Note
Go to the end to download the full example code.
ChemicalDrift - Transport and fate of organic compounds
from opendrift.readers import reader_netCDF_CF_generic
from opendrift.models.chemicaldrift import ChemicalDrift
from opendrift.readers.reader_constant import Reader as ConstantReader
from datetime import timedelta, datetime
import matplotlib.pyplot as plt
import numpy as np
o = ChemicalDrift(loglevel=0, seed=0)
# Norkyst
reader_norkyst = reader_netCDF_CF_generic.Reader('https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be')
mixed_layer = ConstantReader({'ocean_mixed_layer_thickness': 40})
o.add_reader([reader_norkyst,mixed_layer])
o.set_config('drift:vertical_mixing', True)
o.set_config('vertical_mixing:diffusivitymodel', 'windspeed_Large1994')
o.set_config('chemical:particle_diameter',30.e-6) # m
o.set_config('chemical:particle_diameter_uncertainty',5.e-6) # m
o.set_config('chemical:sediment:resuspension_critvel',0.15) # m/s
o.set_config('chemical:transformations:volatilization', True)
o.set_config('chemical:transformations:degradation', True)
o.set_config('chemical:transformations:degradation_mode', 'OverallRateConstants')
o.set_config('seed:LMM_fraction',.9)
o.set_config('seed:particle_fraction',.1)
o.set_config('general:coastline_action', 'previous')
o.init_chemical_compound("Phenanthrene")
# Modify half-life times with unrealistic values for this demo
o.set_config('chemical:transformations:t12_W_tot', 6.) # hours
o.set_config('chemical:transformations:t12_S_tot', 12.) # hours
o.list_configspec()
14:17:55 DEBUG opendrift.config:161: Adding 16 config items from __init__
14:17:55 DEBUG opendrift.config:171: Overwriting config item readers:max_number_of_fails
14:17:55 DEBUG opendrift.config:161: Adding 13 config items from __init__
14:17:55 INFO opendrift.models.basemodel:533: OpenDriftSimulation initialised (version 1.11.2 / v1.11.2-43-gce9f6bb)
14:17:55 DEBUG opendrift.config:161: Adding 15 config items from oceandrift
14:17:55 DEBUG opendrift.config:171: Overwriting config item seed:z
14:17:55 DEBUG opendrift.config:161: Adding 71 config items from chemicaldrift
14:17:55 INFO opendrift.readers.reader_netCDF_CF_generic:102: Opening dataset: https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:17:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:119: Finding coordinate variables.
14:17:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:134: Parsing CF grid mapping dictionary: {'grid_mapping_name': 'polar_stereographic', 'straight_vertical_longitude_from_pole': 70.0, 'latitude_of_projection_origin': 90.0, 'standard_parallel': 60.0, 'false_easting': 3192800.0, 'false_northing': 1784000.0, 'semi_major_axis': 6378137.0, 'semi_minor_axis': 6356752.3142, 'proj4': '+proj=stere +lat_0=90 +lat_ts=60 +lon_0=70 +x_0=3192800 +y_0=1784000 +a=6378137 +b=6356752.3142 +units=m +no_defs +type=crs'}
14:17:58 INFO opendrift.readers.reader_netCDF_CF_generic:314: Detected dimensions: {'x': 'X', 'y': 'Y', 'z': 'depth', 'time': 'time'}
14:17:58 DEBUG opendrift.readers.reader_netCDF_CF_generic:340: Skipped variables without standard_name: ['angle', 'tke', 'ubar', 'vbar']
14:17:58 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 25 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 5 m/s and time span of 1:00:00
14:17:58 DEBUG opendrift.readers.basereader:176: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
14:17:58 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_wind', 'y_wind'] -> wind_speed
14:17:58 DEBUG opendrift.readers.basereader.variables:563: Adding variable mapping: ['x_sea_water_velocity', 'y_sea_water_velocity'] -> sea_water_speed
14:17:58 DEBUG opendrift.readers.basereader:176: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
14:17:58 DEBUG opendrift.models.basemodel.environment:328: Added reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:17:58 DEBUG opendrift.models.basemodel.environment:328: Added reader constant_reader
environment:constant:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:x_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:fallback:y_sea_water_velocity [None] float min: -15, max: 15 [m/s] Component of ocean c...
environment:constant:sea_surface_height [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_surface_height [0] float min: None, max: None [None] Fallback value for s...
environment:constant:x_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:x_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:y_wind [None] float min: -50, max: 50 [m/s] Component of wind al...
environment:fallback:y_wind [0] float min: -50, max: 50 [m/s] Component of wind al...
environment:constant:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:fallback:land_binary_mask [None] float min: 0, max: 1 [None] 1 is land, 0 is sea...
environment:constant:sea_floor_depth_below_sea_level [None] float min: -20, max: 12000 [None] Depth of seafloor...
environment:fallback:sea_floor_depth_below_sea_level [10000] float min: -20, max: 12000 [None] Depth of seafloor...
environment:constant:ocean_vertical_diffusivity [None] float min: 0, max: 1 [None] Use constant value f...
environment:fallback:ocean_vertical_diffusivity [0.0001] float min: 0, max: 1 [None] Fallback value for o...
environment:constant:sea_water_temperature [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_temperature [10] float min: None, max: None [None] Fallback value for s...
environment:constant:sea_water_salinity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_salinity [34] float min: None, max: None [None] Fallback value for s...
environment:constant:upward_sea_water_velocity [None] float min: None, max: None [None] Use constant value f...
environment:fallback:upward_sea_water_velocity [0] float min: None, max: None [None] Fallback value for u...
environment:constant:spm [None] float min: None, max: None [None] Use constant value f...
environment:fallback:spm [1] float min: None, max: None [None] Fallback value for s...
environment:constant:ocean_mixed_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:ocean_mixed_layer_thickness [50] float min: None, max: None [None] Fallback value for o...
environment:constant:active_sediment_layer_thickness [None] float min: None, max: None [None] Use constant value f...
environment:fallback:active_sediment_layer_thickness [0.03] float min: None, max: None [None] Fallback value for a...
environment:constant:doc [None] float min: None, max: None [None] Use constant value f...
environment:fallback:doc [0.0] float min: None, max: None [None] Fallback value for d...
environment:constant:sea_water_ph_reported_on_total_scale [None] float min: None, max: None [None] Use constant value f...
environment:fallback:sea_water_ph_reported_on_total_scale [8.1] float min: None, max: None [None] Fallback value for s...
environment:constant:pH_sediment [None] float min: None, max: None [None] Use constant value f...
environment:fallback:pH_sediment [6.9] float min: None, max: None [None] Fallback value for p...
general:use_auto_landmask [True] bool A built-in GSHHG glo...
drift:current_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:current_uncertainty_uniform [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:max_speed [2.0] float min: 0, max: inf [seconds] Typical maximum spee...
readers:max_number_of_fails [1] int min: 0, max: 1000000.0 [number] Readers are discarde...
general:coastline_action [previous] enum ['none', 'stranding', 'previous'] None means that obje...
general:time_step_minutes [60] float min: 0.01, max: 1440 [minutes] Calculation time ste...
general:time_step_output_minutes [None] float min: 1, max: 1440 [minutes] Output time step, i....
seed:ocean_only [True] bool If True, elements se...
seed:number [1] int min: 1, max: 100000000 [1] The number of elemen...
drift:max_age_seconds [None] float min: 0, max: inf [seconds] Elements will be dea...
drift:advection_scheme [euler] enum ['euler', 'runge-kutta', 'runge-kutta4'] Numerical advection ...
drift:horizontal_diffusivity [0] float min: 0, max: 100000 [m2/s] Add horizontal diffu...
drift:profiles_depth [50] float min: 0, max: None [meters] Environment profiles...
drift:wind_uncertainty [0] float min: 0, max: 5 [m/s] Add gaussian perturb...
drift:relative_wind [False] bool If True, wind drift ...
drift:deactivate_north_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_south_of [None] float min: -90, max: 90 [degrees] Elements are deactiv...
drift:deactivate_east_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
drift:deactivate_west_of [None] float min: -360, max: 360 [degrees] Elements are deactiv...
seed:origin_marker [0] float min: None, max: None [None] An integer kept cons...
seed:z [0] float min: -10000, max: 0 [m] Depth below sea leve...
seed:wind_drift_factor [0.02] float min: None, max: None [1] Elements at surface ...
seed:current_drift_factor [1] float min: None, max: None [1] Elements are moved w...
seed:terminal_velocity [0.0] float min: None, max: None [m/s] Terminal rise/sinkin...
seed:diameter [0.0] float min: None, max: None [m] Seeding value of dia...
seed:density [2650.0] float min: None, max: None [kg/m^3] Seeding value of den...
seed:specie [0] float min: None, max: None [] Seeding value of spe...
seed:mass [1000.0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_water [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_degraded_sediment [0] float min: None, max: None [ug] Seeding value of mas...
seed:mass_volatilized [0] float min: None, max: None [ug] Seeding value of mas...
drift:vertical_advection [True] bool Advect elements with...
drift:vertical_mixing [True] bool Activate vertical mi...
vertical_mixing:timestep [60] float min: 0.1, max: 3600 [seconds] Time step used for i...
vertical_mixing:diffusivitymodel [windspeed_Large1994] enum ['environment', 'stepfunction', 'windspeed_Sundby1983', 'windspeed_Large1994', 'gls_tke', 'constant'] Algorithm/source use...
vertical_mixing:background_diffusivity [1.2e-05] float min: 0, max: 1 [m2s-1] Background diffusivi...
vertical_mixing:TSprofiles [False] bool Update T and S profi...
drift:wind_drift_depth [0.1] float min: 0, max: 10 [meters] The direct wind drif...
drift:stokes_drift [True] bool Advection elements w...
drift:stokes_drift_profile [Phillips] enum ['monochromatic', 'exponential', 'Phillips', 'windsea_swell'] Algorithm to calcula...
drift:use_tabularised_stokes_drift [False] bool If True, Stokes drif...
drift:tabularised_stokes_drift_fetch [25000] enum ['5000', '25000', '50000'] The fetch length whe...
general:seafloor_action [lift_to_seafloor] enum ['none', 'lift_to_seafloor', 'deactivate', 'previous'] "deactivate": elemen...
drift:truncate_ocean_model_below_m [None] float min: 0, max: 10000 [m] Ocean model data are...
seed:seafloor [False] bool Elements are seeded ...
chemical:transfer_setup [organics] enum ['Sandnesfj_Al', 'metals', '137Cs_rev', 'custom', 'organics'] ...
chemical:dynamic_partitioning [True] bool Toggle dynamic parti...
chemical:slowly_fraction [False] bool ...
chemical:irreversible_fraction [False] bool ...
chemical:dissolved_diameter [0] float min: 0, max: 0.0001 [m] ...
chemical:particle_diameter [3e-05] float min: 0, max: 0.0001 [m] ...
chemical:particle_concentration_half_depth [20] float min: 0, max: 100 [m] ...
chemical:doc_concentration_half_depth [1000] float min: 0, max: 1000 [m] ...
chemical:particle_diameter_uncertainty [5e-06] float min: 0, max: 0.0001 [m] ...
seed:LMM_fraction [0.9] float min: 0, max: 1 [] ...
seed:particle_fraction [0.1] float min: 0, max: 1 [] ...
chemical:species:LMM [True] bool Toggle LMM species...
chemical:species:LMMcation [False] bool ...
chemical:species:LMManion [False] bool ...
chemical:species:Colloid [False] bool ...
chemical:species:Humic_colloid [False] bool ...
chemical:species:Polymer [False] bool ...
chemical:species:Particle_reversible [True] bool ...
chemical:species:Particle_slowly_reversible [False] bool ...
chemical:species:Particle_irreversible [False] bool ...
chemical:species:Sediment_reversible [True] bool ...
chemical:species:Sediment_slowly_reversible [False] bool ...
chemical:species:Sediment_irreversible [False] bool ...
chemical:transformations:Kd [2.0] float min: 0, max: 1000000000.0 [m3/kg] ...
chemical:transformations:S0 [0.0] float min: 0, max: 100 [PSU] parameter controllin...
chemical:transformations:Dc [1.16e-05] float min: 0, max: 1000000.0 [] ...
chemical:transformations:slow_coeff [0] float min: 0, max: 1000000.0 [] ...
chemical:transformations:volatilization [True] bool Chemical is evaporat...
chemical:transformations:degradation [True] bool Chemical mass is deg...
chemical:transformations:degradation_mode [OverallRateConstants] enum ['OverallRateConstants'] ...
chemical:transformations:dissociation [nondiss] enum ['nondiss', 'acid', 'base', 'amphoter'] ...
chemical:transformations:LogKOW [4.505] float min: -3, max: 10 [Log L/Kg] ...
chemical:transformations:TrefKOW [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_KOC_Sed [-24900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:DeltaH_KOC_DOM [-25900.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:Setchenow [0.3026] float min: 0, max: 1 [L/mol] ...
chemical:transformations:pKa_acid [-1] float min: -1, max: 14 [] ...
chemical:transformations:pKa_base [-1] float min: -1, max: 14 [] ...
chemical:transformations:KOC_DOM [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:KOC_sed [-1] float min: -1, max: 10000000000 [L/KgOC] ...
chemical:transformations:fOC_SPM [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:fOC_sed [0.05] float min: 0.01, max: 0.1 [gOC/g] ...
chemical:transformations:aggregation_rate [0] float min: 0, max: 1 [s-1] ...
chemical:transformations:t12_W_tot [6.0] float min: 1, max: None [hours] half life in water, ...
chemical:transformations:Tref_kWt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kWt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:t12_S_tot [12.0] float min: 1, max: None [hours] half life in sedimen...
chemical:transformations:Tref_kSt [25.0] float min: -3, max: 30 [C] ...
chemical:transformations:DeltaH_kSt [50000.0] float min: -100000.0, max: 100000.0 [J/mol] ...
chemical:transformations:MolWt [178.226] float min: 50, max: 1000 [amu] molecular weight...
chemical:transformations:Henry [4.294e-05] float min: None, max: None [atm m3 mol-1] Henry constant...
chemical:transformations:Vpress [0.0222] float min: None, max: None [Pa] Vapour pressure...
chemical:transformations:Tref_Vpress [25.0] float min: None, max: None [C] Vapour pressure ref ...
chemical:transformations:DeltaH_Vpress [71733.0] float min: -100000.0, max: 115000.0 [J/mol] Enthalpy of volatili...
chemical:transformations:Solub [1.09] float min: None, max: None [g/m3] Solubility...
chemical:transformations:Tref_Solub [25.0] float min: None, max: None [C] Solubility ref temp...
chemical:transformations:DeltaH_Solub [34800.0] float min: -100000.0, max: 100000.0 [J/mol] Enthalpy of solubili...
chemical:sediment:mixing_depth [0.03] float min: 0, max: 100 [m] ...
chemical:sediment:density [2600] float min: 0, max: 10000 [kg/m3] ...
chemical:sediment:effective_fraction [0.9] float min: 0, max: 1 [] ...
chemical:sediment:corr_factor [0.1] float min: 0, max: 10 [] ...
chemical:sediment:porosity [0.6] float min: 0, max: 1 [] ...
chemical:sediment:layer_thickness [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:desorption_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth [1] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_depth_uncert [0.5] float min: 0, max: 100 [m] ...
chemical:sediment:resuspension_critvel [0.15] float min: 0, max: 1 [m/s] ...
chemical:sediment:burial_rate [3e-05] float min: 0, max: 10 [m/year] ...
chemical:sediment:buried_leaking_rate [0] float min: 0, max: 10 [s-1] ...
chemical:compound [Phenanthrene] enum ['Naphthalene', 'Phenanthrene', 'Fluoranthene', 'Benzo-a-anthracene', 'Benzo-a-pyrene', 'Dibenzo-ah-anthracene', 'C1-Naphthalene', 'Acenaphthene', 'Acenaphthylene', 'Fluorene', 'Dibenzothiophene', 'C2-Naphthalene', 'Anthracene', 'C3-Naphthalene', 'C1-Dibenzothiophene', 'Pyrene', 'C1-Phenanthrene', 'C2-Dibenzothiophene', 'C2-Phenanthrene', 'Benzo-b-fluoranthene', 'Chrysene', 'C3-Dibenzothiophene', 'C3-Phenanthrene', 'Benzo-k-fluoranthene', 'Benzo-ghi-perylene', 'Indeno-123cd-pyrene', 'Copper', 'Cadmium', 'Chromium', 'Lead', 'Vanadium', 'Zinc', 'Nickel', None] ...
Seeding 500 lagrangian elements each representign 2mg og target chemical
td=datetime.today()
time = td - timedelta(days=10)
latseed= 57.6; lonseed= 10.6 # Skagen
ntraj=500
iniz=np.random.rand(ntraj) * -10. # seeding the chemicals in the upper 10m
o.seed_elements(lonseed, latseed, z=iniz, radius=2000,number=ntraj,time=time, mass=2e3)
14:17:58 DEBUG opendrift.models.chemicaldrift:870: Partitioning coefficients (Tref,freshwater)
14:17:58 DEBUG opendrift.models.chemicaldrift:871: KOC_sed: 12953.922406542462 L/KgOC
14:17:58 DEBUG opendrift.models.chemicaldrift:872: KOC_SPM: 12953.922406542462 L/KgOC
14:17:58 DEBUG opendrift.models.chemicaldrift:873: KOC_DOM: 3004.29439651874 L/KgOC
14:17:58 DEBUG opendrift.models.chemicaldrift:885: Kd_sed: 647.6961203271231 L/Kg
14:17:58 DEBUG opendrift.models.chemicaldrift:886: Kd_SPM: 647.6961203271231 L/Kg
14:17:58 DEBUG opendrift.models.chemicaldrift:887: Kd_DOM: 1580.2588525688573 L/Kg
14:17:58 DEBUG opendrift.models.chemicaldrift:1104: nspecies: 5
14:17:58 DEBUG opendrift.models.chemicaldrift:1105: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
14:17:58 DEBUG opendrift.models.chemicaldrift:509: Initial partitioning:
14:17:58 DEBUG opendrift.models.chemicaldrift:511: 449 0 LMM
14:17:58 DEBUG opendrift.models.chemicaldrift:511: 0 1 Humic colloid
14:17:58 DEBUG opendrift.models.chemicaldrift:511: 51 2 Particle reversible
14:17:58 DEBUG opendrift.models.chemicaldrift:511: 0 3 Sediment reversible
14:17:58 DEBUG opendrift.models.chemicaldrift:511: 0 4 Sediment slowly reversible
14:17:58 INFO opendrift.models.basemodel.environment:218: Adding a dynamical landmask with max. priority based on assumed maximum speed of 2.0 m/s. Adding a customised landmask may be faster...
14:17:58 DEBUG opendrift.readers.basereader:176: Variable mapping: ['sea_floor_depth_below_sea_level'] -> ['land_binary_mask'] is not activated
14:18:04 DEBUG opendrift.models.basemodel.environment:328: Added reader global_landmask
14:18:04 INFO opendrift.models.basemodel.environment:245: Fallback values will be used for the following variables which have no readers:
14:18:04 INFO opendrift.models.basemodel.environment:248: sea_surface_height: 0.000000
14:18:04 INFO opendrift.models.basemodel.environment:248: ocean_vertical_diffusivity: 0.000100
14:18:04 INFO opendrift.models.basemodel.environment:248: spm: 1.000000
14:18:04 INFO opendrift.models.basemodel.environment:248: active_sediment_layer_thickness: 0.030000
14:18:04 INFO opendrift.models.basemodel.environment:248: doc: 0.000000
14:18:04 INFO opendrift.models.basemodel.environment:248: sea_water_ph_reported_on_total_scale: 8.100000
14:18:04 INFO opendrift.models.basemodel.environment:248: pH_sediment: 6.900000
14:18:04 DEBUG opendrift.models.basemodel:92: Changed mode from Mode.Config to Mode.Ready
Running model
o.run(steps=48*2, time_step=1800, time_step_output=1800)
14:18:04 DEBUG opendrift.models.basemodel:92: Changed mode from Mode.Ready to Mode.Run
14:18:04 DEBUG opendrift.models.basemodel:1751:
------------------------------------------------------
Software and hardware:
OpenDrift version 1.11.2
Platform: Linux, 5.15.0-1056-aws
68.56775665283203 GB memory
36 processors (x86_64)
NumPy version 1.26.4
SciPy version 1.13.0
Matplotlib version 3.8.4
NetCDF4 version 1.6.1
Xarray version 2024.5.0
Python version 3.11.6 | packaged by conda-forge | (main, Oct 3 2023, 10:40:35) [GCC 12.3.0]
------------------------------------------------------
14:18:04 DEBUG opendrift.models.basemodel:1765: No output file is specified, neglecting export_buffer_length
14:18:04 DEBUG opendrift.models.basemodel:1883: Finalizing environment and preparing readers for simulation coverage ([4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]) and time (2024-05-04 14:17:58.364115 to 2024-05-06 14:17:58.364115)
14:18:04 DEBUG opendrift.models.basemodel.environment:180: Preparing https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
14:18:04 DEBUG opendrift.readers.basereader.structured:153: Clearing cache for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be before starting new simulation
14:18:04 DEBUG opendrift.readers.basereader.variables:608: Setting buffer size 11 for reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be, assuming a maximum average speed of 2 m/s and time span of 1:00:00
14:18:04 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:04 DEBUG opendrift.models.basemodel.environment:180: Preparing constant_reader for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
14:18:04 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for constant_reader
14:18:04 DEBUG opendrift.models.basemodel.environment:180: Preparing global_landmask for extent [4.69639997080836, 54.43270917325407, 16.516793922624256, 60.761642023034995]
14:18:04 DEBUG opendrift.readers.basereader.variables:549: Nothing more to prepare for global_landmask
14:18:04 INFO opendrift.models.basemodel:911: Using existing reader for land_binary_mask
14:18:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:04 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:04 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:04 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:04 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:04 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:04 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:04 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:04 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:04 INFO opendrift.models.basemodel:922: All points are in ocean
14:18:04 INFO opendrift.models.chemicaldrift:352: Number of species: 5
14:18:04 INFO opendrift.models.chemicaldrift:354: 0 LMM
14:18:04 INFO opendrift.models.chemicaldrift:354: 1 Humic colloid
14:18:04 INFO opendrift.models.chemicaldrift:354: 2 Particle reversible
14:18:04 INFO opendrift.models.chemicaldrift:354: 3 Sediment reversible
14:18:04 INFO opendrift.models.chemicaldrift:354: 4 Sediment slowly reversible
14:18:04 INFO opendrift.models.chemicaldrift:357: transfer setup: organics
14:18:04 INFO opendrift.models.chemicaldrift:359: nspecies: 5
14:18:04 INFO opendrift.models.chemicaldrift:360: Transfer rates:
[[0.00000000e+00 1.75855513e-08 4.62500000e-07 2.88600000e-02
0.00000000e+00]
[4.06297347e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-06 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]
[9.91290450e-07 0.00000000e+00 0.00000000e+00 0.00000000e+00
3.16887646e-11]
[0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00]]
14:18:04 DEBUG opendrift.models.basemodel:866: to be seeded: 500, already seeded 0
14:18:04 DEBUG opendrift.models.basemodel:884: Released 500 new elements.
14:18:04 WARNING opendrift.models.basemodel:705: Seafloor check not being run because environment is missing. This will happen the first time the function is run but if it happens subsequently there is probably a problem.
14:18:04 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:04 INFO opendrift.models.basemodel:2011: 2024-05-04 14:17:58.364115 - step 1 of 96 - 500 active elements (0 deactivated)
14:18:04 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:04 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:04 DEBUG opendrift.models.basemodel:2030: 57.546223 <- latitude -> 57.64813
14:18:04 DEBUG opendrift.models.basemodel:2035: 10.507113 <- longitude -> 10.70608
14:18:04 DEBUG opendrift.models.basemodel:2040: -9.98847 <- z -> -0.046954762
14:18:04 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:04 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:04 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:04 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:04 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:04 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 14:00:00 (before)
2024-05-04 15:00:00 (after)
14:18:06 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:06 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:06 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:06 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:06 DEBUG opendrift.readers.basereader.structured:291: Fetched env-block (size 36x35x7) for time before (2024-05-04 14:00:00)
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:08 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:08 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 36x35x7) for time after (2024-05-04 15:00:00)
14:18:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 14:00:00) in space (linearNDFast)
14:18:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:08 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 15:00:00) in space (linearNDFast)
14:18:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:08 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 14:00:00, weight 0.70) and
after (2024-05-04 15:00:00, weight 0.30) in time
14:18:08 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4929004930107 and -59.29392585710053 degrees.
14:18:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4929004930107 and -59.29392585710053 degrees.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.106258 (min) 0.0745877 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0862523 (min) 0.111017 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.263766 (min) 1.57666 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.22481 (min) 2.29363 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.53077 (min) 23.882 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 7.95226 (min) 10.2842 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.1558 (min) 27.5649 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000105291 (min) 7.25479e-05 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:08 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:08 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:08 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:08 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:08 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/core/fromnumeric.py:3504: RuntimeWarning: Mean of empty slice.
return _methods._mean(a, axis=axis, dtype=dtype,
/opt/conda/envs/opendrift/lib/python3.11/site-packages/numpy/core/_methods.py:129: RuntimeWarning: invalid value encountered in divide
ret = ret.dtype.type(ret / rcount)
14:18:08 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:08 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
14:18:08 DEBUG opendrift.models.chemicaldrift:1452: old species: [2]
14:18:08 DEBUG opendrift.models.chemicaldrift:1453: new species: [0]
14:18:08 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]]
14:18:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:08 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:08 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:08 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00399599104573676
14:18:08 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:08 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:08 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:08 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:08 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:08 INFO opendrift.models.chemicaldrift:1861: partitioning: [450, 0, 50, 0, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:08 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:08 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:08 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:08 INFO opendrift.models.basemodel:2011: 2024-05-04 14:47:58.364115 - step 2 of 96 - 500 active elements (0 deactivated)
14:18:08 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:08 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:08 DEBUG opendrift.models.basemodel:2030: 57.54522135003065 <- latitude -> 57.6499227832445
14:18:08 DEBUG opendrift.models.basemodel:2035: 10.506432476139953 <- longitude -> 10.702954135800061
14:18:08 DEBUG opendrift.models.basemodel:2040: -19.96475096296818 <- z -> -0.31097333523489984
14:18:08 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 14:00:00 (before)
2024-05-04 15:00:00 (after)
14:18:08 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 14:00:00) in space (linearNDFast)
14:18:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:08 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 15:00:00) in space (linearNDFast)
14:18:08 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:08 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 14:00:00, weight 0.20) and
after (2024-05-04 15:00:00, weight 0.80) in time
14:18:08 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.493578623005995 and -59.29705692022877 degrees.
14:18:08 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.493578623005995 and -59.29705692022877 degrees.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:08 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:08 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:08 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:08 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:08 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:08 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0886228 (min) 0.0751776 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0658389 (min) 0.140209 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.12237 (min) 4.52495 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.58837 (min) 0.974488 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.50993 (min) 23.7386 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.088 (min) 10.3186 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.1672 (min) 32.5458 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000104102 (min) 6.1065e-05 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:08 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:08 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:08 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:08 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:08 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:08 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:08 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:08 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:08 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:18:08 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:18:08 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[0. 0. 0. 3. 0.]
[0. 0. 0. 0. 0.]
[1. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]
[0. 0. 0. 0. 0.]]
14:18:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:08 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:08 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:08 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.014992551588576307
14:18:08 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:08 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:08 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:08 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:08 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:08 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:08 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:08 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:08 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:08 INFO opendrift.models.chemicaldrift:1861: partitioning: [447, 0, 46, 7, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:08 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:08 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:08 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:08 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:08 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:08 INFO opendrift.models.basemodel:2011: 2024-05-04 15:17:58.364115 - step 3 of 96 - 500 active elements (0 deactivated)
14:18:08 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:08 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:08 DEBUG opendrift.models.basemodel:2030: 57.54493114246923 <- latitude -> 57.65218886027178
14:18:08 DEBUG opendrift.models.basemodel:2035: 10.50586390764039 <- longitude -> 10.700430821815422
14:18:08 DEBUG opendrift.models.basemodel:2040: -20.970450341749657 <- z -> -0.08732309403563854
14:18:08 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:08 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:08 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:08 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:08 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:08 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 15:00:00 (before)
2024-05-04 16:00:00 (after)
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:11 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 37x35x7) for time after (2024-05-04 16:00:00)
14:18:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 15:00:00) in space (linearNDFast)
14:18:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 16:00:00) in space (linearNDFast)
14:18:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 15:00:00, weight 0.70) and
after (2024-05-04 16:00:00, weight 0.30) in time
14:18:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494147191910265 and -59.299580239796555 degrees.
14:18:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494147191910265 and -59.299580239796555 degrees.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0743253 (min) 0.0818113 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0398264 (min) 0.157011 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.8477 (min) 6.04821 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.26625 (min) -0.231175 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48294 (min) 23.6199 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.06684 (min) 10.3968 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.1807 (min) 32.6862 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000101833 (min) 6.38073e-05 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:11 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:11 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:11 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 22
14:18:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0]
14:18:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 0 3 3 3 3 3 3]
14:18:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 24. 0.]
[ 0. 0. 0. 0. 0.]
[ 2. 0. 0. 4. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.027238235274388418
14:18:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:11 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [427, 0, 45, 28, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:11 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:11 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:11 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:11 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:11 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:11 INFO opendrift.models.basemodel:2011: 2024-05-04 15:47:58.364115 - step 4 of 96 - 500 active elements (0 deactivated)
14:18:11 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:11 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:11 DEBUG opendrift.models.basemodel:2030: 57.54504030756289 <- latitude -> 57.65466669653229
14:18:11 DEBUG opendrift.models.basemodel:2035: 10.505926817056114 <- longitude -> 10.699057678902262
14:18:11 DEBUG opendrift.models.basemodel:2040: -21.51475715637207 <- z -> -0.012619249374106856
14:18:11 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 15:00:00 (before)
2024-05-04 16:00:00 (after)
14:18:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 15:00:00) in space (linearNDFast)
14:18:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 16:00:00) in space (linearNDFast)
14:18:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 15:00:00, weight 0.20) and
after (2024-05-04 16:00:00, weight 0.80) in time
14:18:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.30095338946268 degrees.
14:18:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.30095338946268 degrees.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.05611 (min) 0.107723 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0277491 (min) 0.160638 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.19479 (min) 6.79237 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.63835 (min) -0.564477 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.5586 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.07648 (min) 10.3994 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2168 (min) 32.7057 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.15261e-05 (min) 8.505e-05 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:11 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:11 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:11 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:11 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 17
14:18:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 41. 0.]
[ 0. 0. 0. 0. 0.]
[ 2. 0. 0. 4. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03384352033599049
14:18:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:11 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:11 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:11 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:18:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [410, 0, 44, 46, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:11 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:11 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:11 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:11 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:11 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:11 INFO opendrift.models.basemodel:2011: 2024-05-04 16:17:58.364115 - step 5 of 96 - 500 active elements (0 deactivated)
14:18:11 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:11 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:11 DEBUG opendrift.models.basemodel:2030: 57.54563737911413 <- latitude -> 57.65637716782075
14:18:11 DEBUG opendrift.models.basemodel:2035: 10.505926817056114 <- longitude -> 10.698455102346687
14:18:11 DEBUG opendrift.models.basemodel:2040: -21.579301354824434 <- z -> 0.0
14:18:11 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 16:00:00 (before)
2024-05-04 17:00:00 (after)
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:12 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:12 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 38x35x7) for time after (2024-05-04 17:00:00)
14:18:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 16:00:00) in space (linearNDFast)
14:18:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 17:00:00) in space (linearNDFast)
14:18:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 16:00:00, weight 0.70) and
after (2024-05-04 17:00:00, weight 0.30) in time
14:18:12 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.3015559536375 degrees.
14:18:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.3015559536375 degrees.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0527943 (min) 0.157273 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00344956 (min) 0.144667 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 5.37004 (min) 6.81326 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.82102 (min) -0.439659 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.5765 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.07755 (min) 10.2763 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3715 (min) 32.6672 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.98087e-05 (min) 0.000104935 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:12 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:12 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:12 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:12 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:12 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:12 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:12 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 27
14:18:12 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0]
14:18:12 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 0 3 3 3 3 3 3 3 3 3 0 3 3 3 3 3 3 3 3 3 3]
14:18:12 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 66. 0.]
[ 0. 0. 0. 0. 0.]
[ 4. 0. 0. 5. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:12 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:12 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03429072693931202
14:18:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:12 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:12 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:12 INFO opendrift.models.chemicaldrift:1861: partitioning: [387, 0, 36, 77, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:12 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:12 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:12 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:12 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:12 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:12 INFO opendrift.models.basemodel:2011: 2024-05-04 16:47:58.364115 - step 6 of 96 - 500 active elements (0 deactivated)
14:18:12 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:12 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:12 DEBUG opendrift.models.basemodel:2030: 57.54637665772239 <- latitude -> 57.65866931682622
14:18:12 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.697372271272375
14:18:12 DEBUG opendrift.models.basemodel:2040: -22.280534744262695 <- z -> -0.15440788508371506
14:18:12 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 16:00:00 (before)
2024-05-04 17:00:00 (after)
14:18:12 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 16:00:00) in space (linearNDFast)
14:18:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:12 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 17:00:00) in space (linearNDFast)
14:18:12 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:12 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 16:00:00, weight 0.20) and
after (2024-05-04 17:00:00, weight 0.80) in time
14:18:12 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.30263879309415 degrees.
14:18:12 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427814807 and -59.30263879309415 degrees.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:12 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:12 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:12 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:12 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:12 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:12 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0656669 (min) 0.195761 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0165692 (min) 0.143318 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: x_wind: 4.89081 (min) 6.33088 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.75111 (min) -0.381402 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.4961 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.076 (min) 10.3896 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2486 (min) 32.6701 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.92462e-05 (min) 0.000126841 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:12 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:12 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:18:12 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:12 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:12 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:12 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:12 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 20
14:18:12 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:12 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:12 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 85. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 11. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:12 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:12 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:12 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.029998954318309633
14:18:12 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:12 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:12 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:12 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:12 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:12 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:12 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:18:12 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:12 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:12 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:12 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:12 INFO opendrift.models.chemicaldrift:1861: partitioning: [369, 0, 31, 100, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:12 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:12 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:12 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:12 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:12 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:12 INFO opendrift.models.basemodel:2011: 2024-05-04 17:17:58.364115 - step 7 of 96 - 500 active elements (0 deactivated)
14:18:12 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:12 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:12 DEBUG opendrift.models.basemodel:2030: 57.54783088026619 <- latitude -> 57.660949155471954
14:18:12 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.698327795645982
14:18:12 DEBUG opendrift.models.basemodel:2040: -22.280532836914062 <- z -> -0.11872921924736135
14:18:12 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:12 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:12 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:12 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:12 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:12 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 17:00:00 (before)
2024-05-04 18:00:00 (after)
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:14 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:14 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 38x35x7) for time after (2024-05-04 18:00:00)
14:18:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 17:00:00) in space (linearNDFast)
14:18:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:14 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 18:00:00) in space (linearNDFast)
14:18:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:14 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 17:00:00, weight 0.70) and
after (2024-05-04 18:00:00, weight 0.30) in time
14:18:14 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30168326465731 degrees.
14:18:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30168326465731 degrees.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0664284 (min) 0.201526 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0361449 (min) 0.155008 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 3.92864 (min) 5.55897 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.80598 (min) -0.654395 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.5262 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.08012 (min) 10.3114 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2572 (min) 32.7056 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -7.00855e-05 (min) 0.000135909 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:14 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:14 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:14 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:14 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:14 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:14 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 23
14:18:14 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:14 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:14 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 108. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 15. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:14 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:14 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:14 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.023249109371785957
14:18:14 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:14 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:14 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:14 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:14 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:14 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:14 INFO opendrift.models.chemicaldrift:1861: partitioning: [346, 0, 27, 127, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:14 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:14 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:14 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:14 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:14 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:14 INFO opendrift.models.basemodel:2011: 2024-05-04 17:47:58.364115 - step 8 of 96 - 500 active elements (0 deactivated)
14:18:14 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:14 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:14 DEBUG opendrift.models.basemodel:2030: 57.54946497451983 <- latitude -> 57.66286597072693
14:18:14 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.701879036184081
14:18:14 DEBUG opendrift.models.basemodel:2040: -22.88792470562281 <- z -> -0.11162623798697568
14:18:14 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 17:00:00 (before)
2024-05-04 18:00:00 (after)
14:18:14 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 17:00:00) in space (linearNDFast)
14:18:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:14 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 18:00:00) in space (linearNDFast)
14:18:14 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:14 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 17:00:00, weight 0.20) and
after (2024-05-04 18:00:00, weight 0.80) in time
14:18:14 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29813202694508 degrees.
14:18:14 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29813202694508 degrees.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:14 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:14 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:14 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:14 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:14 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:14 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0759036 (min) 0.210186 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0519557 (min) 0.15707 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.5794 (min) 4.63224 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.91922 (min) -0.979121 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.5965 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.07597 (min) 10.3113 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3483 (min) 32.7096 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.74716e-05 (min) 0.000144048 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:14 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:14 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:14 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:14 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:14 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:14 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
14:18:14 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0]
14:18:14 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3]
14:18:14 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 119. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 19. 0.]
[ 0. 0. 0. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:14 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:14 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:14 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.016332328499637967
14:18:14 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:14 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:14 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:14 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:14 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:14 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:14 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:14 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
14:18:14 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:14 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
14:18:14 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:14 INFO opendrift.models.chemicaldrift:1861: partitioning: [335, 0, 27, 138, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:14 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:14 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:14 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:14 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:14 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:14 INFO opendrift.models.basemodel:2011: 2024-05-04 18:17:58.364115 - step 9 of 96 - 500 active elements (0 deactivated)
14:18:14 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:14 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:14 DEBUG opendrift.models.basemodel:2030: 57.551060840421464 <- latitude -> 57.66463566717018
14:18:14 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.703251720788597
14:18:14 DEBUG opendrift.models.basemodel:2040: -22.280532836914062 <- z -> -0.14591523285559566
14:18:14 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:14 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:14 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:14 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:14 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:14 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 18:00:00 (before)
2024-05-04 19:00:00 (after)
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:16 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:16 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x35x7) for time after (2024-05-04 19:00:00)
14:18:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 18:00:00) in space (linearNDFast)
14:18:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:16 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 19:00:00) in space (linearNDFast)
14:18:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:16 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 18:00:00, weight 0.70) and
after (2024-05-04 19:00:00, weight 0.30) in time
14:18:16 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.296759344132326 degrees.
14:18:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.296759344132326 degrees.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.091377 (min) 0.201184 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0224212 (min) 0.198702 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.99862 (min) 4.17692 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.56857 (min) -0.559954 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.7004 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.06859 (min) 10.2972 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2646 (min) 32.7134 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.67052e-05 (min) 0.000160484 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:16 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:16 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:16 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:16 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:16 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:16 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:16 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 16
14:18:16 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:16 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:16 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 135. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 20. 0.]
[ 0. 0. 1. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:16 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:16 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:16 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:16 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:16 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0129497466984644
14:18:16 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:16 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:16 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:16 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:16 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
14:18:16 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:16 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
14:18:16 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:16 INFO opendrift.models.chemicaldrift:1861: partitioning: [319, 0, 39, 142, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:16 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:16 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:16 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:16 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:16 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:16 INFO opendrift.models.basemodel:2011: 2024-05-04 18:47:58.364115 - step 10 of 96 - 500 active elements (0 deactivated)
14:18:16 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:16 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:16 DEBUG opendrift.models.basemodel:2030: 57.552560523147854 <- latitude -> 57.66508446249144
14:18:16 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.700933828597222
14:18:16 DEBUG opendrift.models.basemodel:2040: -22.69363142368084 <- z -> 0.0
14:18:16 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 18:00:00 (before)
2024-05-04 19:00:00 (after)
14:18:16 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 18:00:00) in space (linearNDFast)
14:18:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:16 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 19:00:00) in space (linearNDFast)
14:18:16 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:16 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 18:00:00, weight 0.20) and
after (2024-05-04 19:00:00, weight 0.80) in time
14:18:16 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299077234616746 degrees.
14:18:16 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299077234616746 degrees.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:16 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:16 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:16 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:16 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:16 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:16 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.100162 (min) 0.178882 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00977988 (min) 0.181566 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: x_wind: 2.0283 (min) 4.05629 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.911798 (min) 0.0600802 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.8257 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.06027 (min) 10.2529 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2666 (min) 32.7473 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.02251e-05 (min) 0.000166763 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:16 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:16 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:16 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:18:16 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:16 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:16 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:16 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:16 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 22
14:18:16 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:16 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:16 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 157. 0.]
[ 0. 0. 0. 0. 0.]
[ 5. 0. 0. 21. 0.]
[ 0. 0. 14. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:16 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:16 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:16 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:16 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:16 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.011903528225149632
14:18:16 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:16 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:16 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:16 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:16 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:16 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:16 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:16 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:16 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 14
14:18:16 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:16 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 14 elements
14:18:16 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:16 INFO opendrift.models.chemicaldrift:1861: partitioning: [297, 0, 51, 152, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:16 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:16 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:16 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:16 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:16 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:16 INFO opendrift.models.basemodel:2011: 2024-05-04 19:17:58.364115 - step 11 of 96 - 500 active elements (0 deactivated)
14:18:16 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:16 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:16 DEBUG opendrift.models.basemodel:2030: 57.55443076092606 <- latitude -> 57.66503224166343
14:18:16 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.699237025923349
14:18:16 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.16461518586777807
14:18:16 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:16 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:16 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:16 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:16 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:16 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 19:00:00 (before)
2024-05-04 20:00:00 (after)
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:18 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x36x7) for time after (2024-05-04 20:00:00)
14:18:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 19:00:00) in space (linearNDFast)
14:18:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 20:00:00) in space (linearNDFast)
14:18:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 19:00:00, weight 0.70) and
after (2024-05-04 20:00:00, weight 0.30) in time
14:18:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.300774041270074 degrees.
14:18:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.300774041270074 degrees.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.114746 (min) 0.150858 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0367706 (min) 0.195103 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.92207 (min) 3.5308 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.0912355 (min) 0.648456 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.9561 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.05155 (min) 10.2499 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3341 (min) 32.7749 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.75435e-05 (min) 0.000154622 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:18 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:18 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:18 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 18
14:18:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 3 0 0 0 2 0 0 3 0 0 0 0 0 0]
14:18:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0 3 3 3 0 3 3 0 3 3 3 3 3 3]
14:18:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 172. 0.]
[ 0. 0. 0. 0. 0.]
[ 6. 0. 0. 23. 0.]
[ 2. 0. 28. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009152788019828318
14:18:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 27
14:18:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 27 elements
14:18:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [285, 0, 72, 143, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:18 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:18 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:18 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:18 INFO opendrift.models.basemodel:2011: 2024-05-04 19:47:58.364115 - step 12 of 96 - 500 active elements (0 deactivated)
14:18:18 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:18 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:18 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.664679506290746
14:18:18 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.69914611786848
14:18:18 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.23465756391002043
14:18:18 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 19:00:00 (before)
2024-05-04 20:00:00 (after)
14:18:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 19:00:00) in space (linearNDFast)
14:18:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 20:00:00) in space (linearNDFast)
14:18:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 19:00:00, weight 0.20) and
after (2024-05-04 20:00:00, weight 0.80) in time
14:18:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30086494999985 degrees.
14:18:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30086494999985 degrees.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.117676 (min) 0.124666 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0621627 (min) 0.197609 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.65201 (min) 2.75789 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.333147 (min) 1.26305 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.0791 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04074 (min) 10.2498 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2715 (min) 32.7977 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.86188e-06 (min) 0.000146191 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:18 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:18 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:18 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 17
14:18:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0 0]
14:18:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 3 3 3 3 3 3 3 3 3 3 3 3 3]
14:18:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 188. 0.]
[ 0. 0. 0. 0. 0.]
[ 7. 0. 0. 28. 0.]
[ 2. 0. 55. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0059871568141465145
14:18:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 41
14:18:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 41 elements
14:18:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [270, 0, 102, 128, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:18 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:18 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:18 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:18 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:18 INFO opendrift.models.basemodel:2011: 2024-05-04 20:17:58.364115 - step 13 of 96 - 500 active elements (0 deactivated)
14:18:18 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:18 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:18 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.665652262717686
14:18:18 DEBUG opendrift.models.basemodel:2035: 10.505926817056116 <- longitude -> 10.700987438033817
14:18:18 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.26346107660007745
14:18:18 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 20:00:00 (before)
2024-05-04 21:00:00 (after)
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:20 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:20 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x36x7) for time after (2024-05-04 21:00:00)
14:18:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 20:00:00) in space (linearNDFast)
14:18:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 21:00:00) in space (linearNDFast)
14:18:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 20:00:00, weight 0.70) and
after (2024-05-04 21:00:00, weight 0.30) in time
14:18:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299023624216 degrees.
14:18:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299023624216 degrees.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.12767 (min) 0.0966089 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0820118 (min) 0.176182 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.36405 (min) 2.12359 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.976213 (min) 1.76553 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.1833 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03334 (min) 10.2571 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2719 (min) 32.8145 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.54574e-06 (min) 0.00015014 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:20 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 6 elements to seafloor.
14:18:20 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:20 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 13
14:18:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 2 0 0 0 0 0 0 0]
14:18:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 0 3 3 3 3 3 3 3]
14:18:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 200. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 38. 0.]
[ 2. 0. 96. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004500071556868352
14:18:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 22
14:18:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 22 elements
14:18:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [259, 0, 110, 131, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:20 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:20 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:20 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:20 INFO opendrift.models.basemodel:2011: 2024-05-04 20:47:58.364115 - step 14 of 96 - 500 active elements (0 deactivated)
14:18:20 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:20 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:20 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.66773666930588
14:18:20 DEBUG opendrift.models.basemodel:2035: 10.505926817056118 <- longitude -> 10.701815755069799
14:18:20 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.08285489617573788
14:18:20 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 20:00:00 (before)
2024-05-04 21:00:00 (after)
14:18:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 20:00:00) in space (linearNDFast)
14:18:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 21:00:00) in space (linearNDFast)
14:18:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 20:00:00, weight 0.20) and
after (2024-05-04 21:00:00, weight 0.80) in time
14:18:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29819531072031 degrees.
14:18:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29819531072031 degrees.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.131959 (min) 0.0663711 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0988144 (min) 0.169553 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.18739 (min) 1.54843 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.84966 (min) 2.14716 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.26 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0282 (min) 10.2472 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2723 (min) 32.8274 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 9.97879e-06 (min) 0.00013551 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:20 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:20 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:20 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
14:18:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0]
14:18:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3]
14:18:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 209. 0.]
[ 0. 0. 0. 0. 0.]
[ 8. 0. 0. 51. 0.]
[ 2. 0. 118. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004942312571479194
14:18:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 11
14:18:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 11 elements
14:18:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [250, 0, 106, 144, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:20 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:20 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:20 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:20 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:20 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:20 INFO opendrift.models.basemodel:2011: 2024-05-04 21:17:58.364115 - step 15 of 96 - 500 active elements (0 deactivated)
14:18:20 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:20 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:20 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.66974928995652
14:18:20 DEBUG opendrift.models.basemodel:2035: 10.50592681705612 <- longitude -> 10.701515935620089
14:18:20 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.06204314755916884
14:18:20 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 21:00:00 (before)
2024-05-04 22:00:00 (after)
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:22 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:22 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x36x7) for time after (2024-05-04 22:00:00)
14:18:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 21:00:00) in space (linearNDFast)
14:18:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 22:00:00) in space (linearNDFast)
14:18:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 21:00:00, weight 0.70) and
after (2024-05-04 22:00:00, weight 0.30) in time
14:18:22 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29849513007421 degrees.
14:18:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29849513007421 degrees.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.129692 (min) 0.0435105 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.112134 (min) 0.148837 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.07119 (min) 1.29151 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.17798 (min) 2.61041 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.3023 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.02323 (min) 10.2329 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2727 (min) 32.8372 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.13215e-05 (min) 0.000128422 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:22 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:22 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:22 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:22 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:22 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:22 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:18:22 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0 0]
14:18:22 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3]
14:18:22 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 214. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 66. 0.]
[ 2. 0. 129. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:22 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:22 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:22 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.006095856075300738
14:18:22 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:22 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:22 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
14:18:22 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
14:18:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:22 INFO opendrift.models.chemicaldrift:1861: partitioning: [246, 0, 99, 155, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:22 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:22 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:22 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:22 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:22 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:22 INFO opendrift.models.basemodel:2011: 2024-05-04 21:47:58.364115 - step 16 of 96 - 500 active elements (0 deactivated)
14:18:22 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:22 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:22 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:22 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.700107629610029
14:18:22 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.07974290921112559
14:18:22 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 21:00:00 (before)
2024-05-04 22:00:00 (after)
14:18:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 21:00:00) in space (linearNDFast)
14:18:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 22:00:00) in space (linearNDFast)
14:18:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 21:00:00, weight 0.20) and
after (2024-05-04 22:00:00, weight 0.80) in time
14:18:22 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299903445242784 degrees.
14:18:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.299903445242784 degrees.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.119226 (min) 0.0576702 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.119491 (min) 0.128718 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.0359 (min) 1.25192 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.56708 (min) 2.99272 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.3063 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.01836 (min) 10.2129 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2743 (min) 32.8448 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 1.85022e-06 (min) 0.000114594 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:22 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:22 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:22 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:22 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:22 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:22 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
14:18:22 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0]
14:18:22 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3]
14:18:22 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 221. 0.]
[ 0. 0. 0. 0. 0.]
[ 9. 0. 0. 73. 0.]
[ 2. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:22 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:22 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:22 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007583675226466732
14:18:22 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:22 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:22 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:22 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:22 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:22 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:22 INFO opendrift.models.chemicaldrift:1861: partitioning: [239, 0, 83, 178, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:22 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:22 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:22 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:22 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:22 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:22 INFO opendrift.models.basemodel:2011: 2024-05-04 22:17:58.364115 - step 17 of 96 - 500 active elements (0 deactivated)
14:18:22 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:22 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:22 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:22 DEBUG opendrift.models.basemodel:2035: 10.505926817056126 <- longitude -> 10.698088842643214
14:18:22 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.09744596945928055
14:18:22 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 22:00:00 (before)
2024-05-04 23:00:00 (after)
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:24 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:24 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 39x36x7) for time after (2024-05-04 23:00:00)
14:18:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 22:00:00) in space (linearNDFast)
14:18:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:24 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 23:00:00) in space (linearNDFast)
14:18:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:24 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 22:00:00, weight 0.70) and
after (2024-05-04 23:00:00, weight 0.30) in time
14:18:24 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30192222738288 degrees.
14:18:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30192222738288 degrees.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.127452 (min) 0.0648402 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.122688 (min) 0.113317 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.11941 (min) 1.36928 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.59213 (min) 3.23814 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.2727 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.01678 (min) 10.2071 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2759 (min) 32.8521 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.3506e-06 (min) 9.59869e-05 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:24 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:18:24 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:24 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:24 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:24 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:24 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
14:18:24 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 0 0 0 0 0 0 0]
14:18:24 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 3 3 3 3 3 3 3]
14:18:24 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 229. 0.]
[ 0. 0. 0. 0. 0.]
[ 11. 0. 0. 89. 0.]
[ 2. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:24 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:24 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:24 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00891478133349465
14:18:24 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:24 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:24 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:24 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:24 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:24 INFO opendrift.models.chemicaldrift:1861: partitioning: [233, 0, 72, 195, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:24 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:24 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:24 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:24 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:24 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:24 INFO opendrift.models.basemodel:2011: 2024-05-04 22:47:58.364115 - step 18 of 96 - 500 active elements (0 deactivated)
14:18:24 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:24 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:24 DEBUG opendrift.models.basemodel:2030: 57.5551334290122 <- latitude -> 57.67136825582636
14:18:24 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.697958687935424
14:18:24 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.10890445675724902
14:18:24 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 22:00:00 (before)
2024-05-04 23:00:00 (after)
14:18:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 22:00:00) in space (linearNDFast)
14:18:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:24 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-04 23:00:00) in space (linearNDFast)
14:18:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:24 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 22:00:00, weight 0.20) and
after (2024-05-04 23:00:00, weight 0.80) in time
14:18:24 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30205235177045 degrees.
14:18:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.30205235177045 degrees.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.129104 (min) 0.0661438 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.122032 (min) 0.0959931 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.34462 (min) 1.63593 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.37356 (min) 3.31247 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.2217 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.01495 (min) 10.2522 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2971 (min) 32.8586 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.31777e-05 (min) 7.62896e-05 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:24 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:24 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:24 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:24 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:24 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 17
14:18:24 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0]
14:18:24 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 0 3 3 3 3 3]
14:18:24 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 245. 0.]
[ 0. 0. 0. 0. 0.]
[ 12. 0. 0. 98. 0.]
[ 2. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:24 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:24 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:24 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009658106838987122
14:18:24 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:24 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:24 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:24 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:24 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:24 INFO opendrift.models.chemicaldrift:1861: partitioning: [218, 0, 68, 214, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:24 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:24 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:24 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:24 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:24 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:24 INFO opendrift.models.basemodel:2011: 2024-05-04 23:17:58.364115 - step 19 of 96 - 500 active elements (0 deactivated)
14:18:24 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:24 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:24 DEBUG opendrift.models.basemodel:2030: 57.5551334290122 <- latitude -> 57.67136825582636
14:18:24 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.699953428755196
14:18:24 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.09832707223841103
14:18:24 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 23:00:00 (before)
2024-05-05 00:00:00 (after)
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:25 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:25 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x36x7) for time after (2024-05-05 00:00:00)
14:18:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 23:00:00) in space (linearNDFast)
14:18:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 00:00:00) in space (linearNDFast)
14:18:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 23:00:00, weight 0.70) and
after (2024-05-05 00:00:00, weight 0.30) in time
14:18:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.3000576133535 degrees.
14:18:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.3000576133535 degrees.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.144658 (min) 0.059197 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.110351 (min) 0.0826047 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 1.00674 (min) 1.42626 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.16279 (min) 3.00786 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.1892 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.01371 (min) 10.1951 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.45 (min) 32.8594 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.13125e-05 (min) 5.10731e-05 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:25 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:25 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:25 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:25 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 16
14:18:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0]
14:18:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 0 3 3 3 3 3]
14:18:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 260. 0.]
[ 0. 0. 0. 0. 0.]
[ 13. 0. 0. 101. 0.]
[ 2. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00776487773152929
14:18:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [204, 0, 61, 235, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:25 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:25 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:25 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:25 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:25 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:25 INFO opendrift.models.basemodel:2011: 2024-05-04 23:47:58.364115 - step 20 of 96 - 500 active elements (0 deactivated)
14:18:25 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:25 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:25 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:25 DEBUG opendrift.models.basemodel:2035: 10.505926817056118 <- longitude -> 10.701731874189935
14:18:25 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.11613308637445052
14:18:25 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-04 23:00:00 (before)
2024-05-05 00:00:00 (after)
14:18:25 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-04 23:00:00) in space (linearNDFast)
14:18:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:25 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 00:00:00) in space (linearNDFast)
14:18:25 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:25 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-04 23:00:00, weight 0.20) and
after (2024-05-05 00:00:00, weight 0.80) in time
14:18:25 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:25 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:25 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:25 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:25 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:25 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:25 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:25 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.153601 (min) 0.0566331 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.105817 (min) 0.0677846 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.318692 (min) 0.92579 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.86914 (min) 2.49019 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.2222 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0142 (min) 10.1869 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.5225 (min) 32.8563 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.8583e-05 (min) 3.18272e-05 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:25 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:25 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:25 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:25 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:25 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:25 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:25 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
14:18:25 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0 0 0 0 0 0 0 0]
14:18:25 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3 3 3 3 3 3 3 3]
14:18:25 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 270. 0.]
[ 0. 0. 0. 0. 0.]
[ 15. 0. 0. 107. 0.]
[ 2. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:25 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:25 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:25 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004855875935075073
14:18:25 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:25 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:25 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:25 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:25 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:25 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:25 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:25 INFO opendrift.models.chemicaldrift:1861: partitioning: [196, 0, 55, 249, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:25 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:25 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:25 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:25 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:25 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:25 INFO opendrift.models.basemodel:2011: 2024-05-05 00:17:58.364115 - step 21 of 96 - 500 active elements (0 deactivated)
14:18:25 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:25 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:25 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:25 DEBUG opendrift.models.basemodel:2035: 10.505926817056118 <- longitude -> 10.701731874189935
14:18:25 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.15084669945115564
14:18:25 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:25 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:25 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:25 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:25 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:25 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 00:00:00 (before)
2024-05-05 01:00:00 (after)
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:27 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:27 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x36x7) for time after (2024-05-05 01:00:00)
14:18:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 00:00:00) in space (linearNDFast)
14:18:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:27 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 01:00:00) in space (linearNDFast)
14:18:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:27 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 00:00:00, weight 0.70) and
after (2024-05-05 01:00:00, weight 0.30) in time
14:18:27 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.154535 (min) 0.0639054 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.122451 (min) 0.0561387 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.0609604 (min) 0.490098 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.94244 (min) 2.68923 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.2912 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.01766 (min) 10.181 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4096 (min) 32.8466 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.65373e-05 (min) 2.50006e-05 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:27 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:27 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:27 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:27 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:27 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
14:18:27 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 3 0]
14:18:27 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 0 3]
14:18:27 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 276. 0.]
[ 0. 0. 0. 0. 0.]
[ 15. 0. 0. 111. 0.]
[ 3. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:27 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:27 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:27 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0052897073995196425
14:18:27 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:27 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:27 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:27 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:27 INFO opendrift.models.chemicaldrift:1861: partitioning: [191, 0, 48, 261, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:27 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:27 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:27 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:27 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:27 INFO opendrift.models.basemodel:2011: 2024-05-05 00:47:58.364115 - step 22 of 96 - 500 active elements (0 deactivated)
14:18:27 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:27 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:27 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:27 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.701731874189935
14:18:27 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.1856874525967137
14:18:27 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 00:00:00 (before)
2024-05-05 01:00:00 (after)
14:18:27 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 00:00:00) in space (linearNDFast)
14:18:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:27 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 01:00:00) in space (linearNDFast)
14:18:27 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:27 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 00:00:00, weight 0.20) and
after (2024-05-05 01:00:00, weight 0.80) in time
14:18:27 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:27 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:27 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:27 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:27 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:27 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:27 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:27 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.149959 (min) 0.0803347 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.126462 (min) 0.0570957 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.246538 (min) 0.344134 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.20745 (min) 3.22696 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.3756 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0231 (min) 10.1766 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4306 (min) 32.8324 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000102833 (min) 1.97825e-05 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:27 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:27 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:27 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:27 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:27 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:27 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:27 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
14:18:27 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0]
14:18:27 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3]
14:18:27 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 285. 0.]
[ 0. 0. 0. 0. 0.]
[ 15. 0. 0. 118. 0.]
[ 3. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:27 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:27 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:27 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007614330190401973
14:18:27 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:27 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:27 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:27 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:27 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:27 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:27 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:27 INFO opendrift.models.chemicaldrift:1861: partitioning: [182, 0, 44, 274, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:27 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:27 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:27 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:27 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:27 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:27 INFO opendrift.models.basemodel:2011: 2024-05-05 01:17:58.364115 - step 23 of 96 - 500 active elements (0 deactivated)
14:18:27 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:27 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:27 DEBUG opendrift.models.basemodel:2030: 57.55494849931836 <- latitude -> 57.67136825582636
14:18:27 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.701731874189935
14:18:27 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.2780910458305909
14:18:27 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 01:00:00 (before)
2024-05-05 02:00:00 (after)
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:28 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:28 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x36x7) for time after (2024-05-05 02:00:00)
14:18:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 01:00:00) in space (linearNDFast)
14:18:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 02:00:00) in space (linearNDFast)
14:18:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 01:00:00, weight 0.70) and
after (2024-05-05 02:00:00, weight 0.30) in time
14:18:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:28 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:28 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:28 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:28 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:28 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:28 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:28 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:28 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:28 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:28 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.138626 (min) 0.0948405 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.112032 (min) 0.0983336 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.205635 (min) 0.394485 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.20913 (min) 3.14887 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.4683 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0285 (min) 10.1719 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4402 (min) 32.8156 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000110776 (min) 1.53195e-05 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:28 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:28 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:18:28 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:28 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:28 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:28 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:28 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
14:18:28 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 0]
14:18:28 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 3]
14:18:28 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 297. 0.]
[ 0. 0. 0. 0. 0.]
[ 15. 0. 0. 122. 0.]
[ 3. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:28 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:28 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:28 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007281415534488715
14:18:28 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:28 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:28 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:28 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:28 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:28 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:28 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:28 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:28 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:28 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:28 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:28 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:28 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:28 INFO opendrift.models.chemicaldrift:1861: partitioning: [170, 0, 38, 292, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:28 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:28 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:28 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:28 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:28 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:28 INFO opendrift.models.basemodel:2011: 2024-05-05 01:47:58.364115 - step 24 of 96 - 500 active elements (0 deactivated)
14:18:28 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:28 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:28 DEBUG opendrift.models.basemodel:2030: 57.554763581596525 <- latitude -> 57.67136825582636
14:18:28 DEBUG opendrift.models.basemodel:2035: 10.505926817056123 <- longitude -> 10.701731874189935
14:18:28 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.1665783502063392
14:18:28 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:28 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:28 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:28 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:28 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:28 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 01:00:00 (before)
2024-05-05 02:00:00 (after)
14:18:28 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 01:00:00) in space (linearNDFast)
14:18:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:28 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 02:00:00) in space (linearNDFast)
14:18:28 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:28 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 01:00:00, weight 0.20) and
after (2024-05-05 02:00:00, weight 0.80) in time
14:18:28 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.126993 (min) 0.104991 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0852612 (min) 0.143402 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.113169 (min) 0.439548 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: 2.03199 (min) 2.76496 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.2745 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03386 (min) 10.1669 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2345 (min) 32.7971 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000118879 (min) 8.34084e-06 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:29 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:29 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:29 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:29 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:29 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:29 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 3]
14:18:29 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0]
14:18:29 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 299. 0.]
[ 0. 0. 0. 0. 0.]
[ 15. 0. 0. 128. 0.]
[ 4. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:29 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:29 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00554264968701973
14:18:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:29 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:29 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:29 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:29 INFO opendrift.models.chemicaldrift:1861: partitioning: [169, 0, 33, 298, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:29 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:29 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:29 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:29 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:29 INFO opendrift.models.basemodel:2011: 2024-05-05 02:17:58.364115 - step 25 of 96 - 500 active elements (0 deactivated)
14:18:29 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:29 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:29 DEBUG opendrift.models.basemodel:2030: 57.5550080544532 <- latitude -> 57.67136825582636
14:18:29 DEBUG opendrift.models.basemodel:2035: 10.505926817056118 <- longitude -> 10.701731874189939
14:18:29 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.25296104285093995
14:18:29 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 02:00:00 (before)
2024-05-05 03:00:00 (after)
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:30 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:30 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x36x7) for time after (2024-05-05 03:00:00)
14:18:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 02:00:00) in space (linearNDFast)
14:18:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:30 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 03:00:00) in space (linearNDFast)
14:18:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:30 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 02:00:00, weight 0.70) and
after (2024-05-05 03:00:00, weight 0.30) in time
14:18:30 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.110807 (min) 0.109354 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.058411 (min) 0.188975 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.179164 (min) 0.450739 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.80048 (min) 2.6139 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 24.0282 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03889 (min) 10.1619 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.204 (min) 32.7812 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000115692 (min) 1.10522e-05 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:30 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:30 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:30 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:30 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:30 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:30 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:30 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
14:18:30 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0 0 0 0 0 0 0 0]
14:18:30 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3 3 3 3 3 3 3 3]
14:18:30 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 310. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 133. 0.]
[ 4. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:30 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:30 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:30 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004943983360570793
14:18:30 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:30 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:30 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:30 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:30 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:30 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:30 INFO opendrift.models.chemicaldrift:1861: partitioning: [159, 0, 30, 311, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:30 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:30 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:30 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:30 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:30 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:30 INFO opendrift.models.basemodel:2011: 2024-05-05 02:47:58.364115 - step 26 of 96 - 500 active elements (0 deactivated)
14:18:30 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:30 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:30 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67136825582636
14:18:30 DEBUG opendrift.models.basemodel:2035: 10.505926817056121 <- longitude -> 10.701731874189944
14:18:30 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2641821075116913
14:18:30 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 02:00:00 (before)
2024-05-05 03:00:00 (after)
14:18:30 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 02:00:00) in space (linearNDFast)
14:18:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:30 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 03:00:00) in space (linearNDFast)
14:18:30 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:30 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 02:00:00, weight 0.20) and
after (2024-05-05 03:00:00, weight 0.80) in time
14:18:30 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:30 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:30 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:30 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:30 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:30 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:30 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:30 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.098138 (min) 0.111048 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0413818 (min) 0.261754 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.402384 (min) 0.238451 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.48396 (min) 2.41943 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.7939 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0437 (min) 10.1569 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2642 (min) 32.7672 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000103785 (min) 1.87552e-05 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:30 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:30 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:30 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:30 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:30 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:30 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:30 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:30 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 12
14:18:30 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0 0 0 0 3]
14:18:30 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3 3 3 3 0]
14:18:30 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 321. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 135. 0.]
[ 5. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:30 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:30 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:30 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00435087176795597
14:18:30 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:30 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:30 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:30 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:30 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:30 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:30 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:30 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:30 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:30 INFO opendrift.models.chemicaldrift:1861: partitioning: [149, 0, 28, 323, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:30 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:30 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:30 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:30 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:30 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:30 INFO opendrift.models.basemodel:2011: 2024-05-05 03:17:58.364115 - step 27 of 96 - 500 active elements (0 deactivated)
14:18:30 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:30 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:30 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.6716373703728
14:18:30 DEBUG opendrift.models.basemodel:2035: 10.505926817056125 <- longitude -> 10.701731874189944
14:18:30 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.25181075872963754
14:18:30 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:30 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:30 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:30 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:30 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:30 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 03:00:00 (before)
2024-05-05 04:00:00 (after)
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:32 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:32 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x35x7) for time after (2024-05-05 04:00:00)
14:18:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 03:00:00) in space (linearNDFast)
14:18:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 04:00:00) in space (linearNDFast)
14:18:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 03:00:00, weight 0.70) and
after (2024-05-05 04:00:00, weight 0.30) in time
14:18:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.086061 (min) 0.111118 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0206251 (min) 0.291425 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.603472 (min) 0.138361 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.42796 (min) 2.35145 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.5687 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04542 (min) 10.1549 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2713 (min) 32.7585 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.04769e-05 (min) 2.93463e-05 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:32 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:32 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:32 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:32 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:18:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0]
14:18:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3]
14:18:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 326. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 137. 0.]
[ 5. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004262668137488784
14:18:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [144, 0, 28, 328, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:32 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:32 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:32 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:32 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:32 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:32 INFO opendrift.models.basemodel:2011: 2024-05-05 03:47:58.364115 - step 28 of 96 - 500 active elements (0 deactivated)
14:18:32 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:32 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:32 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67482798422698
14:18:32 DEBUG opendrift.models.basemodel:2035: 10.505926817056125 <- longitude -> 10.701731874189944
14:18:32 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.32692286751377564
14:18:32 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 03:00:00 (before)
2024-05-05 04:00:00 (after)
14:18:32 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 03:00:00) in space (linearNDFast)
14:18:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:32 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 04:00:00) in space (linearNDFast)
14:18:32 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:32 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 03:00:00, weight 0.20) and
after (2024-05-05 04:00:00, weight 0.80) in time
14:18:32 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:32 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:32 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:32 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:32 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:32 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:32 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:32 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0624807 (min) 0.167207 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00404309 (min) 0.308291 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.711468 (min) 0.125421 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.54428 (min) 2.36812 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.3738 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04507 (min) 10.1549 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2806 (min) 32.7533 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.97863e-05 (min) 4.26173e-05 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:32 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:32 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:32 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:32 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:32 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:32 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:32 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:32 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:32 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 3 0 0]
14:18:32 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3]
14:18:32 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 329. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 137. 0.]
[ 6. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:32 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:32 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:32 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004422120816777584
14:18:32 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:32 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:32 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:32 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:32 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:32 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:32 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:32 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:32 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:32 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:32 INFO opendrift.models.chemicaldrift:1861: partitioning: [142, 0, 26, 332, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:32 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:32 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:32 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:32 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:32 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:32 INFO opendrift.models.basemodel:2011: 2024-05-05 04:17:58.364115 - step 29 of 96 - 500 active elements (0 deactivated)
14:18:32 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:32 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:32 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.67893029305555
14:18:32 DEBUG opendrift.models.basemodel:2035: 10.505926817056125 <- longitude -> 10.70173187418994
14:18:32 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.41025248033994416
14:18:32 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:32 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:32 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:32 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:32 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:32 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 04:00:00 (before)
2024-05-05 05:00:00 (after)
14:18:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:33 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:33 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 40x35x7) for time after (2024-05-05 05:00:00)
14:18:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 04:00:00) in space (linearNDFast)
14:18:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 05:00:00) in space (linearNDFast)
14:18:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 04:00:00, weight 0.70) and
after (2024-05-05 05:00:00, weight 0.30) in time
14:18:33 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494084269314705 and -59.29827916509341 degrees.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0437008 (min) 0.203165 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0281263 (min) 0.315699 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.746611 (min) 0.0282895 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.46868 (min) 2.4395 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.2016 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04471 (min) 10.1573 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2804 (min) 32.7542 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.4537e-05 (min) 5.32821e-05 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:34 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:34 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:34 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:34 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0]
14:18:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3]
14:18:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 333. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 139. 0.]
[ 6. 0. 130. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004699801462467781
14:18:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:18:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:18:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [138, 0, 28, 334, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:34 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:34 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:34 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:34 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:34 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:34 INFO opendrift.models.basemodel:2011: 2024-05-05 04:47:58.364115 - step 30 of 96 - 500 active elements (0 deactivated)
14:18:34 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:34 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:34 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.68307619701841
14:18:34 DEBUG opendrift.models.basemodel:2035: 10.505926817056125 <- longitude -> 10.701731874189944
14:18:34 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2613066328139133
14:18:34 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 04:00:00 (before)
2024-05-05 05:00:00 (after)
14:18:34 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 04:00:00) in space (linearNDFast)
14:18:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:34 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 05:00:00) in space (linearNDFast)
14:18:34 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:34 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 04:00:00, weight 0.20) and
after (2024-05-05 05:00:00, weight 0.80) in time
14:18:34 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427242647 and -59.29827916509341 degrees.
14:18:34 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49408427242647 and -59.29827916509341 degrees.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:34 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:34 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:34 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:34 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:34 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:34 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0502548 (min) 0.217919 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.051818 (min) 0.303022 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.779546 (min) -0.113144 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.23722 (min) 2.42684 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48835 (min) 23.0689 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04432 (min) 10.1613 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2911 (min) 32.759 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.96771e-05 (min) 6.63571e-05 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:34 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:34 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:34 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:34 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:34 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:34 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:34 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:34 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
14:18:34 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0 0 0]
14:18:34 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3 3 3]
14:18:34 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 341. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 139. 0.]
[ 6. 0. 132. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:34 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:34 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:34 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004698940030796676
14:18:34 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:34 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:34 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:34 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:34 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:34 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:34 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:34 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:34 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:34 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
14:18:34 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:34 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
14:18:34 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:34 INFO opendrift.models.chemicaldrift:1861: partitioning: [130, 0, 27, 343, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:34 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:34 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:34 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:34 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:34 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:34 INFO opendrift.models.basemodel:2011: 2024-05-05 05:17:58.364115 - step 31 of 96 - 500 active elements (0 deactivated)
14:18:34 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:34 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:34 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.686961697507385
14:18:34 DEBUG opendrift.models.basemodel:2035: 10.506037010995488 <- longitude -> 10.701731874189944
14:18:34 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2916876587057538
14:18:34 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:34 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:34 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:34 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:34 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:34 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 05:00:00 (before)
2024-05-05 06:00:00 (after)
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 41x35x7) for time after (2024-05-05 06:00:00)
14:18:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 05:00:00) in space (linearNDFast)
14:18:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 06:00:00) in space (linearNDFast)
14:18:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 05:00:00, weight 0.70) and
after (2024-05-05 06:00:00, weight 0.30) in time
14:18:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49397408298435 and -59.29827916509341 degrees.
14:18:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49397408298435 and -59.29827916509341 degrees.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0590937 (min) 0.240534 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0602535 (min) 0.275098 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.903436 (min) -0.27231 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.14008 (min) 2.43025 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.40766 (min) 22.9594 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04378 (min) 10.161 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3521 (min) 32.7673 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.40861e-05 (min) 7.78542e-05 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:35 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:35 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:35 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:35 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:35 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:35 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:35 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0]
14:18:35 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3]
14:18:35 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 345. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 143. 0.]
[ 6. 0. 135. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:35 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:35 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004861595717805187
14:18:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:35 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:35 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 8
14:18:35 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 8 elements
14:18:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:35 INFO opendrift.models.chemicaldrift:1861: partitioning: [126, 0, 34, 340, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:35 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:35 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:35 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:35 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:35 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:35 INFO opendrift.models.basemodel:2011: 2024-05-05 05:47:58.364115 - step 32 of 96 - 500 active elements (0 deactivated)
14:18:35 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:35 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:35 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.690743331580016
14:18:35 DEBUG opendrift.models.basemodel:2035: 10.506476912326118 <- longitude -> 10.700991257983747
14:18:35 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.341435828228935
14:18:35 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 05:00:00 (before)
2024-05-05 06:00:00 (after)
14:18:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 05:00:00) in space (linearNDFast)
14:18:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 06:00:00) in space (linearNDFast)
14:18:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 05:00:00, weight 0.20) and
after (2024-05-05 06:00:00, weight 0.80) in time
14:18:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49353418174478 and -59.29901978183179 degrees.
14:18:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49353418174478 and -59.29901978183179 degrees.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0667614 (min) 0.276107 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0666314 (min) 0.249474 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.10565 (min) -0.471919 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.0781 (min) 2.477 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.33789 (min) 22.8872 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04314 (min) 10.1614 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3954 (min) 32.778 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.16766e-05 (min) 9.02431e-05 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:36 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:36 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:36 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:36 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:36 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:36 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:36 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:18:36 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:18:36 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 348. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 144. 0.]
[ 6. 0. 143. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:36 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:36 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:36 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0051469493719305745
14:18:36 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:36 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:36 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:36 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:36 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:36 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:36 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 20
14:18:36 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:36 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 20 elements
14:18:36 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:36 INFO opendrift.models.chemicaldrift:1861: partitioning: [123, 0, 48, 329, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:36 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:36 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:36 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:36 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:36 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:36 INFO opendrift.models.basemodel:2011: 2024-05-05 06:17:58.364115 - step 33 of 96 - 500 active elements (0 deactivated)
14:18:36 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:36 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:36 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.69440431585266
14:18:36 DEBUG opendrift.models.basemodel:2035: 10.507282913393603 <- longitude -> 10.700647825217004
14:18:36 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.40452560356693323
14:18:36 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:36 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:36 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:36 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:36 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:36 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:36 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:36 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:36 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 06:00:00 (before)
2024-05-05 07:00:00 (after)
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:37 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:37 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 42x35x7) for time after (2024-05-05 07:00:00)
14:18:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 06:00:00) in space (linearNDFast)
14:18:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 07:00:00) in space (linearNDFast)
14:18:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 06:00:00, weight 0.70) and
after (2024-05-05 07:00:00, weight 0.30) in time
14:18:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4927281738274 and -59.29936322625978 degrees.
14:18:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.4927281738274 and -59.29936322625978 degrees.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0734578 (min) 0.282715 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0721001 (min) 0.239446 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.23509 (min) -0.518633 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.05811 (min) 2.43592 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.27302 (min) 22.8273 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04251 (min) 10.1672 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3864 (min) 32.7861 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -3.80471e-05 (min) 9.89746e-05 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:37 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:37 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:37 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:37 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:37 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:18:37 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:18:37 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 351. 0.]
[ 0. 0. 0. 0. 0.]
[ 16. 0. 0. 150. 0.]
[ 6. 0. 163. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:37 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:37 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:37 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004884943516981132
14:18:37 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:37 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:37 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:37 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:37 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:37 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
14:18:37 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:37 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
14:18:37 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:37 INFO opendrift.models.chemicaldrift:1861: partitioning: [120, 0, 60, 320, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:37 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:37 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:37 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:37 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:37 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:37 INFO opendrift.models.basemodel:2011: 2024-05-05 06:47:58.364115 - step 34 of 96 - 500 active elements (0 deactivated)
14:18:37 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:37 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:37 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.69776683230617
14:18:37 DEBUG opendrift.models.basemodel:2035: 10.508375140611786 <- longitude -> 10.700712379569353
14:18:37 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.3517384550415596
14:18:37 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:37 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 06:00:00 (before)
2024-05-05 07:00:00 (after)
14:18:37 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 06:00:00) in space (linearNDFast)
14:18:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:37 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 07:00:00) in space (linearNDFast)
14:18:37 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:37 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 06:00:00, weight 0.20) and
after (2024-05-05 07:00:00, weight 0.80) in time
14:18:37 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49163593393201 and -59.29929865877493 degrees.
14:18:37 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49163593393201 and -59.29929865877493 degrees.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:37 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:37 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:37 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:37 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:37 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:37 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:37 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:37 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:37 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:37 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:37 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0795043 (min) 0.277125 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.070542 (min) 0.225163 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.29863 (min) -0.485322 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.10601 (min) 2.28427 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.2237 (min) 22.7607 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04111 (min) 10.1755 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3461 (min) 32.7924 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.98728e-05 (min) 0.000105773 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:37 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:37 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:37 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:37 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:37 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:37 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:37 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:37 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
14:18:38 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 0 0 0 0 0]
14:18:38 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 3 3 3 3 3]
14:18:38 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 359. 0.]
[ 0. 0. 0. 0. 0.]
[ 17. 0. 0. 151. 0.]
[ 6. 0. 176. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:38 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:38 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:38 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004445323867189122
14:18:38 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:38 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:38 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:38 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:38 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:38 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 33
14:18:38 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 33 elements
14:18:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:38 INFO opendrift.models.chemicaldrift:1861: partitioning: [113, 0, 85, 302, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:38 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:38 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:38 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:38 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:38 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:38 INFO opendrift.models.basemodel:2011: 2024-05-05 07:17:58.364115 - step 35 of 96 - 500 active elements (0 deactivated)
14:18:38 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:38 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:38 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.700757864832504
14:18:38 DEBUG opendrift.models.basemodel:2035: 10.50966797676792 <- longitude -> 10.7037512631838
14:18:38 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.20804000855063678
14:18:38 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 07:00:00 (before)
2024-05-05 08:00:00 (after)
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:39 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:39 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 43x35x7) for time after (2024-05-05 08:00:00)
14:18:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 07:00:00) in space (linearNDFast)
14:18:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 08:00:00) in space (linearNDFast)
14:18:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 07:00:00, weight 0.70) and
after (2024-05-05 08:00:00, weight 0.30) in time
14:18:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49034310692249 and -59.29625977542278 degrees.
14:18:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49034310692249 and -59.29625977542278 degrees.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0933872 (min) 0.241897 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0641669 (min) 0.216548 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.37972 (min) -0.552328 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.26927 (min) 2.12979 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.18477 (min) 22.7607 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03917 (min) 10.189 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3908 (min) 32.8001 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.58099e-05 (min) 0.000109193 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:39 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:39 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:39 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:39 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:39 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:39 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:39 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:18:39 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 2]
14:18:39 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0]
14:18:39 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 363. 0.]
[ 0. 0. 0. 0. 0.]
[ 18. 0. 0. 158. 0.]
[ 6. 0. 209. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:39 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:39 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:39 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004172419048109934
14:18:39 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:39 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:39 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:39 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:39 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:39 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 15
14:18:39 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:39 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 15 elements
14:18:39 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:39 INFO opendrift.models.chemicaldrift:1861: partitioning: [110, 0, 85, 305, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:39 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:39 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:39 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:39 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:39 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:39 INFO opendrift.models.basemodel:2011: 2024-05-05 07:47:58.364115 - step 36 of 96 - 500 active elements (0 deactivated)
14:18:39 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:39 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:39 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.703898106765365
14:18:39 DEBUG opendrift.models.basemodel:2035: 10.511115856613486 <- longitude -> 10.708012248633999
14:18:39 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.28630542825294897
14:18:39 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:39 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 07:00:00 (before)
2024-05-05 08:00:00 (after)
14:18:39 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 07:00:00) in space (linearNDFast)
14:18:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:39 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 08:00:00) in space (linearNDFast)
14:18:39 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:39 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 07:00:00, weight 0.20) and
after (2024-05-05 08:00:00, weight 0.80) in time
14:18:39 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48889523926336 and -59.291998787942504 degrees.
14:18:39 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48889523926336 and -59.291998787942504 degrees.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:39 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:39 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:39 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:39 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:39 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:39 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:39 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:39 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:39 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:39 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:39 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.106117 (min) 0.188947 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0545682 (min) 0.199758 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.50948 (min) -0.652789 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.43947 (min) 1.97073 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.16456 (min) 22.7607 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03928 (min) 10.2172 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3547 (min) 32.8087 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.37306e-05 (min) 0.000114441 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:39 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:40 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:40 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:40 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 3]
14:18:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0]
14:18:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 365. 0.]
[ 0. 0. 0. 0. 0.]
[ 19. 0. 0. 172. 0.]
[ 7. 0. 224. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004285593164358931
14:18:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
14:18:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
14:18:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [110, 0, 86, 304, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:40 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:40 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:40 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:40 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:40 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:40 INFO opendrift.models.basemodel:2011: 2024-05-05 08:17:58.364115 - step 37 of 96 - 500 active elements (0 deactivated)
14:18:40 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:40 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:40 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.70632337880528
14:18:40 DEBUG opendrift.models.basemodel:2035: 10.512696053994517 <- longitude -> 10.712932134164651
14:18:40 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2521293508043557
14:18:40 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 08:00:00 (before)
2024-05-05 09:00:00 (after)
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:41 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:41 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 43x35x7) for time after (2024-05-05 09:00:00)
14:18:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 08:00:00) in space (linearNDFast)
14:18:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 09:00:00) in space (linearNDFast)
14:18:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 08:00:00, weight 0.70) and
after (2024-05-05 09:00:00, weight 0.30) in time
14:18:41 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.487315030344064 and -59.287078896614716 degrees.
14:18:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.487315030344064 and -59.287078896614716 degrees.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.111312 (min) 0.167547 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0312262 (min) 0.168094 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.77896 (min) -0.996748 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: 1.20033 (min) 1.69422 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.16319 (min) 22.7885 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03933 (min) 10.248 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3459 (min) 32.8183 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.23239e-06 (min) 0.000113945 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:41 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:41 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:41 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:41 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:41 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:41 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:41 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:18:41 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 0 0]
14:18:41 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3]
14:18:41 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 369. 0.]
[ 0. 0. 0. 0. 0.]
[ 19. 0. 0. 183. 0.]
[ 8. 0. 237. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:41 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:41 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:41 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:41 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00423256480756424
14:18:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:18:41 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:41 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:18:41 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:41 INFO opendrift.models.chemicaldrift:1861: partitioning: [107, 0, 73, 320, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:41 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:41 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:41 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:41 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:41 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:41 INFO opendrift.models.basemodel:2011: 2024-05-05 08:47:58.364115 - step 38 of 96 - 500 active elements (0 deactivated)
14:18:41 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:41 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:41 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.708424350118506
14:18:41 DEBUG opendrift.models.basemodel:2035: 10.513524818110788 <- longitude -> 10.716227545388652
14:18:41 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.20251835842406507
14:18:41 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 08:00:00 (before)
2024-05-05 09:00:00 (after)
14:18:41 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 08:00:00) in space (linearNDFast)
14:18:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:41 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 09:00:00) in space (linearNDFast)
14:18:41 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:41 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 08:00:00, weight 0.20) and
after (2024-05-05 09:00:00, weight 0.80) in time
14:18:41 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.283783484418805 degrees.
14:18:41 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.283783484418805 degrees.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:41 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:41 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:41 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:41 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:41 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:41 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.111467 (min) 0.12164 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0179713 (min) 0.135961 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.04129 (min) -1.50193 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.843811 (min) 1.34676 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 22.8203 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.03933 (min) 10.2872 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.349 (min) 32.8287 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.33568e-07 (min) 0.000191266 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:41 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:41 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:41 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:41 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:41 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:41 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:41 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:41 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 0
14:18:41 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:41 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.004139774229971307
14:18:41 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:41 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:41 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:41 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:41 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:41 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:41 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:41 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:41 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:41 INFO opendrift.models.chemicaldrift:1861: partitioning: [107, 0, 64, 329, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:41 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:41 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:41 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:41 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:41 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:41 INFO opendrift.models.basemodel:2011: 2024-05-05 09:17:58.364115 - step 39 of 96 - 500 active elements (0 deactivated)
14:18:41 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:41 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:41 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.70990100855683
14:18:41 DEBUG opendrift.models.basemodel:2035: 10.513524818110788 <- longitude -> 10.71789113138316
14:18:41 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.22823551574173095
14:18:41 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:41 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:41 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:41 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:41 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:41 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 09:00:00 (before)
2024-05-05 10:00:00 (after)
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:43 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:43 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 44x35x7) for time after (2024-05-05 10:00:00)
14:18:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 09:00:00) in space (linearNDFast)
14:18:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 10:00:00) in space (linearNDFast)
14:18:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 09:00:00, weight 0.70) and
after (2024-05-05 10:00:00, weight 0.30) in time
14:18:43 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28211989825473 degrees.
14:18:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28211989825473 degrees.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.104708 (min) 0.114038 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0577652 (min) 0.132532 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.33424 (min) -1.7847 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.715441 (min) 1.21974 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 22.8877 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04022 (min) 10.3257 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3536 (min) 32.839 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: 2.08652e-06 (min) 0.000146951 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:43 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:43 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:43 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:43 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:43 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:43 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:43 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:43 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 3 0 2]
14:18:43 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0]
14:18:43 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 371. 0.]
[ 0. 0. 0. 0. 0.]
[ 20. 0. 0. 207. 0.]
[ 9. 0. 239. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:43 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:43 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:43 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:43 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0046268295503039585
14:18:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:43 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:43 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:43 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:43 INFO opendrift.models.chemicaldrift:1861: partitioning: [107, 0, 60, 333, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:43 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:43 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:43 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:43 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:43 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:43 INFO opendrift.models.basemodel:2011: 2024-05-05 09:47:58.364115 - step 40 of 96 - 500 active elements (0 deactivated)
14:18:43 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:43 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:43 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.71204293865929
14:18:43 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.718056789153572
14:18:43 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.24640753256110148
14:18:43 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 09:00:00 (before)
2024-05-05 10:00:00 (after)
14:18:43 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 09:00:00) in space (linearNDFast)
14:18:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:43 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 10:00:00) in space (linearNDFast)
14:18:43 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:43 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 09:00:00, weight 0.20) and
after (2024-05-05 10:00:00, weight 0.80) in time
14:18:43 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.281954244388125 degrees.
14:18:43 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.281954244388125 degrees.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:43 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:43 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:43 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:43 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:43 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:43 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0933235 (min) 0.0880527 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0935544 (min) 0.156269 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.64768 (min) -1.92576 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.677635 (min) 1.23319 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 22.9489 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.0417 (min) 10.4321 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3939 (min) 32.8495 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -5.81561e-06 (min) 0.000234261 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:43 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:43 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:43 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:43 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:43 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:43 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:43 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:43 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:43 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:18:43 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:18:43 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 374. 0.]
[ 0. 0. 0. 0. 0.]
[ 20. 0. 0. 210. 0.]
[ 9. 0. 239. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:43 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:43 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:43 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:43 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:43 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.005489195468768781
14:18:43 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:43 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:43 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:43 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:43 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:43 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:43 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:43 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:43 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:43 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:43 INFO opendrift.models.chemicaldrift:1861: partitioning: [104, 0, 53, 343, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:43 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:43 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:43 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:43 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:43 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:43 INFO opendrift.models.basemodel:2011: 2024-05-05 10:17:58.364115 - step 41 of 96 - 500 active elements (0 deactivated)
14:18:43 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:43 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:43 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.71456852297511
14:18:43 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.716800992057989
14:18:43 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2852586709922489
14:18:43 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:43 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:43 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:43 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:43 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:43 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 10:00:00 (before)
2024-05-05 11:00:00 (after)
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:45 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:45 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 44x35x7) for time after (2024-05-05 11:00:00)
14:18:45 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 10:00:00) in space (linearNDFast)
14:18:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:45 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 11:00:00) in space (linearNDFast)
14:18:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:45 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 10:00:00, weight 0.70) and
after (2024-05-05 11:00:00, weight 0.30) in time
14:18:45 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28321003385815 degrees.
14:18:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28321003385815 degrees.
14:18:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:45 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:45 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:45 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:45 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.0828723 (min) 0.0765188 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.125907 (min) 0.171445 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.89005 (min) -2.10845 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.516333 (min) 0.990444 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.002 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04529 (min) 10.507 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3701 (min) 32.8567 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -1.57465e-05 (min) 0.000256531 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:45 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:45 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:45 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:45 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:45 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:45 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
14:18:45 DEBUG opendrift.models.chemicaldrift:1452: old species: [0]
14:18:45 DEBUG opendrift.models.chemicaldrift:1453: new species: [3]
14:18:45 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 375. 0.]
[ 0. 0. 0. 0. 0.]
[ 20. 0. 0. 217. 0.]
[ 9. 0. 239. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:45 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:45 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:45 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:45 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:45 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.006302182641053685
14:18:45 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:45 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:45 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:45 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:45 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:45 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:45 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:45 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:45 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:45 INFO opendrift.models.chemicaldrift:1861: partitioning: [103, 0, 49, 348, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:45 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:45 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:45 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:45 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:45 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:45 INFO opendrift.models.basemodel:2011: 2024-05-05 10:47:58.364115 - step 42 of 96 - 500 active elements (0 deactivated)
14:18:45 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:45 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:45 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.71733939079193
14:18:45 DEBUG opendrift.models.basemodel:2035: 10.513524818110792 <- longitude -> 10.714360673304768
14:18:45 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.025316383243516274
14:18:45 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:45 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 10:00:00 (before)
2024-05-05 11:00:00 (after)
14:18:45 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 10:00:00) in space (linearNDFast)
14:18:45 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:46 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 11:00:00) in space (linearNDFast)
14:18:46 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:46 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 10:00:00, weight 0.20) and
after (2024-05-05 11:00:00, weight 0.80) in time
14:18:46 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.285650360111575 degrees.
14:18:46 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.285650360111575 degrees.
14:18:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:46 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:46 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:46 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:46 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:46 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:46 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:46 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:46 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:46 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.116105 (min) 0.0403713 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.188992 (min) 0.0982548 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.08487 (min) -2.35451 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.373786 (min) 0.677188 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.0399 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.04889 (min) 10.5313 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3642 (min) 32.8618 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -2.89752e-05 (min) 0.000204942 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:46 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:46 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:46 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:46 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:46 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:46 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:46 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:46 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:46 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0]
14:18:46 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3]
14:18:46 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 377. 0.]
[ 0. 0. 0. 0. 0.]
[ 21. 0. 0. 221. 0.]
[ 9. 0. 239. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:46 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:46 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:46 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007046619799404513
14:18:46 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:46 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:46 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:46 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:46 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:46 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:46 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:46 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:46 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
14:18:46 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:46 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
14:18:46 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:46 INFO opendrift.models.chemicaldrift:1861: partitioning: [102, 0, 46, 352, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:46 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:46 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:46 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:46 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:46 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:46 INFO opendrift.models.basemodel:2011: 2024-05-05 11:17:58.364115 - step 43 of 96 - 500 active elements (0 deactivated)
14:18:46 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:46 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:46 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.718927376230944
14:18:46 DEBUG opendrift.models.basemodel:2035: 10.513524818110792 <- longitude -> 10.710978495102694
14:18:46 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.027880694915013993
14:18:46 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:46 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:46 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:46 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:46 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:46 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 11:00:00 (before)
2024-05-05 12:00:00 (after)
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:47 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:47 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 45x35x7) for time after (2024-05-05 12:00:00)
14:18:47 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 11:00:00) in space (linearNDFast)
14:18:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:47 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 12:00:00) in space (linearNDFast)
14:18:47 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:47 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 11:00:00, weight 0.70) and
after (2024-05-05 12:00:00, weight 0.30) in time
14:18:47 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28903253102423 degrees.
14:18:47 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28903253102423 degrees.
14:18:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:47 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:47 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:47 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:47 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:47 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:47 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:47 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:47 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:47 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:47 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:47 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:47 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:47 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:47 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.170523 (min) 0.0376529 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.212544 (min) 0.11243 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.12963 (min) -2.38273 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.089977 (min) 0.345399 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.0719 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.05434 (min) 10.5941 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3554 (min) 32.8612 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -4.85358e-05 (min) 0.00021027 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:47 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:47 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:47 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:47 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:47 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:47 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:47 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:47 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:47 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 3]
14:18:47 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0]
14:18:47 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 379. 0.]
[ 0. 0. 0. 0. 0.]
[ 21. 0. 0. 224. 0.]
[ 11. 0. 240. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:47 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:47 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:47 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:47 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:47 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007090048400491451
14:18:47 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:47 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:47 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:47 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:47 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:47 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
14:18:48 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:48 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 6 elements
14:18:48 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:48 INFO opendrift.models.chemicaldrift:1861: partitioning: [102, 0, 48, 350, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:48 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:48 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:48 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:48 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:48 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:48 INFO opendrift.models.basemodel:2011: 2024-05-05 11:47:58.364115 - step 44 of 96 - 500 active elements (0 deactivated)
14:18:48 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:48 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:48 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.7207444555535
14:18:48 DEBUG opendrift.models.basemodel:2035: 10.513524818110792 <- longitude -> 10.707539918237398
14:18:48 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2497499811799253
14:18:48 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 11:00:00 (before)
2024-05-05 12:00:00 (after)
14:18:48 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 11:00:00) in space (linearNDFast)
14:18:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:48 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 12:00:00) in space (linearNDFast)
14:18:48 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:48 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 11:00:00, weight 0.20) and
after (2024-05-05 12:00:00, weight 0.80) in time
14:18:48 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.29247111344225 degrees.
14:18:48 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.29247111344225 degrees.
14:18:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:48 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:48 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:48 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:48 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:48 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:48 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:48 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:48 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:48 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:48 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.214557 (min) 0.0893357 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.248279 (min) 0.243707 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.07397 (min) -2.24546 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.753459 (min) -0.104664 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.0898 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.06158 (min) 10.7086 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3614 (min) 33.2188 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -6.92091e-05 (min) 0.000181644 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:48 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:48 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:48 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:48 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:48 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:48 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:48 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:48 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:48 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 3]
14:18:48 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0]
14:18:48 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 381. 0.]
[ 0. 0. 0. 0. 0.]
[ 21. 0. 0. 228. 0.]
[ 12. 0. 246. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:48 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:48 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:48 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:48 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:48 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00696557108270967
14:18:48 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:48 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:48 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:48 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:48 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 12
14:18:48 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:48 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 12 elements
14:18:48 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:48 INFO opendrift.models.chemicaldrift:1861: partitioning: [101, 0, 54, 345, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:48 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:48 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:48 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:48 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:48 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:48 INFO opendrift.models.basemodel:2011: 2024-05-05 12:17:58.364115 - step 45 of 96 - 500 active elements (0 deactivated)
14:18:48 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:48 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:48 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.72468321192059
14:18:48 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.704729357140243
14:18:48 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.17931173501904035
14:18:48 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:48 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:48 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:48 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:48 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:48 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 12:00:00 (before)
2024-05-05 13:00:00 (after)
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:49 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:49 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 46x35x7) for time after (2024-05-05 13:00:00)
14:18:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 12:00:00) in space (linearNDFast)
14:18:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 13:00:00) in space (linearNDFast)
14:18:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 12:00:00, weight 0.70) and
after (2024-05-05 13:00:00, weight 0.30) in time
14:18:49 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.295281665526595 degrees.
14:18:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.295281665526595 degrees.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.208296 (min) 0.121202 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.261824 (min) 0.312479 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.94397 (min) -2.04709 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.34656 (min) -0.479817 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.1354 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.07048 (min) 10.7123 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4123 (min) 33.1656 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -8.89629e-05 (min) 0.000165542 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:49 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:49 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:49 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:49 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:49 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:49 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0]
14:18:49 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3]
14:18:49 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 383. 0.]
[ 0. 0. 0. 0. 0.]
[ 22. 0. 0. 234. 0.]
[ 12. 0. 258. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:49 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:49 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.007052184241997214
14:18:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 15
14:18:49 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 15 elements
14:18:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:49 INFO opendrift.models.chemicaldrift:1861: partitioning: [100, 0, 59, 341, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:49 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:49 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:49 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:49 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:49 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:49 INFO opendrift.models.basemodel:2011: 2024-05-05 12:47:58.364115 - step 46 of 96 - 500 active elements (0 deactivated)
14:18:49 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:49 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:49 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.729733425345415
14:18:49 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.7033132625325
14:18:49 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.16674923331387098
14:18:49 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 12:00:00 (before)
2024-05-05 13:00:00 (after)
14:18:49 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 12:00:00) in space (linearNDFast)
14:18:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:49 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 13:00:00) in space (linearNDFast)
14:18:49 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:49 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 12:00:00, weight 0.20) and
after (2024-05-05 13:00:00, weight 0.80) in time
14:18:49 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.296697762591066 degrees.
14:18:49 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.296697762591066 degrees.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:49 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:49 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:49 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:49 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:49 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:49 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.194618 (min) 0.119503 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.2636 (min) 0.401628 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.81359 (min) -1.79714 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.87234 (min) -0.805569 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 23.047 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.08048 (min) 10.7916 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4305 (min) 33.1352 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000103251 (min) 0.000167323 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:49 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:49 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:49 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:49 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:49 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:49 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:18:49 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 2 0]
14:18:49 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 3]
14:18:49 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 386. 0.]
[ 0. 0. 0. 0. 0.]
[ 23. 0. 0. 243. 0.]
[ 13. 0. 273. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:49 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:49 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:49 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.008005601987653254
14:18:49 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:49 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:49 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:49 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:49 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:49 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:49 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 21
14:18:49 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:49 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 21 elements
14:18:49 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:49 INFO opendrift.models.chemicaldrift:1861: partitioning: [99, 0, 70, 331, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:49 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:49 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:49 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:49 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:49 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:49 INFO opendrift.models.basemodel:2011: 2024-05-05 13:17:58.364115 - step 47 of 96 - 500 active elements (0 deactivated)
14:18:49 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:49 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:49 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.73622444703203
14:18:49 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.703611186342645
14:18:49 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2833833560336939
14:18:49 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:49 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:49 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:49 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:49 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:49 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 13:00:00 (before)
2024-05-05 14:00:00 (after)
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:52 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:52 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 47x36x7) for time after (2024-05-05 14:00:00)
14:18:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 13:00:00) in space (linearNDFast)
14:18:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:52 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 14:00:00) in space (linearNDFast)
14:18:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:52 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 13:00:00, weight 0.70) and
after (2024-05-05 14:00:00, weight 0.30) in time
14:18:52 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.296399836363584 degrees.
14:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.296399836363584 degrees.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.185182 (min) 0.0966822 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.250784 (min) 0.398772 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.72173 (min) -1.38648 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.36632 (min) -1.26615 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 22.8969 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.09436 (min) 10.8641 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.452 (min) 33.0985 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00011244 (min) 0.000160039 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:52 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:52 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:52 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:52 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:52 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:52 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:52 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
14:18:52 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0]
14:18:52 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3]
14:18:52 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 388. 0.]
[ 0. 0. 0. 0. 0.]
[ 23. 0. 0. 252. 0.]
[ 13. 0. 294. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:52 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:52 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:52 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.00832009339153086
14:18:52 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:52 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:52 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 18
14:18:52 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 18 elements
14:18:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:52 INFO opendrift.models.chemicaldrift:1861: partitioning: [97, 0, 79, 324, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:52 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:52 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:52 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:52 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:52 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:52 INFO opendrift.models.basemodel:2011: 2024-05-05 13:47:58.364115 - step 48 of 96 - 500 active elements (0 deactivated)
14:18:52 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:52 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:52 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.7426693357488
14:18:52 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.704333979197207
14:18:52 DEBUG opendrift.models.basemodel:2040: -22.760677337646484 <- z -> -0.2644191978735017
14:18:52 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 13:00:00 (before)
2024-05-05 14:00:00 (after)
14:18:52 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 13:00:00) in space (linearNDFast)
14:18:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:52 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 14:00:00) in space (linearNDFast)
14:18:52 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:52 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 13:00:00, weight 0.20) and
after (2024-05-05 14:00:00, weight 0.80) in time
14:18:52 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.29567705063634 degrees.
14:18:52 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.29567705063634 degrees.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:52 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:52 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:52 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:52 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:52 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:52 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.173972 (min) 0.1306 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.210699 (min) 0.627046 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.80898 (min) -0.798975 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.83903 (min) -1.78604 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 22.7607 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11085 (min) 10.8789 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4492 (min) 33.077 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000123523 (min) 0.000158149 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:52 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:52 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:52 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:52 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:52 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:52 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:52 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0]
14:18:52 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3]
14:18:52 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 392. 0.]
[ 0. 0. 0. 0. 0.]
[ 23. 0. 0. 261. 0.]
[ 13. 0. 312. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:52 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:52 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:52 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.008901842206139744
14:18:52 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:52 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:52 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:52 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:52 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 29
14:18:52 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:52 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 29 elements
14:18:52 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:52 INFO opendrift.models.chemicaldrift:1861: partitioning: [93, 0, 101, 306, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:52 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:52 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:52 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:52 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:52 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:52 INFO opendrift.models.basemodel:2011: 2024-05-05 14:17:58.364115 - step 49 of 96 - 500 active elements (0 deactivated)
14:18:52 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:52 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:52 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.752803581379844
14:18:52 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.707076279584264
14:18:52 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.2044431086026247
14:18:52 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:52 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:52 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:52 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:52 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:52 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 14:00:00 (before)
2024-05-05 15:00:00 (after)
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:54 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:54 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 49x36x7) for time after (2024-05-05 15:00:00)
14:18:54 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 14:00:00) in space (linearNDFast)
14:18:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:54 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 15:00:00) in space (linearNDFast)
14:18:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:54 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 14:00:00, weight 0.70) and
after (2024-05-05 15:00:00, weight 0.30) in time
14:18:54 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.29293474666053 degrees.
14:18:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.29293474666053 degrees.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.19257 (min) 0.162908 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.183336 (min) 0.730908 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.65343 (min) -0.421816 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.42104 (min) -2.52018 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 27.51 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.127 (min) 10.9108 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4504 (min) 33.0569 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000122636 (min) 0.000165801 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:54 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:54 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:54 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:54 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:54 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:54 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:18:54 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0 0]
14:18:54 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3 3]
14:18:54 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 398. 0.]
[ 0. 0. 0. 0. 0.]
[ 23. 0. 0. 268. 0.]
[ 13. 0. 341. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:54 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:54 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:54 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.009890971413726676
14:18:54 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:54 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:54 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:54 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 6
14:18:54 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 6 elements
14:18:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:54 INFO opendrift.models.chemicaldrift:1861: partitioning: [87, 0, 99, 314, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:54 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:54 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:54 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:54 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:54 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:54 INFO opendrift.models.basemodel:2011: 2024-05-05 14:47:58.364115 - step 50 of 96 - 500 active elements (0 deactivated)
14:18:54 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:54 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:54 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.764616396327575
14:18:54 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.711086633621692
14:18:54 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.34248086585378973
14:18:54 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 14:00:00 (before)
2024-05-05 15:00:00 (after)
14:18:54 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 14:00:00) in space (linearNDFast)
14:18:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:54 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 15:00:00) in space (linearNDFast)
14:18:54 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:54 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 14:00:00, weight 0.20) and
after (2024-05-05 15:00:00, weight 0.80) in time
14:18:54 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.288924390590445 degrees.
14:18:54 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.288924390590445 degrees.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:54 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:54 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:54 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:54 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:54 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:54 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.201086 (min) 0.3367 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.155942 (min) 0.409013 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: x_wind: -2.33348 (min) -0.213295 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.11391 (min) -3.14909 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 36.9605 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.13331 (min) 10.8965 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4248 (min) 34.2432 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000439669 (min) 0.000153208 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:54 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:54 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:54 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:54 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:54 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:54 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:54 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2]
14:18:54 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0]
14:18:54 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 401. 0.]
[ 0. 0. 0. 0. 0.]
[ 24. 0. 0. 276. 0.]
[ 13. 0. 347. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:54 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:54 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:54 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01321201380728131
14:18:54 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:54 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:54 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:54 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:54 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:54 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:18:54 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:54 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:54 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:54 INFO opendrift.models.chemicaldrift:1861: partitioning: [85, 0, 92, 323, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:54 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:54 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:54 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:54 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:54 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:54 INFO opendrift.models.basemodel:2011: 2024-05-05 15:17:58.364115 - step 51 of 96 - 500 active elements (0 deactivated)
14:18:54 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:54 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:54 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.7637247610036
14:18:54 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.715697528277094
14:18:54 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.2373058502832368
14:18:54 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:54 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:54 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:54 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:54 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:54 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 15:00:00 (before)
2024-05-05 16:00:00 (after)
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:55 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:55 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 51x36x7) for time after (2024-05-05 16:00:00)
14:18:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 15:00:00) in space (linearNDFast)
14:18:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 16:00:00) in space (linearNDFast)
14:18:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 15:00:00, weight 0.70) and
after (2024-05-05 16:00:00, weight 0.30) in time
14:18:55 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28431349909411 degrees.
14:18:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.28431349909411 degrees.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.216589 (min) 0.258715 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.137549 (min) 0.473249 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.97754 (min) -0.134357 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.87913 (min) -3.80305 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 36.8664 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12728 (min) 10.8925 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4437 (min) 33.4233 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000118653 (min) 0.000143054 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:55 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:55 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:55 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:55 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:55 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:55 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:55 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:18:55 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 3 0 0 0 0]
14:18:55 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3 3]
14:18:55 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 406. 0.]
[ 0. 0. 0. 0. 0.]
[ 24. 0. 0. 282. 0.]
[ 14. 0. 347. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:55 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:55 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017881627481949738
14:18:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:18:55 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:18:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:55 INFO opendrift.models.chemicaldrift:1861: partitioning: [81, 0, 83, 336, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:55 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:55 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:55 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:55 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:55 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:55 INFO opendrift.models.basemodel:2011: 2024-05-05 15:47:58.364115 - step 52 of 96 - 500 active elements (0 deactivated)
14:18:55 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:55 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:55 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.76683416777739
14:18:55 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.720691990925678
14:18:55 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.2641873249118659
14:18:55 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:55 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 15:00:00 (before)
2024-05-05 16:00:00 (after)
14:18:55 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 15:00:00) in space (linearNDFast)
14:18:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:55 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 16:00:00) in space (linearNDFast)
14:18:55 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:55 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 15:00:00, weight 0.20) and
after (2024-05-05 16:00:00, weight 0.80) in time
14:18:55 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27931901520293 degrees.
14:18:55 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27931901520293 degrees.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:55 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:55 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:55 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:55 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:55 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:55 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:55 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:55 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:55 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:55 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:55 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.213957 (min) 0.243258 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.114522 (min) 0.481999 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.76607 (min) -0.0942624 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: y_wind: -5.69126 (min) -4.34122 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 39.7226 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12392 (min) 10.9344 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4467 (min) 33.1701 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00010701 (min) 0.000139559 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:55 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:55 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:55 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:55 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:55 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:55 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:55 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0]
14:18:55 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3]
14:18:55 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 408. 0.]
[ 0. 0. 0. 0. 0.]
[ 25. 0. 0. 293. 0.]
[ 14. 0. 349. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:55 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:55 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:55 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:55 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:55 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.023615466729270913
14:18:55 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:55 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:55 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:55 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:55 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:55 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:56 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:56 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:56 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:56 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:56 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:56 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
14:18:56 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:56 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
14:18:56 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:56 INFO opendrift.models.chemicaldrift:1861: partitioning: [80, 0, 80, 340, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:56 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:56 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:56 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:56 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:56 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:56 INFO opendrift.models.basemodel:2011: 2024-05-05 16:17:58.364115 - step 53 of 96 - 500 active elements (0 deactivated)
14:18:56 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:56 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:56 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.7691812909595
14:18:56 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.7229393265596
14:18:56 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.4310655721312741
14:18:56 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:56 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:56 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:56 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:56 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:56 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:56 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:56 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:56 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 16:00:00 (before)
2024-05-05 17:00:00 (after)
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:57 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:57 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 52x36x7) for time after (2024-05-05 17:00:00)
14:18:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 16:00:00) in space (linearNDFast)
14:18:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 17:00:00) in space (linearNDFast)
14:18:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 16:00:00, weight 0.70) and
after (2024-05-05 17:00:00, weight 0.30) in time
14:18:57 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.277071683489226 degrees.
14:18:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.277071683489226 degrees.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.19209 (min) 0.294075 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0920083 (min) 0.360195 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.05314 (min) -0.199507 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.33196 (min) -4.70724 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 42.2433 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12586 (min) 10.9514 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4751 (min) 34.0146 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00029254 (min) 0.00013073 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:57 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:57 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:57 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:57 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:57 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:18:57 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 0]
14:18:57 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 3]
14:18:57 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 412. 0.]
[ 0. 0. 0. 0. 0.]
[ 26. 0. 0. 296. 0.]
[ 14. 0. 350. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:57 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:57 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02907686938446118
14:18:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:57 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:18:57 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:18:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:57 INFO opendrift.models.chemicaldrift:1861: partitioning: [77, 0, 78, 345, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:57 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:57 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:57 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:57 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:18:57 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:57 INFO opendrift.models.basemodel:2011: 2024-05-05 16:47:58.364115 - step 54 of 96 - 500 active elements (0 deactivated)
14:18:57 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:57 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:57 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.77220851509235
14:18:57 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.722609262331593
14:18:57 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.3820099859316679
14:18:57 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 16:00:00 (before)
2024-05-05 17:00:00 (after)
14:18:57 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 16:00:00) in space (linearNDFast)
14:18:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:57 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 17:00:00) in space (linearNDFast)
14:18:57 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:57 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 16:00:00, weight 0.20) and
after (2024-05-05 17:00:00, weight 0.80) in time
14:18:57 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27740174866932 degrees.
14:18:57 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27740174866932 degrees.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:57 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:57 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:57 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:57 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:57 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:57 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.211703 (min) 0.319902 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0661775 (min) 0.378364 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.428271 (min) 0.0937859 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.09147 (min) -4.97832 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.1779 (min) 45.3733 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12782 (min) 10.9517 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4643 (min) 34.0944 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000740219 (min) 0.000139008 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:57 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:57 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:57 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:57 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:57 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:57 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:57 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2]
14:18:57 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0]
14:18:57 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 413. 0.]
[ 0. 0. 0. 0. 0.]
[ 29. 0. 0. 299. 0.]
[ 14. 0. 352. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:57 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:57 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:57 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03647538120547057
14:18:57 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:57 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:57 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:57 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:57 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 10
14:18:57 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:57 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 10 elements
14:18:57 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:57 INFO opendrift.models.chemicaldrift:1861: partitioning: [79, 0, 82, 339, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:57 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:57 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:57 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:57 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:57 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:57 INFO opendrift.models.basemodel:2011: 2024-05-05 17:17:58.364115 - step 55 of 96 - 500 active elements (0 deactivated)
14:18:57 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:57 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:57 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.773253711231916
14:18:57 DEBUG opendrift.models.basemodel:2035: 10.513524818110787 <- longitude -> 10.721667788357266
14:18:57 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.3476170534462299
14:18:57 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:57 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:57 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:57 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:57 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:57 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 17:00:00 (before)
2024-05-05 18:00:00 (after)
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:18:59 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:18:59 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 53x36x7) for time after (2024-05-05 18:00:00)
14:18:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 17:00:00) in space (linearNDFast)
14:18:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
/root/project/opendrift/readers/interpolation/interpolators.py:17: RuntimeWarning: overflow encountered in cast
data[mask] = np.finfo(np.float64).min
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 18:00:00) in space (linearNDFast)
14:18:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:18:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 17:00:00, weight 0.70) and
after (2024-05-05 18:00:00, weight 0.30) in time
14:18:59 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27834323079637 degrees.
14:18:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27834323079637 degrees.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.193259 (min) 0.296427 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0421924 (min) 0.488432 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.577891 (min) 0.725095 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.6696 (min) -4.97822 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.04203 (min) 46.8086 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12086 (min) 10.9396 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4989 (min) 33.8266 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000407394 (min) 0.000145696 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:59 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:59 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:59 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:59 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:59 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:59 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:18:59 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:18:59 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:18:59 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 416. 0.]
[ 0. 0. 0. 0. 0.]
[ 29. 0. 0. 302. 0.]
[ 14. 0. 362. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:59 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:59 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04273299738725568
14:18:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 14
14:18:59 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 14 elements
14:18:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:59 INFO opendrift.models.chemicaldrift:1861: partitioning: [76, 0, 91, 333, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:59 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:59 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:59 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:59 INFO opendrift.models.basemodel:2011: 2024-05-05 17:47:58.364115 - step 56 of 96 - 500 active elements (0 deactivated)
14:18:59 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:59 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:59 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.781147653581336
14:18:59 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.72072257255977
14:18:59 DEBUG opendrift.models.basemodel:2040: -22.703298568725586 <- z -> -0.02618254634578021
14:18:59 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 17:00:00 (before)
2024-05-05 18:00:00 (after)
14:18:59 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 17:00:00) in space (linearNDFast)
14:18:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:59 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 18:00:00) in space (linearNDFast)
14:18:59 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:18:59 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 17:00:00, weight 0.20) and
after (2024-05-05 18:00:00, weight 0.80) in time
14:18:59 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:18:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.27928844362308 degrees.
14:18:59 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486279131455 and -59.27928844362308 degrees.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:18:59 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:18:59 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:18:59 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:18:59 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:18:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:18:59 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:18:59 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.147056 (min) 0.469855 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0194422 (min) 0.470897 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.677829 (min) 1.18612 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.08815 (min) -4.87275 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.93357 (min) 54.4622 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12086 (min) 10.9239 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.641 (min) 33.8653 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000369622 (min) 0.000159523 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:18:59 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:18:59 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:18:59 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:18:59 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:18:59 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:18:59 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:18:59 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:18:59 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0]
14:18:59 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3]
14:18:59 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 419. 0.]
[ 0. 0. 0. 0. 0.]
[ 30. 0. 0. 307. 0.]
[ 14. 0. 376. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:18:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:18:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:59 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:18:59 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:18:59 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04756709773254092
14:18:59 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:18:59 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:18:59 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:18:59 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 21
14:18:59 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:18:59 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 21 elements
14:18:59 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:18:59 INFO opendrift.models.chemicaldrift:1861: partitioning: [74, 0, 102, 324, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:18:59 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:18:59 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:18:59 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:18:59 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:18:59 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:18:59 INFO opendrift.models.basemodel:2011: 2024-05-05 18:17:58.364115 - step 57 of 96 - 500 active elements (0 deactivated)
14:18:59 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:18:59 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:18:59 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.78875817834316
14:18:59 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.721242143739213
14:18:59 DEBUG opendrift.models.basemodel:2040: -28.263647079467773 <- z -> -0.08314527345742528
14:18:59 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:18:59 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:18:59 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:18:59 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:18:59 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:18:59 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 18:00:00 (before)
2024-05-05 19:00:00 (after)
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:01 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:01 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 55x36x7) for time after (2024-05-05 19:00:00)
14:19:01 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 18:00:00) in space (linearNDFast)
14:19:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:01 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 19:00:00) in space (linearNDFast)
14:19:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:01 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 18:00:00, weight 0.70) and
after (2024-05-05 19:00:00, weight 0.30) in time
14:19:01 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27876886057532 degrees.
14:19:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27876886057532 degrees.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.377852 (min) 0.376939 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.000405846 (min) 0.491507 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.631304 (min) 1.68423 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.32377 (min) -5.02087 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.86705 (min) 62.0636 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11835 (min) 10.905 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6173 (min) 34.3434 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000913038 (min) 0.000163515 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:01 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:01 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:01 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:01 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:01 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:01 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:01 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 2]
14:19:01 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0]
14:19:01 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 420. 0.]
[ 0. 0. 0. 0. 0.]
[ 31. 0. 0. 316. 0.]
[ 15. 0. 397. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:01 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:01 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:01 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:01 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:01 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05035351144737319
14:19:01 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:01 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:01 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:01 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 14
14:19:01 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:01 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 14 elements
14:19:01 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:01 INFO opendrift.models.chemicaldrift:1861: partitioning: [75, 0, 107, 318, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:01 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:01 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:01 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:01 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:01 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:01 INFO opendrift.models.basemodel:2011: 2024-05-05 18:47:58.364115 - step 58 of 96 - 500 active elements (0 deactivated)
14:19:01 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:01 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:01 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.79562265383564
14:19:01 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.722679571861383
14:19:01 DEBUG opendrift.models.basemodel:2040: -28.164115550351628 <- z -> -0.3352198556162122
14:19:01 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 18:00:00 (before)
2024-05-05 19:00:00 (after)
14:19:01 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 18:00:00) in space (linearNDFast)
14:19:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 19:00:00) in space (linearNDFast)
14:19:01 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:01 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 18:00:00, weight 0.20) and
after (2024-05-05 19:00:00, weight 0.80) in time
14:19:01 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27733144722632 degrees.
14:19:01 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27733144722632 degrees.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:01 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:01 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:01 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:01 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:01 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:01 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.121094 (min) 0.362716 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0161453 (min) 0.508565 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.579875 (min) 2.20633 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.49098 (min) -5.33603 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.8424 (min) 69.073 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11686 (min) 10.8798 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6308 (min) 34.3165 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00102275 (min) 0.000161731 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:01 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:01 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:01 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:01 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:01 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:01 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
14:19:01 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0]
14:19:01 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3]
14:19:01 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 422. 0.]
[ 0. 0. 0. 0. 0.]
[ 31. 0. 0. 324. 0.]
[ 15. 0. 411. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:01 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:01 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:01 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:01 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:01 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05234548392536019
14:19:01 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:01 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:01 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:01 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:01 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:01 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:01 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 32
14:19:01 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:01 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 32 elements
14:19:01 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:01 INFO opendrift.models.chemicaldrift:1861: partitioning: [73, 0, 131, 296, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:01 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:01 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:01 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:01 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:01 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:01 INFO opendrift.models.basemodel:2011: 2024-05-05 19:17:58.364115 - step 59 of 96 - 500 active elements (0 deactivated)
14:19:01 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:01 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:01 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.80215784315733
14:19:01 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.724735010450793
14:19:01 DEBUG opendrift.models.basemodel:2040: -25.117237771666005 <- z -> -0.05166883261648614
14:19:01 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:01 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:01 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:01 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:01 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:01 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 19:00:00 (before)
2024-05-05 20:00:00 (after)
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:03 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:03 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 56x36x7) for time after (2024-05-05 20:00:00)
14:19:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 19:00:00) in space (linearNDFast)
14:19:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 20:00:00) in space (linearNDFast)
14:19:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 19:00:00, weight 0.70) and
after (2024-05-05 20:00:00, weight 0.30) in time
14:19:03 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27527600794986 degrees.
14:19:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27527600794986 degrees.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.121411 (min) 0.364477 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.029813 (min) 0.489226 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.75809 (min) 2.42363 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.53619 (min) -5.44807 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.87459 (min) 75.22 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11581 (min) 10.8532 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.715 (min) 34.2854 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000852174 (min) 0.000153718 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:03 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:03 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:03 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:03 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:03 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:03 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:03 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:03 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0]
14:19:03 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3]
14:19:03 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 426. 0.]
[ 0. 0. 0. 0. 0.]
[ 31. 0. 0. 332. 0.]
[ 15. 0. 443. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:03 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:03 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:03 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:03 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.053074060943942666
14:19:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:03 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:03 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 31
14:19:03 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:03 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 31 elements
14:19:03 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:03 INFO opendrift.models.chemicaldrift:1861: partitioning: [69, 0, 151, 280, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:03 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:03 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:03 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:03 INFO opendrift.models.basemodel:2011: 2024-05-05 19:47:58.364115 - step 60 of 96 - 500 active elements (0 deactivated)
14:19:03 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:03 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:03 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.810064573199256
14:19:03 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.727149160163805
14:19:03 DEBUG opendrift.models.basemodel:2040: -31.500319529999413 <- z -> 0.0
14:19:03 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 19:00:00 (before)
2024-05-05 20:00:00 (after)
14:19:03 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 19:00:00) in space (linearNDFast)
14:19:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:03 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 20:00:00) in space (linearNDFast)
14:19:03 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:03 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 19:00:00, weight 0.20) and
after (2024-05-05 20:00:00, weight 0.80) in time
14:19:03 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286184809667 degrees.
14:19:03 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286184809667 degrees.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:03 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:03 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:03 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:03 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:03 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:03 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.15245 (min) 0.307902 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.0212574 (min) 0.536519 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.07912 (min) 2.43695 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.49843 (min) -5.07468 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.95316 (min) 82.259 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11131 (min) 10.8258 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7019 (min) 34.2513 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000724306 (min) 0.000345351 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:03 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:03 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:03 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:03 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:03 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:03 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:03 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:03 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 3]
14:19:03 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 0]
14:19:03 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 429. 0.]
[ 0. 0. 0. 0. 0.]
[ 32. 0. 0. 343. 0.]
[ 16. 0. 474. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:03 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:03 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:03 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:03 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:03 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.052641812889762406
14:19:03 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:03 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:03 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:03 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:03 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:03 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:03 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:03 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 21
14:19:03 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:03 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 21 elements
14:19:03 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:03 INFO opendrift.models.chemicaldrift:1861: partitioning: [68, 0, 161, 271, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:03 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:03 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:03 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:03 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:03 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:03 INFO opendrift.models.basemodel:2011: 2024-05-05 20:17:58.364115 - step 61 of 96 - 500 active elements (0 deactivated)
14:19:03 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:03 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:03 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.81604557458695
14:19:03 DEBUG opendrift.models.basemodel:2035: 10.513524818110785 <- longitude -> 10.7271491601638
14:19:03 DEBUG opendrift.models.basemodel:2040: -34.985107421875 <- z -> 0.0
14:19:03 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:03 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:03 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:03 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:03 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:03 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 20:00:00 (before)
2024-05-05 21:00:00 (after)
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:05 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:05 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x36x7) for time after (2024-05-05 21:00:00)
14:19:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 20:00:00) in space (linearNDFast)
14:19:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:05 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 21:00:00) in space (linearNDFast)
14:19:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:05 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 20:00:00, weight 0.70) and
after (2024-05-05 21:00:00, weight 0.30) in time
14:19:05 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286184809667 degrees.
14:19:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286184809667 degrees.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.562862 (min) 0.307592 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00963217 (min) 0.369793 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.715104 (min) 2.50555 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -8.12688 (min) -4.94005 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.08121 (min) 87.0909 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11076 (min) 10.8192 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.4478 (min) 34.2135 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000754521 (min) 0.000163184 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:05 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:05 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:05 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:05 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:05 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:05 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:05 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 0]
14:19:05 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 3]
14:19:05 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 432. 0.]
[ 0. 0. 0. 0. 0.]
[ 34. 0. 0. 353. 0.]
[ 16. 0. 495. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:05 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:05 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:05 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:05 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:05 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04801161412711909
14:19:05 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:05 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:05 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:05 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
14:19:05 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:05 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
14:19:05 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:05 INFO opendrift.models.chemicaldrift:1861: partitioning: [67, 0, 159, 274, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:05 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:05 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:05 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:05 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:05 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:05 INFO opendrift.models.basemodel:2011: 2024-05-05 20:47:58.364115 - step 62 of 96 - 500 active elements (0 deactivated)
14:19:05 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:05 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:05 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.821046425760606
14:19:05 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.7271491601638
14:19:05 DEBUG opendrift.models.basemodel:2040: -34.985107421875 <- z -> -0.07469332312743737
14:19:05 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 20:00:00 (before)
2024-05-05 21:00:00 (after)
14:19:05 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 20:00:00) in space (linearNDFast)
14:19:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:05 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 21:00:00) in space (linearNDFast)
14:19:05 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:05 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 20:00:00, weight 0.20) and
after (2024-05-05 21:00:00, weight 0.80) in time
14:19:05 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286185338023 degrees.
14:19:05 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.27286185338023 degrees.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:05 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:05 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:05 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:05 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:05 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:05 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.318614 (min) 0.314129 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0568392 (min) 0.445883 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.0265479 (min) 2.64751 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.77654 (min) -5.11128 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.22029 (min) 91.5322 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11046 (min) 10.7993 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7678 (min) 34.1754 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000539014 (min) 0.000364462 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:05 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:05 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:05 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:05 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:05 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:05 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:05 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:05 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
14:19:05 DEBUG opendrift.models.chemicaldrift:1452: old species: [0]
14:19:05 DEBUG opendrift.models.chemicaldrift:1453: new species: [3]
14:19:05 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 433. 0.]
[ 0. 0. 0. 0. 0.]
[ 34. 0. 0. 360. 0.]
[ 16. 0. 502. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:05 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:05 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:05 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:05 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:05 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.044340005230616104
14:19:05 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:05 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:05 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:05 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:05 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:05 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:05 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:05 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
14:19:05 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:05 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
14:19:05 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:05 INFO opendrift.models.chemicaldrift:1861: partitioning: [66, 0, 159, 275, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:05 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:05 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:05 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:05 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:05 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:05 INFO opendrift.models.basemodel:2011: 2024-05-05 21:17:58.364115 - step 63 of 96 - 500 active elements (0 deactivated)
14:19:05 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:05 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:05 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.82606437220924
14:19:05 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.73321676124339
14:19:05 DEBUG opendrift.models.basemodel:2040: -34.985107421875 <- z -> -0.0749326533004612
14:19:05 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:05 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:05 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:05 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:05 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:05 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 21:00:00 (before)
2024-05-05 22:00:00 (after)
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:07 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:07 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x38x7) for time after (2024-05-05 22:00:00)
14:19:07 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 21:00:00) in space (linearNDFast)
14:19:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:07 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 22:00:00) in space (linearNDFast)
14:19:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 21:00:00, weight 0.70) and
after (2024-05-05 22:00:00, weight 0.30) in time
14:19:07 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.26679426107294 degrees.
14:19:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.26679426107294 degrees.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.122062 (min) 0.273626 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.108426 (min) 0.338839 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.497914 (min) 2.47326 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.58353 (min) -5.39792 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.33907 (min) 95.5371 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10756 (min) 10.771 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6206 (min) 34.1596 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000300983 (min) 0.000235594 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:07 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:07 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:07 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:07 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:07 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:07 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:07 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:07 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 2 0]
14:19:07 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 3]
14:19:07 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 436. 0.]
[ 0. 0. 0. 0. 0.]
[ 35. 0. 0. 367. 0.]
[ 17. 0. 509. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:07 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:07 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:07 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:07 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:07 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04231518537448175
14:19:07 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:07 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:07 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:07 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:07 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
14:19:07 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:07 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
14:19:07 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:07 INFO opendrift.models.chemicaldrift:1861: partitioning: [65, 0, 150, 285, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:07 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:07 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:07 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:07 INFO opendrift.models.basemodel:2011: 2024-05-05 21:47:58.364115 - step 64 of 96 - 500 active elements (0 deactivated)
14:19:07 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:07 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:07 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.826761785636116
14:19:07 DEBUG opendrift.models.basemodel:2035: 10.51352481811079 <- longitude -> 10.737558491643169
14:19:07 DEBUG opendrift.models.basemodel:2040: -34.985107421875 <- z -> 0.0
14:19:07 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 21:00:00 (before)
2024-05-05 22:00:00 (after)
14:19:07 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 21:00:00) in space (linearNDFast)
14:19:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 22:00:00) in space (linearNDFast)
14:19:07 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:07 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 21:00:00, weight 0.20) and
after (2024-05-05 22:00:00, weight 0.80) in time
14:19:07 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.2624525202056 degrees.
14:19:07 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.2624525202056 degrees.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:07 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:07 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:07 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:07 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:07 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:07 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.149911 (min) 0.254309 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.127627 (min) 0.350644 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.614253 (min) 2.06748 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.29596 (min) -5.73185 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.43512 (min) 95.9207 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10675 (min) 10.7704 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7299 (min) 34.1588 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00010063 (min) 0.000238228 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:07 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:07 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:07 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:07 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:07 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:07 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:07 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:07 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:07 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0]
14:19:07 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3]
14:19:07 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 440. 0.]
[ 0. 0. 0. 0. 0.]
[ 36. 0. 0. 379. 0.]
[ 17. 0. 513. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:07 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:07 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:07 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:07 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:07 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.039176523776236524
14:19:07 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:07 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:07 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:07 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:07 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:07 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:07 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:07 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
14:19:07 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:07 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
14:19:07 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:07 INFO opendrift.models.chemicaldrift:1861: partitioning: [62, 0, 144, 294, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:07 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:07 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:07 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:07 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:07 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:07 INFO opendrift.models.basemodel:2011: 2024-05-05 22:17:58.364115 - step 65 of 96 - 500 active elements (0 deactivated)
14:19:07 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:07 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:07 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.827286704568
14:19:07 DEBUG opendrift.models.basemodel:2035: 10.513524818110794 <- longitude -> 10.741633895204805
14:19:07 DEBUG opendrift.models.basemodel:2040: -35.73826667208389 <- z -> 0.0
14:19:07 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:07 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:07 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:07 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:07 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:07 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 22:00:00 (before)
2024-05-05 23:00:00 (after)
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:09 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:09 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x38x7) for time after (2024-05-05 23:00:00)
14:19:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 22:00:00) in space (linearNDFast)
14:19:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 23:00:00) in space (linearNDFast)
14:19:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 22:00:00, weight 0.70) and
after (2024-05-05 23:00:00, weight 0.30) in time
14:19:09 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.258377117452625 degrees.
14:19:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.258377117452625 degrees.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.177172 (min) 0.248328 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.141249 (min) 0.469717 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: 0.234618 (min) 1.90535 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.01082 (min) -5.49368 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.48605 (min) 96.2332 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10562 (min) 10.7401 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.8403 (min) 34.1812 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -9.91368e-05 (min) 0.000260484 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:09 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:09 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:09 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:09 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:09 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:09 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:09 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 10
14:19:09 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 2 0 0 2 0 2]
14:19:09 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 0 3 3 0 3 0]
14:19:09 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 446. 0.]
[ 0. 0. 0. 0. 0.]
[ 40. 0. 0. 388. 0.]
[ 17. 0. 517. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:09 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:09 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:09 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:09 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:09 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03688812701149027
14:19:09 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:09 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:09 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:09 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:09 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 1
14:19:09 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:09 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 1 elements
14:19:09 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:09 INFO opendrift.models.chemicaldrift:1861: partitioning: [60, 0, 131, 309, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:09 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:09 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:09 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:09 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:09 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:09 INFO opendrift.models.basemodel:2011: 2024-05-05 22:47:58.364115 - step 66 of 96 - 500 active elements (0 deactivated)
14:19:09 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:09 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:09 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.827465603807525
14:19:09 DEBUG opendrift.models.basemodel:2035: 10.513524818110794 <- longitude -> 10.746262011357889
14:19:09 DEBUG opendrift.models.basemodel:2040: -35.05342960333687 <- z -> 0.0
14:19:09 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 22:00:00 (before)
2024-05-05 23:00:00 (after)
14:19:09 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 22:00:00) in space (linearNDFast)
14:19:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:09 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-05 23:00:00) in space (linearNDFast)
14:19:09 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:09 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 22:00:00, weight 0.20) and
after (2024-05-05 23:00:00, weight 0.80) in time
14:19:09 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.253748985259726 degrees.
14:19:09 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.486486261465195 and -59.253748985259726 degrees.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:09 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:09 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:09 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:09 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:09 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:09 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.207356 (min) 0.414654 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.227247 (min) 0.537557 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: x_wind: -0.464811 (min) 2.2168 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: y_wind: -7.30994 (min) -4.65593 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.47493 (min) 96.2781 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10357 (min) 10.7211 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3854 (min) 34.1965 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000144722 (min) 0.000387811 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:09 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:09 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:09 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:09 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:09 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:09 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:09 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:09 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0]
14:19:09 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3]
14:19:09 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 450. 0.]
[ 0. 0. 0. 0. 0.]
[ 40. 0. 0. 398. 0.]
[ 17. 0. 518. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:09 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:09 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:09 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:09 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:09 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03895173247557032
14:19:09 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:09 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:09 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:09 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:09 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:09 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:09 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:19:09 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:09 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:09 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:09 INFO opendrift.models.chemicaldrift:1861: partitioning: [56, 0, 123, 321, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:09 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:09 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:09 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:09 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:09 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:09 INFO opendrift.models.basemodel:2011: 2024-05-05 23:17:58.364115 - step 67 of 96 - 500 active elements (0 deactivated)
14:19:09 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:09 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:09 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.82803887597268
14:19:09 DEBUG opendrift.models.basemodel:2035: 10.512670909638377 <- longitude -> 10.751241740796068
14:19:09 DEBUG opendrift.models.basemodel:2040: -36.40712188525831 <- z -> -0.1911761443130664
14:19:09 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:09 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:09 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:09 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:09 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:09 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 23:00:00 (before)
2024-05-06 00:00:00 (after)
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:11 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:11 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 59x38x7) for time after (2024-05-06 00:00:00)
14:19:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 23:00:00) in space (linearNDFast)
14:19:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 00:00:00) in space (linearNDFast)
14:19:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 23:00:00, weight 0.70) and
after (2024-05-06 00:00:00, weight 0.30) in time
14:19:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.487340179088925 and -59.24876926829072 degrees.
14:19:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.487340179088925 and -59.24876926829072 degrees.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.209168 (min) 0.430266 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.25386 (min) 0.540336 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -1.75478 (min) 2.9563 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -6.12611 (min) -3.74568 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.41962 (min) 96.731 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10428 (min) 10.7058 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2489 (min) 34.2734 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000146176 (min) 0.000406206 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:11 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:11 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 3 0]
14:19:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 3]
14:19:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 452. 0.]
[ 0. 0. 0. 0. 0.]
[ 41. 0. 0. 406. 0.]
[ 18. 0. 518. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02751244239104006
14:19:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
14:19:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
14:19:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [56, 0, 119, 325, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:11 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:11 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:11 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:11 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:11 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:11 INFO opendrift.models.basemodel:2011: 2024-05-05 23:47:58.364115 - step 68 of 96 - 500 active elements (0 deactivated)
14:19:11 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:11 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:11 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.82776375627033
14:19:11 DEBUG opendrift.models.basemodel:2035: 10.510695874412557 <- longitude -> 10.756144503263771
14:19:11 DEBUG opendrift.models.basemodel:2040: -36.28772969074921 <- z -> -0.3409632628830824
14:19:11 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-05 23:00:00 (before)
2024-05-06 00:00:00 (after)
14:19:11 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-05 23:00:00) in space (linearNDFast)
14:19:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:11 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 00:00:00) in space (linearNDFast)
14:19:11 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:11 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-05 23:00:00, weight 0.20) and
after (2024-05-06 00:00:00, weight 0.80) in time
14:19:11 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48931521270728 and -59.24386651813793 degrees.
14:19:11 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48931521270728 and -59.24386651813793 degrees.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:11 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:11 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:11 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:11 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:11 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:11 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.253174 (min) 0.179192 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.242002 (min) 0.51504 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.44595 (min) 3.9056 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.94624 (min) -2.51224 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.33505 (min) 96.5335 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.10828 (min) 10.6968 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.5666 (min) 34.2249 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000169761 (min) 0.000426561 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:11 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:11 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 6 elements to seafloor.
14:19:11 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:11 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:11 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:11 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:11 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:19:11 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 2 0]
14:19:11 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 0 3]
14:19:11 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 456. 0.]
[ 0. 0. 0. 0. 0.]
[ 43. 0. 0. 412. 0.]
[ 18. 0. 521. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:11 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:11 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:11 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.022255663297084914
14:19:11 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:11 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:11 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:11 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:11 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:11 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:19:11 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:11 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:19:11 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:11 INFO opendrift.models.chemicaldrift:1861: partitioning: [54, 0, 112, 334, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:11 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:11 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:11 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:11 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:11 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:11 INFO opendrift.models.basemodel:2011: 2024-05-06 00:17:58.364115 - step 69 of 96 - 500 active elements (0 deactivated)
14:19:11 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:11 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:11 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.8273459344248
14:19:11 DEBUG opendrift.models.basemodel:2035: 10.508318596336952 <- longitude -> 10.76088949418033
14:19:11 DEBUG opendrift.models.basemodel:2040: -35.237250475474944 <- z -> -0.16366017057546017
14:19:11 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:11 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:11 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:11 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:11 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:11 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 00:00:00 (before)
2024-05-06 01:00:00 (after)
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:13 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:13 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x38x7) for time after (2024-05-06 01:00:00)
14:19:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 00:00:00) in space (linearNDFast)
14:19:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:13 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 01:00:00) in space (linearNDFast)
14:19:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:13 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 00:00:00, weight 0.70) and
after (2024-05-06 01:00:00, weight 0.30) in time
14:19:13 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.491692491159355 and -59.23912151873255 degrees.
14:19:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.491692491159355 and -59.23912151873255 degrees.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.320329 (min) 0.273818 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.239652 (min) 0.461225 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.9531 (min) 4.18331 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.54231 (min) -1.28295 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.21317 (min) 96.2987 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11167 (min) 10.707 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.9317 (min) 34.2348 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000167303 (min) 0.000384464 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:13 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:13 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:13 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:13 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:13 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:13 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0]
14:19:13 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3]
14:19:13 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 458. 0.]
[ 0. 0. 0. 0. 0.]
[ 43. 0. 0. 419. 0.]
[ 19. 0. 523. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:13 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:13 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:13 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017320351791360253
14:19:13 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:13 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:13 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 0
14:19:13 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:13 INFO opendrift.models.chemicaldrift:1861: partitioning: [53, 0, 108, 339, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:13 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:13 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:13 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:13 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:13 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:13 INFO opendrift.models.basemodel:2011: 2024-05-06 00:47:58.364115 - step 70 of 96 - 500 active elements (0 deactivated)
14:19:13 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:13 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:13 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.827100300013655
14:19:13 DEBUG opendrift.models.basemodel:2035: 10.505634380049946 <- longitude -> 10.765046130983533
14:19:13 DEBUG opendrift.models.basemodel:2040: -35.186635243246435 <- z -> -0.2732906935094938
14:19:13 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 00:00:00 (before)
2024-05-06 01:00:00 (after)
14:19:13 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 00:00:00) in space (linearNDFast)
14:19:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:13 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 01:00:00) in space (linearNDFast)
14:19:13 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:13 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 00:00:00, weight 0.20) and
after (2024-05-06 01:00:00, weight 0.80) in time
14:19:13 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494376706649255 and -59.23496487766159 degrees.
14:19:13 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.494376706649255 and -59.23496487766159 degrees.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:13 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:13 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:13 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:13 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:13 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:13 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.259263 (min) 0.222028 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.234634 (min) 0.585239 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: x_wind: -3.71769 (min) 4.01158 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.44597 (min) -0.438799 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 9.07149 (min) 96.2946 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11231 (min) 10.6934 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.3052 (min) 34.2473 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000224835 (min) 0.000312179 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:13 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:13 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:13 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:13 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:13 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:13 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:13 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 2]
14:19:13 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 0]
14:19:13 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 460. 0.]
[ 0. 0. 0. 0. 0.]
[ 45. 0. 0. 423. 0.]
[ 19. 0. 523. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:13 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:13 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:13 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.014314712542376106
14:19:13 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:13 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:13 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:13 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:13 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 2
14:19:13 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:13 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 2 elements
14:19:13 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:13 INFO opendrift.models.chemicaldrift:1861: partitioning: [53, 0, 100, 347, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:13 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:13 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:13 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:13 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:13 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:13 INFO opendrift.models.basemodel:2011: 2024-05-06 01:17:58.364115 - step 71 of 96 - 500 active elements (0 deactivated)
14:19:13 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:13 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:13 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.82687032221979
14:19:13 DEBUG opendrift.models.basemodel:2035: 10.50309233103943 <- longitude -> 10.768435614183618
14:19:13 DEBUG opendrift.models.basemodel:2040: -33.92257500759199 <- z -> -0.2652949858753698
14:19:13 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:13 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:13 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:13 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:13 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:13 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 01:00:00 (before)
2024-05-06 02:00:00 (after)
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:15 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:15 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x39x7) for time after (2024-05-06 02:00:00)
14:19:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 01:00:00) in space (linearNDFast)
14:19:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 02:00:00) in space (linearNDFast)
14:19:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 01:00:00, weight 0.70) and
after (2024-05-06 02:00:00, weight 0.30) in time
14:19:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49691876698036 and -59.23157539840933 degrees.
14:19:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49691876698036 and -59.23157539840933 degrees.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.327992 (min) 0.432152 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.208534 (min) 0.572808 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.12818 (min) 3.57031 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.49712 (min) 0.515058 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.93125 (min) 96.362 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11518 (min) 10.6783 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.2945 (min) 34.2541 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000434021 (min) 0.000241294 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:15 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:15 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0]
14:19:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3]
14:19:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 463. 0.]
[ 0. 0. 0. 0. 0.]
[ 45. 0. 0. 431. 0.]
[ 19. 0. 525. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.013938372815833577
14:19:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 24
14:19:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 24 elements
14:19:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [50, 0, 120, 330, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:15 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:15 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:15 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:15 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:15 INFO opendrift.models.basemodel:2011: 2024-05-06 01:47:58.364115 - step 72 of 96 - 500 active elements (0 deactivated)
14:19:15 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:15 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:15 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.82870576085033
14:19:15 DEBUG opendrift.models.basemodel:2035: 10.50069452168621 <- longitude -> 10.770831881586837
14:19:15 DEBUG opendrift.models.basemodel:2040: -34.101566772610106 <- z -> -0.36238378135230487
14:19:15 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 01:00:00 (before)
2024-05-06 02:00:00 (after)
14:19:15 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 01:00:00) in space (linearNDFast)
14:19:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:15 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 02:00:00) in space (linearNDFast)
14:19:15 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:15 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 01:00:00, weight 0.20) and
after (2024-05-06 02:00:00, weight 0.80) in time
14:19:15 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49931656805745 and -59.22917912935427 degrees.
14:19:15 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49931656805745 and -59.22917912935427 degrees.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:15 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:15 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:15 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:15 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:15 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:15 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.47977 (min) 0.365201 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.174739 (min) 0.716583 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.92193 (min) 2.94866 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.983773 (min) 1.46981 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.79357 (min) 98.0789 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11933 (min) 10.6827 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.9152 (min) 34.2755 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000452017 (min) 0.000155888 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:15 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:15 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:15 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:15 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:15 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:15 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:15 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:15 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 0 0 0]
14:19:15 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3]
14:19:15 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 466. 0.]
[ 0. 0. 0. 0. 0.]
[ 45. 0. 0. 435. 0.]
[ 20. 0. 549. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:15 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:15 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:15 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.017514521667439064
14:19:15 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:15 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:15 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:15 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 37
14:19:15 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:15 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 37 elements
14:19:15 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:15 INFO opendrift.models.chemicaldrift:1861: partitioning: [48, 0, 143, 309, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:15 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:15 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:15 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:15 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:15 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:15 INFO opendrift.models.basemodel:2011: 2024-05-06 02:17:58.364115 - step 73 of 96 - 500 active elements (0 deactivated)
14:19:15 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:15 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:15 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.83412880740449
14:19:15 DEBUG opendrift.models.basemodel:2035: 10.498559436729488 <- longitude -> 10.772192766427494
14:19:15 DEBUG opendrift.models.basemodel:2040: -32.66510700320124 <- z -> -0.8423464127326488
14:19:15 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:15 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:15 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:15 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:15 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:15 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 02:00:00 (before)
2024-05-06 03:00:00 (after)
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:18 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:18 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x41x7) for time after (2024-05-06 03:00:00)
14:19:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 02:00:00) in space (linearNDFast)
14:19:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 03:00:00) in space (linearNDFast)
14:19:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 02:00:00, weight 0.70) and
after (2024-05-06 03:00:00, weight 0.30) in time
14:19:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.501451658745765 and -59.22781823177475 degrees.
14:19:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.501451658745765 and -59.22781823177475 degrees.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.512531 (min) 0.33887 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.177157 (min) 0.696471 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.13077 (min) 1.96476 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.39557 (min) 2.3668 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.66623 (min) 102.091 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12004 (min) 10.6741 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.9288 (min) 34.2916 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000581507 (min) 0.000117251 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:18 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:18 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 2 0]
14:19:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 0 3]
14:19:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 470. 0.]
[ 0. 0. 0. 0. 0.]
[ 46. 0. 0. 449. 0.]
[ 20. 0. 586. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01911379780850556
14:19:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 4
14:19:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 4 elements
14:19:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [45, 0, 132, 323, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:18 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:18 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:18 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:18 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:18 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:18 INFO opendrift.models.basemodel:2011: 2024-05-06 02:47:58.364115 - step 74 of 96 - 500 active elements (0 deactivated)
14:19:18 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:18 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:18 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.83906591647128
14:19:18 DEBUG opendrift.models.basemodel:2035: 10.49678731633285 <- longitude -> 10.772543597169106
14:19:18 DEBUG opendrift.models.basemodel:2040: -29.44157421808262 <- z -> -0.5053018252203612
14:19:18 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 02:00:00 (before)
2024-05-06 03:00:00 (after)
14:19:18 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 02:00:00) in space (linearNDFast)
14:19:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:18 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 03:00:00) in space (linearNDFast)
14:19:18 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:18 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 02:00:00, weight 0.20) and
after (2024-05-06 03:00:00, weight 0.80) in time
14:19:18 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.503223769555035 and -59.22746741317539 degrees.
14:19:18 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.503223769555035 and -59.22746741317539 degrees.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:18 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:18 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:18 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:18 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:18 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:18 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.493872 (min) 0.272703 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.171073 (min) 0.849752 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.01423 (min) 0.777419 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.186204 (min) 3.23056 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.5635 (min) 105.243 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12004 (min) 10.6671 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.9304 (min) 34.3616 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000692423 (min) 0.000208725 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:18 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:18 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:18 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:18 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:18 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:18 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
14:19:18 DEBUG opendrift.models.chemicaldrift:1452: old species: [0]
14:19:18 DEBUG opendrift.models.chemicaldrift:1453: new species: [3]
14:19:18 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 471. 0.]
[ 0. 0. 0. 0. 0.]
[ 46. 0. 0. 463. 0.]
[ 20. 0. 590. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:18 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:18 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:18 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.018197439276995346
14:19:18 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:18 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:18 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:18 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:18 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:18 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
14:19:18 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:18 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
14:19:18 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:18 INFO opendrift.models.chemicaldrift:1861: partitioning: [44, 0, 127, 329, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:18 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:18 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:18 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:18 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:18 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:18 INFO opendrift.models.basemodel:2011: 2024-05-06 03:17:58.364115 - step 75 of 96 - 500 active elements (0 deactivated)
14:19:18 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:18 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:18 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.841848959589235
14:19:18 DEBUG opendrift.models.basemodel:2035: 10.495391631823056 <- longitude -> 10.771964419850866
14:19:18 DEBUG opendrift.models.basemodel:2040: -32.1534793038863 <- z -> -0.1399676170729099
14:19:18 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:18 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:18 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:18 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:18 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:18 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 03:00:00 (before)
2024-05-06 04:00:00 (after)
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:20 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:20 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x44x7) for time after (2024-05-06 04:00:00)
14:19:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 03:00:00) in space (linearNDFast)
14:19:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 04:00:00) in space (linearNDFast)
14:19:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 03:00:00, weight 0.70) and
after (2024-05-06 04:00:00, weight 0.30) in time
14:19:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.504619465119646 and -59.22804660112163 degrees.
14:19:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.504619465119646 and -59.22804660112163 degrees.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.498073 (min) 0.349745 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.154331 (min) 0.743264 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: -4.97162 (min) -0.147347 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.175768 (min) 2.98989 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.50914 (min) 106.53 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11897 (min) 10.6596 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4171 (min) 34.3811 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000771708 (min) 0.000239713 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:20 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:20 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
14:19:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0]
14:19:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3]
14:19:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 472. 0.]
[ 0. 0. 0. 0. 0.]
[ 47. 0. 0. 475. 0.]
[ 20. 0. 597. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01788728327513461
14:19:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
14:19:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
14:19:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [44, 0, 123, 333, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:20 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:20 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:20 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:20 INFO opendrift.models.basemodel:2011: 2024-05-06 03:47:58.364115 - step 76 of 96 - 500 active elements (0 deactivated)
14:19:20 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:20 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:20 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84385062206759
14:19:20 DEBUG opendrift.models.basemodel:2035: 10.494386784502238 <- longitude -> 10.770687262500765
14:19:20 DEBUG opendrift.models.basemodel:2040: -31.865190925137167 <- z -> -0.4041125209040361
14:19:20 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 03:00:00 (before)
2024-05-06 04:00:00 (after)
14:19:20 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 03:00:00) in space (linearNDFast)
14:19:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:20 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 04:00:00) in space (linearNDFast)
14:19:20 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:20 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 03:00:00, weight 0.20) and
after (2024-05-06 04:00:00, weight 0.80) in time
14:19:20 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50562431061297 and -59.2293237438808 degrees.
14:19:20 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50562431061297 and -59.2293237438808 degrees.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:20 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:20 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:20 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:20 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:20 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:20 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.384412 (min) 0.378624 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.13113 (min) 0.690782 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.00437 (min) -1.17886 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.374376 (min) 2.08333 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.40837 (min) 106.739 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11591 (min) 10.6509 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4118 (min) 34.3332 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000769404 (min) 0.000317835 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:20 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:20 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:20 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:20 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:20 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:20 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
14:19:20 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 2 0 0 0 3]
14:19:20 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0 3 3 3 0]
14:19:20 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 477. 0.]
[ 0. 0. 0. 0. 0.]
[ 49. 0. 0. 485. 0.]
[ 21. 0. 604. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:20 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:20 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:20 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.018202290125831813
14:19:20 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:20 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:20 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:20 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:20 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 15
14:19:20 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:20 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 15 elements
14:19:20 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:20 INFO opendrift.models.chemicaldrift:1861: partitioning: [42, 0, 124, 334, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:20 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:20 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:20 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:20 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:20 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:20 INFO opendrift.models.basemodel:2011: 2024-05-06 04:17:58.364115 - step 77 of 96 - 500 active elements (0 deactivated)
14:19:20 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:20 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:20 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.843980667073865
14:19:20 DEBUG opendrift.models.basemodel:2035: 10.493791822089749 <- longitude -> 10.768856339093906
14:19:20 DEBUG opendrift.models.basemodel:2040: -32.980669411605675 <- z -> -0.23022655873722142
14:19:20 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:20 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:20 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:20 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:20 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:20 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 04:00:00 (before)
2024-05-06 05:00:00 (after)
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:22 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:22 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 57x45x7) for time after (2024-05-06 05:00:00)
14:19:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 04:00:00) in space (linearNDFast)
14:19:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 05:00:00) in space (linearNDFast)
14:19:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 04:00:00, weight 0.70) and
after (2024-05-06 05:00:00, weight 0.30) in time
14:19:22 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50621926139946 and -59.231154673697205 degrees.
14:19:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50621926139946 and -59.231154673697205 degrees.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.375439 (min) 0.359041 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.101197 (min) 1.04775 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.08422 (min) -2.36671 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.333722 (min) 1.67211 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.32503 (min) 106.117 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11456 (min) 10.6458 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.532 (min) 34.3476 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000744496 (min) 0.000328207 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:22 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:22 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:22 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:22 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:22 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:22 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 9
14:19:22 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 0 0 2 2 0]
14:19:22 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 3 3 0 0 3]
14:19:22 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 481. 0.]
[ 0. 0. 0. 0. 0.]
[ 54. 0. 0. 497. 0.]
[ 21. 0. 619. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:22 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:22 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:22 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01876359759054031
14:19:22 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:22 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:22 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 1 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 4 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 2 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 19
14:19:22 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 19 elements
14:19:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:22 INFO opendrift.models.chemicaldrift:1861: partitioning: [43, 0, 131, 326, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:22 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:22 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:22 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:22 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:22 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:22 INFO opendrift.models.basemodel:2011: 2024-05-06 04:47:58.364115 - step 78 of 96 - 500 active elements (0 deactivated)
14:19:22 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:22 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:22 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84385434504307
14:19:22 DEBUG opendrift.models.basemodel:2035: 10.493612354751377 <- longitude -> 10.766715478666375
14:19:22 DEBUG opendrift.models.basemodel:2040: -33.1874692937952 <- z -> -0.18776291378893217
14:19:22 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 04:00:00 (before)
2024-05-06 05:00:00 (after)
14:19:22 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 04:00:00) in space (linearNDFast)
14:19:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 05:00:00) in space (linearNDFast)
14:19:22 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:22 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 04:00:00, weight 0.20) and
after (2024-05-06 05:00:00, weight 0.80) in time
14:19:22 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.506398739865 and -59.233295526821756 degrees.
14:19:22 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.506398739865 and -59.233295526821756 degrees.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:22 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:22 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:22 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:22 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:22 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:22 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.436662 (min) 0.422789 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0670344 (min) 0.976612 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.1592 (min) -3.48317 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: y_wind: 0.0783848 (min) 1.69074 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.2595 (min) 105.659 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11423 (min) 10.6369 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.1689 (min) 34.4231 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.0006748 (min) 0.000836828 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:22 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:22 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:22 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:22 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:22 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:22 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:22 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 4
14:19:22 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 3]
14:19:22 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 0]
14:19:22 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 483. 0.]
[ 0. 0. 0. 0. 0.]
[ 55. 0. 0. 504. 0.]
[ 22. 0. 638. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:22 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:22 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:22 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.01924262244359884
14:19:22 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:22 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:22 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:22 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 5 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:22 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:22 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:22 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 34
14:19:22 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:22 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 34 elements
14:19:22 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:22 INFO opendrift.models.chemicaldrift:1861: partitioning: [43, 0, 151, 306, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:22 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:22 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:22 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:22 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:22 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:22 INFO opendrift.models.basemodel:2011: 2024-05-06 05:17:58.364115 - step 79 of 96 - 500 active elements (0 deactivated)
14:19:22 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:22 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:22 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84357036473121
14:19:22 DEBUG opendrift.models.basemodel:2035: 10.493868908974404 <- longitude -> 10.765494678518571
14:19:22 DEBUG opendrift.models.basemodel:2040: -34.22826385498047 <- z -> -0.1985144740988407
14:19:22 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:22 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:22 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:22 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:22 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:22 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 05:00:00 (before)
2024-05-06 06:00:00 (after)
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:24 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:24 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 58x46x7) for time after (2024-05-06 06:00:00)
14:19:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 05:00:00) in space (linearNDFast)
14:19:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:24 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 06:00:00) in space (linearNDFast)
14:19:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:24 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 05:00:00, weight 0.70) and
after (2024-05-06 06:00:00, weight 0.30) in time
14:19:24 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50614217728848 and -59.234516330800574 degrees.
14:19:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50614217728848 and -59.234516330800574 degrees.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.38132 (min) 0.499214 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0323595 (min) 0.872204 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.41865 (min) -4.12005 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.370654 (min) 1.31419 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.22798 (min) 105.114 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11356 (min) 10.6314 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.1764 (min) 34.4321 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000704201 (min) 0.00100156 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:24 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:24 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:24 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:24 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:24 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:24 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:24 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0]
14:19:24 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3]
14:19:24 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 485. 0.]
[ 0. 0. 0. 0. 0.]
[ 56. 0. 0. 517. 0.]
[ 22. 0. 672. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:24 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:24 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:24 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.02132061275744904
14:19:24 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:24 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:24 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:24 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:24 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 38
14:19:24 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 38 elements
14:19:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:24 INFO opendrift.models.chemicaldrift:1861: partitioning: [42, 0, 176, 282, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:24 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:24 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:24 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:24 INFO opendrift.models.basemodel:2011: 2024-05-06 05:47:58.364115 - step 80 of 96 - 500 active elements (0 deactivated)
14:19:24 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:24 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:24 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84339942157582
14:19:24 DEBUG opendrift.models.basemodel:2035: 10.494549660983926 <- longitude -> 10.768645392195188
14:19:24 DEBUG opendrift.models.basemodel:2040: -35.93336406575726 <- z -> -0.0579195466313005
14:19:24 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 05:00:00 (before)
2024-05-06 06:00:00 (after)
14:19:24 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 05:00:00) in space (linearNDFast)
14:19:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:24 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 06:00:00) in space (linearNDFast)
14:19:24 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:24 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 05:00:00, weight 0.20) and
after (2024-05-06 06:00:00, weight 0.80) in time
14:19:24 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50546142414665 and -59.23136561310772 degrees.
14:19:24 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50546142414665 and -59.23136561310772 degrees.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:24 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:24 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:24 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:24 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:24 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:24 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.353644 (min) 0.520877 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.00942514 (min) 0.733712 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: x_wind: -5.74333 (min) -4.41427 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: y_wind: -0.931647 (min) 1.79809 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.22347 (min) 104.439 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.1126 (min) 10.6304 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.577 (min) 34.4354 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000851257 (min) 0.00106913 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:24 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:24 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:24 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:24 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:24 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:24 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:24 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:24 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2]
14:19:24 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0]
14:19:24 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 486. 0.]
[ 0. 0. 0. 0. 0.]
[ 58. 0. 0. 529. 0.]
[ 22. 0. 710. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:24 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:24 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:24 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.024467343216695532
14:19:24 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:24 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:24 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:24 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 6 elements penetrated seafloor, lifting up
14:19:24 DEBUG opendrift.models.oceandrift:618: 7 elements penetrated seafloor, lifting up
14:19:24 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 71
14:19:24 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:24 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 71 elements
14:19:24 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:24 INFO opendrift.models.chemicaldrift:1861: partitioning: [43, 0, 235, 222, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:24 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:24 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:24 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:24 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:24 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:24 INFO opendrift.models.basemodel:2011: 2024-05-06 06:17:58.364115 - step 81 of 96 - 500 active elements (0 deactivated)
14:19:24 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:24 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:24 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.843246805252456
14:19:24 DEBUG opendrift.models.basemodel:2035: 10.495667340841045 <- longitude -> 10.771304975966016
14:19:24 DEBUG opendrift.models.basemodel:2040: -36.53030397284498 <- z -> 0.0
14:19:24 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:24 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:24 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:24 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:24 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:24 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 06:00:00 (before)
2024-05-06 07:00:00 (after)
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:26 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:26 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 60x47x7) for time after (2024-05-06 07:00:00)
14:19:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 06:00:00) in space (linearNDFast)
14:19:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 07:00:00) in space (linearNDFast)
14:19:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 06:00:00, weight 0.70) and
after (2024-05-06 07:00:00, weight 0.30) in time
14:19:26 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.504343733014736 and -59.22870602715753 degrees.
14:19:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.504343733014736 and -59.22870602715753 degrees.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.409721 (min) 0.620213 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: 0.00723171 (min) 0.863459 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -6.60394 (min) -4.47484 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -1.61673 (min) 0.757384 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.2617 (min) 103.507 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.1122 (min) 10.6347 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4472 (min) 34.435 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000967924 (min) 0.000599666 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:26 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:19:26 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:26 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:26 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:26 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:26 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 1
14:19:26 DEBUG opendrift.models.chemicaldrift:1452: old species: [2]
14:19:26 DEBUG opendrift.models.chemicaldrift:1453: new species: [0]
14:19:26 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 486. 0.]
[ 0. 0. 0. 0. 0.]
[ 59. 0. 0. 539. 0.]
[ 22. 0. 781. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:26 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:26 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:26 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.03340770298910741
14:19:26 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:26 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:26 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:26 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:618: 8 elements penetrated seafloor, lifting up
14:19:26 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:26 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:26 DEBUG opendrift.models.oceandrift:618: 3 elements penetrated seafloor, lifting up
14:19:26 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 80
14:19:26 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:26 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 80 elements
14:19:26 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:26 INFO opendrift.models.chemicaldrift:1861: partitioning: [44, 0, 296, 160, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:26 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:26 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:26 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:26 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:26 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:26 INFO opendrift.models.basemodel:2011: 2024-05-06 06:47:58.364115 - step 82 of 96 - 500 active elements (0 deactivated)
14:19:26 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:26 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:26 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84347522981248
14:19:26 DEBUG opendrift.models.basemodel:2035: 10.497268755438148 <- longitude -> 10.773566026616189
14:19:26 DEBUG opendrift.models.basemodel:2040: -35.12515615855792 <- z -> 0.0
14:19:26 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:26 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 06:00:00 (before)
2024-05-06 07:00:00 (after)
14:19:26 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 06:00:00) in space (linearNDFast)
14:19:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:26 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 07:00:00) in space (linearNDFast)
14:19:26 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:26 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 06:00:00, weight 0.20) and
after (2024-05-06 07:00:00, weight 0.80) in time
14:19:26 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.502742321202085 and -59.22644497314782 degrees.
14:19:26 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.502742321202085 and -59.22644497314782 degrees.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:26 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:26 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:26 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:26 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:26 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:26 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:26 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:26 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:26 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:26 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:26 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.475662 (min) 0.525587 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0316453 (min) 0.798085 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.52526 (min) -4.04872 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.08708 (min) 1.24175 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.25424 (min) 105.519 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11218 (min) 10.6396 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.4611 (min) 34.4197 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00120018 (min) 0.000443827 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:26 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:27 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:27 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:27 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:27 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:27 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:27 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:27 DEBUG opendrift.models.chemicaldrift:1452: old species: [3 2 2 2 0]
14:19:27 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 3]
14:19:27 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 487. 0.]
[ 0. 0. 0. 0. 0.]
[ 62. 0. 0. 557. 0.]
[ 23. 0. 861. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:27 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:27 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:27 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.043763763929970015
14:19:27 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:27 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:27 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:27 DEBUG opendrift.models.oceandrift:618: 37 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 10 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:27 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:27 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:27 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:27 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 65
14:19:27 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:27 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 65 elements
14:19:27 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:27 INFO opendrift.models.chemicaldrift:1861: partitioning: [47, 0, 344, 109, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:27 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:27 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:27 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:27 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:27 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:27 INFO opendrift.models.basemodel:2011: 2024-05-06 07:17:58.364115 - step 83 of 96 - 500 active elements (0 deactivated)
14:19:27 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:27 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:27 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.847564982329175
14:19:27 DEBUG opendrift.models.basemodel:2035: 10.499348760986729 <- longitude -> 10.775844448609021
14:19:27 DEBUG opendrift.models.basemodel:2040: -33.333660800313375 <- z -> -0.20333607144117716
14:19:27 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:27 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:27 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:27 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:27 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:27 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:27 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:27 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:27 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 07:00:00 (before)
2024-05-06 08:00:00 (after)
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:29 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:29 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 61x48x7) for time after (2024-05-06 08:00:00)
14:19:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 07:00:00) in space (linearNDFast)
14:19:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 08:00:00) in space (linearNDFast)
14:19:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 07:00:00, weight 0.70) and
after (2024-05-06 08:00:00, weight 0.30) in time
14:19:29 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50066232885728 and -59.22416654965072 degrees.
14:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50066232885728 and -59.22416654965072 degrees.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.28319 (min) 0.503805 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0415182 (min) 0.7154 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.88591 (min) -3.65523 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.50632 (min) 0.0421208 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.20076 (min) 109.279 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11126 (min) 10.651 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6872 (min) 34.391 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.00115143 (min) 0.000473719 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:29 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:29 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:29 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:29 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:29 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:29 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:29 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 2 0 0]
14:19:29 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 0 3 3]
14:19:29 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 491. 0.]
[ 0. 0. 0. 0. 0.]
[ 63. 0. 0. 571. 0.]
[ 23. 0. 926. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:29 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:29 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04890924358069812
14:19:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 40
14:19:29 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 40 elements
14:19:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:29 INFO opendrift.models.chemicaldrift:1861: partitioning: [44, 0, 363, 93, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:29 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:29 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:29 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:29 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:29 INFO opendrift.models.basemodel:2011: 2024-05-06 07:47:58.364115 - step 84 of 96 - 500 active elements (0 deactivated)
14:19:29 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:29 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:29 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84705258054687
14:19:29 DEBUG opendrift.models.basemodel:2035: 10.501794492869713 <- longitude -> 10.77821016345026
14:19:29 DEBUG opendrift.models.basemodel:2040: -36.4611225636613 <- z -> -0.009636479614050647
14:19:29 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 07:00:00 (before)
2024-05-06 08:00:00 (after)
14:19:29 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 07:00:00) in space (linearNDFast)
14:19:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:29 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 08:00:00) in space (linearNDFast)
14:19:29 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:29 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 07:00:00, weight 0.20) and
after (2024-05-06 08:00:00, weight 0.80) in time
14:19:29 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49821658681736 and -59.22180083315556 degrees.
14:19:29 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49821658681736 and -59.22180083315556 degrees.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:29 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:29 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:29 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:29 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:29 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:29 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.726586 (min) 0.492244 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0460459 (min) 0.687663 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.90189 (min) -3.0347 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: y_wind: -2.85896 (min) -0.59798 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.16537 (min) 109.1 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11123 (min) 10.6658 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6954 (min) 34.3874 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000773749 (min) 0.000398282 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:29 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:29 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:29 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:29 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:29 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:29 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:29 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 3
14:19:29 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2]
14:19:29 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0]
14:19:29 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 492. 0.]
[ 0. 0. 0. 0. 0.]
[ 65. 0. 0. 591. 0.]
[ 23. 0. 966. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:29 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:29 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:29 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05048551172913925
14:19:29 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:29 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:29 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:29 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:29 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:29 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:29 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 23
14:19:29 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:29 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 23 elements
14:19:29 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:29 INFO opendrift.models.chemicaldrift:1861: partitioning: [45, 0, 360, 95, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:29 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:29 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:29 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:29 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:29 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:29 INFO opendrift.models.basemodel:2011: 2024-05-06 08:17:58.364115 - step 85 of 96 - 500 active elements (0 deactivated)
14:19:29 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:29 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:29 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84685462140044
14:19:29 DEBUG opendrift.models.basemodel:2035: 10.504732262123227 <- longitude -> 10.780486331551412
14:19:29 DEBUG opendrift.models.basemodel:2040: -35.89795561049234 <- z -> -0.27731236714436874
14:19:29 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:29 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:29 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:29 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:29 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:29 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 08:00:00 (before)
2024-05-06 09:00:00 (after)
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:33 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:33 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 61x49x7) for time after (2024-05-06 09:00:00)
14:19:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 08:00:00) in space (linearNDFast)
14:19:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 09:00:00) in space (linearNDFast)
14:19:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 08:00:00, weight 0.70) and
after (2024-05-06 09:00:00, weight 0.30) in time
14:19:33 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.495278810547255 and -59.21952466361465 degrees.
14:19:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.495278810547255 and -59.21952466361465 degrees.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.587765 (min) 0.437453 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.053145 (min) 0.712969 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.22272 (min) -3.87861 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.5431 (min) -1.7244 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.16715 (min) 109.01 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11312 (min) 10.6799 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7542 (min) 34.3795 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000588651 (min) 0.000394569 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:33 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:33 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:33 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:33 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:33 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:19:33 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 2 2]
14:19:33 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 0 0]
14:19:33 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 493. 0.]
[ 0. 0. 0. 0. 0.]
[ 70. 0. 0. 615. 0.]
[ 23. 0. 989. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:33 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:33 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:33 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:33 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05526493234605538
14:19:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:33 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 4 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 26
14:19:33 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:33 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 26 elements
14:19:33 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:33 INFO opendrift.models.chemicaldrift:1861: partitioning: [49, 0, 352, 99, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:33 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:33 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:33 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:33 INFO opendrift.models.basemodel:2011: 2024-05-06 08:47:58.364115 - step 86 of 96 - 500 active elements (0 deactivated)
14:19:33 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:33 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:33 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84659868705493
14:19:33 DEBUG opendrift.models.basemodel:2035: 10.507789432274826 <- longitude -> 10.78281258720379
14:19:33 DEBUG opendrift.models.basemodel:2040: -37.01543426513672 <- z -> -0.4104919678425354
14:19:33 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 08:00:00 (before)
2024-05-06 09:00:00 (after)
14:19:33 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 08:00:00) in space (linearNDFast)
14:19:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:33 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 09:00:00) in space (linearNDFast)
14:19:33 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:33 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 08:00:00, weight 0.20) and
after (2024-05-06 09:00:00, weight 0.80) in time
14:19:33 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49222164319906 and -59.21719840408003 degrees.
14:19:33 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.49222164319906 and -59.21719840408003 degrees.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:33 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:33 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:33 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:33 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:33 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:33 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.597273 (min) 0.306887 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0540297 (min) 0.56554 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.71607 (min) -5.762 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.61482 (min) -2.94539 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.19974 (min) 108.846 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11312 (min) 10.6914 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7332 (min) 34.3712 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000469046 (min) 0.0004056 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:33 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:33 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:33 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:33 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:33 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:33 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:19:33 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 2 2 2 2]
14:19:33 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 0 0 0 0]
14:19:33 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 493. 0.]
[ 0. 0. 0. 0. 0.]
[ 76. 0. 0. 644. 0.]
[ 23. 0. 1015. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:33 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:33 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:33 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:33 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:33 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0635528017583266
14:19:33 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:33 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:33 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:33 DEBUG opendrift.models.oceandrift:618: 38 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 34 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 4 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:33 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:33 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:33 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:33 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 11
14:19:33 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:33 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 11 elements
14:19:33 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:33 INFO opendrift.models.chemicaldrift:1861: partitioning: [55, 0, 339, 106, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:33 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:33 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:33 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:33 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:33 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:33 INFO opendrift.models.basemodel:2011: 2024-05-06 09:17:58.364115 - step 87 of 96 - 500 active elements (0 deactivated)
14:19:33 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:33 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:33 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84842791527946
14:19:33 DEBUG opendrift.models.basemodel:2035: 10.51032640253751 <- longitude -> 10.785830053012933
14:19:33 DEBUG opendrift.models.basemodel:2040: -38.190861103302055 <- z -> 0.0
14:19:33 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:33 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:33 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:33 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:33 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:33 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 09:00:00 (before)
2024-05-06 10:00:00 (after)
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:35 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:35 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 62x50x7) for time after (2024-05-06 10:00:00)
14:19:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 09:00:00) in space (linearNDFast)
14:19:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 10:00:00) in space (linearNDFast)
14:19:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 1 elements, expanding data 1
14:19:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 09:00:00, weight 0.70) and
after (2024-05-06 10:00:00, weight 0.30) in time
14:19:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48968467197323 and -59.214180938239465 degrees.
14:19:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48968467197323 and -59.214180938239465 degrees.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.851855 (min) 0.303225 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.0870553 (min) 0.588805 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.91369 (min) -6.90902 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.89267 (min) -3.10558 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.23823 (min) 108.89 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.11596 (min) 10.704 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.6976 (min) 34.3944 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000390923 (min) 0.00044775 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:35 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:35 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:35 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:35 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:35 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:35 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:35 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:19:35 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 2 2 2 2]
14:19:35 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 0 0 0]
14:19:35 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 494. 0.]
[ 0. 0. 0. 0. 0.]
[ 81. 0. 0. 662. 0.]
[ 23. 0. 1026. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:35 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:35 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06706038206109526
14:19:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:35 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 36 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 13
14:19:35 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 13 elements
14:19:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:35 INFO opendrift.models.chemicaldrift:1861: partitioning: [59, 0, 325, 116, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:35 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:35 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:35 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:35 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:35 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:35 INFO opendrift.models.basemodel:2011: 2024-05-06 09:47:58.364115 - step 88 of 96 - 500 active elements (0 deactivated)
14:19:35 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:35 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:35 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.849543388157265
14:19:35 DEBUG opendrift.models.basemodel:2035: 10.512737412431276 <- longitude -> 10.789299724972931
14:19:35 DEBUG opendrift.models.basemodel:2040: -37.11373519897461 <- z -> -0.03222928771108334
14:19:35 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 09:00:00 (before)
2024-05-06 10:00:00 (after)
14:19:35 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 09:00:00) in space (linearNDFast)
14:19:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:35 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 10:00:00) in space (linearNDFast)
14:19:35 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:35 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 09:00:00, weight 0.20) and
after (2024-05-06 10:00:00, weight 0.80) in time
14:19:35 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48727366200829 and -59.21071126700242 degrees.
14:19:35 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.48727366200829 and -59.21071126700242 degrees.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:35 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:35 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:35 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:35 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:35 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:35 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.72614 (min) 0.294287 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.169742 (min) 0.528269 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.81101 (min) -7.51809 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.94243 (min) -3.28621 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.30104 (min) 109.626 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12071 (min) 10.7154 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7978 (min) 34.3845 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000405532 (min) 0.000627639 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:35 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:35 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:35 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:35 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:35 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:35 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:35 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:35 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:35 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 3 2 0 2]
14:19:35 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 0 3 0]
14:19:35 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 496. 0.]
[ 0. 0. 0. 0. 0.]
[ 83. 0. 0. 684. 0.]
[ 24. 0. 1039. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:35 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:35 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:35 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06646133400391091
14:19:35 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:35 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:35 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:35 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 44 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 35 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:35 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:35 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:35 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:35 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 12
14:19:35 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:35 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 12 elements
14:19:35 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:35 INFO opendrift.models.chemicaldrift:1861: partitioning: [60, 0, 313, 127, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:35 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:35 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:35 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:35 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:35 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:35 INFO opendrift.models.basemodel:2011: 2024-05-06 10:17:58.364115 - step 89 of 96 - 500 active elements (0 deactivated)
14:19:35 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:35 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:35 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84918568204118
14:19:35 DEBUG opendrift.models.basemodel:2035: 10.499302672792687 <- longitude -> 10.793877656150176
14:19:35 DEBUG opendrift.models.basemodel:2040: -39.1934278907438 <- z -> -0.02273608307177699
14:19:35 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:35 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:35 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:35 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:35 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:35 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 10:00:00 (before)
2024-05-06 11:00:00 (after)
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:38 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:38 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 61x52x7) for time after (2024-05-06 11:00:00)
14:19:38 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 10:00:00) in space (linearNDFast)
14:19:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:38 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 11:00:00) in space (linearNDFast)
14:19:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:38 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 10:00:00, weight 0.70) and
after (2024-05-06 11:00:00, weight 0.30) in time
14:19:38 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50070833404538 and -59.206133335292876 degrees.
14:19:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50070833404538 and -59.206133335292876 degrees.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.233911 (min) 0.307873 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.191142 (min) 0.577343 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.58076 (min) -7.684 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: -3.94196 (min) -3.34975 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.34185 (min) 109.491 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.1212 (min) 10.7237 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.918 (min) 34.4254 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000264921 (min) 0.000790329 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:38 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:38 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:38 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:38 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:38 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 6
14:19:38 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2 0 2 0 2]
14:19:38 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0 3 0 3 0]
14:19:38 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 498. 0.]
[ 0. 0. 0. 0. 0.]
[ 87. 0. 0. 706. 0.]
[ 24. 0. 1051. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:38 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:38 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:38 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.06339105737070376
14:19:38 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:38 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:38 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 4 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 33 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 16
14:19:38 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 16 elements
14:19:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:38 INFO opendrift.models.chemicaldrift:1861: partitioning: [62, 0, 302, 136, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:38 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:38 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:38 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:38 INFO opendrift.models.basemodel:2011: 2024-05-06 10:47:58.364115 - step 90 of 96 - 500 active elements (0 deactivated)
14:19:38 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:38 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:38 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84881730961106
14:19:38 DEBUG opendrift.models.basemodel:2035: 10.49221362290768 <- longitude -> 10.798836305920071
14:19:38 DEBUG opendrift.models.basemodel:2040: -39.32215726511801 <- z -> 0.0
14:19:38 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 10:00:00 (before)
2024-05-06 11:00:00 (after)
14:19:38 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 10:00:00) in space (linearNDFast)
14:19:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:38 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 11:00:00) in space (linearNDFast)
14:19:38 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:38 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 10:00:00, weight 0.20) and
after (2024-05-06 11:00:00, weight 0.80) in time
14:19:38 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50779738634979 and -59.20117468322809 degrees.
14:19:38 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.50779738634979 and -59.20117468322809 degrees.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:38 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:38 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:38 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:38 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:38 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:38 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.636793 (min) 0.257301 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.276545 (min) 0.583841 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: x_wind: -8.07152 (min) -7.12265 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.209 (min) -3.3381 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.35009 (min) 109.328 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12166 (min) 10.7331 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.8832 (min) 34.4295 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000239271 (min) 0.000834421 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:38 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:38 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:38 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:38 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:38 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:38 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:38 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
14:19:38 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 2 0 0]
14:19:38 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 0 3 3]
14:19:38 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 504. 0.]
[ 0. 0. 0. 0. 0.]
[ 88. 0. 0. 729. 0.]
[ 24. 0. 1067. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:38 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:38 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:38 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.0567105841521365
14:19:38 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:38 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:38 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:38 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:38 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:38 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:38 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 12
14:19:38 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:38 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 12 elements
14:19:38 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:38 INFO opendrift.models.chemicaldrift:1861: partitioning: [57, 0, 300, 143, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:38 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:38 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:38 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:38 DEBUG opendrift.models.basemodel:725: No elements hit seafloor.
14:19:38 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:38 INFO opendrift.models.basemodel:2011: 2024-05-06 11:17:58.364115 - step 91 of 96 - 500 active elements (0 deactivated)
14:19:38 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:38 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:38 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84832451021731
14:19:38 DEBUG opendrift.models.basemodel:2035: 10.47291387082093 <- longitude -> 10.804993576340596
14:19:38 DEBUG opendrift.models.basemodel:2040: -39.06114569168928 <- z -> 0.0
14:19:38 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:38 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:38 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:38 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:38 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:38 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 11:00:00 (before)
2024-05-06 12:00:00 (after)
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:40 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:40 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 61x54x7) for time after (2024-05-06 12:00:00)
14:19:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 11:00:00) in space (linearNDFast)
14:19:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 12:00:00) in space (linearNDFast)
14:19:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 2 elements, expanding data 1
14:19:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 11:00:00, weight 0.70) and
after (2024-05-06 12:00:00, weight 0.30) in time
14:19:40 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.52709715333637 and -59.195017415729055 degrees.
14:19:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.52709715333637 and -59.195017415729055 degrees.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.576162 (min) 0.267127 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.334997 (min) 0.540481 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.81848 (min) -6.51152 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.32818 (min) -3.25817 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.31986 (min) 109.102 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.12717 (min) 10.7408 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7892 (min) 34.424 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000236618 (min) 0.000881353 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:40 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:40 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 11
14:19:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 2 0 0 2 0 0 2 2 2 2]
14:19:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 0 3 3 0 3 3 0 0 0 0]
14:19:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 509. 0.]
[ 0. 0. 0. 0. 0.]
[ 94. 0. 0. 742. 0.]
[ 24. 0. 1079. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05382797252675402
14:19:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:40 DEBUG opendrift.models.oceandrift:618: 30 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
14:19:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
14:19:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [58, 0, 287, 155, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:40 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:40 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:40 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:40 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:40 INFO opendrift.models.basemodel:2011: 2024-05-06 11:47:58.364115 - step 92 of 96 - 500 active elements (0 deactivated)
14:19:40 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:40 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:40 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.84825230016169
14:19:40 DEBUG opendrift.models.basemodel:2035: 10.467343717525795 <- longitude -> 10.807605223553024
14:19:40 DEBUG opendrift.models.basemodel:2040: -38.72294289833522 <- z -> 0.0
14:19:40 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 11:00:00 (before)
2024-05-06 12:00:00 (after)
14:19:40 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 11:00:00) in space (linearNDFast)
14:19:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:40 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 12:00:00) in space (linearNDFast)
14:19:40 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:40 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 11:00:00, weight 0.20) and
after (2024-05-06 12:00:00, weight 0.80) in time
14:19:40 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.53266729758898 and -59.192405763455056 degrees.
14:19:40 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.53266729758898 and -59.192405763455056 degrees.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:40 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:40 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:40 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:40 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:40 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:40 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.59975 (min) 0.294426 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.373124 (min) 0.4527 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.43117 (min) -6.08983 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.23798 (min) -3.12991 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.26063 (min) 109.184 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.13569 (min) 10.7473 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.412 (min) 34.3933 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000272029 (min) 0.000791256 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:40 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:40 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 5 elements to seafloor.
14:19:40 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:40 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:40 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:40 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:40 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 5
14:19:40 DEBUG opendrift.models.chemicaldrift:1452: old species: [0 0 0 0 0]
14:19:40 DEBUG opendrift.models.chemicaldrift:1453: new species: [3 3 3 3 3]
14:19:40 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 514. 0.]
[ 0. 0. 0. 0. 0.]
[ 94. 0. 0. 752. 0.]
[ 24. 0. 1082. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:40 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:40 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:40 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.04993037550443522
14:19:40 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:40 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:40 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 28 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:40 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:40 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:40 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 8
14:19:40 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:40 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 8 elements
14:19:40 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:40 INFO opendrift.models.chemicaldrift:1861: partitioning: [53, 0, 282, 165, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:40 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:40 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:40 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:40 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:40 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:40 INFO opendrift.models.basemodel:2011: 2024-05-06 12:17:58.364115 - step 93 of 96 - 500 active elements (0 deactivated)
14:19:40 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:40 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:40 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.85457515046677
14:19:40 DEBUG opendrift.models.basemodel:2035: 10.460760942475261 <- longitude -> 10.814879019744117
14:19:40 DEBUG opendrift.models.basemodel:2040: -39.04771279631908 <- z -> -0.1922492691362081
14:19:40 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:40 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:40 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:40 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:40 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:40 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 12:00:00 (before)
2024-05-06 13:00:00 (after)
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:42 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:42 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 62x54x7) for time after (2024-05-06 13:00:00)
14:19:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 12:00:00) in space (linearNDFast)
14:19:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 13:00:00) in space (linearNDFast)
14:19:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 12:00:00, weight 0.70) and
after (2024-05-06 13:00:00, weight 0.30) in time
14:19:42 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.53925006917749 and -59.18513197308458 degrees.
14:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.53925006917749 and -59.18513197308458 degrees.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.562972 (min) 0.322833 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.407337 (min) 0.520547 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.13418 (min) -6.11236 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.20484 (min) -3.26508 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.18017 (min) 112.565 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.14179 (min) 10.753 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.5 (min) 34.4261 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000222384 (min) 0.000752296 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:42 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 6 elements to seafloor.
14:19:42 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:42 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:42 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:42 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:42 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 2
14:19:42 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 2]
14:19:42 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 0]
14:19:42 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 514. 0.]
[ 0. 0. 0. 0. 0.]
[ 96. 0. 0. 765. 0.]
[ 24. 0. 1090. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:42 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:42 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.047600781829155704
14:19:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:42 DEBUG opendrift.models.oceandrift:618: 32 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 3
14:19:42 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 3 elements
14:19:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:42 INFO opendrift.models.chemicaldrift:1861: partitioning: [55, 0, 271, 174, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:42 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:42 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:42 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:42 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:42 INFO opendrift.models.basemodel:2011: 2024-05-06 12:47:58.364115 - step 94 of 96 - 500 active elements (0 deactivated)
14:19:42 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:42 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:42 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.855595700755806
14:19:42 DEBUG opendrift.models.basemodel:2035: 10.45570128808432 <- longitude -> 10.79783004296683
14:19:42 DEBUG opendrift.models.basemodel:2040: -37.96519546515814 <- z -> -0.0779363236584597
14:19:42 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 12:00:00 (before)
2024-05-06 13:00:00 (after)
14:19:42 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 12:00:00) in space (linearNDFast)
14:19:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:42 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 13:00:00) in space (linearNDFast)
14:19:42 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:42 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 12:00:00, weight 0.20) and
after (2024-05-06 13:00:00, weight 0.80) in time
14:19:42 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.54430972933362 and -59.20218093995139 degrees.
14:19:42 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.54430972933362 and -59.20218093995139 degrees.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:42 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:42 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:42 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:42 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:42 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:42 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.748853 (min) 0.297271 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.400033 (min) 0.58713 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.37568 (min) -5.96403 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.19344 (min) -3.56827 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.09151 (min) 113.069 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.15085 (min) 10.7585 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 21.7381 (min) 34.4123 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000254979 (min) 0.000665172 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:42 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:42 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 6 elements to seafloor.
14:19:42 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:42 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:42 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:42 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:42 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 8
14:19:42 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 2 0 2 2 0 2]
14:19:42 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 0 3 0 0 3 0]
14:19:42 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 517. 0.]
[ 0. 0. 0. 0. 0.]
[ 101. 0. 0. 777. 0.]
[ 24. 0. 1093. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:42 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:42 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:42 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.050256212034030426
14:19:42 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:42 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:42 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:42 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 31 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 29 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:42 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:42 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 7
14:19:42 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:42 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 7 elements
14:19:42 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:42 INFO opendrift.models.chemicaldrift:1861: partitioning: [57, 0, 258, 185, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:42 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:42 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:42 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:42 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:42 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:42 INFO opendrift.models.basemodel:2011: 2024-05-06 13:17:58.364115 - step 95 of 96 - 500 active elements (0 deactivated)
14:19:42 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:42 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:42 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.85508744347691
14:19:42 DEBUG opendrift.models.basemodel:2035: 10.4515998856939 <- longitude -> 10.804245692746841
14:19:42 DEBUG opendrift.models.basemodel:2040: -38.56347824947155 <- z -> 0.0
14:19:42 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:42 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:42 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:42 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:42 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:42 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 13:00:00 (before)
2024-05-06 14:00:00 (after)
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking sea_floor_depth_below_sea_level for invalid values
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_wind for invalid values
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_wind for invalid values
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking x_sea_water_velocity for invalid values
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking y_sea_water_velocity for invalid values
14:19:44 DEBUG opendrift.readers.interpolation.structured:53: Filled NaN-values toward seafloor for :['y_sea_water_velocity', 'x_sea_water_velocity', 'sea_water_salinity', 'sea_water_temperature', 'upward_sea_water_velocity']
14:19:44 DEBUG opendrift.readers.basereader.structured:313: Fetched env-block (size 62x55x7) for time after (2024-05-06 14:00:00)
14:19:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 13:00:00) in space (linearNDFast)
14:19:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:44 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 14:00:00) in space (linearNDFast)
14:19:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift:135: Linear2DInterpolator informational: NaN values for 3 elements, expanding data 1
14:19:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 13:00:00, weight 0.70) and
after (2024-05-06 14:00:00, weight 0.30) in time
14:19:44 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.54841113129697 and -59.19576529246763 degrees.
14:19:44 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.54841113129697 and -59.19576529246763 degrees.
14:19:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:44 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:44 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:44 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:44 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:44 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:44 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:44 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:44 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:44 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.65103 (min) 0.339379 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.380047 (min) 0.539476 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.59465 (min) -6.14104 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.18215 (min) -3.60681 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.09151 (min) 112.737 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.16238 (min) 10.759 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.8719 (min) 34.378 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000227252 (min) 0.00057008 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:44 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:44 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:44 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:44 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:44 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:44 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:44 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
14:19:44 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 0 0 0 2]
14:19:44 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 3 3 3 0]
14:19:44 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 522. 0.]
[ 0. 0. 0. 0. 0.]
[ 103. 0. 0. 792. 0.]
[ 24. 0. 1100. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:44 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:44 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:44 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05212368998325451
14:19:44 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:44 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:44 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 13 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:44 DEBUG opendrift.models.oceandrift:618: 20 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:44 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:44 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 35
14:19:44 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:44 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 35 elements
14:19:44 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:44 INFO opendrift.models.chemicaldrift:1861: partitioning: [54, 0, 280, 166, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:44 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:44 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:44 DEBUG opendrift.models.basemodel:866: to be seeded: 0, already seeded 500
14:19:44 DEBUG opendrift.models.basemodel:730: Lifting 4 elements to seafloor.
14:19:44 DEBUG opendrift.models.basemodel:2010: ======================================================================
14:19:44 INFO opendrift.models.basemodel:2011: 2024-05-06 13:47:58.364115 - step 96 of 96 - 500 active elements (0 deactivated)
14:19:44 DEBUG opendrift.models.basemodel:2017: 0 elements scheduled.
14:19:44 DEBUG opendrift.models.basemodel:2019: ======================================================================
14:19:44 DEBUG opendrift.models.basemodel:2030: 57.555133429012194 <- latitude -> 57.863146276470104
14:19:44 DEBUG opendrift.models.basemodel:2035: 10.44616227151035 <- longitude -> 10.799532430601595
14:19:44 DEBUG opendrift.models.basemodel:2040: -36.0539521727743 <- z -> -0.31897220563968554
14:19:44 DEBUG opendrift.models.basemodel:2041: ---------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:608: Variable group ['sea_floor_depth_below_sea_level', 'x_wind', 'y_wind', 'sea_water_salinity', 'sea_water_temperature', 'x_sea_water_velocity', 'y_sea_water_velocity', 'upward_sea_water_velocity']
14:19:44 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:613: Calling reader https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be
14:19:44 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:44 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:44 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from https://thredds.met.no/thredds/dodsC/sea/norkyst800m/1h/aggregate_be covering 500 elements
14:19:44 DEBUG opendrift.readers.basereader.structured:222: Reader time:
2024-05-06 13:00:00 (before)
2024-05-06 14:00:00 (after)
14:19:44 DEBUG opendrift.readers.basereader.structured:334: Interpolating before (2024-05-06 13:00:00) in space (linearNDFast)
14:19:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:44 DEBUG opendrift.readers.basereader.structured:340: Interpolating after (2024-05-06 14:00:00) in space (linearNDFast)
14:19:44 DEBUG opendrift.readers.interpolation.structured:80: Initialising interpolator.
14:19:44 DEBUG opendrift.readers.basereader.structured:355: Interpolating before (2024-05-06 13:00:00, weight 0.20) and
after (2024-05-06 14:00:00, weight 0.80) in time
14:19:44 DEBUG opendrift.readers.basereader.structured:368: Interpolating profiles in time
14:19:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.553848755868586 and -59.20047854659377 degrees.
14:19:45 DEBUG opendrift.readers.basereader.variables:96: Rotating vectors between -59.553848755868586 and -59.20047854659377 degrees.
14:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['ocean_mixed_layer_thickness']
14:19:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader constant_reader
14:19:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from constant_reader covering 500 elements
14:19:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 0.0001 for ocean_vertical_diffusivity for all profiles
14:19:45 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 10 for sea_water_temperature for 0 profiles
14:19:45 DEBUG opendrift.models.basemodel.environment:814: Using fallback value 34 for sea_water_salinity for 0 profiles
14:19:45 DEBUG opendrift.models.basemodel.environment:803: Using fallback value 8.1 for sea_water_ph_reported_on_total_scale for all profiles
14:19:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:45 DEBUG opendrift.models.basemodel.environment:905: x_sea_water_velocity: -0.512575 (min) 0.263979 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: y_sea_water_velocity: -0.316048 (min) 0.648965 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_surface_height: 0 (min) 0 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: x_wind: -7.74979 (min) -6.46417 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: y_wind: -4.17619 (min) -3.4224 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_floor_depth_below_sea_level: 8.09151 (min) 115.238 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: ocean_vertical_diffusivity: 0.0001 (min) 0.0001 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_temperature: 6.16912 (min) 10.76 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_salinity: 20.8139 (min) 34.3914 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: upward_sea_water_velocity: -0.000348032 (min) 0.000421897 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: spm: 1 (min) 1 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: ocean_mixed_layer_thickness: 40 (min) 40 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: active_sediment_layer_thickness: 0.03 (min) 0.03 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: doc: 0 (min) 0 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: sea_water_ph_reported_on_total_scale: 8.1 (min) 8.1 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:905: pH_sediment: 6.9 (min) 6.9 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:45 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:45 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:45 DEBUG opendrift.models.basemodel:2082: Calling ChemicalDrift.update()
14:19:45 DEBUG opendrift.models.chemicaldrift:1667: Calculating overall degradation using overall rate constants
14:19:45 DEBUG opendrift.models.chemicaldrift:1722: Calculating: volatilization
14:19:45 INFO opendrift.models.chemicaldrift:1435: Number of transformations: 7
14:19:45 DEBUG opendrift.models.chemicaldrift:1452: old species: [2 0 0 3 0 2 0]
14:19:45 DEBUG opendrift.models.chemicaldrift:1453: new species: [0 3 3 0 3 0 3]
14:19:45 DEBUG opendrift.models.chemicaldrift:1460: Number of transformations total:
[[ 0. 0. 0. 526. 0.]
[ 0. 0. 0. 0. 0.]
[ 105. 0. 0. 803. 0.]
[ 25. 0. 1135. 0. 0.]
[ 0. 0. 0. 0. 0.]]
14:19:45 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 0 elements
14:19:45 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:45 DEBUG opendrift.models.chemicaldrift:1504: Adding uncertainty for desorption from sediments: 0.5 m
14:19:45 DEBUG opendrift.models.oceandrift:530: Using functional expression for diffusivity
14:19:45 DEBUG opendrift.models.oceandrift:535: Diffusivities are in range 0.0 to 0.05264858077711284
14:19:45 DEBUG opendrift.models.oceandrift:554: TSprofiles deactivated for vertical mixing
14:19:45 DEBUG opendrift.models.oceandrift:568: Vertical mixing module:windspeed_Large1994
14:19:45 DEBUG opendrift.models.oceandrift:571: Turbulent diffusion with random walk scheme using 30 fast time steps of dt=60s
14:19:45 DEBUG opendrift.models.oceandrift:618: 27 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 9 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 12 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 11 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 14 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 17 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 24 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 18 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 2 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 2 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 26 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 22 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 19 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 25 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 3 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 3 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 15 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 21 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 16 elements penetrated seafloor, lifting up
14:19:45 DEBUG opendrift.models.oceandrift:636: 1 elements reached seafloor, interacting with bottom
14:19:45 DEBUG opendrift.models.basemodel:730: Lifting 1 elements to seafloor.
14:19:45 DEBUG opendrift.models.oceandrift:618: 23 elements penetrated seafloor, lifting up
14:19:45 INFO opendrift.models.chemicaldrift:1633: Number of resuspended particles: 28
14:19:45 DEBUG opendrift.models.chemicaldrift:1638: Adding uncertainty for resuspension from sediments: 0.5 m
14:19:45 DEBUG opendrift.models.chemicaldrift:1539: Updated particle diameter for 28 elements
14:19:45 DEBUG opendrift.models.chemicaldrift:1543: Adding uncertainty for particle diameter: 5e-06 m
14:19:45 INFO opendrift.models.chemicaldrift:1861: partitioning: [53, 0, 291, 156, 0] ['LMM', 'Humic colloid', 'Particle reversible', 'Sediment reversible', 'Sediment slowly reversible']
14:19:45 DEBUG opendrift.models.basemodel:1634: Horizontal diffusivity is 0, no random walk.
14:19:45 DEBUG opendrift.models.basemodel:2097: 500 active elements (0 deactivated)
14:19:45 DEBUG opendrift.models.basemodel:2126: Cleaning up
14:19:45 DEBUG opendrift.models.basemodel.environment:607: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:608: Variable group ['land_binary_mask']
14:19:45 DEBUG opendrift.models.basemodel.environment:609: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:613: Calling reader global_landmask
14:19:45 DEBUG opendrift.models.basemodel.environment:614: ----------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:630: Data needed for 500 elements
14:19:45 DEBUG opendrift.readers.basereader.variables:752: Fetching variables from global_landmask covering 500 elements
14:19:45 DEBUG opendrift.readers.basereader.continuous:37: Fetched env-before
14:19:45 DEBUG opendrift.readers.basereader.variables:633: Checking land_binary_mask for invalid values
14:19:45 DEBUG opendrift.readers.basereader.variables:788: Reader projection is latlon - rotation of vectors is not needed.
14:19:45 DEBUG opendrift.models.basemodel.environment:764: Obtained data for all elements.
14:19:45 DEBUG opendrift.models.basemodel.environment:777: ---------------------------------------
14:19:45 DEBUG opendrift.models.basemodel.environment:778: Finished processing all variable groups
14:19:45 DEBUG opendrift.models.basemodel.environment:903: ------------ SUMMARY -------------
14:19:45 DEBUG opendrift.models.basemodel.environment:905: land_binary_mask: 0 (min) 0 (max)
14:19:45 DEBUG opendrift.models.basemodel.environment:907: ---------------------------------
14:19:45 DEBUG opendrift.models.basemodel:686: No elements hit coastline.
14:19:45 DEBUG opendrift.models.basemodel:1683: No elements to deactivate
14:19:45 DEBUG opendrift.models.basemodel:92: Changed mode from Mode.Run to Mode.Result
Print and plot results
print('Final speciation:')
for isp,sp in enumerate(o.name_species):
print ('{:32}: {:>6}'.format(sp,sum(o.elements.specie==isp)))
print('Number of transformations:')
for isp in range(o.nspecies):
print('{}'.format(['{:>9}'.format(np.int32(item)) for item in o.ntransformations[isp,:]]) )
mass = o.get_property('mass')
mass_d = o.get_property('mass_degraded')
mass_v = o.get_property('mass_volatilized')
m_pre = sum(mass[0][-1])
m_deg = sum(mass_d[0][-1])
m_vol = sum(mass_v[0][-1])
m_tot = m_pre + m_deg + m_vol
print('Mass budget for target chemical:')
print('mass preserved : {:.3f}'.format(m_pre * 1e-6),' g {:.3f}'.format(m_pre/m_tot*100),'%')
print('mass degraded : {:.3f}'.format(m_deg * 1e-6),' g {:.3f}'.format(m_deg/m_tot*100),'%')
print('mass volatilized : {:.3f}'.format(m_vol * 1e-6),' g {:.3f}'.format(m_vol/m_tot*100),'%')
legend=['dissolved', '', 'SPM', 'sediment', '']
o.animation_profile(color='specie',
markersize='mass',
markersize_scaling=30,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
legend = legend,
legend_loc = 3,
fps = 10
)
Final speciation:
LMM : 53
Humic colloid : 0
Particle reversible : 291
Sediment reversible : 156
Sediment slowly reversible : 0
Number of transformations:
[' 0', ' 0', ' 0', ' 526', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
[' 105', ' 0', ' 0', ' 818', ' 0']
[' 25', ' 0', ' 1163', ' 0', ' 0']
[' 0', ' 0', ' 0', ' 0', ' 0']
Mass budget for target chemical:
mass preserved : 0.458 g 45.787 %
mass degraded : 0.541 g 54.108 %
mass volatilized : 0.001 g 0.105 %
14:19:45 DEBUG opendrift.models.basemodel:3010: Saving animation..
14:19:45 INFO opendrift.models.basemodel:4570: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_0.gif...
14:19:56 DEBUG opendrift.models.basemodel:4608: MPLBACKEND = agg
14:19:56 DEBUG opendrift.models.basemodel:4609: DISPLAY = None
14:19:56 DEBUG opendrift.models.basemodel:4610: Time to save animation: 0:00:11.596614
14:19:56 INFO opendrift.models.basemodel:3217: Time to make animation: 0:00:11.767734
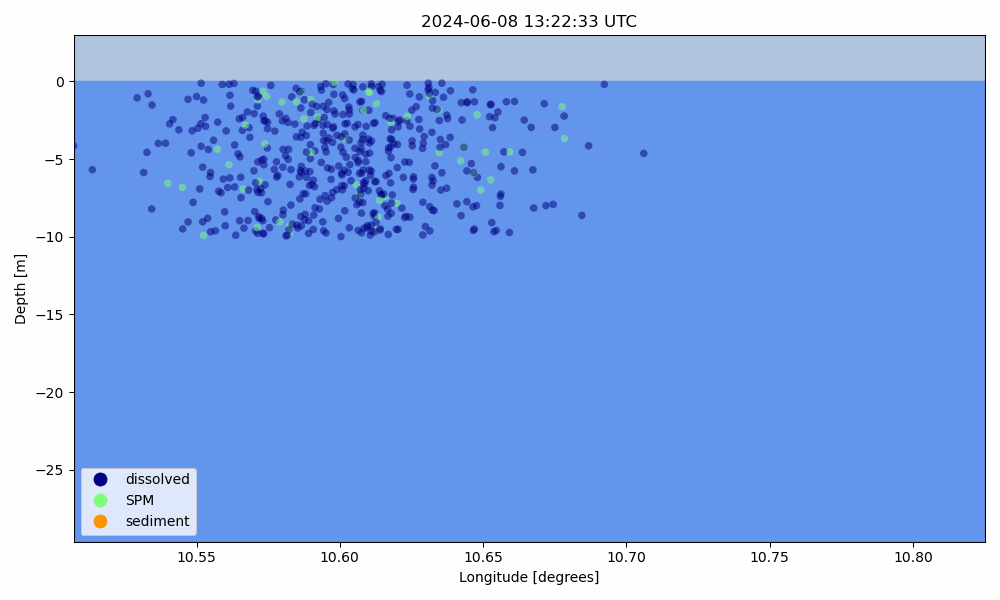
o.animation(color='specie',
markersize='mass',
markersize_scaling=100,
alpha=.5,
vmin=0,vmax=o.nspecies-1,
colorbar=False,
fast = True,
legend = legend,
legend_loc = 3,
fps=10
)
14:19:56 DEBUG opendrift.models.basemodel:2333: Setting up map: corners=None, fast=True, lscale=None
14:19:56 WARNING opendrift.models.basemodel:2379: Plotting fast. This will make your plots less accurate.
14:19:58 DEBUG opendrift.models.basemodel:3010: Saving animation..
14:19:58 INFO opendrift.models.basemodel:4570: Saving animation to /root/project/docs/source/gallery/animations/example_chemicaldrift_1.gif...
14:20:55 DEBUG opendrift.models.basemodel:4608: MPLBACKEND = agg
14:20:55 DEBUG opendrift.models.basemodel:4609: DISPLAY = None
14:20:55 DEBUG opendrift.models.basemodel:4610: Time to save animation: 0:00:56.684078
14:20:55 INFO opendrift.models.basemodel:3003: Time to make animation: 0:00:58.552901
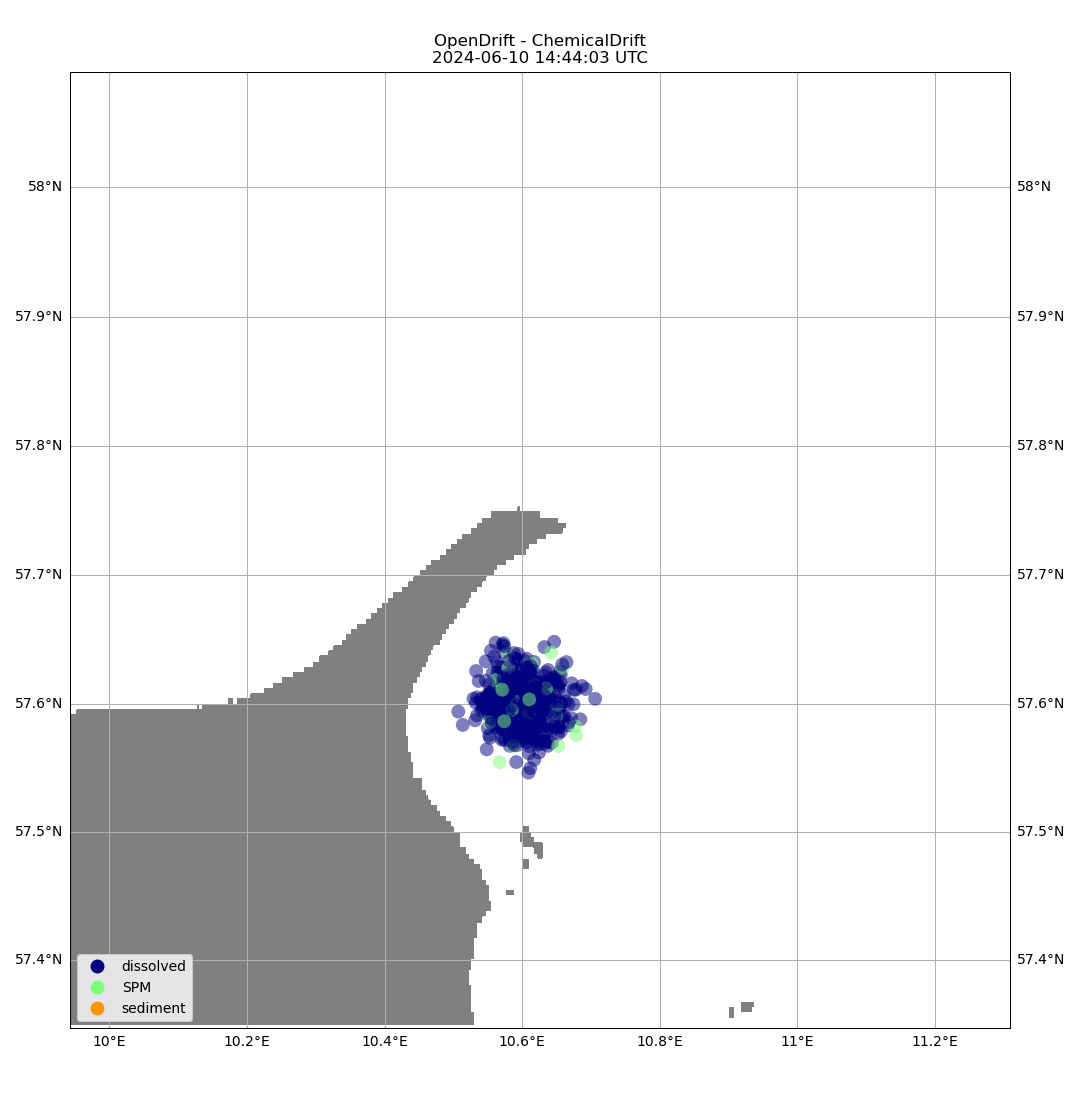
o.plot_mass(legend = legend,
time_unit = 'hours',
title = 'Chemical mass budget')
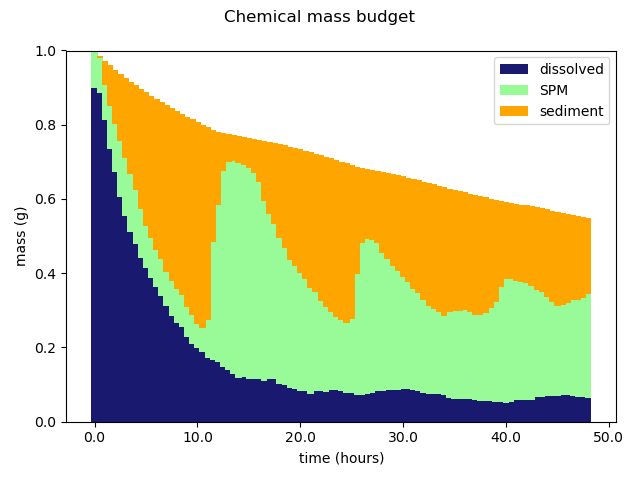
dissolved : 0.03854771875g (8.418928161838084%)
SPM : 0.2796795625g (61.08278833029008%)
sediment : 0.139642390625g (30.498283507871836%)
/root/project/opendrift/models/chemicaldrift.py:3006: UserWarning: set_ticklabels() should only be used with a fixed number of ticks, i.e. after set_ticks() or using a FixedLocator.
ax.axes.get_xaxis().set_ticklabels(np.round(ax.axes.get_xticks() * time_conversion_factor))
Total running time of the script: (3 minutes 1.342 seconds)