Note
Go to the end to download the full example code.
Plotting wave spectra data, using the accessor syntax#
from pathlib import Path
from trajan.readers.omb import read_omb_csv
import coloredlogs
import datetime
import matplotlib.pyplot as plt
# adjust the level of information printed
# coloredlogs.install(level='error')
coloredlogs.install(level='debug')
# load the data from an example file with several buoys and a bit of wave spectra data
path_to_test_data = Path.cwd().parent / "tests" / "test_data" / "csv" / "omb3.csv"
xr_data = read_omb_csv(path_to_test_data)
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG reading /home/runner/work/trajan/trajan/tests/test_data/csv/omb3.csv..
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG omb_dataframe at index 94 is:
Date Time (UTC) 16/Jun/2022 18:27:19
Device drifter_2
Direction MO
Payload NaN
Approx Lat/Lng 74.36745,3.3274
Payload (Text) NaN
Length (Bytes) 0
Credits 1
Name: 94, dtype: object
this is empty (Length (Bytes) is 0), drop
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG start applying sliding_filter_nsigma
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG done applying sliding_filter_nsigma
/home/runner/micromamba/envs/trajan/lib/python3.11/site-packages/xarray/core/indexing.py:1559: UserWarning: no explicit representation of timezones available for np.datetime64
array[key] = value
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG start applying sliding_filter_nsigma
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG done applying sliding_filter_nsigma
/home/runner/micromamba/envs/trajan/lib/python3.11/site-packages/xarray/core/indexing.py:1559: UserWarning: no explicit representation of timezones available for np.datetime64
array[key] = value
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG start applying sliding_filter_nsigma
2025-04-07 09:28:12 fv-az1340-240 trajan.readers.omb[3337] DEBUG done applying sliding_filter_nsigma
/home/runner/micromamba/envs/trajan/lib/python3.11/site-packages/xarray/core/indexing.py:1559: UserWarning: no explicit representation of timezones available for np.datetime64
array[key] = value
2025-04-07 09:28:12 fv-az1340-240 trajan.accessor[3337] DEBUG Detecting trajectory dimension
2025-04-07 09:28:12 fv-az1340-240 trajan.accessor[3337] DEBUG Detecting time-variable for "obs"..
2025-04-07 09:28:12 fv-az1340-240 trajan.accessor[3337] DEBUG Detected obs-dim: obs, detected time-variable: time.
2025-04-07 09:28:12 fv-az1340-240 trajan.accessor[3337] DEBUG Detected un-structured (2D) trajectory dataset
2025-04-07 09:28:12 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:28:12 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:28:12 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:28:12 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
# if no axis is provided, an axis will be generated automatically
# by default, we "decorate", i.e. label axis etc
xr_data.isel(trajectory=0).processed_elevation_energy_spectrum.wave.plot(
xr_data.isel(trajectory=0).time_waves_imu.squeeze(),
)
plt.show()
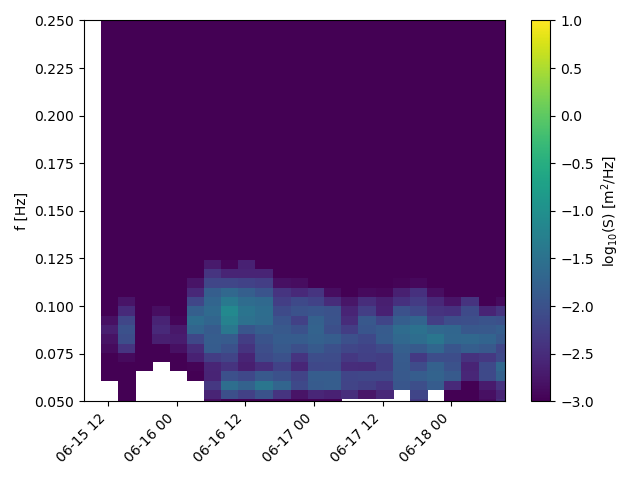
2025-04-07 09:28:12 fv-az1340-240 trajan.waves[3337] DEBUG Setting up new plot object.
2025-04-07 09:28:12 fv-az1340-240 matplotlib.colorbar[3337] DEBUG locator: <matplotlib.ticker.AutoLocator object at 0x7efc26f1ff50>
# it is also possible to provide an axis on which to plot
# in this case, this will likely be part of a larger figure, and the user will likely want to put the
# labels etc themselves; remember to switch off decoration
# a plot with 3 lines, 2 columns
fig, ax = plt.subplots(3, 2)
ax_out, pclr = xr_data.isel(trajectory=0).processed_elevation_energy_spectrum.wave.plot(
xr_data.isel(trajectory=0).time_waves_imu.squeeze(),
# plot on the second line, first column
ax=ax[1, 0],
decorate=False,
)
# the user can make the plot to their liking
ax[1, 0].set_xticks(ax[1, 0].get_xticks(), ax[1, 0].get_xticklabels(), rotation=45, ha='right')
ax[1,0].set_ylim([0.05, 0.25])
ax[1,0].set_ylabel("f [Hz]")
plt.tight_layout()
cbar = plt.colorbar(pclr, ax=ax, orientation='vertical', shrink=0.8)
cbar.set_label('log$_{10}$(S) [m$^2$/Hz]')
plt.show()
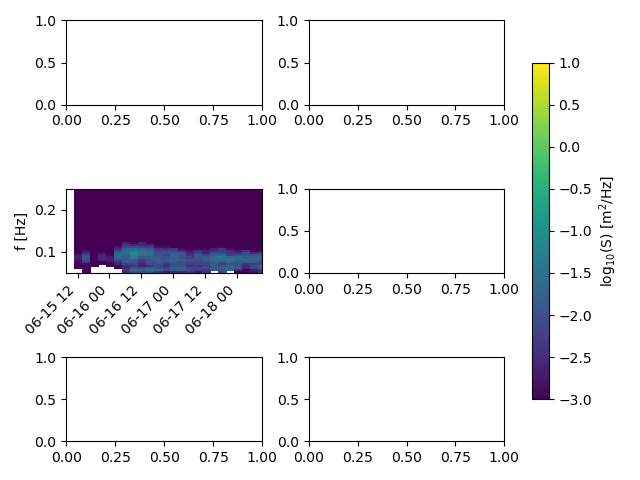
2025-04-07 09:28:13 fv-az1340-240 trajan.waves[3337] DEBUG Setting up new plot object.
2025-04-07 09:28:13 fv-az1340-240 matplotlib.colorbar[3337] DEBUG locator: <matplotlib.ticker.AutoLocator object at 0x7efc26a4c250>
Total running time of the script: (0 minutes 0.633 seconds)