Note
Go to the end to download the full example code.
Cone seeding
from datetime import datetime, timedelta
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.openoil import OpenOil
o = OpenOil(loglevel=20) # Set loglevel to 0 for debug information
09:44:31 INFO opendrift:507: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-21-g950aeec)
Using live data from Thredds
o.add_readers_from_list([
'https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be'])
Adjusting some configuration
o.set_config('processes:dispersion', True)
o.set_config('processes:evaporation', False)
o.set_config('processes:emulsification', True)
Seed elements along cone, e.g. ship track with increasing uncertainty in position
latstart = 68.988911
lonstart = 16.040701
latend = 69.991446
lonend = 17.760061
time = [datetime.utcnow(), datetime.utcnow() + timedelta(hours=12)]
o.seed_cone(lon=[lonstart, lonend], lat=[latstart, latend],
oil_type='EKOFISK', radius=[100, 800], number=10000, time=[time])
print(o)
/root/project/examples/example_cone.py:31: DeprecationWarning: datetime.datetime.utcnow() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.now(datetime.UTC).
time = [datetime.utcnow(), datetime.utcnow() + timedelta(hours=12)]
09:44:31 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
09:44:35 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_height: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: upward_sea_water_velocity: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_ice_area_fraction: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_ice_x_velocity: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_ice_y_velocity: 0.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_water_temperature: 10.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_water_salinity: 34.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: sea_floor_depth_below_sea_level: 10000.000000
09:44:35 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.020000
09:44:35 INFO opendrift.models.basemodel.environment:232: ocean_mixed_layer_thickness: 50.000000
09:44:35 INFO opendrift.models.openoil.adios.dirjs:86: Querying ADIOS database for oil: EKOFISK
09:44:35 WARNING opendrift.models.openoil.adios.dirjs:90: Several oils found with name: EKOFISK: ['AD00328', 'AD00329', 'AD00332', 'AD00333', 'AD01944', 'AD02094', 'AD02463', 'AD02558', 'NO00013', 'NO00014', 'NO00015', 'NO00016'], using first.
09:44:35 INFO opendrift.models.openoil.openoil:1712: Using density 809.002835 and viscosity 3.3498550728972226e-06 of oiltype EKOFISK
===========================
Model: OpenOil (OpenDrift version 1.14.2)
0 active Oil particles (0 deactivated, 10000 scheduled)
-------------------
Environment variables:
-----
land_binary_mask
1) global_landmask
-----
Readers not added for the following variables:
ocean_mixed_layer_thickness
ocean_vertical_diffusivity
sea_floor_depth_below_sea_level
sea_ice_area_fraction
sea_ice_x_velocity
sea_ice_y_velocity
sea_surface_height
sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment
sea_surface_wave_period_at_variance_spectral_density_maximum
sea_surface_wave_significant_height
sea_surface_wave_stokes_drift_x_velocity
sea_surface_wave_stokes_drift_y_velocity
sea_water_salinity
sea_water_temperature
upward_sea_water_velocity
x_sea_water_velocity
x_wind
y_sea_water_velocity
y_wind
---
Lazy readers:
LazyReader: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
Discarded readers:
===========================
Running model for 24 hours
o.run(steps=24*2, time_step=1800, time_step_output=3600)
09:44:35 INFO opendrift:911: Using existing reader for land_binary_mask
09:44:35 INFO opendrift:940: All points are in ocean
09:44:35 INFO opendrift.models.openoil.openoil:687: Oil-water surface tension is 0.027884 Nm
09:44:35 INFO opendrift.models.openoil.openoil:700: Max water fraction not available for EKOFISK, using default
09:44:35 INFO opendrift:2102: 2025-05-28 09:44:31.224386 - step 1 of 48 - 417 active elements (0 deactivated)
09:44:35 INFO opendrift.readers:61: Opening file with xr.open_dataset
09:44:37 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
09:44:40 INFO opendrift:2102: 2025-05-28 10:14:31.224386 - step 2 of 48 - 834 active elements (0 deactivated)
09:44:42 INFO opendrift:2102: 2025-05-28 10:44:31.224386 - step 3 of 48 - 1250 active elements (0 deactivated)
09:44:42 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:42 INFO opendrift:2102: 2025-05-28 11:14:31.224386 - step 4 of 48 - 1667 active elements (0 deactivated)
09:44:44 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:44 INFO opendrift:2102: 2025-05-28 11:44:31.224386 - step 5 of 48 - 2084 active elements (0 deactivated)
09:44:44 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:44 INFO opendrift:2102: 2025-05-28 12:14:31.224386 - step 6 of 48 - 2500 active elements (0 deactivated)
09:44:46 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:46 INFO opendrift:2102: 2025-05-28 12:44:31.224386 - step 7 of 48 - 2917 active elements (0 deactivated)
09:44:46 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:46 INFO opendrift:2102: 2025-05-28 13:14:31.224386 - step 8 of 48 - 3334 active elements (0 deactivated)
09:44:48 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:48 INFO opendrift:2102: 2025-05-28 13:44:31.224386 - step 9 of 48 - 3750 active elements (0 deactivated)
09:44:48 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:49 INFO opendrift:2102: 2025-05-28 14:14:31.224386 - step 10 of 48 - 4167 active elements (0 deactivated)
09:44:50 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:51 INFO opendrift:2102: 2025-05-28 14:44:31.224386 - step 11 of 48 - 4583 active elements (0 deactivated)
09:44:51 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:51 INFO opendrift:2102: 2025-05-28 15:14:31.224386 - step 12 of 48 - 5000 active elements (0 deactivated)
09:44:53 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:53 INFO opendrift:2102: 2025-05-28 15:44:31.224386 - step 13 of 48 - 5417 active elements (0 deactivated)
09:44:53 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:53 INFO opendrift:2102: 2025-05-28 16:14:31.224386 - step 14 of 48 - 5833 active elements (0 deactivated)
09:44:55 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:55 INFO opendrift:2102: 2025-05-28 16:44:31.224386 - step 15 of 48 - 6250 active elements (0 deactivated)
09:44:55 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:56 INFO opendrift:2102: 2025-05-28 17:14:31.224386 - step 16 of 48 - 6667 active elements (0 deactivated)
09:44:58 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:58 INFO opendrift:2102: 2025-05-28 17:44:31.224386 - step 17 of 48 - 7083 active elements (0 deactivated)
09:44:58 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:44:58 INFO opendrift:2102: 2025-05-28 18:14:31.224386 - step 18 of 48 - 7500 active elements (0 deactivated)
09:45:01 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:01 INFO opendrift:2102: 2025-05-28 18:44:31.224386 - step 19 of 48 - 7916 active elements (0 deactivated)
09:45:01 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:01 INFO opendrift:2102: 2025-05-28 19:14:31.224386 - step 20 of 48 - 8333 active elements (0 deactivated)
09:45:03 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:04 INFO opendrift:2102: 2025-05-28 19:44:31.224386 - step 21 of 48 - 8750 active elements (0 deactivated)
09:45:05 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:05 INFO opendrift:2102: 2025-05-28 20:14:31.224386 - step 22 of 48 - 9166 active elements (0 deactivated)
09:45:08 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:10 INFO opendrift:2102: 2025-05-28 20:44:31.224386 - step 23 of 48 - 9581 active elements (2 deactivated)
09:45:10 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:11 INFO opendrift:2102: 2025-05-28 21:14:31.224386 - step 24 of 48 - 9995 active elements (5 deactivated)
09:45:13 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:13 INFO opendrift:2102: 2025-05-28 21:44:31.224386 - step 25 of 48 - 9988 active elements (12 deactivated)
09:45:13 WARNING opendrift.readers.basereader.structured:326: Data block from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be not large enough to cover element positions within timestep. Buffer size (8) must be increased. See `Variables.set_buffer_size`.
09:45:14 INFO opendrift:2102: 2025-05-28 22:14:31.224386 - step 26 of 48 - 9979 active elements (21 deactivated)
09:45:16 INFO opendrift:2102: 2025-05-28 22:44:31.224386 - step 27 of 48 - 9976 active elements (24 deactivated)
09:45:17 INFO opendrift:2102: 2025-05-28 23:14:31.224386 - step 28 of 48 - 9972 active elements (28 deactivated)
09:45:19 INFO opendrift:2102: 2025-05-28 23:44:31.224386 - step 29 of 48 - 9968 active elements (32 deactivated)
09:45:19 INFO opendrift:2102: 2025-05-29 00:14:31.224386 - step 30 of 48 - 9965 active elements (35 deactivated)
09:45:22 INFO opendrift:2102: 2025-05-29 00:44:31.224386 - step 31 of 48 - 9961 active elements (39 deactivated)
09:45:22 INFO opendrift:2102: 2025-05-29 01:14:31.224386 - step 32 of 48 - 9950 active elements (50 deactivated)
09:45:24 INFO opendrift:2102: 2025-05-29 01:44:31.224386 - step 33 of 48 - 9937 active elements (63 deactivated)
09:45:25 INFO opendrift:2102: 2025-05-29 02:14:31.224386 - step 34 of 48 - 9926 active elements (74 deactivated)
09:45:27 INFO opendrift:2102: 2025-05-29 02:44:31.224386 - step 35 of 48 - 9912 active elements (88 deactivated)
09:45:27 INFO opendrift:2102: 2025-05-29 03:14:31.224386 - step 36 of 48 - 9896 active elements (104 deactivated)
09:45:30 INFO opendrift:2102: 2025-05-29 03:44:31.224386 - step 37 of 48 - 9882 active elements (118 deactivated)
09:45:30 INFO opendrift:2102: 2025-05-29 04:14:31.224386 - step 38 of 48 - 9861 active elements (139 deactivated)
09:45:33 INFO opendrift:2102: 2025-05-29 04:44:31.224386 - step 39 of 48 - 9851 active elements (149 deactivated)
09:45:33 INFO opendrift:2102: 2025-05-29 05:14:31.224386 - step 40 of 48 - 9832 active elements (168 deactivated)
09:45:36 INFO opendrift:2102: 2025-05-29 05:44:31.224386 - step 41 of 48 - 9810 active elements (190 deactivated)
09:45:36 INFO opendrift:2102: 2025-05-29 06:14:31.224386 - step 42 of 48 - 9795 active elements (205 deactivated)
09:45:39 INFO opendrift:2102: 2025-05-29 06:44:31.224386 - step 43 of 48 - 9778 active elements (222 deactivated)
09:45:39 INFO opendrift:2102: 2025-05-29 07:14:31.224386 - step 44 of 48 - 9766 active elements (234 deactivated)
09:45:41 INFO opendrift:2102: 2025-05-29 07:44:31.224386 - step 45 of 48 - 9753 active elements (247 deactivated)
09:45:42 INFO opendrift:2102: 2025-05-29 08:14:31.224386 - step 46 of 48 - 9742 active elements (258 deactivated)
09:45:44 INFO opendrift:2102: 2025-05-29 08:44:31.224386 - step 47 of 48 - 9736 active elements (264 deactivated)
09:45:44 INFO opendrift:2102: 2025-05-29 09:14:31.224386 - step 48 of 48 - 9723 active elements (277 deactivated)
Print and plot results
print(o)
===========================
--------------------
Reader performance:
--------------------
global_landmask
0:00:01.5 total
0:00:00.0 preparing
0:00:01.5 reading
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
0:00:56.4 total
0:00:00.0 preparing
0:00:55.3 reading
0:00:02.6 interpolation
0:00:00.0 interpolation_time
0:00:01.1 rotating vectors
0:00:00.0 masking
--------------------
Performance:
1:16.1 total time
4.0 configuration
0.1 preparing main loop
0.0 moving elements to ocean
1:12.0 main loop
7.9 updating elements
0.0 oil weathering
0.0 updating viscosities
0.0 updating densities
0.0 emulsification
0.0 dispersion
7.2 vertical mixing
0.0 cleaning up
--------------------
===========================
Model: OpenOil (OpenDrift version 1.14.2)
9710 active Oil particles (290 deactivated, 0 scheduled)
-------------------
Environment variables:
-----
land_binary_mask
1) global_landmask
-----
sea_floor_depth_below_sea_level
sea_surface_height
sea_water_salinity
sea_water_temperature
upward_sea_water_velocity
x_sea_water_velocity
x_wind
y_sea_water_velocity
y_wind
1) https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
-----
Readers not added for the following variables:
ocean_mixed_layer_thickness
ocean_vertical_diffusivity
sea_ice_area_fraction
sea_ice_x_velocity
sea_ice_y_velocity
sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment
sea_surface_wave_period_at_variance_spectral_density_maximum
sea_surface_wave_significant_height
sea_surface_wave_stokes_drift_x_velocity
sea_surface_wave_stokes_drift_y_velocity
Discarded readers:
Time:
Start: 2025-05-28 09:44:31.224386 UTC
Present: 2025-05-29 09:44:31.224386 UTC
Calculation steps: 48 * 0:30:00 - total time: 1 day, 0:00:00
Output steps: 25 * 1:00:00
===========================
Add text label on the map
text = [{'s': 'Senja', 'x': 17.3, 'y': 69.3, 'fontsize': 20, 'color': 'g',
'backgroundcolor': 'white', 'bbox': dict(facecolor='white', alpha=0.8), 'zorder': 1000}]
o.animation(fast=False, ocean_color='skyblue', land_color='burlywood', text=text)
09:46:07 INFO opendrift:4609: Saving animation to /root/project/docs/source/gallery/animations/example_cone_0.gif...
09:46:29 INFO opendrift:3062: Time to make animation: 0:00:41.547630
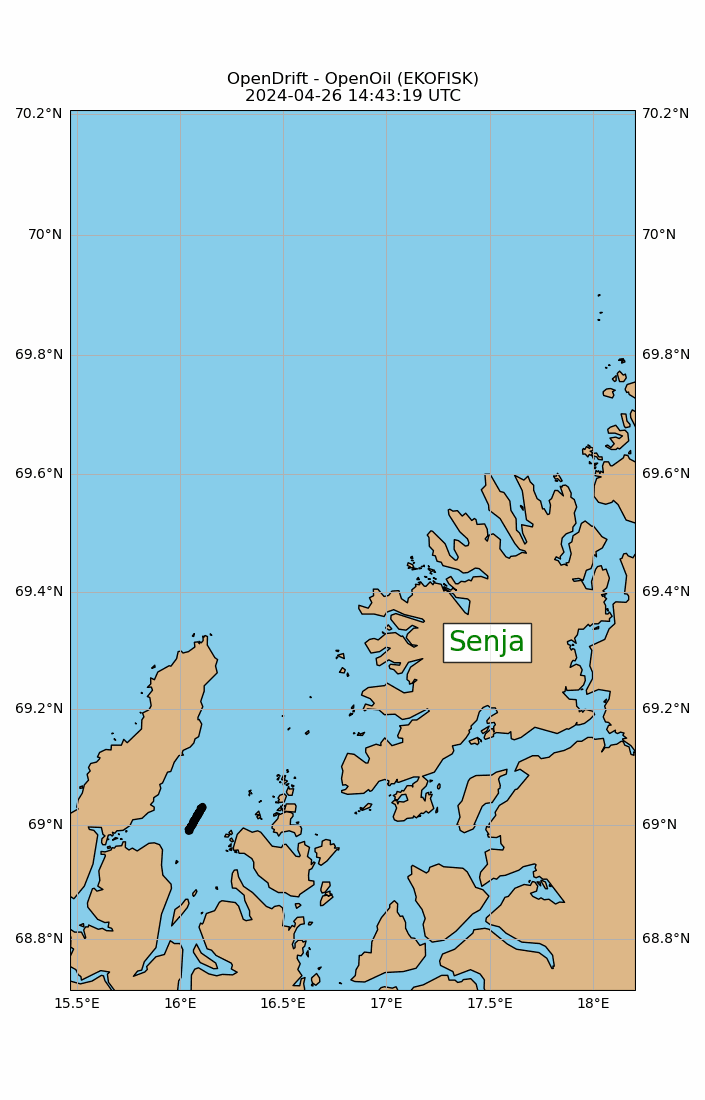
o.plot(fast=True, ocean_color='skyblue', land_color='dimgray', text=text)
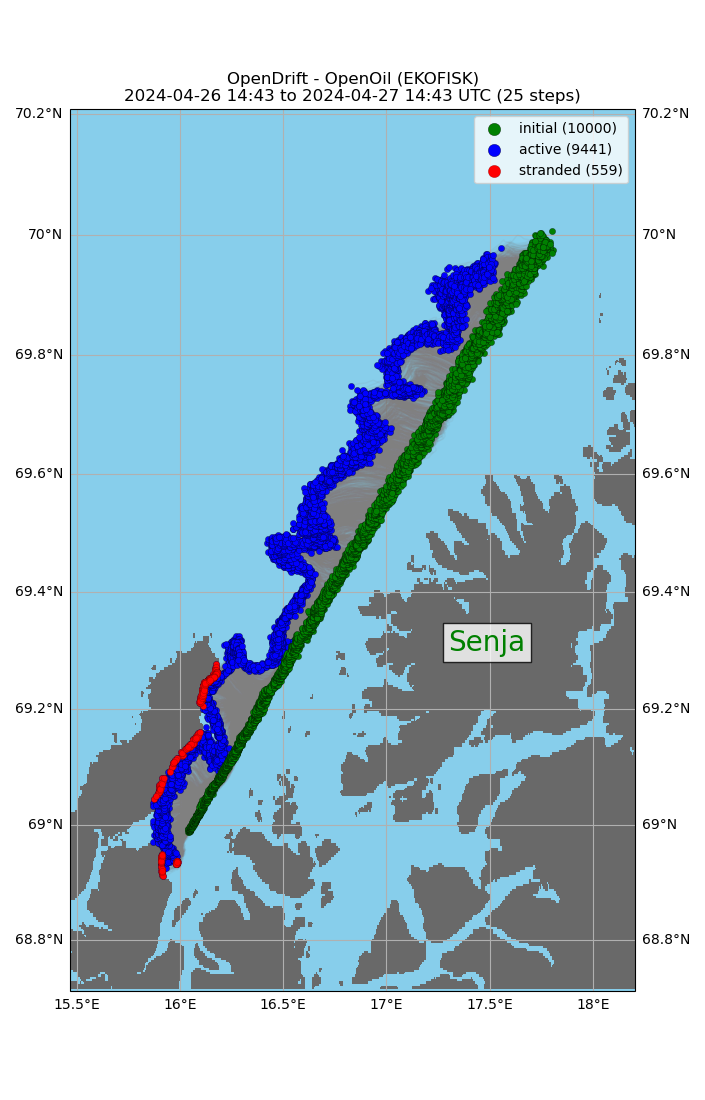
09:46:29 WARNING opendrift:2459: Plotting fast. This will make your plots less accurate.
(<GeoAxes: title={'center': 'OpenDrift - OpenOil (EKOFISK)\n2025-05-28 09:44 to 2025-05-29 09:44 UTC (25 steps)'}>, <Figure size 793.295x1100 with 1 Axes>)
Total running time of the script: (2 minutes 32.410 seconds)