Note
Go to the end to download the full example code.
Analysing huge output files
import os
from datetime import datetime, timedelta
import opendrift
from opendrift.models.oceandrift import OceanDrift
from opendrift.readers import reader_oscillating
First make a simulation with two seedings, marked by origin_marker
o = OceanDrift(loglevel=10)
o.set_config('drift:horizontal_diffusivity', 10)
t1 = datetime.now()
t2 = t1 + timedelta(hours=6)
number = 10000
outfile = 'simulation.nc' # Raw simulation output
reader_x = reader_oscillating.Reader('x_sea_water_velocity',
amplitude=1, zero_time=t1)
reader_y = reader_oscillating.Reader('y_sea_water_velocity',
amplitude=1, zero_time=t2)
o.add_reader([reader_x, reader_y])
o.seed_elements(time=t1, lon=4, lat=60, number=number,
origin_marker=0)
o.seed_elements(time=[t1, t2], lon=4.2, lat=60.4, number=number,
origin_marker=1)
o.run(duration=timedelta(hours=24),
time_step=900, time_step_output=1800, outfile=outfile)
18:33:44 DEBUG opendrift.config:168: Adding 18 config items from __init__
18:33:44 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
18:33:44 DEBUG opendrift.config:168: Adding 5 config items from __init__
18:33:44 INFO opendrift:513: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-74-gdbb4afa)
18:33:44 DEBUG opendrift.config:168: Adding 17 config items from oceandrift
18:33:44 DEBUG opendrift.config:178: Overwriting config item seed:z
18:33:44 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
18:33:44 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
18:33:44 DEBUG opendrift.models.basemodel.environment:312: Added reader oscillating_reader
18:33:44 DEBUG opendrift.models.basemodel.environment:312: Added reader oscillating_reader_0
18:33:44 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
18:33:44 DEBUG opendrift.readers.basereader:186: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
18:33:48 DEBUG opendrift.models.basemodel.environment:312: Added reader global_landmask
18:33:48 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_height: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: x_wind: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: y_wind: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: upward_sea_water_velocity: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: ocean_vertical_diffusivity: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_period_at_variance_spectral_density_maximum: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_to_direction: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_swell_wave_significant_height: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_to_direction: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_mean_period: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_surface_wind_wave_significant_height: 0.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: ocean_mixed_layer_thickness: 50.000000
18:33:48 INFO opendrift.models.basemodel.environment:232: sea_floor_depth_below_sea_level: 10000.000000
18:33:48 DEBUG opendrift:100: Changed mode from Mode.Config to Mode.Ready
18:33:48 DEBUG opendrift:100: Changed mode from Mode.Ready to Mode.Run
18:33:48 DEBUG opendrift:1797:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.14.2
Platform: Linux, 6.8.0-1029-aws
4.0 GB memory
36 processors (x86_64)
NumPy version 2.3.0
SciPy version 1.15.2
Matplotlib version 3.10.3
NetCDF4 version 1.7.2
Xarray version 2025.6.1
ADIOS (adios_db) version 1.2.5
Copernicusmarine version 2.1.2
Python version 3.13.5 | packaged by conda-forge | (main, Jun 16 2025, 08:27:50) [GCC 13.3.0]
------------------------------------------------------
18:33:48 INFO opendrift:1825: Skipping environment variable ocean_vertical_diffusivity because of condition ['drift:vertical_mixing', 'is', False]
18:33:48 DEBUG opendrift:1938: Finalizing environment and preparing readers for simulation coverage ([ 0.86752815 58.44324324 7.33247166 61.95675828]) and time (2025-06-30 18:33:44.415110 to 2025-07-01 18:33:44.415110)
18:33:48 DEBUG opendrift.models.basemodel.environment:168: Preparing oscillating_reader for extent [ 0.86752815 58.44324324 7.33247166 61.95675828]
18:33:48 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:168: Preparing oscillating_reader_0 for extent [ 0.86752815 58.44324324 7.33247166 61.95675828]
18:33:48 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:168: Preparing global_landmask for extent [ 0.86752815 58.44324324 7.33247166 61.95675828]
18:33:48 DEBUG opendrift.readers.basereader.variables:555: Nothing more to prepare for global_landmask
18:33:48 DEBUG opendrift:2025: Initial self.result, size Frozen({'trajectory': 20000, 'time': 49})
18:33:48 DEBUG opendrift.export.io_netcdf:24: Initialising output netCDF file {self.outfile_name}
18:33:48 INFO opendrift:889: Using existing reader for land_binary_mask
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 INFO opendrift:918: All points are in ocean
18:33:48 INFO opendrift:2051: Storing previous position of elements for coastline interaction
18:33:48 DEBUG opendrift:846: to be seeded: 20000, already seeded 0
18:33:48 DEBUG opendrift:868: Released 10417 new elements.
18:33:48 DEBUG opendrift:2111: ======================================================================
18:33:48 INFO opendrift:2112: 2025-06-30 18:33:44.415110 - step 1 of 96 - 10417 active elements (0 deactivated)
18:33:48 DEBUG opendrift:2118: 9583 elements scheduled.
18:33:48 DEBUG opendrift:2120: ======================================================================
18:33:48 DEBUG opendrift:2131: 60.0 <- latitude -> 60.400001525878906
18:33:48 DEBUG opendrift:2131: 4.0 <- longitude -> 4.199999809265137
18:33:48 DEBUG opendrift:2129: z = 0.0
18:33:48 DEBUG opendrift:2132: ---------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10417 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.707107 (min) -0.707107 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:48 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:48 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift:623: No elements hit coastline.
18:33:48 DEBUG opendrift:1729: No elements to deactivate
18:33:48 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:48 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:48 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:48 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:48 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0004783844377588917 and 0.6708176047456992 m/s
18:33:48 DEBUG opendrift:846: to be seeded: 9583, already seeded 10417
18:33:48 DEBUG opendrift:868: Released 417 new elements.
18:33:48 DEBUG opendrift:2111: ======================================================================
18:33:48 INFO opendrift:2112: 2025-06-30 18:48:44.415110 - step 2 of 96 - 10834 active elements (0 deactivated)
18:33:48 DEBUG opendrift:2118: 9166 elements scheduled.
18:33:48 DEBUG opendrift:2120: ======================================================================
18:33:48 DEBUG opendrift:2131: 59.9889332079791 <- latitude -> 60.400001525878906
18:33:48 DEBUG opendrift:2131: 3.991009050284454 <- longitude -> 4.207835745653981
18:33:48 DEBUG opendrift:2129: z = 0.0
18:33:48 DEBUG opendrift:2132: ---------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10834 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0327191 (min) 0.0327191 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.683592 (min) -0.683592 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:48 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:48 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift:623: No elements hit coastline.
18:33:48 DEBUG opendrift:1729: No elements to deactivate
18:33:48 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:48 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:48 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:48 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:48 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0024255923287307634 and 0.6978153828635182 m/s
18:33:48 DEBUG opendrift:846: to be seeded: 9166, already seeded 10834
18:33:48 DEBUG opendrift:868: Released 416 new elements.
18:33:48 DEBUG opendrift:2111: ======================================================================
18:33:48 INFO opendrift:2112: 2025-06-30 19:03:44.415110 - step 3 of 96 - 11250 active elements (0 deactivated)
18:33:48 DEBUG opendrift:2118: 8750 elements scheduled.
18:33:48 DEBUG opendrift:2120: ======================================================================
18:33:48 DEBUG opendrift:2131: 59.98182961294819 <- latitude -> 60.400001525878906
18:33:48 DEBUG opendrift:2131: 3.986598282230568 <- longitude -> 4.210790500351989
18:33:48 DEBUG opendrift:2129: z = 0.0
18:33:48 DEBUG opendrift:2132: ---------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 11250 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0654031 (min) 0.0654031 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.659346 (min) -0.659346 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:48 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:48 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift:623: No elements hit coastline.
18:33:48 DEBUG opendrift:1729: No elements to deactivate
18:33:48 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:48 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:48 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:48 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:48 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0005759790513010039 and 0.7233143349503885 m/s
18:33:48 DEBUG opendrift:846: to be seeded: 8750, already seeded 11250
18:33:48 DEBUG opendrift:868: Released 417 new elements.
18:33:48 DEBUG opendrift:2111: ======================================================================
18:33:48 INFO opendrift:2112: 2025-06-30 19:18:44.415110 - step 4 of 96 - 11667 active elements (0 deactivated)
18:33:48 DEBUG opendrift:2118: 8333 elements scheduled.
18:33:48 DEBUG opendrift:2120: ======================================================================
18:33:48 DEBUG opendrift:2131: 59.975713166460615 <- latitude -> 60.400001525878906
18:33:48 DEBUG opendrift:2131: 3.986302604144529 <- longitude -> 4.218286740994067
18:33:48 DEBUG opendrift:2129: z = 0.0
18:33:48 DEBUG opendrift:2132: ---------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 11667 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0980171 (min) 0.0980171 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.634393 (min) -0.634393 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:48 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:48 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift:623: No elements hit coastline.
18:33:48 DEBUG opendrift:1729: No elements to deactivate
18:33:48 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:48 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:48 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:48 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:48 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036481103053630963 and 0.6646006747283487 m/s
18:33:48 DEBUG opendrift:846: to be seeded: 8333, already seeded 11667
18:33:48 DEBUG opendrift:868: Released 417 new elements.
18:33:48 DEBUG opendrift:2111: ======================================================================
18:33:48 INFO opendrift:2112: 2025-06-30 19:33:44.415110 - step 5 of 96 - 12084 active elements (0 deactivated)
18:33:48 DEBUG opendrift:2118: 7916 elements scheduled.
18:33:48 DEBUG opendrift:2120: ======================================================================
18:33:48 DEBUG opendrift:2131: 59.969656648486364 <- latitude -> 60.400001525878906
18:33:48 DEBUG opendrift:2131: 3.986569837452591 <- longitude -> 4.220227839911713
18:33:48 DEBUG opendrift:2129: z = 0.0
18:33:48 DEBUG opendrift:2132: ---------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:48 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:48 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 12084 elements
18:33:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:48 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:48 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:48 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:48 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:48 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:48 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.130526 (min) 0.130526 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.608761 (min) -0.608761 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:48 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:48 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:48 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:48 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:48 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020626844287717425 and 0.6570493443891587 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 7916, already seeded 12084
18:33:49 DEBUG opendrift:868: Released 416 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 19:48:44.415110 - step 6 of 96 - 12500 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 7500 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.961566125830345 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.9852073947532656 <- longitude -> 4.225880754288525
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 12500 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.162895 (min) 0.162895 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.582478 (min) -0.582478 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0001716675067009026 and 0.6528664837575173 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 7500, already seeded 12500
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 20:03:44.415110 - step 7 of 96 - 12917 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 7083 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.956765962432726 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.9879167606069466 <- longitude -> 4.2243103230375665
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 12917 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.19509 (min) 0.19509 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.55557 (min) -0.55557 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0006925187291057659 and 0.6399626248197438 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 7083, already seeded 12917
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 20:18:44.415110 - step 8 of 96 - 13334 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 6666 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.952711534974405 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.990163823674436 <- longitude -> 4.231318595551444
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 13334 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.227076 (min) 0.227076 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.528068 (min) -0.528068 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019837497791843515 and 0.7116543008246453 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 6666, already seeded 13334
18:33:49 DEBUG opendrift:868: Released 416 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 20:33:44.415110 - step 9 of 96 - 13750 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 6250 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.94750759737051 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.990923044202252 <- longitude -> 4.236394815105278
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 13750 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.258819 (min) 0.258819 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.5 (min) -0.5 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0013470725761360893 and 0.618411477222436 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 6250, already seeded 13750
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 20:48:44.415110 - step 10 of 96 - 14167 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 5833 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.94196782943895 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.9934962984158 <- longitude -> 4.239614967299764
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 14167 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.290285 (min) 0.290285 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.471397 (min) -0.471397 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012777836943165392 and 0.6133511246127163 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 5833, already seeded 14167
18:33:49 DEBUG opendrift:868: Released 416 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 21:03:44.415110 - step 11 of 96 - 14583 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 5417 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.93764986150843 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 3.9951272394293897 <- longitude -> 4.247002134180496
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 14583 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.321439 (min) 0.321439 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.442289 (min) -0.442289 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012446753818979822 and 0.654562649378931 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 5417, already seeded 14583
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 21:18:44.415110 - step 12 of 96 - 15000 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 5000 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.93370527277088 <- latitude -> 60.40013310701376
18:33:49 DEBUG opendrift:2131: 3.998872849854091 <- longitude -> 4.252692090534838
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 15000 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.35225 (min) 0.35225 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.412707 (min) -0.412707 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018328320656149759 and 0.6063211906196811 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 5000, already seeded 15000
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 21:33:44.415110 - step 13 of 96 - 15417 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 4583 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.928484920745234 <- latitude -> 60.400001525878906
18:33:49 DEBUG opendrift:2131: 4.003181979596534 <- longitude -> 4.2579998091393065
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 15417 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.382683 (min) 0.382683 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.382683 (min) -0.382683 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001956701948502457 and 0.6666777125583642 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 4583, already seeded 15417
18:33:49 DEBUG opendrift:868: Released 416 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 21:48:44.415110 - step 14 of 96 - 15833 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 4167 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.9248812533476 <- latitude -> 60.400293627631
18:33:49 DEBUG opendrift:2131: 4.0090732347992954 <- longitude -> 4.264089254374051
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 15833 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.412707 (min) 0.412707 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.35225 (min) -0.35225 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001967897448752019 and 0.6556102905940424 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 4167, already seeded 15833
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 22:03:44.415110 - step 15 of 96 - 16250 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 3750 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.92030957923807 <- latitude -> 60.40106597932256
18:33:49 DEBUG opendrift:2131: 4.013477799122551 <- longitude -> 4.271119658302645
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 16250 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.442289 (min) 0.442289 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.321439 (min) -0.321439 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0008582891768491151 and 0.6538565831550766 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 3750, already seeded 16250
18:33:49 DEBUG opendrift:868: Released 417 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 22:18:44.415110 - step 16 of 96 - 16667 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 3333 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.91554880496981 <- latitude -> 60.401284599310515
18:33:49 DEBUG opendrift:2131: 4.017297692353883 <- longitude -> 4.2813955598255635
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 16667 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.471397 (min) 0.471397 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.290285 (min) -0.290285 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:49 DEBUG opendrift:1729: No elements to deactivate
18:33:49 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:49 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:49 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:49 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:49 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0010749043173384658 and 0.6017224887114345 m/s
18:33:49 DEBUG opendrift:846: to be seeded: 3333, already seeded 16667
18:33:49 DEBUG opendrift:868: Released 416 new elements.
18:33:49 DEBUG opendrift:2111: ======================================================================
18:33:49 INFO opendrift:2112: 2025-06-30 22:33:44.415110 - step 17 of 96 - 17083 active elements (0 deactivated)
18:33:49 DEBUG opendrift:2118: 2917 elements scheduled.
18:33:49 DEBUG opendrift:2120: ======================================================================
18:33:49 DEBUG opendrift:2131: 59.912799497586136 <- latitude -> 60.400880738105094
18:33:49 DEBUG opendrift:2131: 4.024482770479216 <- longitude -> 4.291163082191727
18:33:49 DEBUG opendrift:2129: z = 0.0
18:33:49 DEBUG opendrift:2132: ---------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:49 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:49 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 17083 elements
18:33:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:49 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:49 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:49 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:49 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:49 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:49 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.5 (min) 0.5 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.258819 (min) -0.258819 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:49 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:49 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:49 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:49 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:49 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0009981821062900402 and 0.6661203474158527 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 2917, already seeded 17083
18:33:50 DEBUG opendrift:868: Released 417 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-06-30 22:48:44.415110 - step 18 of 96 - 17500 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 2500 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.910377358643714 <- latitude -> 60.40102088787448
18:33:50 DEBUG opendrift:2131: 4.034468408347627 <- longitude -> 4.302448469542373
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 17500 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.528068 (min) 0.528068 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.227076 (min) -0.227076 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00021801065656003164 and 0.7485461444861935 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 2500, already seeded 17500
18:33:50 DEBUG opendrift:868: Released 416 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-06-30 23:03:44.415110 - step 19 of 96 - 17916 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 2084 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90663710157564 <- latitude -> 60.40169336098914
18:33:50 DEBUG opendrift:2131: 4.04244076132154 <- longitude -> 4.312854090972568
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 17916 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.55557 (min) 0.55557 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.19509 (min) -0.19509 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001997235124461775 and 0.6652693857647132 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 2084, already seeded 17916
18:33:50 DEBUG opendrift:868: Released 417 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-06-30 23:18:44.415110 - step 20 of 96 - 18333 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 1667 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90356381339163 <- latitude -> 60.402536662763566
18:33:50 DEBUG opendrift:2131: 4.0493281364868245 <- longitude -> 4.323011580022753
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 18333 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.582478 (min) 0.582478 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.162895 (min) -0.162895 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0016039308398587808 and 0.683000830967989 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 1667, already seeded 18333
18:33:50 DEBUG opendrift:868: Released 417 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-06-30 23:33:44.415110 - step 21 of 96 - 18750 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 1250 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90408856642368 <- latitude -> 60.40251523663189
18:33:50 DEBUG opendrift:2131: 4.058483138550159 <- longitude -> 4.333665583662468
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 18750 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.608761 (min) 0.608761 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.130526 (min) -0.130526 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036990750871861693 and 0.7446689692863168 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 1250, already seeded 18750
18:33:50 DEBUG opendrift:868: Released 416 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-06-30 23:48:44.415110 - step 22 of 96 - 19166 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 834 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90385218058455 <- latitude -> 60.40342310011279
18:33:50 DEBUG opendrift:2131: 4.067826273407681 <- longitude -> 4.341711046255121
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19166 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.634393 (min) 0.634393 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0980171 (min) -0.0980171 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00019165716172213915 and 0.6396977686373392 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 834, already seeded 19166
18:33:50 DEBUG opendrift:868: Released 417 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-07-01 00:03:44.415110 - step 23 of 96 - 19583 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 417 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.902983176818964 <- latitude -> 60.40370110750315
18:33:50 DEBUG opendrift:2131: 4.07730574605288 <- longitude -> 4.35581178381932
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19583 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.659346 (min) 0.659346 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0654031 (min) -0.0654031 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0005936550504541482 and 0.743740193946451 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 417, already seeded 19583
18:33:50 DEBUG opendrift:868: Released 417 new elements.
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-07-01 00:18:44.415110 - step 24 of 96 - 20000 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 0 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.9015616667983 <- latitude -> 60.403782204352815
18:33:50 DEBUG opendrift:2131: 4.086470527137045 <- longitude -> 4.3658405369581414
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.683592 (min) 0.683592 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: -0.0327191 (min) -0.0327191 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0008935162994609504 and 0.6667605295541306 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-07-01 00:33:44.415110 - step 25 of 96 - 20000 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 0 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90311309538322 <- latitude -> 60.40480666937984
18:33:50 DEBUG opendrift:2131: 4.096864218374361 <- longitude -> 4.376247253394024
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.707107 (min) 0.707107 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011338357964871093 and 0.644038055310478 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-07-01 00:48:44.415110 - step 26 of 96 - 20000 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 0 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90287354514482 <- latitude -> 60.405815070341816
18:33:50 DEBUG opendrift:2131: 4.10702244158585 <- longitude -> 4.390070440082197
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:50 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.729864 (min) 0.729864 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0327191 (min) 0.0327191 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:50 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:50 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:50 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:50 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:50 DEBUG opendrift:623: No elements hit coastline.
18:33:50 DEBUG opendrift:1729: No elements to deactivate
18:33:50 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:50 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:50 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:50 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:50 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0010555899984309677 and 0.6536829843858957 m/s
18:33:50 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:50 DEBUG opendrift:2111: ======================================================================
18:33:50 INFO opendrift:2112: 2025-07-01 01:03:44.415110 - step 27 of 96 - 20000 active elements (0 deactivated)
18:33:50 DEBUG opendrift:2118: 0 elements scheduled.
18:33:50 DEBUG opendrift:2120: ======================================================================
18:33:50 DEBUG opendrift:2131: 59.90166104111249 <- latitude -> 60.40667784113546
18:33:50 DEBUG opendrift:2131: 4.1137959179843495 <- longitude -> 4.403285643870159
18:33:50 DEBUG opendrift:2129: z = 0.0
18:33:50 DEBUG opendrift:2132: ---------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:50 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:50 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:50 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:50 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:50 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:50 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:50 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:50 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.75184 (min) 0.75184 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0654031 (min) 0.0654031 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018863816073306804 and 0.6718534574900351 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 01:18:44.415110 - step 28 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.901885641100165 <- latitude -> 60.407998162742686
18:33:51 DEBUG opendrift:2131: 4.127892701183806 <- longitude -> 4.417909186553076
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.77301 (min) 0.77301 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.0980171 (min) 0.0980171 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002116237652365572 and 0.6882531345546973 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 01:33:44.415110 - step 29 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.90330578857828 <- latitude -> 60.41012859637172
18:33:51 DEBUG opendrift:2131: 4.138434642522627 <- longitude -> 4.43403890567364
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.793353 (min) 0.793353 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.130526 (min) 0.130526 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0007103531159978684 and 0.674080334030248 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 01:48:44.415110 - step 30 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.9024997649947 <- latitude -> 60.41111039288447
18:33:51 DEBUG opendrift:2131: 4.153552924006332 <- longitude -> 4.445909525397751
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.812847 (min) 0.812847 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.162895 (min) 0.162895 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012757928908164675 and 0.662120589730336 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 02:03:44.415110 - step 31 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.905087833653816 <- latitude -> 60.41337456472657
18:33:51 DEBUG opendrift:2131: 4.166354642650994 <- longitude -> 4.464466678829797
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.83147 (min) 0.83147 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.19509 (min) 0.19509 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001143053337813005 and 0.7186634827578383 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 02:18:44.415110 - step 32 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.906127244350344 <- latitude -> 60.41619802062936
18:33:51 DEBUG opendrift:2131: 4.178696338449474 <- longitude -> 4.478927571971532
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.849202 (min) 0.849202 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.227076 (min) 0.227076 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0004403873312388031 and 0.6158548807702673 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 02:33:44.415110 - step 33 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.908130174434525 <- latitude -> 60.41934410942158
18:33:51 DEBUG opendrift:2131: 4.188912912819961 <- longitude -> 4.493630199517747
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.866025 (min) 0.866025 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.258819 (min) 0.258819 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001120124502003005 and 0.658877962761667 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 02:48:44.415110 - step 34 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.90877657980711 <- latitude -> 60.4221569217412
18:33:51 DEBUG opendrift:2131: 4.203106484616184 <- longitude -> 4.50869181798516
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.881921 (min) 0.881921 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.290285 (min) 0.290285 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:51 DEBUG opendrift:1729: No elements to deactivate
18:33:51 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:51 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:51 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:51 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:51 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0017248076190992403 and 0.7661966211520362 m/s
18:33:51 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:51 DEBUG opendrift:2111: ======================================================================
18:33:51 INFO opendrift:2112: 2025-07-01 03:03:44.415110 - step 35 of 96 - 20000 active elements (0 deactivated)
18:33:51 DEBUG opendrift:2118: 0 elements scheduled.
18:33:51 DEBUG opendrift:2120: ======================================================================
18:33:51 DEBUG opendrift:2131: 59.90959155425649 <- latitude -> 60.42506820731998
18:33:51 DEBUG opendrift:2131: 4.218132364989313 <- longitude -> 4.523874825698244
18:33:51 DEBUG opendrift:2129: z = 0.0
18:33:51 DEBUG opendrift:2132: ---------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:51 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:51 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:51 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:51 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:51 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:51 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:51 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:51 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:51 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.896873 (min) 0.896873 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.321439 (min) 0.321439 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:51 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:51 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:51 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:51 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:51 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0014906549108621282 and 0.6326984745705948 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 03:18:44.415110 - step 36 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.911372669667436 <- latitude -> 60.42726798974072
18:33:52 DEBUG opendrift:2131: 4.228953840563955 <- longitude -> 4.541075195915172
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.910864 (min) 0.910864 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.35225 (min) 0.35225 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0012346637955292202 and 0.6454728418243137 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 03:33:44.415110 - step 37 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.913331269297366 <- latitude -> 60.430696610959004
18:33:52 DEBUG opendrift:2131: 4.246188248959151 <- longitude -> 4.557615415984443
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.92388 (min) 0.92388 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.382683 (min) 0.382683 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019104925498583097 and 0.7178986843973044 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 03:48:44.415110 - step 38 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.91853706559563 <- latitude -> 60.43527776395597
18:33:52 DEBUG opendrift:2131: 4.257347987579247 <- longitude -> 4.575037810806312
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.935906 (min) 0.935906 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.412707 (min) 0.412707 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0008334997975556808 and 0.6763909118887844 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 04:03:44.415110 - step 39 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.92203329526542 <- latitude -> 60.43964269969196
18:33:52 DEBUG opendrift:2131: 4.270035780152167 <- longitude -> 4.587913278797062
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.94693 (min) 0.94693 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.442289 (min) 0.442289 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0016273526975542673 and 0.6663615002156348 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 04:18:44.415110 - step 40 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.925743161341714 <- latitude -> 60.44368051305642
18:33:52 DEBUG opendrift:2131: 4.290493840547837 <- longitude -> 4.60363385903801
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.95694 (min) 0.95694 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.471397 (min) 0.471397 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0001850873502866946 and 0.632668220386991 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 04:33:44.415110 - step 41 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.928749783781306 <- latitude -> 60.45028740777853
18:33:52 DEBUG opendrift:2131: 4.299850885432803 <- longitude -> 4.617380856866025
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.965926 (min) 0.965926 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.5 (min) 0.5 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0015835789584519478 and 0.6826808654752956 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 04:48:44.415110 - step 42 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.932252324290744 <- latitude -> 60.45419211199763
18:33:52 DEBUG opendrift:2131: 4.317069028871556 <- longitude -> 4.6306725911394375
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.973877 (min) 0.973877 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.528068 (min) 0.528068 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0015878403240043462 and 0.6532421108491367 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 05:03:44.415110 - step 43 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.93481294649023 <- latitude -> 60.45802852347255
18:33:52 DEBUG opendrift:2131: 4.328373400403119 <- longitude -> 4.651551556117374
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:52 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.980785 (min) 0.980785 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.55557 (min) 0.55557 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:52 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:52 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:52 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:52 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:52 DEBUG opendrift:623: No elements hit coastline.
18:33:52 DEBUG opendrift:1729: No elements to deactivate
18:33:52 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:52 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:52 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:52 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:52 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001516365238598295 and 0.6484546794312046 m/s
18:33:52 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:52 DEBUG opendrift:2111: ======================================================================
18:33:52 INFO opendrift:2112: 2025-07-01 05:18:44.415110 - step 44 of 96 - 20000 active elements (0 deactivated)
18:33:52 DEBUG opendrift:2118: 0 elements scheduled.
18:33:52 DEBUG opendrift:2120: ======================================================================
18:33:52 DEBUG opendrift:2131: 59.937363174601856 <- latitude -> 60.46200923050674
18:33:52 DEBUG opendrift:2131: 4.342070821989857 <- longitude -> 4.666206970379621
18:33:52 DEBUG opendrift:2129: z = 0.0
18:33:52 DEBUG opendrift:2132: ---------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:52 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:52 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:52 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:52 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:52 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:52 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:52 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.986643 (min) 0.986643 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.582478 (min) 0.582478 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0017133649318304862 and 0.6667984640990559 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 05:33:44.415110 - step 45 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.94289887139466 <- latitude -> 60.466735965854056
18:33:53 DEBUG opendrift:2131: 4.356940876280607 <- longitude -> 4.6815402738372525
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.991445 (min) 0.991445 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.608761 (min) 0.608761 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002747788368445282 and 0.7360139614071095 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 05:48:44.415110 - step 46 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.94656178673707 <- latitude -> 60.47304995541367
18:33:53 DEBUG opendrift:2131: 4.375342357354464 <- longitude -> 4.696118218906989
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.995185 (min) 0.995185 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.634393 (min) 0.634393 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019102526596760404 and 0.7167953635925663 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 06:03:44.415110 - step 47 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.95031655947 <- latitude -> 60.47908039109224
18:33:53 DEBUG opendrift:2131: 4.385385762714923 <- longitude -> 4.714458142418321
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.997859 (min) 0.997859 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.659346 (min) 0.659346 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00018069244752100862 and 0.6348784056743623 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 06:18:44.415110 - step 48 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.9546724546952 <- latitude -> 60.48657383259642
18:33:53 DEBUG opendrift:2131: 4.401749585371887 <- longitude -> 4.7356950504452495
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.999465 (min) 0.999465 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.683592 (min) 0.683592 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002027839943802982 and 0.7055052557587911 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 06:33:44.415110 - step 49 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.95744448247602 <- latitude -> 60.49147261082811
18:33:53 DEBUG opendrift:2131: 4.419103132647014 <- longitude -> 4.748091669921553
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 1 (min) 1 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.707107 (min) 0.707107 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020421469399316654 and 0.6616856469321197 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 06:48:44.415110 - step 50 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.96259676838757 <- latitude -> 60.49686032787409
18:33:53 DEBUG opendrift:2131: 4.434404968150022 <- longitude -> 4.76575399019886
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.999465 (min) 0.999465 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.729864 (min) 0.729864 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002474977411255848 and 0.6549270565747503 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 07:03:44.415110 - step 51 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.971044401719624 <- latitude -> 60.505067502968245
18:33:53 DEBUG opendrift:2131: 4.446445912819859 <- longitude -> 4.778871472777117
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.997859 (min) 0.997859 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.75184 (min) 0.75184 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001394557425952194 and 0.6034335668891109 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 07:18:44.415110 - step 52 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.97880140676547 <- latitude -> 60.512439054912804
18:33:53 DEBUG opendrift:2131: 4.45943671845521 <- longitude -> 4.794167977121989
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.995185 (min) 0.995185 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.77301 (min) 0.77301 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:53 DEBUG opendrift:1729: No elements to deactivate
18:33:53 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:53 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:53 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:53 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:53 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019181642261345978 and 0.650211524998927 m/s
18:33:53 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:53 DEBUG opendrift:2111: ======================================================================
18:33:53 INFO opendrift:2112: 2025-07-01 07:33:44.415110 - step 53 of 96 - 20000 active elements (0 deactivated)
18:33:53 DEBUG opendrift:2118: 0 elements scheduled.
18:33:53 DEBUG opendrift:2120: ======================================================================
18:33:53 DEBUG opendrift:2131: 59.98353899777482 <- latitude -> 60.51950454950763
18:33:53 DEBUG opendrift:2131: 4.47695232315072 <- longitude -> 4.8192085142176
18:33:53 DEBUG opendrift:2129: z = 0.0
18:33:53 DEBUG opendrift:2132: ---------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:53 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:53 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:53 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:53 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:53 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:53 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:53 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:53 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:53 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.991445 (min) 0.991445 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.793353 (min) 0.793353 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:53 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:53 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:53 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:53 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:53 DEBUG opendrift:623: No elements hit coastline.
18:33:54 DEBUG opendrift:1729: No elements to deactivate
18:33:54 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:54 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:54 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:54 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:54 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0010539043123736992 and 0.6310421781391603 m/s
18:33:54 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:54 DEBUG opendrift:2111: ======================================================================
18:33:54 INFO opendrift:2112: 2025-07-01 07:48:44.415110 - step 54 of 96 - 20000 active elements (0 deactivated)
18:33:54 DEBUG opendrift:2118: 0 elements scheduled.
18:33:54 DEBUG opendrift:2120: ======================================================================
18:33:54 DEBUG opendrift:2131: 59.98910053749985 <- latitude -> 60.52828738674665
18:33:54 DEBUG opendrift:2131: 4.49235704049861 <- longitude -> 4.8369686158008065
18:33:54 DEBUG opendrift:2129: z = 0.0
18:33:54 DEBUG opendrift:2132: ---------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:54 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:54 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:54 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:54 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:54 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:54 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:54 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:54 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:54 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.986643 (min) 0.986643 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.812847 (min) 0.812847 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:54 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:54 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:54 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:54 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:54 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:54 DEBUG opendrift:623: No elements hit coastline.
18:33:54 DEBUG opendrift:1729: No elements to deactivate
18:33:54 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:54 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:54 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:54 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:54 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001660485658366536 and 0.6728553733600551 m/s
18:33:54 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:54 DEBUG opendrift:2111: ======================================================================
18:33:54 INFO opendrift:2112: 2025-07-01 08:03:44.415110 - step 55 of 96 - 20000 active elements (0 deactivated)
18:33:54 DEBUG opendrift:2118: 0 elements scheduled.
18:33:54 DEBUG opendrift:2120: ======================================================================
18:33:54 DEBUG opendrift:2131: 59.99657482706746 <- latitude -> 60.536042604429184
18:33:54 DEBUG opendrift:2131: 4.50865754666652 <- longitude -> 4.8543955940462125
18:33:54 DEBUG opendrift:2129: z = 0.0
18:33:54 DEBUG opendrift:2132: ---------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:54 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:54 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:54 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:54 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:54 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:54 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:54 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:54 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:54 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:54 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:55 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.980785 (min) 0.980785 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.83147 (min) 0.83147 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:55 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:55 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:55 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:55 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:55 DEBUG opendrift:623: No elements hit coastline.
18:33:55 DEBUG opendrift:1729: No elements to deactivate
18:33:55 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:55 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:55 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:55 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:55 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0023961718747631286 and 0.7089049851505758 m/s
18:33:55 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:55 DEBUG opendrift:2111: ======================================================================
18:33:55 INFO opendrift:2112: 2025-07-01 08:18:44.415110 - step 56 of 96 - 20000 active elements (0 deactivated)
18:33:55 DEBUG opendrift:2118: 0 elements scheduled.
18:33:55 DEBUG opendrift:2120: ======================================================================
18:33:55 DEBUG opendrift:2131: 60.002093295234005 <- latitude -> 60.54228849107933
18:33:55 DEBUG opendrift:2131: 4.525568561644843 <- longitude -> 4.873788184570426
18:33:55 DEBUG opendrift:2129: z = 0.0
18:33:55 DEBUG opendrift:2132: ---------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:55 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:55 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:55 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:55 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:55 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:614: Data needed for 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 20000 elements
18:33:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:55 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:55 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:55 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:55 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:55 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:55 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:55 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.973877 (min) 0.973877 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.849202 (min) 0.849202 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:55 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:55 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:55 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:55 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:55 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:55 DEBUG opendrift:626: 4 elements hit land, moving them to the coastline.
18:33:55 DEBUG opendrift:1702: Added status stranded
18:33:55 DEBUG opendrift:1713: 4 elements scheduled for deactivation (stranded)
18:33:55 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:33:57 DEBUG opendrift:1733: Removed 4 elements.
18:33:57 DEBUG opendrift:1736: Removed 4 values from environment.
18:33:57 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:57 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:57 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:57 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:57 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011827906588158666 and 0.6916539463575451 m/s
18:33:57 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:57 DEBUG opendrift:2111: ======================================================================
18:33:57 INFO opendrift:2112: 2025-07-01 08:33:44.415110 - step 57 of 96 - 19996 active elements (4 deactivated)
18:33:57 DEBUG opendrift:2118: 0 elements scheduled.
18:33:57 DEBUG opendrift:2120: ======================================================================
18:33:57 DEBUG opendrift:2131: 60.008563836349396 <- latitude -> 60.550510011852964
18:33:57 DEBUG opendrift:2131: 4.542283262934586 <- longitude -> 4.876354891503847
18:33:57 DEBUG opendrift:2129: z = 0.0
18:33:57 DEBUG opendrift:2132: ---------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19996 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:57 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.965926 (min) 0.965926 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.866025 (min) 0.866025 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:57 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:57 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:57 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift:626: 27 elements hit land, moving them to the coastline.
18:33:57 DEBUG opendrift:1713: 27 elements scheduled for deactivation (stranded)
18:33:57 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:33:57 DEBUG opendrift:1733: Removed 27 elements.
18:33:57 DEBUG opendrift:1736: Removed 27 values from environment.
18:33:57 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:57 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:57 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:57 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:57 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0023570944391652264 and 0.6772708540135095 m/s
18:33:57 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:57 DEBUG opendrift:2111: ======================================================================
18:33:57 INFO opendrift:2112: 2025-07-01 08:48:44.415110 - step 58 of 96 - 19969 active elements (31 deactivated)
18:33:57 DEBUG opendrift:2118: 0 elements scheduled.
18:33:57 DEBUG opendrift:2120: ======================================================================
18:33:57 DEBUG opendrift:2131: 60.015168940350776 <- latitude -> 60.5573610053044
18:33:57 DEBUG opendrift:2131: 4.557595604627869 <- longitude -> 4.893479228003766
18:33:57 DEBUG opendrift:2129: z = 0.0
18:33:57 DEBUG opendrift:2132: ---------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19969 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:57 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.95694 (min) 0.95694 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.881921 (min) 0.881921 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:57 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:57 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:57 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift:626: 147 elements hit land, moving them to the coastline.
18:33:57 DEBUG opendrift:1713: 147 elements scheduled for deactivation (stranded)
18:33:57 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:33:57 DEBUG opendrift:1733: Removed 147 elements.
18:33:57 DEBUG opendrift:1736: Removed 147 values from environment.
18:33:57 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:57 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:57 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:57 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:57 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011598415890844125 and 0.663569127987229 m/s
18:33:57 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:57 DEBUG opendrift:2111: ======================================================================
18:33:57 INFO opendrift:2112: 2025-07-01 09:03:44.415110 - step 59 of 96 - 19822 active elements (178 deactivated)
18:33:57 DEBUG opendrift:2118: 0 elements scheduled.
18:33:57 DEBUG opendrift:2120: ======================================================================
18:33:57 DEBUG opendrift:2131: 60.02260232814449 <- latitude -> 60.563830581795244
18:33:57 DEBUG opendrift:2131: 4.570928409641453 <- longitude -> 4.910648966590062
18:33:57 DEBUG opendrift:2129: z = 0.0
18:33:57 DEBUG opendrift:2132: ---------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:57 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:57 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19822 elements
18:33:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:57 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:57 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:57 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:57 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:57 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:57 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.94693 (min) 0.94693 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.896873 (min) 0.896873 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:57 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:57 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:57 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:57 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:57 DEBUG opendrift:626: 336 elements hit land, moving them to the coastline.
18:33:57 DEBUG opendrift:1713: 336 elements scheduled for deactivation (stranded)
18:33:57 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:33:58 DEBUG opendrift:1733: Removed 336 elements.
18:33:58 DEBUG opendrift:1736: Removed 336 values from environment.
18:33:58 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:58 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:58 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:58 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:58 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00164897258768246 and 0.6523343715279821 m/s
18:33:58 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:58 DEBUG opendrift:2111: ======================================================================
18:33:58 INFO opendrift:2112: 2025-07-01 09:18:44.415110 - step 60 of 96 - 19486 active elements (514 deactivated)
18:33:58 DEBUG opendrift:2118: 0 elements scheduled.
18:33:58 DEBUG opendrift:2120: ======================================================================
18:33:58 DEBUG opendrift:2131: 60.02747637123181 <- latitude -> 60.57168543677169
18:33:58 DEBUG opendrift:2131: 4.585213485994837 <- longitude -> 4.925514299116738
18:33:58 DEBUG opendrift:2129: z = 0.0
18:33:58 DEBUG opendrift:2132: ---------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:58 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:58 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:58 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:58 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:58 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:58 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:58 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:614: Data needed for 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 19486 elements
18:33:58 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:58 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:58 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:58 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:58 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:58 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:58 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:58 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.935906 (min) 0.935906 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.910864 (min) 0.910864 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:58 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:58 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:58 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:58 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:58 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:58 DEBUG opendrift:626: 749 elements hit land, moving them to the coastline.
18:33:58 DEBUG opendrift:1713: 749 elements scheduled for deactivation (stranded)
18:33:58 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:33:59 DEBUG opendrift:1733: Removed 749 elements.
18:33:59 DEBUG opendrift:1736: Removed 749 values from environment.
18:33:59 DEBUG opendrift:2166: Calling OceanDrift.update()
18:33:59 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:33:59 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:33:59 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:33:59 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0013272287265555032 and 0.7594377401004461 m/s
18:33:59 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:33:59 DEBUG opendrift:2111: ======================================================================
18:33:59 INFO opendrift:2112: 2025-07-01 09:33:44.415110 - step 61 of 96 - 18737 active elements (1263 deactivated)
18:33:59 DEBUG opendrift:2118: 0 elements scheduled.
18:33:59 DEBUG opendrift:2120: ======================================================================
18:33:59 DEBUG opendrift:2131: 60.03479416438543 <- latitude -> 60.578137762092766
18:33:59 DEBUG opendrift:2131: 4.601259214723544 <- longitude -> 4.941896291331746
18:33:59 DEBUG opendrift:2129: z = 0.0
18:33:59 DEBUG opendrift:2132: ---------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:33:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:33:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:59 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:33:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:33:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:33:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:59 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:33:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:59 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:33:59 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:33:59 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:614: Data needed for 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 18737 elements
18:33:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:33:59 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:33:59 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:33:59 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:33:59 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:33:59 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:33:59 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:33:59 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.92388 (min) 0.92388 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.92388 (min) 0.92388 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:33:59 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:33:59 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:33:59 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:33:59 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:33:59 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:33:59 DEBUG opendrift:626: 2076 elements hit land, moving them to the coastline.
18:33:59 DEBUG opendrift:1713: 2076 elements scheduled for deactivation (stranded)
18:33:59 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:01 DEBUG opendrift:1733: Removed 2076 elements.
18:34:01 DEBUG opendrift:1736: Removed 2076 values from environment.
18:34:01 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:01 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:01 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:01 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:01 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0016511758340700016 and 0.684031226724656 m/s
18:34:01 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:01 DEBUG opendrift:2111: ======================================================================
18:34:01 INFO opendrift:2112: 2025-07-01 09:48:44.415110 - step 62 of 96 - 16661 active elements (3339 deactivated)
18:34:01 DEBUG opendrift:2118: 0 elements scheduled.
18:34:01 DEBUG opendrift:2120: ======================================================================
18:34:01 DEBUG opendrift:2131: 60.04046190548857 <- latitude -> 60.58627949303191
18:34:01 DEBUG opendrift:2131: 4.614503812843648 <- longitude -> 4.945477457432173
18:34:01 DEBUG opendrift:2129: z = 0.0
18:34:01 DEBUG opendrift:2132: ---------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:01 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:01 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:01 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:01 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:01 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:614: Data needed for 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 16661 elements
18:34:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:01 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:01 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:01 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:01 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:01 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:01 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:01 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.910864 (min) 0.910864 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.935906 (min) 0.935906 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:01 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:01 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:01 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:01 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:01 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:01 DEBUG opendrift:626: 2844 elements hit land, moving them to the coastline.
18:34:01 DEBUG opendrift:1713: 2844 elements scheduled for deactivation (stranded)
18:34:01 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:04 DEBUG opendrift:1733: Removed 2844 elements.
18:34:04 DEBUG opendrift:1736: Removed 2844 values from environment.
18:34:04 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:04 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:04 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:04 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:04 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0007569779142612818 and 0.6531890048392434 m/s
18:34:04 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:04 DEBUG opendrift:2111: ======================================================================
18:34:04 INFO opendrift:2112: 2025-07-01 10:03:44.415110 - step 63 of 96 - 13817 active elements (6183 deactivated)
18:34:04 DEBUG opendrift:2118: 0 elements scheduled.
18:34:04 DEBUG opendrift:2120: ======================================================================
18:34:04 DEBUG opendrift:2131: 60.047053908630275 <- latitude -> 60.592138060342336
18:34:04 DEBUG opendrift:2131: 4.629558694678762 <- longitude -> 4.9607121876677995
18:34:04 DEBUG opendrift:2129: z = 0.0
18:34:04 DEBUG opendrift:2132: ---------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:04 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:04 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:04 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:04 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:04 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:614: Data needed for 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 13817 elements
18:34:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:04 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:04 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:04 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:04 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:04 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:04 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:04 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.896873 (min) 0.896873 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.94693 (min) 0.94693 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:04 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:04 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:04 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:04 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:04 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:04 DEBUG opendrift:626: 1965 elements hit land, moving them to the coastline.
18:34:04 DEBUG opendrift:1713: 1965 elements scheduled for deactivation (stranded)
18:34:04 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:06 DEBUG opendrift:1733: Removed 1965 elements.
18:34:06 DEBUG opendrift:1736: Removed 1965 values from environment.
18:34:06 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:06 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:06 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:06 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:06 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0018089601521269901 and 0.6546975323774888 m/s
18:34:06 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:06 DEBUG opendrift:2111: ======================================================================
18:34:06 INFO opendrift:2112: 2025-07-01 10:18:44.415110 - step 64 of 96 - 11852 active elements (8148 deactivated)
18:34:06 DEBUG opendrift:2118: 0 elements scheduled.
18:34:06 DEBUG opendrift:2120: ======================================================================
18:34:06 DEBUG opendrift:2131: 60.05771703452828 <- latitude -> 60.5935609717592
18:34:06 DEBUG opendrift:2131: 4.645391004273493 <- longitude -> 4.979306387181799
18:34:06 DEBUG opendrift:2129: z = 0.0
18:34:06 DEBUG opendrift:2132: ---------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:06 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:06 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:06 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:06 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:06 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:614: Data needed for 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 11852 elements
18:34:06 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:06 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:06 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:06 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:06 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:06 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:06 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:06 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.881921 (min) 0.881921 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.95694 (min) 0.95694 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:06 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:06 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:06 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:06 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:06 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:06 DEBUG opendrift:626: 995 elements hit land, moving them to the coastline.
18:34:06 DEBUG opendrift:1713: 995 elements scheduled for deactivation (stranded)
18:34:06 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:07 DEBUG opendrift:1733: Removed 995 elements.
18:34:07 DEBUG opendrift:1736: Removed 995 values from environment.
18:34:07 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:07 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:07 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:07 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:07 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00098800643290302 and 0.663937184290865 m/s
18:34:07 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:07 DEBUG opendrift:2111: ======================================================================
18:34:07 INFO opendrift:2112: 2025-07-01 10:33:44.415110 - step 65 of 96 - 10857 active elements (9143 deactivated)
18:34:07 DEBUG opendrift:2118: 0 elements scheduled.
18:34:07 DEBUG opendrift:2120: ======================================================================
18:34:07 DEBUG opendrift:2131: 60.06386488273323 <- latitude -> 60.60060719965694
18:34:07 DEBUG opendrift:2131: 4.660323696647748 <- longitude -> 4.996376434077517
18:34:07 DEBUG opendrift:2129: z = 0.0
18:34:07 DEBUG opendrift:2132: ---------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10857 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.866025 (min) 0.866025 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.965926 (min) 0.965926 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:07 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:07 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:07 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:07 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:07 DEBUG opendrift:626: 358 elements hit land, moving them to the coastline.
18:34:07 DEBUG opendrift:1713: 358 elements scheduled for deactivation (stranded)
18:34:07 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:07 DEBUG opendrift:1733: Removed 358 elements.
18:34:07 DEBUG opendrift:1736: Removed 358 values from environment.
18:34:07 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:07 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:07 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:07 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:07 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011333363261569228 and 0.6430834906825046 m/s
18:34:07 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:07 DEBUG opendrift:2111: ======================================================================
18:34:07 INFO opendrift:2112: 2025-07-01 10:48:44.415110 - step 66 of 96 - 10499 active elements (9501 deactivated)
18:34:07 DEBUG opendrift:2118: 0 elements scheduled.
18:34:07 DEBUG opendrift:2120: ======================================================================
18:34:07 DEBUG opendrift:2131: 60.07125456697098 <- latitude -> 60.6086219974744
18:34:07 DEBUG opendrift:2131: 4.676752676660054 <- longitude -> 5.011398156074519
18:34:07 DEBUG opendrift:2129: z = 0.0
18:34:07 DEBUG opendrift:2132: ---------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10499 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:07 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:07 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:07 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:07 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.849202 (min) 0.849202 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.973877 (min) 0.973877 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:07 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:07 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:07 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:07 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:07 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:07 DEBUG opendrift:626: 197 elements hit land, moving them to the coastline.
18:34:07 DEBUG opendrift:1713: 197 elements scheduled for deactivation (stranded)
18:34:07 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:07 DEBUG opendrift:1733: Removed 197 elements.
18:34:07 DEBUG opendrift:1736: Removed 197 values from environment.
18:34:07 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:07 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:07 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:07 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:07 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0025688858155714198 and 0.697840524171302 m/s
18:34:07 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:07 DEBUG opendrift:2111: ======================================================================
18:34:07 INFO opendrift:2112: 2025-07-01 11:03:44.415110 - step 67 of 96 - 10302 active elements (9698 deactivated)
18:34:07 DEBUG opendrift:2118: 0 elements scheduled.
18:34:07 DEBUG opendrift:2120: ======================================================================
18:34:07 DEBUG opendrift:2131: 60.07878719635157 <- latitude -> 60.603995010967466
18:34:07 DEBUG opendrift:2131: 4.692632038159043 <- longitude -> 5.007923860581697
18:34:07 DEBUG opendrift:2129: z = 0.0
18:34:07 DEBUG opendrift:2132: ---------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:07 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:07 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:07 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10302 elements
18:34:07 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10302 elements
18:34:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:07 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:07 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10302 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10302 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10302 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10302 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.83147 (min) 0.83147 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.980785 (min) 0.980785 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 114 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 114 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 114 elements.
18:34:08 DEBUG opendrift:1736: Removed 114 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0009320201089410899 and 0.6058736669927178 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 11:18:44.415110 - step 68 of 96 - 10188 active elements (9812 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.08668300283819 <- latitude -> 60.61389420811267
18:34:08 DEBUG opendrift:2131: 4.70565187221939 <- longitude -> 5.009397222434042
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10188 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.812847 (min) 0.812847 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.986643 (min) 0.986643 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 73 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 73 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 73 elements.
18:34:08 DEBUG opendrift:1736: Removed 73 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003703570430123482 and 0.6498524702802314 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 11:33:44.415110 - step 69 of 96 - 10115 active elements (9885 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.09418769332153 <- latitude -> 60.62133421673067
18:34:08 DEBUG opendrift:2131: 4.714678442413302 <- longitude -> 5.012518836307634
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10115 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.793353 (min) 0.793353 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.991445 (min) 0.991445 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 36 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 36 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 36 elements.
18:34:08 DEBUG opendrift:1736: Removed 36 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003969870087277251 and 0.6243343314043794 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 11:48:44.415110 - step 70 of 96 - 10079 active elements (9921 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.09966605283215 <- latitude -> 60.630346947269196
18:34:08 DEBUG opendrift:2131: 4.72896899633981 <- longitude -> 5.008661530385605
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10079 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.77301 (min) 0.77301 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.995185 (min) 0.995185 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 38 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 38 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 38 elements.
18:34:08 DEBUG opendrift:1736: Removed 38 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001138025570186333 and 0.6622485975234808 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 12:03:44.415110 - step 71 of 96 - 10041 active elements (9959 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.10715889043236 <- latitude -> 60.63891100088855
18:34:08 DEBUG opendrift:2131: 4.738962333126467 <- longitude -> 5.022162721155703
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10041 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.75184 (min) 0.75184 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.997859 (min) 0.997859 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 7 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 7 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 7 elements.
18:34:08 DEBUG opendrift:1736: Removed 7 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00016322267094997023 and 0.6089492139668994 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 12:18:44.415110 - step 72 of 96 - 10034 active elements (9966 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.115129018845764 <- latitude -> 60.649053449791325
18:34:08 DEBUG opendrift:2131: 4.753019053492832 <- longitude -> 5.001020316989357
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10034 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.729864 (min) 0.729864 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.999465 (min) 0.999465 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 5 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 5 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 5 elements.
18:34:08 DEBUG opendrift:1736: Removed 5 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0022564047625173718 and 0.6825196278601715 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 12:33:44.415110 - step 73 of 96 - 10029 active elements (9971 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.12087738345298 <- latitude -> 60.652143533534044
18:34:08 DEBUG opendrift:2131: 4.761647673802501 <- longitude -> 5.005712084013138
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10029 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.707107 (min) 0.707107 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 1 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 13 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 13 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:08 DEBUG opendrift:1733: Removed 13 elements.
18:34:08 DEBUG opendrift:1736: Removed 13 values from environment.
18:34:08 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:08 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:08 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:08 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:08 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0010065387997015127 and 0.6298916356404459 m/s
18:34:08 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:08 DEBUG opendrift:2111: ======================================================================
18:34:08 INFO opendrift:2112: 2025-07-01 12:48:44.415110 - step 74 of 96 - 10016 active elements (9984 deactivated)
18:34:08 DEBUG opendrift:2118: 0 elements scheduled.
18:34:08 DEBUG opendrift:2120: ======================================================================
18:34:08 DEBUG opendrift:2131: 60.129724627136326 <- latitude -> 60.65443193199735
18:34:08 DEBUG opendrift:2131: 4.773651821142362 <- longitude -> 4.963947427390377
18:34:08 DEBUG opendrift:2129: z = 0.0
18:34:08 DEBUG opendrift:2132: ---------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:08 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:08 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10016 elements
18:34:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:08 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:08 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:08 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:08 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:08 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:08 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.683592 (min) 0.683592 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.999465 (min) 0.999465 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:08 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:08 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:08 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:08 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:08 DEBUG opendrift:626: 11 elements hit land, moving them to the coastline.
18:34:08 DEBUG opendrift:1713: 11 elements scheduled for deactivation (stranded)
18:34:08 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 11 elements.
18:34:09 DEBUG opendrift:1736: Removed 11 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0028180305614185566 and 0.7584247039609127 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 13:03:44.415110 - step 75 of 96 - 10005 active elements (9995 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.13865213026085 <- latitude -> 60.66312332219496
18:34:09 DEBUG opendrift:2131: 4.7814345446659505 <- longitude -> 4.962932378987756
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10005 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.659346 (min) 0.659346 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.997859 (min) 0.997859 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 4 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 4 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 4 elements.
18:34:09 DEBUG opendrift:1736: Removed 4 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0017819557790387075 and 0.5742552817162931 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 13:18:44.415110 - step 76 of 96 - 10001 active elements (9999 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.14621228356114 <- latitude -> 60.67080721872613
18:34:09 DEBUG opendrift:2131: 4.791899772047216 <- longitude -> 4.969012048327577
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10001 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.634393 (min) 0.634393 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.995185 (min) 0.995185 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 1 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 1 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 1 elements.
18:34:09 DEBUG opendrift:1736: Removed 1 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0017411288922463033 and 0.6754836561544258 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 13:33:44.415110 - step 77 of 96 - 10000 active elements (10000 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.15461074559141 <- latitude -> 60.24090836781567
18:34:09 DEBUG opendrift:2131: 4.802380445279483 <- longitude -> 4.955051183496601
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.608761 (min) 0.608761 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.991445 (min) 0.991445 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:623: No elements hit coastline.
18:34:09 DEBUG opendrift:1729: No elements to deactivate
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0016316951805623089 and 0.6161712620439994 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 13:48:44.415110 - step 78 of 96 - 10000 active elements (10000 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.16104115623111 <- latitude -> 60.24873334529896
18:34:09 DEBUG opendrift:2131: 4.813599800414429 <- longitude -> 4.965662344112914
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.582478 (min) 0.582478 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.986643 (min) 0.986643 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:623: No elements hit coastline.
18:34:09 DEBUG opendrift:1729: No elements to deactivate
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0011315609477084564 and 0.6453528598856837 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 14:03:44.415110 - step 79 of 96 - 10000 active elements (10000 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.16776132204743 <- latitude -> 60.25517245143333
18:34:09 DEBUG opendrift:2131: 4.823437205867416 <- longitude -> 4.975472791246047
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.55557 (min) 0.55557 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.980785 (min) 0.980785 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:623: No elements hit coastline.
18:34:09 DEBUG opendrift:1729: No elements to deactivate
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0019162957521953827 and 0.66099199464259 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 14:18:44.415110 - step 80 of 96 - 10000 active elements (10000 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.174760030461485 <- latitude -> 60.26493738687162
18:34:09 DEBUG opendrift:2131: 4.832244329626053 <- longitude -> 4.9835333117084515
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 10000 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.528068 (min) 0.528068 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.973877 (min) 0.973877 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 7 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 7 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 7 elements.
18:34:09 DEBUG opendrift:1736: Removed 7 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020865111364467112 and 0.6735607366679655 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 14:33:44.415110 - step 81 of 96 - 9993 active elements (10007 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.18173410839751 <- latitude -> 60.27402683401922
18:34:09 DEBUG opendrift:2131: 4.837186793422456 <- longitude -> 4.992553348208767
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 9993 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.5 (min) 0.5 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.965926 (min) 0.965926 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 39 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 39 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 39 elements.
18:34:09 DEBUG opendrift:1736: Removed 39 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002538706838830736 and 0.7378004987242909 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 14:48:44.415110 - step 82 of 96 - 9954 active elements (10046 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.189541900111244 <- latitude -> 60.28226487986754
18:34:09 DEBUG opendrift:2131: 4.845141520085394 <- longitude -> 5.00014181027662
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 9954 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.471397 (min) 0.471397 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.95694 (min) 0.95694 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 225 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 225 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:09 DEBUG opendrift:1733: Removed 225 elements.
18:34:09 DEBUG opendrift:1736: Removed 225 values from environment.
18:34:09 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:09 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:09 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:09 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:09 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.002150883722605878 and 0.636511853267026 m/s
18:34:09 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:09 DEBUG opendrift:2111: ======================================================================
18:34:09 INFO opendrift:2112: 2025-07-01 15:03:44.415110 - step 83 of 96 - 9729 active elements (10271 deactivated)
18:34:09 DEBUG opendrift:2118: 0 elements scheduled.
18:34:09 DEBUG opendrift:2120: ======================================================================
18:34:09 DEBUG opendrift:2131: 60.19727227851145 <- latitude -> 60.2901610060884
18:34:09 DEBUG opendrift:2131: 4.851257963960245 <- longitude -> 4.995951739738295
18:34:09 DEBUG opendrift:2129: z = 0.0
18:34:09 DEBUG opendrift:2132: ---------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:09 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:09 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 9729 elements
18:34:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:09 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:09 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:09 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:09 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:09 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:09 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.442289 (min) 0.442289 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.94693 (min) 0.94693 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:09 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:09 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:09 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:09 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:09 DEBUG opendrift:626: 688 elements hit land, moving them to the coastline.
18:34:09 DEBUG opendrift:1713: 688 elements scheduled for deactivation (stranded)
18:34:09 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:10 DEBUG opendrift:1733: Removed 688 elements.
18:34:10 DEBUG opendrift:1736: Removed 688 values from environment.
18:34:10 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:10 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:10 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:10 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:10 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003719262309967598 and 0.626402984948736 m/s
18:34:10 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:10 DEBUG opendrift:2111: ======================================================================
18:34:10 INFO opendrift:2112: 2025-07-01 15:18:44.415110 - step 84 of 96 - 9041 active elements (10959 deactivated)
18:34:10 DEBUG opendrift:2118: 0 elements scheduled.
18:34:10 DEBUG opendrift:2120: ======================================================================
18:34:10 DEBUG opendrift:2131: 60.20586302483343 <- latitude -> 60.30018944094358
18:34:10 DEBUG opendrift:2131: 4.859973601644811 <- longitude -> 4.996941891076716
18:34:10 DEBUG opendrift:2129: z = 0.0
18:34:10 DEBUG opendrift:2132: ---------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:10 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:10 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:10 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:10 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:10 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:10 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:10 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:614: Data needed for 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 9041 elements
18:34:10 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:10 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:10 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:10 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:10 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:10 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:10 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:10 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.412707 (min) 0.412707 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.935906 (min) 0.935906 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:10 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:10 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:10 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:10 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:10 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:10 DEBUG opendrift:626: 1273 elements hit land, moving them to the coastline.
18:34:10 DEBUG opendrift:1713: 1273 elements scheduled for deactivation (stranded)
18:34:10 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:11 DEBUG opendrift:1733: Removed 1273 elements.
18:34:11 DEBUG opendrift:1736: Removed 1273 values from environment.
18:34:11 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:11 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:11 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:11 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:11 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.001250974418126693 and 0.5912709351509505 m/s
18:34:11 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:11 DEBUG opendrift:2111: ======================================================================
18:34:11 INFO opendrift:2112: 2025-07-01 15:33:44.415110 - step 85 of 96 - 7768 active elements (12232 deactivated)
18:34:11 DEBUG opendrift:2118: 0 elements scheduled.
18:34:11 DEBUG opendrift:2120: ======================================================================
18:34:11 DEBUG opendrift:2131: 60.213047110849246 <- latitude -> 60.30780380699134
18:34:11 DEBUG opendrift:2131: 4.865611941445716 <- longitude -> 4.99478257292857
18:34:11 DEBUG opendrift:2129: z = 0.0
18:34:11 DEBUG opendrift:2132: ---------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 7768 elements
18:34:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 7768 elements
18:34:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:11 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 7768 elements
18:34:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 7768 elements
18:34:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:11 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:11 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:11 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:11 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:11 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:11 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:11 DEBUG opendrift.models.basemodel.environment:614: Data needed for 7768 elements
18:34:11 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 7768 elements
18:34:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:12 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:12 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:12 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:12 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:12 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:12 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:12 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.382683 (min) 0.382683 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.92388 (min) 0.92388 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:12 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:12 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:12 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:12 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:12 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:12 DEBUG opendrift:626: 1801 elements hit land, moving them to the coastline.
18:34:12 DEBUG opendrift:1713: 1801 elements scheduled for deactivation (stranded)
18:34:12 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:13 DEBUG opendrift:1733: Removed 1801 elements.
18:34:13 DEBUG opendrift:1736: Removed 1801 values from environment.
18:34:13 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:13 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:13 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:13 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:13 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0016718127211672027 and 0.6530367841284331 m/s
18:34:13 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:13 DEBUG opendrift:2111: ======================================================================
18:34:13 INFO opendrift:2112: 2025-07-01 15:48:44.415110 - step 86 of 96 - 5967 active elements (14033 deactivated)
18:34:13 DEBUG opendrift:2118: 0 elements scheduled.
18:34:13 DEBUG opendrift:2120: ======================================================================
18:34:13 DEBUG opendrift:2131: 60.22042814309364 <- latitude -> 60.316488986870624
18:34:13 DEBUG opendrift:2131: 4.87262340792464 <- longitude -> 4.997762372971645
18:34:13 DEBUG opendrift:2129: z = 0.0
18:34:13 DEBUG opendrift:2132: ---------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:13 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:13 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:13 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:13 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:13 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:614: Data needed for 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 5967 elements
18:34:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:13 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:13 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:13 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:13 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:13 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:13 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:13 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.35225 (min) 0.35225 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.910864 (min) 0.910864 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:13 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:13 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:13 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:13 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:13 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:13 DEBUG opendrift:626: 1697 elements hit land, moving them to the coastline.
18:34:13 DEBUG opendrift:1713: 1697 elements scheduled for deactivation (stranded)
18:34:13 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:15 DEBUG opendrift:1733: Removed 1697 elements.
18:34:15 DEBUG opendrift:1736: Removed 1697 values from environment.
18:34:15 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:15 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:15 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:15 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:15 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003827724985333203 and 0.5849336035140908 m/s
18:34:15 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:15 DEBUG opendrift:2111: ======================================================================
18:34:15 INFO opendrift:2112: 2025-07-01 16:03:44.415110 - step 87 of 96 - 4270 active elements (15730 deactivated)
18:34:15 DEBUG opendrift:2118: 0 elements scheduled.
18:34:15 DEBUG opendrift:2120: ======================================================================
18:34:15 DEBUG opendrift:2131: 60.2277676523252 <- latitude -> 60.32279209880877
18:34:15 DEBUG opendrift:2131: 4.8759094441831605 <- longitude -> 5.00205953473389
18:34:15 DEBUG opendrift:2129: z = 0.0
18:34:15 DEBUG opendrift:2132: ---------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:15 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:15 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:15 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:15 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:15 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:614: Data needed for 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 4270 elements
18:34:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:15 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:15 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:15 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:15 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:15 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:15 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:15 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.321439 (min) 0.321439 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.896873 (min) 0.896873 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:15 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:15 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:15 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:15 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:15 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:15 DEBUG opendrift:626: 1320 elements hit land, moving them to the coastline.
18:34:15 DEBUG opendrift:1713: 1320 elements scheduled for deactivation (stranded)
18:34:15 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:16 DEBUG opendrift:1733: Removed 1320 elements.
18:34:16 DEBUG opendrift:1736: Removed 1320 values from environment.
18:34:16 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:16 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:16 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:16 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:16 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0020590302394972258 and 0.6015461753780741 m/s
18:34:16 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:16 DEBUG opendrift:2111: ======================================================================
18:34:16 INFO opendrift:2112: 2025-07-01 16:18:44.415110 - step 88 of 96 - 2950 active elements (17050 deactivated)
18:34:16 DEBUG opendrift:2118: 0 elements scheduled.
18:34:16 DEBUG opendrift:2120: ======================================================================
18:34:16 DEBUG opendrift:2131: 60.234522417785016 <- latitude -> 60.329679513947795
18:34:16 DEBUG opendrift:2131: 4.883100547348347 <- longitude -> 4.9970975915306095
18:34:16 DEBUG opendrift:2129: z = 0.0
18:34:16 DEBUG opendrift:2132: ---------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:16 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:16 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:16 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:16 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:16 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:16 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:16 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 2950 elements
18:34:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:16 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:16 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:16 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:16 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:16 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:16 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:16 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.290285 (min) 0.290285 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.881921 (min) 0.881921 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:16 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:16 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:16 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:16 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:16 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:16 DEBUG opendrift:626: 888 elements hit land, moving them to the coastline.
18:34:16 DEBUG opendrift:1713: 888 elements scheduled for deactivation (stranded)
18:34:16 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:17 DEBUG opendrift:1733: Removed 888 elements.
18:34:17 DEBUG opendrift:1736: Removed 888 values from environment.
18:34:17 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:17 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:17 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:17 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:17 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.006086767919096393 and 0.5464493884756085 m/s
18:34:17 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:17 DEBUG opendrift:2111: ======================================================================
18:34:17 INFO opendrift:2112: 2025-07-01 16:33:44.415110 - step 89 of 96 - 2062 active elements (17938 deactivated)
18:34:17 DEBUG opendrift:2118: 0 elements scheduled.
18:34:17 DEBUG opendrift:2120: ======================================================================
18:34:17 DEBUG opendrift:2131: 60.24073851133438 <- latitude -> 60.33692210547657
18:34:17 DEBUG opendrift:2131: 4.887757890029407 <- longitude -> 4.996561696532032
18:34:17 DEBUG opendrift:2129: z = 0.0
18:34:17 DEBUG opendrift:2132: ---------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:17 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:17 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:17 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:17 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:17 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:614: Data needed for 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 2062 elements
18:34:17 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:17 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:17 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:17 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:17 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:17 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:17 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:17 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.258819 (min) 0.258819 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.866025 (min) 0.866025 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:17 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:17 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:17 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:17 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:17 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:17 DEBUG opendrift:626: 626 elements hit land, moving them to the coastline.
18:34:17 DEBUG opendrift:1713: 626 elements scheduled for deactivation (stranded)
18:34:17 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:18 DEBUG opendrift:1733: Removed 626 elements.
18:34:18 DEBUG opendrift:1736: Removed 626 values from environment.
18:34:18 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:18 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:18 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:18 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:18 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.00735776119099111 and 0.555084375359102 m/s
18:34:18 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:18 DEBUG opendrift:2111: ======================================================================
18:34:18 INFO opendrift:2112: 2025-07-01 16:48:44.415110 - step 90 of 96 - 1436 active elements (18564 deactivated)
18:34:18 DEBUG opendrift:2118: 0 elements scheduled.
18:34:18 DEBUG opendrift:2120: ======================================================================
18:34:18 DEBUG opendrift:2131: 60.24758385341792 <- latitude -> 60.33006664671505
18:34:18 DEBUG opendrift:2131: 4.889343635855528 <- longitude -> 4.992725823397902
18:34:18 DEBUG opendrift:2129: z = 0.0
18:34:18 DEBUG opendrift:2132: ---------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 1436 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:18 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.227076 (min) 0.227076 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.849202 (min) 0.849202 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:18 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:18 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:18 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift:626: 474 elements hit land, moving them to the coastline.
18:34:18 DEBUG opendrift:1713: 474 elements scheduled for deactivation (stranded)
18:34:18 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:18 DEBUG opendrift:1733: Removed 474 elements.
18:34:18 DEBUG opendrift:1736: Removed 474 values from environment.
18:34:18 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:18 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:18 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:18 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:18 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.005026491836975483 and 0.5968454080119526 m/s
18:34:18 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:18 DEBUG opendrift:2111: ======================================================================
18:34:18 INFO opendrift:2112: 2025-07-01 17:03:44.415110 - step 91 of 96 - 962 active elements (19038 deactivated)
18:34:18 DEBUG opendrift:2118: 0 elements scheduled.
18:34:18 DEBUG opendrift:2120: ======================================================================
18:34:18 DEBUG opendrift:2131: 60.25246791173796 <- latitude -> 60.33735367206825
18:34:18 DEBUG opendrift:2131: 4.891895878372277 <- longitude -> 4.983970017375232
18:34:18 DEBUG opendrift:2129: z = 0.0
18:34:18 DEBUG opendrift:2132: ---------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 962 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 962 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 962 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 962 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 962 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 962 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:18 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.19509 (min) 0.19509 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.83147 (min) 0.83147 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:18 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:18 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:18 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift:626: 333 elements hit land, moving them to the coastline.
18:34:18 DEBUG opendrift:1713: 333 elements scheduled for deactivation (stranded)
18:34:18 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:18 DEBUG opendrift:1733: Removed 333 elements.
18:34:18 DEBUG opendrift:1736: Removed 333 values from environment.
18:34:18 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:18 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:18 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:18 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:18 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.003164621732593731 and 0.5191505235689248 m/s
18:34:18 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:18 DEBUG opendrift:2111: ======================================================================
18:34:18 INFO opendrift:2112: 2025-07-01 17:18:44.415110 - step 92 of 96 - 629 active elements (19371 deactivated)
18:34:18 DEBUG opendrift:2118: 0 elements scheduled.
18:34:18 DEBUG opendrift:2120: ======================================================================
18:34:18 DEBUG opendrift:2131: 60.26948700635353 <- latitude -> 60.342355405677765
18:34:18 DEBUG opendrift:2131: 4.894769593183221 <- longitude -> 4.988017607350394
18:34:18 DEBUG opendrift:2129: z = 0.0
18:34:18 DEBUG opendrift:2132: ---------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 629 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 629 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 629 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 629 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:18 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:18 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:614: Data needed for 629 elements
18:34:18 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 629 elements
18:34:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:18 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:18 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:18 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:18 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:18 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:18 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.162895 (min) 0.162895 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.812847 (min) 0.812847 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:18 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:18 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:18 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:18 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:18 DEBUG opendrift:626: 246 elements hit land, moving them to the coastline.
18:34:18 DEBUG opendrift:1713: 246 elements scheduled for deactivation (stranded)
18:34:18 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:1733: Removed 246 elements.
18:34:19 DEBUG opendrift:1736: Removed 246 values from environment.
18:34:19 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:19 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:19 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:19 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:19 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.011138388642781258 and 0.6007170551308516 m/s
18:34:19 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:19 DEBUG opendrift:2111: ======================================================================
18:34:19 INFO opendrift:2112: 2025-07-01 17:33:44.415110 - step 93 of 96 - 383 active elements (19617 deactivated)
18:34:19 DEBUG opendrift:2118: 0 elements scheduled.
18:34:19 DEBUG opendrift:2120: ======================================================================
18:34:19 DEBUG opendrift:2131: 60.2751737528635 <- latitude -> 60.34952108320693
18:34:19 DEBUG opendrift:2131: 4.894900622042819 <- longitude -> 4.9931119083181175
18:34:19 DEBUG opendrift:2129: z = 0.0
18:34:19 DEBUG opendrift:2132: ---------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 383 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 383 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 383 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 383 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 383 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 383 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.130526 (min) 0.130526 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.793353 (min) 0.793353 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:19 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:19 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:19 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift:626: 158 elements hit land, moving them to the coastline.
18:34:19 DEBUG opendrift:1713: 158 elements scheduled for deactivation (stranded)
18:34:19 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:1733: Removed 158 elements.
18:34:19 DEBUG opendrift:1736: Removed 158 values from environment.
18:34:19 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:19 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:19 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:19 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:19 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.008625528964109612 and 0.7093539729858529 m/s
18:34:19 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:19 DEBUG opendrift:2111: ======================================================================
18:34:19 INFO opendrift:2112: 2025-07-01 17:48:44.415110 - step 94 of 96 - 225 active elements (19775 deactivated)
18:34:19 DEBUG opendrift:2118: 0 elements scheduled.
18:34:19 DEBUG opendrift:2120: ======================================================================
18:34:19 DEBUG opendrift:2131: 60.282717420877376 <- latitude -> 60.351081225246375
18:34:19 DEBUG opendrift:2131: 4.894756097605529 <- longitude -> 4.976379784830244
18:34:19 DEBUG opendrift:2129: z = 0.0
18:34:19 DEBUG opendrift:2132: ---------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 225 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 225 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 225 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 225 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 225 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 225 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0980171 (min) 0.0980171 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.77301 (min) 0.77301 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:19 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:19 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:19 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift:626: 70 elements hit land, moving them to the coastline.
18:34:19 DEBUG opendrift:1713: 70 elements scheduled for deactivation (stranded)
18:34:19 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:1733: Removed 70 elements.
18:34:19 DEBUG opendrift:1736: Removed 70 values from environment.
18:34:19 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:19 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:19 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:19 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:19 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.01960645690467423 and 0.4708328812908272 m/s
18:34:19 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:19 DEBUG opendrift:2111: ======================================================================
18:34:19 INFO opendrift:2112: 2025-07-01 18:03:44.415110 - step 95 of 96 - 155 active elements (19845 deactivated)
18:34:19 DEBUG opendrift:2118: 0 elements scheduled.
18:34:19 DEBUG opendrift:2120: ======================================================================
18:34:19 DEBUG opendrift:2131: 60.289663826940505 <- latitude -> 60.35103190804412
18:34:19 DEBUG opendrift:2131: 4.897515906023744 <- longitude -> 4.979461404251399
18:34:19 DEBUG opendrift:2129: z = 0.0
18:34:19 DEBUG opendrift:2132: ---------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 155 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 155 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 155 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 155 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 155 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 155 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0654031 (min) 0.0654031 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.75184 (min) 0.75184 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:19 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:19 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:19 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift:626: 56 elements hit land, moving them to the coastline.
18:34:19 DEBUG opendrift:1713: 56 elements scheduled for deactivation (stranded)
18:34:19 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:1733: Removed 56 elements.
18:34:19 DEBUG opendrift:1736: Removed 56 values from environment.
18:34:19 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:19 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:19 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:19 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:19 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.007399925388815132 and 0.46640679562131393 m/s
18:34:19 DEBUG opendrift:846: to be seeded: 0, already seeded 20000
18:34:19 DEBUG opendrift:2111: ======================================================================
18:34:19 INFO opendrift:2112: 2025-07-01 18:18:44.415110 - step 96 of 96 - 99 active elements (19901 deactivated)
18:34:19 DEBUG opendrift:2118: 0 elements scheduled.
18:34:19 DEBUG opendrift:2120: ======================================================================
18:34:19 DEBUG opendrift:2131: 60.295997247199786 <- latitude -> 60.35703455906534
18:34:19 DEBUG opendrift:2131: 4.906495640167651 <- longitude -> 4.976021110240112
18:34:19 DEBUG opendrift:2129: z = 0.0
18:34:19 DEBUG opendrift:2132: ---------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['x_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 99 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader covering 99 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking x_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['y_sea_water_velocity']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader oscillating_reader_0
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 99 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from oscillating_reader_0 covering 99 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking y_sea_water_velocity for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 99 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 99 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_sea_water_velocity: 0.0327191 (min) 0.0327191 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_sea_water_velocity: 0.729864 (min) 0.729864 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: x_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: y_wind: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: upward_sea_water_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_x_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_stokes_drift_y_velocity: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_period_at_variance_spectral_density_maximum: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_peak_period_from_variance_spectral_density: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_swell_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_to_direction: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_mean_period: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_surface_wind_wave_significant_height: 0 (min) 0 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: ocean_mixed_layer_thickness: 50 (min) 50 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: sea_floor_depth_below_sea_level: 10000 (min) 10000 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:19 DEBUG opendrift.models.physics_methods:810: Calculating Hs from wind, min: 0.000000, mean: 0.000000, max: 0.000000
18:34:19 DEBUG opendrift.models.physics_methods:868: Calculating wave period Tm02 from wind
18:34:19 DEBUG opendrift.models.physics_methods:878: min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift.models.physics_methods:818: Calculating wave period from wind, min: 1.256637, mean: 1.256637, max: 1.256637
18:34:19 DEBUG opendrift:626: 39 elements hit land, moving them to the coastline.
18:34:19 DEBUG opendrift:1713: 39 elements scheduled for deactivation (stranded)
18:34:19 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:1733: Removed 39 elements.
18:34:19 DEBUG opendrift:1736: Removed 39 values from environment.
18:34:19 DEBUG opendrift:2166: Calling OceanDrift.update()
18:34:19 DEBUG opendrift.models.physics_methods:722: No wind for wind-sheared ocean drift
18:34:19 DEBUG opendrift.models.physics_methods:750: No Stokes drift velocity available
18:34:19 DEBUG opendrift.models.oceandrift:313: Vertical advection, excluding elements at surface
18:34:19 DEBUG opendrift:1691: Moving elements according to horizontal diffusivity of 10.0, with speeds between 0.0036460707565725728 and 0.351805971324279 m/s
18:34:19 DEBUG opendrift:2204: Cleaning up
18:34:19 DEBUG opendrift.models.basemodel.environment:591: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:592: Variable group ['land_binary_mask']
18:34:19 DEBUG opendrift.models.basemodel.environment:593: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:597: Calling reader global_landmask
18:34:19 DEBUG opendrift.models.basemodel.environment:598: ----------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:614: Data needed for 60 elements
18:34:19 DEBUG opendrift.readers.basereader.variables:761: Fetching variables from global_landmask covering 60 elements
18:34:19 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
18:34:19 DEBUG opendrift.readers.basereader.variables:639: Checking land_binary_mask for invalid values
18:34:19 DEBUG opendrift.readers.basereader.variables:797: Reader projection is latlon - rotation of vectors is not needed.
18:34:19 DEBUG opendrift.models.basemodel.environment:748: Obtained data for all elements.
18:34:19 DEBUG opendrift.models.basemodel.environment:761: ---------------------------------------
18:34:19 DEBUG opendrift.models.basemodel.environment:762: Finished processing all variable groups
18:34:19 DEBUG opendrift.models.basemodel.environment:887: ------------ SUMMARY -------------
18:34:19 DEBUG opendrift.models.basemodel.environment:889: land_binary_mask: 0 (min) 1 (max)
18:34:19 DEBUG opendrift.models.basemodel.environment:891: ---------------------------------
18:34:19 DEBUG opendrift:626: 21 elements hit land, moving them to the coastline.
18:34:19 DEBUG opendrift:1713: 21 elements scheduled for deactivation (stranded)
18:34:19 DEBUG opendrift:1715: (z: 0.000000 to 0.000000)
18:34:19 DEBUG opendrift:2290: Updating minval and maxval
18:34:19 DEBUG opendrift:2370: Writing to file
18:34:19 DEBUG opendrift.export.io_netcdf:33: Initialising output netCDF file simulation.nc with 49 timesteps
18:34:20 DEBUG opendrift:2215: Finalising and closing output file: simulation.nc
18:34:20 DEBUG opendrift.export.io_netcdf:67: Closing netCDF-file
18:34:20 DEBUG opendrift.export.io_netcdf:77: Closed netCDF-file
18:34:20 DEBUG gribapi.bindings:58: eccodes lib search: trying to find binary wheel
18:34:20 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes.libs
18:34:20 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes/.dylibs
18:34:20 DEBUG gribapi.bindings:65: eccodes lib search: looking in /opt/conda/envs/opendrift/lib/python3.13/site-packages/eccodes
18:34:20 DEBUG gribapi.bindings:91: eccodes lib search: did not find library from wheel; try to find as separate lib
18:34:20 DEBUG gribapi.bindings:99: eccodes lib search: findlibs returned /opt/conda/envs/opendrift/lib/libeccodes.so
18:34:20 DEBUG opendrift.export.io_netcdf:90: Making netCDF file CDM compliant with fixed dimensions, and compressing with {'zlib': True, 'complevel': 6}
18:34:22 DEBUG opendrift:1733: Removed 21 elements.
18:34:22 DEBUG opendrift:1736: Removed 21 values from environment.
18:34:22 DEBUG opendrift:100: Changed mode from Mode.Run to Mode.Result
Opening the output file lazily with Xarray. This will work even if the file is too large to fit in memory, as it will read and process data chuck-by-chunk directly from file using Dask. (See also example_river_runoff.py) See also TrajAn: https://opendrift.github.io/trajan/
oa = opendrift.open(outfile)
18:34:23 DEBUG opendrift.models.oceandrift:116: No machine learning correction available.
18:34:23 DEBUG opendrift.config:168: Adding 42 config items from environment
18:34:23 DEBUG opendrift.config:168: Adding 5 config items from environment
18:34:23 DEBUG opendrift.config:168: Adding 18 config items from __init__
18:34:23 DEBUG opendrift.config:178: Overwriting config item readers:max_number_of_fails
18:34:23 DEBUG opendrift.config:168: Adding 5 config items from __init__
18:34:23 INFO opendrift:513: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-74-gdbb4afa)
18:34:23 DEBUG opendrift.config:168: Adding 17 config items from oceandrift
18:34:23 DEBUG opendrift.config:178: Overwriting config item seed:z
18:34:23 DEBUG opendrift.export.io_netcdf:105: Importing from simulation.nc
18:34:23 DEBUG opendrift:1733: Removed 19961 elements.
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:x_sea_water_velocity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:x_sea_water_velocity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:y_sea_water_velocity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:y_sea_water_velocity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_height -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_height -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:x_wind -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:x_wind -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:y_wind -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:y_wind -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:upward_sea_water_velocity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:upward_sea_water_velocity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:ocean_vertical_diffusivity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:ocean_vertical_diffusivity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wave_significant_height -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wave_significant_height -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_x_velocity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_x_velocity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wave_stokes_drift_y_velocity -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wave_stokes_drift_y_velocity -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wave_period_at_variance_spectral_density_maximum -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wave_period_at_variance_spectral_density_maximum -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_swell_wave_to_direction -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_swell_wave_to_direction -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_swell_wave_peak_period_from_variance_spectral_density -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_swell_wave_peak_period_from_variance_spectral_density -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_swell_wave_significant_height -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_swell_wave_significant_height -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wind_wave_to_direction -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wind_wave_to_direction -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wind_wave_mean_period -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wind_wave_mean_period -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_surface_wind_wave_significant_height -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_surface_wind_wave_significant_height -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:ocean_mixed_layer_thickness -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:ocean_mixed_layer_thickness -> 50
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:sea_floor_depth_below_sea_level -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:sea_floor_depth_below_sea_level -> 10000
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:constant:land_binary_mask -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: environment:fallback:land_binary_mask -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:use_auto_landmask -> True
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:current_uncertainty -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:current_uncertainty_uniform -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:max_speed -> 2.0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: readers:max_number_of_fails -> 1
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:simulation_name ->
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:coastline_action -> stranding
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:coastline_approximation_precision -> 0.001
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:time_step_minutes -> 60
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:time_step_output_minutes -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:ocean_only -> True
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:number -> 1
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:max_age_seconds -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:advection_scheme -> euler
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:horizontal_diffusivity -> 10.0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:profiles_depth -> 50
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:wind_uncertainty -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:relative_wind -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:deactivate_north_of -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:deactivate_south_of -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:deactivate_east_of -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:deactivate_west_of -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:origin_marker -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:z -> 0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:wind_drift_factor -> 0.02
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:current_drift_factor -> 1
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:terminal_velocity -> 0.0
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:vertical_advection -> True
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:vertical_advection_at_surface -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:vertical_mixing -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:vertical_mixing_at_surface -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: vertical_mixing:timestep -> 60
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: vertical_mixing:diffusivitymodel -> environment
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: vertical_mixing:background_diffusivity -> 1.2e-05
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: vertical_mixing:TSprofiles -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:wind_drift_depth -> 0.1
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:stokes_drift -> True
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:stokes_drift_profile -> Phillips
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:use_tabularised_stokes_drift -> False
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:tabularised_stokes_drift_fetch -> 25000
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: general:seafloor_action -> lift_to_seafloor
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: drift:truncate_ocean_model_below_m -> None
18:34:23 DEBUG opendrift.export.io_netcdf:150: Setting imported config: seed:seafloor -> False
18:34:23 INFO opendrift:82: Returning <class 'opendrift.models.oceandrift.OceanDrift'> object
Calculating histogram The histogram may be stored/cached to a netCDF file for later re-use, as the calculation may be time consuming for huge output files.
h = oa.get_histogram(pixelsize_m=500)
18:34:23 INFO opendrift:3910: calculating for origin_marker 0...
18:34:23 INFO opendrift:3910: calculating for origin_marker 1...
Plot the cumulative coverage of first seeding (origin_marker=0)
b=h.isel(origin_marker=0).sum(dim='time')
oa.plot(background=b.where(b>0), fast=True, show_elements=False, vmin=0, vmax=1000, clabel='First seeding')
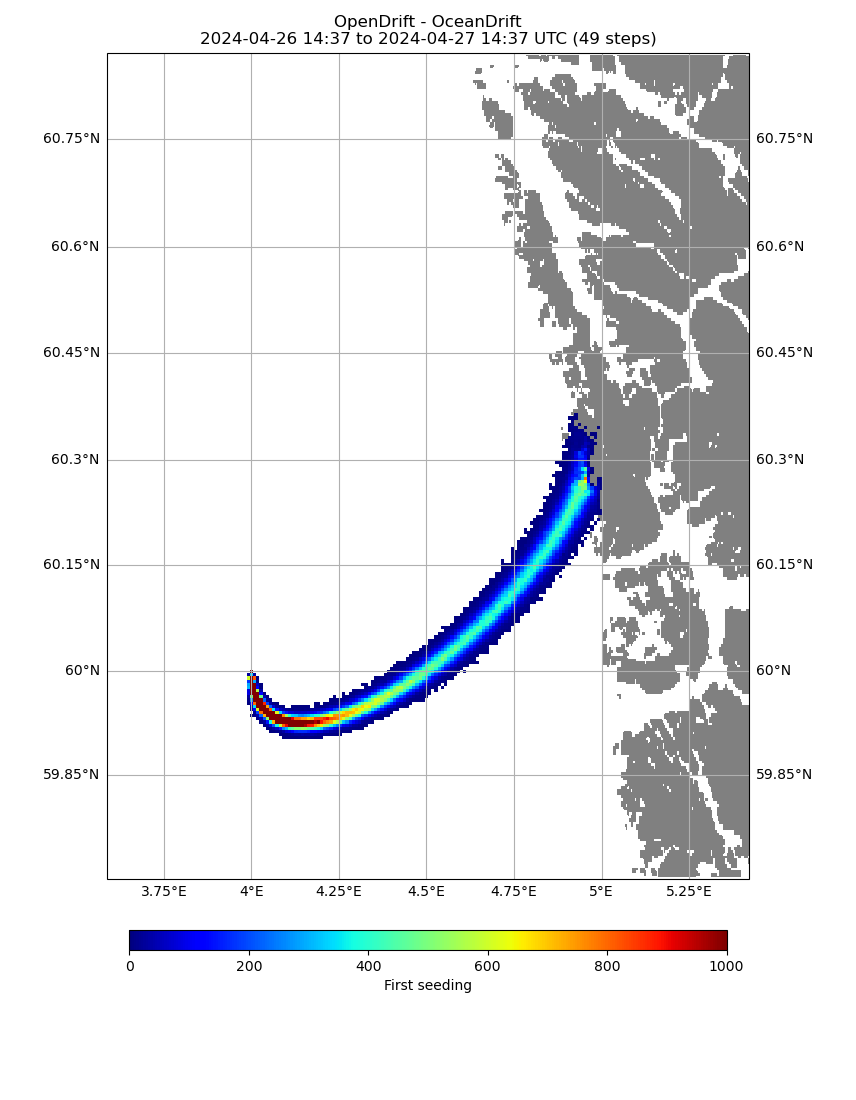
18:34:23 DEBUG opendrift:2437: Setting up map: corners=None, fast=True, lscale=None
18:34:23 WARNING opendrift:2468: Plotting fast. This will make your plots less accurate.
(<GeoAxes: title={'center': 'OpenDrift - OceanDrift\n2025-06-30 18:33 to 2025-07-01 18:33 UTC (49 steps)'}>, <Figure size 858.236x1100 with 2 Axes>)
Making two animations, for each of the two seedings / origin_markers. The calculated density fields may be stored/cached to a netCDF file for later re-use, as their calculation may be time consuming for huge output files. Note that other analysis/plotting methods are not yet adapted to datasets opened lazily with open_xarray
for om in [0, 1]:
background=h.isel(origin_marker=om)
oa.animation(background=background.where(background>0), bgalpha=1,
corners=[4.0, 6, 59.5, 61], fast=False, show_elements=False, vmin=0, vmax=200)
# Cleaning up
os.remove(outfile)
18:35:23 DEBUG opendrift:2437: Setting up map: corners=[4.0, 6, 59.5, 61], fast=False, lscale=None
18:35:23 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 1) with Cartopy shapereader...
18:35:36 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 5) with Cartopy shapereader...
18:35:36 DEBUG opendrift.readers.reader_global_landmask:84: Loading shapes ('h' level 6) with Cartopy shapereader...
18:35:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
/opt/conda/envs/opendrift/lib/python3.13/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'vmin', 'vmax' will be ignored
result = super().scatter(*args, **kwargs)
18:35:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:37 DEBUG opendrift:3078: Saving animation..
18:35:37 INFO opendrift:4630: Saving animation to /root/project/docs/source/gallery/animations/example_huge_output_0.gif...
18:35:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:38 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:38 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:38 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:39 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:40 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:41 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:42 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:43 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:44 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:44 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:44 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:45 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:46 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:47 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:48 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:49 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:50 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:50 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:50 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:51 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:52 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:53 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:54 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:55 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:56 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:57 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:58 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:35:59 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:00 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:01 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:02 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:03 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:04 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:05 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:08 DEBUG opendrift:4668: MPLBACKEND = agg
18:36:08 DEBUG opendrift:4669: DISPLAY = None
18:36:08 DEBUG opendrift:4670: Time to save animation: 0:00:31.354792
18:36:08 INFO opendrift:3071: Time to make animation: 0:00:45.948984
18:36:08 DEBUG opendrift:2437: Setting up map: corners=[4.0, 6, 59.5, 61], fast=False, lscale=None
18:36:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
/opt/conda/envs/opendrift/lib/python3.13/site-packages/cartopy/mpl/geoaxes.py:1692: UserWarning: No data for colormapping provided via 'c'. Parameters 'vmin', 'vmax' will be ignored
result = super().scatter(*args, **kwargs)
18:36:09 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:09 DEBUG opendrift:3078: Saving animation..
18:36:10 INFO opendrift:4630: Saving animation to /root/project/docs/source/gallery/animations/example_huge_output_1.gif...
18:36:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:10 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:11 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:12 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:13 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:14 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:15 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:16 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:17 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:18 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:19 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:20 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:21 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:22 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:23 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:24 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:25 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:26 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:27 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:28 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:29 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:30 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:31 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:32 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:33 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:34 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:35 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:36 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:37 DEBUG opendrift.readers.reader_global_landmask:123: Adding GSHHG shapes from cartopy, scale: h, extent: (3.999999999999999, 6.0, 59.5, 60.99999999999999)..
18:36:40 DEBUG opendrift:4668: MPLBACKEND = agg
18:36:40 DEBUG opendrift:4669: DISPLAY = None
18:36:40 DEBUG opendrift:4670: Time to save animation: 0:00:30.707432
18:36:40 INFO opendrift:3071: Time to make animation: 0:00:32.002226
First seeding
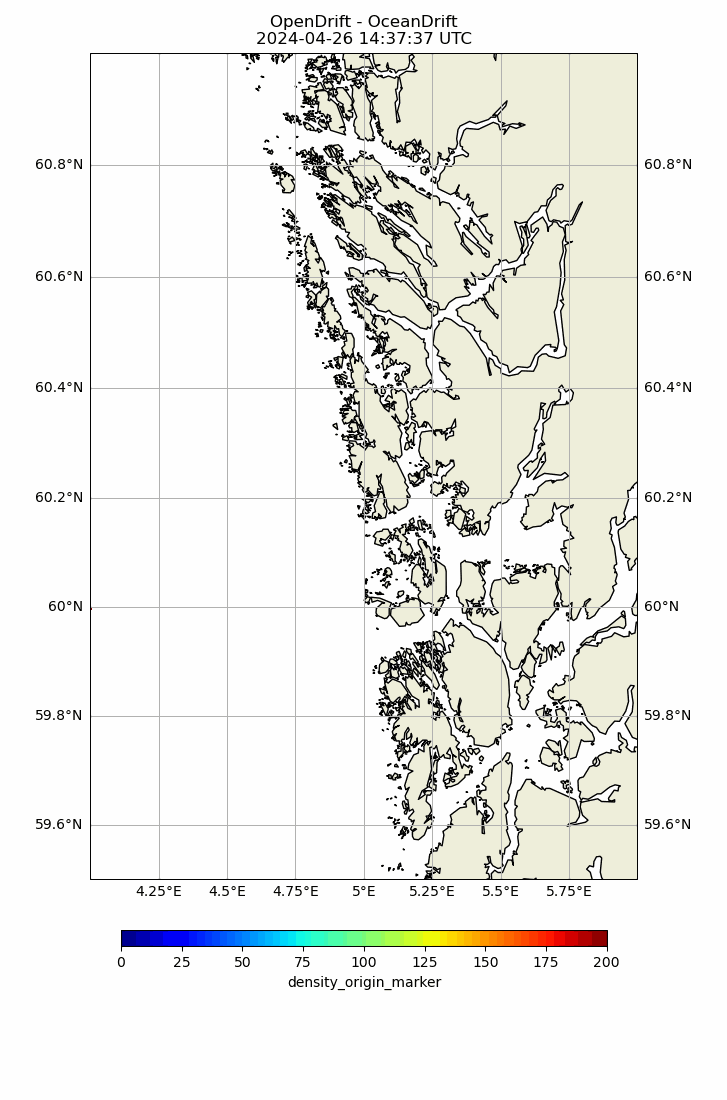
Second seeding
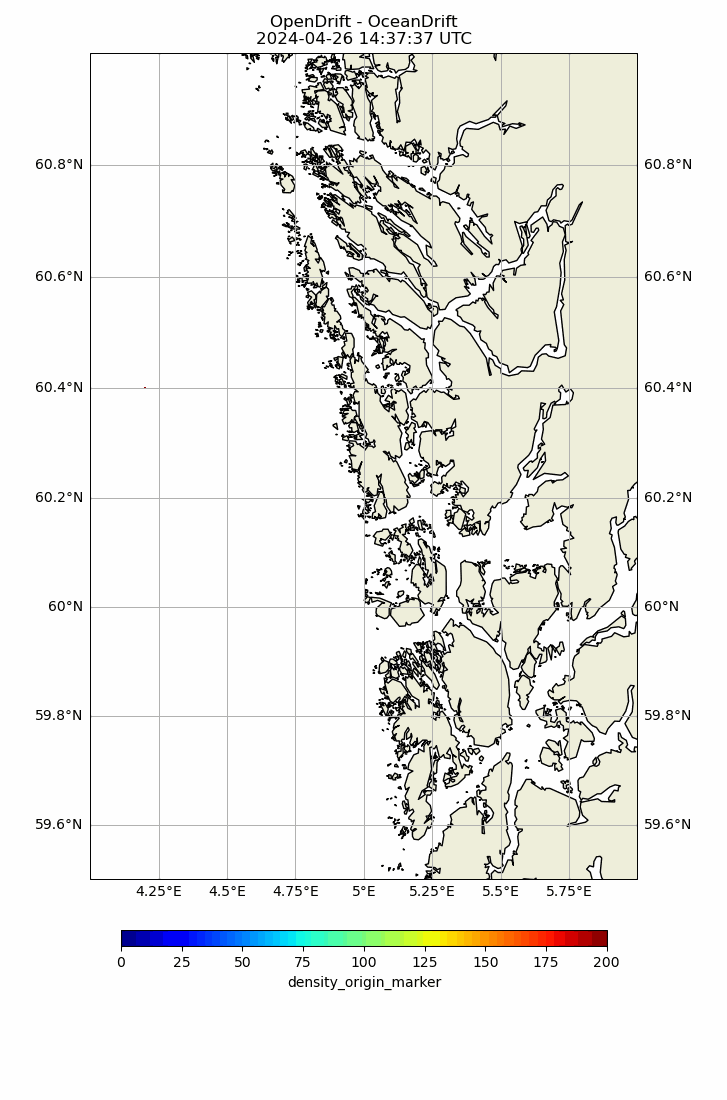
Total running time of the script: (3 minutes 1.522 seconds)