Note
Go to the end to download the full example code.
Sediment drift with resuspension
from datetime import timedelta, datetime
from opendrift.readers import reader_oscillating
from opendrift.models.sedimentdrift import SedimentDrift
Constructing an artificial current field where x- and y-components are oscilating with different amplitude and period
reader_oscx = reader_oscillating.Reader('x_sea_water_velocity',
amplitude=0.6, zero_time=datetime.utcnow())
reader_oscy = reader_oscillating.Reader('y_sea_water_velocity',
amplitude=.3, period_seconds=3600*5, zero_time=datetime.utcnow())
o = SedimentDrift(loglevel=50) # 0 for debug output
if True:
o.add_reader([reader_oscx, reader_oscy])
o.set_config('environment:fallback:y_wind', -6)
o.set_config('environment:fallback:x_wind', -3)
o.set_config('environment:fallback:sea_floor_depth_below_sea_level', 30) # 100m depth
else: # Using live data from Thredds instead of oscillating currents
o.add_readers_from_list([
'https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be'])
/root/project/examples/example_sediments_resuspension.py:14: DeprecationWarning: datetime.datetime.utcnow() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.now(datetime.UTC).
amplitude=0.6, zero_time=datetime.utcnow())
/root/project/examples/example_sediments_resuspension.py:16: DeprecationWarning: datetime.datetime.utcnow() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.now(datetime.UTC).
amplitude=.3, period_seconds=3600*5, zero_time=datetime.utcnow())
Set threshold for bottom resuspension
o.set_config('vertical_mixing:resuspension_threshold', .5)
# Adding some horizontal and vertical diffusion
o.set_config('drift:current_uncertainty', 0.1)
o.set_config('drift:wind_uncertainty', 1)
o.set_config('vertical_mixing:diffusivitymodel', 'windspeed_Large1994')
#o.set_config('vertical_mixing:diffusivitymodel', 'environment')
Seeding sediments
o.seed_elements(lon=4.65, lat=60, number=10000,
time=[datetime.utcnow(), datetime.utcnow()+timedelta(hours=6)],
terminal_velocity=-.01) # 1 cm/s settling speed
o.run(time_step=1800, time_step_output=1800, duration=timedelta(hours=72))
/root/project/examples/example_sediments_resuspension.py:42: DeprecationWarning: datetime.datetime.utcnow() is deprecated and scheduled for removal in a future version. Use timezone-aware objects to represent datetimes in UTC: datetime.datetime.now(datetime.UTC).
time=[datetime.utcnow(), datetime.utcnow()+timedelta(hours=6)],
<xarray.Dataset> Size: 145MB Dimensions: ( trajectory: 10000, time: 145) Coordinates: * trajectory (trajectory) int64 80kB ... * time (time) datetime64[ns] 1kB ... Data variables: (12/25) status (trajectory, time) float32 6MB ... moving (trajectory, time) float32 6MB ... age_seconds (trajectory, time) float32 6MB ... origin_marker (trajectory, time) float32 6MB ... lon (trajectory, time) float32 6MB ... lat (trajectory, time) float32 6MB ... ... ... sea_surface_wave_period_at_variance_spectral_density_maximum (trajectory, time) float32 6MB ... sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment (trajectory, time) float32 6MB ... land_binary_mask (trajectory, time) float32 6MB ... ocean_vertical_diffusivity (trajectory, time) float32 6MB ... ocean_mixed_layer_thickness (trajectory, time) float32 6MB ... sea_floor_depth_below_sea_level (trajectory, time) float32 6MB ... Attributes: (12/124) Conventions: ... standard_name_vocabulary: ... featureType: ... title: ... summary: ... keywords: ... ... ... geospatial_lon_units: ... geospatial_lon_resolution: ... runtime: ... geospatial_vertical_min: ... geospatial_vertical_max: ... geospatial_vertical_positive: ...
xarray.Dataset
- trajectory: 10000
- time: 145
- trajectory(trajectory)int640 1 2 3 4 ... 9996 9997 9998 9999
- cf_role :
- trajectory_id
- dtype :
- <class 'numpy.int32'>
array([ 0, 1, 2, ..., 9997, 9998, 9999], shape=(10000,))
- time(time)datetime64[ns]2025-05-16T15:26:28.190006 ... 2...
- standard_name :
- time
- long_name :
- time
- dtype :
- <class 'numpy.float64'>
array(['2025-05-16T15:26:28.190006000', '2025-05-16T15:56:28.190006000', '2025-05-16T16:26:28.190006000', '2025-05-16T16:56:28.190006000', '2025-05-16T17:26:28.190006000', '2025-05-16T17:56:28.190006000', '2025-05-16T18:26:28.190006000', '2025-05-16T18:56:28.190006000', '2025-05-16T19:26:28.190006000', '2025-05-16T19:56:28.190006000', '2025-05-16T20:26:28.190006000', '2025-05-16T20:56:28.190006000', '2025-05-16T21:26:28.190006000', '2025-05-16T21:56:28.190006000', '2025-05-16T22:26:28.190006000', '2025-05-16T22:56:28.190006000', '2025-05-16T23:26:28.190006000', '2025-05-16T23:56:28.190006000', '2025-05-17T00:26:28.190006000', '2025-05-17T00:56:28.190006000', '2025-05-17T01:26:28.190006000', '2025-05-17T01:56:28.190006000', '2025-05-17T02:26:28.190006000', '2025-05-17T02:56:28.190006000', '2025-05-17T03:26:28.190006000', '2025-05-17T03:56:28.190006000', '2025-05-17T04:26:28.190006000', '2025-05-17T04:56:28.190006000', '2025-05-17T05:26:28.190006000', '2025-05-17T05:56:28.190006000', '2025-05-17T06:26:28.190006000', '2025-05-17T06:56:28.190006000', '2025-05-17T07:26:28.190006000', '2025-05-17T07:56:28.190006000', '2025-05-17T08:26:28.190006000', '2025-05-17T08:56:28.190006000', '2025-05-17T09:26:28.190006000', '2025-05-17T09:56:28.190006000', '2025-05-17T10:26:28.190006000', '2025-05-17T10:56:28.190006000', '2025-05-17T11:26:28.190006000', '2025-05-17T11:56:28.190006000', '2025-05-17T12:26:28.190006000', '2025-05-17T12:56:28.190006000', '2025-05-17T13:26:28.190006000', '2025-05-17T13:56:28.190006000', '2025-05-17T14:26:28.190006000', '2025-05-17T14:56:28.190006000', '2025-05-17T15:26:28.190006000', '2025-05-17T15:56:28.190006000', '2025-05-17T16:26:28.190006000', '2025-05-17T16:56:28.190006000', '2025-05-17T17:26:28.190006000', '2025-05-17T17:56:28.190006000', '2025-05-17T18:26:28.190006000', '2025-05-17T18:56:28.190006000', '2025-05-17T19:26:28.190006000', '2025-05-17T19:56:28.190006000', '2025-05-17T20:26:28.190006000', '2025-05-17T20:56:28.190006000', '2025-05-17T21:26:28.190006000', '2025-05-17T21:56:28.190006000', '2025-05-17T22:26:28.190006000', '2025-05-17T22:56:28.190006000', '2025-05-17T23:26:28.190006000', '2025-05-17T23:56:28.190006000', '2025-05-18T00:26:28.190006000', '2025-05-18T00:56:28.190006000', '2025-05-18T01:26:28.190006000', '2025-05-18T01:56:28.190006000', '2025-05-18T02:26:28.190006000', '2025-05-18T02:56:28.190006000', '2025-05-18T03:26:28.190006000', '2025-05-18T03:56:28.190006000', '2025-05-18T04:26:28.190006000', '2025-05-18T04:56:28.190006000', '2025-05-18T05:26:28.190006000', '2025-05-18T05:56:28.190006000', '2025-05-18T06:26:28.190006000', '2025-05-18T06:56:28.190006000', '2025-05-18T07:26:28.190006000', '2025-05-18T07:56:28.190006000', '2025-05-18T08:26:28.190006000', '2025-05-18T08:56:28.190006000', '2025-05-18T09:26:28.190006000', '2025-05-18T09:56:28.190006000', '2025-05-18T10:26:28.190006000', '2025-05-18T10:56:28.190006000', '2025-05-18T11:26:28.190006000', '2025-05-18T11:56:28.190006000', '2025-05-18T12:26:28.190006000', '2025-05-18T12:56:28.190006000', '2025-05-18T13:26:28.190006000', '2025-05-18T13:56:28.190006000', '2025-05-18T14:26:28.190006000', '2025-05-18T14:56:28.190006000', '2025-05-18T15:26:28.190006000', '2025-05-18T15:56:28.190006000', '2025-05-18T16:26:28.190006000', '2025-05-18T16:56:28.190006000', '2025-05-18T17:26:28.190006000', '2025-05-18T17:56:28.190006000', '2025-05-18T18:26:28.190006000', '2025-05-18T18:56:28.190006000', '2025-05-18T19:26:28.190006000', '2025-05-18T19:56:28.190006000', '2025-05-18T20:26:28.190006000', '2025-05-18T20:56:28.190006000', '2025-05-18T21:26:28.190006000', '2025-05-18T21:56:28.190006000', '2025-05-18T22:26:28.190006000', '2025-05-18T22:56:28.190006000', '2025-05-18T23:26:28.190006000', '2025-05-18T23:56:28.190006000', '2025-05-19T00:26:28.190006000', '2025-05-19T00:56:28.190006000', '2025-05-19T01:26:28.190006000', '2025-05-19T01:56:28.190006000', '2025-05-19T02:26:28.190006000', '2025-05-19T02:56:28.190006000', '2025-05-19T03:26:28.190006000', '2025-05-19T03:56:28.190006000', '2025-05-19T04:26:28.190006000', '2025-05-19T04:56:28.190006000', '2025-05-19T05:26:28.190006000', '2025-05-19T05:56:28.190006000', '2025-05-19T06:26:28.190006000', '2025-05-19T06:56:28.190006000', '2025-05-19T07:26:28.190006000', '2025-05-19T07:56:28.190006000', '2025-05-19T08:26:28.190006000', '2025-05-19T08:56:28.190006000', '2025-05-19T09:26:28.190006000', '2025-05-19T09:56:28.190006000', '2025-05-19T10:26:28.190006000', '2025-05-19T10:56:28.190006000', '2025-05-19T11:26:28.190006000', '2025-05-19T11:56:28.190006000', '2025-05-19T12:26:28.190006000', '2025-05-19T12:56:28.190006000', '2025-05-19T13:26:28.190006000', '2025-05-19T13:56:28.190006000', '2025-05-19T14:26:28.190006000', '2025-05-19T14:56:28.190006000', '2025-05-19T15:26:28.190006000'], dtype='datetime64[ns]')
- status(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- valid_range :
- [0 0]
- flag_values :
- [0]
- flag_meanings :
- active
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- moving(trajectory, time)float321.0 1.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- minval :
- 0
- maxval :
- 1
array([[ 1., 1., 0., ..., 0., 0., 1.], [ 1., 0., 0., ..., 0., 0., 0.], [ 1., 1., 1., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- age_seconds(trajectory, time)float320.0 1.8e+03 ... 2.376e+05 2.394e+05
- dtype :
- <class 'numpy.float32'>
- units :
- s
- minval :
- 0.0
- maxval :
- 259200.0
array([[ 0., 1800., 3600., ..., 255600., 257400., 259200.], [ 0., 1800., 3600., ..., 255600., 257400., 259200.], [ 0., 1800., 3600., ..., 255600., 257400., 259200.], ..., [ nan, nan, nan, ..., 235800., 237600., 239400.], [ nan, nan, nan, ..., 235800., 237600., 239400.], [ nan, nan, nan, ..., 235800., 237600., 239400.]], shape=(10000, 145), dtype=float32)
- origin_marker(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.int32'>
- unit :
- description :
- An integer kept constant during the simulation. Different values may be used for different seedings, to separate elements during analysis. With GUI, only a single seeding is possible.
- flag_values :
- [0]
- flag_meanings :
- Seed_0
- minval :
- 0
- maxval :
- 0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- lon(trajectory, time)float324.65 4.654 4.655 ... 4.98 4.98 4.98
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_east
- standard_name :
- longitude
- long_name :
- longitude
- axis :
- X
- minval :
- 4.4029746
- maxval :
- 5.258207
array([[4.65 , 4.6537676, 4.654725 , ..., 5.06053 , 5.06053 , 5.06053 ], [4.65 , 4.649303 , 4.649303 , ..., 5.0203714, 5.0203714, 5.0203714], [4.65 , 4.6506047, 4.648504 , ..., 5.1139092, 5.1139092, 5.1139092], ..., [ nan, nan, nan, ..., 4.9113955, 4.9113955, 4.9113955], [ nan, nan, nan, ..., 4.99289 , 4.99289 , 4.99289 ], [ nan, nan, nan, ..., 4.979738 , 4.979738 , 4.979738 ]], shape=(10000, 145), dtype=float32)
- lat(trajectory, time)float3260.0 60.0 60.0 ... 59.98 59.98
- dtype :
- <class 'numpy.float32'>
- units :
- degrees_north
- standard_name :
- latitude
- long_name :
- latitude
- axis :
- Y
- minval :
- 59.90722
- maxval :
- 60.09805
array([[60. , 59.99876 , 59.999546, ..., 60.00605 , 60.00605 , 60.00605 ], [60. , 59.99921 , 59.99921 , ..., 59.979485, 59.979485, 59.979485], [60. , 59.99663 , 59.99691 , ..., 59.96593 , 59.96593 , 59.96593 ], ..., [ nan, nan, nan, ..., 59.983097, 59.983097, 59.983097], [ nan, nan, nan, ..., 59.971367, 59.971367, 59.971367], [ nan, nan, nan, ..., 59.983715, 59.983715, 59.983715]], shape=(10000, 145), dtype=float32)
- z(trajectory, time)float320.0 -18.3 -30.37 ... -30.09 -30.09
- dtype :
- <class 'numpy.float32'>
- units :
- m
- standard_name :
- z
- long_name :
- vertical position
- axis :
- Z
- positive :
- up
- minval :
- -30.599998
- maxval :
- 0.0
array([[ 0. , -18.304564, -30.371801, ..., -30.323664, -30.323664, -30.313663], [ 0. , -30.42179 , -30.42179 , ..., -30.31842 , -30.31842 , -30.31842 ], [ 0. , -18.376421, -27.266197, ..., -30.202114, -30.202114, -30.202114], ..., [ nan, nan, nan, ..., -30.1376 , -30.1376 , -30.1376 ], [ nan, nan, nan, ..., -30.272543, -30.272543, -30.272543], [ nan, nan, nan, ..., -30.09179 , -30.09179 , -30.09179 ]], shape=(10000, 145), dtype=float32)
- wind_drift_factor(trajectory, time)float320.02 0.02 0.02 ... 0.02 0.02 0.02
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements at surface are moved with this fraction of the vind vector, in addition to currents and Stokes drift
- minval :
- 0.02
- maxval :
- 0.02
array([[0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], ..., [ nan, nan, nan, ..., 0.02, 0.02, 0.02], [ nan, nan, nan, ..., 0.02, 0.02, 0.02], [ nan, nan, nan, ..., 0.02, 0.02, 0.02]], shape=(10000, 145), dtype=float32)
- current_drift_factor(trajectory, time)float321.0 1.0 1.0 1.0 ... 1.0 1.0 1.0 1.0
- dtype :
- <class 'numpy.float32'>
- units :
- 1
- description :
- Elements are moved with this fraction of the current vector, in addition to currents and Stokes drift
- minval :
- 1.0
- maxval :
- 1.0
array([[ 1., 1., 1., ..., 1., 1., 1.], [ 1., 1., 1., ..., 1., 1., 1.], [ 1., 1., 1., ..., 1., 1., 1.], ..., [nan, nan, nan, ..., 1., 1., 1.], [nan, nan, nan, ..., 1., 1., 1.], [nan, nan, nan, ..., 1., 1., 1.]], shape=(10000, 145), dtype=float32)
- terminal_velocity(trajectory, time)float32-0.01 -0.01 -0.01 ... -0.01 -0.01
- dtype :
- <class 'numpy.float32'>
- units :
- m/s
- minval :
- -0.01
- maxval :
- -0.01
array([[-0.01, -0.01, -0.01, ..., -0.01, -0.01, -0.01], [-0.01, -0.01, -0.01, ..., -0.01, -0.01, -0.01], [-0.01, -0.01, -0.01, ..., -0.01, -0.01, -0.01], ..., [ nan, nan, nan, ..., -0.01, -0.01, -0.01], [ nan, nan, nan, ..., -0.01, -0.01, -0.01], [ nan, nan, nan, ..., -0.01, -0.01, -0.01]], shape=(10000, 145), dtype=float32)
- settled(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- dtype :
- <class 'numpy.uint8'>
- units :
- 1
- minval :
- 0
- maxval :
- 0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- x_sea_water_velocity(trajectory, time)float320.1764 0.02968 ... -0.08856
- minval :
- -1.0181197
- maxval :
- 1.0368029
array([[ 0.17641065, 0.02968033, 0.10041013, ..., 0.31032115, 0.09746931, 0.09746931], [ 0.04002114, 0.08297281, 0.11504182, ..., 0.21695563, -0.02193519, -0.02193519], [ 0.09787922, -0.06513286, 0.00946982, ..., 0.10161462, 0.08584288, 0.08584288], ..., [ nan, nan, nan, ..., -0.01610638, 0.07293681, 0.07293681], [ nan, nan, nan, ..., 0.14972326, 0.13996379, 0.13996379], [ nan, nan, nan, ..., 0.05969651, -0.08855508, -0.08855508]], shape=(10000, 145), dtype=float32)
- y_sea_water_velocity(trajectory, time)float320.00517 0.04866 ... 0.3579 0.3579
- minval :
- -0.7497906
- maxval :
- 0.71832526
array([[ 0.00517046, 0.04866335, 0.1517693 , ..., 0.21628278, 0.5807201 , 0.5807201 ], [ 0.08674064, -0.07284551, 0.23437034, ..., 0.24177785, 0.26389685, 0.26389685], [-0.08481908, 0.01732874, 0.15258786, ..., 0.19646394, 0.3284302 , 0.3284302 ], ..., [ nan, nan, nan, ..., 0.18403158, 0.37294105, 0.37294105], [ nan, nan, nan, ..., 0.23300138, 0.1592499 , 0.1592499 ], [ nan, nan, nan, ..., 0.21412936, 0.3578778 , 0.3578778 ]], shape=(10000, 145), dtype=float32)
- sea_surface_height(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- upward_sea_water_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- x_wind(trajectory, time)float32-2.981 -4.244 ... -3.338 -3.338
- minval :
- -8.002298
- maxval :
- 1.8089983
array([[-2.981078 , -4.243658 , -2.8865137, ..., -4.9380593, -3.34425 , -3.34425 ], [-3.082034 , -2.827815 , -2.0777311, ..., -3.5350225, -2.32015 , -2.32015 ], [-3.957158 , -2.3757708, -1.5997821, ..., -2.5414686, -4.458367 , -4.458367 ], ..., [ nan, nan, nan, ..., -2.7222812, -1.0365078, -1.0365078], [ nan, nan, nan, ..., -2.0151415, -3.3912978, -3.3912978], [ nan, nan, nan, ..., -2.4119408, -3.3377228, -3.3377228]], shape=(10000, 145), dtype=float32)
- y_wind(trajectory, time)float32-4.097 -5.883 ... -5.671 -5.671
- minval :
- -10.789874
- maxval :
- -1.0925639
array([[-4.0973816, -5.883371 , -6.234717 , ..., -7.2351575, -5.630373 , -5.630373 ], [-6.7756643, -6.090957 , -4.563066 , ..., -6.344755 , -7.9961433, -7.9961433], [-6.18809 , -5.2804637, -5.106999 , ..., -4.6859593, -7.3998036, -7.3998036], ..., [ nan, nan, nan, ..., -5.7076945, -6.708225 , -6.708225 ], [ nan, nan, nan, ..., -5.610828 , -5.963494 , -5.963494 ], [ nan, nan, nan, ..., -6.3788004, -5.67101 , -5.67101 ]], shape=(10000, 145), dtype=float32)
- sea_surface_wave_stokes_drift_x_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- sea_surface_wave_stokes_drift_y_velocity(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- sea_surface_wave_period_at_variance_spectral_density_maximum(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment(trajectory, time)float324.33 6.198 5.871 ... 5.623 5.623
- minval :
- 1.5306671
- maxval :
- 9.854761
array([[4.329676 , 6.1984577, 5.870633 , ..., 7.484886 , 5.5956483, 5.5956483], [6.3604164, 5.738093 , 4.284174 , ..., 6.2060866, 7.114278 , 7.114278 ], [6.276234 , 4.947642 , 4.5728745, ..., 4.555 , 7.3818607, 7.3818607], ..., [ nan, nan, nan, ..., 5.403376 , 5.8 , 5.8 ], [ nan, nan, nan, ..., 5.09412 , 5.861952 , 5.861952 ], [ nan, nan, nan, ..., 5.8271236, 5.622699 , 5.622699 ]], shape=(10000, 145), dtype=float32)
- land_binary_mask(trajectory, time)float320.0 0.0 0.0 0.0 ... 0.0 0.0 0.0 0.0
- minval :
- 0.0
- maxval :
- 0.0
array([[ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], [ 0., 0., 0., ..., 0., 0., 0.], ..., [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.], [nan, nan, nan, ..., 0., 0., 0.]], shape=(10000, 145), dtype=float32)
- ocean_vertical_diffusivity(trajectory, time)float320.02 0.02 0.02 ... 0.02 0.02 0.02
- minval :
- 0.02
- maxval :
- 0.02
array([[0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], [0.02, 0.02, 0.02, ..., 0.02, 0.02, 0.02], ..., [ nan, nan, nan, ..., 0.02, 0.02, 0.02], [ nan, nan, nan, ..., 0.02, 0.02, 0.02], [ nan, nan, nan, ..., 0.02, 0.02, 0.02]], shape=(10000, 145), dtype=float32)
- ocean_mixed_layer_thickness(trajectory, time)float3250.0 50.0 50.0 ... 50.0 50.0 50.0
- minval :
- 50.0
- maxval :
- 50.0
array([[50., 50., 50., ..., 50., 50., 50.], [50., 50., 50., ..., 50., 50., 50.], [50., 50., 50., ..., 50., 50., 50.], ..., [nan, nan, nan, ..., 50., 50., 50.], [nan, nan, nan, ..., 50., 50., 50.], [nan, nan, nan, ..., 50., 50., 50.]], shape=(10000, 145), dtype=float32)
- sea_floor_depth_below_sea_level(trajectory, time)float3230.0 30.0 30.0 ... 30.0 30.0 30.0
- minval :
- 30.0
- maxval :
- 30.0
array([[30., 30., 30., ..., 30., 30., 30.], [30., 30., 30., ..., 30., 30., 30.], [30., 30., 30., ..., 30., 30., 30.], ..., [nan, nan, nan, ..., 30., 30., 30.], [nan, nan, nan, ..., 30., 30., 30.], [nan, nan, nan, ..., 30., 30., 30.]], shape=(10000, 145), dtype=float32)
- trajectoryPandasIndex
PandasIndex(Index([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, ... 9990, 9991, 9992, 9993, 9994, 9995, 9996, 9997, 9998, 9999], dtype='int64', name='trajectory', length=10000))
- timePandasIndex
PandasIndex(DatetimeIndex(['2025-05-16 15:26:28.190006', '2025-05-16 15:56:28.190006', '2025-05-16 16:26:28.190006', '2025-05-16 16:56:28.190006', '2025-05-16 17:26:28.190006', '2025-05-16 17:56:28.190006', '2025-05-16 18:26:28.190006', '2025-05-16 18:56:28.190006', '2025-05-16 19:26:28.190006', '2025-05-16 19:56:28.190006', ... '2025-05-19 10:56:28.190006', '2025-05-19 11:26:28.190006', '2025-05-19 11:56:28.190006', '2025-05-19 12:26:28.190006', '2025-05-19 12:56:28.190006', '2025-05-19 13:26:28.190006', '2025-05-19 13:56:28.190006', '2025-05-19 14:26:28.190006', '2025-05-19 14:56:28.190006', '2025-05-19 15:26:28.190006'], dtype='datetime64[ns]', name='time', length=145, freq='30min'))
- Conventions :
- CF-1.11, ACDD-1.3
- standard_name_vocabulary :
- CF Standard Name Table v85
- featureType :
- trajectory
- title :
- OpenDrift trajectory simulation
- summary :
- Output from simulation with OpenDrift framework
- keywords :
- trajectory, drift, lagrangian, simulation
- history :
- Created 2025-05-16 15:26:32.810605
- date_created :
- 2025-05-16T15:26:32.810624
- source :
- Output from simulation with OpenDrift
- model_url :
- https://github.com/OpenDrift/opendrift
- opendrift_class :
- SedimentDrift
- opendrift_module :
- opendrift.models.sedimentdrift
- readers :
- odict_keys(['oscillating_reader', 'oscillating_reader_0', 'global_landmask'])
- time_coverage_start :
- 2025-05-16 15:26:28.190006
- time_step_calculation :
- 0:30:00
- time_step_output :
- 0:30:00
- config_environment:constant:x_sea_water_velocity :
- None
- config_environment:fallback:x_sea_water_velocity :
- 0
- config_environment:constant:y_sea_water_velocity :
- None
- config_environment:fallback:y_sea_water_velocity :
- 0
- config_environment:constant:sea_surface_height :
- None
- config_environment:fallback:sea_surface_height :
- 0
- config_environment:constant:upward_sea_water_velocity :
- None
- config_environment:fallback:upward_sea_water_velocity :
- 0
- config_environment:constant:x_wind :
- None
- config_environment:fallback:x_wind :
- -3.0
- config_environment:constant:y_wind :
- None
- config_environment:fallback:y_wind :
- -6.0
- config_environment:constant:sea_surface_wave_stokes_drift_x_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_x_velocity :
- 0
- config_environment:constant:sea_surface_wave_stokes_drift_y_velocity :
- None
- config_environment:fallback:sea_surface_wave_stokes_drift_y_velocity :
- 0
- config_environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum :
- None
- config_environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- config_environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- None
- config_environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- config_environment:constant:land_binary_mask :
- None
- config_environment:fallback:land_binary_mask :
- None
- config_environment:constant:ocean_vertical_diffusivity :
- None
- config_environment:fallback:ocean_vertical_diffusivity :
- 0.02
- config_environment:constant:ocean_mixed_layer_thickness :
- None
- config_environment:fallback:ocean_mixed_layer_thickness :
- 50
- config_environment:constant:sea_floor_depth_below_sea_level :
- None
- config_environment:fallback:sea_floor_depth_below_sea_level :
- 30.0
- config_general:use_auto_landmask :
- True
- config_drift:current_uncertainty :
- 0.1
- config_drift:current_uncertainty_uniform :
- 0
- config_drift:max_speed :
- 2.0
- config_readers:max_number_of_fails :
- 1
- config_general:simulation_name :
- config_general:coastline_action :
- previous
- config_general:coastline_approximation_precision :
- 0.001
- config_general:time_step_minutes :
- 60
- config_general:time_step_output_minutes :
- None
- config_seed:ocean_only :
- True
- config_seed:number :
- 1
- config_drift:max_age_seconds :
- None
- config_drift:advection_scheme :
- euler
- config_drift:horizontal_diffusivity :
- 0
- config_drift:profiles_depth :
- 50
- config_drift:wind_uncertainty :
- 1.0
- config_drift:relative_wind :
- False
- config_drift:deactivate_north_of :
- None
- config_drift:deactivate_south_of :
- None
- config_drift:deactivate_east_of :
- None
- config_drift:deactivate_west_of :
- None
- config_seed:origin_marker :
- 0
- config_seed:z :
- 0
- config_seed:wind_drift_factor :
- 0.02
- config_seed:current_drift_factor :
- 1
- config_seed:terminal_velocity :
- -0.001
- config_seed:settled :
- 0
- config_drift:vertical_advection :
- True
- config_drift:vertical_advection_at_surface :
- True
- config_drift:vertical_mixing :
- True
- config_drift:vertical_mixing_at_surface :
- True
- config_vertical_mixing:timestep :
- 60
- config_vertical_mixing:diffusivitymodel :
- windspeed_Large1994
- config_vertical_mixing:background_diffusivity :
- 1.2e-05
- config_vertical_mixing:TSprofiles :
- False
- config_drift:wind_drift_depth :
- 0.1
- config_drift:stokes_drift :
- True
- config_drift:stokes_drift_profile :
- Phillips
- config_drift:use_tabularised_stokes_drift :
- False
- config_drift:tabularised_stokes_drift_fetch :
- 25000
- config_general:seafloor_action :
- lift_to_seafloor
- config_drift:truncate_ocean_model_below_m :
- None
- config_seed:seafloor :
- False
- config_vertical_mixing:resuspension_threshold :
- 0.5
- opendrift_version :
- 1.14.0
- seed_geojson :
- {"features": [], "type": "FeatureCollection"}
- simulation_time :
- 2025-05-16 15:26:32.969369
- reader_x_sea_water_velocity :
- ['oscillating_reader']
- reader_y_sea_water_velocity :
- ['oscillating_reader_0']
- reader_sea_surface_height :
- 0
- reader_upward_sea_water_velocity :
- 0
- reader_x_wind :
- -3.0
- reader_y_wind :
- -6.0
- reader_sea_surface_wave_stokes_drift_x_velocity :
- 0
- reader_sea_surface_wave_stokes_drift_y_velocity :
- 0
- reader_sea_surface_wave_period_at_variance_spectral_density_maximum :
- 0
- reader_sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment :
- 0
- reader_land_binary_mask :
- ['global_landmask']
- reader_ocean_vertical_diffusivity :
- 0.02
- reader_ocean_mixed_layer_thickness :
- 50
- reader_sea_floor_depth_below_sea_level :
- 30.0
- time_coverage_end :
- 2025-05-19 15:26:28.190006
- time_coverage_duration :
- P3DT0H0M0S
- time_coverage_resolution :
- P0DT0H30M0S
- performance :
- -------------------- Reader performance: -------------------- oscillating_reader 0:00:00.0 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 masking -------------------- oscillating_reader_0 0:00:00.0 total 0:00:00.0 preparing 0:00:00.0 reading 0:00:00.0 masking -------------------- global_landmask 0:00:03.5 total 0:00:00.0 preparing 0:00:03.4 reading 0:00:00.0 masking -------------------- Performance: 35.0 total time 4.5 configuration 0.3 preparing main loop 0.0 moving elements to ocean 30.1 main loop 15.8 updating elements 14.8 vertical mixing 0.0 cleaning up --------------------
- geospatial_bounds_crs :
- EPSG:4326
- geospatial_bounds_vertical_crs :
- EPSG:5831
- geospatial_lat_min :
- 59.90722
- geospatial_lat_max :
- 60.09805
- geospatial_lat_units :
- degrees_north
- geospatial_lat_resolution :
- point
- geospatial_lon_min :
- 4.4029746
- geospatial_lon_max :
- 5.258207
- geospatial_lon_units :
- degrees_east
- geospatial_lon_resolution :
- point
- runtime :
- 0:00:35.034362
- geospatial_vertical_min :
- -30.599998
- geospatial_vertical_max :
- 0.0
- geospatial_vertical_positive :
- up
Plotting the depth vs time
o.plot_property('z')
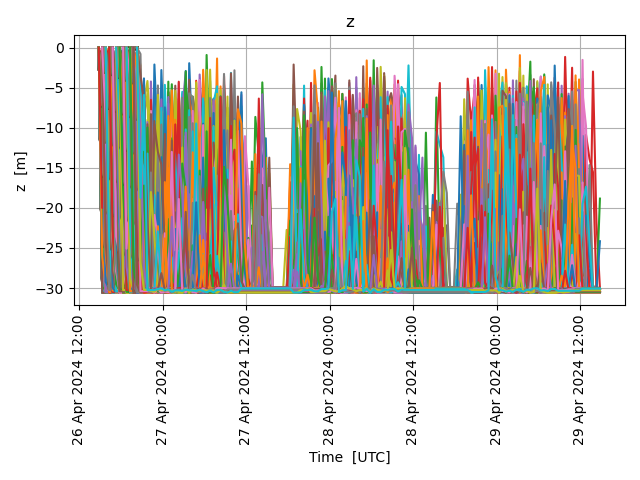
Animate sediment particles
o.animation(color='moving', colorbar=False, legend=['Sedimented', 'Moving'], fast=True, buffer=.01)
#o.animation_profile()
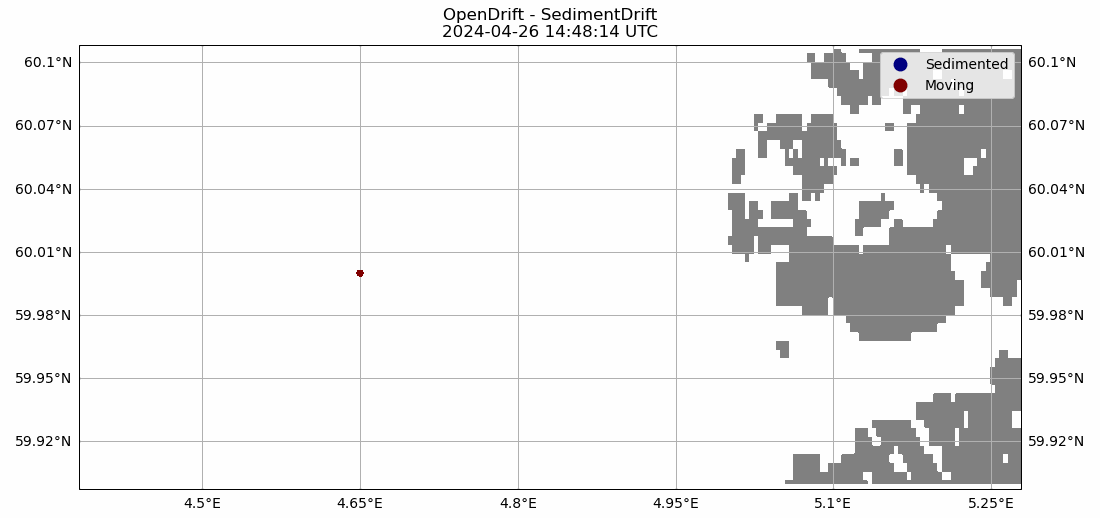
Total running time of the script: (3 minutes 23.279 seconds)