Note
Go to the end to download the full example code.
Ship drift
from datetime import datetime
from opendrift.models.shipdrift import ShipDrift
o = ShipDrift(loglevel=20)
o.add_readers_from_list([
'https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml',
'https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc',
'https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml'
])
07:27:33 INFO opendrift:513: OpenDriftSimulation initialised (version 1.14.2 / v1.14.2-90-g1dd1995)
Seed ship elements at defined position and time Note: beam/length ratio is larger than allowed, but is then clipped internally
o.seed_elements(lon=5.0, lat=63.0, radius=1000, number=1000,
time=datetime.now(),
length=80.0, beam=20.0, height=9.0, draft=4.0)
07:27:33 WARNING opendrift.models.shipdrift:181: Ratio of beam to length should be in range 0.12 to 0.18, given range is 0.25-0.25. Using border value.
07:27:33 INFO opendrift.models.basemodel.environment:206: Adding a global landmask from GSHHG
07:27:37 INFO opendrift.models.basemodel.environment:229: Fallback values will be used for the following variables which have no readers:
07:27:37 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_x_velocity: 0.000000
07:27:37 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_stokes_drift_y_velocity: 0.000000
07:27:37 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_significant_height: 0.000000
07:27:37 INFO opendrift.models.basemodel.environment:232: sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment: 0.000000
Running model
o.run(steps=24, stop_on_error=True)
07:27:37 INFO opendrift:1743: Storing previous values of element property lon because of condition (('general:coastline_action', 'in', ['stranding', 'previous']), 'or', ('general:seafloor_action', 'in', ['previous']))
07:27:37 INFO opendrift:1743: Storing previous values of element property lat because of condition (('general:coastline_action', 'in', ['stranding', 'previous']), 'or', ('general:seafloor_action', 'in', ['previous']))
07:27:37 INFO opendrift:899: Using existing reader for land_binary_mask
07:27:37 INFO opendrift:928: All points are in ocean
07:27:37 INFO opendrift:2035: 2025-07-17 07:27:33.413417 - step 1 of 24 - 1000 active elements (0 deactivated)
07:27:37 INFO opendrift.readers:61: Opening file with xr.open_dataset
07:27:39 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'x': 'x', 'y': 'y', 'time': 'time'}
07:27:39 INFO opendrift.readers:61: Opening file with xr.open_dataset
07:27:40 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'time': 'time', 'x': 'x', 'y': 'y'}
07:27:40 INFO opendrift.readers:61: Opening file with xr.open_dataset
07:27:42 INFO opendrift.readers.reader_netCDF_CF_generic:332: Detected dimensions: {'y': 'rlat', 'x': 'rlon', 'time': 'time'}
07:27:43 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:43 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:44 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:44 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:45 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:45 INFO opendrift:2035: 2025-07-17 08:27:33.413417 - step 2 of 24 - 1000 active elements (0 deactivated)
07:27:46 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:46 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:46 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:46 INFO opendrift:2035: 2025-07-17 09:27:33.413417 - step 3 of 24 - 1000 active elements (0 deactivated)
07:27:47 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:47 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:48 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:48 INFO opendrift:2035: 2025-07-17 10:27:33.413417 - step 4 of 24 - 1000 active elements (0 deactivated)
07:27:49 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:49 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:50 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:50 INFO opendrift:2035: 2025-07-17 11:27:33.413417 - step 5 of 24 - 1000 active elements (0 deactivated)
07:27:51 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:51 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:52 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:52 INFO opendrift:2035: 2025-07-17 12:27:33.413417 - step 6 of 24 - 1000 active elements (0 deactivated)
07:27:53 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:53 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:54 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:54 INFO opendrift:2035: 2025-07-17 13:27:33.413417 - step 7 of 24 - 1000 active elements (0 deactivated)
07:27:55 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:55 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:56 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:56 INFO opendrift:2035: 2025-07-17 14:27:33.413417 - step 8 of 24 - 1000 active elements (0 deactivated)
07:27:57 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:57 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:57 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:57 INFO opendrift:2035: 2025-07-17 15:27:33.413417 - step 9 of 24 - 1000 active elements (0 deactivated)
07:27:58 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:58 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:27:59 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:27:59 INFO opendrift:2035: 2025-07-17 16:27:33.413417 - step 10 of 24 - 1000 active elements (0 deactivated)
07:28:00 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:00 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:01 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:01 INFO opendrift:2035: 2025-07-17 17:27:33.413417 - step 11 of 24 - 1000 active elements (0 deactivated)
07:28:02 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:02 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:03 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:03 INFO opendrift:2035: 2025-07-17 18:27:33.413417 - step 12 of 24 - 1000 active elements (0 deactivated)
07:28:04 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:04 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:05 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:05 INFO opendrift:2035: 2025-07-17 19:27:33.413417 - step 13 of 24 - 1000 active elements (0 deactivated)
07:28:06 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:06 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:07 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:07 INFO opendrift:2035: 2025-07-17 20:27:33.413417 - step 14 of 24 - 1000 active elements (0 deactivated)
07:28:08 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:08 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:09 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:09 INFO opendrift:2035: 2025-07-17 21:27:33.413417 - step 15 of 24 - 1000 active elements (0 deactivated)
07:28:10 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:10 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:10 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:10 INFO opendrift:2035: 2025-07-17 22:27:33.413417 - step 16 of 24 - 1000 active elements (0 deactivated)
07:28:12 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:12 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:12 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:12 INFO opendrift:2035: 2025-07-17 23:27:33.413417 - step 17 of 24 - 1000 active elements (0 deactivated)
07:28:14 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:14 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:15 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:15 INFO opendrift:2035: 2025-07-18 00:27:33.413417 - step 18 of 24 - 1000 active elements (0 deactivated)
07:28:16 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:16 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:17 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:17 INFO opendrift:2035: 2025-07-18 01:27:33.413417 - step 19 of 24 - 1000 active elements (0 deactivated)
07:28:18 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:18 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:19 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:19 INFO opendrift:2035: 2025-07-18 02:27:33.413417 - step 20 of 24 - 1000 active elements (0 deactivated)
07:28:20 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:20 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:21 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:21 INFO opendrift:2035: 2025-07-18 03:27:33.413417 - step 21 of 24 - 1000 active elements (0 deactivated)
07:28:22 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:22 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:23 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:23 INFO opendrift:2035: 2025-07-18 04:27:33.413417 - step 22 of 24 - 1000 active elements (0 deactivated)
07:28:25 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:25 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:25 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:25 INFO opendrift:2035: 2025-07-18 05:27:33.413417 - step 23 of 24 - 1000 active elements (0 deactivated)
07:28:27 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:27 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:28 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
07:28:28 INFO opendrift:2035: 2025-07-18 06:27:33.413417 - step 24 of 24 - 1000 active elements (0 deactivated)
07:28:29 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:29 WARNING opendrift.readers.interpolation.structured:63: Ensemble data currently not extrapolated towards seafloor
07:28:30 INFO opendrift.models.shipdrift:311: Using Stokes drift direction as wave direction
Print and plot results
print(o)
o.plot(linecolor='orientation')
#o.animation(color='orientation', markersize=20, filename="orientation.gif", legend=['left','right'],colorbar=False,cmap='bwr')
o.animation(color='orientation', legend=['left','right'], markersize=20, colorbar=False, cmap='bwr')
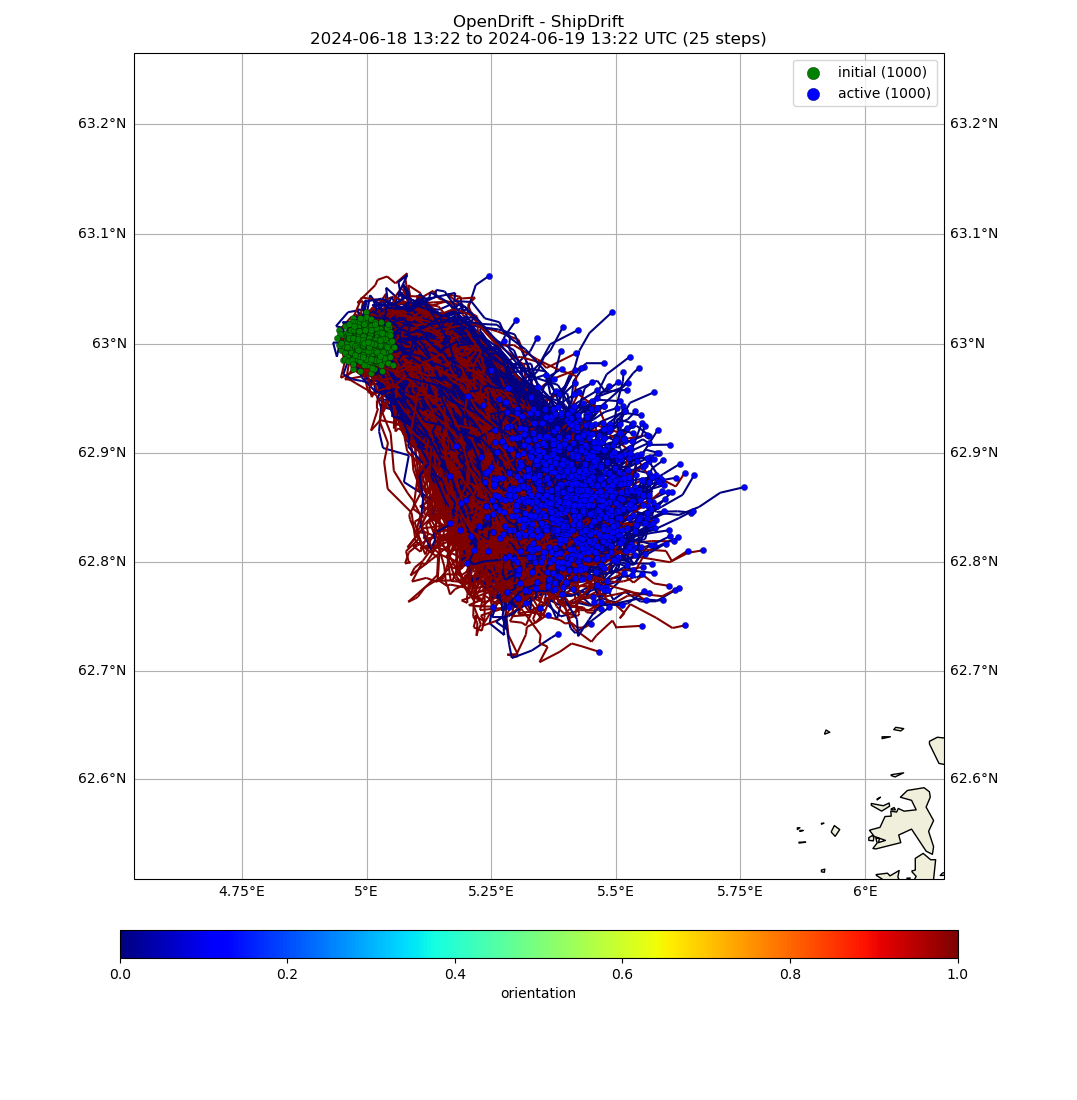
===========================
--------------------
Reader performance:
--------------------
global_landmask
0:00:00.0 total
0:00:00.0 preparing
0:00:00.0 reading
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml
0:00:15.8 total
0:00:00.0 preparing
0:00:15.7 reading
0:00:00.0 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc
0:00:14.4 total
0:00:00.0 preparing
0:00:14.3 reading
0:00:00.3 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
0:00:16.4 total
0:00:00.0 preparing
0:00:16.3 reading
0:00:00.0 interpolation
0:00:00.0 interpolation_time
0:00:00.0 rotating vectors
0:00:00.0 masking
--------------------
Performance:
57.0 total time
4.1 configuration
0.0 preparing main loop
0.0 moving elements to ocean
52.7 main loop
0.5 updating elements
0.0 cleaning up
--------------------
===========================
Model: ShipDrift (OpenDrift version 1.14.2)
1000 active ShipObject particles (0 deactivated, 0 scheduled)
-------------------
Environment variables:
-----
land_binary_mask
1) global_landmask
-----
x_sea_water_velocity
y_sea_water_velocity
1) https://thredds.met.no/thredds/dodsC/cmems/topaz6/dataset-topaz6-arc-15min-3km-be.ncml
2) https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
-----
x_wind
y_wind
1) https://thredds.met.no/thredds/dodsC/mepslatest/meps_lagged_6_h_latest_2_5km_latest.nc
-----
sea_surface_wave_mean_period_from_variance_spectral_density_second_frequency_moment
sea_surface_wave_significant_height
sea_surface_wave_stokes_drift_x_velocity
sea_surface_wave_stokes_drift_y_velocity
1) https://thredds.met.no/thredds/dodsC/cmems/mywavewam3km/dataset-wam-arctic-1hr3km-be.ncml
Discarded readers:
Time:
Start: 2025-07-17 07:27:33.413417 UTC
Present: 2025-07-18 07:27:33.413417 UTC
Calculation steps: 24 * 1:00:00 - total time: 1 day, 0:00:00
Output steps: 25 * 1:00:00
===========================
07:28:46 INFO opendrift:4553: Saving animation to /root/project/docs/source/gallery/animations/example_shipdrift_0.gif...
07:29:02 INFO opendrift:2994: Time to make animation: 0:00:16.826914
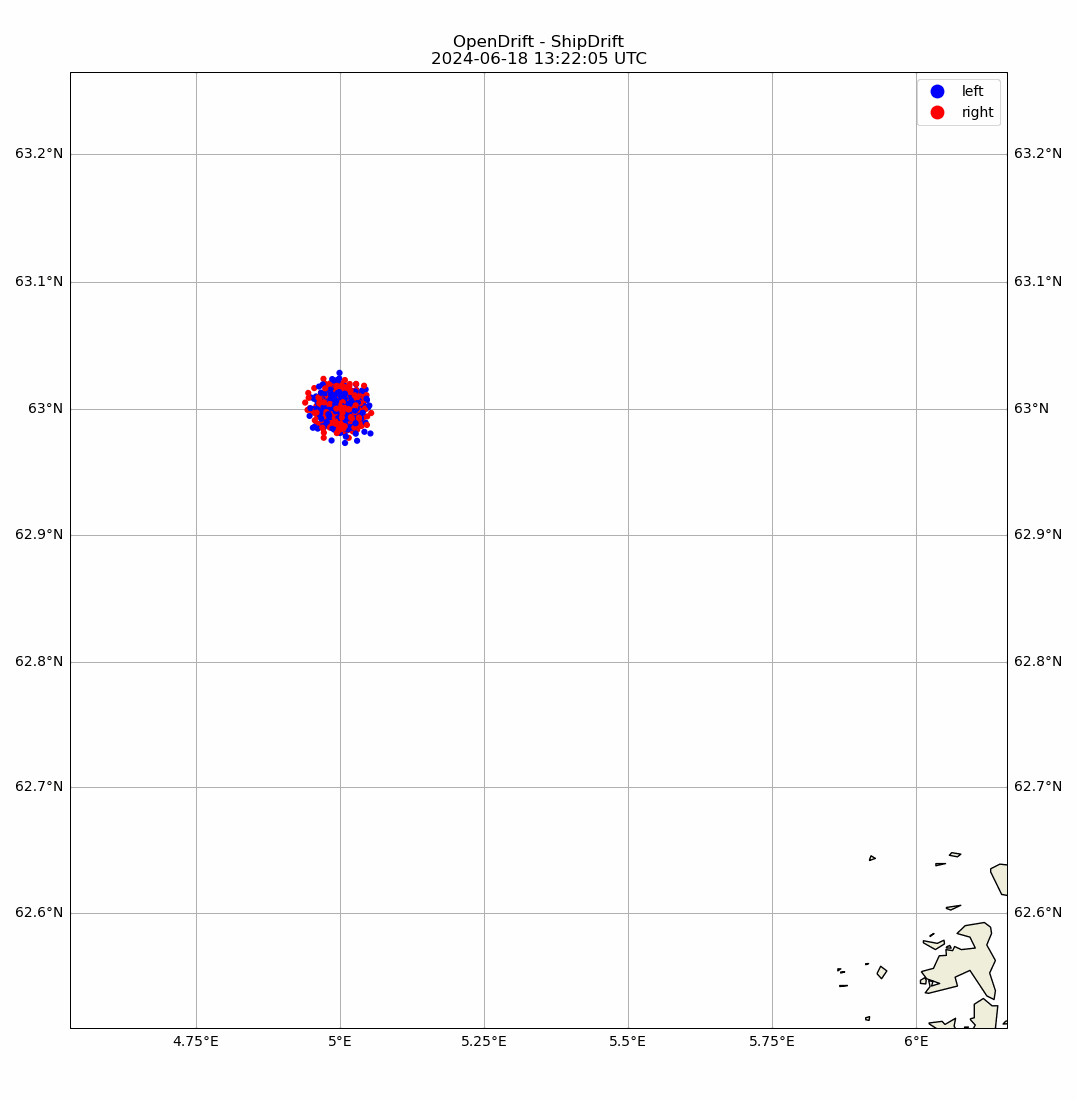
Total running time of the script: (1 minutes 36.102 seconds)