59 68.59
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_north
- standard_name :
- latitude
- long_name :
- latitude
- axis :
- Y
- minval :
- 68.3
- maxval :
- 68.59417
array([[68.3 , 68.30504 , 68.31006 , 68.31506 , 68.320076, 68.32511 , 68.33018 , 68.33526 , 68.34038 , 68.345535, 68.35074 , 68.355995, 68.36129 , 68.366615, 68.371956, 68.37731 , 68.382675, 68.38801 , 68.39326 , 68.39841 , 68.403465, 68.408394, 68.41305 , 68.417404, 68.42145 , 68.42516 , 68.42843 , 68.43123 , 68.43356 , 68.43544 , 68.43685 , 68.43788 , 68.43864 , 68.43913 , 68.4394 , 68.43945 , 68.43932 , 68.43902 , 68.438614, 68.43815 , 68.437706, 68.43727 , 68.43684 , 68.4364 , 68.43595 , 68.43547 , 68.434944, 68.43437 , 68.43373 ], [68.3 , 68.30538 , 68.31077 , 68.31615 , 68.32156 , 68.32701 , 68.33251 , 68.33805 , 68.34365 , 68.34932 , 68.355064, 68.36088 , 68.36676 , 68.37269 , 68.378654, 68.38467 , 68.39071 , 68.39667 , 68.40255 , 68.40833 , 68.41398 , 68.419334, 68.4244 , 68.429146, 68.43352 , 68.437416, 68.440834, 68.44378 , 68.44626 , 68.448296, 68.449905, 68.45109 , 68.45201 , 68.4527 , 68.45323 , 68.45369 , 68.45407 , 68.4544 , 68.45468 , 68.454926, 68.45517 , 68.45546 , 68.45581 , 68.45624 , 68.45672 , 68.45723 , 68.45776 , 68.45828 , 68.45878 ], [68.3 , 68.305466, 68.310936, 68.31641 , 68.321915, 68.32747 , 68.33307 , 68.33872 , 68.34444 , 68.35023 , 68.3561 , 68.36205 , ... 68.552635, 68.55583 , 68.55908 , 68.5624 , 68.56577 , 68.56921 , 68.5727 ], [68.3 , 68.30618 , 68.31243 , 68.318756, 68.32517 , 68.331696, 68.33833 , 68.3451 , 68.35201 , 68.35911 , 68.36639 , 68.37388 , 68.381584, 68.3895 , 68.39765 , 68.40607 , 68.41468 , 68.42343 , 68.43231 , 68.44126 , 68.45006 , 68.45865 , 68.46701 , 68.47501 , 68.482635, 68.48986 , 68.49664 , 68.502975, 68.50889 , 68.51441 , 68.519585, 68.5244 , 68.52885 , 68.53305 , 68.53705 , 68.540825, 68.54438 , 68.54783 , 68.551254, 68.55469 , 68.55812 , 68.56156 , 68.56506 , 68.56863 , 68.572266, 68.575966, 68.579735, 68.58357 , 68.58746 ], [68.3 , 68.30649 , 68.31297 , 68.31954 , 68.32621 , 68.332985, 68.339874, 68.34688 , 68.35403 , 68.36135 , 68.368866, 68.376595, 68.38454 , 68.392715, 68.40114 , 68.40985 , 68.41876 , 68.42778 , 68.43687 , 68.44594 , 68.454796, 68.46342 , 68.47179 , 68.47978 , 68.48738 , 68.49459 , 68.501335, 68.50763 , 68.51348 , 68.51898 , 68.52414 , 68.52894 , 68.5334 , 68.53766 , 68.541725, 68.54557 , 68.549225, 68.55283 , 68.556404, 68.55996 , 68.563515, 68.567116, 68.57078 , 68.57451 , 68.57831 , 68.58217 , 68.586105, 68.5901 , 68.59417 ]], dtype=float32)
- z(trajectory, time)float320.0 0.0 0.0 ... -115.1 -115.1
- dtype :
- <class 'numpy.float32'>
- units :
- m
- standard_name :
- z
- long_name :
- vertical position
- axis :
- Z
- positive :
- up
- minval :
- -145.01424
- maxval :
- 0.0
array([[ 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. , 0. ], [ -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666, -16.666666], ... -133.33333 , -133.33333 , -133.33333 , -133.33333 , -133.33333 , -133.33333 , -133.33333 , -133.33333 , -131.41325 , -128.85619 , -126.01974 , -122.9878 , -119.90839 , -117.0533 , -115.41541 , -113.82893 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 , -112.26823 ], [-145.01424 , -144.87401 , -144.74911 , -144.36847 , -143.82245 , -143.24442 , -142.6316 , -141.81143 , -140.51796 , -139.19302 , -137.83368 , -136.21394 , -133.72862 , -131.17 , -128.52863 , -125.6285 , -122.8036 , -120.2668 , -118.13629 , -117.171906, -116.0738 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 , -115.08563 ]], dtype=float32)
- wind_drift_factor(trajectory, time)float320.02 0.02 0.02 ... 0.02 0.02 0.02
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements at surface are moved with this fraction of the vind vector, in addition to currents and Stokes drift
- minval :
- 0.02
- maxval :
- 0.02
array([[0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], ... [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02, 0.02]], dtype=float32)
- current_drift_factor(trajectory, time)float321.0 1.0 1.0 1.0 ... 1.0 1.0 1.0 1.0
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements are moved with this fraction of the current vector, in addition to currents and Stokes drift
- minval :
- 1.0
- maxval :
- 1.0
array([[1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.], [1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1., 1.]], dtype=float32)
- terminal_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.float32'>
- units :
- m/s
- description :
- Terminal rise/sinking velocity (buoyancy) in the ocean column
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- x_sea_water_velocity(trajectory, time)float320.2178 0.2155 ... 0.1938 0.1938
- minval :
- 0.118565544
- maxval :
- 0.3001663
array([[0.21777777, 0.21545067, 0.2132618 , 0.21079648, 0.2083422 , 0.20605281, 0.2039207 , 0.20225573, 0.20168716, 0.20120266, 0.20080034, 0.20047873, 0.20270751, 0.20579736, 0.20874374, 0.21153906, 0.21539982, 0.22158226, 0.2275157 , 0.23296289, 0.23803417, 0.24403134, 0.24915522, 0.25334987, 0.25655964, 0.25446016, 0.25108165, 0.2466141 , 0.24110752, 0.23461373, 0.22614922, 0.2191382 , 0.21204276, 0.20492344, 0.19783202, 0.19081222, 0.1839002 , 0.17597562, 0.16783683, 0.16240461, 0.15709898, 0.15181313, 0.14651436, 0.14118521, 0.13579106, 0.13030447, 0.12470538, 0.11897684, 0.11897684], [0.2192253 , 0.21727958, 0.21547648, 0.21333621, 0.21125531, 0.20934872, 0.20761031, 0.20673552, 0.20665354, 0.20667434, 0.20679782, 0.20790711, 0.21181197, 0.2156398 , 0.2193527 , 0.22346848, 0.230919 , 0.23794858, 0.24452795, 0.25095916, 0.2582848 , 0.2647446 , 0.27023676, 0.27422866, 0.27627125, 0.2736009 , 0.26976508, 0.26432118, 0.25530574, 0.24610128, 0.23670971, 0.22971338, 0.22267051, 0.21426181, 0.2052268 , 0.19660135, 0.18834873, 0.18046285, 0.17292516, 0.16678956, 0.16213115, 0.15693322, 0.15096428, 0.14486519, 0.13859972, 0.13214022, 0.12546542, 0.11856554, 0.11856554], ... [0.17533877, 0.17592897, 0.17653665, 0.17668588, 0.17670536, 0.17679545, 0.1769613 , 0.17775631, 0.17938623, 0.1810896 , 0.18287674, 0.18505068, 0.18785359, 0.1907077 , 0.19623776, 0.20346212, 0.21101733, 0.21762422, 0.2239881 , 0.23367026, 0.242143 , 0.25007492, 0.2619095 , 0.27257577, 0.2822089 , 0.28738585, 0.29096282, 0.2934672 , 0.29284006, 0.2890917 , 0.28539792, 0.28173923, 0.27486598, 0.26742718, 0.26023686, 0.25321493, 0.24532743, 0.23741648, 0.23010796, 0.22289653, 0.21578917, 0.21061267, 0.20584795, 0.20124502, 0.19681688, 0.19254379, 0.19000815, 0.18822806, 0.18822806], [0.15043677, 0.15911993, 0.16029304, 0.16105926, 0.1620712 , 0.1634181 , 0.16488941, 0.16694108, 0.17055982, 0.17503694, 0.17959538, 0.18448184, 0.19046292, 0.19730091, 0.20352405, 0.21057321, 0.21855998, 0.22607863, 0.23390174, 0.24468729, 0.25378567, 0.26240188, 0.2733279 , 0.2827766 , 0.29139742, 0.29510835, 0.2981386 , 0.3001663 , 0.29745543, 0.29319862, 0.28906536, 0.2844426 , 0.27660614, 0.26910424, 0.2618664 , 0.25469312, 0.24644902, 0.23835176, 0.23073938, 0.22370881, 0.21771643, 0.21300875, 0.20847057, 0.20410022, 0.19990537, 0.1968546 , 0.19525443, 0.19375178, 0.19375178]], dtype=float32)
- y_sea_water_velocity(trajectory, time)float320.1561 0.1555 ... 0.1259 0.1259
- minval :
- -0.019770665
- maxval :
- 0.2814455
array([[ 0.15607086, 0.15554969, 0.15510467, 0.15541995, 0.15606135, 0.15678473, 0.15758719, 0.15856382, 0.15990366, 0.16129543, 0.16274352, 0.16425245, 0.16502911, 0.1654955 , 0.16587101, 0.16615961, 0.16537085, 0.16250855, 0.15976694, 0.15665936, 0.15279867, 0.14415245, 0.13499486, 0.12534146, 0.11520682, 0.1012563 , 0.08678375, 0.07240164, 0.05812557, 0.04396812, 0.03193604, 0.02328417, 0.01541318, 0.00826761, 0.0017907 , -0.00407424, -0.00938269, -0.01242779, -0.01439667, -0.0138239 , -0.01343467, -0.01332635, -0.01352201, -0.01404891, -0.01492121, -0.0161547 , -0.01776611, -0.01977067, -0.01977067], [ 0.16675992, 0.16673814, 0.16680989, 0.16774926, 0.16897722, 0.17031899, 0.17177476, 0.17356856, 0.17567413, 0.17787509, 0.18017764, 0.18231519, 0.18368554, 0.18502057, 0.18629679, 0.18707652, 0.184837 , 0.1822286 , 0.17926425, 0.17484504, 0.16609682, 0.15688252, 0.14713491, 0.13547803, 0.1208234 , 0.10597734, 0.09129336, 0.07689638, 0.0632377 , 0.04984454, 0.03679437, 0.0285824 , 0.02119374, 0.01669037, 0.0141272 , 0.01197461, 0.01018607, 0.00872066, 0.00753946, 0.0076609 , 0.00898523, 0.0108624 , 0.01321585, 0.01491849, 0.01597105, 0.01637709, 0.01615671, 0.01530157, 0.01530157], ... 0.205792 , 0.20961186, 0.21418263, 0.21976346, 0.22571278, 0.23206848, 0.23869516, 0.2454445 , 0.252637 , 0.26075166, 0.2668943 , 0.2711104 , 0.275131 , 0.277432 , 0.2724725 , 0.26620898, 0.2591578 , 0.24805212, 0.23610283, 0.22398153, 0.21013412, 0.19643995, 0.18316737, 0.17122768, 0.16040094, 0.14925545, 0.13773166, 0.13033654, 0.12384185, 0.11715408, 0.11027838, 0.10691322, 0.10615879, 0.10625352, 0.10642767, 0.10668318, 0.10850827, 0.110562 , 0.11263681, 0.11474307, 0.11685684, 0.11879344, 0.12065318, 0.12065318], [ 0.20100544, 0.20093648, 0.20354295, 0.20660089, 0.20991282, 0.21339844, 0.21714078, 0.22148281, 0.22698253, 0.23287135, 0.23932894, 0.24625422, 0.2532499 , 0.26109403, 0.2698768 , 0.2763139 , 0.27943572, 0.2814455 , 0.2811526 , 0.27453867, 0.26722825, 0.25925094, 0.24769355, 0.23568195, 0.22337246, 0.2090588 , 0.19500133, 0.18154928, 0.17038731, 0.15975763, 0.1487996 , 0.1382913 , 0.13217588, 0.12585527, 0.11934759, 0.11318252, 0.1118938 , 0.11068968, 0.11012092, 0.11034349, 0.11143039, 0.11351069, 0.11561003, 0.11772675, 0.11986552, 0.12193 , 0.12389946, 0.12588099, 0.12588099]], dtype=float32)
- sea_surface_height(trajectory, time)float320.2854 0.2812 ... 0.05152 0.05152
- minval :
- 0.051515914
- maxval :
- 0.28540272
array([[0.28540272, 0.2812017 , 0.276999 , 0.27285415, 0.2687156 , 0.26455802, 0.2603817 , 0.25619876, 0.2520374 , 0.24786821, 0.24369088, 0.23950505, 0.23528627, 0.23107833, 0.2269104 , 0.22278343, 0.21863396, 0.21438165, 0.21007995, 0.20587197, 0.2017246 , 0.19729513, 0.1930232 , 0.1889123 , 0.18496472, 0.18050021, 0.17614698, 0.17196655, 0.1679462 , 0.16407263, 0.1599146 , 0.1555083 , 0.15115999, 0.14685762, 0.14259027, 0.13834815, 0.1341224 , 0.1298457 , 0.12553546, 0.12100461, 0.11643305, 0.11182591, 0.10718326, 0.10250226, 0.09778567, 0.09303512, 0.08825127, 0.08343484, 0.08343484], [0.28540272, 0.28109884, 0.27678967, 0.27254432, 0.2682953 , 0.26402342, 0.2597287 , 0.25543693, 0.2511532 , 0.24685735, 0.24254864, 0.23821759, 0.2338546 , 0.22952223, 0.225227 , 0.22094181, 0.21652341, 0.21223313, 0.20807572, 0.20396101, 0.1994234 , 0.19499667, 0.19074538, 0.18652667, 0.18221723, 0.1778024 , 0.17358197, 0.16949405, 0.16529553, 0.16122408, 0.15726681, 0.15292713, 0.14864475, 0.14432648, 0.13997553, 0.13562529, 0.13127124, 0.12690629, 0.12252565, 0.11804716, 0.11343692, 0.10879739, 0.10413493, 0.09943146, 0.09469016, 0.08991387, 0.08510381, 0.08026421, 0.08026421], ... [0.28540272, 0.28045514, 0.27548242, 0.27055195, 0.2656143 , 0.26063758, 0.25561887, 0.25057667, 0.24552022, 0.24041677, 0.23526177, 0.2300322 , 0.2247081 , 0.21932389, 0.21386921, 0.20821318, 0.20244792, 0.19669795, 0.1908759 , 0.18471298, 0.17870706, 0.1728705 , 0.16683923, 0.161076 , 0.1556066 , 0.15001646, 0.14463788, 0.13953431, 0.13461299, 0.12980133, 0.12514721, 0.12065138, 0.11635115, 0.11211787, 0.10792167, 0.10377625, 0.09980991, 0.09577451, 0.09153784, 0.08726655, 0.08295994, 0.07876721, 0.07456399, 0.07032844, 0.06605382, 0.06174701, 0.05748867, 0.05325147, 0.05325147], [0.28540272, 0.28013945, 0.27493706, 0.26976678, 0.26459694, 0.25939363, 0.25415686, 0.24889778, 0.24364138, 0.23835422, 0.23303959, 0.22767916, 0.22223374, 0.21675272, 0.21123278, 0.20550938, 0.19956245, 0.19356126, 0.18751128, 0.18124422, 0.17523038, 0.16942108, 0.16352342, 0.15796328, 0.15273243, 0.14735445, 0.14216775, 0.1371664 , 0.13229874, 0.12755896, 0.12296897, 0.11853773, 0.11428108, 0.11005366, 0.10586087, 0.10173333, 0.09781931, 0.09387718, 0.08978038, 0.08549014, 0.08123682, 0.07704145, 0.07281708, 0.06856142, 0.06426974, 0.0599923 , 0.05575633, 0.05151591, 0.05151591]], dtype=float32)
- x_wind(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- y_wind(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- upward_sea_water_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- ocean_vertical_diffusivity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_significant_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_stokes_drift_x_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_stokes_drift_y_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_period_at_variance_spectral_density_maximum(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment(trajectory, time)float321.257 1.257 1.257 ... 1.257 1.257
- minval :
- 1.2566371
- maxval :
- 1.2566371
array([[1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371], [1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371], [1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, ... 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371], [1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371], [1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371, 1.2566371]], dtype=float32)
- sea_surface_swell_wave_to_direction(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_swell_wave_peak_period_from_variance_spectral_density(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_swell_wave_significant_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_to_direction(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_mean_period(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- sea_surface_wind_wave_significant_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- surface_downward_x_stress(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- surface_downward_y_stress(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- turbulent_kinetic_energy(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- turbulent_generic_length_scale(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- ocean_mixed_layer_thickness(trajectory, time)float3250.0 50.0 50.0 ... 50.0 50.0 50.0
- minval :
- 50.0
- maxval :
- 50.0
array([[50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.], [50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50., 50.]], dtype=float32)
- sea_floor_depth_below_sea_level(trajectory, time)float32144.7 144.7 144.6 ... 143.7 143.7
- minval :
- 107.6641
- maxval :
- 144.72884
array([[144.72884 , 144.67424 , 144.6002 , 144.30568 , 143.96576 , 143.65767 , 143.3785 , 142.93623 , 141.91878 , 140.89528 , 139.8654 , 138.82877 , 137.1374 , 135.19044 , 133.22884 , 131.25375 , 129.11626 , 126.668396, 124.27837 , 121.89397 , 119.55142 , 117.628296, 115.73457 , 113.87106 , 112.039215, 111.36639 , 110.95084 , 110.55869 , 110.18916 , 109.84257 , 110.828445, 112.30263 , 113.79114 , 115.28778 , 116.78696 , 118.28357 , 119.77307 , 121.562546, 123.384125, 125.303024, 127.125626, 128.85107 , 130.48381 , 132.0233 , 133.47668 , 134.84901 , 136.14339 , 137.36278 , 137.36278 ], [144.72884 , 144.66356 , 144.58049 , 144.2391 , 143.8663 , 143.51973 , 143.19626 , 142.4873 , 141.39041 , 140.28313 , 139.16487 , 137.80542 , 135.7154 , 133.59741 , 131.45569 , 129.23035 , 126.573006, 123.88356 , 121.16234 , 118.52008 , 116.37885 , 114.29669 , 112.23932 , 110.72584 , 110.23054 , 109.754944, 109.29574 , 109.07313 , 110.09598 , 111.03415 , 111.9059 , 113.49078 , 115.09282 , 117.21597 , 119.55218 , 121.724945, 123.75304 , 125.6416 , 127.40048 , 129.13023 , 130.86588 , 132.50969 , 134.01079 , 135.3498 , 136.53848 , 137.58821 , 138.5125 , 139.31827 , 139.31827 ], ... [144.72884 , 144.6164 , 144.5049 , 144.1212 , 143.61858 , 143.09724 , 142.5536 , 141.70453 , 140.44 , 139.1459 , 137.82034 , 136.10562 , 133.67987 , 131.19392 , 128.64232 , 125.81152 , 122.785355, 119.711685, 116.86243 , 115.2307 , 113.65023 , 112.09536 , 112.90028 , 113.74682 , 114.44146 , 116.12817 , 117.968475, 119.52127 , 121.412285, 123.853355, 126.0007 , 127.844955, 129.91066 , 131.90047 , 133.70627 , 135.31909 , 136.17201 , 137.24503 , 139.02635 , 140.70847 , 142.29993 , 142.88258 , 143.26743 , 143.59062 , 143.86256 , 144.08842 , 143.79185 , 143.24458 , 143.24458 ], [144.72884 , 144.59387 , 144.47418 , 144.09871 , 143.55785 , 142.98502 , 142.37746 , 141.56253 , 140.27432 , 138.95467 , 137.60065 , 135.98627 , 133.5064 , 130.95325 , 128.3174 , 125.42299 , 122.604034, 120.07324 , 117.948784, 116.99066 , 115.89857 , 114.916214, 116.00521 , 116.702225, 116.924835, 118.18411 , 119.60415 , 121.221954, 123.581436, 126.06091 , 128.24051 , 130.20837 , 132.43906 , 134.48305 , 136.34373 , 137.93037 , 138.54556 , 139.06775 , 140.10555 , 141.83392 , 142.99713 , 143.50024 , 143.93463 , 144.3091 , 144.63338 , 144.62416 , 144.17418 , 143.6664 , 143.6664 ]], dtype=float32)
- land_binary_mask(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.], [0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0., 0.]], dtype=float32)
- trajectoryPandasIndex
PandasIndex(Index([0, 1, 2, 3, 4, 5, 6, 7, 8, 9], dtype='int64', name='trajectory'))
- timePandasIndex
PandasIndex(DatetimeIndex(['2016-02-02 12:00:00', '2016-02-02 13:00:00', '2016-02-02 14:00:00', '2016-02-02 15:00:00', '2016-02-02 16:00:00', '2016-02-02 17:00:00', '2016-02-02 18:00:00', '2016-02-02 19:00:00', '2016-02-02 20:00:00', '2016-02-02 21:00:00', '2016-02-02 22:00:00', '2016-02-02 23:00:00', '2016-02-03 00:00:00', '2016-02-03 01:00:00', '2016-02-03 02:00:00', '2016-02-03 03:00:00', '2016-02-03 04:00:00', '2016-02-03 05:00:00', '2016-02-03 06:00:00', '2016-02-03 07:00:00', '2016-02-03 08:00:00', '2016-02-03 09:00:00', '2016-02-03 10:00:00', '2016-02-03 11:00:00', '2016-02-03 12:00:00', '2016-02-03 13:00:00', '2016-02-03 14:00:00', '2016-02-03 15:00:00', '2016-02-03 16:00:00', '2016-02-03 17:00:00', '2016-02-03 18:00:00', '2016-02-03 19:00:00', '2016-02-03 20:00:00', '2016-02-03 21:00:00', '2016-02-03 22:00:00', '2016-02-03 23:00:00', '2016-02-04 00:00:00', '2016-02-04 01:00:00', '2016-02-04 02:00:00', '2016-02-04 03:00:00', '2016-02-04 04:00:00', '2016-02-04 05:00:00', '2016-02-04 06:00:00', '2016-02-04 07:00:00', '2016-02-04 08:00:00', '2016-02-04 09:00:00', '2016-02-04 10:00:00', '2016-02-04 11:00:00', '2016-02-04 12:00:00'], dtype='datetime64[ns]', name='time', freq='h'))
- Conventions :
- CF-1.11, ACDD-1.3
- standard_name_vocabulary :
- CF Standard Name Table v85
- featureType :
- trajectory
- title :
- OpenDrift trajectory simulation
- summary :
- Output from simulation with OpenDrift framework
- keywords :
- trajectory, drift, lagrangian, simulation
- history :
- Created 2025-06-26 09:59:09.179401
- date_created :
- 2025-06-26T09:59:09.179407
- source :
- Output from simulation with OpenDrift
- model_url :
- https://github.com/OpenDrift/opendrift
- opendrift_class :
- OceanDrift
- opendrift_module :
- opendrift.models.oceandrift
- readers :
- odict_keys(['roms native', 'global_landmask'])
- time_coverage_start :
- 2016-02-02 12:00:00
- time_step_calculation :
- 1:00:00
- time_step_output :
- 1:00:00
- config_environment:constant:x_sea_water_velocity :
- None
- config_environment:fallback:x_sea_water_velocity :
- 0
- config_environment:constant:y_sea_water_velocity :
- None
- config_environment:fallback:y_sea_water_velocity :
- 0
- config_environment:constant:sea_surface_height :
- None
- config_environment:fallback:sea_surface_height :
- 0
- config_environment:constant:x_wind :
- None
- config_environment:fallback:x_wind :
- 0
- config_environment:constant:y_wind :
- None
- config_environment:fallback:y_wind :
- 0
- config_environment:constant:upward_sea_water_velocity :
- None
- config_environment:fallback:upward_sea_water_velocity :
- 0
- config_environment:constant:ocean_vertical_diffusivity :
- None
- config_environment:fallback:ocean_vertical_diffusivity :
- 0
- config_environment:constant:sea_surface_wave_significant_height :
- None
- config_environment:fallback:sea_surface_wave_significant_height :
- 0
- config_environment:constant:sea_surface_wave_stokes_drift_x_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_x_velocity :
- 0
- config_environment:constant:sea_surface_wave_stokes_drift_y_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_y_velocity :
- 0
- config_environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum :
- None
- config_environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- config_environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- None
- config_environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- config_environment:constant:sea_surface_swell_wave_to_direction :
- None
- config_environment:fallback:sea_surface_swell_wave_to_direction :
- 0
- config_environment:constant:sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- None
- config_environment:fallback:sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- 0
- config_environment:constant:sea_surface_swell_wave_significant_height :
- None
- config_environment:fallback:sea_surface_swell_wave_significant_height :
- 0
- config_environment:constant:sea_surface_wind_wave_to_direction :
- None
- config_environment:fallback:sea_surface_wind_wave_to_direction :
- 0
- config_environment:constant:sea_surface_wind_wave_mean_period :
- None
- config_environment:fallback:sea_surface_wind_wave_mean_period :
- 0
- config_environment:constant:sea_surface_wind_wave_significant_height :
- None
- config_environment:fallback:sea_surface_wind_wave_significant_height :
- 0
- config_environment:constant:surface_downward_x_stress :
- None
- config_environment:fallback:surface_downward_x_stress :
- 0
- config_environment:constant:surface_downward_y_stress :
- None
- config_environment:fallback:surface_downward_y_stress :
- 0
- config_environment:constant:turbulent_kinetic_energy :
- None
- config_environment:fallback:turbulent_kinetic_energy :
- 0
- config_environment:constant:turbulent_generic_length_scale :
- None
- config_environment:fallback:turbulent_generic_length_scale :
- 0
- config_environment:constant:ocean_mixed_layer_thickness :
- None
- config_environment:fallback:ocean_mixed_layer_thickness :
- 50
- config_environment:constant:sea_floor_depth_below_sea_level :
- None
- config_environment:fallback:sea_floor_depth_below_sea_level :
- 10000
- config_environment:constant:land_binary_mask :
- None
- config_environment:fallback:land_binary_mask :
- None
- config_general:use_auto_landmask :
- True
- config_drift:current_uncertainty :
- 0
- config_drift:current_uncertainty_uniform :
- 0
- config_drift:max_speed :
- 2.0
- config_readers:max_number_of_fails :
- 1
- config_general:simulation_name :
- config_general:coastline_action :
- stranding
- config_general:coastline_approximation_precision :
- 0.001
- config_general:time_step_minutes :
- 60
- config_general:time_step_output_minutes :
- None
- config_seed:ocean_only :
- True
- config_seed:number :
- 1
- config_drift:max_age_seconds :
- None
- config_drift:advection_scheme :
- euler
- config_drift:horizontal_diffusivity :
- 0
- config_drift:profiles_depth :
- 50
- config_drift:wind_uncertainty :
- 0
- config_drift:relative_wind :
- False
- config_drift:deactivate_north_of :
- None
- config_drift:deactivate_south_of :
- None
- config_drift:deactivate_east_of :
- None
- config_drift:deactivate_west_of :
- None
- config_seed:origin_marker :
- 0
- config_seed:z :
- 0
- config_seed:wind_drift_factor :
- 0.02
- config_seed:current_drift_factor :
- 1
- config_seed:terminal_velocity :
- 0.0
- config_drift:vertical_advection :
- True
- config_drift:vertical_advection_at_surface :
- False
- config_drift:vertical_mixing :
- False
- config_drift:vertical_mixing_at_surface :
- False
- config_vertical_mixing:timestep :
- 60
- config_vertical_mixing:diffusivitymodel :
- environment
- config_vertical_mixing:background_diffusivity :
- 1.2e-05
- config_vertical_mixing:TSprofiles :
- False
- config_drift:wind_drift_depth :
- 0.1
- config_drift:stokes_drift :
- True
- config_drift:stokes_drift_profile :
- Phillips
- config_drift:use_tabularised_stokes_drift :
- False
- config_drift:tabularised_stokes_drift_fetch :
- 25000
- config_general:seafloor_action :
- lift_to_seafloor
- config_drift:truncate_ocean_model_below_m :
- None
- config_seed:seafloor :
- False
- opendrift_version :
- 1.14.2
- seed_geojson :
- {"features": [], "type": "FeatureCollection"}
- simulation_time :
- 2025-06-26 09:59:09.184707
- reader_x_sea_water_velocity :
- ['roms native']
- reader_y_sea_water_velocity :
- ['roms native']
- reader_sea_surface_height :
- ['roms native']
- reader_x_wind :
- 0
- reader_y_wind :
- 0
- reader_upward_sea_water_velocity :
- 0
- reader_ocean_vertical_diffusivity :
- 0
- reader_sea_surface_wave_significant_height :
- 0
- reader_sea_surface_wave_stokes_drift_x_velocity :
- 0
- reader_sea_surface_wave_stokes_drift_y_velocity :
- 0
- reader_sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- reader_sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- reader_sea_surface_swell_wave_to_direction :
- 0
- reader_sea_surface_swell_wave_peak_period_from_variance_spectral_density :
- 0
- reader_sea_surface_swell_wave_significant_height :
- 0
- reader_sea_surface_wind_wave_to_direction :
- 0
- reader_sea_surface_wind_wave_mean_period :
- 0
- reader_sea_surface_wind_wave_significant_height :
- 0
- reader_surface_downward_x_stress :
- 0
- reader_surface_downward_y_stress :
- 0
- reader_turbulent_kinetic_energy :
- 0
- reader_turbulent_generic_length_scale :
- 0
- reader_ocean_mixed_layer_thickness :
- 50
- reader_sea_floor_depth_below_sea_level :
- ['roms native']
- reader_land_binary_mask :
- ['global_landmask']
- time_coverage_end :
- 2016-02-04 12:00:00
- time_coverage_duration :
- P2DT0H0M0S
- time_coverage_resolution :
- P0DT1H0M0S
- performance :
- -------------------- Reader performance: -------------------- roms native 0:00:00.3 total 0:00:00.0 preparing 0:00:00.3 reading 0:00:00.1 interpolation 0:00:00.0 interpolation_time 0:00:00.0 rotating vectors 0:00:00.0 masking -------------------- global_landmask 0:00:00.0 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 masking -------------------- Performance: 7.4 total time 5.4 configuration 0.0 preparing main loop 0.0 moving elements to ocean 1.9 main loop 0.0 updating elements 0.0 cleaning up --------------------
- geospatial_bounds_crs :
- EPSG:4326
- geospatial_bounds_vertical_crs :
- EPSG:5831
- geospatial_lat_min :
- 68.3
- geospatial_lat_max :
- 68.59417
- geospatial_lat_units :
- degrees_north
- geospatial_lat_resolution :
- point
- geospatial_lon_min :
- 12.0
- geospatial_lon_max :
- 12.947239
- geospatial_lon_units :
- degrees_east
- geospatial_lon_resolution :
- point
- runtime :
- 0:00:07.440083
- geospatial_vertical_min :
- -145.01424
- geospatial_vertical_max :
- 0.0
- geospatial_vertical_positive :
- up
Print and plot results, with lines colored by particle depth
print(o)
o.plot(linecolor='z', fast=True)
#o.animation()
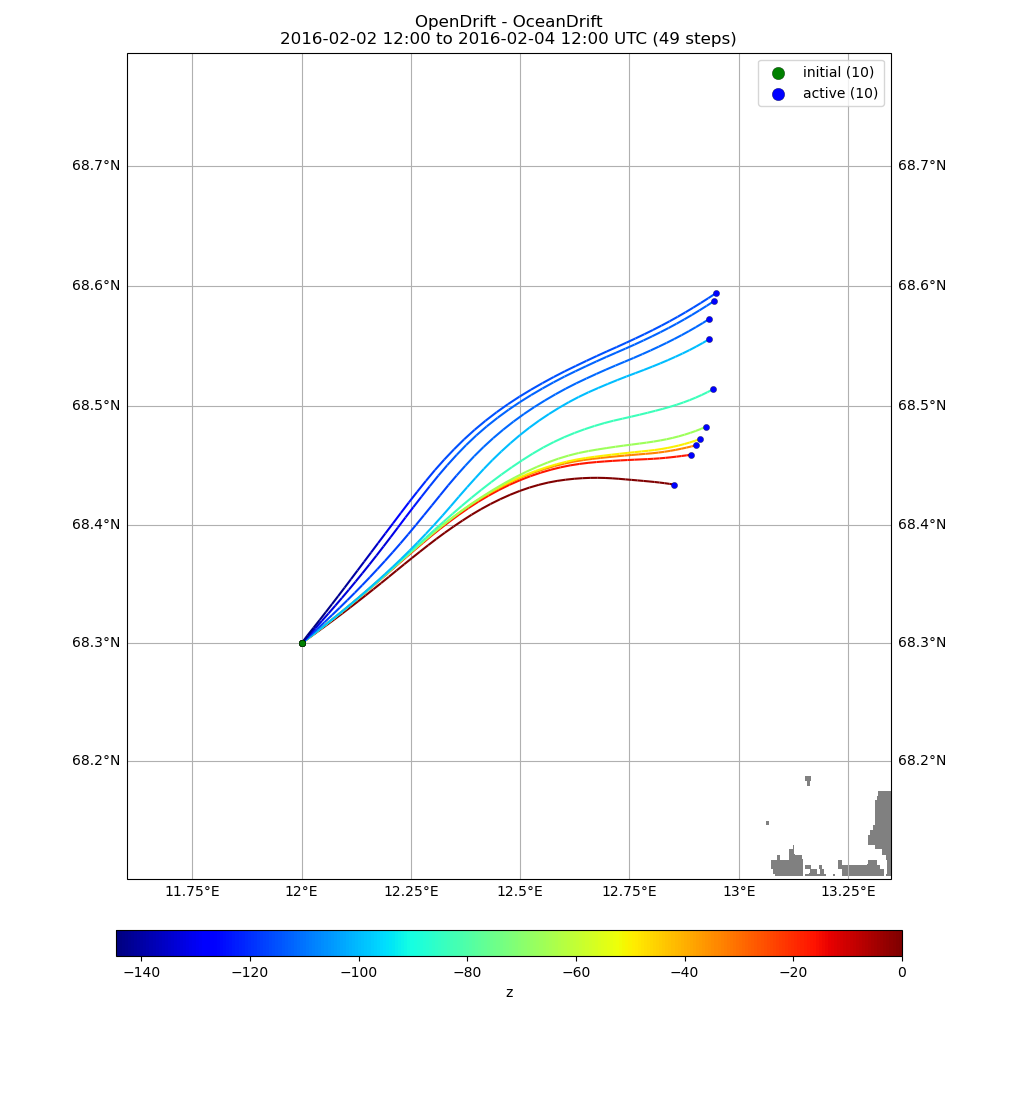
===========================
--------------------
Reader performance:
--------------------
roms native
0:00:00.3 total
0:00:00.0 preparing
0:00:00.3 reading
0:00:00.1 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
global_landmask
0:00:00.0 total
0:00:00.0 preparing
0:00:00.0 reading
0:00:00.0 masking
--------------------
Performance:
7.4 total time
5.4 configuration
0.0 preparing main loop
0.0 moving elements to ocean
1.9 main loop
0.0 updating elements
0.0 cleaning up
--------------------
===========================
Model: OceanDrift (OpenDrift version 1.14.2)
10 active Lagrangian3DArray particles (0 deactivated, 0 scheduled)
-------------------
Environment variables:
-----
sea_floor_depth_below_sea_level
sea_surface_height
x_sea_water_velocity
y_sea_water_velocity
1) roms native
-----
land_binary_mask
1) global_landmask
-----
Readers not added for the following variables:
ocean_mixed_layer_thickness
ocean_vertical_diffusivity
sea_surface_swell_wave_peak_period_from_variance_spectral_density
sea_surface_swell_wave_significant_height
sea_surface_swell_wave_to_direction
sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment
sea_surface_wave_period_at_variance_spectral_density_maximum
sea_surface_wave_significant_height
sea_surface_wave_stokes_drift_x_velocity
sea_surface_wave_stokes_drift_y_velocity
sea_surface_wind_wave_mean_period
sea_surface_wind_wave_significant_height
sea_surface_wind_wave_to_direction
surface_downward_x_stress
surface_downward_y_stress
turbulent_generic_length_scale
turbulent_kinetic_energy
upward_sea_water_velocity
x_wind
y_wind
Discarded readers:
Time:
Start: 2016-02-02 12:00:00 UTC
Present: 2016-02-04 12:00:00 UTC
Calculation steps: 48 * 1:00:00 - total time: 2 days, 0:00:00
Output steps: 49 * 1:00:00
===========================
09:59:11 WARNING opendrift:2452: Plotting fast. This will make your plots less accurate.
(<GeoAxes: title={'center': 'OpenDrift - OceanDrift\n2016-02-02 12:00 to 2016-02-04 12:00 UTC (49 steps)'}>, <Figure size 1017.13x1100 with 2 Axes>)
Total running time of the script: (0 minutes 15.863 seconds)