Note
Go to the end to download the full example code.
Analysing output from Parcels (Zarr format)
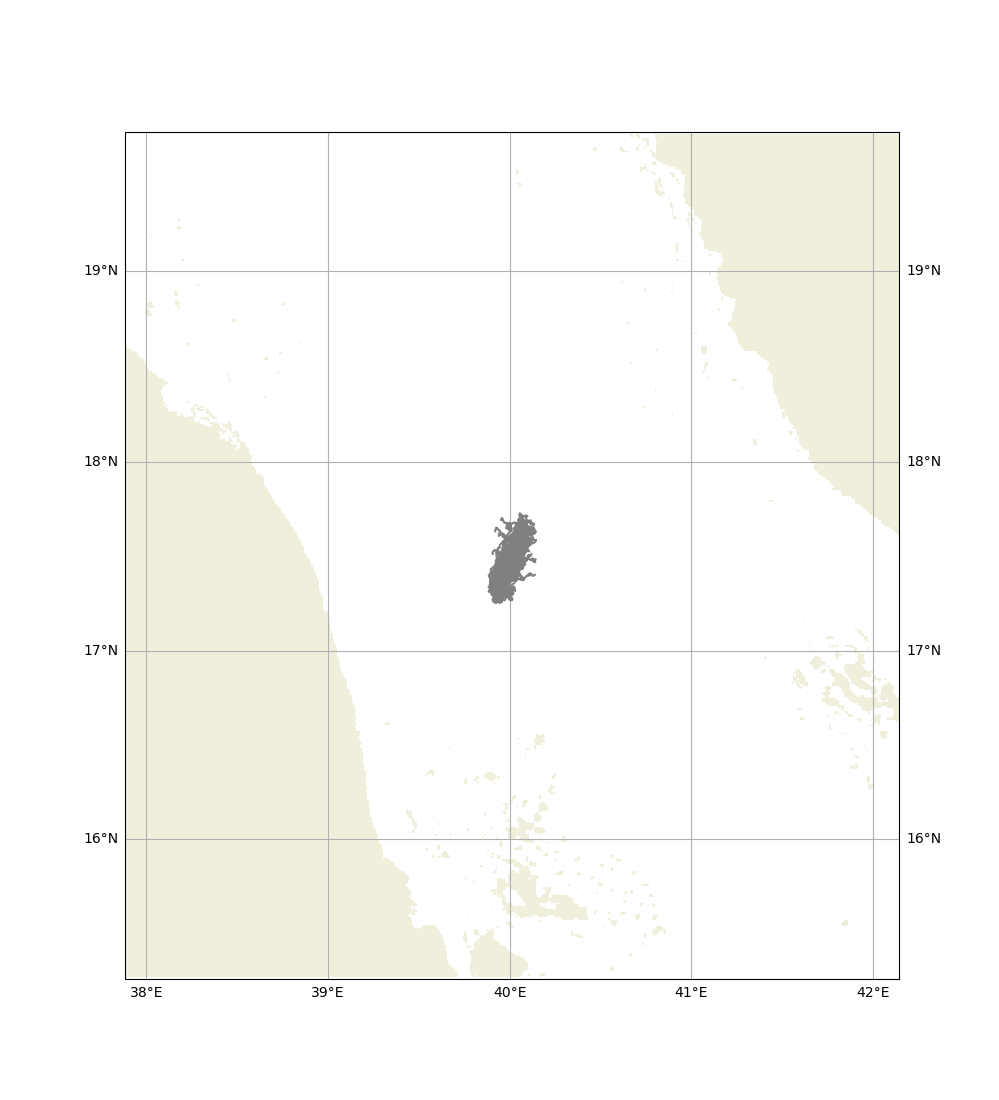
<xarray.Dataset> Size: 123kB
Dimensions: (trajectory: 100, obs: 61)
Coordinates:
* obs (obs) int32 244B 0 1 2 3 4 5 6 7 8 ... 53 54 55 56 57 58 59 60
* trajectory (trajectory) int64 800B 700 701 702 703 704 ... 796 797 798 799
Data variables:
lat (trajectory, obs) float32 24kB ...
lon (trajectory, obs) float32 24kB ...
time (trajectory, obs) datetime64[ns] 49kB ...
z (trajectory, obs) float32 24kB ...
Attributes:
Conventions: CF-1.6/CF-1.7
feature_type: trajectory
ncei_template_version: NCEI_NetCDF_Trajectory_Template_v2.0
parcels_mesh: spherical
parcels_version: 2.4.0
import matplotlib.pyplot as plt
import xarray as xr
import trajan as ta
ds = xr.open_dataset('../tests/test_data/parcels.zarr', engine='zarr')
print(ds)
ds.traj.plot(land='mask', margin=2)
#ds.mean('trajectory', skipna=True).traj.plot(color='r', label='Mean trajectory')
plt.show()
Total running time of the script: (0 minutes 5.154 seconds)