Note
Go to the end to download the full example code.
Reading and using a contiguous ragged dataset#
import matplotlib.pyplot as plt
from pathlib import Path
import xarray as xr
import trajan
import coloredlogs
# the test data: a small extract from the Sofar dataset https://sofar-spotter-archive.s3.amazonaws.com/index.html
path_to_test_data = Path.cwd().parent / "tests" / "test_data" / "xr_spotter_bulk_test_data.nc"
xr_data = xr.load_dataset(path_to_test_data)
# this is a ragged contiguous dataset; the data variables are 1D arrays
xr_data
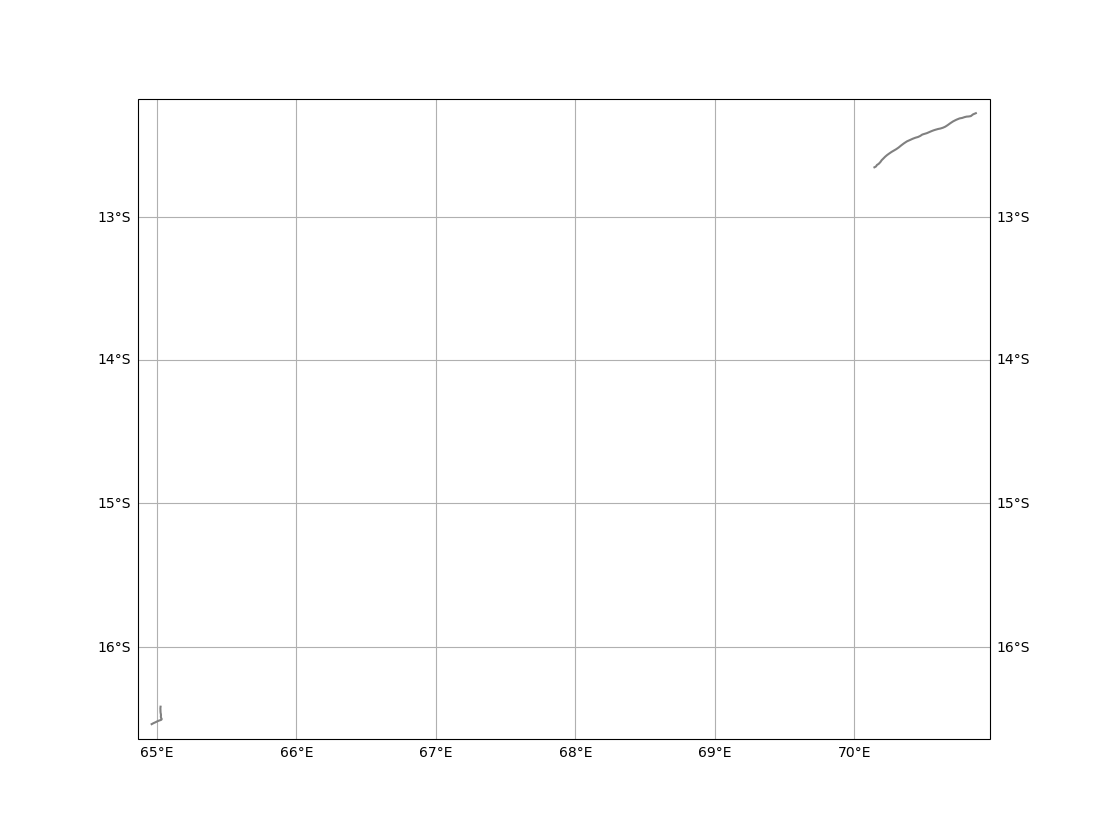
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG Detecting trajectory dimension
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG 1D storage dataset; detected: obs_dim = 'index', timecoord = 'time', trajectory_dim = 'trajectory', rowsize
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG Detecting trajectory dimension
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG Detecting time-variable for "obs"..
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG Detected obs-dim: obs, detected time-variable: time.
2025-04-07 09:25:37 fv-az1340-240 trajan.accessor[3337] DEBUG Detected un-structured (2D) trajectory dataset
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG Setting up new plot object.
2025-04-07 09:25:37 fv-az1340-240 trajan.plot[3337] DEBUG Plotting lines
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.plot[3337] DEBUG Creating new figure and axes..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
2025-04-07 09:25:37 fv-az1340-240 trajan.traj[3337] DEBUG No grid-mapping specified, checking if coordinates are lon/lat..
/home/runner/micromamba/envs/trajan/lib/python3.11/site-packages/shapely/creation.py:218: RuntimeWarning: invalid value encountered in linestrings
return lib.linestrings(coords, np.intc(handle_nan), out=out, **kwargs)
# it is possible to get a 2d-array dataset version using trajan:
# compare:
print(f"{xr_data = }")
# with:
xr_data_as_2darray = xr_data.traj.to_2d()
print(f"{xr_data_as_2darray = }")
# naturally, the 2darray version can be dumped to disk if you want:
xr_data_as_2darray.to_netcdf("./xr_spotter_bulk_test_data_as_2darray.nc")
xr_data = <xarray.Dataset> Size: 5kB
Dimensions: (index: 65, trajectory: 2)
Coordinates:
time (index) datetime64[ns] 520B 2022-03-15T04:07:25 .....
* trajectory (trajectory) <U11 88B 'SPOT-010102' 'SPOT-010103'
Dimensions without coordinates: index
Data variables:
significantWaveHeight (index) float64 520B 1.736 1.817 ... 1.263 1.136
peakPeriod (index) float64 520B 7.877 8.533 ... 7.314 7.314
meanPeriod (index) float64 520B 6.804 6.652 ... 6.629 6.497
peakDirection (index) float64 520B 147.9 155.5 ... 105.2 102.2
peakDirectionalSpread (index) float64 520B 30.37 25.48 26.04 ... 37.2 38.09
meanDirection (index) float64 520B 146.7 144.5 ... 127.3 120.8
meanDirectionalSpread (index) float64 520B 40.34 40.47 ... 50.39 51.12
latitude (index) float64 520B -16.41 -16.43 ... -12.64 -12.65
longitude (index) float64 520B 65.03 65.03 ... 70.15 70.14
rowsize (trajectory) int64 16B 20 45
Attributes:
title: Sofar Spotter Data Archive - Bulk Wave Parameters
institution: Sofar Ocean
source: Spotter wave buoy
creation_date: 2023-10-18 00:43:55.333537
author: Isabel A. Houghton
email: isabel.houghton@sofarocean.com
references: https://content.sofarocean.com/hubfs/Spotter%20product%20...
modified: modified to only keep a couple of buoys, to be used as a ...
xr_data_as_2darray = <xarray.Dataset> Size: 7kB
Dimensions: (trajectory: 2, obs: 45)
Coordinates:
* trajectory (trajectory) <U11 88B 'SPOT-010102' 'SPOT-010103'
Dimensions without coordinates: obs
Data variables:
time (trajectory, obs) datetime64[ns] 720B 2022-03-15T0...
significantWaveHeight (trajectory, obs) float64 720B 1.736 1.817 ... 1.136
peakPeriod (trajectory, obs) float64 720B 7.877 8.533 ... 7.314
meanPeriod (trajectory, obs) float64 720B 6.804 6.652 ... 6.497
peakDirection (trajectory, obs) float64 720B 147.9 155.5 ... 102.2
peakDirectionalSpread (trajectory, obs) float64 720B 30.37 25.48 ... 38.09
meanDirection (trajectory, obs) float64 720B 146.7 144.5 ... 120.8
meanDirectionalSpread (trajectory, obs) float64 720B 40.34 40.47 ... 51.12
lat (trajectory, obs) float64 720B -16.41 ... -12.65
lon (trajectory, obs) float64 720B 65.03 65.03 ... 70.14
Attributes:
title: Sofar Spotter Data Archive - Bulk Wave Parameters
institution: Sofar Ocean
source: Spotter wave buoy
creation_date: 2023-10-18 00:43:55.333537
author: Isabel A. Houghton
email: isabel.houghton@sofarocean.com
references: https://content.sofarocean.com/hubfs/Spotter%20product%...
modified: modified to only keep a couple of buoys, to be used as ...
trajan_modified: this was initially a contiguous ragged Dataset, which w...
Total running time of the script: (0 minutes 4.168 seconds)